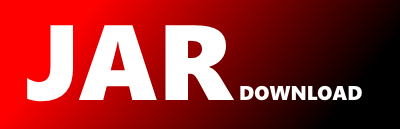
com.streamsend.pillar.Migration.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pillar Show documentation
Show all versions of pillar Show documentation
Pillar manages migrations for your Cassandra data stores.
package com.streamsend.pillar
import java.util.Date
import com.datastax.driver.core.Session
import com.datastax.driver.core.querybuilder.QueryBuilder
object Migration {
def apply(description: String, authoredAt: Date, up: String): Migration = {
new IrreversibleMigration(description, authoredAt, up)
}
def apply(description: String, authoredAt: Date, up: String, down: Option[String]): Migration = {
down match {
case Some(downStatement) =>
new ReversibleMigration(description, authoredAt, up, downStatement)
case None =>
new ReversibleMigrationWithNoOpDown(description, authoredAt, up)
}
}
}
trait Migration {
val description: String
val authoredAt: Date
val up: String
def key: MigrationKey = MigrationKey(authoredAt, description)
def authoredAfter(date: Date): Boolean = {
authoredAt.after(date)
}
def authoredBefore(date: Date): Boolean = {
authoredAt.compareTo(date) <= 0
}
def executeUpStatement(session: Session) {
session.execute(up)
insertIntoAppliedMigrations(session)
}
def executeDownStatement(session: Session)
protected def deleteFromAppliedMigrations(session: Session) {
session.execute(QueryBuilder.
delete().
from("applied_migrations").
where(QueryBuilder.eq("authored_at", authoredAt)).
and(QueryBuilder.eq("description", description))
)
}
private def insertIntoAppliedMigrations(session: Session) {
session.execute(QueryBuilder.
insertInto("applied_migrations").
value("authored_at", authoredAt).
value("description", description).
value("applied_at", System.currentTimeMillis())
)
}
}
class IrreversibleMigration(val description: String, val authoredAt: Date, val up: String) extends Migration {
def executeDownStatement(session: Session) {
throw new IrreversibleMigrationException(this)
}
}
class ReversibleMigrationWithNoOpDown(val description: String, val authoredAt: Date, val up: String) extends Migration {
def executeDownStatement(session: Session) {
deleteFromAppliedMigrations(session)
}
}
class ReversibleMigration(val description: String, val authoredAt: Date, val up: String, val down: String) extends Migration {
def executeDownStatement(session: Session) {
session.execute(down)
deleteFromAppliedMigrations(session)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy