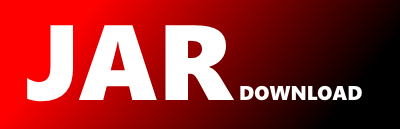
com.streamsets.pipeline.sdk.BatchContextSdkImpl Maven / Gradle / Ivy
/*
* Copyright (c) 2021 StreamSets Inc.
*/
package com.streamsets.pipeline.sdk;
import com.streamsets.datacollector.runner.StageContext;
import com.streamsets.pipeline.api.BatchContext;
import com.streamsets.pipeline.api.BatchMaker;
import com.streamsets.pipeline.api.ErrorCode;
import com.streamsets.pipeline.api.EventRecord;
import com.streamsets.pipeline.api.Record;
import java.util.Collection;
import java.util.List;
/**
* SDK implementation of the BatchContext interface
*/
public class BatchContextSdkImpl implements BatchContext {
private BatchMaker batchMaker;
private StageContext context;
public BatchContextSdkImpl(BatchMaker batchMaker, StageContext context) {
this.batchMaker = batchMaker;
this.context = context;
}
@Override
public BatchMaker getBatchMaker() {
return batchMaker;
}
@Override
public void toError(Record record, Exception exception) {
context.toError(record, exception);
}
@Override
public void toError(Record record, String errorMessage) {
context.toError(record, errorMessage);
}
@Override
public void toError(Record record, ErrorCode errorCode, Object... args) {
context.toError(record, errorCode, args);
}
@Override
public void toEvent(EventRecord record) {
context.toEvent(record);
}
@Override
public void complete(Record record) {
context.complete(record);
}
@Override
public void complete(Collection records) {
context.complete(records);
}
@Override
public List getSourceResponseRecords() {
return context.getSourceResponseSink().getResponseRecords();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy