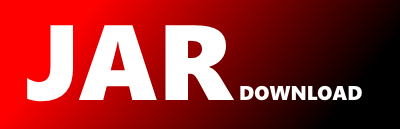
com.sleepycat.je.utilint.CollectionUtils Maven / Gradle / Ivy
The newest version!
/*-
* Copyright (C) 2002, 2018, Oracle and/or its affiliates. All rights reserved.
*
* This file was distributed by Oracle as part of a version of Oracle Berkeley
* DB Java Edition made available at:
*
* http://www.oracle.com/technetwork/database/database-technologies/berkeleydb/downloads/index.html
*
* Please see the LICENSE file included in the top-level directory of the
* appropriate version of Oracle Berkeley DB Java Edition for a copy of the
* license and additional information.
*/
package com.sleepycat.je.utilint;
import static java.util.Collections.emptySet;
import java.io.Serializable;
import java.util.AbstractMap;
import java.util.AbstractSet;
import java.util.Comparator;
import java.util.Iterator;
import java.util.NoSuchElementException;
import java.util.Set;
import java.util.SortedMap;
import java.util.SortedSet;
/**
* Java Collection utilities.
*/
public final class CollectionUtils {
/** This class cannot be instantiated. */
private CollectionUtils() {
throw new AssertionError();
}
/**
* An empty, unmodifiable, serializable, sorted set used for
* emptySortedSet.
*/
private static class EmptySortedSet extends AbstractSet
implements SortedSet, Serializable {
private static final long serialVersionUID = 1;
@SuppressWarnings("rawtypes")
static final SortedSet> INSTANCE = new EmptySortedSet
© 2015 - 2024 Weber Informatics LLC | Privacy Policy