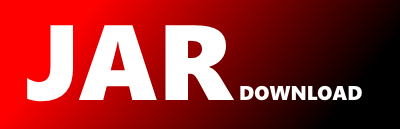
com.sleepycat.persist.impl.EntityInput Maven / Gradle / Ivy
The newest version!
/*-
* Copyright (C) 2002, 2018, Oracle and/or its affiliates. All rights reserved.
*
* This file was distributed by Oracle as part of a version of Oracle Berkeley
* DB Java Edition made available at:
*
* http://www.oracle.com/technetwork/database/database-technologies/berkeleydb/downloads/index.html
*
* Please see the LICENSE file included in the top-level directory of the
* appropriate version of Oracle Berkeley DB Java Edition for a copy of the
* license and additional information.
*/
package com.sleepycat.persist.impl;
import java.math.BigDecimal;
import java.math.BigInteger;
/**
* Used for reading object fields.
*
* Unlike TupleInput, Strings are returned by {@link #readObject} when using
* this class.
*
* @author Mark Hayes
*/
public interface EntityInput {
/**
* Returns the Catalog associated with this input.
*/
Catalog getCatalog();
/**
* Return whether this input is in raw mode, i.e., whether it is returning
* raw instances.
*/
boolean isRawAccess();
/**
* Changes raw mode and returns the original mode, which is normally
* restored later. For temporarily changing the mode during a conversion.
*/
boolean setRawAccess(boolean rawAccessParam);
/**
* Called via Accessor to read all fields with reference types, except for
* the primary key field and composite key fields (see readKeyObject
* below).
*/
Object readObject()
throws RefreshException;
/**
* Called for a primary key field or a composite key field with a reference
* type.
*
* For such key fields, no formatId is present nor can the object
* already be present in the visited object set.
*/
Object readKeyObject(Format format)
throws RefreshException;
/**
* Called for a String field, that is not a primary key field or a
* composite key field with a reference type.
*
* For the new String format, no formatId is present nor can the object
* already be present in the visited object set. For the old String
* format, this method simply calls readObject for compatibility.
*/
Object readStringObject()
throws RefreshException;
/**
* Called via Accessor.readSecKeyFields for a primary key field with a
* reference type. This method must be called before reading any other
* fields.
*/
void registerPriKeyObject(Object o);
/**
* Called via Accessor.readSecKeyFields for a primary String key field.
* This method must be called before reading any other fields.
*/
void registerPriStringKeyObject(Object o);
/**
* Called by ObjectArrayFormat and PrimitiveArrayFormat to read the array
* length.
*/
int readArrayLength();
/**
* Called by EnumFormat to read and return index of the enum constant.
*/
int readEnumConstant(String[] names);
/**
* Called via PersistKeyCreator to skip fields prior to the secondary key
* field. Also called during class evolution so skip deleted fields.
*/
void skipField(Format declaredFormat)
throws RefreshException;
/* The following methods are a subset of the methods in TupleInput. */
String readString()
throws RefreshException;
char readChar()
throws RefreshException;
boolean readBoolean()
throws RefreshException;
byte readByte()
throws RefreshException;
short readShort()
throws RefreshException;
int readInt()
throws RefreshException;
long readLong()
throws RefreshException;
float readSortedFloat()
throws RefreshException;
double readSortedDouble()
throws RefreshException;
BigInteger readBigInteger()
throws RefreshException;
BigDecimal readSortedBigDecimal()
throws RefreshException;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy