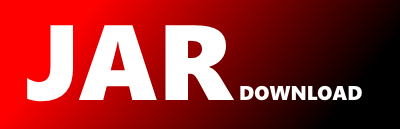
com.stripe.model.issuing.Authorization Maven / Gradle / Ivy
// Generated by delombok at Fri Dec 20 23:44:59 CET 2019
package com.stripe.model.issuing;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.model.BalanceTransaction;
import com.stripe.model.BalanceTransactionSource;
import com.stripe.model.ExpandableField;
import com.stripe.model.MetadataStore;
import com.stripe.model.StripeObject;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.issuing.AuthorizationApproveParams;
import com.stripe.param.issuing.AuthorizationDeclineParams;
import com.stripe.param.issuing.AuthorizationListParams;
import com.stripe.param.issuing.AuthorizationRetrieveParams;
import com.stripe.param.issuing.AuthorizationUpdateParams;
import java.util.List;
import java.util.Map;
public class Authorization extends ApiResource implements MetadataStore, BalanceTransactionSource {
/**
* Whether the authorization has been approved.
*/
@SerializedName("approved")
Boolean approved;
/**
* How the card details were provided.
*
* One of `chip`, `contactless`, `keyed_in`, `online`, or `swipe`.
*/
@SerializedName("authorization_method")
String authorizationMethod;
/**
* The amount that has been authorized. This will be `0` when the object is created, and increase
* after it has been approved.
*/
@SerializedName("authorized_amount")
Long authorizedAmount;
/**
* The currency that was presented to the cardholder for the authorization. Three-letter [ISO
* currency code](https://www.iso.org/iso-4217-currency-codes.html), in lowercase. Must be a
* [supported currency](https://stripe.com/docs/currencies).
*/
@SerializedName("authorized_currency")
String authorizedCurrency;
@SerializedName("balance_transactions")
List balanceTransactions;
@SerializedName("card")
Card card;
/**
* The cardholder to whom this authorization belongs.
*/
@SerializedName("cardholder")
ExpandableField cardholder;
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* The amount the authorization is expected to be in `held_currency`. When Stripe holds funds from
* you, this is the amount reserved for the authorization. This will be `0` when the object is
* created, and increase after it has been approved. For multi-currency transactions,
* `held_amount` can be used to determine the expected exchange rate.
*/
@SerializedName("held_amount")
Long heldAmount;
/**
* The currency of the [held
* amount](https://stripe.com/docs/api#issuing_authorization_object-held_amount). This will always
* be the card currency.
*/
@SerializedName("held_currency")
String heldCurrency;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
@SerializedName("is_held_amount_controllable")
Boolean isHeldAmountControllable;
/**
* Has the value `true` if the object exists in live mode or the value `false` if the object
* exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
@SerializedName("merchant_data")
MerchantData merchantData;
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to `issuing.authorization`.
*/
@SerializedName("object")
String object;
/**
* The amount the user is requesting to be authorized. This field will only be non-zero during an
* `issuing.authorization.request` webhook.
*/
@SerializedName("pending_authorized_amount")
Long pendingAuthorizedAmount;
/**
* The additional amount Stripe will hold if the authorization is approved. This field will only
* be non-zero during an `issuing.authorization.request` webhook.
*/
@SerializedName("pending_held_amount")
Long pendingHeldAmount;
@SerializedName("request_history")
List requestHistory;
/**
* The current status of the authorization in its lifecycle.
*
* One of `closed`, `pending`, or `reversed`.
*/
@SerializedName("status")
String status;
@SerializedName("transactions")
List transactions;
@SerializedName("verification_data")
VerificationData verificationData;
/**
* What, if any, digital wallet was used for this authorization. One of `apple_pay`, `google_pay`,
* or `samsung_pay`.
*/
@SerializedName("wallet_provider")
String walletProvider;
/**
* Get id of expandable `cardholder` object.
*/
public String getCardholder() {
return (this.cardholder != null) ? this.cardholder.getId() : null;
}
public void setCardholder(String id) {
this.cardholder = ApiResource.setExpandableFieldId(id, this.cardholder);
}
/**
* Get expanded `cardholder`.
*/
public Cardholder getCardholderObject() {
return (this.cardholder != null) ? this.cardholder.getExpanded() : null;
}
public void setCardholderObject(Cardholder expandableObject) {
this.cardholder = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Returns a list of Issuing Authorization
objects. The objects are sorted in
* descending order by creation date, with the most recently created object appearing first.
*/
public static AuthorizationCollection list(Map params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of Issuing Authorization
objects. The objects are sorted in
* descending order by creation date, with the most recently created object appearing first.
*/
public static AuthorizationCollection list(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/issuing/authorizations");
return ApiResource.requestCollection(url, params, AuthorizationCollection.class, options);
}
/**
* Returns a list of Issuing Authorization
objects. The objects are sorted in
* descending order by creation date, with the most recently created object appearing first.
*/
public static AuthorizationCollection list(AuthorizationListParams params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of Issuing Authorization
objects. The objects are sorted in
* descending order by creation date, with the most recently created object appearing first.
*/
public static AuthorizationCollection list(AuthorizationListParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/issuing/authorizations");
return ApiResource.requestCollection(url, params, AuthorizationCollection.class, options);
}
/**
* Retrieves an Issuing Authorization
object.
*/
public static Authorization retrieve(String authorization) throws StripeException {
return retrieve(authorization, (Map) null, (RequestOptions) null);
}
/**
* Retrieves an Issuing Authorization
object.
*/
public static Authorization retrieve(String authorization, RequestOptions options) throws StripeException {
return retrieve(authorization, (Map) null, options);
}
/**
* Retrieves an Issuing Authorization
object.
*/
public static Authorization retrieve(String authorization, Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/issuing/authorizations/%s", ApiResource.urlEncodeId(authorization)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Authorization.class, options);
}
/**
* Retrieves an Issuing Authorization
object.
*/
public static Authorization retrieve(String authorization, AuthorizationRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/issuing/authorizations/%s", ApiResource.urlEncodeId(authorization)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Authorization.class, options);
}
/**
* Updates the specified Issuing Authorization
object by setting the values of the
* parameters passed. Any parameters not provided will be left unchanged.
*/
@Override
public Authorization update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates the specified Issuing Authorization
object by setting the values of the
* parameters passed. Any parameters not provided will be left unchanged.
*/
@Override
public Authorization update(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/issuing/authorizations/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Authorization.class, options);
}
/**
* Updates the specified Issuing Authorization
object by setting the values of the
* parameters passed. Any parameters not provided will be left unchanged.
*/
public Authorization update(AuthorizationUpdateParams params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates the specified Issuing Authorization
object by setting the values of the
* parameters passed. Any parameters not provided will be left unchanged.
*/
public Authorization update(AuthorizationUpdateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/issuing/authorizations/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Authorization.class, options);
}
/**
* Approves a pending Issuing Authorization
object.
*/
public Authorization approve() throws StripeException {
return approve((Map) null, (RequestOptions) null);
}
/**
* Approves a pending Issuing Authorization
object.
*/
public Authorization approve(RequestOptions options) throws StripeException {
return approve((Map) null, options);
}
/**
* Approves a pending Issuing Authorization
object.
*/
public Authorization approve(Map params) throws StripeException {
return approve(params, (RequestOptions) null);
}
/**
* Approves a pending Issuing Authorization
object.
*/
public Authorization approve(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/issuing/authorizations/%s/approve", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Authorization.class, options);
}
/**
* Approves a pending Issuing Authorization
object.
*/
public Authorization approve(AuthorizationApproveParams params) throws StripeException {
return approve(params, (RequestOptions) null);
}
/**
* Approves a pending Issuing Authorization
object.
*/
public Authorization approve(AuthorizationApproveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/issuing/authorizations/%s/approve", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Authorization.class, options);
}
/**
* Declines a pending Issuing Authorization
object.
*/
public Authorization decline() throws StripeException {
return decline((Map) null, (RequestOptions) null);
}
/**
* Declines a pending Issuing Authorization
object.
*/
public Authorization decline(RequestOptions options) throws StripeException {
return decline((Map) null, options);
}
/**
* Declines a pending Issuing Authorization
object.
*/
public Authorization decline(Map params) throws StripeException {
return decline(params, (RequestOptions) null);
}
/**
* Declines a pending Issuing Authorization
object.
*/
public Authorization decline(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/issuing/authorizations/%s/decline", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Authorization.class, options);
}
/**
* Declines a pending Issuing Authorization
object.
*/
public Authorization decline(AuthorizationDeclineParams params) throws StripeException {
return decline(params, (RequestOptions) null);
}
/**
* Declines a pending Issuing Authorization
object.
*/
public Authorization decline(AuthorizationDeclineParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/issuing/authorizations/%s/decline", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Authorization.class, options);
}
public static class RequestHistory extends StripeObject {
/**
* Whether this request was approved.
*/
@SerializedName("approved")
Boolean approved;
/**
* The amount that was authorized at the time of this request.
*/
@SerializedName("authorized_amount")
Long authorizedAmount;
/**
* The currency that was presented to the cardholder for the authorization. Three-letter [ISO
* currency code](https://www.iso.org/iso-4217-currency-codes.html), in lowercase. Must be a
* [supported currency](https://stripe.com/docs/currencies).
*/
@SerializedName("authorized_currency")
String authorizedCurrency;
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* The amount Stripe held from your account to fund the authorization, if the request was
* approved.
*/
@SerializedName("held_amount")
Long heldAmount;
/**
* The currency of the [held
* amount](https://stripe.com/docs/api#issuing_authorization_object-held_amount).
*/
@SerializedName("held_currency")
String heldCurrency;
/**
* The reason for the approval or decline.
*
* One of `account_compliance_disabled`, `account_inactive`, `authentication_failed`,
* `authorization_controls`, `card_active`, `card_inactive`, `cardholder_inactive`,
* `cardholder_verification_required`, `insufficient_funds`, `not_allowed`, `suspected_fraud`,
* `webhook_approved`, `webhook_declined`, or `webhook_timeout`.
*/
@SerializedName("reason")
String reason;
/**
* When an authorization is declined due to `authorization_controls`, this array contains
* details about the authorization controls that were violated. Otherwise, it is empty.
*/
@SerializedName("violated_authorization_controls")
List violatedAuthorizationControls;
public static class ViolatedAuthorizationControl extends StripeObject {
/**
* Entity which the authorization control acts on. One of `account`, `card`, or `cardholder`.
*/
@SerializedName("entity")
String entity;
@SerializedName("name")
String name;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getEntity() {
return this.entity;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getName() {
return this.name;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEntity(final String entity) {
this.entity = entity;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setName(final String name) {
this.name = name;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Authorization.RequestHistory.ViolatedAuthorizationControl)) return false;
final Authorization.RequestHistory.ViolatedAuthorizationControl other = (Authorization.RequestHistory.ViolatedAuthorizationControl) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$entity = this.getEntity();
final java.lang.Object other$entity = other.getEntity();
if (this$entity == null ? other$entity != null : !this$entity.equals(other$entity)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Authorization.RequestHistory.ViolatedAuthorizationControl;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $entity = this.getEntity();
result = result * PRIME + ($entity == null ? 43 : $entity.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
return result;
}
}
/**
* Whether this request was approved.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getApproved() {
return this.approved;
}
/**
* The amount that was authorized at the time of this request.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAuthorizedAmount() {
return this.authorizedAmount;
}
/**
* The currency that was presented to the cardholder for the authorization. Three-letter [ISO
* currency code](https://www.iso.org/iso-4217-currency-codes.html), in lowercase. Must be a
* [supported currency](https://stripe.com/docs/currencies).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAuthorizedCurrency() {
return this.authorizedCurrency;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
/**
* The amount Stripe held from your account to fund the authorization, if the request was
* approved.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getHeldAmount() {
return this.heldAmount;
}
/**
* The currency of the [held
* amount](https://stripe.com/docs/api#issuing_authorization_object-held_amount).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getHeldCurrency() {
return this.heldCurrency;
}
/**
* The reason for the approval or decline.
*
* One of `account_compliance_disabled`, `account_inactive`, `authentication_failed`,
* `authorization_controls`, `card_active`, `card_inactive`, `cardholder_inactive`,
* `cardholder_verification_required`, `insufficient_funds`, `not_allowed`, `suspected_fraud`,
* `webhook_approved`, `webhook_declined`, or `webhook_timeout`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReason() {
return this.reason;
}
/**
* When an authorization is declined due to `authorization_controls`, this array contains
* details about the authorization controls that were violated. Otherwise, it is empty.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getViolatedAuthorizationControls() {
return this.violatedAuthorizationControls;
}
/**
* Whether this request was approved.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setApproved(final Boolean approved) {
this.approved = approved;
}
/**
* The amount that was authorized at the time of this request.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAuthorizedAmount(final Long authorizedAmount) {
this.authorizedAmount = authorizedAmount;
}
/**
* The currency that was presented to the cardholder for the authorization. Three-letter [ISO
* currency code](https://www.iso.org/iso-4217-currency-codes.html), in lowercase. Must be a
* [supported currency](https://stripe.com/docs/currencies).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAuthorizedCurrency(final String authorizedCurrency) {
this.authorizedCurrency = authorizedCurrency;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* The amount Stripe held from your account to fund the authorization, if the request was
* approved.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setHeldAmount(final Long heldAmount) {
this.heldAmount = heldAmount;
}
/**
* The currency of the [held
* amount](https://stripe.com/docs/api#issuing_authorization_object-held_amount).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setHeldCurrency(final String heldCurrency) {
this.heldCurrency = heldCurrency;
}
/**
* The reason for the approval or decline.
*
* One of `account_compliance_disabled`, `account_inactive`, `authentication_failed`,
* `authorization_controls`, `card_active`, `card_inactive`, `cardholder_inactive`,
* `cardholder_verification_required`, `insufficient_funds`, `not_allowed`, `suspected_fraud`,
* `webhook_approved`, `webhook_declined`, or `webhook_timeout`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReason(final String reason) {
this.reason = reason;
}
/**
* When an authorization is declined due to `authorization_controls`, this array contains
* details about the authorization controls that were violated. Otherwise, it is empty.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setViolatedAuthorizationControls(final List violatedAuthorizationControls) {
this.violatedAuthorizationControls = violatedAuthorizationControls;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Authorization.RequestHistory)) return false;
final Authorization.RequestHistory other = (Authorization.RequestHistory) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$approved = this.getApproved();
final java.lang.Object other$approved = other.getApproved();
if (this$approved == null ? other$approved != null : !this$approved.equals(other$approved)) return false;
final java.lang.Object this$authorizedAmount = this.getAuthorizedAmount();
final java.lang.Object other$authorizedAmount = other.getAuthorizedAmount();
if (this$authorizedAmount == null ? other$authorizedAmount != null : !this$authorizedAmount.equals(other$authorizedAmount)) return false;
final java.lang.Object this$authorizedCurrency = this.getAuthorizedCurrency();
final java.lang.Object other$authorizedCurrency = other.getAuthorizedCurrency();
if (this$authorizedCurrency == null ? other$authorizedCurrency != null : !this$authorizedCurrency.equals(other$authorizedCurrency)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$heldAmount = this.getHeldAmount();
final java.lang.Object other$heldAmount = other.getHeldAmount();
if (this$heldAmount == null ? other$heldAmount != null : !this$heldAmount.equals(other$heldAmount)) return false;
final java.lang.Object this$heldCurrency = this.getHeldCurrency();
final java.lang.Object other$heldCurrency = other.getHeldCurrency();
if (this$heldCurrency == null ? other$heldCurrency != null : !this$heldCurrency.equals(other$heldCurrency)) return false;
final java.lang.Object this$reason = this.getReason();
final java.lang.Object other$reason = other.getReason();
if (this$reason == null ? other$reason != null : !this$reason.equals(other$reason)) return false;
final java.lang.Object this$violatedAuthorizationControls = this.getViolatedAuthorizationControls();
final java.lang.Object other$violatedAuthorizationControls = other.getViolatedAuthorizationControls();
if (this$violatedAuthorizationControls == null ? other$violatedAuthorizationControls != null : !this$violatedAuthorizationControls.equals(other$violatedAuthorizationControls)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Authorization.RequestHistory;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $approved = this.getApproved();
result = result * PRIME + ($approved == null ? 43 : $approved.hashCode());
final java.lang.Object $authorizedAmount = this.getAuthorizedAmount();
result = result * PRIME + ($authorizedAmount == null ? 43 : $authorizedAmount.hashCode());
final java.lang.Object $authorizedCurrency = this.getAuthorizedCurrency();
result = result * PRIME + ($authorizedCurrency == null ? 43 : $authorizedCurrency.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $heldAmount = this.getHeldAmount();
result = result * PRIME + ($heldAmount == null ? 43 : $heldAmount.hashCode());
final java.lang.Object $heldCurrency = this.getHeldCurrency();
result = result * PRIME + ($heldCurrency == null ? 43 : $heldCurrency.hashCode());
final java.lang.Object $reason = this.getReason();
result = result * PRIME + ($reason == null ? 43 : $reason.hashCode());
final java.lang.Object $violatedAuthorizationControls = this.getViolatedAuthorizationControls();
result = result * PRIME + ($violatedAuthorizationControls == null ? 43 : $violatedAuthorizationControls.hashCode());
return result;
}
}
/**
* Name of the authorization control. One of `allowed_categories`, `blocked_categories`,
* `max_amount`, `max_approvals`, or `spending_limits`.
*/
public static class VerificationData extends StripeObject {
/**
* Whether the cardholder provided an address first line and if it matched the cardholder’s
* `billing.address.line1`. One of `match`, `mismatch`, or `not_provided`.
*/
@SerializedName("address_line1_check")
String addressLine1Check;
/**
* Whether the cardholder provided a zip (or postal code) and if it matched the cardholder’s
* `billing.address.postal_code`. One of `match`, `mismatch`, or `not_provided`.
*/
@SerializedName("address_zip_check")
String addressZipCheck;
/** One of `exempt`, `failure`, `none`, or `success`. */
@SerializedName("authentication")
String authentication;
/**
* Whether the cardholder provided a CVC and if it matched Stripe’s record. One of `match`,
* `mismatch`, or `not_provided`.
*/
@SerializedName("cvc_check")
String cvcCheck;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAddressLine1Check() {
return this.addressLine1Check;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAddressZipCheck() {
return this.addressZipCheck;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAuthentication() {
return this.authentication;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCvcCheck() {
return this.cvcCheck;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddressLine1Check(final String addressLine1Check) {
this.addressLine1Check = addressLine1Check;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddressZipCheck(final String addressZipCheck) {
this.addressZipCheck = addressZipCheck;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAuthentication(final String authentication) {
this.authentication = authentication;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCvcCheck(final String cvcCheck) {
this.cvcCheck = cvcCheck;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Authorization.VerificationData)) return false;
final Authorization.VerificationData other = (Authorization.VerificationData) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$addressLine1Check = this.getAddressLine1Check();
final java.lang.Object other$addressLine1Check = other.getAddressLine1Check();
if (this$addressLine1Check == null ? other$addressLine1Check != null : !this$addressLine1Check.equals(other$addressLine1Check)) return false;
final java.lang.Object this$addressZipCheck = this.getAddressZipCheck();
final java.lang.Object other$addressZipCheck = other.getAddressZipCheck();
if (this$addressZipCheck == null ? other$addressZipCheck != null : !this$addressZipCheck.equals(other$addressZipCheck)) return false;
final java.lang.Object this$authentication = this.getAuthentication();
final java.lang.Object other$authentication = other.getAuthentication();
if (this$authentication == null ? other$authentication != null : !this$authentication.equals(other$authentication)) return false;
final java.lang.Object this$cvcCheck = this.getCvcCheck();
final java.lang.Object other$cvcCheck = other.getCvcCheck();
if (this$cvcCheck == null ? other$cvcCheck != null : !this$cvcCheck.equals(other$cvcCheck)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Authorization.VerificationData;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $addressLine1Check = this.getAddressLine1Check();
result = result * PRIME + ($addressLine1Check == null ? 43 : $addressLine1Check.hashCode());
final java.lang.Object $addressZipCheck = this.getAddressZipCheck();
result = result * PRIME + ($addressZipCheck == null ? 43 : $addressZipCheck.hashCode());
final java.lang.Object $authentication = this.getAuthentication();
result = result * PRIME + ($authentication == null ? 43 : $authentication.hashCode());
final java.lang.Object $cvcCheck = this.getCvcCheck();
result = result * PRIME + ($cvcCheck == null ? 43 : $cvcCheck.hashCode());
return result;
}
}
/**
* Whether the authorization has been approved.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getApproved() {
return this.approved;
}
/**
* How the card details were provided.
*
* One of `chip`, `contactless`, `keyed_in`, `online`, or `swipe`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAuthorizationMethod() {
return this.authorizationMethod;
}
/**
* The amount that has been authorized. This will be `0` when the object is created, and increase
* after it has been approved.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAuthorizedAmount() {
return this.authorizedAmount;
}
/**
* The currency that was presented to the cardholder for the authorization. Three-letter [ISO
* currency code](https://www.iso.org/iso-4217-currency-codes.html), in lowercase. Must be a
* [supported currency](https://stripe.com/docs/currencies).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAuthorizedCurrency() {
return this.authorizedCurrency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getBalanceTransactions() {
return this.balanceTransactions;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Card getCard() {
return this.card;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
/**
* The amount the authorization is expected to be in `held_currency`. When Stripe holds funds from
* you, this is the amount reserved for the authorization. This will be `0` when the object is
* created, and increase after it has been approved. For multi-currency transactions,
* `held_amount` can be used to determine the expected exchange rate.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getHeldAmount() {
return this.heldAmount;
}
/**
* The currency of the [held
* amount](https://stripe.com/docs/api#issuing_authorization_object-held_amount). This will always
* be the card currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getHeldCurrency() {
return this.heldCurrency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getIsHeldAmountControllable() {
return this.isHeldAmountControllable;
}
/**
* Has the value `true` if the object exists in live mode or the value `false` if the object
* exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public MerchantData getMerchantData() {
return this.merchantData;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to `issuing.authorization`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* The amount the user is requesting to be authorized. This field will only be non-zero during an
* `issuing.authorization.request` webhook.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getPendingAuthorizedAmount() {
return this.pendingAuthorizedAmount;
}
/**
* The additional amount Stripe will hold if the authorization is approved. This field will only
* be non-zero during an `issuing.authorization.request` webhook.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getPendingHeldAmount() {
return this.pendingHeldAmount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getRequestHistory() {
return this.requestHistory;
}
/**
* The current status of the authorization in its lifecycle.
*
* One of `closed`, `pending`, or `reversed`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getTransactions() {
return this.transactions;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public VerificationData getVerificationData() {
return this.verificationData;
}
/**
* What, if any, digital wallet was used for this authorization. One of `apple_pay`, `google_pay`,
* or `samsung_pay`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getWalletProvider() {
return this.walletProvider;
}
/**
* Whether the authorization has been approved.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setApproved(final Boolean approved) {
this.approved = approved;
}
/**
* How the card details were provided.
*
* One of `chip`, `contactless`, `keyed_in`, `online`, or `swipe`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAuthorizationMethod(final String authorizationMethod) {
this.authorizationMethod = authorizationMethod;
}
/**
* The amount that has been authorized. This will be `0` when the object is created, and increase
* after it has been approved.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAuthorizedAmount(final Long authorizedAmount) {
this.authorizedAmount = authorizedAmount;
}
/**
* The currency that was presented to the cardholder for the authorization. Three-letter [ISO
* currency code](https://www.iso.org/iso-4217-currency-codes.html), in lowercase. Must be a
* [supported currency](https://stripe.com/docs/currencies).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAuthorizedCurrency(final String authorizedCurrency) {
this.authorizedCurrency = authorizedCurrency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBalanceTransactions(final List balanceTransactions) {
this.balanceTransactions = balanceTransactions;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCard(final Card card) {
this.card = card;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* The amount the authorization is expected to be in `held_currency`. When Stripe holds funds from
* you, this is the amount reserved for the authorization. This will be `0` when the object is
* created, and increase after it has been approved. For multi-currency transactions,
* `held_amount` can be used to determine the expected exchange rate.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setHeldAmount(final Long heldAmount) {
this.heldAmount = heldAmount;
}
/**
* The currency of the [held
* amount](https://stripe.com/docs/api#issuing_authorization_object-held_amount). This will always
* be the card currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setHeldCurrency(final String heldCurrency) {
this.heldCurrency = heldCurrency;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIsHeldAmountControllable(final Boolean isHeldAmountControllable) {
this.isHeldAmountControllable = isHeldAmountControllable;
}
/**
* Has the value `true` if the object exists in live mode or the value `false` if the object
* exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMerchantData(final MerchantData merchantData) {
this.merchantData = merchantData;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to `issuing.authorization`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* The amount the user is requesting to be authorized. This field will only be non-zero during an
* `issuing.authorization.request` webhook.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPendingAuthorizedAmount(final Long pendingAuthorizedAmount) {
this.pendingAuthorizedAmount = pendingAuthorizedAmount;
}
/**
* The additional amount Stripe will hold if the authorization is approved. This field will only
* be non-zero during an `issuing.authorization.request` webhook.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPendingHeldAmount(final Long pendingHeldAmount) {
this.pendingHeldAmount = pendingHeldAmount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRequestHistory(final List requestHistory) {
this.requestHistory = requestHistory;
}
/**
* The current status of the authorization in its lifecycle.
*
* One of `closed`, `pending`, or `reversed`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTransactions(final List transactions) {
this.transactions = transactions;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVerificationData(final VerificationData verificationData) {
this.verificationData = verificationData;
}
/**
* What, if any, digital wallet was used for this authorization. One of `apple_pay`, `google_pay`,
* or `samsung_pay`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setWalletProvider(final String walletProvider) {
this.walletProvider = walletProvider;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Authorization)) return false;
final Authorization other = (Authorization) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$approved = this.getApproved();
final java.lang.Object other$approved = other.getApproved();
if (this$approved == null ? other$approved != null : !this$approved.equals(other$approved)) return false;
final java.lang.Object this$authorizationMethod = this.getAuthorizationMethod();
final java.lang.Object other$authorizationMethod = other.getAuthorizationMethod();
if (this$authorizationMethod == null ? other$authorizationMethod != null : !this$authorizationMethod.equals(other$authorizationMethod)) return false;
final java.lang.Object this$authorizedAmount = this.getAuthorizedAmount();
final java.lang.Object other$authorizedAmount = other.getAuthorizedAmount();
if (this$authorizedAmount == null ? other$authorizedAmount != null : !this$authorizedAmount.equals(other$authorizedAmount)) return false;
final java.lang.Object this$authorizedCurrency = this.getAuthorizedCurrency();
final java.lang.Object other$authorizedCurrency = other.getAuthorizedCurrency();
if (this$authorizedCurrency == null ? other$authorizedCurrency != null : !this$authorizedCurrency.equals(other$authorizedCurrency)) return false;
final java.lang.Object this$balanceTransactions = this.getBalanceTransactions();
final java.lang.Object other$balanceTransactions = other.getBalanceTransactions();
if (this$balanceTransactions == null ? other$balanceTransactions != null : !this$balanceTransactions.equals(other$balanceTransactions)) return false;
final java.lang.Object this$card = this.getCard();
final java.lang.Object other$card = other.getCard();
if (this$card == null ? other$card != null : !this$card.equals(other$card)) return false;
final java.lang.Object this$cardholder = this.getCardholder();
final java.lang.Object other$cardholder = other.getCardholder();
if (this$cardholder == null ? other$cardholder != null : !this$cardholder.equals(other$cardholder)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$heldAmount = this.getHeldAmount();
final java.lang.Object other$heldAmount = other.getHeldAmount();
if (this$heldAmount == null ? other$heldAmount != null : !this$heldAmount.equals(other$heldAmount)) return false;
final java.lang.Object this$heldCurrency = this.getHeldCurrency();
final java.lang.Object other$heldCurrency = other.getHeldCurrency();
if (this$heldCurrency == null ? other$heldCurrency != null : !this$heldCurrency.equals(other$heldCurrency)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$isHeldAmountControllable = this.getIsHeldAmountControllable();
final java.lang.Object other$isHeldAmountControllable = other.getIsHeldAmountControllable();
if (this$isHeldAmountControllable == null ? other$isHeldAmountControllable != null : !this$isHeldAmountControllable.equals(other$isHeldAmountControllable)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$merchantData = this.getMerchantData();
final java.lang.Object other$merchantData = other.getMerchantData();
if (this$merchantData == null ? other$merchantData != null : !this$merchantData.equals(other$merchantData)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$pendingAuthorizedAmount = this.getPendingAuthorizedAmount();
final java.lang.Object other$pendingAuthorizedAmount = other.getPendingAuthorizedAmount();
if (this$pendingAuthorizedAmount == null ? other$pendingAuthorizedAmount != null : !this$pendingAuthorizedAmount.equals(other$pendingAuthorizedAmount)) return false;
final java.lang.Object this$pendingHeldAmount = this.getPendingHeldAmount();
final java.lang.Object other$pendingHeldAmount = other.getPendingHeldAmount();
if (this$pendingHeldAmount == null ? other$pendingHeldAmount != null : !this$pendingHeldAmount.equals(other$pendingHeldAmount)) return false;
final java.lang.Object this$requestHistory = this.getRequestHistory();
final java.lang.Object other$requestHistory = other.getRequestHistory();
if (this$requestHistory == null ? other$requestHistory != null : !this$requestHistory.equals(other$requestHistory)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$transactions = this.getTransactions();
final java.lang.Object other$transactions = other.getTransactions();
if (this$transactions == null ? other$transactions != null : !this$transactions.equals(other$transactions)) return false;
final java.lang.Object this$verificationData = this.getVerificationData();
final java.lang.Object other$verificationData = other.getVerificationData();
if (this$verificationData == null ? other$verificationData != null : !this$verificationData.equals(other$verificationData)) return false;
final java.lang.Object this$walletProvider = this.getWalletProvider();
final java.lang.Object other$walletProvider = other.getWalletProvider();
if (this$walletProvider == null ? other$walletProvider != null : !this$walletProvider.equals(other$walletProvider)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Authorization;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $approved = this.getApproved();
result = result * PRIME + ($approved == null ? 43 : $approved.hashCode());
final java.lang.Object $authorizationMethod = this.getAuthorizationMethod();
result = result * PRIME + ($authorizationMethod == null ? 43 : $authorizationMethod.hashCode());
final java.lang.Object $authorizedAmount = this.getAuthorizedAmount();
result = result * PRIME + ($authorizedAmount == null ? 43 : $authorizedAmount.hashCode());
final java.lang.Object $authorizedCurrency = this.getAuthorizedCurrency();
result = result * PRIME + ($authorizedCurrency == null ? 43 : $authorizedCurrency.hashCode());
final java.lang.Object $balanceTransactions = this.getBalanceTransactions();
result = result * PRIME + ($balanceTransactions == null ? 43 : $balanceTransactions.hashCode());
final java.lang.Object $card = this.getCard();
result = result * PRIME + ($card == null ? 43 : $card.hashCode());
final java.lang.Object $cardholder = this.getCardholder();
result = result * PRIME + ($cardholder == null ? 43 : $cardholder.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $heldAmount = this.getHeldAmount();
result = result * PRIME + ($heldAmount == null ? 43 : $heldAmount.hashCode());
final java.lang.Object $heldCurrency = this.getHeldCurrency();
result = result * PRIME + ($heldCurrency == null ? 43 : $heldCurrency.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $isHeldAmountControllable = this.getIsHeldAmountControllable();
result = result * PRIME + ($isHeldAmountControllable == null ? 43 : $isHeldAmountControllable.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $merchantData = this.getMerchantData();
result = result * PRIME + ($merchantData == null ? 43 : $merchantData.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $pendingAuthorizedAmount = this.getPendingAuthorizedAmount();
result = result * PRIME + ($pendingAuthorizedAmount == null ? 43 : $pendingAuthorizedAmount.hashCode());
final java.lang.Object $pendingHeldAmount = this.getPendingHeldAmount();
result = result * PRIME + ($pendingHeldAmount == null ? 43 : $pendingHeldAmount.hashCode());
final java.lang.Object $requestHistory = this.getRequestHistory();
result = result * PRIME + ($requestHistory == null ? 43 : $requestHistory.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $transactions = this.getTransactions();
result = result * PRIME + ($transactions == null ? 43 : $transactions.hashCode());
final java.lang.Object $verificationData = this.getVerificationData();
result = result * PRIME + ($verificationData == null ? 43 : $verificationData.hashCode());
final java.lang.Object $walletProvider = this.getWalletProvider();
result = result * PRIME + ($walletProvider == null ? 43 : $walletProvider.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
}