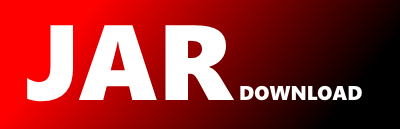
com.sleepycat.persist.ForwardCursor Maven / Gradle / Ivy
The newest version!
/*-
* Copyright (C) 2002, 2018, Oracle and/or its affiliates. All rights reserved.
*
* This file was distributed by Oracle as part of a version of Oracle Berkeley
* DB Java Edition made available at:
*
* http://www.oracle.com/technetwork/database/database-technologies/berkeleydb/downloads/index.html
*
* Please see the LICENSE file included in the top-level directory of the
* appropriate version of Oracle Berkeley DB Java Edition for a copy of the
* license and additional information.
*/
package com.sleepycat.persist;
import java.io.Closeable;
import java.util.Iterator;
import com.sleepycat.je.DatabaseException;
/* */
import com.sleepycat.je.EnvironmentFailureException ; // for javadoc
/* */
import com.sleepycat.je.LockMode;
/* */
import com.sleepycat.je.OperationFailureException ; // for javadoc
/* */
/**
* Cursor operations limited to traversing forward. See {@link EntityCursor}
* for general information on cursors.
*
* {@code ForwardCursor} objects are not thread-safe. Cursors
* should be opened, used and closed by a single thread.
*
* WARNING: Cursors must always be closed to prevent resource leaks
* which could lead to the index becoming unusable or cause an
* OutOfMemoryError
. To ensure that a cursor is closed in the
* face of exceptions, close it in a finally block.
*
* @author Mark Hayes
*/
public interface ForwardCursor extends Iterable
/* */
, Closeable
/* */
{
/**
* Moves the cursor to the next value and returns it, or returns null
* if there are no more values in the cursor range. If the cursor is
* uninitialized, this method returns the first value.
*
* {@link LockMode#DEFAULT} is used implicitly.
*
* @return the next value, or null if there are no more values in the
* cursor range.
*
*
* @throws OperationFailureException if one of the Read Operation
* Failures occurs.
*
* @throws EnvironmentFailureException if an unexpected, internal or
* environment-wide failure occurs.
*
*
* @throws DatabaseException the base class for all BDB exceptions.
*/
V next()
throws DatabaseException;
/**
* Moves the cursor to the next value and returns it, or returns null
* if there are no more values in the cursor range. If the cursor is
* uninitialized, this method returns the first value.
*
* @param lockMode the lock mode to use for this operation, or null to
* use {@link LockMode#DEFAULT}.
*
* @return the next value, or null if there are no more values in the
* cursor range.
*
*
* @throws OperationFailureException if one of the Read Operation
* Failures occurs.
*
* @throws EnvironmentFailureException if an unexpected, internal or
* environment-wide failure occurs.
*
*
* @throws DatabaseException the base class for all BDB exceptions.
*/
V next(LockMode lockMode)
throws DatabaseException;
/**
* Returns an iterator over the key range, starting with the value
* following the current position or at the first value if the cursor is
* uninitialized.
*
* {@link LockMode#DEFAULT} is used implicitly.
*
* @return the iterator.
*/
Iterator iterator();
/**
* Returns an iterator over the key range, starting with the value
* following the current position or at the first value if the cursor is
* uninitialized.
*
* @param lockMode the lock mode to use for all operations performed
* using the iterator, or null to use {@link LockMode#DEFAULT}.
*
* @return the iterator.
*/
Iterator iterator(LockMode lockMode);
/**
* Closes the cursor.
*
* @throws DatabaseException the base class for all BDB exceptions.
*/
void close()
throws DatabaseException;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy