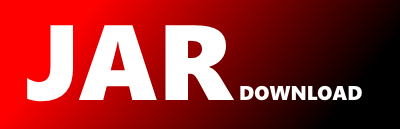
com.stripe.model.StripeCollection Maven / Gradle / Ivy
// Generated by delombok at Wed Jan 15 15:10:53 PST 2020
package com.stripe.model;
import com.stripe.net.RequestOptions;
import java.util.List;
import java.util.Map;
/**
* Provides a representation of a single page worth of data from the Stripe API.
*
* The following code will have the effect of iterating through a single page worth of invoice
* data retrieve from the API:
*
*
*
*
{@code
* foreach (Invoice invoice : Invoice.list(...).getData()) {
* System.out.println("Current invoice = " + invoice.toString());
* }
* }
*
* The class also provides a helper for iterating over collections that may be longer than a
* single page:
*
*
*
*
{@code
* foreach (Invoice invoice : Invoice.list(...).autoPagingIterable()) {
* System.out.println("Current invoice = " + invoice.toString());
* }
* }
*/
public abstract class StripeCollection extends StripeObject implements StripeCollectionInterface {
String object;
List data;
Boolean hasMore;
String url;
private RequestOptions requestOptions;
private Map requestParams;
public Iterable autoPagingIterable() {
return new PagingIterable<>(this);
}
public Iterable autoPagingIterable(Map params) {
this.setRequestParams(params);
return new PagingIterable<>(this);
}
/**
* Constructs an iterable that can be used to iterate across all objects across all pages. As page
* boundaries are encountered, the next page will be fetched automatically for continued
* iteration.
*
* @param params request parameters (will override the parameters from the initial list request)
* @param options request options (will override the options from the initial list request)
*/
public Iterable autoPagingIterable(Map params, RequestOptions options) {
this.setRequestOptions(options);
this.setRequestParams(params);
return new PagingIterable<>(this);
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setData(final List data) {
this.data = data;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setHasMore(final Boolean hasMore) {
this.hasMore = hasMore;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUrl(final String url) {
this.url = url;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof StripeCollection)) return false;
final StripeCollection> other = (StripeCollection>) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$data = this.getData();
final java.lang.Object other$data = other.getData();
if (this$data == null ? other$data != null : !this$data.equals(other$data)) return false;
final java.lang.Object this$hasMore = this.getHasMore();
final java.lang.Object other$hasMore = other.getHasMore();
if (this$hasMore == null ? other$hasMore != null : !this$hasMore.equals(other$hasMore)) return false;
final java.lang.Object this$url = this.getUrl();
final java.lang.Object other$url = other.getUrl();
if (this$url == null ? other$url != null : !this$url.equals(other$url)) return false;
final java.lang.Object this$requestOptions = this.getRequestOptions();
final java.lang.Object other$requestOptions = other.getRequestOptions();
if (this$requestOptions == null ? other$requestOptions != null : !this$requestOptions.equals(other$requestOptions)) return false;
final java.lang.Object this$requestParams = this.getRequestParams();
final java.lang.Object other$requestParams = other.getRequestParams();
if (this$requestParams == null ? other$requestParams != null : !this$requestParams.equals(other$requestParams)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof StripeCollection;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $data = this.getData();
result = result * PRIME + ($data == null ? 43 : $data.hashCode());
final java.lang.Object $hasMore = this.getHasMore();
result = result * PRIME + ($hasMore == null ? 43 : $hasMore.hashCode());
final java.lang.Object $url = this.getUrl();
result = result * PRIME + ($url == null ? 43 : $url.hashCode());
final java.lang.Object $requestOptions = this.getRequestOptions();
result = result * PRIME + ($requestOptions == null ? 43 : $requestOptions.hashCode());
final java.lang.Object $requestParams = this.getRequestParams();
result = result * PRIME + ($requestParams == null ? 43 : $requestParams.hashCode());
return result;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getData() {
return this.data;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getHasMore() {
return this.hasMore;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUrl() {
return this.url;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public RequestOptions getRequestOptions() {
return this.requestOptions;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRequestOptions(final RequestOptions requestOptions) {
this.requestOptions = requestOptions;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getRequestParams() {
return this.requestParams;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRequestParams(final Map requestParams) {
this.requestParams = requestParams;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy