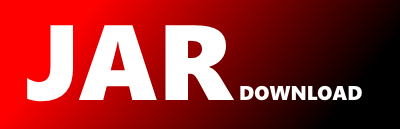
com.stripe.param.checkout.SessionCreateParams Maven / Gradle / Ivy
// Generated by delombok at Tue Jun 18 17:32:51 PDT 2019
// Generated by com.stripe.generator.entity.SdkBuilder
package com.stripe.param.checkout;
import com.google.gson.annotations.SerializedName;
import com.stripe.net.ApiRequestParams;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class SessionCreateParams extends ApiRequestParams {
/**
* Specify whether Checkout should collect the customer's billing address. If set to `required`,
* Checkout will always collect the customer's billing address. If left blank or set to `auto`
* Checkout will only collect the billing address when necessary.
*/
@SerializedName("billing_address_collection")
BillingAddressCollection billingAddressCollection;
/**
* The URL the customer will be directed to if they decide to cancel payment and return to your
* website.
*/
@SerializedName("cancel_url")
String cancelUrl;
/**
* A unique string to reference the Checkout Session. This can be a customer ID, a cart ID, or
* similar, and can be used to reconcile the session with your internal systems.
*/
@SerializedName("client_reference_id")
String clientReferenceId;
/**
* ID of an existing customer paying for this session, if one exists. May only be used with
* line_items. Usage with subscription_data is not yet available. If blank, Checkout will create a
* new customer object based on information provided during the session. The email stored on the
* customer will be used to prefill the email field on the Checkout page. If the customer changes
* their email on the Checkout page, the Customer object will be updated with the new email.
*/
@SerializedName("customer")
String customer;
/**
* If provided, this value will be used when the Customer object is created. If not provided,
* customers will be asked to enter their email address. Use this parameter to prefill customer
* data if you already have an email on file. To access information about the customer once a
* session is complete, use the `customer` field.
*/
@SerializedName("customer_email")
String customerEmail;
/**
* Specifies which fields in the response should be expanded.
*/
@SerializedName("expand")
List expand;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* A list of items the customer is purchasing. Use this parameter for one-time payments. To create
* subscriptions, use `subscription_data.items`.
*/
@SerializedName("line_items")
List lineItems;
/**
* The IETF language tag of the locale Checkout is displayed in. If blank or `auto`, the browser's
* locale is used. Supported values are `auto`, `da`, `de`, `en`, `es`, `fi`, `fr`, `it`, `ja`,
* `nb`, `nl`, `pl`, `pt`, `sv`, or `zh`.
*/
@SerializedName("locale")
Locale locale;
/**
* A subset of parameters to be passed to PaymentIntent creation.
*/
@SerializedName("payment_intent_data")
PaymentIntentData paymentIntentData;
/**
* A list of the types of payment methods (e.g. card) this Checkout Session is allowed to accept.
* The only supported value today is `card`.
*/
@SerializedName("payment_method_types")
List paymentMethodTypes;
/**
* Describes the type of transaction being performed by Checkout in order to customize relevant
* text on the page, such as the submit button. `submit_type` can only be specified on Checkout
* Sessions using line items or a SKU, but not Checkout Sessions for subscriptions. Supported
* values are `auto`, `book`, `donate`, or `pay`.
*/
@SerializedName("submit_type")
SubmitType submitType;
/**
* A subset of parameters to be passed to subscription creation.
*/
@SerializedName("subscription_data")
SubscriptionData subscriptionData;
/**
* The URL to which Stripe should send customers when payment is complete. If you’d like access to
* the Checkout Session for the successful payment, read more about it in our guide on [fulfilling
* your payments with webhooks](/docs/payments/checkout/fulfillment#webhooks).
*/
@SerializedName("success_url")
String successUrl;
private SessionCreateParams(BillingAddressCollection billingAddressCollection, String cancelUrl, String clientReferenceId, String customer, String customerEmail, List expand, Map extraParams, List lineItems, Locale locale, PaymentIntentData paymentIntentData, List paymentMethodTypes, SubmitType submitType, SubscriptionData subscriptionData, String successUrl) {
this.billingAddressCollection = billingAddressCollection;
this.cancelUrl = cancelUrl;
this.clientReferenceId = clientReferenceId;
this.customer = customer;
this.customerEmail = customerEmail;
this.expand = expand;
this.extraParams = extraParams;
this.lineItems = lineItems;
this.locale = locale;
this.paymentIntentData = paymentIntentData;
this.paymentMethodTypes = paymentMethodTypes;
this.submitType = submitType;
this.subscriptionData = subscriptionData;
this.successUrl = successUrl;
}
public static Builder builder() {
return new com.stripe.param.checkout.SessionCreateParams.Builder();
}
public static class Builder {
private BillingAddressCollection billingAddressCollection;
private String cancelUrl;
private String clientReferenceId;
private String customer;
private String customerEmail;
private List expand;
private Map extraParams;
private List lineItems;
private Locale locale;
private PaymentIntentData paymentIntentData;
private List paymentMethodTypes;
private SubmitType submitType;
private SubscriptionData subscriptionData;
private String successUrl;
/**
* Finalize and obtain parameter instance from this builder.
*/
public SessionCreateParams build() {
return new SessionCreateParams(this.billingAddressCollection, this.cancelUrl, this.clientReferenceId, this.customer, this.customerEmail, this.expand, this.extraParams, this.lineItems, this.locale, this.paymentIntentData, this.paymentMethodTypes, this.submitType, this.subscriptionData, this.successUrl);
}
/**
* Specify whether Checkout should collect the customer's billing address. If set to `required`,
* Checkout will always collect the customer's billing address. If left blank or set to `auto`
* Checkout will only collect the billing address when necessary.
*/
public Builder setBillingAddressCollection(BillingAddressCollection billingAddressCollection) {
this.billingAddressCollection = billingAddressCollection;
return this;
}
/**
* The URL the customer will be directed to if they decide to cancel payment and return to your
* website.
*/
public Builder setCancelUrl(String cancelUrl) {
this.cancelUrl = cancelUrl;
return this;
}
/**
* A unique string to reference the Checkout Session. This can be a customer ID, a cart ID, or
* similar, and can be used to reconcile the session with your internal systems.
*/
public Builder setClientReferenceId(String clientReferenceId) {
this.clientReferenceId = clientReferenceId;
return this;
}
/**
* ID of an existing customer paying for this session, if one exists. May only be used with
* line_items. Usage with subscription_data is not yet available. If blank, Checkout will create
* a new customer object based on information provided during the session. The email stored on
* the customer will be used to prefill the email field on the Checkout page. If the customer
* changes their email on the Checkout page, the Customer object will be updated with the new
* email.
*/
public Builder setCustomer(String customer) {
this.customer = customer;
return this;
}
/**
* If provided, this value will be used when the Customer object is created. If not provided,
* customers will be asked to enter their email address. Use this parameter to prefill customer
* data if you already have an email on file. To access information about the customer once a
* session is complete, use the `customer` field.
*/
public Builder setCustomerEmail(String customerEmail) {
this.customerEmail = customerEmail;
return this;
}
/**
* Add an element to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SessionCreateParams#expand} for the field documentation.
*/
public Builder addExpand(String element) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.add(element);
return this;
}
/**
* Add all elements to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SessionCreateParams#expand} for the field documentation.
*/
public Builder addAllExpand(List elements) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.addAll(elements);
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SessionCreateParams#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SessionCreateParams#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Add an element to `lineItems` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* SessionCreateParams#lineItems} for the field documentation.
*/
public Builder addLineItem(LineItem element) {
if (this.lineItems == null) {
this.lineItems = new ArrayList<>();
}
this.lineItems.add(element);
return this;
}
/**
* Add all elements to `lineItems` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* SessionCreateParams#lineItems} for the field documentation.
*/
public Builder addAllLineItem(List elements) {
if (this.lineItems == null) {
this.lineItems = new ArrayList<>();
}
this.lineItems.addAll(elements);
return this;
}
/**
* The IETF language tag of the locale Checkout is displayed in. If blank or `auto`, the
* browser's locale is used. Supported values are `auto`, `da`, `de`, `en`, `es`, `fi`, `fr`,
* `it`, `ja`, `nb`, `nl`, `pl`, `pt`, `sv`, or `zh`.
*/
public Builder setLocale(Locale locale) {
this.locale = locale;
return this;
}
/**
* A subset of parameters to be passed to PaymentIntent creation.
*/
public Builder setPaymentIntentData(PaymentIntentData paymentIntentData) {
this.paymentIntentData = paymentIntentData;
return this;
}
/**
* Add an element to `paymentMethodTypes` list. A list is initialized for the first `add/addAll`
* call, and subsequent calls adds additional elements to the original list. See {@link
* SessionCreateParams#paymentMethodTypes} for the field documentation.
*/
public Builder addPaymentMethodType(PaymentMethodType element) {
if (this.paymentMethodTypes == null) {
this.paymentMethodTypes = new ArrayList<>();
}
this.paymentMethodTypes.add(element);
return this;
}
/**
* Add all elements to `paymentMethodTypes` list. A list is initialized for the first
* `add/addAll` call, and subsequent calls adds additional elements to the original list. See
* {@link SessionCreateParams#paymentMethodTypes} for the field documentation.
*/
public Builder addAllPaymentMethodType(List elements) {
if (this.paymentMethodTypes == null) {
this.paymentMethodTypes = new ArrayList<>();
}
this.paymentMethodTypes.addAll(elements);
return this;
}
/**
* Describes the type of transaction being performed by Checkout in order to customize relevant
* text on the page, such as the submit button. `submit_type` can only be specified on Checkout
* Sessions using line items or a SKU, but not Checkout Sessions for subscriptions. Supported
* values are `auto`, `book`, `donate`, or `pay`.
*/
public Builder setSubmitType(SubmitType submitType) {
this.submitType = submitType;
return this;
}
/**
* A subset of parameters to be passed to subscription creation.
*/
public Builder setSubscriptionData(SubscriptionData subscriptionData) {
this.subscriptionData = subscriptionData;
return this;
}
/**
* The URL to which Stripe should send customers when payment is complete. If you’d like access
* to the Checkout Session for the successful payment, read more about it in our guide on
* [fulfilling your payments with webhooks](/docs/payments/checkout/fulfillment#webhooks).
*/
public Builder setSuccessUrl(String successUrl) {
this.successUrl = successUrl;
return this;
}
}
public static class LineItem {
/**
* The amount to be collected per unit of the line item.
*/
@SerializedName("amount")
Long amount;
/**
* Three-letter [ISO currency code](https://www.iso.org/iso-4217-currency-codes.html), in
* lowercase. Must be a [supported currency](https://stripe.com/docs/currencies).
*/
@SerializedName("currency")
String currency;
/**
* The description for the line item.
*/
@SerializedName("description")
String description;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* A list of images representing this line item.
*/
@SerializedName("images")
List images;
/**
* The name for the line item.
*/
@SerializedName("name")
String name;
/**
* The quantity of the line item being purchased.
*/
@SerializedName("quantity")
Long quantity;
private LineItem(Long amount, String currency, String description, Map extraParams, List images, String name, Long quantity) {
this.amount = amount;
this.currency = currency;
this.description = description;
this.extraParams = extraParams;
this.images = images;
this.name = name;
this.quantity = quantity;
}
public static Builder builder() {
return new com.stripe.param.checkout.SessionCreateParams.LineItem.Builder();
}
public static class Builder {
private Long amount;
private String currency;
private String description;
private Map extraParams;
private List images;
private String name;
private Long quantity;
/** Finalize and obtain parameter instance from this builder. */
public LineItem build() {
return new LineItem(this.amount, this.currency, this.description, this.extraParams, this.images, this.name, this.quantity);
}
/** The amount to be collected per unit of the line item. */
public Builder setAmount(Long amount) {
this.amount = amount;
return this;
}
/**
* Three-letter [ISO currency code](https://www.iso.org/iso-4217-currency-codes.html), in
* lowercase. Must be a [supported currency](https://stripe.com/docs/currencies).
*/
public Builder setCurrency(String currency) {
this.currency = currency;
return this;
}
/** The description for the line item. */
public Builder setDescription(String description) {
this.description = description;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SessionCreateParams.LineItem#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SessionCreateParams.LineItem#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Add an element to `images` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SessionCreateParams.LineItem#images} for the field documentation.
*/
public Builder addImage(String element) {
if (this.images == null) {
this.images = new ArrayList<>();
}
this.images.add(element);
return this;
}
/**
* Add all elements to `images` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* SessionCreateParams.LineItem#images} for the field documentation.
*/
public Builder addAllImage(List elements) {
if (this.images == null) {
this.images = new ArrayList<>();
}
this.images.addAll(elements);
return this;
}
/** The name for the line item. */
public Builder setName(String name) {
this.name = name;
return this;
}
public Builder setQuantity(Long quantity) {
this.quantity = quantity;
return this;
}
}
}
/**
* The quantity of the line item being purchased.
*/
public static class PaymentIntentData {
/**
* The amount of the application fee (if any) that will be applied to the payment and
* transferred to the application owner's Stripe account. To use an application fee, the request
* must be made on behalf of another account, using the `Stripe-Account` header or an OAuth key.
* For more information, see the PaymentIntents [use case for connected
* accounts](https://stripe.com/docs/payments/payment-intents/use-cases#connected-accounts).
*/
@SerializedName("application_fee_amount")
Long applicationFeeAmount;
/**
* Capture method of this PaymentIntent, one of `automatic` or `manual`.
*/
@SerializedName("capture_method")
CaptureMethod captureMethod;
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
*/
@SerializedName("description")
String description;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* The Stripe account ID for which these funds are intended. For details, see the PaymentIntents
* [use case for connected
* accounts](https://stripe.com/docs/payments/payment-intents/use-cases#connected-accounts).
*/
@SerializedName("on_behalf_of")
String onBehalfOf;
/**
* Email address that the receipt for the resulting payment will be sent to.
*/
@SerializedName("receipt_email")
String receiptEmail;
/**
* Shipping information for this payment.
*/
@SerializedName("shipping")
Shipping shipping;
/**
* Extra information about the payment. This will appear on your customer's statement when this
* payment succeeds in creating a charge.
*/
@SerializedName("statement_descriptor")
String statementDescriptor;
/**
* The parameters used to automatically create a Transfer when the payment succeeds. For more
* information, see the PaymentIntents [use case for connected
* accounts](https://stripe.com/docs/payments/payment-intents/use-cases#connected-accounts).
*/
@SerializedName("transfer_data")
TransferData transferData;
private PaymentIntentData(Long applicationFeeAmount, CaptureMethod captureMethod, String description, Map extraParams, Map metadata, String onBehalfOf, String receiptEmail, Shipping shipping, String statementDescriptor, TransferData transferData) {
this.applicationFeeAmount = applicationFeeAmount;
this.captureMethod = captureMethod;
this.description = description;
this.extraParams = extraParams;
this.metadata = metadata;
this.onBehalfOf = onBehalfOf;
this.receiptEmail = receiptEmail;
this.shipping = shipping;
this.statementDescriptor = statementDescriptor;
this.transferData = transferData;
}
public static Builder builder() {
return new com.stripe.param.checkout.SessionCreateParams.PaymentIntentData.Builder();
}
public static class Builder {
private Long applicationFeeAmount;
private CaptureMethod captureMethod;
private String description;
private Map extraParams;
private Map metadata;
private String onBehalfOf;
private String receiptEmail;
private Shipping shipping;
private String statementDescriptor;
private TransferData transferData;
/**
* Finalize and obtain parameter instance from this builder.
*/
public PaymentIntentData build() {
return new PaymentIntentData(this.applicationFeeAmount, this.captureMethod, this.description, this.extraParams, this.metadata, this.onBehalfOf, this.receiptEmail, this.shipping, this.statementDescriptor, this.transferData);
}
/**
* The amount of the application fee (if any) that will be applied to the payment and
* transferred to the application owner's Stripe account. To use an application fee, the
* request must be made on behalf of another account, using the `Stripe-Account` header or an
* OAuth key. For more information, see the PaymentIntents [use case for connected
* accounts](https://stripe.com/docs/payments/payment-intents/use-cases#connected-accounts).
*/
public Builder setApplicationFeeAmount(Long applicationFeeAmount) {
this.applicationFeeAmount = applicationFeeAmount;
return this;
}
/**
* Capture method of this PaymentIntent, one of `automatic` or `manual`.
*/
public Builder setCaptureMethod(CaptureMethod captureMethod) {
this.captureMethod = captureMethod;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
*/
public Builder setDescription(String description) {
this.description = description;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SessionCreateParams.PaymentIntentData#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SessionCreateParams.PaymentIntentData#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Add a key/value pair to `metadata` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SessionCreateParams.PaymentIntentData#metadata} for the field documentation.
*/
public Builder putMetadata(String key, String value) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `metadata` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SessionCreateParams.PaymentIntentData#metadata} for the field documentation.
*/
public Builder putAllMetadata(Map map) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.putAll(map);
return this;
}
/**
* The Stripe account ID for which these funds are intended. For details, see the
* PaymentIntents [use case for connected
* accounts](https://stripe.com/docs/payments/payment-intents/use-cases#connected-accounts).
*/
public Builder setOnBehalfOf(String onBehalfOf) {
this.onBehalfOf = onBehalfOf;
return this;
}
/**
* Email address that the receipt for the resulting payment will be sent to.
*/
public Builder setReceiptEmail(String receiptEmail) {
this.receiptEmail = receiptEmail;
return this;
}
/**
* Shipping information for this payment.
*/
public Builder setShipping(Shipping shipping) {
this.shipping = shipping;
return this;
}
/**
* Extra information about the payment. This will appear on your customer's statement when
* this payment succeeds in creating a charge.
*/
public Builder setStatementDescriptor(String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
return this;
}
/**
* The parameters used to automatically create a Transfer when the payment succeeds. For more
* information, see the PaymentIntents [use case for connected
* accounts](https://stripe.com/docs/payments/payment-intents/use-cases#connected-accounts).
*/
public Builder setTransferData(TransferData transferData) {
this.transferData = transferData;
return this;
}
}
public static class Shipping {
/**
* Shipping address.
*/
@SerializedName("address")
Address address;
/**
* The delivery service that shipped a physical product, such as Fedex, UPS, USPS, etc.
*/
@SerializedName("carrier")
String carrier;
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Recipient name.
*/
@SerializedName("name")
String name;
/**
* Recipient phone (including extension).
*/
@SerializedName("phone")
String phone;
/**
* The tracking number for a physical product, obtained from the delivery service. If multiple
* tracking numbers were generated for this purchase, please separate them with commas.
*/
@SerializedName("tracking_number")
String trackingNumber;
private Shipping(Address address, String carrier, Map extraParams, String name, String phone, String trackingNumber) {
this.address = address;
this.carrier = carrier;
this.extraParams = extraParams;
this.name = name;
this.phone = phone;
this.trackingNumber = trackingNumber;
}
public static Builder builder() {
return new com.stripe.param.checkout.SessionCreateParams.PaymentIntentData.Shipping.Builder();
}
public static class Builder {
private Address address;
private String carrier;
private Map extraParams;
private String name;
private String phone;
private String trackingNumber;
/**
* Finalize and obtain parameter instance from this builder.
*/
public Shipping build() {
return new Shipping(this.address, this.carrier, this.extraParams, this.name, this.phone, this.trackingNumber);
}
/**
* Shipping address.
*/
public Builder setAddress(Address address) {
this.address = address;
return this;
}
/**
* The delivery service that shipped a physical product, such as Fedex, UPS, USPS, etc.
*/
public Builder setCarrier(String carrier) {
this.carrier = carrier;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SessionCreateParams.PaymentIntentData.Shipping#extraParams} for the field
* documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SessionCreateParams.PaymentIntentData.Shipping#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Recipient name.
*/
public Builder setName(String name) {
this.name = name;
return this;
}
/**
* Recipient phone (including extension).
*/
public Builder setPhone(String phone) {
this.phone = phone;
return this;
}
/**
* The tracking number for a physical product, obtained from the delivery service. If
* multiple tracking numbers were generated for this purchase, please separate them with
* commas.
*/
public Builder setTrackingNumber(String trackingNumber) {
this.trackingNumber = trackingNumber;
return this;
}
}
public static class Address {
@SerializedName("city")
String city;
@SerializedName("country")
String country;
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field
* (serialized) name in this param object. Effectively, this map is flattened to its parent
* instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
@SerializedName("line1")
String line1;
@SerializedName("line2")
String line2;
@SerializedName("postal_code")
String postalCode;
@SerializedName("state")
String state;
private Address(String city, String country, Map extraParams, String line1, String line2, String postalCode, String state) {
this.city = city;
this.country = country;
this.extraParams = extraParams;
this.line1 = line1;
this.line2 = line2;
this.postalCode = postalCode;
this.state = state;
}
public static Builder builder() {
return new com.stripe.param.checkout.SessionCreateParams.PaymentIntentData.Shipping.Address.Builder();
}
public static class Builder {
private String city;
private String country;
private Map extraParams;
private String line1;
private String line2;
private String postalCode;
private String state;
/** Finalize and obtain parameter instance from this builder. */
public Address build() {
return new Address(this.city, this.country, this.extraParams, this.line1, this.line2, this.postalCode, this.state);
}
public Builder setCity(String city) {
this.city = city;
return this;
}
public Builder setCountry(String country) {
this.country = country;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SessionCreateParams.PaymentIntentData.Shipping.Address#extraParams} for
* the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
public Builder setLine1(String line1) {
this.line1 = line1;
return this;
}
public Builder setLine2(String line2) {
this.line2 = line2;
return this;
}
public Builder setPostalCode(String postalCode) {
this.postalCode = postalCode;
return this;
}
public Builder setState(String state) {
this.state = state;
return this;
}
}
}
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SessionCreateParams.PaymentIntentData.Shipping.Address#extraParams} for
* the field documentation.
*/
public static class TransferData {
/**
* If specified, successful charges will be attributed to the destination account for tax
* reporting, and the funds from charges will be transferred to the destination account. The
* ID of the resulting transfer will be returned on the successful charge's `transfer` field.
*/
@SerializedName("destination")
String destination;
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
private TransferData(String destination, Map extraParams) {
this.destination = destination;
this.extraParams = extraParams;
}
public static Builder builder() {
return new com.stripe.param.checkout.SessionCreateParams.PaymentIntentData.TransferData.Builder();
}
public static class Builder {
private String destination;
private Map extraParams;
/** Finalize and obtain parameter instance from this builder. */
public TransferData build() {
return new TransferData(this.destination, this.extraParams);
}
/**
* If specified, successful charges will be attributed to the destination account for tax
* reporting, and the funds from charges will be transferred to the destination account. The
* ID of the resulting transfer will be returned on the successful charge's `transfer`
* field.
*/
public Builder setDestination(String destination) {
this.destination = destination;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SessionCreateParams.PaymentIntentData.TransferData#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
}
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SessionCreateParams.PaymentIntentData.TransferData#extraParams} for the
* field documentation.
*/
public enum CaptureMethod implements ApiRequestParams.EnumParam {
@SerializedName("automatic")
AUTOMATIC("automatic"), @SerializedName("manual")
MANUAL("manual");
private final String value;
CaptureMethod(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
}
public static class SubscriptionData {
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* A list of items, each with an attached plan, that the customer is subscribing to. Use this
* parameter for subscriptions. To create one-time payments, use `line_items`.
*/
@SerializedName("items")
List- items;
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@SerializedName("metadata")
Map
metadata;
/**
* Unix timestamp representing the end of the trial period the customer will get before being
* charged for the first time. Has to be at least 48h in the future.
*/
@SerializedName("trial_end")
Long trialEnd;
/**
* Integer representing the number of trial period days before the customer is charged for the
* first time.Has to be at least 1.
*/
@SerializedName("trial_period_days")
Long trialPeriodDays;
private SubscriptionData(Map extraParams, List- items, Map
metadata, Long trialEnd, Long trialPeriodDays) {
this.extraParams = extraParams;
this.items = items;
this.metadata = metadata;
this.trialEnd = trialEnd;
this.trialPeriodDays = trialPeriodDays;
}
public static Builder builder() {
return new com.stripe.param.checkout.SessionCreateParams.SubscriptionData.Builder();
}
public static class Builder {
private Map extraParams;
private List- items;
private Map
metadata;
private Long trialEnd;
private Long trialPeriodDays;
/**
* Finalize and obtain parameter instance from this builder.
*/
public SubscriptionData build() {
return new SubscriptionData(this.extraParams, this.items, this.metadata, this.trialEnd, this.trialPeriodDays);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SessionCreateParams.SubscriptionData#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SessionCreateParams.SubscriptionData#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Add an element to `items` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SessionCreateParams.SubscriptionData#items} for the field documentation.
*/
public Builder addItem(Item element) {
if (this.items == null) {
this.items = new ArrayList<>();
}
this.items.add(element);
return this;
}
/**
* Add all elements to `items` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* SessionCreateParams.SubscriptionData#items} for the field documentation.
*/
public Builder addAllItem(List- elements) {
if (this.items == null) {
this.items = new ArrayList<>();
}
this.items.addAll(elements);
return this;
}
/**
* Add a key/value pair to `metadata` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SessionCreateParams.SubscriptionData#metadata} for the field documentation.
*/
public Builder putMetadata(String key, String value) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `metadata` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SessionCreateParams.SubscriptionData#metadata} for the field documentation.
*/
public Builder putAllMetadata(Map
map) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.putAll(map);
return this;
}
/**
* Unix timestamp representing the end of the trial period the customer will get before being
* charged for the first time. Has to be at least 48h in the future.
*/
public Builder setTrialEnd(Long trialEnd) {
this.trialEnd = trialEnd;
return this;
}
/**
* Integer representing the number of trial period days before the customer is charged for the
* first time.Has to be at least 1.
*/
public Builder setTrialPeriodDays(Long trialPeriodDays) {
this.trialPeriodDays = trialPeriodDays;
return this;
}
}
public static class Item {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/** Plan ID for this item. */
@SerializedName("plan")
String plan;
/** Quantity for this item. */
@SerializedName("quantity")
Long quantity;
private Item(Map extraParams, String plan, Long quantity) {
this.extraParams = extraParams;
this.plan = plan;
this.quantity = quantity;
}
public static Builder builder() {
return new com.stripe.param.checkout.SessionCreateParams.SubscriptionData.Item.Builder();
}
public static class Builder {
private Map extraParams;
private String plan;
private Long quantity;
/** Finalize and obtain parameter instance from this builder. */
public Item build() {
return new Item(this.extraParams, this.plan, this.quantity);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SessionCreateParams.SubscriptionData.Item#extraParams} for the field
* documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SessionCreateParams.SubscriptionData.Item#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/** Plan ID for this item. */
public Builder setPlan(String plan) {
this.plan = plan;
return this;
}
public Builder setQuantity(Long quantity) {
this.quantity = quantity;
return this;
}
}
}
}
/**
* Quantity for this item.
*/
public enum BillingAddressCollection implements ApiRequestParams.EnumParam {
@SerializedName("auto")
AUTO("auto"), @SerializedName("required")
REQUIRED("required");
private final String value;
BillingAddressCollection(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum Locale implements ApiRequestParams.EnumParam {
@SerializedName("auto")
AUTO("auto"), @SerializedName("da")
DA("da"), @SerializedName("de")
DE("de"), @SerializedName("en")
EN("en"), @SerializedName("es")
ES("es"), @SerializedName("fi")
FI("fi"), @SerializedName("fr")
FR("fr"), @SerializedName("it")
IT("it"), @SerializedName("ja")
JA("ja"), @SerializedName("nb")
NB("nb"), @SerializedName("nl")
NL("nl"), @SerializedName("pl")
PL("pl"), @SerializedName("pt")
PT("pt"), @SerializedName("sv")
SV("sv"), @SerializedName("zh")
ZH("zh");
private final String value;
Locale(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum PaymentMethodType implements ApiRequestParams.EnumParam {
@SerializedName("card")
CARD("card");
private final String value;
PaymentMethodType(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum SubmitType implements ApiRequestParams.EnumParam {
@SerializedName("auto")
AUTO("auto"), @SerializedName("book")
BOOK("book"), @SerializedName("donate")
DONATE("donate"), @SerializedName("pay")
PAY("pay");
private final String value;
SubmitType(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy