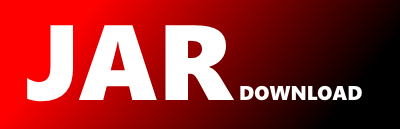
com.stripe.model.Transfer Maven / Gradle / Ivy
// Generated by delombok at Tue Jun 18 17:32:52 PDT 2019
// Generated by com.stripe.generator.entity.SdkBuilder
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.TransferCreateParams;
import com.stripe.param.TransferListParams;
import com.stripe.param.TransferRetrieveParams;
import com.stripe.param.TransferUpdateParams;
import java.util.Map;
public class Transfer extends ApiResource implements BalanceTransactionSource, MetadataStore {
/**
* Amount in %s to be transferred.
*/
@SerializedName("amount")
Long amount;
/**
* Amount in %s reversed (can be less than the amount attribute on the transfer if a partial
* reversal was issued).
*/
@SerializedName("amount_reversed")
Long amountReversed;
/**
* Balance transaction that describes the impact of this transfer on your account balance.
*/
@SerializedName("balance_transaction")
ExpandableField balanceTransaction;
/**
* Time that this record of the transfer was first created.
*/
@SerializedName("created")
Long created;
/**
* Three-letter [ISO currency code](https://www.iso.org/iso-4217-currency-codes.html), in
* lowercase. Must be a [supported currency](https://stripe.com/docs/currencies).
*/
@SerializedName("currency")
String currency;
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
*/
@SerializedName("description")
String description;
/**
* ID of the Stripe account the transfer was sent to.
*/
@SerializedName("destination")
ExpandableField destination;
/**
* If the destination is a Stripe account, this will be the ID of the payment that the destination
* account received for the transfer.
*/
@SerializedName("destination_payment")
ExpandableField destinationPayment;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* Has the value `true` if the object exists in live mode or the value `false` if the object
* exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* A set of key-value pairs that you can attach to a transfer object. It can be useful for storing
* additional information about the transfer in a structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* String representing the object's type. Objects of the same type share the same value.
*/
@SerializedName("object")
String object;
/**
* A list of reversals that have been applied to the transfer.
*/
@SerializedName("reversals")
TransferReversalCollection reversals;
/**
* Whether the transfer has been fully reversed. If the transfer is only partially reversed, this
* attribute will still be false.
*/
@SerializedName("reversed")
Boolean reversed;
/**
* ID of the charge or payment that was used to fund the transfer. If null, the transfer was
* funded from the available balance.
*/
@SerializedName("source_transaction")
ExpandableField sourceTransaction;
/**
* The source balance this transfer came from. One of `card` or `bank_account`.
*/
@SerializedName("source_type")
String sourceType;
/**
* A string that identifies this transaction as part of a group. See the [Connect
* documentation](https://stripe.com/docs/connect/charges-transfers#grouping-transactions) for
* details.
*/
@SerializedName("transfer_group")
String transferGroup;
/**
* Get id of expandable `balanceTransaction` object.
*/
public String getBalanceTransaction() {
return (this.balanceTransaction != null) ? this.balanceTransaction.getId() : null;
}
public void setBalanceTransaction(String id) {
this.balanceTransaction = ApiResource.setExpandableFieldId(id, this.balanceTransaction);
}
/**
* Get expanded `balanceTransaction`.
*/
public BalanceTransaction getBalanceTransactionObject() {
return (this.balanceTransaction != null) ? this.balanceTransaction.getExpanded() : null;
}
public void setBalanceTransactionObject(BalanceTransaction expandableObject) {
this.balanceTransaction = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get id of expandable `destination` object.
*/
public String getDestination() {
return (this.destination != null) ? this.destination.getId() : null;
}
public void setDestination(String id) {
this.destination = ApiResource.setExpandableFieldId(id, this.destination);
}
/**
* Get expanded `destination`.
*/
public Account getDestinationObject() {
return (this.destination != null) ? this.destination.getExpanded() : null;
}
public void setDestinationObject(Account expandableObject) {
this.destination = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get id of expandable `destinationPayment` object.
*/
public String getDestinationPayment() {
return (this.destinationPayment != null) ? this.destinationPayment.getId() : null;
}
public void setDestinationPayment(String id) {
this.destinationPayment = ApiResource.setExpandableFieldId(id, this.destinationPayment);
}
/**
* Get expanded `destinationPayment`.
*/
public Charge getDestinationPaymentObject() {
return (this.destinationPayment != null) ? this.destinationPayment.getExpanded() : null;
}
public void setDestinationPaymentObject(Charge expandableObject) {
this.destinationPayment = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get id of expandable `sourceTransaction` object.
*/
public String getSourceTransaction() {
return (this.sourceTransaction != null) ? this.sourceTransaction.getId() : null;
}
public void setSourceTransaction(String id) {
this.sourceTransaction = ApiResource.setExpandableFieldId(id, this.sourceTransaction);
}
/**
* Get expanded `sourceTransaction`.
*/
public Charge getSourceTransactionObject() {
return (this.sourceTransaction != null) ? this.sourceTransaction.getExpanded() : null;
}
public void setSourceTransactionObject(Charge expandableObject) {
this.sourceTransaction = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* To send funds from your Stripe account to a connected account, you create a new transfer
* object. Your Stripe balance must be able to cover the transfer amount,
* or you’ll receive an “Insufficient Funds” error.
*/
public static Transfer create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* To send funds from your Stripe account to a connected account, you create a new transfer
* object. Your Stripe balance must be able to cover the transfer amount,
* or you’ll receive an “Insufficient Funds” error.
*/
public static Transfer create(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/transfers");
return request(ApiResource.RequestMethod.POST, url, params, Transfer.class, options);
}
/**
* To send funds from your Stripe account to a connected account, you create a new transfer
* object. Your Stripe balance must be able to cover the transfer amount,
* or you’ll receive an “Insufficient Funds” error.
*/
public static Transfer create(TransferCreateParams params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* To send funds from your Stripe account to a connected account, you create a new transfer
* object. Your Stripe balance must be able to cover the transfer amount,
* or you’ll receive an “Insufficient Funds” error.
*/
public static Transfer create(TransferCreateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/transfers");
return request(ApiResource.RequestMethod.POST, url, params, Transfer.class, options);
}
/**
* Returns a list of existing transfers sent to connected accounts. The transfers are returned in
* sorted order, with the most recently created transfers appearing first.
*/
public static TransferCollection list(Map params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of existing transfers sent to connected accounts. The transfers are returned in
* sorted order, with the most recently created transfers appearing first.
*/
public static TransferCollection list(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/transfers");
return requestCollection(url, params, TransferCollection.class, options);
}
/**
* Returns a list of existing transfers sent to connected accounts. The transfers are returned in
* sorted order, with the most recently created transfers appearing first.
*/
public static TransferCollection list(TransferListParams params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of existing transfers sent to connected accounts. The transfers are returned in
* sorted order, with the most recently created transfers appearing first.
*/
public static TransferCollection list(TransferListParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/transfers");
return requestCollection(url, params, TransferCollection.class, options);
}
/**
* Retrieves the details of an existing transfer. Supply the unique transfer ID from either a
* transfer creation request or the transfer list, and Stripe will return the corresponding
* transfer information.
*/
public static Transfer retrieve(String transfer) throws StripeException {
return retrieve(transfer, (Map) null, (RequestOptions) null);
}
/**
* Retrieves the details of an existing transfer. Supply the unique transfer ID from either a
* transfer creation request or the transfer list, and Stripe will return the corresponding
* transfer information.
*/
public static Transfer retrieve(String transfer, RequestOptions options) throws StripeException {
return retrieve(transfer, (Map) null, options);
}
/**
* Retrieves the details of an existing transfer. Supply the unique transfer ID from either a
* transfer creation request or the transfer list, and Stripe will return the corresponding
* transfer information.
*/
public static Transfer retrieve(String transfer, Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/transfers/%s", ApiResource.urlEncodeId(transfer)));
return request(ApiResource.RequestMethod.GET, url, params, Transfer.class, options);
}
/**
* Retrieves the details of an existing transfer. Supply the unique transfer ID from either a
* transfer creation request or the transfer list, and Stripe will return the corresponding
* transfer information.
*/
public static Transfer retrieve(String transfer, TransferRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/transfers/%s", ApiResource.urlEncodeId(transfer)));
return request(ApiResource.RequestMethod.GET, url, params, Transfer.class, options);
}
/**
* Updates the specified transfer by setting the values of the parameters passed. Any parameters
* not provided will be left unchanged.
*
* This request accepts only metadata as an argument.
*/
@Override
public Transfer update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates the specified transfer by setting the values of the parameters passed. Any parameters
* not provided will be left unchanged.
*
* This request accepts only metadata as an argument.
*/
@Override
public Transfer update(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/transfers/%s", ApiResource.urlEncodeId(this.getId())));
return request(ApiResource.RequestMethod.POST, url, params, Transfer.class, options);
}
/**
* Updates the specified transfer by setting the values of the parameters passed. Any parameters
* not provided will be left unchanged.
*
* This request accepts only metadata as an argument.
*/
public Transfer update(TransferUpdateParams params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates the specified transfer by setting the values of the parameters passed. Any parameters
* not provided will be left unchanged.
*
*
This request accepts only metadata as an argument.
*/
public Transfer update(TransferUpdateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/transfers/%s", ApiResource.urlEncodeId(this.getId())));
return request(ApiResource.RequestMethod.POST, url, params, Transfer.class, options);
}
/**
* Amount in %s to be transferred.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
/**
* Amount in %s reversed (can be less than the amount attribute on the transfer if a partial
* reversal was issued).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountReversed() {
return this.amountReversed;
}
/**
* Time that this record of the transfer was first created.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
/**
* Three-letter [ISO currency code](https://www.iso.org/iso-4217-currency-codes.html), in
* lowercase. Must be a [supported currency](https://stripe.com/docs/currencies).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDescription() {
return this.description;
}
/**
* Has the value `true` if the object exists in live mode or the value `false` if the object
* exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* A list of reversals that have been applied to the transfer.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TransferReversalCollection getReversals() {
return this.reversals;
}
/**
* Whether the transfer has been fully reversed. If the transfer is only partially reversed, this
* attribute will still be false.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getReversed() {
return this.reversed;
}
/**
* The source balance this transfer came from. One of `card` or `bank_account`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSourceType() {
return this.sourceType;
}
/**
* A string that identifies this transaction as part of a group. See the [Connect
* documentation](https://stripe.com/docs/connect/charges-transfers#grouping-transactions) for
* details.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTransferGroup() {
return this.transferGroup;
}
/**
* Amount in %s to be transferred.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmount(final Long amount) {
this.amount = amount;
}
/**
* Amount in %s reversed (can be less than the amount attribute on the transfer if a partial
* reversal was issued).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountReversed(final Long amountReversed) {
this.amountReversed = amountReversed;
}
/**
* Time that this record of the transfer was first created.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* Three-letter [ISO currency code](https://www.iso.org/iso-4217-currency-codes.html), in
* lowercase. Must be a [supported currency](https://stripe.com/docs/currencies).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDescription(final String description) {
this.description = description;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* Has the value `true` if the object exists in live mode or the value `false` if the object
* exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* A set of key-value pairs that you can attach to a transfer object. It can be useful for storing
* additional information about the transfer in a structured format.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* A list of reversals that have been applied to the transfer.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReversals(final TransferReversalCollection reversals) {
this.reversals = reversals;
}
/**
* Whether the transfer has been fully reversed. If the transfer is only partially reversed, this
* attribute will still be false.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReversed(final Boolean reversed) {
this.reversed = reversed;
}
/**
* The source balance this transfer came from. One of `card` or `bank_account`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSourceType(final String sourceType) {
this.sourceType = sourceType;
}
/**
* A string that identifies this transaction as part of a group. See the [Connect
* documentation](https://stripe.com/docs/connect/charges-transfers#grouping-transactions) for
* details.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTransferGroup(final String transferGroup) {
this.transferGroup = transferGroup;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Transfer)) return false;
final Transfer other = (Transfer) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$amountReversed = this.getAmountReversed();
final java.lang.Object other$amountReversed = other.getAmountReversed();
if (this$amountReversed == null ? other$amountReversed != null : !this$amountReversed.equals(other$amountReversed)) return false;
final java.lang.Object this$balanceTransaction = this.getBalanceTransaction();
final java.lang.Object other$balanceTransaction = other.getBalanceTransaction();
if (this$balanceTransaction == null ? other$balanceTransaction != null : !this$balanceTransaction.equals(other$balanceTransaction)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$destination = this.getDestination();
final java.lang.Object other$destination = other.getDestination();
if (this$destination == null ? other$destination != null : !this$destination.equals(other$destination)) return false;
final java.lang.Object this$destinationPayment = this.getDestinationPayment();
final java.lang.Object other$destinationPayment = other.getDestinationPayment();
if (this$destinationPayment == null ? other$destinationPayment != null : !this$destinationPayment.equals(other$destinationPayment)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$reversals = this.getReversals();
final java.lang.Object other$reversals = other.getReversals();
if (this$reversals == null ? other$reversals != null : !this$reversals.equals(other$reversals)) return false;
final java.lang.Object this$reversed = this.getReversed();
final java.lang.Object other$reversed = other.getReversed();
if (this$reversed == null ? other$reversed != null : !this$reversed.equals(other$reversed)) return false;
final java.lang.Object this$sourceTransaction = this.getSourceTransaction();
final java.lang.Object other$sourceTransaction = other.getSourceTransaction();
if (this$sourceTransaction == null ? other$sourceTransaction != null : !this$sourceTransaction.equals(other$sourceTransaction)) return false;
final java.lang.Object this$sourceType = this.getSourceType();
final java.lang.Object other$sourceType = other.getSourceType();
if (this$sourceType == null ? other$sourceType != null : !this$sourceType.equals(other$sourceType)) return false;
final java.lang.Object this$transferGroup = this.getTransferGroup();
final java.lang.Object other$transferGroup = other.getTransferGroup();
if (this$transferGroup == null ? other$transferGroup != null : !this$transferGroup.equals(other$transferGroup)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Transfer;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $amountReversed = this.getAmountReversed();
result = result * PRIME + ($amountReversed == null ? 43 : $amountReversed.hashCode());
final java.lang.Object $balanceTransaction = this.getBalanceTransaction();
result = result * PRIME + ($balanceTransaction == null ? 43 : $balanceTransaction.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $destination = this.getDestination();
result = result * PRIME + ($destination == null ? 43 : $destination.hashCode());
final java.lang.Object $destinationPayment = this.getDestinationPayment();
result = result * PRIME + ($destinationPayment == null ? 43 : $destinationPayment.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $reversals = this.getReversals();
result = result * PRIME + ($reversals == null ? 43 : $reversals.hashCode());
final java.lang.Object $reversed = this.getReversed();
result = result * PRIME + ($reversed == null ? 43 : $reversed.hashCode());
final java.lang.Object $sourceTransaction = this.getSourceTransaction();
result = result * PRIME + ($sourceTransaction == null ? 43 : $sourceTransaction.hashCode());
final java.lang.Object $sourceType = this.getSourceType();
result = result * PRIME + ($sourceType == null ? 43 : $sourceType.hashCode());
final java.lang.Object $transferGroup = this.getTransferGroup();
result = result * PRIME + ($transferGroup == null ? 43 : $transferGroup.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
/**
* A set of key-value pairs that you can attach to a transfer object. It can be useful for storing
* additional information about the transfer in a structured format.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
}