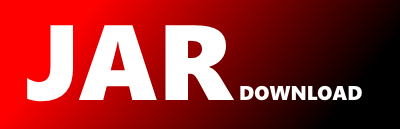
com.stripe.model.Invoice Maven / Gradle / Ivy
// Generated by delombok at Fri Dec 20 23:44:58 CET 2019
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.InvoiceCreateParams;
import com.stripe.param.InvoiceFinalizeInvoiceParams;
import com.stripe.param.InvoiceListParams;
import com.stripe.param.InvoiceMarkUncollectibleParams;
import com.stripe.param.InvoicePayParams;
import com.stripe.param.InvoiceRetrieveParams;
import com.stripe.param.InvoiceSendInvoiceParams;
import com.stripe.param.InvoiceUpcomingParams;
import com.stripe.param.InvoiceUpdateParams;
import com.stripe.param.InvoiceVoidInvoiceParams;
import java.math.BigDecimal;
import java.util.List;
import java.util.Map;
public class Invoice extends ApiResource implements HasId, MetadataStore {
/**
* The country of the business associated with this invoice, most often the business creating the
* invoice.
*/
@SerializedName("account_country")
String accountCountry;
/**
* The public name of the business associated with this invoice, most often the business creating
* the invoice.
*/
@SerializedName("account_name")
String accountName;
/**
* Final amount due at this time for this invoice. If the invoice's total is smaller than the
* minimum charge amount, for example, or if there is account credit that can be applied to the
* invoice, the `amount_due` may be 0. If there is a positive `starting_balance` for the invoice
* (the customer owes money), the `amount_due` will also take that into account. The charge that
* gets generated for the invoice will be for the amount specified in `amount_due`.
*/
@SerializedName("amount_due")
Long amountDue;
/**
* The amount, in %s, that was paid.
*/
@SerializedName("amount_paid")
Long amountPaid;
/**
* The amount remaining, in %s, that is due.
*/
@SerializedName("amount_remaining")
Long amountRemaining;
/**
* The fee in %s that will be applied to the invoice and transferred to the application owner's
* Stripe account when the invoice is paid.
*/
@SerializedName("application_fee_amount")
Long applicationFeeAmount;
/**
* Number of payment attempts made for this invoice, from the perspective of the payment retry
* schedule. Any payment attempt counts as the first attempt, and subsequently only automatic
* retries increment the attempt count. In other words, manual payment attempts after the first
* attempt do not affect the retry schedule.
*/
@SerializedName("attempt_count")
Long attemptCount;
/**
* Whether an attempt has been made to pay the invoice. An invoice is not attempted until 1 hour
* after the `invoice.created` webhook, for example, so you might not want to display that invoice
* as unpaid to your users.
*/
@SerializedName("attempted")
Boolean attempted;
/**
* Controls whether Stripe will perform [automatic
* collection](https://stripe.com/docs/billing/invoices/workflow/#auto_advance) of the invoice.
* When `false`, the invoice's state will not automatically advance without an explicit action.
*/
@SerializedName("auto_advance")
Boolean autoAdvance;
/**
* Indicates the reason why the invoice was created. `subscription_cycle` indicates an invoice
* created by a subscription advancing into a new period. `subscription_create` indicates an
* invoice created due to creating a subscription. `subscription_update` indicates an invoice
* created due to updating a subscription. `subscription` is set for all old invoices to indicate
* either a change to a subscription or a period advancement. `manual` is set for all invoices
* unrelated to a subscription (for example: created via the invoice editor). The `upcoming` value
* is reserved for simulated invoices per the upcoming invoice endpoint. `subscription_threshold`
* indicates an invoice created due to a billing threshold being reached.
*
* One of `automatic_pending_invoice_item_invoice`, `manual`, `subscription`,
* `subscription_create`, `subscription_cycle`, `subscription_threshold`, `subscription_update`,
* or `upcoming`.
*/
@SerializedName("billing_reason")
String billingReason;
/**
* ID of the latest charge generated for this invoice, if any.
*/
@SerializedName("charge")
ExpandableField charge;
/**
* Either `charge_automatically`, or `send_invoice`. When charging automatically, Stripe will
* attempt to pay this invoice using the default source attached to the customer. When sending an
* invoice, Stripe will email this invoice to the customer with payment instructions.
*
* One of `charge_automatically`, or `send_invoice`.
*/
@SerializedName("collection_method")
String collectionMethod;
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* Three-letter [ISO currency code](https://www.iso.org/iso-4217-currency-codes.html), in
* lowercase. Must be a [supported currency](https://stripe.com/docs/currencies).
*/
@SerializedName("currency")
String currency;
/**
* Custom fields displayed on the invoice.
*/
@SerializedName("custom_fields")
List customFields;
@SerializedName("customer")
ExpandableField customer;
/**
* The customer's address. Until the invoice is finalized, this field will equal
* `customer.address`. Once the invoice is finalized, this field will no longer be updated.
*/
@SerializedName("customer_address")
Address customerAddress;
/**
* The customer's email. Until the invoice is finalized, this field will equal `customer.email`.
* Once the invoice is finalized, this field will no longer be updated.
*/
@SerializedName("customer_email")
String customerEmail;
/**
* The customer's name. Until the invoice is finalized, this field will equal `customer.name`.
* Once the invoice is finalized, this field will no longer be updated.
*/
@SerializedName("customer_name")
String customerName;
/**
* The customer's phone number. Until the invoice is finalized, this field will equal
* `customer.phone`. Once the invoice is finalized, this field will no longer be updated.
*/
@SerializedName("customer_phone")
String customerPhone;
/**
* The customer's shipping information. Until the invoice is finalized, this field will equal
* `customer.shipping`. Once the invoice is finalized, this field will no longer be updated.
*/
@SerializedName("customer_shipping")
ShippingDetails customerShipping;
/**
* The customer's tax exempt status. Until the invoice is finalized, this field will equal
* `customer.tax_exempt`. Once the invoice is finalized, this field will no longer be updated.
*
* One of `exempt`, `none`, or `reverse`.
*/
@SerializedName("customer_tax_exempt")
String customerTaxExempt;
/**
* The customer's tax IDs. Until the invoice is finalized, this field will contain the same tax
* IDs as `customer.tax_ids`. Once the invoice is finalized, this field will no longer be updated.
*/
@SerializedName("customer_tax_ids")
List customerTaxIds;
/**
* ID of the default payment method for the invoice. It must belong to the customer associated
* with the invoice. If not set, defaults to the subscription's default payment method, if any, or
* to the default payment method in the customer's invoice settings.
*/
@SerializedName("default_payment_method")
ExpandableField defaultPaymentMethod;
/**
* ID of the default payment source for the invoice. It must belong to the customer associated
* with the invoice and be in a chargeable state. If not set, defaults to the subscription's
* default source, if any, or to the customer's default source.
*/
@SerializedName("default_source")
ExpandableField defaultSource;
/**
* The tax rates applied to this invoice, if any.
*/
@SerializedName("default_tax_rates")
List defaultTaxRates;
/**
* Always true for a deleted object.
*/
@SerializedName("deleted")
Boolean deleted;
/**
* An arbitrary string attached to the object. Often useful for displaying to users. Referenced as
* 'memo' in the Dashboard.
*/
@SerializedName("description")
String description;
@SerializedName("discount")
Discount discount;
/**
* The date on which payment for this invoice is due. This value will be `null` for invoices where
* `collection_method=charge_automatically`.
*/
@SerializedName("due_date")
Long dueDate;
/**
* Ending customer balance after the invoice is finalized. Invoices are finalized approximately an
* hour after successful webhook delivery or when payment collection is attempted for the invoice.
* If the invoice has not been finalized yet, this will be null.
*/
@SerializedName("ending_balance")
Long endingBalance;
/**
* Footer displayed on the invoice.
*/
@SerializedName("footer")
String footer;
/**
* The URL for the hosted invoice page, which allows customers to view and pay an invoice. If the
* invoice has not been finalized yet, this will be null.
*/
@SerializedName("hosted_invoice_url")
String hostedInvoiceUrl;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* The link to download the PDF for the invoice. If the invoice has not been finalized yet, this
* will be null.
*/
@SerializedName("invoice_pdf")
String invoicePdf;
/**
* The individual line items that make up the invoice. `lines` is sorted as follows: invoice items
* in reverse chronological order, followed by the subscription, if any.
*/
@SerializedName("lines")
InvoiceLineItemCollection lines;
/**
* Has the value `true` if the object exists in live mode or the value `false` if the object
* exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* The time at which payment will next be attempted. This value will be `null` for invoices where
* `collection_method=send_invoice`.
*/
@SerializedName("next_payment_attempt")
Long nextPaymentAttempt;
/**
* A unique, identifying string that appears on emails sent to the customer for this invoice. This
* starts with the customer's unique invoice_prefix if it is specified.
*/
@SerializedName("number")
String number;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to `invoice`.
*/
@SerializedName("object")
String object;
/**
* Whether payment was successfully collected for this invoice. An invoice can be paid (most
* commonly) with a charge or with credit from the customer's account balance.
*/
@SerializedName("paid")
Boolean paid;
/**
* The PaymentIntent associated with this invoice. The PaymentIntent is generated when the invoice
* is finalized, and can then be used to pay the invoice. Note that voiding an invoice will cancel
* the PaymentIntent.
*/
@SerializedName("payment_intent")
ExpandableField paymentIntent;
/**
* End of the usage period during which invoice items were added to this invoice.
*/
@SerializedName("period_end")
Long periodEnd;
/**
* Start of the usage period during which invoice items were added to this invoice.
*/
@SerializedName("period_start")
Long periodStart;
/**
* Total amount of all post-payment credit notes issued for this invoice.
*/
@SerializedName("post_payment_credit_notes_amount")
Long postPaymentCreditNotesAmount;
/**
* Total amount of all pre-payment credit notes issued for this invoice.
*/
@SerializedName("pre_payment_credit_notes_amount")
Long prePaymentCreditNotesAmount;
/**
* This is the transaction number that appears on email receipts sent for this invoice.
*/
@SerializedName("receipt_number")
String receiptNumber;
/**
* Starting customer balance before the invoice is finalized. If the invoice has not been
* finalized yet, this will be the current customer balance.
*/
@SerializedName("starting_balance")
Long startingBalance;
/**
* Extra information about an invoice for the customer's credit card statement.
*/
@SerializedName("statement_descriptor")
String statementDescriptor;
/**
* The status of the invoice, one of `draft`, `open`, `paid`, `uncollectible`, or `void`. [Learn
* more](https://stripe.com/docs/billing/invoices/workflow#workflow-overview)
*/
@SerializedName("status")
String status;
@SerializedName("status_transitions")
StatusTransitions statusTransitions;
/**
* The subscription that this invoice was prepared for, if any.
*/
@SerializedName("subscription")
ExpandableField subscription;
/**
* Only set for upcoming invoices that preview prorations. The time used to calculate prorations.
*/
@SerializedName("subscription_proration_date")
Long subscriptionProrationDate;
/**
* Total of all subscriptions, invoice items, and prorations on the invoice before any discount or
* tax is applied.
*/
@SerializedName("subtotal")
Long subtotal;
/**
* The amount of tax on this invoice. This is the sum of all the tax amounts on this invoice.
*/
@SerializedName("tax")
Long tax;
/**
* This percentage of the subtotal has been added to the total amount of the invoice, including
* invoice line items and discounts. This field is inherited from the subscription's `tax_percent`
* field, but can be changed before the invoice is paid. This field defaults to null.
*/
@SerializedName("tax_percent")
BigDecimal taxPercent;
@SerializedName("threshold_reason")
ThresholdReason thresholdReason;
/**
* Total after discounts and taxes.
*/
@SerializedName("total")
Long total;
/**
* The aggregate amounts calculated per tax rate for all line items.
*/
@SerializedName("total_tax_amounts")
List totalTaxAmounts;
/**
* If specified, the funds from the invoice will be transferred to the destination and the ID of
* the resulting transfer will be found on the invoice's charge.
*/
@SerializedName("transfer_data")
TransferData transferData;
/**
* The time at which webhooks for this invoice were successfully delivered (if the invoice had no
* webhooks to deliver, this will match `created`). Invoice payment is delayed until webhooks are
* delivered, or until all webhook delivery attempts have been exhausted.
*/
@SerializedName("webhooks_delivered_at")
Long webhooksDeliveredAt;
/**
* Get id of expandable `charge` object.
*/
public String getCharge() {
return (this.charge != null) ? this.charge.getId() : null;
}
public void setCharge(String id) {
this.charge = ApiResource.setExpandableFieldId(id, this.charge);
}
/**
* Get expanded `charge`.
*/
public Charge getChargeObject() {
return (this.charge != null) ? this.charge.getExpanded() : null;
}
public void setChargeObject(Charge expandableObject) {
this.charge = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get id of expandable `customer` object.
*/
public String getCustomer() {
return (this.customer != null) ? this.customer.getId() : null;
}
public void setCustomer(String id) {
this.customer = ApiResource.setExpandableFieldId(id, this.customer);
}
/**
* Get expanded `customer`.
*/
public Customer getCustomerObject() {
return (this.customer != null) ? this.customer.getExpanded() : null;
}
public void setCustomerObject(Customer expandableObject) {
this.customer = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get id of expandable `defaultPaymentMethod` object.
*/
public String getDefaultPaymentMethod() {
return (this.defaultPaymentMethod != null) ? this.defaultPaymentMethod.getId() : null;
}
public void setDefaultPaymentMethod(String id) {
this.defaultPaymentMethod = ApiResource.setExpandableFieldId(id, this.defaultPaymentMethod);
}
/**
* Get expanded `defaultPaymentMethod`.
*/
public PaymentMethod getDefaultPaymentMethodObject() {
return (this.defaultPaymentMethod != null) ? this.defaultPaymentMethod.getExpanded() : null;
}
public void setDefaultPaymentMethodObject(PaymentMethod expandableObject) {
this.defaultPaymentMethod = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get id of expandable `defaultSource` object.
*/
public String getDefaultSource() {
return (this.defaultSource != null) ? this.defaultSource.getId() : null;
}
public void setDefaultSource(String id) {
this.defaultSource = ApiResource.setExpandableFieldId(id, this.defaultSource);
}
/**
* Get expanded `defaultSource`.
*/
public PaymentSource getDefaultSourceObject() {
return (this.defaultSource != null) ? this.defaultSource.getExpanded() : null;
}
public void setDefaultSourceObject(PaymentSource expandableObject) {
this.defaultSource = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get id of expandable `paymentIntent` object.
*/
public String getPaymentIntent() {
return (this.paymentIntent != null) ? this.paymentIntent.getId() : null;
}
public void setPaymentIntent(String id) {
this.paymentIntent = ApiResource.setExpandableFieldId(id, this.paymentIntent);
}
/**
* Get expanded `paymentIntent`.
*/
public PaymentIntent getPaymentIntentObject() {
return (this.paymentIntent != null) ? this.paymentIntent.getExpanded() : null;
}
public void setPaymentIntentObject(PaymentIntent expandableObject) {
this.paymentIntent = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get id of expandable `subscription` object.
*/
public String getSubscription() {
return (this.subscription != null) ? this.subscription.getId() : null;
}
public void setSubscription(String id) {
this.subscription = ApiResource.setExpandableFieldId(id, this.subscription);
}
/**
* Get expanded `subscription`.
*/
public Subscription getSubscriptionObject() {
return (this.subscription != null) ? this.subscription.getExpanded() : null;
}
public void setSubscriptionObject(Subscription expandableObject) {
this.subscription = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* You can list all invoices, or list the invoices for a specific customer. The invoices are
* returned sorted by creation date, with the most recently created invoices appearing first.
*/
public static InvoiceCollection list(Map params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* You can list all invoices, or list the invoices for a specific customer. The invoices are
* returned sorted by creation date, with the most recently created invoices appearing first.
*/
public static InvoiceCollection list(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/invoices");
return ApiResource.requestCollection(url, params, InvoiceCollection.class, options);
}
/**
* You can list all invoices, or list the invoices for a specific customer. The invoices are
* returned sorted by creation date, with the most recently created invoices appearing first.
*/
public static InvoiceCollection list(InvoiceListParams params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* You can list all invoices, or list the invoices for a specific customer. The invoices are
* returned sorted by creation date, with the most recently created invoices appearing first.
*/
public static InvoiceCollection list(InvoiceListParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/invoices");
return ApiResource.requestCollection(url, params, InvoiceCollection.class, options);
}
/**
* At any time, you can preview the upcoming invoice for a customer. This will show you all the
* charges that are pending, including subscription renewal charges, invoice item charges, etc. It
* will also show you any discount that is applicable to the customer.
*
* Note that when you are viewing an upcoming invoice, you are simply viewing a preview – the
* invoice has not yet been created. As such, the upcoming invoice will not show up in invoice
* listing calls, and you cannot use the API to pay or edit the invoice. If you want to change the
* amount that your customer will be billed, you can add, remove, or update pending invoice items,
* or update the customer’s discount.
*
*
You can preview the effects of updating a subscription, including a preview of what
* proration will take place. To ensure that the actual proration is calculated exactly the same
* as the previewed proration, you should pass a proration_date
parameter when doing
* the actual subscription update. The value passed in should be the same as the
* subscription_proration_date
returned on the upcoming invoice resource. The recommended
* way to get only the prorations being previewed is to consider only proration line items where
* period[start]
is equal to the subscription_proration_date
on the
* upcoming invoice resource.
*/
public static Invoice upcoming() throws StripeException {
return upcoming((Map) null, (RequestOptions) null);
}
/**
* At any time, you can preview the upcoming invoice for a customer. This will show you all the
* charges that are pending, including subscription renewal charges, invoice item charges, etc. It
* will also show you any discount that is applicable to the customer.
*
* Note that when you are viewing an upcoming invoice, you are simply viewing a preview – the
* invoice has not yet been created. As such, the upcoming invoice will not show up in invoice
* listing calls, and you cannot use the API to pay or edit the invoice. If you want to change the
* amount that your customer will be billed, you can add, remove, or update pending invoice items,
* or update the customer’s discount.
*
*
You can preview the effects of updating a subscription, including a preview of what
* proration will take place. To ensure that the actual proration is calculated exactly the same
* as the previewed proration, you should pass a proration_date
parameter when doing
* the actual subscription update. The value passed in should be the same as the
* subscription_proration_date
returned on the upcoming invoice resource. The recommended
* way to get only the prorations being previewed is to consider only proration line items where
* period[start]
is equal to the subscription_proration_date
on the
* upcoming invoice resource.
*/
public static Invoice upcoming(Map params) throws StripeException {
return upcoming(params, (RequestOptions) null);
}
/**
* At any time, you can preview the upcoming invoice for a customer. This will show you all the
* charges that are pending, including subscription renewal charges, invoice item charges, etc. It
* will also show you any discount that is applicable to the customer.
*
* Note that when you are viewing an upcoming invoice, you are simply viewing a preview – the
* invoice has not yet been created. As such, the upcoming invoice will not show up in invoice
* listing calls, and you cannot use the API to pay or edit the invoice. If you want to change the
* amount that your customer will be billed, you can add, remove, or update pending invoice items,
* or update the customer’s discount.
*
*
You can preview the effects of updating a subscription, including a preview of what
* proration will take place. To ensure that the actual proration is calculated exactly the same
* as the previewed proration, you should pass a proration_date
parameter when doing
* the actual subscription update. The value passed in should be the same as the
* subscription_proration_date
returned on the upcoming invoice resource. The recommended
* way to get only the prorations being previewed is to consider only proration line items where
* period[start]
is equal to the subscription_proration_date
on the
* upcoming invoice resource.
*/
public static Invoice upcoming(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/invoices/upcoming");
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Invoice.class, options);
}
/**
* At any time, you can preview the upcoming invoice for a customer. This will show you all the
* charges that are pending, including subscription renewal charges, invoice item charges, etc. It
* will also show you any discount that is applicable to the customer.
*
* Note that when you are viewing an upcoming invoice, you are simply viewing a preview – the
* invoice has not yet been created. As such, the upcoming invoice will not show up in invoice
* listing calls, and you cannot use the API to pay or edit the invoice. If you want to change the
* amount that your customer will be billed, you can add, remove, or update pending invoice items,
* or update the customer’s discount.
*
*
You can preview the effects of updating a subscription, including a preview of what
* proration will take place. To ensure that the actual proration is calculated exactly the same
* as the previewed proration, you should pass a proration_date
parameter when doing
* the actual subscription update. The value passed in should be the same as the
* subscription_proration_date
returned on the upcoming invoice resource. The recommended
* way to get only the prorations being previewed is to consider only proration line items where
* period[start]
is equal to the subscription_proration_date
on the
* upcoming invoice resource.
*/
public static Invoice upcoming(InvoiceUpcomingParams params) throws StripeException {
return upcoming(params, (RequestOptions) null);
}
/**
* At any time, you can preview the upcoming invoice for a customer. This will show you all the
* charges that are pending, including subscription renewal charges, invoice item charges, etc. It
* will also show you any discount that is applicable to the customer.
*
*
Note that when you are viewing an upcoming invoice, you are simply viewing a preview – the
* invoice has not yet been created. As such, the upcoming invoice will not show up in invoice
* listing calls, and you cannot use the API to pay or edit the invoice. If you want to change the
* amount that your customer will be billed, you can add, remove, or update pending invoice items,
* or update the customer’s discount.
*
*
You can preview the effects of updating a subscription, including a preview of what
* proration will take place. To ensure that the actual proration is calculated exactly the same
* as the previewed proration, you should pass a proration_date
parameter when doing
* the actual subscription update. The value passed in should be the same as the
* subscription_proration_date
returned on the upcoming invoice resource. The recommended
* way to get only the prorations being previewed is to consider only proration line items where
* period[start]
is equal to the subscription_proration_date
on the
* upcoming invoice resource.
*/
public static Invoice upcoming(InvoiceUpcomingParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/invoices/upcoming");
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Invoice.class, options);
}
/**
* This endpoint creates a draft invoice for a given customer. The draft invoice created pulls in
* all pending invoice items on that customer, including prorations.
*/
public static Invoice create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* This endpoint creates a draft invoice for a given customer. The draft invoice created pulls in
* all pending invoice items on that customer, including prorations.
*/
public static Invoice create(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/invoices");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Invoice.class, options);
}
/**
* This endpoint creates a draft invoice for a given customer. The draft invoice created pulls in
* all pending invoice items on that customer, including prorations.
*/
public static Invoice create(InvoiceCreateParams params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* This endpoint creates a draft invoice for a given customer. The draft invoice created pulls in
* all pending invoice items on that customer, including prorations.
*/
public static Invoice create(InvoiceCreateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/invoices");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Invoice.class, options);
}
/**
* Retrieves the invoice with the given ID.
*/
public static Invoice retrieve(String invoice) throws StripeException {
return retrieve(invoice, (Map) null, (RequestOptions) null);
}
/**
* Retrieves the invoice with the given ID.
*/
public static Invoice retrieve(String invoice, RequestOptions options) throws StripeException {
return retrieve(invoice, (Map) null, options);
}
/**
* Retrieves the invoice with the given ID.
*/
public static Invoice retrieve(String invoice, Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/invoices/%s", ApiResource.urlEncodeId(invoice)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Invoice.class, options);
}
/**
* Retrieves the invoice with the given ID.
*/
public static Invoice retrieve(String invoice, InvoiceRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/invoices/%s", ApiResource.urlEncodeId(invoice)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Invoice.class, options);
}
/**
* Draft invoices are fully editable. Once an invoice is finalized, monetary values, as well as
* collection_method
, become uneditable.
*
* If you would like to stop the Stripe Billing engine from automatically finalizing,
* reattempting payments on, sending reminders for, or automatically reconciling invoices, pass
* auto_advance=false
.
*/
@Override
public Invoice update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Draft invoices are fully editable. Once an invoice is finalized, monetary values, as well as
* collection_method
, become uneditable.
*
* If you would like to stop the Stripe Billing engine from automatically finalizing,
* reattempting payments on, sending reminders for, or automatically reconciling invoices, pass
* auto_advance=false
.
*/
@Override
public Invoice update(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/invoices/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Invoice.class, options);
}
/**
* Draft invoices are fully editable. Once an invoice is finalized, monetary values, as well as
* collection_method
, become uneditable.
*
* If you would like to stop the Stripe Billing engine from automatically finalizing,
* reattempting payments on, sending reminders for, or automatically reconciling invoices, pass
* auto_advance=false
.
*/
public Invoice update(InvoiceUpdateParams params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Draft invoices are fully editable. Once an invoice is finalized, monetary values, as well as
* collection_method
, become uneditable.
*
*
If you would like to stop the Stripe Billing engine from automatically finalizing,
* reattempting payments on, sending reminders for, or automatically reconciling invoices, pass
* auto_advance=false
.
*/
public Invoice update(InvoiceUpdateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/invoices/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Invoice.class, options);
}
/**
* Permanently deletes a draft invoice. This cannot be undone. Attempts to delete invoices that
* are no longer in a draft state will fail; once an invoice has been finalized, it must be voided.
*/
public Invoice delete() throws StripeException {
return delete((Map) null, (RequestOptions) null);
}
/**
* Permanently deletes a draft invoice. This cannot be undone. Attempts to delete invoices that
* are no longer in a draft state will fail; once an invoice has been finalized, it must be voided.
*/
public Invoice delete(RequestOptions options) throws StripeException {
return delete((Map) null, options);
}
/**
* Permanently deletes a draft invoice. This cannot be undone. Attempts to delete invoices that
* are no longer in a draft state will fail; once an invoice has been finalized, it must be voided.
*/
public Invoice delete(Map params) throws StripeException {
return delete(params, (RequestOptions) null);
}
/**
* Permanently deletes a draft invoice. This cannot be undone. Attempts to delete invoices that
* are no longer in a draft state will fail; once an invoice has been finalized, it must be voided.
*/
public Invoice delete(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/invoices/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.DELETE, url, params, Invoice.class, options);
}
/**
* Stripe automatically creates and then attempts to collect payment on invoices for customers on
* subscriptions according to your subscriptions settings.
* However, if you’d like to attempt payment on an invoice out of the normal collection schedule
* or for some other reason, you can do so.
*/
public Invoice pay() throws StripeException {
return pay((Map) null, (RequestOptions) null);
}
/**
* Stripe automatically creates and then attempts to collect payment on invoices for customers on
* subscriptions according to your subscriptions settings.
* However, if you’d like to attempt payment on an invoice out of the normal collection schedule
* or for some other reason, you can do so.
*/
public Invoice pay(RequestOptions options) throws StripeException {
return pay((Map) null, options);
}
/**
* Stripe automatically creates and then attempts to collect payment on invoices for customers on
* subscriptions according to your subscriptions settings.
* However, if you’d like to attempt payment on an invoice out of the normal collection schedule
* or for some other reason, you can do so.
*/
public Invoice pay(Map params) throws StripeException {
return pay(params, (RequestOptions) null);
}
/**
* Stripe automatically creates and then attempts to collect payment on invoices for customers on
* subscriptions according to your subscriptions settings.
* However, if you’d like to attempt payment on an invoice out of the normal collection schedule
* or for some other reason, you can do so.
*/
public Invoice pay(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/invoices/%s/pay", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Invoice.class, options);
}
/**
* Stripe automatically creates and then attempts to collect payment on invoices for customers on
* subscriptions according to your subscriptions settings.
* However, if you’d like to attempt payment on an invoice out of the normal collection schedule
* or for some other reason, you can do so.
*/
public Invoice pay(InvoicePayParams params) throws StripeException {
return pay(params, (RequestOptions) null);
}
/**
* Stripe automatically creates and then attempts to collect payment on invoices for customers on
* subscriptions according to your subscriptions settings.
* However, if you’d like to attempt payment on an invoice out of the normal collection schedule
* or for some other reason, you can do so.
*/
public Invoice pay(InvoicePayParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/invoices/%s/pay", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Invoice.class, options);
}
/**
* Stripe automatically finalizes drafts before sending and attempting payment on invoices.
* However, if you’d like to finalize a draft invoice manually, you can do so using this method.
*/
public Invoice finalizeInvoice() throws StripeException {
return finalizeInvoice((Map) null, (RequestOptions) null);
}
/**
* Stripe automatically finalizes drafts before sending and attempting payment on invoices.
* However, if you’d like to finalize a draft invoice manually, you can do so using this method.
*/
public Invoice finalizeInvoice(RequestOptions options) throws StripeException {
return finalizeInvoice((Map) null, options);
}
/**
* Stripe automatically finalizes drafts before sending and attempting payment on invoices.
* However, if you’d like to finalize a draft invoice manually, you can do so using this method.
*/
public Invoice finalizeInvoice(Map params) throws StripeException {
return finalizeInvoice(params, (RequestOptions) null);
}
/**
* Stripe automatically finalizes drafts before sending and attempting payment on invoices.
* However, if you’d like to finalize a draft invoice manually, you can do so using this method.
*/
public Invoice finalizeInvoice(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/invoices/%s/finalize", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Invoice.class, options);
}
/**
* Stripe automatically finalizes drafts before sending and attempting payment on invoices.
* However, if you’d like to finalize a draft invoice manually, you can do so using this method.
*/
public Invoice finalizeInvoice(InvoiceFinalizeInvoiceParams params) throws StripeException {
return finalizeInvoice(params, (RequestOptions) null);
}
/**
* Stripe automatically finalizes drafts before sending and attempting payment on invoices.
* However, if you’d like to finalize a draft invoice manually, you can do so using this method.
*/
public Invoice finalizeInvoice(InvoiceFinalizeInvoiceParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/invoices/%s/finalize", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Invoice.class, options);
}
/**
* Stripe will automatically send invoices to customers according to your subscriptions settings.
* However, if you’d like to manually send an invoice to your customer out of the normal schedule,
* you can do so. When sending invoices that have already been paid, there will be no reference to
* the payment in the email.
*
* Requests made in test-mode result in no emails being sent, despite sending an
* invoice.sent
event.
*/
public Invoice sendInvoice() throws StripeException {
return sendInvoice((Map) null, (RequestOptions) null);
}
/**
* Stripe will automatically send invoices to customers according to your subscriptions settings.
* However, if you’d like to manually send an invoice to your customer out of the normal schedule,
* you can do so. When sending invoices that have already been paid, there will be no reference to
* the payment in the email.
*
* Requests made in test-mode result in no emails being sent, despite sending an
* invoice.sent
event.
*/
public Invoice sendInvoice(RequestOptions options) throws StripeException {
return sendInvoice((Map) null, options);
}
/**
* Stripe will automatically send invoices to customers according to your subscriptions settings.
* However, if you’d like to manually send an invoice to your customer out of the normal schedule,
* you can do so. When sending invoices that have already been paid, there will be no reference to
* the payment in the email.
*
* Requests made in test-mode result in no emails being sent, despite sending an
* invoice.sent
event.
*/
public Invoice sendInvoice(Map params) throws StripeException {
return sendInvoice(params, (RequestOptions) null);
}
/**
* Stripe will automatically send invoices to customers according to your subscriptions settings.
* However, if you’d like to manually send an invoice to your customer out of the normal schedule,
* you can do so. When sending invoices that have already been paid, there will be no reference to
* the payment in the email.
*
* Requests made in test-mode result in no emails being sent, despite sending an
* invoice.sent
event.
*/
public Invoice sendInvoice(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/invoices/%s/send", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Invoice.class, options);
}
/**
* Stripe will automatically send invoices to customers according to your subscriptions settings.
* However, if you’d like to manually send an invoice to your customer out of the normal schedule,
* you can do so. When sending invoices that have already been paid, there will be no reference to
* the payment in the email.
*
* Requests made in test-mode result in no emails being sent, despite sending an
* invoice.sent
event.
*/
public Invoice sendInvoice(InvoiceSendInvoiceParams params) throws StripeException {
return sendInvoice(params, (RequestOptions) null);
}
/**
* Stripe will automatically send invoices to customers according to your subscriptions settings.
* However, if you’d like to manually send an invoice to your customer out of the normal schedule,
* you can do so. When sending invoices that have already been paid, there will be no reference to
* the payment in the email.
*
*
Requests made in test-mode result in no emails being sent, despite sending an
* invoice.sent
event.
*/
public Invoice sendInvoice(InvoiceSendInvoiceParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/invoices/%s/send", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Invoice.class, options);
}
/**
* Marking an invoice as uncollectible is useful for keeping track of bad debts that can be
* written off for accounting purposes.
*/
public Invoice markUncollectible() throws StripeException {
return markUncollectible((Map) null, (RequestOptions) null);
}
/**
* Marking an invoice as uncollectible is useful for keeping track of bad debts that can be
* written off for accounting purposes.
*/
public Invoice markUncollectible(RequestOptions options) throws StripeException {
return markUncollectible((Map) null, options);
}
/**
* Marking an invoice as uncollectible is useful for keeping track of bad debts that can be
* written off for accounting purposes.
*/
public Invoice markUncollectible(Map params) throws StripeException {
return markUncollectible(params, (RequestOptions) null);
}
/**
* Marking an invoice as uncollectible is useful for keeping track of bad debts that can be
* written off for accounting purposes.
*/
public Invoice markUncollectible(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/invoices/%s/mark_uncollectible", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Invoice.class, options);
}
/**
* Marking an invoice as uncollectible is useful for keeping track of bad debts that can be
* written off for accounting purposes.
*/
public Invoice markUncollectible(InvoiceMarkUncollectibleParams params) throws StripeException {
return markUncollectible(params, (RequestOptions) null);
}
/**
* Marking an invoice as uncollectible is useful for keeping track of bad debts that can be
* written off for accounting purposes.
*/
public Invoice markUncollectible(InvoiceMarkUncollectibleParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/invoices/%s/mark_uncollectible", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Invoice.class, options);
}
/**
* Mark a finalized invoice as void. This cannot be undone. Voiding an invoice is similar to deletion, however it only applies to finalized invoices and
* maintains a papertrail where the invoice can still be found.
*/
public Invoice voidInvoice() throws StripeException {
return voidInvoice((Map) null, (RequestOptions) null);
}
/**
* Mark a finalized invoice as void. This cannot be undone. Voiding an invoice is similar to deletion, however it only applies to finalized invoices and
* maintains a papertrail where the invoice can still be found.
*/
public Invoice voidInvoice(RequestOptions options) throws StripeException {
return voidInvoice((Map) null, options);
}
/**
* Mark a finalized invoice as void. This cannot be undone. Voiding an invoice is similar to deletion, however it only applies to finalized invoices and
* maintains a papertrail where the invoice can still be found.
*/
public Invoice voidInvoice(Map params) throws StripeException {
return voidInvoice(params, (RequestOptions) null);
}
/**
* Mark a finalized invoice as void. This cannot be undone. Voiding an invoice is similar to deletion, however it only applies to finalized invoices and
* maintains a papertrail where the invoice can still be found.
*/
public Invoice voidInvoice(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/invoices/%s/void", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Invoice.class, options);
}
/**
* Mark a finalized invoice as void. This cannot be undone. Voiding an invoice is similar to deletion, however it only applies to finalized invoices and
* maintains a papertrail where the invoice can still be found.
*/
public Invoice voidInvoice(InvoiceVoidInvoiceParams params) throws StripeException {
return voidInvoice(params, (RequestOptions) null);
}
/**
* Mark a finalized invoice as void. This cannot be undone. Voiding an invoice is similar to deletion, however it only applies to finalized invoices and
* maintains a papertrail where the invoice can still be found.
*/
public Invoice voidInvoice(InvoiceVoidInvoiceParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/invoices/%s/void", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Invoice.class, options);
}
public static class CustomField extends StripeObject {
/**
* The name of the custom field.
*/
@SerializedName("name")
String name;
/**
* The value of the custom field.
*/
@SerializedName("value")
String value;
/**
* The name of the custom field.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getName() {
return this.name;
}
/**
* The value of the custom field.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
/**
* The name of the custom field.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setName(final String name) {
this.name = name;
}
/**
* The value of the custom field.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setValue(final String value) {
this.value = value;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Invoice.CustomField)) return false;
final Invoice.CustomField other = (Invoice.CustomField) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$value = this.getValue();
final java.lang.Object other$value = other.getValue();
if (this$value == null ? other$value != null : !this$value.equals(other$value)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Invoice.CustomField;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $value = this.getValue();
result = result * PRIME + ($value == null ? 43 : $value.hashCode());
return result;
}
}
public static class CustomerTaxId extends StripeObject {
/**
* The type of the tax ID, one of `au_abn`, `ch_vat`, `eu_vat`, `in_gst`, `mx_rfc`, `no_vat`,
* `nz_gst`, `unknown`, or `za_vat`.
*/
@SerializedName("type")
String type;
/**
* The value of the tax ID.
*/
@SerializedName("value")
String value;
/**
* The type of the tax ID, one of `au_abn`, `ch_vat`, `eu_vat`, `in_gst`, `mx_rfc`, `no_vat`,
* `nz_gst`, `unknown`, or `za_vat`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
/**
* The value of the tax ID.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
/**
* The type of the tax ID, one of `au_abn`, `ch_vat`, `eu_vat`, `in_gst`, `mx_rfc`, `no_vat`,
* `nz_gst`, `unknown`, or `za_vat`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
/**
* The value of the tax ID.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setValue(final String value) {
this.value = value;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Invoice.CustomerTaxId)) return false;
final Invoice.CustomerTaxId other = (Invoice.CustomerTaxId) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final java.lang.Object this$value = this.getValue();
final java.lang.Object other$value = other.getValue();
if (this$value == null ? other$value != null : !this$value.equals(other$value)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Invoice.CustomerTaxId;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final java.lang.Object $value = this.getValue();
result = result * PRIME + ($value == null ? 43 : $value.hashCode());
return result;
}
}
public static class StatusTransitions extends StripeObject {
/**
* The time that the invoice draft was finalized.
*/
@SerializedName("finalized_at")
Long finalizedAt;
/**
* The time that the invoice was marked uncollectible.
*/
@SerializedName("marked_uncollectible_at")
Long markedUncollectibleAt;
/**
* The time that the invoice was paid.
*/
@SerializedName("paid_at")
Long paidAt;
/**
* The time that the invoice was voided.
*/
@SerializedName("voided_at")
Long voidedAt;
/**
* The time that the invoice draft was finalized.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getFinalizedAt() {
return this.finalizedAt;
}
/**
* The time that the invoice was marked uncollectible.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getMarkedUncollectibleAt() {
return this.markedUncollectibleAt;
}
/**
* The time that the invoice was paid.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getPaidAt() {
return this.paidAt;
}
/**
* The time that the invoice was voided.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getVoidedAt() {
return this.voidedAt;
}
/**
* The time that the invoice draft was finalized.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFinalizedAt(final Long finalizedAt) {
this.finalizedAt = finalizedAt;
}
/**
* The time that the invoice was marked uncollectible.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMarkedUncollectibleAt(final Long markedUncollectibleAt) {
this.markedUncollectibleAt = markedUncollectibleAt;
}
/**
* The time that the invoice was paid.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPaidAt(final Long paidAt) {
this.paidAt = paidAt;
}
/**
* The time that the invoice was voided.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVoidedAt(final Long voidedAt) {
this.voidedAt = voidedAt;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Invoice.StatusTransitions)) return false;
final Invoice.StatusTransitions other = (Invoice.StatusTransitions) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$finalizedAt = this.getFinalizedAt();
final java.lang.Object other$finalizedAt = other.getFinalizedAt();
if (this$finalizedAt == null ? other$finalizedAt != null : !this$finalizedAt.equals(other$finalizedAt)) return false;
final java.lang.Object this$markedUncollectibleAt = this.getMarkedUncollectibleAt();
final java.lang.Object other$markedUncollectibleAt = other.getMarkedUncollectibleAt();
if (this$markedUncollectibleAt == null ? other$markedUncollectibleAt != null : !this$markedUncollectibleAt.equals(other$markedUncollectibleAt)) return false;
final java.lang.Object this$paidAt = this.getPaidAt();
final java.lang.Object other$paidAt = other.getPaidAt();
if (this$paidAt == null ? other$paidAt != null : !this$paidAt.equals(other$paidAt)) return false;
final java.lang.Object this$voidedAt = this.getVoidedAt();
final java.lang.Object other$voidedAt = other.getVoidedAt();
if (this$voidedAt == null ? other$voidedAt != null : !this$voidedAt.equals(other$voidedAt)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Invoice.StatusTransitions;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $finalizedAt = this.getFinalizedAt();
result = result * PRIME + ($finalizedAt == null ? 43 : $finalizedAt.hashCode());
final java.lang.Object $markedUncollectibleAt = this.getMarkedUncollectibleAt();
result = result * PRIME + ($markedUncollectibleAt == null ? 43 : $markedUncollectibleAt.hashCode());
final java.lang.Object $paidAt = this.getPaidAt();
result = result * PRIME + ($paidAt == null ? 43 : $paidAt.hashCode());
final java.lang.Object $voidedAt = this.getVoidedAt();
result = result * PRIME + ($voidedAt == null ? 43 : $voidedAt.hashCode());
return result;
}
}
public static class TaxAmount extends StripeObject {
/**
* The amount, in %s, of the tax.
*/
@SerializedName("amount")
Long amount;
/**
* Whether this tax amount is inclusive or exclusive.
*/
@SerializedName("inclusive")
Boolean inclusive;
/**
* The tax rate that was applied to get this tax amount.
*/
@SerializedName("tax_rate")
ExpandableField taxRate;
/**
* Get id of expandable `taxRate` object.
*/
public String getTaxRate() {
return (this.taxRate != null) ? this.taxRate.getId() : null;
}
public void setTaxRate(String id) {
this.taxRate = ApiResource.setExpandableFieldId(id, this.taxRate);
}
/**
* Get expanded `taxRate`.
*/
public TaxRate getTaxRateObject() {
return (this.taxRate != null) ? this.taxRate.getExpanded() : null;
}
public void setTaxRateObject(TaxRate expandableObject) {
this.taxRate = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* The amount, in %s, of the tax.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
/**
* Whether this tax amount is inclusive or exclusive.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getInclusive() {
return this.inclusive;
}
/**
* The amount, in %s, of the tax.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmount(final Long amount) {
this.amount = amount;
}
/**
* Whether this tax amount is inclusive or exclusive.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setInclusive(final Boolean inclusive) {
this.inclusive = inclusive;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Invoice.TaxAmount)) return false;
final Invoice.TaxAmount other = (Invoice.TaxAmount) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$inclusive = this.getInclusive();
final java.lang.Object other$inclusive = other.getInclusive();
if (this$inclusive == null ? other$inclusive != null : !this$inclusive.equals(other$inclusive)) return false;
final java.lang.Object this$taxRate = this.getTaxRate();
final java.lang.Object other$taxRate = other.getTaxRate();
if (this$taxRate == null ? other$taxRate != null : !this$taxRate.equals(other$taxRate)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Invoice.TaxAmount;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $inclusive = this.getInclusive();
result = result * PRIME + ($inclusive == null ? 43 : $inclusive.hashCode());
final java.lang.Object $taxRate = this.getTaxRate();
result = result * PRIME + ($taxRate == null ? 43 : $taxRate.hashCode());
return result;
}
}
public static class ThresholdItemReason extends StripeObject {
/**
* The IDs of the line items that triggered the threshold invoice.
*/
@SerializedName("line_item_ids")
List lineItemIds;
/**
* The quantity threshold boundary that applied to the given line item.
*/
@SerializedName("usage_gte")
Long usageGte;
/**
* The IDs of the line items that triggered the threshold invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getLineItemIds() {
return this.lineItemIds;
}
/**
* The quantity threshold boundary that applied to the given line item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getUsageGte() {
return this.usageGte;
}
/**
* The IDs of the line items that triggered the threshold invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLineItemIds(final List lineItemIds) {
this.lineItemIds = lineItemIds;
}
/**
* The quantity threshold boundary that applied to the given line item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsageGte(final Long usageGte) {
this.usageGte = usageGte;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Invoice.ThresholdItemReason)) return false;
final Invoice.ThresholdItemReason other = (Invoice.ThresholdItemReason) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$lineItemIds = this.getLineItemIds();
final java.lang.Object other$lineItemIds = other.getLineItemIds();
if (this$lineItemIds == null ? other$lineItemIds != null : !this$lineItemIds.equals(other$lineItemIds)) return false;
final java.lang.Object this$usageGte = this.getUsageGte();
final java.lang.Object other$usageGte = other.getUsageGte();
if (this$usageGte == null ? other$usageGte != null : !this$usageGte.equals(other$usageGte)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Invoice.ThresholdItemReason;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $lineItemIds = this.getLineItemIds();
result = result * PRIME + ($lineItemIds == null ? 43 : $lineItemIds.hashCode());
final java.lang.Object $usageGte = this.getUsageGte();
result = result * PRIME + ($usageGte == null ? 43 : $usageGte.hashCode());
return result;
}
}
public static class ThresholdReason extends StripeObject {
/**
* The total invoice amount threshold boundary if it triggered the threshold invoice.
*/
@SerializedName("amount_gte")
Long amountGte;
/**
* Indicates which line items triggered a threshold invoice.
*/
@SerializedName("item_reasons")
List itemReasons;
/**
* The total invoice amount threshold boundary if it triggered the threshold invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountGte() {
return this.amountGte;
}
/**
* Indicates which line items triggered a threshold invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getItemReasons() {
return this.itemReasons;
}
/**
* The total invoice amount threshold boundary if it triggered the threshold invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountGte(final Long amountGte) {
this.amountGte = amountGte;
}
/**
* Indicates which line items triggered a threshold invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setItemReasons(final List itemReasons) {
this.itemReasons = itemReasons;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Invoice.ThresholdReason)) return false;
final Invoice.ThresholdReason other = (Invoice.ThresholdReason) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amountGte = this.getAmountGte();
final java.lang.Object other$amountGte = other.getAmountGte();
if (this$amountGte == null ? other$amountGte != null : !this$amountGte.equals(other$amountGte)) return false;
final java.lang.Object this$itemReasons = this.getItemReasons();
final java.lang.Object other$itemReasons = other.getItemReasons();
if (this$itemReasons == null ? other$itemReasons != null : !this$itemReasons.equals(other$itemReasons)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Invoice.ThresholdReason;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amountGte = this.getAmountGte();
result = result * PRIME + ($amountGte == null ? 43 : $amountGte.hashCode());
final java.lang.Object $itemReasons = this.getItemReasons();
result = result * PRIME + ($itemReasons == null ? 43 : $itemReasons.hashCode());
return result;
}
}
public static class TransferData extends StripeObject {
/**
* The account (if any) where funds from the payment will be transferred to upon payment
* success.
*/
@SerializedName("destination")
ExpandableField destination;
/** Get id of expandable `destination` object. */
public String getDestination() {
return (this.destination != null) ? this.destination.getId() : null;
}
public void setDestination(String id) {
this.destination = ApiResource.setExpandableFieldId(id, this.destination);
}
/** Get expanded `destination`. */
public Account getDestinationObject() {
return (this.destination != null) ? this.destination.getExpanded() : null;
}
public void setDestinationObject(Account expandableObject) {
this.destination = new ExpandableField(expandableObject.getId(), expandableObject);
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Invoice.TransferData)) return false;
final Invoice.TransferData other = (Invoice.TransferData) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$destination = this.getDestination();
final java.lang.Object other$destination = other.getDestination();
if (this$destination == null ? other$destination != null : !this$destination.equals(other$destination)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Invoice.TransferData;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $destination = this.getDestination();
result = result * PRIME + ($destination == null ? 43 : $destination.hashCode());
return result;
}
}
/**
* The country of the business associated with this invoice, most often the business creating the
* invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAccountCountry() {
return this.accountCountry;
}
/**
* The public name of the business associated with this invoice, most often the business creating
* the invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAccountName() {
return this.accountName;
}
/**
* Final amount due at this time for this invoice. If the invoice's total is smaller than the
* minimum charge amount, for example, or if there is account credit that can be applied to the
* invoice, the `amount_due` may be 0. If there is a positive `starting_balance` for the invoice
* (the customer owes money), the `amount_due` will also take that into account. The charge that
* gets generated for the invoice will be for the amount specified in `amount_due`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountDue() {
return this.amountDue;
}
/**
* The amount, in %s, that was paid.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountPaid() {
return this.amountPaid;
}
/**
* The amount remaining, in %s, that is due.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountRemaining() {
return this.amountRemaining;
}
/**
* The fee in %s that will be applied to the invoice and transferred to the application owner's
* Stripe account when the invoice is paid.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getApplicationFeeAmount() {
return this.applicationFeeAmount;
}
/**
* Number of payment attempts made for this invoice, from the perspective of the payment retry
* schedule. Any payment attempt counts as the first attempt, and subsequently only automatic
* retries increment the attempt count. In other words, manual payment attempts after the first
* attempt do not affect the retry schedule.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAttemptCount() {
return this.attemptCount;
}
/**
* Whether an attempt has been made to pay the invoice. An invoice is not attempted until 1 hour
* after the `invoice.created` webhook, for example, so you might not want to display that invoice
* as unpaid to your users.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getAttempted() {
return this.attempted;
}
/**
* Controls whether Stripe will perform [automatic
* collection](https://stripe.com/docs/billing/invoices/workflow/#auto_advance) of the invoice.
* When `false`, the invoice's state will not automatically advance without an explicit action.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getAutoAdvance() {
return this.autoAdvance;
}
/**
* Indicates the reason why the invoice was created. `subscription_cycle` indicates an invoice
* created by a subscription advancing into a new period. `subscription_create` indicates an
* invoice created due to creating a subscription. `subscription_update` indicates an invoice
* created due to updating a subscription. `subscription` is set for all old invoices to indicate
* either a change to a subscription or a period advancement. `manual` is set for all invoices
* unrelated to a subscription (for example: created via the invoice editor). The `upcoming` value
* is reserved for simulated invoices per the upcoming invoice endpoint. `subscription_threshold`
* indicates an invoice created due to a billing threshold being reached.
*
* One of `automatic_pending_invoice_item_invoice`, `manual`, `subscription`,
* `subscription_create`, `subscription_cycle`, `subscription_threshold`, `subscription_update`,
* or `upcoming`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBillingReason() {
return this.billingReason;
}
/**
* Either `charge_automatically`, or `send_invoice`. When charging automatically, Stripe will
* attempt to pay this invoice using the default source attached to the customer. When sending an
* invoice, Stripe will email this invoice to the customer with payment instructions.
*
*
One of `charge_automatically`, or `send_invoice`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCollectionMethod() {
return this.collectionMethod;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
/**
* Three-letter [ISO currency code](https://www.iso.org/iso-4217-currency-codes.html), in
* lowercase. Must be a [supported currency](https://stripe.com/docs/currencies).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
/**
* Custom fields displayed on the invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getCustomFields() {
return this.customFields;
}
/**
* The customer's address. Until the invoice is finalized, this field will equal
* `customer.address`. Once the invoice is finalized, this field will no longer be updated.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Address getCustomerAddress() {
return this.customerAddress;
}
/**
* The customer's email. Until the invoice is finalized, this field will equal `customer.email`.
* Once the invoice is finalized, this field will no longer be updated.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCustomerEmail() {
return this.customerEmail;
}
/**
* The customer's name. Until the invoice is finalized, this field will equal `customer.name`.
* Once the invoice is finalized, this field will no longer be updated.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCustomerName() {
return this.customerName;
}
/**
* The customer's phone number. Until the invoice is finalized, this field will equal
* `customer.phone`. Once the invoice is finalized, this field will no longer be updated.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCustomerPhone() {
return this.customerPhone;
}
/**
* The customer's shipping information. Until the invoice is finalized, this field will equal
* `customer.shipping`. Once the invoice is finalized, this field will no longer be updated.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ShippingDetails getCustomerShipping() {
return this.customerShipping;
}
/**
* The customer's tax exempt status. Until the invoice is finalized, this field will equal
* `customer.tax_exempt`. Once the invoice is finalized, this field will no longer be updated.
*
* One of `exempt`, `none`, or `reverse`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCustomerTaxExempt() {
return this.customerTaxExempt;
}
/**
* The customer's tax IDs. Until the invoice is finalized, this field will contain the same tax
* IDs as `customer.tax_ids`. Once the invoice is finalized, this field will no longer be updated.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getCustomerTaxIds() {
return this.customerTaxIds;
}
/**
* The tax rates applied to this invoice, if any.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getDefaultTaxRates() {
return this.defaultTaxRates;
}
/**
* Always true for a deleted object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getDeleted() {
return this.deleted;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users. Referenced as
* 'memo' in the Dashboard.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDescription() {
return this.description;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Discount getDiscount() {
return this.discount;
}
/**
* The date on which payment for this invoice is due. This value will be `null` for invoices where
* `collection_method=charge_automatically`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDueDate() {
return this.dueDate;
}
/**
* Ending customer balance after the invoice is finalized. Invoices are finalized approximately an
* hour after successful webhook delivery or when payment collection is attempted for the invoice.
* If the invoice has not been finalized yet, this will be null.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getEndingBalance() {
return this.endingBalance;
}
/**
* Footer displayed on the invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFooter() {
return this.footer;
}
/**
* The URL for the hosted invoice page, which allows customers to view and pay an invoice. If the
* invoice has not been finalized yet, this will be null.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getHostedInvoiceUrl() {
return this.hostedInvoiceUrl;
}
/**
* The link to download the PDF for the invoice. If the invoice has not been finalized yet, this
* will be null.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getInvoicePdf() {
return this.invoicePdf;
}
/**
* The individual line items that make up the invoice. `lines` is sorted as follows: invoice items
* in reverse chronological order, followed by the subscription, if any.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public InvoiceLineItemCollection getLines() {
return this.lines;
}
/**
* Has the value `true` if the object exists in live mode or the value `false` if the object
* exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* The time at which payment will next be attempted. This value will be `null` for invoices where
* `collection_method=send_invoice`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getNextPaymentAttempt() {
return this.nextPaymentAttempt;
}
/**
* A unique, identifying string that appears on emails sent to the customer for this invoice. This
* starts with the customer's unique invoice_prefix if it is specified.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getNumber() {
return this.number;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to `invoice`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* Whether payment was successfully collected for this invoice. An invoice can be paid (most
* commonly) with a charge or with credit from the customer's account balance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getPaid() {
return this.paid;
}
/**
* End of the usage period during which invoice items were added to this invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getPeriodEnd() {
return this.periodEnd;
}
/**
* Start of the usage period during which invoice items were added to this invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getPeriodStart() {
return this.periodStart;
}
/**
* Total amount of all post-payment credit notes issued for this invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getPostPaymentCreditNotesAmount() {
return this.postPaymentCreditNotesAmount;
}
/**
* Total amount of all pre-payment credit notes issued for this invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getPrePaymentCreditNotesAmount() {
return this.prePaymentCreditNotesAmount;
}
/**
* This is the transaction number that appears on email receipts sent for this invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReceiptNumber() {
return this.receiptNumber;
}
/**
* Starting customer balance before the invoice is finalized. If the invoice has not been
* finalized yet, this will be the current customer balance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getStartingBalance() {
return this.startingBalance;
}
/**
* Extra information about an invoice for the customer's credit card statement.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatementDescriptor() {
return this.statementDescriptor;
}
/**
* The status of the invoice, one of `draft`, `open`, `paid`, `uncollectible`, or `void`. [Learn
* more](https://stripe.com/docs/billing/invoices/workflow#workflow-overview)
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public StatusTransitions getStatusTransitions() {
return this.statusTransitions;
}
/**
* Only set for upcoming invoices that preview prorations. The time used to calculate prorations.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getSubscriptionProrationDate() {
return this.subscriptionProrationDate;
}
/**
* Total of all subscriptions, invoice items, and prorations on the invoice before any discount or
* tax is applied.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getSubtotal() {
return this.subtotal;
}
/**
* The amount of tax on this invoice. This is the sum of all the tax amounts on this invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getTax() {
return this.tax;
}
/**
* This percentage of the subtotal has been added to the total amount of the invoice, including
* invoice line items and discounts. This field is inherited from the subscription's `tax_percent`
* field, but can be changed before the invoice is paid. This field defaults to null.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BigDecimal getTaxPercent() {
return this.taxPercent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ThresholdReason getThresholdReason() {
return this.thresholdReason;
}
/**
* Total after discounts and taxes.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getTotal() {
return this.total;
}
/**
* The aggregate amounts calculated per tax rate for all line items.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getTotalTaxAmounts() {
return this.totalTaxAmounts;
}
/**
* If specified, the funds from the invoice will be transferred to the destination and the ID of
* the resulting transfer will be found on the invoice's charge.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TransferData getTransferData() {
return this.transferData;
}
/**
* The time at which webhooks for this invoice were successfully delivered (if the invoice had no
* webhooks to deliver, this will match `created`). Invoice payment is delayed until webhooks are
* delivered, or until all webhook delivery attempts have been exhausted.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getWebhooksDeliveredAt() {
return this.webhooksDeliveredAt;
}
/**
* The country of the business associated with this invoice, most often the business creating the
* invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAccountCountry(final String accountCountry) {
this.accountCountry = accountCountry;
}
/**
* The public name of the business associated with this invoice, most often the business creating
* the invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAccountName(final String accountName) {
this.accountName = accountName;
}
/**
* Final amount due at this time for this invoice. If the invoice's total is smaller than the
* minimum charge amount, for example, or if there is account credit that can be applied to the
* invoice, the `amount_due` may be 0. If there is a positive `starting_balance` for the invoice
* (the customer owes money), the `amount_due` will also take that into account. The charge that
* gets generated for the invoice will be for the amount specified in `amount_due`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountDue(final Long amountDue) {
this.amountDue = amountDue;
}
/**
* The amount, in %s, that was paid.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountPaid(final Long amountPaid) {
this.amountPaid = amountPaid;
}
/**
* The amount remaining, in %s, that is due.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountRemaining(final Long amountRemaining) {
this.amountRemaining = amountRemaining;
}
/**
* The fee in %s that will be applied to the invoice and transferred to the application owner's
* Stripe account when the invoice is paid.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setApplicationFeeAmount(final Long applicationFeeAmount) {
this.applicationFeeAmount = applicationFeeAmount;
}
/**
* Number of payment attempts made for this invoice, from the perspective of the payment retry
* schedule. Any payment attempt counts as the first attempt, and subsequently only automatic
* retries increment the attempt count. In other words, manual payment attempts after the first
* attempt do not affect the retry schedule.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAttemptCount(final Long attemptCount) {
this.attemptCount = attemptCount;
}
/**
* Whether an attempt has been made to pay the invoice. An invoice is not attempted until 1 hour
* after the `invoice.created` webhook, for example, so you might not want to display that invoice
* as unpaid to your users.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAttempted(final Boolean attempted) {
this.attempted = attempted;
}
/**
* Controls whether Stripe will perform [automatic
* collection](https://stripe.com/docs/billing/invoices/workflow/#auto_advance) of the invoice.
* When `false`, the invoice's state will not automatically advance without an explicit action.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAutoAdvance(final Boolean autoAdvance) {
this.autoAdvance = autoAdvance;
}
/**
* Indicates the reason why the invoice was created. `subscription_cycle` indicates an invoice
* created by a subscription advancing into a new period. `subscription_create` indicates an
* invoice created due to creating a subscription. `subscription_update` indicates an invoice
* created due to updating a subscription. `subscription` is set for all old invoices to indicate
* either a change to a subscription or a period advancement. `manual` is set for all invoices
* unrelated to a subscription (for example: created via the invoice editor). The `upcoming` value
* is reserved for simulated invoices per the upcoming invoice endpoint. `subscription_threshold`
* indicates an invoice created due to a billing threshold being reached.
*
* One of `automatic_pending_invoice_item_invoice`, `manual`, `subscription`,
* `subscription_create`, `subscription_cycle`, `subscription_threshold`, `subscription_update`,
* or `upcoming`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBillingReason(final String billingReason) {
this.billingReason = billingReason;
}
/**
* Either `charge_automatically`, or `send_invoice`. When charging automatically, Stripe will
* attempt to pay this invoice using the default source attached to the customer. When sending an
* invoice, Stripe will email this invoice to the customer with payment instructions.
*
*
One of `charge_automatically`, or `send_invoice`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCollectionMethod(final String collectionMethod) {
this.collectionMethod = collectionMethod;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* Three-letter [ISO currency code](https://www.iso.org/iso-4217-currency-codes.html), in
* lowercase. Must be a [supported currency](https://stripe.com/docs/currencies).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
/**
* Custom fields displayed on the invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCustomFields(final List customFields) {
this.customFields = customFields;
}
/**
* The customer's address. Until the invoice is finalized, this field will equal
* `customer.address`. Once the invoice is finalized, this field will no longer be updated.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCustomerAddress(final Address customerAddress) {
this.customerAddress = customerAddress;
}
/**
* The customer's email. Until the invoice is finalized, this field will equal `customer.email`.
* Once the invoice is finalized, this field will no longer be updated.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCustomerEmail(final String customerEmail) {
this.customerEmail = customerEmail;
}
/**
* The customer's name. Until the invoice is finalized, this field will equal `customer.name`.
* Once the invoice is finalized, this field will no longer be updated.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCustomerName(final String customerName) {
this.customerName = customerName;
}
/**
* The customer's phone number. Until the invoice is finalized, this field will equal
* `customer.phone`. Once the invoice is finalized, this field will no longer be updated.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCustomerPhone(final String customerPhone) {
this.customerPhone = customerPhone;
}
/**
* The customer's shipping information. Until the invoice is finalized, this field will equal
* `customer.shipping`. Once the invoice is finalized, this field will no longer be updated.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCustomerShipping(final ShippingDetails customerShipping) {
this.customerShipping = customerShipping;
}
/**
* The customer's tax exempt status. Until the invoice is finalized, this field will equal
* `customer.tax_exempt`. Once the invoice is finalized, this field will no longer be updated.
*
* One of `exempt`, `none`, or `reverse`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCustomerTaxExempt(final String customerTaxExempt) {
this.customerTaxExempt = customerTaxExempt;
}
/**
* The customer's tax IDs. Until the invoice is finalized, this field will contain the same tax
* IDs as `customer.tax_ids`. Once the invoice is finalized, this field will no longer be updated.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCustomerTaxIds(final List customerTaxIds) {
this.customerTaxIds = customerTaxIds;
}
/**
* The tax rates applied to this invoice, if any.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDefaultTaxRates(final List defaultTaxRates) {
this.defaultTaxRates = defaultTaxRates;
}
/**
* Always true for a deleted object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDeleted(final Boolean deleted) {
this.deleted = deleted;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users. Referenced as
* 'memo' in the Dashboard.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDescription(final String description) {
this.description = description;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDiscount(final Discount discount) {
this.discount = discount;
}
/**
* The date on which payment for this invoice is due. This value will be `null` for invoices where
* `collection_method=charge_automatically`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDueDate(final Long dueDate) {
this.dueDate = dueDate;
}
/**
* Ending customer balance after the invoice is finalized. Invoices are finalized approximately an
* hour after successful webhook delivery or when payment collection is attempted for the invoice.
* If the invoice has not been finalized yet, this will be null.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEndingBalance(final Long endingBalance) {
this.endingBalance = endingBalance;
}
/**
* Footer displayed on the invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFooter(final String footer) {
this.footer = footer;
}
/**
* The URL for the hosted invoice page, which allows customers to view and pay an invoice. If the
* invoice has not been finalized yet, this will be null.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setHostedInvoiceUrl(final String hostedInvoiceUrl) {
this.hostedInvoiceUrl = hostedInvoiceUrl;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* The link to download the PDF for the invoice. If the invoice has not been finalized yet, this
* will be null.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setInvoicePdf(final String invoicePdf) {
this.invoicePdf = invoicePdf;
}
/**
* The individual line items that make up the invoice. `lines` is sorted as follows: invoice items
* in reverse chronological order, followed by the subscription, if any.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLines(final InvoiceLineItemCollection lines) {
this.lines = lines;
}
/**
* Has the value `true` if the object exists in live mode or the value `false` if the object
* exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
/**
* The time at which payment will next be attempted. This value will be `null` for invoices where
* `collection_method=send_invoice`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setNextPaymentAttempt(final Long nextPaymentAttempt) {
this.nextPaymentAttempt = nextPaymentAttempt;
}
/**
* A unique, identifying string that appears on emails sent to the customer for this invoice. This
* starts with the customer's unique invoice_prefix if it is specified.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setNumber(final String number) {
this.number = number;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to `invoice`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* Whether payment was successfully collected for this invoice. An invoice can be paid (most
* commonly) with a charge or with credit from the customer's account balance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPaid(final Boolean paid) {
this.paid = paid;
}
/**
* End of the usage period during which invoice items were added to this invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPeriodEnd(final Long periodEnd) {
this.periodEnd = periodEnd;
}
/**
* Start of the usage period during which invoice items were added to this invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPeriodStart(final Long periodStart) {
this.periodStart = periodStart;
}
/**
* Total amount of all post-payment credit notes issued for this invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPostPaymentCreditNotesAmount(final Long postPaymentCreditNotesAmount) {
this.postPaymentCreditNotesAmount = postPaymentCreditNotesAmount;
}
/**
* Total amount of all pre-payment credit notes issued for this invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPrePaymentCreditNotesAmount(final Long prePaymentCreditNotesAmount) {
this.prePaymentCreditNotesAmount = prePaymentCreditNotesAmount;
}
/**
* This is the transaction number that appears on email receipts sent for this invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReceiptNumber(final String receiptNumber) {
this.receiptNumber = receiptNumber;
}
/**
* Starting customer balance before the invoice is finalized. If the invoice has not been
* finalized yet, this will be the current customer balance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStartingBalance(final Long startingBalance) {
this.startingBalance = startingBalance;
}
/**
* Extra information about an invoice for the customer's credit card statement.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatementDescriptor(final String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
}
/**
* The status of the invoice, one of `draft`, `open`, `paid`, `uncollectible`, or `void`. [Learn
* more](https://stripe.com/docs/billing/invoices/workflow#workflow-overview)
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatusTransitions(final StatusTransitions statusTransitions) {
this.statusTransitions = statusTransitions;
}
/**
* Only set for upcoming invoices that preview prorations. The time used to calculate prorations.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSubscriptionProrationDate(final Long subscriptionProrationDate) {
this.subscriptionProrationDate = subscriptionProrationDate;
}
/**
* Total of all subscriptions, invoice items, and prorations on the invoice before any discount or
* tax is applied.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSubtotal(final Long subtotal) {
this.subtotal = subtotal;
}
/**
* The amount of tax on this invoice. This is the sum of all the tax amounts on this invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTax(final Long tax) {
this.tax = tax;
}
/**
* This percentage of the subtotal has been added to the total amount of the invoice, including
* invoice line items and discounts. This field is inherited from the subscription's `tax_percent`
* field, but can be changed before the invoice is paid. This field defaults to null.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxPercent(final BigDecimal taxPercent) {
this.taxPercent = taxPercent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setThresholdReason(final ThresholdReason thresholdReason) {
this.thresholdReason = thresholdReason;
}
/**
* Total after discounts and taxes.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTotal(final Long total) {
this.total = total;
}
/**
* The aggregate amounts calculated per tax rate for all line items.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTotalTaxAmounts(final List totalTaxAmounts) {
this.totalTaxAmounts = totalTaxAmounts;
}
/**
* If specified, the funds from the invoice will be transferred to the destination and the ID of
* the resulting transfer will be found on the invoice's charge.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTransferData(final TransferData transferData) {
this.transferData = transferData;
}
/**
* The time at which webhooks for this invoice were successfully delivered (if the invoice had no
* webhooks to deliver, this will match `created`). Invoice payment is delayed until webhooks are
* delivered, or until all webhook delivery attempts have been exhausted.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setWebhooksDeliveredAt(final Long webhooksDeliveredAt) {
this.webhooksDeliveredAt = webhooksDeliveredAt;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Invoice)) return false;
final Invoice other = (Invoice) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$accountCountry = this.getAccountCountry();
final java.lang.Object other$accountCountry = other.getAccountCountry();
if (this$accountCountry == null ? other$accountCountry != null : !this$accountCountry.equals(other$accountCountry)) return false;
final java.lang.Object this$accountName = this.getAccountName();
final java.lang.Object other$accountName = other.getAccountName();
if (this$accountName == null ? other$accountName != null : !this$accountName.equals(other$accountName)) return false;
final java.lang.Object this$amountDue = this.getAmountDue();
final java.lang.Object other$amountDue = other.getAmountDue();
if (this$amountDue == null ? other$amountDue != null : !this$amountDue.equals(other$amountDue)) return false;
final java.lang.Object this$amountPaid = this.getAmountPaid();
final java.lang.Object other$amountPaid = other.getAmountPaid();
if (this$amountPaid == null ? other$amountPaid != null : !this$amountPaid.equals(other$amountPaid)) return false;
final java.lang.Object this$amountRemaining = this.getAmountRemaining();
final java.lang.Object other$amountRemaining = other.getAmountRemaining();
if (this$amountRemaining == null ? other$amountRemaining != null : !this$amountRemaining.equals(other$amountRemaining)) return false;
final java.lang.Object this$applicationFeeAmount = this.getApplicationFeeAmount();
final java.lang.Object other$applicationFeeAmount = other.getApplicationFeeAmount();
if (this$applicationFeeAmount == null ? other$applicationFeeAmount != null : !this$applicationFeeAmount.equals(other$applicationFeeAmount)) return false;
final java.lang.Object this$attemptCount = this.getAttemptCount();
final java.lang.Object other$attemptCount = other.getAttemptCount();
if (this$attemptCount == null ? other$attemptCount != null : !this$attemptCount.equals(other$attemptCount)) return false;
final java.lang.Object this$attempted = this.getAttempted();
final java.lang.Object other$attempted = other.getAttempted();
if (this$attempted == null ? other$attempted != null : !this$attempted.equals(other$attempted)) return false;
final java.lang.Object this$autoAdvance = this.getAutoAdvance();
final java.lang.Object other$autoAdvance = other.getAutoAdvance();
if (this$autoAdvance == null ? other$autoAdvance != null : !this$autoAdvance.equals(other$autoAdvance)) return false;
final java.lang.Object this$billingReason = this.getBillingReason();
final java.lang.Object other$billingReason = other.getBillingReason();
if (this$billingReason == null ? other$billingReason != null : !this$billingReason.equals(other$billingReason)) return false;
final java.lang.Object this$charge = this.getCharge();
final java.lang.Object other$charge = other.getCharge();
if (this$charge == null ? other$charge != null : !this$charge.equals(other$charge)) return false;
final java.lang.Object this$collectionMethod = this.getCollectionMethod();
final java.lang.Object other$collectionMethod = other.getCollectionMethod();
if (this$collectionMethod == null ? other$collectionMethod != null : !this$collectionMethod.equals(other$collectionMethod)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
final java.lang.Object this$customFields = this.getCustomFields();
final java.lang.Object other$customFields = other.getCustomFields();
if (this$customFields == null ? other$customFields != null : !this$customFields.equals(other$customFields)) return false;
final java.lang.Object this$customer = this.getCustomer();
final java.lang.Object other$customer = other.getCustomer();
if (this$customer == null ? other$customer != null : !this$customer.equals(other$customer)) return false;
final java.lang.Object this$customerAddress = this.getCustomerAddress();
final java.lang.Object other$customerAddress = other.getCustomerAddress();
if (this$customerAddress == null ? other$customerAddress != null : !this$customerAddress.equals(other$customerAddress)) return false;
final java.lang.Object this$customerEmail = this.getCustomerEmail();
final java.lang.Object other$customerEmail = other.getCustomerEmail();
if (this$customerEmail == null ? other$customerEmail != null : !this$customerEmail.equals(other$customerEmail)) return false;
final java.lang.Object this$customerName = this.getCustomerName();
final java.lang.Object other$customerName = other.getCustomerName();
if (this$customerName == null ? other$customerName != null : !this$customerName.equals(other$customerName)) return false;
final java.lang.Object this$customerPhone = this.getCustomerPhone();
final java.lang.Object other$customerPhone = other.getCustomerPhone();
if (this$customerPhone == null ? other$customerPhone != null : !this$customerPhone.equals(other$customerPhone)) return false;
final java.lang.Object this$customerShipping = this.getCustomerShipping();
final java.lang.Object other$customerShipping = other.getCustomerShipping();
if (this$customerShipping == null ? other$customerShipping != null : !this$customerShipping.equals(other$customerShipping)) return false;
final java.lang.Object this$customerTaxExempt = this.getCustomerTaxExempt();
final java.lang.Object other$customerTaxExempt = other.getCustomerTaxExempt();
if (this$customerTaxExempt == null ? other$customerTaxExempt != null : !this$customerTaxExempt.equals(other$customerTaxExempt)) return false;
final java.lang.Object this$customerTaxIds = this.getCustomerTaxIds();
final java.lang.Object other$customerTaxIds = other.getCustomerTaxIds();
if (this$customerTaxIds == null ? other$customerTaxIds != null : !this$customerTaxIds.equals(other$customerTaxIds)) return false;
final java.lang.Object this$defaultPaymentMethod = this.getDefaultPaymentMethod();
final java.lang.Object other$defaultPaymentMethod = other.getDefaultPaymentMethod();
if (this$defaultPaymentMethod == null ? other$defaultPaymentMethod != null : !this$defaultPaymentMethod.equals(other$defaultPaymentMethod)) return false;
final java.lang.Object this$defaultSource = this.getDefaultSource();
final java.lang.Object other$defaultSource = other.getDefaultSource();
if (this$defaultSource == null ? other$defaultSource != null : !this$defaultSource.equals(other$defaultSource)) return false;
final java.lang.Object this$defaultTaxRates = this.getDefaultTaxRates();
final java.lang.Object other$defaultTaxRates = other.getDefaultTaxRates();
if (this$defaultTaxRates == null ? other$defaultTaxRates != null : !this$defaultTaxRates.equals(other$defaultTaxRates)) return false;
final java.lang.Object this$deleted = this.getDeleted();
final java.lang.Object other$deleted = other.getDeleted();
if (this$deleted == null ? other$deleted != null : !this$deleted.equals(other$deleted)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$discount = this.getDiscount();
final java.lang.Object other$discount = other.getDiscount();
if (this$discount == null ? other$discount != null : !this$discount.equals(other$discount)) return false;
final java.lang.Object this$dueDate = this.getDueDate();
final java.lang.Object other$dueDate = other.getDueDate();
if (this$dueDate == null ? other$dueDate != null : !this$dueDate.equals(other$dueDate)) return false;
final java.lang.Object this$endingBalance = this.getEndingBalance();
final java.lang.Object other$endingBalance = other.getEndingBalance();
if (this$endingBalance == null ? other$endingBalance != null : !this$endingBalance.equals(other$endingBalance)) return false;
final java.lang.Object this$footer = this.getFooter();
final java.lang.Object other$footer = other.getFooter();
if (this$footer == null ? other$footer != null : !this$footer.equals(other$footer)) return false;
final java.lang.Object this$hostedInvoiceUrl = this.getHostedInvoiceUrl();
final java.lang.Object other$hostedInvoiceUrl = other.getHostedInvoiceUrl();
if (this$hostedInvoiceUrl == null ? other$hostedInvoiceUrl != null : !this$hostedInvoiceUrl.equals(other$hostedInvoiceUrl)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$invoicePdf = this.getInvoicePdf();
final java.lang.Object other$invoicePdf = other.getInvoicePdf();
if (this$invoicePdf == null ? other$invoicePdf != null : !this$invoicePdf.equals(other$invoicePdf)) return false;
final java.lang.Object this$lines = this.getLines();
final java.lang.Object other$lines = other.getLines();
if (this$lines == null ? other$lines != null : !this$lines.equals(other$lines)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$nextPaymentAttempt = this.getNextPaymentAttempt();
final java.lang.Object other$nextPaymentAttempt = other.getNextPaymentAttempt();
if (this$nextPaymentAttempt == null ? other$nextPaymentAttempt != null : !this$nextPaymentAttempt.equals(other$nextPaymentAttempt)) return false;
final java.lang.Object this$number = this.getNumber();
final java.lang.Object other$number = other.getNumber();
if (this$number == null ? other$number != null : !this$number.equals(other$number)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$paid = this.getPaid();
final java.lang.Object other$paid = other.getPaid();
if (this$paid == null ? other$paid != null : !this$paid.equals(other$paid)) return false;
final java.lang.Object this$paymentIntent = this.getPaymentIntent();
final java.lang.Object other$paymentIntent = other.getPaymentIntent();
if (this$paymentIntent == null ? other$paymentIntent != null : !this$paymentIntent.equals(other$paymentIntent)) return false;
final java.lang.Object this$periodEnd = this.getPeriodEnd();
final java.lang.Object other$periodEnd = other.getPeriodEnd();
if (this$periodEnd == null ? other$periodEnd != null : !this$periodEnd.equals(other$periodEnd)) return false;
final java.lang.Object this$periodStart = this.getPeriodStart();
final java.lang.Object other$periodStart = other.getPeriodStart();
if (this$periodStart == null ? other$periodStart != null : !this$periodStart.equals(other$periodStart)) return false;
final java.lang.Object this$postPaymentCreditNotesAmount = this.getPostPaymentCreditNotesAmount();
final java.lang.Object other$postPaymentCreditNotesAmount = other.getPostPaymentCreditNotesAmount();
if (this$postPaymentCreditNotesAmount == null ? other$postPaymentCreditNotesAmount != null : !this$postPaymentCreditNotesAmount.equals(other$postPaymentCreditNotesAmount)) return false;
final java.lang.Object this$prePaymentCreditNotesAmount = this.getPrePaymentCreditNotesAmount();
final java.lang.Object other$prePaymentCreditNotesAmount = other.getPrePaymentCreditNotesAmount();
if (this$prePaymentCreditNotesAmount == null ? other$prePaymentCreditNotesAmount != null : !this$prePaymentCreditNotesAmount.equals(other$prePaymentCreditNotesAmount)) return false;
final java.lang.Object this$receiptNumber = this.getReceiptNumber();
final java.lang.Object other$receiptNumber = other.getReceiptNumber();
if (this$receiptNumber == null ? other$receiptNumber != null : !this$receiptNumber.equals(other$receiptNumber)) return false;
final java.lang.Object this$startingBalance = this.getStartingBalance();
final java.lang.Object other$startingBalance = other.getStartingBalance();
if (this$startingBalance == null ? other$startingBalance != null : !this$startingBalance.equals(other$startingBalance)) return false;
final java.lang.Object this$statementDescriptor = this.getStatementDescriptor();
final java.lang.Object other$statementDescriptor = other.getStatementDescriptor();
if (this$statementDescriptor == null ? other$statementDescriptor != null : !this$statementDescriptor.equals(other$statementDescriptor)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$statusTransitions = this.getStatusTransitions();
final java.lang.Object other$statusTransitions = other.getStatusTransitions();
if (this$statusTransitions == null ? other$statusTransitions != null : !this$statusTransitions.equals(other$statusTransitions)) return false;
final java.lang.Object this$subscription = this.getSubscription();
final java.lang.Object other$subscription = other.getSubscription();
if (this$subscription == null ? other$subscription != null : !this$subscription.equals(other$subscription)) return false;
final java.lang.Object this$subscriptionProrationDate = this.getSubscriptionProrationDate();
final java.lang.Object other$subscriptionProrationDate = other.getSubscriptionProrationDate();
if (this$subscriptionProrationDate == null ? other$subscriptionProrationDate != null : !this$subscriptionProrationDate.equals(other$subscriptionProrationDate)) return false;
final java.lang.Object this$subtotal = this.getSubtotal();
final java.lang.Object other$subtotal = other.getSubtotal();
if (this$subtotal == null ? other$subtotal != null : !this$subtotal.equals(other$subtotal)) return false;
final java.lang.Object this$tax = this.getTax();
final java.lang.Object other$tax = other.getTax();
if (this$tax == null ? other$tax != null : !this$tax.equals(other$tax)) return false;
final java.lang.Object this$taxPercent = this.getTaxPercent();
final java.lang.Object other$taxPercent = other.getTaxPercent();
if (this$taxPercent == null ? other$taxPercent != null : !this$taxPercent.equals(other$taxPercent)) return false;
final java.lang.Object this$thresholdReason = this.getThresholdReason();
final java.lang.Object other$thresholdReason = other.getThresholdReason();
if (this$thresholdReason == null ? other$thresholdReason != null : !this$thresholdReason.equals(other$thresholdReason)) return false;
final java.lang.Object this$total = this.getTotal();
final java.lang.Object other$total = other.getTotal();
if (this$total == null ? other$total != null : !this$total.equals(other$total)) return false;
final java.lang.Object this$totalTaxAmounts = this.getTotalTaxAmounts();
final java.lang.Object other$totalTaxAmounts = other.getTotalTaxAmounts();
if (this$totalTaxAmounts == null ? other$totalTaxAmounts != null : !this$totalTaxAmounts.equals(other$totalTaxAmounts)) return false;
final java.lang.Object this$transferData = this.getTransferData();
final java.lang.Object other$transferData = other.getTransferData();
if (this$transferData == null ? other$transferData != null : !this$transferData.equals(other$transferData)) return false;
final java.lang.Object this$webhooksDeliveredAt = this.getWebhooksDeliveredAt();
final java.lang.Object other$webhooksDeliveredAt = other.getWebhooksDeliveredAt();
if (this$webhooksDeliveredAt == null ? other$webhooksDeliveredAt != null : !this$webhooksDeliveredAt.equals(other$webhooksDeliveredAt)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Invoice;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $accountCountry = this.getAccountCountry();
result = result * PRIME + ($accountCountry == null ? 43 : $accountCountry.hashCode());
final java.lang.Object $accountName = this.getAccountName();
result = result * PRIME + ($accountName == null ? 43 : $accountName.hashCode());
final java.lang.Object $amountDue = this.getAmountDue();
result = result * PRIME + ($amountDue == null ? 43 : $amountDue.hashCode());
final java.lang.Object $amountPaid = this.getAmountPaid();
result = result * PRIME + ($amountPaid == null ? 43 : $amountPaid.hashCode());
final java.lang.Object $amountRemaining = this.getAmountRemaining();
result = result * PRIME + ($amountRemaining == null ? 43 : $amountRemaining.hashCode());
final java.lang.Object $applicationFeeAmount = this.getApplicationFeeAmount();
result = result * PRIME + ($applicationFeeAmount == null ? 43 : $applicationFeeAmount.hashCode());
final java.lang.Object $attemptCount = this.getAttemptCount();
result = result * PRIME + ($attemptCount == null ? 43 : $attemptCount.hashCode());
final java.lang.Object $attempted = this.getAttempted();
result = result * PRIME + ($attempted == null ? 43 : $attempted.hashCode());
final java.lang.Object $autoAdvance = this.getAutoAdvance();
result = result * PRIME + ($autoAdvance == null ? 43 : $autoAdvance.hashCode());
final java.lang.Object $billingReason = this.getBillingReason();
result = result * PRIME + ($billingReason == null ? 43 : $billingReason.hashCode());
final java.lang.Object $charge = this.getCharge();
result = result * PRIME + ($charge == null ? 43 : $charge.hashCode());
final java.lang.Object $collectionMethod = this.getCollectionMethod();
result = result * PRIME + ($collectionMethod == null ? 43 : $collectionMethod.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
final java.lang.Object $customFields = this.getCustomFields();
result = result * PRIME + ($customFields == null ? 43 : $customFields.hashCode());
final java.lang.Object $customer = this.getCustomer();
result = result * PRIME + ($customer == null ? 43 : $customer.hashCode());
final java.lang.Object $customerAddress = this.getCustomerAddress();
result = result * PRIME + ($customerAddress == null ? 43 : $customerAddress.hashCode());
final java.lang.Object $customerEmail = this.getCustomerEmail();
result = result * PRIME + ($customerEmail == null ? 43 : $customerEmail.hashCode());
final java.lang.Object $customerName = this.getCustomerName();
result = result * PRIME + ($customerName == null ? 43 : $customerName.hashCode());
final java.lang.Object $customerPhone = this.getCustomerPhone();
result = result * PRIME + ($customerPhone == null ? 43 : $customerPhone.hashCode());
final java.lang.Object $customerShipping = this.getCustomerShipping();
result = result * PRIME + ($customerShipping == null ? 43 : $customerShipping.hashCode());
final java.lang.Object $customerTaxExempt = this.getCustomerTaxExempt();
result = result * PRIME + ($customerTaxExempt == null ? 43 : $customerTaxExempt.hashCode());
final java.lang.Object $customerTaxIds = this.getCustomerTaxIds();
result = result * PRIME + ($customerTaxIds == null ? 43 : $customerTaxIds.hashCode());
final java.lang.Object $defaultPaymentMethod = this.getDefaultPaymentMethod();
result = result * PRIME + ($defaultPaymentMethod == null ? 43 : $defaultPaymentMethod.hashCode());
final java.lang.Object $defaultSource = this.getDefaultSource();
result = result * PRIME + ($defaultSource == null ? 43 : $defaultSource.hashCode());
final java.lang.Object $defaultTaxRates = this.getDefaultTaxRates();
result = result * PRIME + ($defaultTaxRates == null ? 43 : $defaultTaxRates.hashCode());
final java.lang.Object $deleted = this.getDeleted();
result = result * PRIME + ($deleted == null ? 43 : $deleted.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $discount = this.getDiscount();
result = result * PRIME + ($discount == null ? 43 : $discount.hashCode());
final java.lang.Object $dueDate = this.getDueDate();
result = result * PRIME + ($dueDate == null ? 43 : $dueDate.hashCode());
final java.lang.Object $endingBalance = this.getEndingBalance();
result = result * PRIME + ($endingBalance == null ? 43 : $endingBalance.hashCode());
final java.lang.Object $footer = this.getFooter();
result = result * PRIME + ($footer == null ? 43 : $footer.hashCode());
final java.lang.Object $hostedInvoiceUrl = this.getHostedInvoiceUrl();
result = result * PRIME + ($hostedInvoiceUrl == null ? 43 : $hostedInvoiceUrl.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $invoicePdf = this.getInvoicePdf();
result = result * PRIME + ($invoicePdf == null ? 43 : $invoicePdf.hashCode());
final java.lang.Object $lines = this.getLines();
result = result * PRIME + ($lines == null ? 43 : $lines.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $nextPaymentAttempt = this.getNextPaymentAttempt();
result = result * PRIME + ($nextPaymentAttempt == null ? 43 : $nextPaymentAttempt.hashCode());
final java.lang.Object $number = this.getNumber();
result = result * PRIME + ($number == null ? 43 : $number.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $paid = this.getPaid();
result = result * PRIME + ($paid == null ? 43 : $paid.hashCode());
final java.lang.Object $paymentIntent = this.getPaymentIntent();
result = result * PRIME + ($paymentIntent == null ? 43 : $paymentIntent.hashCode());
final java.lang.Object $periodEnd = this.getPeriodEnd();
result = result * PRIME + ($periodEnd == null ? 43 : $periodEnd.hashCode());
final java.lang.Object $periodStart = this.getPeriodStart();
result = result * PRIME + ($periodStart == null ? 43 : $periodStart.hashCode());
final java.lang.Object $postPaymentCreditNotesAmount = this.getPostPaymentCreditNotesAmount();
result = result * PRIME + ($postPaymentCreditNotesAmount == null ? 43 : $postPaymentCreditNotesAmount.hashCode());
final java.lang.Object $prePaymentCreditNotesAmount = this.getPrePaymentCreditNotesAmount();
result = result * PRIME + ($prePaymentCreditNotesAmount == null ? 43 : $prePaymentCreditNotesAmount.hashCode());
final java.lang.Object $receiptNumber = this.getReceiptNumber();
result = result * PRIME + ($receiptNumber == null ? 43 : $receiptNumber.hashCode());
final java.lang.Object $startingBalance = this.getStartingBalance();
result = result * PRIME + ($startingBalance == null ? 43 : $startingBalance.hashCode());
final java.lang.Object $statementDescriptor = this.getStatementDescriptor();
result = result * PRIME + ($statementDescriptor == null ? 43 : $statementDescriptor.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $statusTransitions = this.getStatusTransitions();
result = result * PRIME + ($statusTransitions == null ? 43 : $statusTransitions.hashCode());
final java.lang.Object $subscription = this.getSubscription();
result = result * PRIME + ($subscription == null ? 43 : $subscription.hashCode());
final java.lang.Object $subscriptionProrationDate = this.getSubscriptionProrationDate();
result = result * PRIME + ($subscriptionProrationDate == null ? 43 : $subscriptionProrationDate.hashCode());
final java.lang.Object $subtotal = this.getSubtotal();
result = result * PRIME + ($subtotal == null ? 43 : $subtotal.hashCode());
final java.lang.Object $tax = this.getTax();
result = result * PRIME + ($tax == null ? 43 : $tax.hashCode());
final java.lang.Object $taxPercent = this.getTaxPercent();
result = result * PRIME + ($taxPercent == null ? 43 : $taxPercent.hashCode());
final java.lang.Object $thresholdReason = this.getThresholdReason();
result = result * PRIME + ($thresholdReason == null ? 43 : $thresholdReason.hashCode());
final java.lang.Object $total = this.getTotal();
result = result * PRIME + ($total == null ? 43 : $total.hashCode());
final java.lang.Object $totalTaxAmounts = this.getTotalTaxAmounts();
result = result * PRIME + ($totalTaxAmounts == null ? 43 : $totalTaxAmounts.hashCode());
final java.lang.Object $transferData = this.getTransferData();
result = result * PRIME + ($transferData == null ? 43 : $transferData.hashCode());
final java.lang.Object $webhooksDeliveredAt = this.getWebhooksDeliveredAt();
result = result * PRIME + ($webhooksDeliveredAt == null ? 43 : $webhooksDeliveredAt.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
}