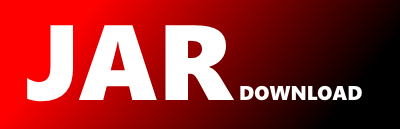
sts.oda.client.api.DefaultApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of on-demand-sdk-java Show documentation
Show all versions of on-demand-sdk-java Show documentation
Sterling On Demand Java SDK
/*
* on-demand-api
* The Sterling On Demand API allows you to integrate background checks into your platform and manage the process from end-to-end.
*
* OpenAPI spec version: 2018-05-08T16:44:26.238-07:00
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package sts.oda.client.api;
import sts.oda.client.ApiCallback;
import sts.oda.client.ApiClient;
import sts.oda.client.ApiException;
import sts.oda.client.ApiResponse;
import sts.oda.client.Configuration;
import sts.oda.client.Pair;
import sts.oda.client.ProgressRequestBody;
import sts.oda.client.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import sts.oda.client.model.AdverseActionRequest;
import sts.oda.client.model.BillingCodesResponse;
import sts.oda.client.model.CandidateDocumentResponse;
import sts.oda.client.model.CandidatePortalLinkResponse;
import sts.oda.client.model.CandidateRequest;
import sts.oda.client.model.CandidateResponse;
import sts.oda.client.model.ChargeRequest;
import sts.oda.client.model.ChargeResponse;
import sts.oda.client.model.ErrorResponse;
import sts.oda.client.model.HealthResponse;
import sts.oda.client.model.IdentityRequest;
import sts.oda.client.model.IdentityResponse;
import sts.oda.client.model.InviteResponse;
import sts.oda.client.model.OneTimeReportLinkResponse;
import sts.oda.client.model.PackagePriceResponse;
import sts.oda.client.model.PackageResponse;
import sts.oda.client.model.ReferenceCodesResponse;
import sts.oda.client.model.ScreeningDetailsResponse;
import sts.oda.client.model.ScreeningRequest;
import sts.oda.client.model.ScreeningResponse;
import sts.oda.client.model.SubscriptionEventsResponse;
import sts.oda.client.model.SubscriptionRequest;
import sts.oda.client.model.SubscriptionResponse;
import sts.oda.client.model.TokenRequest;
import sts.oda.client.model.TokenResponse;
import sts.oda.client.model.TrustedUserRequest;
import sts.oda.client.model.TrustedUserResponse;
import sts.oda.client.model.VerificationRequest;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class DefaultApi {
private ApiClient apiClient;
public DefaultApi() {
this(Configuration.getDefaultApiClient());
}
public DefaultApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for billingCodesGet
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call billingCodesGetCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/billing-codes";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call billingCodesGetValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = billingCodesGetCall(progressListener, progressRequestListener);
return call;
}
/**
* Get a list of valid billing codes
* Get a list of valid billing codes
* @return BillingCodesResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public BillingCodesResponse billingCodesGet() throws ApiException {
ApiResponse resp = billingCodesGetWithHttpInfo();
return resp.getData();
}
/**
* Get a list of valid billing codes
* Get a list of valid billing codes
* @return ApiResponse<BillingCodesResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse billingCodesGetWithHttpInfo() throws ApiException {
com.squareup.okhttp.Call call = billingCodesGetValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a list of valid billing codes (asynchronously)
* Get a list of valid billing codes
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call billingCodesGetAsync(final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = billingCodesGetValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for candidatesGet
* @param limit (optional)
* @param offset (optional)
* @param givenName (optional)
* @param familyName (optional)
* @param clientReferenceId (optional)
* @param email (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call candidatesGetCall(String limit, String offset, String givenName, String familyName, String clientReferenceId, String email, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/candidates";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPair("offset", offset));
if (givenName != null)
localVarQueryParams.addAll(apiClient.parameterToPair("givenName", givenName));
if (familyName != null)
localVarQueryParams.addAll(apiClient.parameterToPair("familyName", familyName));
if (clientReferenceId != null)
localVarQueryParams.addAll(apiClient.parameterToPair("clientReferenceId", clientReferenceId));
if (email != null)
localVarQueryParams.addAll(apiClient.parameterToPair("email", email));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call candidatesGetValidateBeforeCall(String limit, String offset, String givenName, String familyName, String clientReferenceId, String email, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = candidatesGetCall(limit, offset, givenName, familyName, clientReferenceId, email, progressListener, progressRequestListener);
return call;
}
/**
* Get a list of Candidates
* Get a list of Candidates
* @param limit (optional)
* @param offset (optional)
* @param givenName (optional)
* @param familyName (optional)
* @param clientReferenceId (optional)
* @param email (optional)
* @return List<CandidateResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public List candidatesGet(String limit, String offset, String givenName, String familyName, String clientReferenceId, String email) throws ApiException {
ApiResponse> resp = candidatesGetWithHttpInfo(limit, offset, givenName, familyName, clientReferenceId, email);
return resp.getData();
}
/**
* Get a list of Candidates
* Get a list of Candidates
* @param limit (optional)
* @param offset (optional)
* @param givenName (optional)
* @param familyName (optional)
* @param clientReferenceId (optional)
* @param email (optional)
* @return ApiResponse<List<CandidateResponse>>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse> candidatesGetWithHttpInfo(String limit, String offset, String givenName, String familyName, String clientReferenceId, String email) throws ApiException {
com.squareup.okhttp.Call call = candidatesGetValidateBeforeCall(limit, offset, givenName, familyName, clientReferenceId, email, null, null);
Type localVarReturnType = new TypeToken>(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a list of Candidates (asynchronously)
* Get a list of Candidates
* @param limit (optional)
* @param offset (optional)
* @param givenName (optional)
* @param familyName (optional)
* @param clientReferenceId (optional)
* @param email (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call candidatesGetAsync(String limit, String offset, String givenName, String familyName, String clientReferenceId, String email, final ApiCallback> callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = candidatesGetValidateBeforeCall(limit, offset, givenName, familyName, clientReferenceId, email, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken>(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for candidatesIdDocumentsGet
* @param id (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call candidatesIdDocumentsGetCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/candidates/{id}/documents"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call candidatesIdDocumentsGetValidateBeforeCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling candidatesIdDocumentsGet(Async)");
}
com.squareup.okhttp.Call call = candidatesIdDocumentsGetCall(id, progressListener, progressRequestListener);
return call;
}
/**
* List documents attached to a candidate
* List documents attached to a candidate
* @param id (required)
* @return List<CandidateDocumentResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public List candidatesIdDocumentsGet(String id) throws ApiException {
ApiResponse> resp = candidatesIdDocumentsGetWithHttpInfo(id);
return resp.getData();
}
/**
* List documents attached to a candidate
* List documents attached to a candidate
* @param id (required)
* @return ApiResponse<List<CandidateDocumentResponse>>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse> candidatesIdDocumentsGetWithHttpInfo(String id) throws ApiException {
com.squareup.okhttp.Call call = candidatesIdDocumentsGetValidateBeforeCall(id, null, null);
Type localVarReturnType = new TypeToken>(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* List documents attached to a candidate (asynchronously)
* List documents attached to a candidate
* @param id (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call candidatesIdDocumentsGetAsync(String id, final ApiCallback> callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = candidatesIdDocumentsGetValidateBeforeCall(id, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken>(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for candidatesIdDocumentsPost
* @param id (required)
* @param contentType (optional)
* @param fileName (optional)
* @param body (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call candidatesIdDocumentsPostCall(String id, String contentType, String fileName, byte[] body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/candidates/{id}/documents"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (fileName != null)
localVarQueryParams.addAll(apiClient.parameterToPair("fileName", fileName));
Map localVarHeaderParams = new HashMap();
if (contentType != null)
localVarHeaderParams.put("Content-Type", apiClient.parameterToString(contentType));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/octet-stream"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call candidatesIdDocumentsPostValidateBeforeCall(String id, String contentType, String fileName, byte[] body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling candidatesIdDocumentsPost(Async)");
}
com.squareup.okhttp.Call call = candidatesIdDocumentsPostCall(id, contentType, fileName, body, progressListener, progressRequestListener);
return call;
}
/**
* Attach a document to a candidate
* Attach a document to a candidate
* @param id (required)
* @param contentType (optional)
* @param fileName (optional)
* @param body (optional)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void candidatesIdDocumentsPost(String id, String contentType, String fileName, byte[] body) throws ApiException {
candidatesIdDocumentsPostWithHttpInfo(id, contentType, fileName, body);
}
/**
* Attach a document to a candidate
* Attach a document to a candidate
* @param id (required)
* @param contentType (optional)
* @param fileName (optional)
* @param body (optional)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse candidatesIdDocumentsPostWithHttpInfo(String id, String contentType, String fileName, byte[] body) throws ApiException {
com.squareup.okhttp.Call call = candidatesIdDocumentsPostValidateBeforeCall(id, contentType, fileName, body, null, null);
return apiClient.execute(call);
}
/**
* Attach a document to a candidate (asynchronously)
* Attach a document to a candidate
* @param id (required)
* @param contentType (optional)
* @param fileName (optional)
* @param body (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call candidatesIdDocumentsPostAsync(String id, String contentType, String fileName, byte[] body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = candidatesIdDocumentsPostValidateBeforeCall(id, contentType, fileName, body, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for candidatesIdGet
* @param id (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call candidatesIdGetCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/candidates/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call candidatesIdGetValidateBeforeCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling candidatesIdGet(Async)");
}
com.squareup.okhttp.Call call = candidatesIdGetCall(id, progressListener, progressRequestListener);
return call;
}
/**
* Get a specific Candidate
* Get a specific Candidate
* @param id (required)
* @return CandidateResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CandidateResponse candidatesIdGet(String id) throws ApiException {
ApiResponse resp = candidatesIdGetWithHttpInfo(id);
return resp.getData();
}
/**
* Get a specific Candidate
* Get a specific Candidate
* @param id (required)
* @return ApiResponse<CandidateResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse candidatesIdGetWithHttpInfo(String id) throws ApiException {
com.squareup.okhttp.Call call = candidatesIdGetValidateBeforeCall(id, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a specific Candidate (asynchronously)
* Get a specific Candidate
* @param id (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call candidatesIdGetAsync(String id, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = candidatesIdGetValidateBeforeCall(id, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for candidatesIdLinksPost
* @param id (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call candidatesIdLinksPostCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/candidates/{id}/links"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call candidatesIdLinksPostValidateBeforeCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling candidatesIdLinksPost(Async)");
}
com.squareup.okhttp.Call call = candidatesIdLinksPostCall(id, progressListener, progressRequestListener);
return call;
}
/**
* Get links to the Candidate portal for use by the Candidate
* Get links to the Candidate portal for use by the Candidate
* @param id (required)
* @return CandidatePortalLinkResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CandidatePortalLinkResponse candidatesIdLinksPost(String id) throws ApiException {
ApiResponse resp = candidatesIdLinksPostWithHttpInfo(id);
return resp.getData();
}
/**
* Get links to the Candidate portal for use by the Candidate
* Get links to the Candidate portal for use by the Candidate
* @param id (required)
* @return ApiResponse<CandidatePortalLinkResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse candidatesIdLinksPostWithHttpInfo(String id) throws ApiException {
com.squareup.okhttp.Call call = candidatesIdLinksPostValidateBeforeCall(id, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get links to the Candidate portal for use by the Candidate (asynchronously)
* Get links to the Candidate portal for use by the Candidate
* @param id (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call candidatesIdLinksPostAsync(String id, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = candidatesIdLinksPostValidateBeforeCall(id, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for candidatesIdPut
* @param id (required)
* @param candidateRequest (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call candidatesIdPutCall(String id, CandidateRequest candidateRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = candidateRequest;
// create path and map variables
String localVarPath = "/candidates/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call candidatesIdPutValidateBeforeCall(String id, CandidateRequest candidateRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling candidatesIdPut(Async)");
}
// verify the required parameter 'candidateRequest' is set
if (candidateRequest == null) {
throw new ApiException("Missing the required parameter 'candidateRequest' when calling candidatesIdPut(Async)");
}
com.squareup.okhttp.Call call = candidatesIdPutCall(id, candidateRequest, progressListener, progressRequestListener);
return call;
}
/**
* Update a candidate
* Update a candidate
* @param id (required)
* @param candidateRequest (required)
* @return CandidateResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CandidateResponse candidatesIdPut(String id, CandidateRequest candidateRequest) throws ApiException {
ApiResponse resp = candidatesIdPutWithHttpInfo(id, candidateRequest);
return resp.getData();
}
/**
* Update a candidate
* Update a candidate
* @param id (required)
* @param candidateRequest (required)
* @return ApiResponse<CandidateResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse candidatesIdPutWithHttpInfo(String id, CandidateRequest candidateRequest) throws ApiException {
com.squareup.okhttp.Call call = candidatesIdPutValidateBeforeCall(id, candidateRequest, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Update a candidate (asynchronously)
* Update a candidate
* @param id (required)
* @param candidateRequest (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call candidatesIdPutAsync(String id, CandidateRequest candidateRequest, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = candidatesIdPutValidateBeforeCall(id, candidateRequest, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for candidatesIdTrustPost
* @param id (required)
* @param trustedUserRequest (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call candidatesIdTrustPostCall(String id, TrustedUserRequest trustedUserRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = trustedUserRequest;
// create path and map variables
String localVarPath = "/candidates/{id}/trust"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call candidatesIdTrustPostValidateBeforeCall(String id, TrustedUserRequest trustedUserRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling candidatesIdTrustPost(Async)");
}
// verify the required parameter 'trustedUserRequest' is set
if (trustedUserRequest == null) {
throw new ApiException("Missing the required parameter 'trustedUserRequest' when calling candidatesIdTrustPost(Async)");
}
com.squareup.okhttp.Call call = candidatesIdTrustPostCall(id, trustedUserRequest, progressListener, progressRequestListener);
return call;
}
/**
* Trust a candidate
* Trust a candidate
* @param id (required)
* @param trustedUserRequest (required)
* @return TrustedUserResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TrustedUserResponse candidatesIdTrustPost(String id, TrustedUserRequest trustedUserRequest) throws ApiException {
ApiResponse resp = candidatesIdTrustPostWithHttpInfo(id, trustedUserRequest);
return resp.getData();
}
/**
* Trust a candidate
* Trust a candidate
* @param id (required)
* @param trustedUserRequest (required)
* @return ApiResponse<TrustedUserResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse candidatesIdTrustPostWithHttpInfo(String id, TrustedUserRequest trustedUserRequest) throws ApiException {
com.squareup.okhttp.Call call = candidatesIdTrustPostValidateBeforeCall(id, trustedUserRequest, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Trust a candidate (asynchronously)
* Trust a candidate
* @param id (required)
* @param trustedUserRequest (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call candidatesIdTrustPostAsync(String id, TrustedUserRequest trustedUserRequest, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = candidatesIdTrustPostValidateBeforeCall(id, trustedUserRequest, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for candidatesPost
* @param candidateRequest (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call candidatesPostCall(CandidateRequest candidateRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = candidateRequest;
// create path and map variables
String localVarPath = "/candidates";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call candidatesPostValidateBeforeCall(CandidateRequest candidateRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'candidateRequest' is set
if (candidateRequest == null) {
throw new ApiException("Missing the required parameter 'candidateRequest' when calling candidatesPost(Async)");
}
com.squareup.okhttp.Call call = candidatesPostCall(candidateRequest, progressListener, progressRequestListener);
return call;
}
/**
* Create a candidate
* Create a candidate
* @param candidateRequest (required)
* @return CandidateResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CandidateResponse candidatesPost(CandidateRequest candidateRequest) throws ApiException {
ApiResponse resp = candidatesPostWithHttpInfo(candidateRequest);
return resp.getData();
}
/**
* Create a candidate
* Create a candidate
* @param candidateRequest (required)
* @return ApiResponse<CandidateResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse candidatesPostWithHttpInfo(CandidateRequest candidateRequest) throws ApiException {
com.squareup.okhttp.Call call = candidatesPostValidateBeforeCall(candidateRequest, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Create a candidate (asynchronously)
* Create a candidate
* @param candidateRequest (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call candidatesPostAsync(CandidateRequest candidateRequest, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = candidatesPostValidateBeforeCall(candidateRequest, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for chargesPost
* @param chargeRequest (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call chargesPostCall(ChargeRequest chargeRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = chargeRequest;
// create path and map variables
String localVarPath = "/charges";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call chargesPostValidateBeforeCall(ChargeRequest chargeRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'chargeRequest' is set
if (chargeRequest == null) {
throw new ApiException("Missing the required parameter 'chargeRequest' when calling chargesPost(Async)");
}
com.squareup.okhttp.Call call = chargesPostCall(chargeRequest, progressListener, progressRequestListener);
return call;
}
/**
* Create a capture
* Create a capture
* @param chargeRequest (required)
* @return ChargeResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ChargeResponse chargesPost(ChargeRequest chargeRequest) throws ApiException {
ApiResponse resp = chargesPostWithHttpInfo(chargeRequest);
return resp.getData();
}
/**
* Create a capture
* Create a capture
* @param chargeRequest (required)
* @return ApiResponse<ChargeResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse chargesPostWithHttpInfo(ChargeRequest chargeRequest) throws ApiException {
com.squareup.okhttp.Call call = chargesPostValidateBeforeCall(chargeRequest, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Create a capture (asynchronously)
* Create a capture
* @param chargeRequest (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call chargesPostAsync(ChargeRequest chargeRequest, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = chargesPostValidateBeforeCall(chargeRequest, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for healthGet
* @param deep (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call healthGetCall(String deep, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/health";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (deep != null)
localVarQueryParams.addAll(apiClient.parameterToPair("deep", deep));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call healthGetValidateBeforeCall(String deep, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = healthGetCall(deep, progressListener, progressRequestListener);
return call;
}
/**
* Check the health of the API
* Check the health of the API
* @param deep (optional)
* @return HealthResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public HealthResponse healthGet(String deep) throws ApiException {
ApiResponse resp = healthGetWithHttpInfo(deep);
return resp.getData();
}
/**
* Check the health of the API
* Check the health of the API
* @param deep (optional)
* @return ApiResponse<HealthResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse healthGetWithHttpInfo(String deep) throws ApiException {
com.squareup.okhttp.Call call = healthGetValidateBeforeCall(deep, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Check the health of the API (asynchronously)
* Check the health of the API
* @param deep (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call healthGetAsync(String deep, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = healthGetValidateBeforeCall(deep, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for identitiesIdGet
* @param id (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call identitiesIdGetCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/identities/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call identitiesIdGetValidateBeforeCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling identitiesIdGet(Async)");
}
com.squareup.okhttp.Call call = identitiesIdGetCall(id, progressListener, progressRequestListener);
return call;
}
/**
* Get a verified identity
* Get a verified identity
* @param id (required)
* @return IdentityResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public IdentityResponse identitiesIdGet(String id) throws ApiException {
ApiResponse resp = identitiesIdGetWithHttpInfo(id);
return resp.getData();
}
/**
* Get a verified identity
* Get a verified identity
* @param id (required)
* @return ApiResponse<IdentityResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse identitiesIdGetWithHttpInfo(String id) throws ApiException {
com.squareup.okhttp.Call call = identitiesIdGetValidateBeforeCall(id, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a verified identity (asynchronously)
* Get a verified identity
* @param id (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call identitiesIdGetAsync(String id, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = identitiesIdGetValidateBeforeCall(id, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for identitiesIdRetryPost
* @param id (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call identitiesIdRetryPostCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/identities/{id}/retry"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call identitiesIdRetryPostValidateBeforeCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling identitiesIdRetryPost(Async)");
}
com.squareup.okhttp.Call call = identitiesIdRetryPostCall(id, progressListener, progressRequestListener);
return call;
}
/**
* Retry creating an identity and get a new set of questions
* Retry creating an identity and get a new set of questions
* @param id (required)
* @return IdentityResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public IdentityResponse identitiesIdRetryPost(String id) throws ApiException {
ApiResponse resp = identitiesIdRetryPostWithHttpInfo(id);
return resp.getData();
}
/**
* Retry creating an identity and get a new set of questions
* Retry creating an identity and get a new set of questions
* @param id (required)
* @return ApiResponse<IdentityResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse identitiesIdRetryPostWithHttpInfo(String id) throws ApiException {
com.squareup.okhttp.Call call = identitiesIdRetryPostValidateBeforeCall(id, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Retry creating an identity and get a new set of questions (asynchronously)
* Retry creating an identity and get a new set of questions
* @param id (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call identitiesIdRetryPostAsync(String id, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = identitiesIdRetryPostValidateBeforeCall(id, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for identitiesIdVerificationPut
* @param id (required)
* @param verificationRequest (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call identitiesIdVerificationPutCall(String id, VerificationRequest verificationRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = verificationRequest;
// create path and map variables
String localVarPath = "/identities/{id}/verification"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call identitiesIdVerificationPutValidateBeforeCall(String id, VerificationRequest verificationRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling identitiesIdVerificationPut(Async)");
}
// verify the required parameter 'verificationRequest' is set
if (verificationRequest == null) {
throw new ApiException("Missing the required parameter 'verificationRequest' when calling identitiesIdVerificationPut(Async)");
}
com.squareup.okhttp.Call call = identitiesIdVerificationPutCall(id, verificationRequest, progressListener, progressRequestListener);
return call;
}
/**
* Verify an identity by providing correct answers
* Verify an identity by providing correct answers
* @param id (required)
* @param verificationRequest (required)
* @return IdentityResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public IdentityResponse identitiesIdVerificationPut(String id, VerificationRequest verificationRequest) throws ApiException {
ApiResponse resp = identitiesIdVerificationPutWithHttpInfo(id, verificationRequest);
return resp.getData();
}
/**
* Verify an identity by providing correct answers
* Verify an identity by providing correct answers
* @param id (required)
* @param verificationRequest (required)
* @return ApiResponse<IdentityResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse identitiesIdVerificationPutWithHttpInfo(String id, VerificationRequest verificationRequest) throws ApiException {
com.squareup.okhttp.Call call = identitiesIdVerificationPutValidateBeforeCall(id, verificationRequest, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Verify an identity by providing correct answers (asynchronously)
* Verify an identity by providing correct answers
* @param id (required)
* @param verificationRequest (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call identitiesIdVerificationPutAsync(String id, VerificationRequest verificationRequest, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = identitiesIdVerificationPutValidateBeforeCall(id, verificationRequest, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for identitiesPost
* @param identityRequest (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call identitiesPostCall(IdentityRequest identityRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = identityRequest;
// create path and map variables
String localVarPath = "/identities";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call identitiesPostValidateBeforeCall(IdentityRequest identityRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'identityRequest' is set
if (identityRequest == null) {
throw new ApiException("Missing the required parameter 'identityRequest' when calling identitiesPost(Async)");
}
com.squareup.okhttp.Call call = identitiesPostCall(identityRequest, progressListener, progressRequestListener);
return call;
}
/**
* Get a list of questions needing to be answered before an identity can be created
* Get a list of questions needing to be answered before an identity can be created
* @param identityRequest (required)
* @return IdentityResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public IdentityResponse identitiesPost(IdentityRequest identityRequest) throws ApiException {
ApiResponse resp = identitiesPostWithHttpInfo(identityRequest);
return resp.getData();
}
/**
* Get a list of questions needing to be answered before an identity can be created
* Get a list of questions needing to be answered before an identity can be created
* @param identityRequest (required)
* @return ApiResponse<IdentityResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse identitiesPostWithHttpInfo(IdentityRequest identityRequest) throws ApiException {
com.squareup.okhttp.Call call = identitiesPostValidateBeforeCall(identityRequest, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a list of questions needing to be answered before an identity can be created (asynchronously)
* Get a list of questions needing to be answered before an identity can be created
* @param identityRequest (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call identitiesPostAsync(IdentityRequest identityRequest, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = identitiesPostValidateBeforeCall(identityRequest, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for packagesGet
* @param all (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call packagesGetCall(String all, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/packages";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (all != null)
localVarQueryParams.addAll(apiClient.parameterToPair("all", all));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call packagesGetValidateBeforeCall(String all, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = packagesGetCall(all, progressListener, progressRequestListener);
return call;
}
/**
* Get a list of Packages
* Get a list of Packages
* @param all (optional)
* @return List<PackageResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public List packagesGet(String all) throws ApiException {
ApiResponse> resp = packagesGetWithHttpInfo(all);
return resp.getData();
}
/**
* Get a list of Packages
* Get a list of Packages
* @param all (optional)
* @return ApiResponse<List<PackageResponse>>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse> packagesGetWithHttpInfo(String all) throws ApiException {
com.squareup.okhttp.Call call = packagesGetValidateBeforeCall(all, null, null);
Type localVarReturnType = new TypeToken>(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a list of Packages (asynchronously)
* Get a list of Packages
* @param all (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call packagesGetAsync(String all, final ApiCallback> callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = packagesGetValidateBeforeCall(all, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken>(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for packagesIdPriceGet
* @param id (required)
* @param candidateId (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call packagesIdPriceGetCall(String id, String candidateId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/packages/{id}/price"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (candidateId != null)
localVarQueryParams.addAll(apiClient.parameterToPair("candidateId", candidateId));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call packagesIdPriceGetValidateBeforeCall(String id, String candidateId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling packagesIdPriceGet(Async)");
}
// verify the required parameter 'candidateId' is set
if (candidateId == null) {
throw new ApiException("Missing the required parameter 'candidateId' when calling packagesIdPriceGet(Async)");
}
com.squareup.okhttp.Call call = packagesIdPriceGetCall(id, candidateId, progressListener, progressRequestListener);
return call;
}
/**
* Get the estimated price of a Package
* Get the estimated price of a Package
* @param id (required)
* @param candidateId (required)
* @return PackagePriceResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public PackagePriceResponse packagesIdPriceGet(String id, String candidateId) throws ApiException {
ApiResponse resp = packagesIdPriceGetWithHttpInfo(id, candidateId);
return resp.getData();
}
/**
* Get the estimated price of a Package
* Get the estimated price of a Package
* @param id (required)
* @param candidateId (required)
* @return ApiResponse<PackagePriceResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse packagesIdPriceGetWithHttpInfo(String id, String candidateId) throws ApiException {
com.squareup.okhttp.Call call = packagesIdPriceGetValidateBeforeCall(id, candidateId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get the estimated price of a Package (asynchronously)
* Get the estimated price of a Package
* @param id (required)
* @param candidateId (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call packagesIdPriceGetAsync(String id, String candidateId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = packagesIdPriceGetValidateBeforeCall(id, candidateId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for referenceCodesGet
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call referenceCodesGetCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reference-codes";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call referenceCodesGetValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = referenceCodesGetCall(progressListener, progressRequestListener);
return call;
}
/**
* Return a list of valid Reference Codes
* Return a list of valid Reference Codes
* @return ReferenceCodesResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ReferenceCodesResponse referenceCodesGet() throws ApiException {
ApiResponse resp = referenceCodesGetWithHttpInfo();
return resp.getData();
}
/**
* Return a list of valid Reference Codes
* Return a list of valid Reference Codes
* @return ApiResponse<ReferenceCodesResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse referenceCodesGetWithHttpInfo() throws ApiException {
com.squareup.okhttp.Call call = referenceCodesGetValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Return a list of valid Reference Codes (asynchronously)
* Return a list of valid Reference Codes
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call referenceCodesGetAsync(final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = referenceCodesGetValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for screeningsIdAdverseActionsPost
* @param id (required)
* @param adverseActionRequest (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call screeningsIdAdverseActionsPostCall(String id, AdverseActionRequest adverseActionRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = adverseActionRequest;
// create path and map variables
String localVarPath = "/screenings/{id}/adverse-actions"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"*/*"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call screeningsIdAdverseActionsPostValidateBeforeCall(String id, AdverseActionRequest adverseActionRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling screeningsIdAdverseActionsPost(Async)");
}
// verify the required parameter 'adverseActionRequest' is set
if (adverseActionRequest == null) {
throw new ApiException("Missing the required parameter 'adverseActionRequest' when calling screeningsIdAdverseActionsPost(Async)");
}
com.squareup.okhttp.Call call = screeningsIdAdverseActionsPostCall(id, adverseActionRequest, progressListener, progressRequestListener);
return call;
}
/**
* Create an adverse action on a Screening
* Create an adverse action on a Screening
* @param id (required)
* @param adverseActionRequest (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void screeningsIdAdverseActionsPost(String id, AdverseActionRequest adverseActionRequest) throws ApiException {
screeningsIdAdverseActionsPostWithHttpInfo(id, adverseActionRequest);
}
/**
* Create an adverse action on a Screening
* Create an adverse action on a Screening
* @param id (required)
* @param adverseActionRequest (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse screeningsIdAdverseActionsPostWithHttpInfo(String id, AdverseActionRequest adverseActionRequest) throws ApiException {
com.squareup.okhttp.Call call = screeningsIdAdverseActionsPostValidateBeforeCall(id, adverseActionRequest, null, null);
return apiClient.execute(call);
}
/**
* Create an adverse action on a Screening (asynchronously)
* Create an adverse action on a Screening
* @param id (required)
* @param adverseActionRequest (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call screeningsIdAdverseActionsPostAsync(String id, AdverseActionRequest adverseActionRequest, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = screeningsIdAdverseActionsPostValidateBeforeCall(id, adverseActionRequest, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for screeningsIdDetailsGet
* @param id (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call screeningsIdDetailsGetCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/screenings/{id}/details"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map