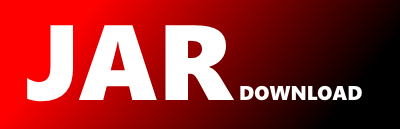
com.stripe.model.Account Maven / Gradle / Ivy
// Generated by delombok at Wed May 29 17:45:06 PDT 2019
// Generated by com.stripe.generator.entity.SdkBuilder
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.AccountCapabilitiesParams;
import com.stripe.param.AccountCreateParams;
import com.stripe.param.AccountListParams;
import com.stripe.param.AccountPersonsParams;
import com.stripe.param.AccountRejectParams;
import com.stripe.param.AccountRetrieveParams;
import com.stripe.param.AccountUpdateParams;
import java.util.List;
import java.util.Map;
public class Account extends ApiResource implements PaymentSource, MetadataStore {
/**
* Optional information related to the business.
*/
@SerializedName("business_profile")
BusinessProfile businessProfile;
/**
* The business type. Can be `individual` or `company`.
*/
@SerializedName("business_type")
String businessType;
@SerializedName("capabilities")
Capabilities capabilities;
/**
* Whether the account can create live charges.
*/
@SerializedName("charges_enabled")
Boolean chargesEnabled;
@SerializedName("company")
Company company;
/**
* The account's country.
*/
@SerializedName("country")
String country;
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* Three-letter ISO currency code representing the default currency for the account. This must be
* a currency that [Stripe supports in the account's country](https://stripe.com/docs/payouts).
*/
@SerializedName("default_currency")
String defaultCurrency;
/**
* Always true for a deleted object.
*/
@SerializedName("deleted")
Boolean deleted;
/**
* Whether account details have been submitted. Standard accounts cannot receive payouts before
* this is true.
*/
@SerializedName("details_submitted")
Boolean detailsSubmitted;
/**
* The primary user's email address.
*/
@SerializedName("email")
String email;
/**
* External accounts (bank accounts and debit cards) currently attached to this account.
*/
@SerializedName("external_accounts")
ExternalAccountCollection externalAccounts;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
@SerializedName("individual")
Person individual;
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* String representing the object's type. Objects of the same type share the same value.
*/
@SerializedName("object")
String object;
/**
* Whether Stripe can send payouts to this account.
*/
@SerializedName("payouts_enabled")
Boolean payoutsEnabled;
@SerializedName("requirements")
Requirements requirements;
/**
* Account options for customizing how the account functions within Stripe.
*/
@SerializedName("settings")
Settings settings;
@SerializedName("tos_acceptance")
TosAcceptance tosAcceptance;
/**
* The Stripe account type. Can be `standard`, `express`, or `custom`.
*/
@SerializedName("type")
String type;
/**
* Retrieves the details of an account.
*/
public static Account retrieve() throws StripeException {
return retrieve((Map) null, (RequestOptions) null);
}
/**
* Retrieves the details of an account.
*/
public static Account retrieve(RequestOptions options) throws StripeException {
return retrieve((Map) null, options);
}
/**
* Retrieves the details of an account.
*/
public static Account retrieve(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/account");
return request(ApiResource.RequestMethod.GET, url, params, Account.class, options);
}
/**
* Retrieves the details of an account.
*/
public static Account retrieve(AccountRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/account");
return request(ApiResource.RequestMethod.GET, url, params, Account.class, options);
}
/**
* Retrieves the details of an account.
*/
public static Account retrieve(String account) throws StripeException {
return retrieve(account, (Map) null, (RequestOptions) null);
}
/**
* Retrieves the details of an account.
*/
public static Account retrieve(String account, RequestOptions options) throws StripeException {
return retrieve(account, (Map) null, options);
}
/**
* Retrieves the details of an account.
*/
public static Account retrieve(String account, Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/accounts/%s", ApiResource.urlEncodeId(account)));
return request(ApiResource.RequestMethod.GET, url, params, Account.class, options);
}
/**
* Retrieves the details of an account.
*/
public static Account retrieve(String account, AccountRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/accounts/%s", ApiResource.urlEncodeId(account)));
return request(ApiResource.RequestMethod.GET, url, params, Account.class, options);
}
/**
* Updates a connected Express or Custom account by setting
* the values of the parameters passed. Any parameters not provided are left unchanged. Most
* parameters can be changed only for Custom accounts. (These are marked Custom
* Only below.) Parameters marked Custom and Express are supported by
* both account types.
*
* To update your own account, use the Dashboard. Refer to our Connect documentation to learn more about updating
* accounts.
*/
@Override
public Account update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates a connected Express or Custom account by setting
* the values of the parameters passed. Any parameters not provided are left unchanged. Most
* parameters can be changed only for Custom accounts. (These are marked Custom
* Only below.) Parameters marked Custom and Express are supported by
* both account types.
*
* To update your own account, use the Dashboard. Refer to our Connect documentation to learn more about updating
* accounts.
*/
@Override
public Account update(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/accounts/%s", ApiResource.urlEncodeId(this.getId())));
return request(ApiResource.RequestMethod.POST, url, params, Account.class, options);
}
/**
* Updates a connected Express or Custom account by setting
* the values of the parameters passed. Any parameters not provided are left unchanged. Most
* parameters can be changed only for Custom accounts. (These are marked Custom
* Only below.) Parameters marked Custom and Express are supported by
* both account types.
*
* To update your own account, use the Dashboard. Refer to our Connect documentation to learn more about updating
* accounts.
*/
public Account update(AccountUpdateParams params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates a connected Express or Custom account by setting
* the values of the parameters passed. Any parameters not provided are left unchanged. Most
* parameters can be changed only for Custom accounts. (These are marked Custom
* Only below.) Parameters marked Custom and Express are supported by
* both account types.
*
*
To update your own account, use the Dashboard. Refer to our Connect documentation to learn more about updating
* accounts.
*/
public Account update(AccountUpdateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/accounts/%s", ApiResource.urlEncodeId(this.getId())));
return request(ApiResource.RequestMethod.POST, url, params, Account.class, options);
}
/**
* Returns a list of accounts connected to your platform via Connect.
* If you’re not a platform, the list is empty.
*/
public static AccountCollection list(Map params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of accounts connected to your platform via Connect.
* If you’re not a platform, the list is empty.
*/
public static AccountCollection list(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/accounts");
return requestCollection(url, params, AccountCollection.class, options);
}
/**
* Returns a list of accounts connected to your platform via Connect.
* If you’re not a platform, the list is empty.
*/
public static AccountCollection list(AccountListParams params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of accounts connected to your platform via Connect.
* If you’re not a platform, the list is empty.
*/
public static AccountCollection list(AccountListParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/accounts");
return requestCollection(url, params, AccountCollection.class, options);
}
/**
* With Connect, you can create Stripe accounts for your users. To do
* this, you’ll first need to register your platform.
*
* For Standard accounts, parameters other than country
, email
, and
* type
are used to prefill the account application that we ask the account holder to
* complete.
*/
public static Account create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* With Connect, you can create Stripe accounts for your users. To do
* this, you’ll first need to register your platform.
*
* For Standard accounts, parameters other than country
, email
, and
* type
are used to prefill the account application that we ask the account holder to
* complete.
*/
public static Account create(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/accounts");
return request(ApiResource.RequestMethod.POST, url, params, Account.class, options);
}
/**
* With Connect, you can create Stripe accounts for your users. To do
* this, you’ll first need to register your platform.
*
* For Standard accounts, parameters other than country
, email
, and
* type
are used to prefill the account application that we ask the account holder to
* complete.
*/
public static Account create(AccountCreateParams params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* With Connect, you can create Stripe accounts for your users. To do
* this, you’ll first need to register your platform.
*
*
For Standard accounts, parameters other than country
, email
, and
* type
are used to prefill the account application that we ask the account holder to
* complete.
*/
public static Account create(AccountCreateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/accounts");
return request(ApiResource.RequestMethod.POST, url, params, Account.class, options);
}
/**
* With Connect, you may delete Custom accounts you manage.
*
*
Custom accounts created using test-mode keys can be deleted at any time. Custom accounts
* created using live-mode keys may only be deleted once all balances are zero.
*
*
If you are looking to close your own account, use the data tab in your account settings instead.
*/
public Account delete() throws StripeException {
return delete((Map) null, (RequestOptions) null);
}
/**
* With Connect, you may delete Custom accounts you manage.
*
* Custom accounts created using test-mode keys can be deleted at any time. Custom accounts
* created using live-mode keys may only be deleted once all balances are zero.
*
*
If you are looking to close your own account, use the data tab in your account settings instead.
*/
public Account delete(RequestOptions options) throws StripeException {
return delete((Map) null, options);
}
/**
* With Connect, you may delete Custom accounts you manage.
*
* Custom accounts created using test-mode keys can be deleted at any time. Custom accounts
* created using live-mode keys may only be deleted once all balances are zero.
*
*
If you are looking to close your own account, use the data tab in your account settings instead.
*/
public Account delete(Map params) throws StripeException {
return delete(params, (RequestOptions) null);
}
/**
* With Connect, you may delete Custom accounts you manage.
*
* Custom accounts created using test-mode keys can be deleted at any time. Custom accounts
* created using live-mode keys may only be deleted once all balances are zero.
*
*
If you are looking to close your own account, use the data tab in your account settings instead.
*/
public Account delete(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/accounts/%s", ApiResource.urlEncodeId(this.getId())));
return request(ApiResource.RequestMethod.DELETE, url, params, Account.class, options);
}
/**
* With Connect, you may flag accounts as suspicious.
*
* Test-mode Custom and Express accounts can be rejected at any time. Accounts created using
* live-mode keys may only be rejected once all balances are zero.
*/
public Account reject(Map params) throws StripeException {
return reject(params, (RequestOptions) null);
}
/**
* With Connect, you may flag accounts as suspicious.
*
* Test-mode Custom and Express accounts can be rejected at any time. Accounts created using
* live-mode keys may only be rejected once all balances are zero.
*/
public Account reject(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/accounts/%s/reject", ApiResource.urlEncodeId(this.getId())));
return request(ApiResource.RequestMethod.POST, url, params, Account.class, options);
}
/**
* With Connect, you may flag accounts as suspicious.
*
* Test-mode Custom and Express accounts can be rejected at any time. Accounts created using
* live-mode keys may only be rejected once all balances are zero.
*/
public Account reject(AccountRejectParams params) throws StripeException {
return reject(params, (RequestOptions) null);
}
/**
* With Connect, you may flag accounts as suspicious.
*
*
Test-mode Custom and Express accounts can be rejected at any time. Accounts created using
* live-mode keys may only be rejected once all balances are zero.
*/
public Account reject(AccountRejectParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/accounts/%s/reject", ApiResource.urlEncodeId(this.getId())));
return request(ApiResource.RequestMethod.POST, url, params, Account.class, options);
}
/**
* Returns a list of people associated with the account’s legal entity. The people are returned
* sorted by creation date, with the most recent people appearing first.
*/
public PersonCollection persons() throws StripeException {
return persons((Map) null, (RequestOptions) null);
}
/**
* Returns a list of people associated with the account’s legal entity. The people are returned
* sorted by creation date, with the most recent people appearing first.
*/
public PersonCollection persons(Map params) throws StripeException {
return persons(params, (RequestOptions) null);
}
/**
* Returns a list of people associated with the account’s legal entity. The people are returned
* sorted by creation date, with the most recent people appearing first.
*/
public PersonCollection persons(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/accounts/%s/persons", ApiResource.urlEncodeId(this.getId())));
return requestCollection(url, params, PersonCollection.class, options);
}
/**
* Returns a list of people associated with the account’s legal entity. The people are returned
* sorted by creation date, with the most recent people appearing first.
*/
public PersonCollection persons(AccountPersonsParams params) throws StripeException {
return persons(params, (RequestOptions) null);
}
/**
* Returns a list of people associated with the account’s legal entity. The people are returned
* sorted by creation date, with the most recent people appearing first.
*/
public PersonCollection persons(AccountPersonsParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/accounts/%s/persons", ApiResource.urlEncodeId(this.getId())));
return requestCollection(url, params, PersonCollection.class, options);
}
/**
* Returns a list of capabilities associated with the account. The capabilities are returned
* sorted by creation date, with the most recent capability appearing first.
*/
public CapabilityCollection capabilities() throws StripeException {
return capabilities((Map) null, (RequestOptions) null);
}
/**
* Returns a list of capabilities associated with the account. The capabilities are returned
* sorted by creation date, with the most recent capability appearing first.
*/
public CapabilityCollection capabilities(Map params) throws StripeException {
return capabilities(params, (RequestOptions) null);
}
/**
* Returns a list of capabilities associated with the account. The capabilities are returned
* sorted by creation date, with the most recent capability appearing first.
*/
public CapabilityCollection capabilities(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/accounts/%s/capabilities", ApiResource.urlEncodeId(this.getId())));
return requestCollection(url, params, CapabilityCollection.class, options);
}
/**
* Returns a list of capabilities associated with the account. The capabilities are returned
* sorted by creation date, with the most recent capability appearing first.
*/
public CapabilityCollection capabilities(AccountCapabilitiesParams params) throws StripeException {
return capabilities(params, (RequestOptions) null);
}
/**
* Returns a list of capabilities associated with the account. The capabilities are returned
* sorted by creation date, with the most recent capability appearing first.
*/
public CapabilityCollection capabilities(AccountCapabilitiesParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/accounts/%s/capabilities", ApiResource.urlEncodeId(this.getId())));
return requestCollection(url, params, CapabilityCollection.class, options);
}
public static class BusinessProfile extends StripeObject {
/**
* The merchant category code for the account. MCCs are used to classify businesses based on the
* goods or services they provide.
*/
@SerializedName("mcc")
String mcc;
/**
* The customer-facing business name.
*/
@SerializedName("name")
String name;
/**
* Internal-only description of the product sold or service provided by the business. It's used
* by Stripe for risk and underwriting purposes.
*/
@SerializedName("product_description")
String productDescription;
/**
* A publicly available mailing address for sending support issues to.
*/
@SerializedName("support_address")
Address supportAddress;
/**
* A publicly available email address for sending support issues to.
*/
@SerializedName("support_email")
String supportEmail;
/**
* A publicly available phone number to call with support issues.
*/
@SerializedName("support_phone")
String supportPhone;
/**
* A publicly available website for handling support issues.
*/
@SerializedName("support_url")
String supportUrl;
/**
* The business's publicly available website.
*/
@SerializedName("url")
String url;
/**
* The merchant category code for the account. MCCs are used to classify businesses based on the
* goods or services they provide.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getMcc() {
return this.mcc;
}
/**
* The customer-facing business name.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getName() {
return this.name;
}
/**
* Internal-only description of the product sold or service provided by the business. It's used
* by Stripe for risk and underwriting purposes.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getProductDescription() {
return this.productDescription;
}
/**
* A publicly available mailing address for sending support issues to.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Address getSupportAddress() {
return this.supportAddress;
}
/**
* A publicly available email address for sending support issues to.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSupportEmail() {
return this.supportEmail;
}
/**
* A publicly available phone number to call with support issues.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSupportPhone() {
return this.supportPhone;
}
/**
* A publicly available website for handling support issues.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSupportUrl() {
return this.supportUrl;
}
/**
* The business's publicly available website.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUrl() {
return this.url;
}
/**
* The merchant category code for the account. MCCs are used to classify businesses based on the
* goods or services they provide.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMcc(final String mcc) {
this.mcc = mcc;
}
/**
* The customer-facing business name.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setName(final String name) {
this.name = name;
}
/**
* Internal-only description of the product sold or service provided by the business. It's used
* by Stripe for risk and underwriting purposes.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setProductDescription(final String productDescription) {
this.productDescription = productDescription;
}
/**
* A publicly available mailing address for sending support issues to.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSupportAddress(final Address supportAddress) {
this.supportAddress = supportAddress;
}
/**
* A publicly available email address for sending support issues to.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSupportEmail(final String supportEmail) {
this.supportEmail = supportEmail;
}
/**
* A publicly available phone number to call with support issues.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSupportPhone(final String supportPhone) {
this.supportPhone = supportPhone;
}
/**
* A publicly available website for handling support issues.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSupportUrl(final String supportUrl) {
this.supportUrl = supportUrl;
}
/**
* The business's publicly available website.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUrl(final String url) {
this.url = url;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Account.BusinessProfile)) return false;
final Account.BusinessProfile other = (Account.BusinessProfile) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$mcc = this.getMcc();
final java.lang.Object other$mcc = other.getMcc();
if (this$mcc == null ? other$mcc != null : !this$mcc.equals(other$mcc)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$productDescription = this.getProductDescription();
final java.lang.Object other$productDescription = other.getProductDescription();
if (this$productDescription == null ? other$productDescription != null : !this$productDescription.equals(other$productDescription)) return false;
final java.lang.Object this$supportAddress = this.getSupportAddress();
final java.lang.Object other$supportAddress = other.getSupportAddress();
if (this$supportAddress == null ? other$supportAddress != null : !this$supportAddress.equals(other$supportAddress)) return false;
final java.lang.Object this$supportEmail = this.getSupportEmail();
final java.lang.Object other$supportEmail = other.getSupportEmail();
if (this$supportEmail == null ? other$supportEmail != null : !this$supportEmail.equals(other$supportEmail)) return false;
final java.lang.Object this$supportPhone = this.getSupportPhone();
final java.lang.Object other$supportPhone = other.getSupportPhone();
if (this$supportPhone == null ? other$supportPhone != null : !this$supportPhone.equals(other$supportPhone)) return false;
final java.lang.Object this$supportUrl = this.getSupportUrl();
final java.lang.Object other$supportUrl = other.getSupportUrl();
if (this$supportUrl == null ? other$supportUrl != null : !this$supportUrl.equals(other$supportUrl)) return false;
final java.lang.Object this$url = this.getUrl();
final java.lang.Object other$url = other.getUrl();
if (this$url == null ? other$url != null : !this$url.equals(other$url)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Account.BusinessProfile;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $mcc = this.getMcc();
result = result * PRIME + ($mcc == null ? 43 : $mcc.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $productDescription = this.getProductDescription();
result = result * PRIME + ($productDescription == null ? 43 : $productDescription.hashCode());
final java.lang.Object $supportAddress = this.getSupportAddress();
result = result * PRIME + ($supportAddress == null ? 43 : $supportAddress.hashCode());
final java.lang.Object $supportEmail = this.getSupportEmail();
result = result * PRIME + ($supportEmail == null ? 43 : $supportEmail.hashCode());
final java.lang.Object $supportPhone = this.getSupportPhone();
result = result * PRIME + ($supportPhone == null ? 43 : $supportPhone.hashCode());
final java.lang.Object $supportUrl = this.getSupportUrl();
result = result * PRIME + ($supportUrl == null ? 43 : $supportUrl.hashCode());
final java.lang.Object $url = this.getUrl();
result = result * PRIME + ($url == null ? 43 : $url.hashCode());
return result;
}
}
public static class Capabilities extends StripeObject {
/**
* The status of the card payments capability of the account, or whether the account can
* directly process credit and debit card charges.
*/
@SerializedName("card_payments")
String cardPayments;
/**
* The status of the legacy payments capability of the account.
*/
@SerializedName("legacy_payments")
String legacyPayments;
/**
* The status of the platform payments capability of the account, or whether your platform can
* process charges on behalf of the account.
*/
@SerializedName("platform_payments")
String platformPayments;
/**
* The status of the card payments capability of the account, or whether the account can
* directly process credit and debit card charges.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCardPayments() {
return this.cardPayments;
}
/**
* The status of the legacy payments capability of the account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLegacyPayments() {
return this.legacyPayments;
}
/**
* The status of the platform payments capability of the account, or whether your platform can
* process charges on behalf of the account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPlatformPayments() {
return this.platformPayments;
}
/**
* The status of the card payments capability of the account, or whether the account can
* directly process credit and debit card charges.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCardPayments(final String cardPayments) {
this.cardPayments = cardPayments;
}
/**
* The status of the legacy payments capability of the account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLegacyPayments(final String legacyPayments) {
this.legacyPayments = legacyPayments;
}
/**
* The status of the platform payments capability of the account, or whether your platform can
* process charges on behalf of the account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPlatformPayments(final String platformPayments) {
this.platformPayments = platformPayments;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Account.Capabilities)) return false;
final Account.Capabilities other = (Account.Capabilities) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$cardPayments = this.getCardPayments();
final java.lang.Object other$cardPayments = other.getCardPayments();
if (this$cardPayments == null ? other$cardPayments != null : !this$cardPayments.equals(other$cardPayments)) return false;
final java.lang.Object this$legacyPayments = this.getLegacyPayments();
final java.lang.Object other$legacyPayments = other.getLegacyPayments();
if (this$legacyPayments == null ? other$legacyPayments != null : !this$legacyPayments.equals(other$legacyPayments)) return false;
final java.lang.Object this$platformPayments = this.getPlatformPayments();
final java.lang.Object other$platformPayments = other.getPlatformPayments();
if (this$platformPayments == null ? other$platformPayments != null : !this$platformPayments.equals(other$platformPayments)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Account.Capabilities;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $cardPayments = this.getCardPayments();
result = result * PRIME + ($cardPayments == null ? 43 : $cardPayments.hashCode());
final java.lang.Object $legacyPayments = this.getLegacyPayments();
result = result * PRIME + ($legacyPayments == null ? 43 : $legacyPayments.hashCode());
final java.lang.Object $platformPayments = this.getPlatformPayments();
result = result * PRIME + ($platformPayments == null ? 43 : $platformPayments.hashCode());
return result;
}
}
public static class Company extends StripeObject {
@SerializedName("address")
Address address;
/**
* The Kana variation of the company's primary address (Japan only).
*/
@SerializedName("address_kana")
Person.JapanAddress addressKana;
/**
* The Kanji variation of the company's primary address (Japan only).
*/
@SerializedName("address_kanji")
Person.JapanAddress addressKanji;
/**
* Whether the company's directors have been provided. This Boolean will be `true` if you've
* manually indicated that all directors are provided via [the `directors_provided`
* parameter](https://stripe.com/docs/api/accounts/update#update_account-company-directors_provided).
*/
@SerializedName("directors_provided")
Boolean directorsProvided;
/**
* The company's legal name.
*/
@SerializedName("name")
String name;
/**
* The Kana variation of the company's legal name Japan only).
*/
@SerializedName("name_kana")
String nameKana;
/**
* The Kanji variation of the company's legal name (Japan only).
*/
@SerializedName("name_kanji")
String nameKanji;
/**
* Whether the company's owners have been provided. This Boolean will be `true` if you've
* manually indicated that all owners are provided via [the `owners_provided`
* parameter](https://stripe.com/docs/api/accounts/update#update_account-company-owners_provided),
* or if Stripe determined that all owners were provided. Stripe determines ownership
* requirements using both the number of owners provided and their total percent ownership
* (calculated by adding the `percent_ownership` of each owner together).
*/
@SerializedName("owners_provided")
Boolean ownersProvided;
/**
* The company's phone number (used for verification).
*/
@SerializedName("phone")
String phone;
/**
* Whether the company's business ID number was provided.
*/
@SerializedName("tax_id_provided")
Boolean taxIdProvided;
/**
* The jurisdiction in which the `tax_id` is registered (Germany-based companies only).
*/
@SerializedName("tax_id_registrar")
String taxIdRegistrar;
/**
* Whether the company's business VAT number was provided.
*/
@SerializedName("vat_id_provided")
Boolean vatIdProvided;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Address getAddress() {
return this.address;
}
/**
* The Kana variation of the company's primary address (Japan only).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Person.JapanAddress getAddressKana() {
return this.addressKana;
}
/**
* The Kanji variation of the company's primary address (Japan only).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Person.JapanAddress getAddressKanji() {
return this.addressKanji;
}
/**
* Whether the company's directors have been provided. This Boolean will be `true` if you've
* manually indicated that all directors are provided via [the `directors_provided`
* parameter](https://stripe.com/docs/api/accounts/update#update_account-company-directors_provided).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getDirectorsProvided() {
return this.directorsProvided;
}
/**
* The company's legal name.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getName() {
return this.name;
}
/**
* The Kana variation of the company's legal name Japan only).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getNameKana() {
return this.nameKana;
}
/**
* The Kanji variation of the company's legal name (Japan only).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getNameKanji() {
return this.nameKanji;
}
/**
* Whether the company's owners have been provided. This Boolean will be `true` if you've
* manually indicated that all owners are provided via [the `owners_provided`
* parameter](https://stripe.com/docs/api/accounts/update#update_account-company-owners_provided),
* or if Stripe determined that all owners were provided. Stripe determines ownership
* requirements using both the number of owners provided and their total percent ownership
* (calculated by adding the `percent_ownership` of each owner together).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getOwnersProvided() {
return this.ownersProvided;
}
/**
* The company's phone number (used for verification).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPhone() {
return this.phone;
}
/**
* Whether the company's business ID number was provided.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getTaxIdProvided() {
return this.taxIdProvided;
}
/**
* The jurisdiction in which the `tax_id` is registered (Germany-based companies only).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTaxIdRegistrar() {
return this.taxIdRegistrar;
}
/**
* Whether the company's business VAT number was provided.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getVatIdProvided() {
return this.vatIdProvided;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddress(final Address address) {
this.address = address;
}
/**
* The Kana variation of the company's primary address (Japan only).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddressKana(final Person.JapanAddress addressKana) {
this.addressKana = addressKana;
}
/**
* The Kanji variation of the company's primary address (Japan only).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddressKanji(final Person.JapanAddress addressKanji) {
this.addressKanji = addressKanji;
}
/**
* Whether the company's directors have been provided. This Boolean will be `true` if you've
* manually indicated that all directors are provided via [the `directors_provided`
* parameter](https://stripe.com/docs/api/accounts/update#update_account-company-directors_provided).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDirectorsProvided(final Boolean directorsProvided) {
this.directorsProvided = directorsProvided;
}
/**
* The company's legal name.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setName(final String name) {
this.name = name;
}
/**
* The Kana variation of the company's legal name Japan only).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setNameKana(final String nameKana) {
this.nameKana = nameKana;
}
/**
* The Kanji variation of the company's legal name (Japan only).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setNameKanji(final String nameKanji) {
this.nameKanji = nameKanji;
}
/**
* Whether the company's owners have been provided. This Boolean will be `true` if you've
* manually indicated that all owners are provided via [the `owners_provided`
* parameter](https://stripe.com/docs/api/accounts/update#update_account-company-owners_provided),
* or if Stripe determined that all owners were provided. Stripe determines ownership
* requirements using both the number of owners provided and their total percent ownership
* (calculated by adding the `percent_ownership` of each owner together).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setOwnersProvided(final Boolean ownersProvided) {
this.ownersProvided = ownersProvided;
}
/**
* The company's phone number (used for verification).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPhone(final String phone) {
this.phone = phone;
}
/**
* Whether the company's business ID number was provided.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxIdProvided(final Boolean taxIdProvided) {
this.taxIdProvided = taxIdProvided;
}
/**
* The jurisdiction in which the `tax_id` is registered (Germany-based companies only).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxIdRegistrar(final String taxIdRegistrar) {
this.taxIdRegistrar = taxIdRegistrar;
}
/**
* Whether the company's business VAT number was provided.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVatIdProvided(final Boolean vatIdProvided) {
this.vatIdProvided = vatIdProvided;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Account.Company)) return false;
final Account.Company other = (Account.Company) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$address = this.getAddress();
final java.lang.Object other$address = other.getAddress();
if (this$address == null ? other$address != null : !this$address.equals(other$address)) return false;
final java.lang.Object this$addressKana = this.getAddressKana();
final java.lang.Object other$addressKana = other.getAddressKana();
if (this$addressKana == null ? other$addressKana != null : !this$addressKana.equals(other$addressKana)) return false;
final java.lang.Object this$addressKanji = this.getAddressKanji();
final java.lang.Object other$addressKanji = other.getAddressKanji();
if (this$addressKanji == null ? other$addressKanji != null : !this$addressKanji.equals(other$addressKanji)) return false;
final java.lang.Object this$directorsProvided = this.getDirectorsProvided();
final java.lang.Object other$directorsProvided = other.getDirectorsProvided();
if (this$directorsProvided == null ? other$directorsProvided != null : !this$directorsProvided.equals(other$directorsProvided)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$nameKana = this.getNameKana();
final java.lang.Object other$nameKana = other.getNameKana();
if (this$nameKana == null ? other$nameKana != null : !this$nameKana.equals(other$nameKana)) return false;
final java.lang.Object this$nameKanji = this.getNameKanji();
final java.lang.Object other$nameKanji = other.getNameKanji();
if (this$nameKanji == null ? other$nameKanji != null : !this$nameKanji.equals(other$nameKanji)) return false;
final java.lang.Object this$ownersProvided = this.getOwnersProvided();
final java.lang.Object other$ownersProvided = other.getOwnersProvided();
if (this$ownersProvided == null ? other$ownersProvided != null : !this$ownersProvided.equals(other$ownersProvided)) return false;
final java.lang.Object this$phone = this.getPhone();
final java.lang.Object other$phone = other.getPhone();
if (this$phone == null ? other$phone != null : !this$phone.equals(other$phone)) return false;
final java.lang.Object this$taxIdProvided = this.getTaxIdProvided();
final java.lang.Object other$taxIdProvided = other.getTaxIdProvided();
if (this$taxIdProvided == null ? other$taxIdProvided != null : !this$taxIdProvided.equals(other$taxIdProvided)) return false;
final java.lang.Object this$taxIdRegistrar = this.getTaxIdRegistrar();
final java.lang.Object other$taxIdRegistrar = other.getTaxIdRegistrar();
if (this$taxIdRegistrar == null ? other$taxIdRegistrar != null : !this$taxIdRegistrar.equals(other$taxIdRegistrar)) return false;
final java.lang.Object this$vatIdProvided = this.getVatIdProvided();
final java.lang.Object other$vatIdProvided = other.getVatIdProvided();
if (this$vatIdProvided == null ? other$vatIdProvided != null : !this$vatIdProvided.equals(other$vatIdProvided)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Account.Company;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $address = this.getAddress();
result = result * PRIME + ($address == null ? 43 : $address.hashCode());
final java.lang.Object $addressKana = this.getAddressKana();
result = result * PRIME + ($addressKana == null ? 43 : $addressKana.hashCode());
final java.lang.Object $addressKanji = this.getAddressKanji();
result = result * PRIME + ($addressKanji == null ? 43 : $addressKanji.hashCode());
final java.lang.Object $directorsProvided = this.getDirectorsProvided();
result = result * PRIME + ($directorsProvided == null ? 43 : $directorsProvided.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $nameKana = this.getNameKana();
result = result * PRIME + ($nameKana == null ? 43 : $nameKana.hashCode());
final java.lang.Object $nameKanji = this.getNameKanji();
result = result * PRIME + ($nameKanji == null ? 43 : $nameKanji.hashCode());
final java.lang.Object $ownersProvided = this.getOwnersProvided();
result = result * PRIME + ($ownersProvided == null ? 43 : $ownersProvided.hashCode());
final java.lang.Object $phone = this.getPhone();
result = result * PRIME + ($phone == null ? 43 : $phone.hashCode());
final java.lang.Object $taxIdProvided = this.getTaxIdProvided();
result = result * PRIME + ($taxIdProvided == null ? 43 : $taxIdProvided.hashCode());
final java.lang.Object $taxIdRegistrar = this.getTaxIdRegistrar();
result = result * PRIME + ($taxIdRegistrar == null ? 43 : $taxIdRegistrar.hashCode());
final java.lang.Object $vatIdProvided = this.getVatIdProvided();
result = result * PRIME + ($vatIdProvided == null ? 43 : $vatIdProvided.hashCode());
return result;
}
}
public static class DeclineChargeOn extends StripeObject {
/**
* Whether Stripe automatically declines charges with an incorrect ZIP or postal code. This
* setting only applies when a ZIP or postal code is provided and they fail bank verification.
*/
@SerializedName("avs_failure")
Boolean avsFailure;
/**
* Whether Stripe automatically declines charges with an incorrect CVC. This setting only
* applies when a CVC is provided and it fails bank verification.
*/
@SerializedName("cvc_failure")
Boolean cvcFailure;
/**
* Whether Stripe automatically declines charges with an incorrect ZIP or postal code. This
* setting only applies when a ZIP or postal code is provided and they fail bank verification.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getAvsFailure() {
return this.avsFailure;
}
/**
* Whether Stripe automatically declines charges with an incorrect CVC. This setting only
* applies when a CVC is provided and it fails bank verification.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getCvcFailure() {
return this.cvcFailure;
}
/**
* Whether Stripe automatically declines charges with an incorrect ZIP or postal code. This
* setting only applies when a ZIP or postal code is provided and they fail bank verification.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAvsFailure(final Boolean avsFailure) {
this.avsFailure = avsFailure;
}
/**
* Whether Stripe automatically declines charges with an incorrect CVC. This setting only
* applies when a CVC is provided and it fails bank verification.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCvcFailure(final Boolean cvcFailure) {
this.cvcFailure = cvcFailure;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Account.DeclineChargeOn)) return false;
final Account.DeclineChargeOn other = (Account.DeclineChargeOn) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$avsFailure = this.getAvsFailure();
final java.lang.Object other$avsFailure = other.getAvsFailure();
if (this$avsFailure == null ? other$avsFailure != null : !this$avsFailure.equals(other$avsFailure)) return false;
final java.lang.Object this$cvcFailure = this.getCvcFailure();
final java.lang.Object other$cvcFailure = other.getCvcFailure();
if (this$cvcFailure == null ? other$cvcFailure != null : !this$cvcFailure.equals(other$cvcFailure)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Account.DeclineChargeOn;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $avsFailure = this.getAvsFailure();
result = result * PRIME + ($avsFailure == null ? 43 : $avsFailure.hashCode());
final java.lang.Object $cvcFailure = this.getCvcFailure();
result = result * PRIME + ($cvcFailure == null ? 43 : $cvcFailure.hashCode());
return result;
}
}
public static class PayoutSchedule extends StripeObject {
/**
* The number of days charges for the account will be held before being paid out.
*/
@SerializedName("delay_days")
Long delayDays;
/**
* How frequently funds will be paid out. One of `manual` (payouts only created via API call),
* `daily`, `weekly`, or `monthly`.
*/
@SerializedName("interval")
String interval;
/**
* The day of the month funds will be paid out. Only shown if `interval` is monthly. Payouts
* scheduled between the 29th and 31st of the month are sent on the last day of shorter months.
*/
@SerializedName("monthly_anchor")
Long monthlyAnchor;
/**
* The day of the week funds will be paid out, of the style 'monday', 'tuesday', etc. Only shown
* if `interval` is weekly.
*/
@SerializedName("weekly_anchor")
String weeklyAnchor;
/**
* The number of days charges for the account will be held before being paid out.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDelayDays() {
return this.delayDays;
}
/**
* How frequently funds will be paid out. One of `manual` (payouts only created via API call),
* `daily`, `weekly`, or `monthly`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getInterval() {
return this.interval;
}
/**
* The day of the month funds will be paid out. Only shown if `interval` is monthly. Payouts
* scheduled between the 29th and 31st of the month are sent on the last day of shorter months.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getMonthlyAnchor() {
return this.monthlyAnchor;
}
/**
* The day of the week funds will be paid out, of the style 'monday', 'tuesday', etc. Only shown
* if `interval` is weekly.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getWeeklyAnchor() {
return this.weeklyAnchor;
}
/**
* The number of days charges for the account will be held before being paid out.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDelayDays(final Long delayDays) {
this.delayDays = delayDays;
}
/**
* How frequently funds will be paid out. One of `manual` (payouts only created via API call),
* `daily`, `weekly`, or `monthly`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setInterval(final String interval) {
this.interval = interval;
}
/**
* The day of the month funds will be paid out. Only shown if `interval` is monthly. Payouts
* scheduled between the 29th and 31st of the month are sent on the last day of shorter months.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMonthlyAnchor(final Long monthlyAnchor) {
this.monthlyAnchor = monthlyAnchor;
}
/**
* The day of the week funds will be paid out, of the style 'monday', 'tuesday', etc. Only shown
* if `interval` is weekly.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setWeeklyAnchor(final String weeklyAnchor) {
this.weeklyAnchor = weeklyAnchor;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Account.PayoutSchedule)) return false;
final Account.PayoutSchedule other = (Account.PayoutSchedule) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$delayDays = this.getDelayDays();
final java.lang.Object other$delayDays = other.getDelayDays();
if (this$delayDays == null ? other$delayDays != null : !this$delayDays.equals(other$delayDays)) return false;
final java.lang.Object this$interval = this.getInterval();
final java.lang.Object other$interval = other.getInterval();
if (this$interval == null ? other$interval != null : !this$interval.equals(other$interval)) return false;
final java.lang.Object this$monthlyAnchor = this.getMonthlyAnchor();
final java.lang.Object other$monthlyAnchor = other.getMonthlyAnchor();
if (this$monthlyAnchor == null ? other$monthlyAnchor != null : !this$monthlyAnchor.equals(other$monthlyAnchor)) return false;
final java.lang.Object this$weeklyAnchor = this.getWeeklyAnchor();
final java.lang.Object other$weeklyAnchor = other.getWeeklyAnchor();
if (this$weeklyAnchor == null ? other$weeklyAnchor != null : !this$weeklyAnchor.equals(other$weeklyAnchor)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Account.PayoutSchedule;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $delayDays = this.getDelayDays();
result = result * PRIME + ($delayDays == null ? 43 : $delayDays.hashCode());
final java.lang.Object $interval = this.getInterval();
result = result * PRIME + ($interval == null ? 43 : $interval.hashCode());
final java.lang.Object $monthlyAnchor = this.getMonthlyAnchor();
result = result * PRIME + ($monthlyAnchor == null ? 43 : $monthlyAnchor.hashCode());
final java.lang.Object $weeklyAnchor = this.getWeeklyAnchor();
result = result * PRIME + ($weeklyAnchor == null ? 43 : $weeklyAnchor.hashCode());
return result;
}
}
public static class Requirements extends StripeObject {
/**
* The date the fields in `currently_due` must be collected by to keep payouts enabled for the
* account. These fields might block payouts sooner if the next threshold is reached before
* these fields are collected.
*/
@SerializedName("current_deadline")
Long currentDeadline;
/**
* The fields that need to be collected to keep the account enabled. If not collected by the
* `current_deadline`, these fields appear in `past_due` as well, and the account is disabled.
*/
@SerializedName("currently_due")
List currentlyDue;
/**
* If the account is disabled, this string describes why the account can’t create charges or
* receive payouts. Can be `requirements.past_due`, `requirements.pending_verification`,
* `rejected.fraud`, `rejected.terms_of_service`, `rejected.listed`, `rejected.other`, `listed`,
* `under_review`, or `other`.
*/
@SerializedName("disabled_reason")
String disabledReason;
/**
* The fields that need to be collected assuming all volume thresholds are reached. As they
* become required, these fields appear in `currently_due` as well, and the `current_deadline`
* is set.
*/
@SerializedName("eventually_due")
List eventuallyDue;
/**
* The fields that weren't collected by the `current_deadline`. These fields need to be
* collected to re-enable the account.
*/
@SerializedName("past_due")
List pastDue;
/**
* The date the fields in `currently_due` must be collected by to keep payouts enabled for the
* account. These fields might block payouts sooner if the next threshold is reached before
* these fields are collected.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCurrentDeadline() {
return this.currentDeadline;
}
/**
* The fields that need to be collected to keep the account enabled. If not collected by the
* `current_deadline`, these fields appear in `past_due` as well, and the account is disabled.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getCurrentlyDue() {
return this.currentlyDue;
}
/**
* If the account is disabled, this string describes why the account can’t create charges or
* receive payouts. Can be `requirements.past_due`, `requirements.pending_verification`,
* `rejected.fraud`, `rejected.terms_of_service`, `rejected.listed`, `rejected.other`, `listed`,
* `under_review`, or `other`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDisabledReason() {
return this.disabledReason;
}
/**
* The fields that need to be collected assuming all volume thresholds are reached. As they
* become required, these fields appear in `currently_due` as well, and the `current_deadline`
* is set.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getEventuallyDue() {
return this.eventuallyDue;
}
/**
* The fields that weren't collected by the `current_deadline`. These fields need to be
* collected to re-enable the account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getPastDue() {
return this.pastDue;
}
/**
* The date the fields in `currently_due` must be collected by to keep payouts enabled for the
* account. These fields might block payouts sooner if the next threshold is reached before
* these fields are collected.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrentDeadline(final Long currentDeadline) {
this.currentDeadline = currentDeadline;
}
/**
* The fields that need to be collected to keep the account enabled. If not collected by the
* `current_deadline`, these fields appear in `past_due` as well, and the account is disabled.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrentlyDue(final List currentlyDue) {
this.currentlyDue = currentlyDue;
}
/**
* If the account is disabled, this string describes why the account can’t create charges or
* receive payouts. Can be `requirements.past_due`, `requirements.pending_verification`,
* `rejected.fraud`, `rejected.terms_of_service`, `rejected.listed`, `rejected.other`, `listed`,
* `under_review`, or `other`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDisabledReason(final String disabledReason) {
this.disabledReason = disabledReason;
}
/**
* The fields that need to be collected assuming all volume thresholds are reached. As they
* become required, these fields appear in `currently_due` as well, and the `current_deadline`
* is set.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEventuallyDue(final List eventuallyDue) {
this.eventuallyDue = eventuallyDue;
}
/**
* The fields that weren't collected by the `current_deadline`. These fields need to be
* collected to re-enable the account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPastDue(final List pastDue) {
this.pastDue = pastDue;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Account.Requirements)) return false;
final Account.Requirements other = (Account.Requirements) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$currentDeadline = this.getCurrentDeadline();
final java.lang.Object other$currentDeadline = other.getCurrentDeadline();
if (this$currentDeadline == null ? other$currentDeadline != null : !this$currentDeadline.equals(other$currentDeadline)) return false;
final java.lang.Object this$currentlyDue = this.getCurrentlyDue();
final java.lang.Object other$currentlyDue = other.getCurrentlyDue();
if (this$currentlyDue == null ? other$currentlyDue != null : !this$currentlyDue.equals(other$currentlyDue)) return false;
final java.lang.Object this$disabledReason = this.getDisabledReason();
final java.lang.Object other$disabledReason = other.getDisabledReason();
if (this$disabledReason == null ? other$disabledReason != null : !this$disabledReason.equals(other$disabledReason)) return false;
final java.lang.Object this$eventuallyDue = this.getEventuallyDue();
final java.lang.Object other$eventuallyDue = other.getEventuallyDue();
if (this$eventuallyDue == null ? other$eventuallyDue != null : !this$eventuallyDue.equals(other$eventuallyDue)) return false;
final java.lang.Object this$pastDue = this.getPastDue();
final java.lang.Object other$pastDue = other.getPastDue();
if (this$pastDue == null ? other$pastDue != null : !this$pastDue.equals(other$pastDue)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Account.Requirements;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $currentDeadline = this.getCurrentDeadline();
result = result * PRIME + ($currentDeadline == null ? 43 : $currentDeadline.hashCode());
final java.lang.Object $currentlyDue = this.getCurrentlyDue();
result = result * PRIME + ($currentlyDue == null ? 43 : $currentlyDue.hashCode());
final java.lang.Object $disabledReason = this.getDisabledReason();
result = result * PRIME + ($disabledReason == null ? 43 : $disabledReason.hashCode());
final java.lang.Object $eventuallyDue = this.getEventuallyDue();
result = result * PRIME + ($eventuallyDue == null ? 43 : $eventuallyDue.hashCode());
final java.lang.Object $pastDue = this.getPastDue();
result = result * PRIME + ($pastDue == null ? 43 : $pastDue.hashCode());
return result;
}
}
public static class Settings extends StripeObject {
@SerializedName("branding")
SettingsBranding branding;
@SerializedName("card_payments")
SettingsCardPayments cardPayments;
@SerializedName("dashboard")
SettingsDashboard dashboard;
@SerializedName("payments")
SettingsPayments payments;
@SerializedName("payouts")
SettingsPayouts payouts;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SettingsBranding getBranding() {
return this.branding;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SettingsCardPayments getCardPayments() {
return this.cardPayments;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SettingsDashboard getDashboard() {
return this.dashboard;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SettingsPayments getPayments() {
return this.payments;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SettingsPayouts getPayouts() {
return this.payouts;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBranding(final SettingsBranding branding) {
this.branding = branding;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCardPayments(final SettingsCardPayments cardPayments) {
this.cardPayments = cardPayments;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDashboard(final SettingsDashboard dashboard) {
this.dashboard = dashboard;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPayments(final SettingsPayments payments) {
this.payments = payments;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPayouts(final SettingsPayouts payouts) {
this.payouts = payouts;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Account.Settings)) return false;
final Account.Settings other = (Account.Settings) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$branding = this.getBranding();
final java.lang.Object other$branding = other.getBranding();
if (this$branding == null ? other$branding != null : !this$branding.equals(other$branding)) return false;
final java.lang.Object this$cardPayments = this.getCardPayments();
final java.lang.Object other$cardPayments = other.getCardPayments();
if (this$cardPayments == null ? other$cardPayments != null : !this$cardPayments.equals(other$cardPayments)) return false;
final java.lang.Object this$dashboard = this.getDashboard();
final java.lang.Object other$dashboard = other.getDashboard();
if (this$dashboard == null ? other$dashboard != null : !this$dashboard.equals(other$dashboard)) return false;
final java.lang.Object this$payments = this.getPayments();
final java.lang.Object other$payments = other.getPayments();
if (this$payments == null ? other$payments != null : !this$payments.equals(other$payments)) return false;
final java.lang.Object this$payouts = this.getPayouts();
final java.lang.Object other$payouts = other.getPayouts();
if (this$payouts == null ? other$payouts != null : !this$payouts.equals(other$payouts)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Account.Settings;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $branding = this.getBranding();
result = result * PRIME + ($branding == null ? 43 : $branding.hashCode());
final java.lang.Object $cardPayments = this.getCardPayments();
result = result * PRIME + ($cardPayments == null ? 43 : $cardPayments.hashCode());
final java.lang.Object $dashboard = this.getDashboard();
result = result * PRIME + ($dashboard == null ? 43 : $dashboard.hashCode());
final java.lang.Object $payments = this.getPayments();
result = result * PRIME + ($payments == null ? 43 : $payments.hashCode());
final java.lang.Object $payouts = this.getPayouts();
result = result * PRIME + ($payouts == null ? 43 : $payouts.hashCode());
return result;
}
}
public static class SettingsBranding extends StripeObject {
/**
* (ID of a [file upload](https://stripe.com/docs/guides/file-upload)) An icon for the account.
* Must be square and at least 128px x 128px.
*/
@SerializedName("icon")
ExpandableField icon;
/**
* (ID of a [file upload](https://stripe.com/docs/guides/file-upload)) A logo for the account
* that will be used in Checkout instead of the icon and without the account's name next to it
* if provided. Must be at least 128px x 128px.
*/
@SerializedName("logo")
ExpandableField logo;
/**
* A CSS hex color value representing the primary branding color for this account.
*/
@SerializedName("primary_color")
String primaryColor;
/**
* Get id of expandable `icon` object.
*/
public String getIcon() {
return (this.icon != null) ? this.icon.getId() : null;
}
public void setIcon(String id) {
this.icon = ApiResource.setExpandableFieldId(id, this.icon);
}
/**
* Get expanded `icon`.
*/
public File getIconObject() {
return (this.icon != null) ? this.icon.getExpanded() : null;
}
public void setIconObject(File expandableObject) {
this.icon = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get id of expandable `logo` object.
*/
public String getLogo() {
return (this.logo != null) ? this.logo.getId() : null;
}
public void setLogo(String id) {
this.logo = ApiResource.setExpandableFieldId(id, this.logo);
}
/**
* Get expanded `logo`.
*/
public File getLogoObject() {
return (this.logo != null) ? this.logo.getExpanded() : null;
}
public void setLogoObject(File expandableObject) {
this.logo = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* A CSS hex color value representing the primary branding color for this account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPrimaryColor() {
return this.primaryColor;
}
/**
* A CSS hex color value representing the primary branding color for this account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPrimaryColor(final String primaryColor) {
this.primaryColor = primaryColor;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Account.SettingsBranding)) return false;
final Account.SettingsBranding other = (Account.SettingsBranding) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$icon = this.getIcon();
final java.lang.Object other$icon = other.getIcon();
if (this$icon == null ? other$icon != null : !this$icon.equals(other$icon)) return false;
final java.lang.Object this$logo = this.getLogo();
final java.lang.Object other$logo = other.getLogo();
if (this$logo == null ? other$logo != null : !this$logo.equals(other$logo)) return false;
final java.lang.Object this$primaryColor = this.getPrimaryColor();
final java.lang.Object other$primaryColor = other.getPrimaryColor();
if (this$primaryColor == null ? other$primaryColor != null : !this$primaryColor.equals(other$primaryColor)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Account.SettingsBranding;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $icon = this.getIcon();
result = result * PRIME + ($icon == null ? 43 : $icon.hashCode());
final java.lang.Object $logo = this.getLogo();
result = result * PRIME + ($logo == null ? 43 : $logo.hashCode());
final java.lang.Object $primaryColor = this.getPrimaryColor();
result = result * PRIME + ($primaryColor == null ? 43 : $primaryColor.hashCode());
return result;
}
}
public static class SettingsCardPayments extends StripeObject {
@SerializedName("decline_on")
DeclineChargeOn declineOn;
/**
* The default text that appears on credit card statements when a charge is made. This field
* prefixes any dynamic `statement_descriptor` specified on the charge.
* `statement_descriptor_prefix` is useful for maximizing descriptor space for the dynamic
* portion.
*/
@SerializedName("statement_descriptor_prefix")
String statementDescriptorPrefix;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public DeclineChargeOn getDeclineOn() {
return this.declineOn;
}
/**
* The default text that appears on credit card statements when a charge is made. This field
* prefixes any dynamic `statement_descriptor` specified on the charge.
* `statement_descriptor_prefix` is useful for maximizing descriptor space for the dynamic
* portion.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatementDescriptorPrefix() {
return this.statementDescriptorPrefix;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDeclineOn(final DeclineChargeOn declineOn) {
this.declineOn = declineOn;
}
/**
* The default text that appears on credit card statements when a charge is made. This field
* prefixes any dynamic `statement_descriptor` specified on the charge.
* `statement_descriptor_prefix` is useful for maximizing descriptor space for the dynamic
* portion.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatementDescriptorPrefix(final String statementDescriptorPrefix) {
this.statementDescriptorPrefix = statementDescriptorPrefix;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Account.SettingsCardPayments)) return false;
final Account.SettingsCardPayments other = (Account.SettingsCardPayments) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$declineOn = this.getDeclineOn();
final java.lang.Object other$declineOn = other.getDeclineOn();
if (this$declineOn == null ? other$declineOn != null : !this$declineOn.equals(other$declineOn)) return false;
final java.lang.Object this$statementDescriptorPrefix = this.getStatementDescriptorPrefix();
final java.lang.Object other$statementDescriptorPrefix = other.getStatementDescriptorPrefix();
if (this$statementDescriptorPrefix == null ? other$statementDescriptorPrefix != null : !this$statementDescriptorPrefix.equals(other$statementDescriptorPrefix)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Account.SettingsCardPayments;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $declineOn = this.getDeclineOn();
result = result * PRIME + ($declineOn == null ? 43 : $declineOn.hashCode());
final java.lang.Object $statementDescriptorPrefix = this.getStatementDescriptorPrefix();
result = result * PRIME + ($statementDescriptorPrefix == null ? 43 : $statementDescriptorPrefix.hashCode());
return result;
}
}
public static class SettingsDashboard extends StripeObject {
/**
* The display name for this account. This is used on the Stripe Dashboard to differentiate
* between accounts.
*/
@SerializedName("display_name")
String displayName;
/**
* The timezone used in the Stripe Dashboard for this account. A list of possible time zone
* values is maintained at the [IANA Time Zone Database](http://www.iana.org/time-zones).
*/
@SerializedName("timezone")
String timezone;
/**
* The display name for this account. This is used on the Stripe Dashboard to differentiate
* between accounts.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDisplayName() {
return this.displayName;
}
/**
* The timezone used in the Stripe Dashboard for this account. A list of possible time zone
* values is maintained at the [IANA Time Zone Database](http://www.iana.org/time-zones).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTimezone() {
return this.timezone;
}
/**
* The display name for this account. This is used on the Stripe Dashboard to differentiate
* between accounts.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDisplayName(final String displayName) {
this.displayName = displayName;
}
/**
* The timezone used in the Stripe Dashboard for this account. A list of possible time zone
* values is maintained at the [IANA Time Zone Database](http://www.iana.org/time-zones).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTimezone(final String timezone) {
this.timezone = timezone;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Account.SettingsDashboard)) return false;
final Account.SettingsDashboard other = (Account.SettingsDashboard) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$displayName = this.getDisplayName();
final java.lang.Object other$displayName = other.getDisplayName();
if (this$displayName == null ? other$displayName != null : !this$displayName.equals(other$displayName)) return false;
final java.lang.Object this$timezone = this.getTimezone();
final java.lang.Object other$timezone = other.getTimezone();
if (this$timezone == null ? other$timezone != null : !this$timezone.equals(other$timezone)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Account.SettingsDashboard;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $displayName = this.getDisplayName();
result = result * PRIME + ($displayName == null ? 43 : $displayName.hashCode());
final java.lang.Object $timezone = this.getTimezone();
result = result * PRIME + ($timezone == null ? 43 : $timezone.hashCode());
return result;
}
}
public static class SettingsPayments extends StripeObject {
/**
* The default text that appears on credit card statements when a charge is made. This field
* prefixes any dynamic `statement_descriptor` specified on the charge.
*/
@SerializedName("statement_descriptor")
String statementDescriptor;
/**
* The Kana variation of the default text that appears on credit card statements when a charge
* is made (Japan only).
*/
@SerializedName("statement_descriptor_kana")
String statementDescriptorKana;
/**
* The Kanji variation of the default text that appears on credit card statements when a charge
* is made (Japan only).
*/
@SerializedName("statement_descriptor_kanji")
String statementDescriptorKanji;
/**
* The default text that appears on credit card statements when a charge is made. This field
* prefixes any dynamic `statement_descriptor` specified on the charge.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatementDescriptor() {
return this.statementDescriptor;
}
/**
* The Kana variation of the default text that appears on credit card statements when a charge
* is made (Japan only).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatementDescriptorKana() {
return this.statementDescriptorKana;
}
/**
* The Kanji variation of the default text that appears on credit card statements when a charge
* is made (Japan only).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatementDescriptorKanji() {
return this.statementDescriptorKanji;
}
/**
* The default text that appears on credit card statements when a charge is made. This field
* prefixes any dynamic `statement_descriptor` specified on the charge.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatementDescriptor(final String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
}
/**
* The Kana variation of the default text that appears on credit card statements when a charge
* is made (Japan only).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatementDescriptorKana(final String statementDescriptorKana) {
this.statementDescriptorKana = statementDescriptorKana;
}
/**
* The Kanji variation of the default text that appears on credit card statements when a charge
* is made (Japan only).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatementDescriptorKanji(final String statementDescriptorKanji) {
this.statementDescriptorKanji = statementDescriptorKanji;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Account.SettingsPayments)) return false;
final Account.SettingsPayments other = (Account.SettingsPayments) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$statementDescriptor = this.getStatementDescriptor();
final java.lang.Object other$statementDescriptor = other.getStatementDescriptor();
if (this$statementDescriptor == null ? other$statementDescriptor != null : !this$statementDescriptor.equals(other$statementDescriptor)) return false;
final java.lang.Object this$statementDescriptorKana = this.getStatementDescriptorKana();
final java.lang.Object other$statementDescriptorKana = other.getStatementDescriptorKana();
if (this$statementDescriptorKana == null ? other$statementDescriptorKana != null : !this$statementDescriptorKana.equals(other$statementDescriptorKana)) return false;
final java.lang.Object this$statementDescriptorKanji = this.getStatementDescriptorKanji();
final java.lang.Object other$statementDescriptorKanji = other.getStatementDescriptorKanji();
if (this$statementDescriptorKanji == null ? other$statementDescriptorKanji != null : !this$statementDescriptorKanji.equals(other$statementDescriptorKanji)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Account.SettingsPayments;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $statementDescriptor = this.getStatementDescriptor();
result = result * PRIME + ($statementDescriptor == null ? 43 : $statementDescriptor.hashCode());
final java.lang.Object $statementDescriptorKana = this.getStatementDescriptorKana();
result = result * PRIME + ($statementDescriptorKana == null ? 43 : $statementDescriptorKana.hashCode());
final java.lang.Object $statementDescriptorKanji = this.getStatementDescriptorKanji();
result = result * PRIME + ($statementDescriptorKanji == null ? 43 : $statementDescriptorKanji.hashCode());
return result;
}
}
public static class SettingsPayouts extends StripeObject {
/**
* A Boolean indicating if Stripe should try to reclaim negative balances from an attached bank
* account. See our [Understanding Connect Account
* Balances](https://stripe.com/docs/connect/account-balances) documentation for details.
* Default value is `true` for Express accounts and `false` for Custom accounts.
*/
@SerializedName("debit_negative_balances")
Boolean debitNegativeBalances;
@SerializedName("schedule")
PayoutSchedule schedule;
/**
* The text that appears on the bank account statement for payouts. If not set, this defaults to
* the platform's bank descriptor as set in the Dashboard.
*/
@SerializedName("statement_descriptor")
String statementDescriptor;
/**
* A Boolean indicating if Stripe should try to reclaim negative balances from an attached bank
* account. See our [Understanding Connect Account
* Balances](https://stripe.com/docs/connect/account-balances) documentation for details.
* Default value is `true` for Express accounts and `false` for Custom accounts.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getDebitNegativeBalances() {
return this.debitNegativeBalances;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PayoutSchedule getSchedule() {
return this.schedule;
}
/**
* The text that appears on the bank account statement for payouts. If not set, this defaults to
* the platform's bank descriptor as set in the Dashboard.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatementDescriptor() {
return this.statementDescriptor;
}
/**
* A Boolean indicating if Stripe should try to reclaim negative balances from an attached bank
* account. See our [Understanding Connect Account
* Balances](https://stripe.com/docs/connect/account-balances) documentation for details.
* Default value is `true` for Express accounts and `false` for Custom accounts.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDebitNegativeBalances(final Boolean debitNegativeBalances) {
this.debitNegativeBalances = debitNegativeBalances;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSchedule(final PayoutSchedule schedule) {
this.schedule = schedule;
}
/**
* The text that appears on the bank account statement for payouts. If not set, this defaults to
* the platform's bank descriptor as set in the Dashboard.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatementDescriptor(final String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Account.SettingsPayouts)) return false;
final Account.SettingsPayouts other = (Account.SettingsPayouts) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$debitNegativeBalances = this.getDebitNegativeBalances();
final java.lang.Object other$debitNegativeBalances = other.getDebitNegativeBalances();
if (this$debitNegativeBalances == null ? other$debitNegativeBalances != null : !this$debitNegativeBalances.equals(other$debitNegativeBalances)) return false;
final java.lang.Object this$schedule = this.getSchedule();
final java.lang.Object other$schedule = other.getSchedule();
if (this$schedule == null ? other$schedule != null : !this$schedule.equals(other$schedule)) return false;
final java.lang.Object this$statementDescriptor = this.getStatementDescriptor();
final java.lang.Object other$statementDescriptor = other.getStatementDescriptor();
if (this$statementDescriptor == null ? other$statementDescriptor != null : !this$statementDescriptor.equals(other$statementDescriptor)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Account.SettingsPayouts;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $debitNegativeBalances = this.getDebitNegativeBalances();
result = result * PRIME + ($debitNegativeBalances == null ? 43 : $debitNegativeBalances.hashCode());
final java.lang.Object $schedule = this.getSchedule();
result = result * PRIME + ($schedule == null ? 43 : $schedule.hashCode());
final java.lang.Object $statementDescriptor = this.getStatementDescriptor();
result = result * PRIME + ($statementDescriptor == null ? 43 : $statementDescriptor.hashCode());
return result;
}
}
public static class TosAcceptance extends StripeObject {
/**
* The Unix timestamp marking when the Stripe Services Agreement was accepted by the account
* representative.
*/
@SerializedName("date")
Long date;
/**
* The IP address from which the Stripe Services Agreement was accepted by the account
* representative.
*/
@SerializedName("ip")
String ip;
/**
* The user agent of the browser from which the Stripe Services Agreement was accepted by the
* account representative.
*/
@SerializedName("user_agent")
String userAgent;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDate() {
return this.date;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getIp() {
return this.ip;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUserAgent() {
return this.userAgent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDate(final Long date) {
this.date = date;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIp(final String ip) {
this.ip = ip;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUserAgent(final String userAgent) {
this.userAgent = userAgent;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Account.TosAcceptance)) return false;
final Account.TosAcceptance other = (Account.TosAcceptance) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$date = this.getDate();
final java.lang.Object other$date = other.getDate();
if (this$date == null ? other$date != null : !this$date.equals(other$date)) return false;
final java.lang.Object this$ip = this.getIp();
final java.lang.Object other$ip = other.getIp();
if (this$ip == null ? other$ip != null : !this$ip.equals(other$ip)) return false;
final java.lang.Object this$userAgent = this.getUserAgent();
final java.lang.Object other$userAgent = other.getUserAgent();
if (this$userAgent == null ? other$userAgent != null : !this$userAgent.equals(other$userAgent)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Account.TosAcceptance;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $date = this.getDate();
result = result * PRIME + ($date == null ? 43 : $date.hashCode());
final java.lang.Object $ip = this.getIp();
result = result * PRIME + ($ip == null ? 43 : $ip.hashCode());
final java.lang.Object $userAgent = this.getUserAgent();
result = result * PRIME + ($userAgent == null ? 43 : $userAgent.hashCode());
return result;
}
}
/**
* Optional information related to the business.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BusinessProfile getBusinessProfile() {
return this.businessProfile;
}
/**
* The business type. Can be `individual` or `company`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBusinessType() {
return this.businessType;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Capabilities getCapabilities() {
return this.capabilities;
}
/**
* Whether the account can create live charges.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getChargesEnabled() {
return this.chargesEnabled;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Company getCompany() {
return this.company;
}
/**
* The account's country.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCountry() {
return this.country;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
/**
* Three-letter ISO currency code representing the default currency for the account. This must be
* a currency that [Stripe supports in the account's country](https://stripe.com/docs/payouts).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDefaultCurrency() {
return this.defaultCurrency;
}
/**
* Always true for a deleted object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getDeleted() {
return this.deleted;
}
/**
* Whether account details have been submitted. Standard accounts cannot receive payouts before
* this is true.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getDetailsSubmitted() {
return this.detailsSubmitted;
}
/**
* The primary user's email address.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getEmail() {
return this.email;
}
/**
* External accounts (bank accounts and debit cards) currently attached to this account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ExternalAccountCollection getExternalAccounts() {
return this.externalAccounts;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Person getIndividual() {
return this.individual;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* Whether Stripe can send payouts to this account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getPayoutsEnabled() {
return this.payoutsEnabled;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Requirements getRequirements() {
return this.requirements;
}
/**
* Account options for customizing how the account functions within Stripe.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Settings getSettings() {
return this.settings;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TosAcceptance getTosAcceptance() {
return this.tosAcceptance;
}
/**
* The Stripe account type. Can be `standard`, `express`, or `custom`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
/**
* Optional information related to the business.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBusinessProfile(final BusinessProfile businessProfile) {
this.businessProfile = businessProfile;
}
/**
* The business type. Can be `individual` or `company`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBusinessType(final String businessType) {
this.businessType = businessType;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCapabilities(final Capabilities capabilities) {
this.capabilities = capabilities;
}
/**
* Whether the account can create live charges.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setChargesEnabled(final Boolean chargesEnabled) {
this.chargesEnabled = chargesEnabled;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCompany(final Company company) {
this.company = company;
}
/**
* The account's country.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCountry(final String country) {
this.country = country;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* Three-letter ISO currency code representing the default currency for the account. This must be
* a currency that [Stripe supports in the account's country](https://stripe.com/docs/payouts).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDefaultCurrency(final String defaultCurrency) {
this.defaultCurrency = defaultCurrency;
}
/**
* Always true for a deleted object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDeleted(final Boolean deleted) {
this.deleted = deleted;
}
/**
* Whether account details have been submitted. Standard accounts cannot receive payouts before
* this is true.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDetailsSubmitted(final Boolean detailsSubmitted) {
this.detailsSubmitted = detailsSubmitted;
}
/**
* The primary user's email address.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEmail(final String email) {
this.email = email;
}
/**
* External accounts (bank accounts and debit cards) currently attached to this account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setExternalAccounts(final ExternalAccountCollection externalAccounts) {
this.externalAccounts = externalAccounts;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIndividual(final Person individual) {
this.individual = individual;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* Whether Stripe can send payouts to this account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPayoutsEnabled(final Boolean payoutsEnabled) {
this.payoutsEnabled = payoutsEnabled;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRequirements(final Requirements requirements) {
this.requirements = requirements;
}
/**
* Account options for customizing how the account functions within Stripe.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSettings(final Settings settings) {
this.settings = settings;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTosAcceptance(final TosAcceptance tosAcceptance) {
this.tosAcceptance = tosAcceptance;
}
/**
* The Stripe account type. Can be `standard`, `express`, or `custom`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Account)) return false;
final Account other = (Account) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$businessProfile = this.getBusinessProfile();
final java.lang.Object other$businessProfile = other.getBusinessProfile();
if (this$businessProfile == null ? other$businessProfile != null : !this$businessProfile.equals(other$businessProfile)) return false;
final java.lang.Object this$businessType = this.getBusinessType();
final java.lang.Object other$businessType = other.getBusinessType();
if (this$businessType == null ? other$businessType != null : !this$businessType.equals(other$businessType)) return false;
final java.lang.Object this$capabilities = this.getCapabilities();
final java.lang.Object other$capabilities = other.getCapabilities();
if (this$capabilities == null ? other$capabilities != null : !this$capabilities.equals(other$capabilities)) return false;
final java.lang.Object this$chargesEnabled = this.getChargesEnabled();
final java.lang.Object other$chargesEnabled = other.getChargesEnabled();
if (this$chargesEnabled == null ? other$chargesEnabled != null : !this$chargesEnabled.equals(other$chargesEnabled)) return false;
final java.lang.Object this$company = this.getCompany();
final java.lang.Object other$company = other.getCompany();
if (this$company == null ? other$company != null : !this$company.equals(other$company)) return false;
final java.lang.Object this$country = this.getCountry();
final java.lang.Object other$country = other.getCountry();
if (this$country == null ? other$country != null : !this$country.equals(other$country)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$defaultCurrency = this.getDefaultCurrency();
final java.lang.Object other$defaultCurrency = other.getDefaultCurrency();
if (this$defaultCurrency == null ? other$defaultCurrency != null : !this$defaultCurrency.equals(other$defaultCurrency)) return false;
final java.lang.Object this$deleted = this.getDeleted();
final java.lang.Object other$deleted = other.getDeleted();
if (this$deleted == null ? other$deleted != null : !this$deleted.equals(other$deleted)) return false;
final java.lang.Object this$detailsSubmitted = this.getDetailsSubmitted();
final java.lang.Object other$detailsSubmitted = other.getDetailsSubmitted();
if (this$detailsSubmitted == null ? other$detailsSubmitted != null : !this$detailsSubmitted.equals(other$detailsSubmitted)) return false;
final java.lang.Object this$email = this.getEmail();
final java.lang.Object other$email = other.getEmail();
if (this$email == null ? other$email != null : !this$email.equals(other$email)) return false;
final java.lang.Object this$externalAccounts = this.getExternalAccounts();
final java.lang.Object other$externalAccounts = other.getExternalAccounts();
if (this$externalAccounts == null ? other$externalAccounts != null : !this$externalAccounts.equals(other$externalAccounts)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$individual = this.getIndividual();
final java.lang.Object other$individual = other.getIndividual();
if (this$individual == null ? other$individual != null : !this$individual.equals(other$individual)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$payoutsEnabled = this.getPayoutsEnabled();
final java.lang.Object other$payoutsEnabled = other.getPayoutsEnabled();
if (this$payoutsEnabled == null ? other$payoutsEnabled != null : !this$payoutsEnabled.equals(other$payoutsEnabled)) return false;
final java.lang.Object this$requirements = this.getRequirements();
final java.lang.Object other$requirements = other.getRequirements();
if (this$requirements == null ? other$requirements != null : !this$requirements.equals(other$requirements)) return false;
final java.lang.Object this$settings = this.getSettings();
final java.lang.Object other$settings = other.getSettings();
if (this$settings == null ? other$settings != null : !this$settings.equals(other$settings)) return false;
final java.lang.Object this$tosAcceptance = this.getTosAcceptance();
final java.lang.Object other$tosAcceptance = other.getTosAcceptance();
if (this$tosAcceptance == null ? other$tosAcceptance != null : !this$tosAcceptance.equals(other$tosAcceptance)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Account;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $businessProfile = this.getBusinessProfile();
result = result * PRIME + ($businessProfile == null ? 43 : $businessProfile.hashCode());
final java.lang.Object $businessType = this.getBusinessType();
result = result * PRIME + ($businessType == null ? 43 : $businessType.hashCode());
final java.lang.Object $capabilities = this.getCapabilities();
result = result * PRIME + ($capabilities == null ? 43 : $capabilities.hashCode());
final java.lang.Object $chargesEnabled = this.getChargesEnabled();
result = result * PRIME + ($chargesEnabled == null ? 43 : $chargesEnabled.hashCode());
final java.lang.Object $company = this.getCompany();
result = result * PRIME + ($company == null ? 43 : $company.hashCode());
final java.lang.Object $country = this.getCountry();
result = result * PRIME + ($country == null ? 43 : $country.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $defaultCurrency = this.getDefaultCurrency();
result = result * PRIME + ($defaultCurrency == null ? 43 : $defaultCurrency.hashCode());
final java.lang.Object $deleted = this.getDeleted();
result = result * PRIME + ($deleted == null ? 43 : $deleted.hashCode());
final java.lang.Object $detailsSubmitted = this.getDetailsSubmitted();
result = result * PRIME + ($detailsSubmitted == null ? 43 : $detailsSubmitted.hashCode());
final java.lang.Object $email = this.getEmail();
result = result * PRIME + ($email == null ? 43 : $email.hashCode());
final java.lang.Object $externalAccounts = this.getExternalAccounts();
result = result * PRIME + ($externalAccounts == null ? 43 : $externalAccounts.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $individual = this.getIndividual();
result = result * PRIME + ($individual == null ? 43 : $individual.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $payoutsEnabled = this.getPayoutsEnabled();
result = result * PRIME + ($payoutsEnabled == null ? 43 : $payoutsEnabled.hashCode());
final java.lang.Object $requirements = this.getRequirements();
result = result * PRIME + ($requirements == null ? 43 : $requirements.hashCode());
final java.lang.Object $settings = this.getSettings();
result = result * PRIME + ($settings == null ? 43 : $settings.hashCode());
final java.lang.Object $tosAcceptance = this.getTosAcceptance();
result = result * PRIME + ($tosAcceptance == null ? 43 : $tosAcceptance.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
}