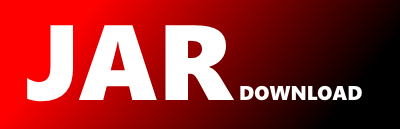
com.stripe.net.RequestTelemetry Maven / Gradle / Ivy
// Generated by delombok at Wed Jan 15 15:10:52 PST 2020
package com.stripe.net;
import com.google.gson.Gson;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import java.time.Duration;
import java.util.Optional;
import java.util.concurrent.ConcurrentLinkedQueue;
/**
* Helper class used by {@link LiveStripeResponseGetter} to manage request telemetry.
*/
class RequestTelemetry {
/**
* The name of the header used to send request telemetry in requests.
*/
public static final String HEADER_NAME = "X-Stripe-Client-Telemetry";
private static final int MAX_REQUEST_METRICS_QUEUE_SIZE = 100;
private static final Gson gson = new Gson();
private static ConcurrentLinkedQueue prevRequestMetrics = new ConcurrentLinkedQueue();
/**
* Returns an {@link Optional} containing the value of the {@code X-Stripe-Telemetry} header to
* add to the request. If the header is already present in the request, or if there is available
* metrics, or if telemetry is disabled, then the returned {@code Optional} is empty.
*
* @param headers the request headers
*/
public Optional getHeaderValue(HttpHeaders headers) {
if (headers.firstValue(HEADER_NAME).isPresent()) {
return Optional.empty();
}
RequestMetrics requestMetrics = prevRequestMetrics.poll();
if (requestMetrics == null) {
return Optional.empty();
}
if (!Stripe.enableTelemetry) {
return Optional.empty();
}
ClientTelemetryPayload payload = new ClientTelemetryPayload(requestMetrics);
return Optional.of(gson.toJson(payload));
}
/**
* If telemetry is enabled and the queue is not full, then enqueue a new metrics item; otherwise,
* do nothing.
*
* @param response the Stripe response
* @param duration the request duration
*/
public void maybeEnqueueMetrics(StripeResponse response, Duration duration) {
if (!Stripe.enableTelemetry) {
return;
}
if (response.requestId() == null) {
return;
}
if (prevRequestMetrics.size() >= MAX_REQUEST_METRICS_QUEUE_SIZE) {
return;
}
RequestMetrics metrics = new RequestMetrics(response.requestId(), duration.toMillis());
prevRequestMetrics.add(metrics);
}
private static class ClientTelemetryPayload {
@SerializedName("last_request_metrics")
private final RequestMetrics lastRequestMetrics;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ClientTelemetryPayload(final RequestMetrics lastRequestMetrics) {
this.lastRequestMetrics = lastRequestMetrics;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public RequestMetrics getLastRequestMetrics() {
return this.lastRequestMetrics;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof RequestTelemetry.ClientTelemetryPayload)) return false;
final RequestTelemetry.ClientTelemetryPayload other = (RequestTelemetry.ClientTelemetryPayload) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$lastRequestMetrics = this.getLastRequestMetrics();
final java.lang.Object other$lastRequestMetrics = other.getLastRequestMetrics();
if (this$lastRequestMetrics == null ? other$lastRequestMetrics != null : !this$lastRequestMetrics.equals(other$lastRequestMetrics)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof RequestTelemetry.ClientTelemetryPayload;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $lastRequestMetrics = this.getLastRequestMetrics();
result = result * PRIME + ($lastRequestMetrics == null ? 43 : $lastRequestMetrics.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "RequestTelemetry.ClientTelemetryPayload(lastRequestMetrics=" + this.getLastRequestMetrics() + ")";
}
}
private static class RequestMetrics {
@SerializedName("request_id")
private final String requestId;
@SerializedName("request_duration_ms")
private final long requestDurationMs;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public RequestMetrics(final String requestId, final long requestDurationMs) {
this.requestId = requestId;
this.requestDurationMs = requestDurationMs;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getRequestId() {
return this.requestId;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public long getRequestDurationMs() {
return this.requestDurationMs;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof RequestTelemetry.RequestMetrics)) return false;
final RequestTelemetry.RequestMetrics other = (RequestTelemetry.RequestMetrics) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$requestId = this.getRequestId();
final java.lang.Object other$requestId = other.getRequestId();
if (this$requestId == null ? other$requestId != null : !this$requestId.equals(other$requestId)) return false;
if (this.getRequestDurationMs() != other.getRequestDurationMs()) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof RequestTelemetry.RequestMetrics;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $requestId = this.getRequestId();
result = result * PRIME + ($requestId == null ? 43 : $requestId.hashCode());
final long $requestDurationMs = this.getRequestDurationMs();
result = result * PRIME + (int) ($requestDurationMs >>> 32 ^ $requestDurationMs);
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "RequestTelemetry.RequestMetrics(requestId=" + this.getRequestId() + ", requestDurationMs=" + this.getRequestDurationMs() + ")";
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy