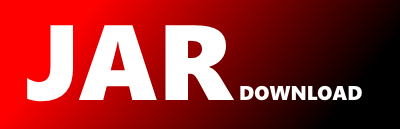
com.streamsets.pipeline.sdk.ExecutorRunner Maven / Gradle / Ivy
/*
* Copyright (c) 2021 StreamSets Inc.
*/
package com.streamsets.pipeline.sdk;
import com.streamsets.datacollector.main.RuntimeInfo;
import com.streamsets.datacollector.runner.BatchImpl;
import com.streamsets.pipeline.api.DeliveryGuarantee;
import com.streamsets.pipeline.api.ExecutionMode;
import com.streamsets.pipeline.api.Executor;
import com.streamsets.pipeline.api.OnRecordError;
import com.streamsets.pipeline.api.Record;
import com.streamsets.pipeline.api.StageException;
import com.streamsets.pipeline.api.StageType;
import com.streamsets.pipeline.api.impl.Utils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Collections;
import java.util.List;
import java.util.Map;
public class ExecutorRunner extends StageRunner {
private static final Logger LOG = LoggerFactory.getLogger(TargetRunner.class);
@SuppressWarnings("unchecked")
public ExecutorRunner(
Class executorClass,
Executor executor,
Map configuration,
boolean isPreview,
OnRecordError onRecordError,
Map constants,
Map stageSdcConf,
ExecutionMode executionMode,
DeliveryGuarantee deliveryGuarantee,
String resourcesDir,
RuntimeInfo runtimeInfo,
List services
) {
super(executorClass,
executor,
StageType.EXECUTOR,
configuration,
Collections.EMPTY_LIST,
isPreview,
onRecordError,
constants,
stageSdcConf,
executionMode,
deliveryGuarantee,
resourcesDir,
runtimeInfo,
services
);
}
@SuppressWarnings("unchecked")
public ExecutorRunner(
Class executorClass,
Map configuration,
boolean isPreview,
OnRecordError onRecordError,
Map constants,
Map stageSdcConf,
ExecutionMode executionMode,
DeliveryGuarantee deliveryGuarantee,
String resourcesDir,
RuntimeInfo runtimeInfo,
List services
) {
super(executorClass,
StageType.EXECUTOR,
configuration,
Collections.EMPTY_LIST,
isPreview,
onRecordError,
constants,
stageSdcConf,
executionMode,
deliveryGuarantee,
resourcesDir,
runtimeInfo,
services
);
}
public void runWrite(List inputRecords) throws StageException {
LOG.debug("Stage '{}' write starts", getInfo().getInstanceName());
ensureStatus(Status.INITIALIZED);
BatchImpl batch = new BatchImpl(getInfo().getInstanceName(), "sdk", "sourceOffset", inputRecords);
getStage().write(batch);
LOG.debug("Stage '{}' write ends", getInfo().getInstanceName());
}
public static class Builder extends StageRunner.Builder {
public Builder(Class extends Executor> executorClass, Executor executor) {
super((Class)executorClass, executor);
}
@SuppressWarnings("unchecked")
public Builder(Class extends Executor> executorClass) {
super((Class) executorClass);
}
@Override
public ExecutorRunner build() {
Utils.checkState(outputLanes.isEmpty(), "An Executor cannot have output streams");
if(stage != null) {
return new ExecutorRunner(
stageClass,
stage,
configs,
isPreview,
onRecordError,
constants,
stageSdcConf,
executionMode,
deliveryGuarantee,
resourcesDir,
runtimeInfo,
services
);
} else {
return new ExecutorRunner(
stageClass,
configs,
isPreview,
onRecordError,
constants,
stageSdcConf,
executionMode,
deliveryGuarantee,
resourcesDir,
runtimeInfo,
services
);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy