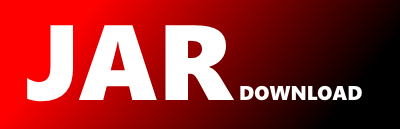
com.stratio.deep.entity.Cells Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deep-commons Show documentation
Show all versions of deep-commons Show documentation
stratio deep common utility classes
/*
* Copyright 2014, Stratio.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package com.stratio.deep.entity;
import com.stratio.deep.exception.DeepGenericException;
import java.io.Serializable;
import java.nio.ByteBuffer;
import java.util.*;
/**
* Represents a tuple inside the Cassandra's datastore. A Cells object basically is an ordered
* collection of {@link com.stratio.deep.entity.Cell} objects, plus a few utility methods to access
* specific cells in the row.
*
* @author Luca Rosellini
*/
public class Cells implements Iterable, Serializable {
private static final long serialVersionUID = 3074521612130550380L;
private List cells = new ArrayList<>();
/**
* Default constructor.
*/
public Cells() {
}
/**
* Builds a new Cells object containing the provided cells.
*
* @param cells the array of Cells we want to use to create the Cells object.
*/
public Cells(Cell... cells) {
Collections.addAll(this.cells, cells);
}
/**
* Adds a new Cell object to this Cells instance.
*
* @param c the Cell we want to add to this Cells object.
* @return either true/false if the Cell has been added successfully or not.
*/
public boolean add(Cell c) {
if (c == null) {
throw new DeepGenericException(new IllegalArgumentException("cell parameter cannot be null"));
}
return cells.add(c);
}
/**
* Replaces the cell having the same name that the given one with the given Cell object.
*
* @param c the Cell to replace the one in the Cells object.
* @return either true/false if the Cell has been successfully replace or not.
*/
public boolean replaceByName(Cell c) {
if (c == null) {
throw new DeepGenericException(new IllegalArgumentException("cell parameter cannot be null"));
}
boolean cellFound = false;
int position = 0;
Iterator cellsIt = cells.iterator();
while (!cellFound && cellsIt.hasNext()) {
Cell currentCell = cellsIt.next();
if (currentCell.getCellName().equals(c.getCellName())) {
cellFound = true;
} else {
position++;
}
}
if (cellFound) {
cells.remove(position);
return cells.add(c);
}
return false;
}
/**
* Removes the cell with the given cell name.
*
* @param cellName the name of the cell to be removed.
* @return either true/false if the Cell has been successfully removed or not.
*/
public boolean remove(String cellName) {
if (cellName == null) {
throw new DeepGenericException(new IllegalArgumentException(
"cell name parameter cannot be null"));
}
Iterator cellsIt = cells.iterator();
while (cellsIt.hasNext()) {
Cell currentCell = cellsIt.next();
if (currentCell.getCellName().equals(cellName)) {
return cells.remove(currentCell);
}
}
return false;
}
/**
* {@inheritDoc}
*/
@Override
public boolean equals(Object obj) {
if (!(obj instanceof Cells)) {
return false;
}
Cells o = (Cells) obj;
if (cells.size() != this.size()) {
return false;
}
for (Cell cell : cells) {
Cell otherCell = o.getCellByName(cell.getCellName());
if (otherCell == null) {
return false;
}
if (!otherCell.equals(cell)) {
return false;
}
}
return true;
}
/**
* Returns the cell at position idx.
*
* @param idx the index position of the Cell we want to retrieve.
* @return Returns the cell at position idx.
*/
public Cell getCellByIdx(int idx) {
return cells.get(idx);
}
/**
* Returns the Cell whose name is cellName, or null if this Cells object contains no cell whose
* name is cellName.
*
* @param cellName the name of the Cell we want to retrieve from this Cells object.
* @return the Cell whose name is cellName contained in this Cells object. null if no cell named
* cellName is present.
*/
public Cell getCellByName(String cellName) {
for (Cell c : cells) {
if (c.getCellName().equals(cellName)) {
return c;
}
}
return null;
}
/**
* @return Returns an immutable collection of Cell objects contained in this Cells.
*/
public Collection getCells() {
return Collections.unmodifiableList(cells);
}
/**
* Converts every Cell contained in this object to an ArrayBuffer. In order to perform the
* conversion we use the appropriate Cassandra marshaller for the Cell.
*
* @return a collection of Cell(s) values converted to byte buffers using the appropriate
* marshaller.
*/
public Collection getDecomposedCellValues() {
List res = new ArrayList<>();
for (Cell c : cells) {
res.add(c.getDecomposedCellValue());
}
return res;
}
/**
* Converts every Cell contained in this object to an ArrayBuffer. In order to perform the
* conversion we use the appropriate Cassandra marshaller for the Cell.
*
* @return a collection of Cell(s) values.
*/
public Collection | | | | |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy