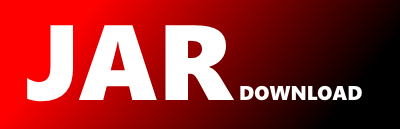
com.stripe.model.Capability Maven / Gradle / Ivy
// Generated by delombok at Fri Dec 20 23:44:59 CET 2019
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.CapabilityUpdateParams;
import java.util.List;
import java.util.Map;
public class Capability extends ApiResource implements HasId {
/**
* The account for which the capability enables functionality.
*/
@SerializedName("account")
ExpandableField account;
/**
* The identifier for the capability.
*/
@SerializedName("id")
String id;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to `capability`.
*/
@SerializedName("object")
String object;
/**
* Whether the capability has been requested.
*/
@SerializedName("requested")
Boolean requested;
/**
* Time at which the capability was requested. Measured in seconds since the Unix epoch.
*/
@SerializedName("requested_at")
Long requestedAt;
@SerializedName("requirements")
Requirements requirements;
/**
* The status of the capability. Can be `active`, `inactive`, `pending`, or `unrequested`.
*/
@SerializedName("status")
String status;
/**
* Get id of expandable `account` object.
*/
public String getAccount() {
return (this.account != null) ? this.account.getId() : null;
}
public void setAccount(String id) {
this.account = ApiResource.setExpandableFieldId(id, this.account);
}
/**
* Get expanded `account`.
*/
public Account getAccountObject() {
return (this.account != null) ? this.account.getExpanded() : null;
}
public void setAccountObject(Account expandableObject) {
this.account = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Updates an existing Account Capability.
*/
public Capability update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates an existing Account Capability.
*/
public Capability update(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/accounts/%s/capabilities/%s", ApiResource.urlEncodeId(this.getAccount()), ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Capability.class, options);
}
/**
* Updates an existing Account Capability.
*/
public Capability update(CapabilityUpdateParams params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates an existing Account Capability.
*/
public Capability update(CapabilityUpdateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/accounts/%s/capabilities/%s", ApiResource.urlEncodeId(this.getAccount()), ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Capability.class, options);
}
public static class Requirements extends StripeObject {
/**
* The date the fields in `currently_due` must be collected by to keep the capability enabled
* for the account.
*/
@SerializedName("current_deadline")
Long currentDeadline;
/**
* The fields that need to be collected to keep the capability enabled. If not collected by the
* `current_deadline`, these fields appear in `past_due` as well, and the capability is
* disabled.
*/
@SerializedName("currently_due")
List currentlyDue;
/**
* If the capability is disabled, this string describes why. Possible values are
* `requirement.fields_needed`, `pending.onboarding`, `pending.review`, `rejected_fraud`, or
* `rejected.other`.
*/
@SerializedName("disabled_reason")
String disabledReason;
/**
* The fields that need to be collected assuming all volume thresholds are reached. As they
* become required, these fields appear in `currently_due` as well, and the `current_deadline`
* is set.
*/
@SerializedName("eventually_due")
List eventuallyDue;
/**
* The fields that weren't collected by the `current_deadline`. These fields need to be
* collected to enable the capability for the account.
*/
@SerializedName("past_due")
List pastDue;
/**
* Fields that may become required depending on the results of verification or review. An empty
* array unless an asynchronous verification is pending. If verification fails, the fields in
* this array become required and move to `currently_due` or `past_due`.
*/
@SerializedName("pending_verification")
List pendingVerification;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCurrentDeadline() {
return this.currentDeadline;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getCurrentlyDue() {
return this.currentlyDue;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDisabledReason() {
return this.disabledReason;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getEventuallyDue() {
return this.eventuallyDue;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getPastDue() {
return this.pastDue;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getPendingVerification() {
return this.pendingVerification;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrentDeadline(final Long currentDeadline) {
this.currentDeadline = currentDeadline;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrentlyDue(final List currentlyDue) {
this.currentlyDue = currentlyDue;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDisabledReason(final String disabledReason) {
this.disabledReason = disabledReason;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEventuallyDue(final List eventuallyDue) {
this.eventuallyDue = eventuallyDue;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPastDue(final List pastDue) {
this.pastDue = pastDue;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPendingVerification(final List pendingVerification) {
this.pendingVerification = pendingVerification;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Capability.Requirements)) return false;
final Capability.Requirements other = (Capability.Requirements) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$currentDeadline = this.getCurrentDeadline();
final java.lang.Object other$currentDeadline = other.getCurrentDeadline();
if (this$currentDeadline == null ? other$currentDeadline != null : !this$currentDeadline.equals(other$currentDeadline)) return false;
final java.lang.Object this$currentlyDue = this.getCurrentlyDue();
final java.lang.Object other$currentlyDue = other.getCurrentlyDue();
if (this$currentlyDue == null ? other$currentlyDue != null : !this$currentlyDue.equals(other$currentlyDue)) return false;
final java.lang.Object this$disabledReason = this.getDisabledReason();
final java.lang.Object other$disabledReason = other.getDisabledReason();
if (this$disabledReason == null ? other$disabledReason != null : !this$disabledReason.equals(other$disabledReason)) return false;
final java.lang.Object this$eventuallyDue = this.getEventuallyDue();
final java.lang.Object other$eventuallyDue = other.getEventuallyDue();
if (this$eventuallyDue == null ? other$eventuallyDue != null : !this$eventuallyDue.equals(other$eventuallyDue)) return false;
final java.lang.Object this$pastDue = this.getPastDue();
final java.lang.Object other$pastDue = other.getPastDue();
if (this$pastDue == null ? other$pastDue != null : !this$pastDue.equals(other$pastDue)) return false;
final java.lang.Object this$pendingVerification = this.getPendingVerification();
final java.lang.Object other$pendingVerification = other.getPendingVerification();
if (this$pendingVerification == null ? other$pendingVerification != null : !this$pendingVerification.equals(other$pendingVerification)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Capability.Requirements;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $currentDeadline = this.getCurrentDeadline();
result = result * PRIME + ($currentDeadline == null ? 43 : $currentDeadline.hashCode());
final java.lang.Object $currentlyDue = this.getCurrentlyDue();
result = result * PRIME + ($currentlyDue == null ? 43 : $currentlyDue.hashCode());
final java.lang.Object $disabledReason = this.getDisabledReason();
result = result * PRIME + ($disabledReason == null ? 43 : $disabledReason.hashCode());
final java.lang.Object $eventuallyDue = this.getEventuallyDue();
result = result * PRIME + ($eventuallyDue == null ? 43 : $eventuallyDue.hashCode());
final java.lang.Object $pastDue = this.getPastDue();
result = result * PRIME + ($pastDue == null ? 43 : $pastDue.hashCode());
final java.lang.Object $pendingVerification = this.getPendingVerification();
result = result * PRIME + ($pendingVerification == null ? 43 : $pendingVerification.hashCode());
return result;
}
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to `capability`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* Whether the capability has been requested.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getRequested() {
return this.requested;
}
/**
* Time at which the capability was requested. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getRequestedAt() {
return this.requestedAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Requirements getRequirements() {
return this.requirements;
}
/**
* The status of the capability. Can be `active`, `inactive`, `pending`, or `unrequested`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
/**
* The identifier for the capability.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to `capability`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* Whether the capability has been requested.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRequested(final Boolean requested) {
this.requested = requested;
}
/**
* Time at which the capability was requested. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRequestedAt(final Long requestedAt) {
this.requestedAt = requestedAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRequirements(final Requirements requirements) {
this.requirements = requirements;
}
/**
* The status of the capability. Can be `active`, `inactive`, `pending`, or `unrequested`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Capability)) return false;
final Capability other = (Capability) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$account = this.getAccount();
final java.lang.Object other$account = other.getAccount();
if (this$account == null ? other$account != null : !this$account.equals(other$account)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$requested = this.getRequested();
final java.lang.Object other$requested = other.getRequested();
if (this$requested == null ? other$requested != null : !this$requested.equals(other$requested)) return false;
final java.lang.Object this$requestedAt = this.getRequestedAt();
final java.lang.Object other$requestedAt = other.getRequestedAt();
if (this$requestedAt == null ? other$requestedAt != null : !this$requestedAt.equals(other$requestedAt)) return false;
final java.lang.Object this$requirements = this.getRequirements();
final java.lang.Object other$requirements = other.getRequirements();
if (this$requirements == null ? other$requirements != null : !this$requirements.equals(other$requirements)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Capability;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $account = this.getAccount();
result = result * PRIME + ($account == null ? 43 : $account.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $requested = this.getRequested();
result = result * PRIME + ($requested == null ? 43 : $requested.hashCode());
final java.lang.Object $requestedAt = this.getRequestedAt();
result = result * PRIME + ($requestedAt == null ? 43 : $requestedAt.hashCode());
final java.lang.Object $requirements = this.getRequirements();
result = result * PRIME + ($requirements == null ? 43 : $requirements.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
return result;
}
/**
* The identifier for the capability.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
}