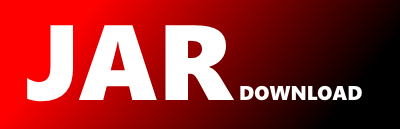
com.streamsets.pipeline.sdk.ServiceRunner Maven / Gradle / Ivy
/*
* Copyright (c) 2021 StreamSets Inc.
*/
package com.streamsets.pipeline.sdk;
import com.codahale.metrics.MetricRegistry;
import com.streamsets.datacollector.email.EmailSender;
import com.streamsets.datacollector.main.RuntimeInfo;
import com.streamsets.datacollector.runner.ServiceContext;
import com.streamsets.datacollector.util.Configuration;
import com.streamsets.datacollector.util.ContainerError;
import com.streamsets.pipeline.api.ConfigIssue;
import com.streamsets.pipeline.api.Stage;
import com.streamsets.pipeline.api.StageException;
import com.streamsets.pipeline.api.impl.Utils;
import com.streamsets.pipeline.api.service.Service;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class ServiceRunner extends ProtoRunner {
private static final Logger LOG = LoggerFactory.getLogger(ServiceRunner.class);
private final S service;
private final Class serviceClass;
private final ServiceContext serviceContext;
protected ServiceRunner(
S service,
Class serviceClass,
Map stageSdcConf,
Map configs,
Map constants,
String resourcesDir,
RuntimeInfo runtimeInfo
) {
this.service = service;
this.serviceClass = serviceClass;
try {
configureObject(service, configs);
} catch (Exception ex) {
throw new RuntimeException(ex);
}
this.serviceContext = new ServiceContext(
new Configuration(),
ElUtil.getConfigToElDefMap(serviceClass),
constants,
new EmailSender(new Configuration()),
new MetricRegistry(),
"myPipeline",
"0",
0,
"stageName",
"serviceName",
resourcesDir
);
Configuration sdcConfiguration = new Configuration();
stageSdcConf.forEach((k, v) -> sdcConfiguration.set("stage.conf_" + k, v));
status = Status.CREATED;
}
public S getService() {
return service;
}
public Class getServiceClass() {
return serviceClass;
}
public Service.Context getContext() {
return serviceContext;
}
public List runValidateConfigs() {
try {
LOG.debug("Service '{}' validateConfigs starts", serviceClass.getCanonicalName());
ensureStatus(Status.CREATED);
try {
return ((Service)service).init(getContext());
} finally {
((Service)service).destroy();
}
} finally {
LOG.debug("Service '{}' validateConfigs done", serviceClass.getCanonicalName());
}
}
@SuppressWarnings("unchecked")
public void runInit() throws StageException {
LOG.debug("Service '{}' init starts", serviceClass.getCanonicalName());
ensureStatus(Status.CREATED);
List issues = ((Service)service).init(getContext());
if (!issues.isEmpty()) {
List list = new ArrayList<>(issues.size());
for (Stage.ConfigIssue issue : issues) {
list.add(issue.toString());
}
throw new StageException(ContainerError.CONTAINER_0010, list);
}
status = Status.INITIALIZED;
LOG.debug("Service '{}' init ends", serviceClass.getCanonicalName());
}
public void runDestroy() throws StageException {
LOG.debug("Service '{}' destroy starts", serviceClass.getCanonicalName());
ensureStatus(Status.INITIALIZED);
((Service)service).destroy();
status = Status.DESTROYED;
LOG.debug("Service '{}' destroy ends", serviceClass.getCanonicalName());
}
public static class Builder {
final S service;
final Class serviceClass;
final Map stageSdcConf;
final Map configs;
final Map constants;
String resourcesDir;
RuntimeInfo runtimeInfo;
public Builder(Class serviceClass, S service) {
this.service = service;
this.serviceClass = serviceClass;
configs = new HashMap<>();
this.constants = new HashMap<>();
this.stageSdcConf = new HashMap<>();
this.runtimeInfo = new SdkRuntimeInfo("", null, null);
}
public B setResourcesDir(String resourcesDir) {
this.resourcesDir = resourcesDir;
return (B) this;
}
@SuppressWarnings("unchecked")
public B addConfiguration(String name, Object value) {
configs.put(Utils.checkNotNull(name, "name"), value);
return (B) this;
}
@SuppressWarnings("unchecked")
public B addStageSdcConfiguration(String name, String value) {
stageSdcConf.put(Utils.checkNotNull(name, "name"), value);
return (B) this;
}
public B addConstants(Map constants) {
this.constants.putAll(constants);
return (B) this;
}
public B setRuntimeInfo(RuntimeInfo runtimeInfo) {
this.runtimeInfo = runtimeInfo;
return (B) this;
}
public ServiceRunner build() {
return new ServiceRunner<>(
service,
serviceClass,
stageSdcConf,
configs,
constants,
resourcesDir,
runtimeInfo
);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy