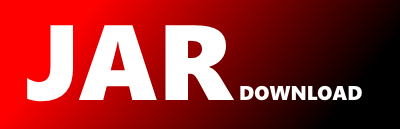
com.stripe.param.SubscriptionUpdateParams Maven / Gradle / Ivy
// Generated by delombok at Wed Jan 15 15:10:52 PST 2020
package com.stripe.param;
import com.google.gson.annotations.SerializedName;
import com.stripe.net.ApiRequestParams;
import com.stripe.param.common.EmptyParam;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class SubscriptionUpdateParams extends ApiRequestParams {
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents the
* percentage of the subscription invoice subtotal that will be transferred to the application
* owner's Stripe account. The request must be made by a platform account on a connected account
* in order to set an application fee percentage. For more information, see the application fees
* [documentation](https://stripe.com/docs/connect/subscriptions#collecting-fees-on-subscriptions).
*/
@SerializedName("application_fee_percent")
BigDecimal applicationFeePercent;
/**
* Either `now` or `unchanged`. Setting the value to `now` resets the subscription's billing cycle
* anchor to the current time. For more information, see the billing cycle
* [documentation](https://stripe.com/docs/billing/subscriptions/billing-cycle).
*/
@SerializedName("billing_cycle_anchor")
BillingCycleAnchor billingCycleAnchor;
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. Pass an empty string to remove previously-defined thresholds.
*/
@SerializedName("billing_thresholds")
Object billingThresholds;
/**
* A timestamp at which the subscription should cancel. If set to a date before the current period
* ends this will cause a proration if `prorate=true`.
*/
@SerializedName("cancel_at")
Object cancelAt;
/**
* Boolean indicating whether this subscription should cancel at the end of the current period.
*/
@SerializedName("cancel_at_period_end")
Boolean cancelAtPeriodEnd;
/**
* Either `charge_automatically`, or `send_invoice`. When charging automatically, Stripe will
* attempt to pay this subscription at the end of the cycle using the default source attached to
* the customer. When sending an invoice, Stripe will email your customer an invoice with payment
* instructions. Defaults to `charge_automatically`.
*/
@SerializedName("collection_method")
CollectionMethod collectionMethod;
/**
* The code of the coupon to apply to this subscription. A coupon applied to a subscription will
* only affect invoices created for that particular subscription.
*/
@SerializedName("coupon")
Object coupon;
/**
* Number of days a customer has to pay invoices generated by this subscription. Valid only for
* subscriptions where `collection_method` is set to `send_invoice`.
*/
@SerializedName("days_until_due")
Long daysUntilDue;
/**
* ID of the default payment method for the subscription. It must belong to the customer
* associated with the subscription. If not set, invoices will use the default payment method in
* the customer's invoice settings.
*/
@SerializedName("default_payment_method")
Object defaultPaymentMethod;
/**
* ID of the default payment source for the subscription. It must belong to the customer
* associated with the subscription and be in a chargeable state. If not set, defaults to the
* customer's default source.
*/
@SerializedName("default_source")
Object defaultSource;
/**
* The tax rates that will apply to any subscription item that does not have `tax_rates` set.
* Invoices created will have their `default_tax_rates` populated from the subscription. Pass an
* empty string to remove previously-defined tax rates.
*/
@SerializedName("default_tax_rates")
Object defaultTaxRates;
/**
* Specifies which fields in the response should be expanded.
*/
@SerializedName("expand")
List expand;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* List of subscription items, each with an attached plan.
*/
@SerializedName("items")
List- items;
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset by
* posting an empty value to them. All keys can be unset by posting an empty value to `metadata`.
*/
@SerializedName("metadata")
Map
metadata;
/**
* Indicates if a customer is on or off-session while an invoice payment is attempted.
*/
@SerializedName("off_session")
Boolean offSession;
/**
* Use `allow_incomplete` to create subscriptions with `status=incomplete` if the first invoice
* cannot be paid. Creating subscriptions with this status allows you to manage scenarios where
* additional user actions are needed to pay a subscription's invoice. For example, SCA regulation
* may require 3DS authentication to complete payment. See the [SCA Migration
* Guide](https://stripe.com/docs/billing/migration/strong-customer-authentication) for Billing to
* learn more. This is the default behavior.
*
* Use `error_if_incomplete` if you want Stripe to return an HTTP 402 status code if a
* subscription's first invoice cannot be paid. For example, if a payment method requires 3DS
* authentication due to SCA regulation and further user action is needed, this parameter does not
* create a subscription and returns an error instead. This was the default behavior for API
* versions prior to 2019-03-14. See the [changelog](https://stripe.com/docs/upgrades#2019-03-14)
* to learn more.
*/
@SerializedName("payment_behavior")
PaymentBehavior paymentBehavior;
/**
* Specifies an interval for how often to bill for any pending invoice items. It is analogous to
* calling [Create an invoice](https://stripe.com/docs/api#create_invoice) for the given
* subscription at the specified interval.
*/
@SerializedName("pending_invoice_item_interval")
Object pendingInvoiceItemInterval;
/**
* Boolean (defaults to `true`) telling us whether to [credit for unused
* time](https://stripe.com/docs/subscriptions/billing-cycle#prorations) when the billing cycle
* changes (e.g. when switching plans, resetting `billing_cycle_anchor=now`, or starting a trial),
* or if an item's `quantity` changes. If `false`, the anchor period will be free (similar to a
* trial) and no proration adjustments will be created.
*/
@SerializedName("prorate")
Boolean prorate;
/**
* Determines how to handle
* [prorations](https://stripe.com/docs/subscriptions/billing-cycle#prorations) when the billing
* cycle changes (e.g., when switching plans, resetting `billing_cycle_anchor=now`, or starting a
* trial), or if an item's `quantity` changes. The value defaults to `create_prorations`,
* indicating that proration invoice items should be created. Prorations can be disabled by
* setting the value to `none`. Passing `always_invoice` will cause an invoice to immediately be
* created for any prorations.
*/
@SerializedName("proration_behavior")
ProrationBehavior prorationBehavior;
/**
* If set, the proration will be calculated as though the subscription was updated at the given
* time. This can be used to apply exactly the same proration that was previewed with [upcoming
* invoice](#retrieve_customer_invoice) endpoint. It can also be used to implement custom
* proration logic, such as prorating by day instead of by second, by providing the time that you
* wish to use for proration calculations.
*/
@SerializedName("proration_date")
Long prorationDate;
/**
* A non-negative decimal (with at most four decimal places) between 0 and 100. This represents
* the percentage of the subscription invoice subtotal that will be calculated and added as tax to
* the final amount in each billing period. For example, a plan which charges $10/month with a
* `tax_percent` of `20.0` will charge $12 per invoice. To unset a previously-set value, pass an
* empty string. This field has been deprecated and will be removed in a future API version, for
* further information view the [migration docs](https://stripe.com/docs/billing/migration/taxes)
* for `tax_rates`.
*/
@SerializedName("tax_percent")
Object taxPercent;
/**
* If specified, the funds from the subscription's invoices will be transferred to the destination
* and the ID of the resulting transfers will be found on the resulting charges. This will be
* unset if you POST an empty value.
*/
@SerializedName("transfer_data")
Object transferData;
/**
* Unix timestamp representing the end of the trial period the customer will get before being
* charged for the first time. This will always overwrite any trials that might apply via a
* subscribed plan. If set, trial_end will override the default trial period of the plan the
* customer is being subscribed to. The special value `now` can be provided to end the customer's
* trial immediately. Can be at most two years from `billing_cycle_anchor`.
*/
@SerializedName("trial_end")
Object trialEnd;
/**
* Indicates if a plan's `trial_period_days` should be applied to the subscription. Setting
* `trial_end` per subscription is preferred, and this defaults to `false`. Setting this flag to
* `true` together with `trial_end` is not allowed.
*/
@SerializedName("trial_from_plan")
Boolean trialFromPlan;
private SubscriptionUpdateParams(BigDecimal applicationFeePercent, BillingCycleAnchor billingCycleAnchor, Object billingThresholds, Object cancelAt, Boolean cancelAtPeriodEnd, CollectionMethod collectionMethod, Object coupon, Long daysUntilDue, Object defaultPaymentMethod, Object defaultSource, Object defaultTaxRates, List expand, Map extraParams, List- items, Map
metadata, Boolean offSession, PaymentBehavior paymentBehavior, Object pendingInvoiceItemInterval, Boolean prorate, ProrationBehavior prorationBehavior, Long prorationDate, Object taxPercent, Object transferData, Object trialEnd, Boolean trialFromPlan) {
this.applicationFeePercent = applicationFeePercent;
this.billingCycleAnchor = billingCycleAnchor;
this.billingThresholds = billingThresholds;
this.cancelAt = cancelAt;
this.cancelAtPeriodEnd = cancelAtPeriodEnd;
this.collectionMethod = collectionMethod;
this.coupon = coupon;
this.daysUntilDue = daysUntilDue;
this.defaultPaymentMethod = defaultPaymentMethod;
this.defaultSource = defaultSource;
this.defaultTaxRates = defaultTaxRates;
this.expand = expand;
this.extraParams = extraParams;
this.items = items;
this.metadata = metadata;
this.offSession = offSession;
this.paymentBehavior = paymentBehavior;
this.pendingInvoiceItemInterval = pendingInvoiceItemInterval;
this.prorate = prorate;
this.prorationBehavior = prorationBehavior;
this.prorationDate = prorationDate;
this.taxPercent = taxPercent;
this.transferData = transferData;
this.trialEnd = trialEnd;
this.trialFromPlan = trialFromPlan;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private BigDecimal applicationFeePercent;
private BillingCycleAnchor billingCycleAnchor;
private Object billingThresholds;
private Object cancelAt;
private Boolean cancelAtPeriodEnd;
private CollectionMethod collectionMethod;
private Object coupon;
private Long daysUntilDue;
private Object defaultPaymentMethod;
private Object defaultSource;
private Object defaultTaxRates;
private List expand;
private Map extraParams;
private List- items;
private Map
metadata;
private Boolean offSession;
private PaymentBehavior paymentBehavior;
private Object pendingInvoiceItemInterval;
private Boolean prorate;
private ProrationBehavior prorationBehavior;
private Long prorationDate;
private Object taxPercent;
private Object transferData;
private Object trialEnd;
private Boolean trialFromPlan;
/**
* Finalize and obtain parameter instance from this builder.
*/
public SubscriptionUpdateParams build() {
return new SubscriptionUpdateParams(this.applicationFeePercent, this.billingCycleAnchor, this.billingThresholds, this.cancelAt, this.cancelAtPeriodEnd, this.collectionMethod, this.coupon, this.daysUntilDue, this.defaultPaymentMethod, this.defaultSource, this.defaultTaxRates, this.expand, this.extraParams, this.items, this.metadata, this.offSession, this.paymentBehavior, this.pendingInvoiceItemInterval, this.prorate, this.prorationBehavior, this.prorationDate, this.taxPercent, this.transferData, this.trialEnd, this.trialFromPlan);
}
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents
* the percentage of the subscription invoice subtotal that will be transferred to the
* application owner's Stripe account. The request must be made by a platform account on a
* connected account in order to set an application fee percentage. For more information, see
* the application fees
* [documentation](https://stripe.com/docs/connect/subscriptions#collecting-fees-on-subscriptions).
*/
public Builder setApplicationFeePercent(BigDecimal applicationFeePercent) {
this.applicationFeePercent = applicationFeePercent;
return this;
}
/**
* Either `now` or `unchanged`. Setting the value to `now` resets the subscription's billing
* cycle anchor to the current time. For more information, see the billing cycle
* [documentation](https://stripe.com/docs/billing/subscriptions/billing-cycle).
*/
public Builder setBillingCycleAnchor(BillingCycleAnchor billingCycleAnchor) {
this.billingCycleAnchor = billingCycleAnchor;
return this;
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. Pass an empty string to remove previously-defined thresholds.
*/
public Builder setBillingThresholds(BillingThresholds billingThresholds) {
this.billingThresholds = billingThresholds;
return this;
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. Pass an empty string to remove previously-defined thresholds.
*/
public Builder setBillingThresholds(EmptyParam billingThresholds) {
this.billingThresholds = billingThresholds;
return this;
}
/**
* A timestamp at which the subscription should cancel. If set to a date before the current
* period ends this will cause a proration if `prorate=true`.
*/
public Builder setCancelAt(Long cancelAt) {
this.cancelAt = cancelAt;
return this;
}
/**
* A timestamp at which the subscription should cancel. If set to a date before the current
* period ends this will cause a proration if `prorate=true`.
*/
public Builder setCancelAt(EmptyParam cancelAt) {
this.cancelAt = cancelAt;
return this;
}
/**
* Boolean indicating whether this subscription should cancel at the end of the current period.
*/
public Builder setCancelAtPeriodEnd(Boolean cancelAtPeriodEnd) {
this.cancelAtPeriodEnd = cancelAtPeriodEnd;
return this;
}
/**
* Either `charge_automatically`, or `send_invoice`. When charging automatically, Stripe will
* attempt to pay this subscription at the end of the cycle using the default source attached to
* the customer. When sending an invoice, Stripe will email your customer an invoice with
* payment instructions. Defaults to `charge_automatically`.
*/
public Builder setCollectionMethod(CollectionMethod collectionMethod) {
this.collectionMethod = collectionMethod;
return this;
}
/**
* The code of the coupon to apply to this subscription. A coupon applied to a subscription will
* only affect invoices created for that particular subscription.
*/
public Builder setCoupon(String coupon) {
this.coupon = coupon;
return this;
}
/**
* The code of the coupon to apply to this subscription. A coupon applied to a subscription will
* only affect invoices created for that particular subscription.
*/
public Builder setCoupon(EmptyParam coupon) {
this.coupon = coupon;
return this;
}
/**
* Number of days a customer has to pay invoices generated by this subscription. Valid only for
* subscriptions where `collection_method` is set to `send_invoice`.
*/
public Builder setDaysUntilDue(Long daysUntilDue) {
this.daysUntilDue = daysUntilDue;
return this;
}
/**
* ID of the default payment method for the subscription. It must belong to the customer
* associated with the subscription. If not set, invoices will use the default payment method in
* the customer's invoice settings.
*/
public Builder setDefaultPaymentMethod(String defaultPaymentMethod) {
this.defaultPaymentMethod = defaultPaymentMethod;
return this;
}
/**
* ID of the default payment method for the subscription. It must belong to the customer
* associated with the subscription. If not set, invoices will use the default payment method in
* the customer's invoice settings.
*/
public Builder setDefaultPaymentMethod(EmptyParam defaultPaymentMethod) {
this.defaultPaymentMethod = defaultPaymentMethod;
return this;
}
/**
* ID of the default payment source for the subscription. It must belong to the customer
* associated with the subscription and be in a chargeable state. If not set, defaults to the
* customer's default source.
*/
public Builder setDefaultSource(String defaultSource) {
this.defaultSource = defaultSource;
return this;
}
/**
* ID of the default payment source for the subscription. It must belong to the customer
* associated with the subscription and be in a chargeable state. If not set, defaults to the
* customer's default source.
*/
public Builder setDefaultSource(EmptyParam defaultSource) {
this.defaultSource = defaultSource;
return this;
}
/**
* Add an element to `defaultTaxRates` list. A list is initialized for the first `add/addAll`
* call, and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams#defaultTaxRates} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addDefaultTaxRate(String element) {
if (this.defaultTaxRates == null || this.defaultTaxRates instanceof EmptyParam) {
this.defaultTaxRates = new ArrayList();
}
((List) this.defaultTaxRates).add(element);
return this;
}
/**
* Add all elements to `defaultTaxRates` list. A list is initialized for the first `add/addAll`
* call, and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams#defaultTaxRates} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addAllDefaultTaxRate(List elements) {
if (this.defaultTaxRates == null || this.defaultTaxRates instanceof EmptyParam) {
this.defaultTaxRates = new ArrayList();
}
((List) this.defaultTaxRates).addAll(elements);
return this;
}
/**
* The tax rates that will apply to any subscription item that does not have `tax_rates` set.
* Invoices created will have their `default_tax_rates` populated from the subscription. Pass an
* empty string to remove previously-defined tax rates.
*/
public Builder setDefaultTaxRates(EmptyParam defaultTaxRates) {
this.defaultTaxRates = defaultTaxRates;
return this;
}
/**
* The tax rates that will apply to any subscription item that does not have `tax_rates` set.
* Invoices created will have their `default_tax_rates` populated from the subscription. Pass an
* empty string to remove previously-defined tax rates.
*/
public Builder setDefaultTaxRates(List defaultTaxRates) {
this.defaultTaxRates = defaultTaxRates;
return this;
}
/**
* Add an element to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams#expand} for the field documentation.
*/
public Builder addExpand(String element) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.add(element);
return this;
}
/**
* Add all elements to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams#expand} for the field documentation.
*/
public Builder addAllExpand(List elements) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.addAll(elements);
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionUpdateParams#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionUpdateParams#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Add an element to `items` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams#items} for the field documentation.
*/
public Builder addItem(Item element) {
if (this.items == null) {
this.items = new ArrayList<>();
}
this.items.add(element);
return this;
}
/**
* Add all elements to `items` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams#items} for the field documentation.
*/
public Builder addAllItem(List- elements) {
if (this.items == null) {
this.items = new ArrayList<>();
}
this.items.addAll(elements);
return this;
}
/**
* Add a key/value pair to `metadata` map. A map is initialized for the first `put/putAll` call,
* and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionUpdateParams#metadata} for the field documentation.
*/
public Builder putMetadata(String key, String value) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `metadata` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionUpdateParams#metadata} for the field documentation.
*/
public Builder putAllMetadata(Map
map) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.putAll(map);
return this;
}
/**
* Indicates if a customer is on or off-session while an invoice payment is attempted.
*/
public Builder setOffSession(Boolean offSession) {
this.offSession = offSession;
return this;
}
/**
* Use `allow_incomplete` to create subscriptions with `status=incomplete` if the first invoice
* cannot be paid. Creating subscriptions with this status allows you to manage scenarios where
* additional user actions are needed to pay a subscription's invoice. For example, SCA
* regulation may require 3DS authentication to complete payment. See the [SCA Migration
* Guide](https://stripe.com/docs/billing/migration/strong-customer-authentication) for Billing
* to learn more. This is the default behavior.
*
* Use `error_if_incomplete` if you want Stripe to return an HTTP 402 status code if a
* subscription's first invoice cannot be paid. For example, if a payment method requires 3DS
* authentication due to SCA regulation and further user action is needed, this parameter does
* not create a subscription and returns an error instead. This was the default behavior for API
* versions prior to 2019-03-14. See the
* [changelog](https://stripe.com/docs/upgrades#2019-03-14) to learn more.
*/
public Builder setPaymentBehavior(PaymentBehavior paymentBehavior) {
this.paymentBehavior = paymentBehavior;
return this;
}
/**
* Specifies an interval for how often to bill for any pending invoice items. It is analogous to
* calling [Create an invoice](https://stripe.com/docs/api#create_invoice) for the given
* subscription at the specified interval.
*/
public Builder setPendingInvoiceItemInterval(PendingInvoiceItemInterval pendingInvoiceItemInterval) {
this.pendingInvoiceItemInterval = pendingInvoiceItemInterval;
return this;
}
/**
* Specifies an interval for how often to bill for any pending invoice items. It is analogous to
* calling [Create an invoice](https://stripe.com/docs/api#create_invoice) for the given
* subscription at the specified interval.
*/
public Builder setPendingInvoiceItemInterval(EmptyParam pendingInvoiceItemInterval) {
this.pendingInvoiceItemInterval = pendingInvoiceItemInterval;
return this;
}
/**
* Boolean (defaults to `true`) telling us whether to [credit for unused
* time](https://stripe.com/docs/subscriptions/billing-cycle#prorations) when the billing cycle
* changes (e.g. when switching plans, resetting `billing_cycle_anchor=now`, or starting a
* trial), or if an item's `quantity` changes. If `false`, the anchor period will be free
* (similar to a trial) and no proration adjustments will be created.
*/
public Builder setProrate(Boolean prorate) {
this.prorate = prorate;
return this;
}
/**
* Determines how to handle
* [prorations](https://stripe.com/docs/subscriptions/billing-cycle#prorations) when the billing
* cycle changes (e.g., when switching plans, resetting `billing_cycle_anchor=now`, or starting
* a trial), or if an item's `quantity` changes. The value defaults to `create_prorations`,
* indicating that proration invoice items should be created. Prorations can be disabled by
* setting the value to `none`. Passing `always_invoice` will cause an invoice to immediately be
* created for any prorations.
*/
public Builder setProrationBehavior(ProrationBehavior prorationBehavior) {
this.prorationBehavior = prorationBehavior;
return this;
}
/**
* If set, the proration will be calculated as though the subscription was updated at the given
* time. This can be used to apply exactly the same proration that was previewed with [upcoming
* invoice](#retrieve_customer_invoice) endpoint. It can also be used to implement custom
* proration logic, such as prorating by day instead of by second, by providing the time that
* you wish to use for proration calculations.
*/
public Builder setProrationDate(Long prorationDate) {
this.prorationDate = prorationDate;
return this;
}
/**
* A non-negative decimal (with at most four decimal places) between 0 and 100. This represents
* the percentage of the subscription invoice subtotal that will be calculated and added as tax
* to the final amount in each billing period. For example, a plan which charges $10/month with
* a `tax_percent` of `20.0` will charge $12 per invoice. To unset a previously-set value, pass
* an empty string. This field has been deprecated and will be removed in a future API version,
* for further information view the [migration
* docs](https://stripe.com/docs/billing/migration/taxes) for `tax_rates`.
*/
public Builder setTaxPercent(BigDecimal taxPercent) {
this.taxPercent = taxPercent;
return this;
}
/**
* A non-negative decimal (with at most four decimal places) between 0 and 100. This represents
* the percentage of the subscription invoice subtotal that will be calculated and added as tax
* to the final amount in each billing period. For example, a plan which charges $10/month with
* a `tax_percent` of `20.0` will charge $12 per invoice. To unset a previously-set value, pass
* an empty string. This field has been deprecated and will be removed in a future API version,
* for further information view the [migration
* docs](https://stripe.com/docs/billing/migration/taxes) for `tax_rates`.
*/
public Builder setTaxPercent(EmptyParam taxPercent) {
this.taxPercent = taxPercent;
return this;
}
/**
* If specified, the funds from the subscription's invoices will be transferred to the
* destination and the ID of the resulting transfers will be found on the resulting charges.
* This will be unset if you POST an empty value.
*/
public Builder setTransferData(TransferData transferData) {
this.transferData = transferData;
return this;
}
/**
* If specified, the funds from the subscription's invoices will be transferred to the
* destination and the ID of the resulting transfers will be found on the resulting charges.
* This will be unset if you POST an empty value.
*/
public Builder setTransferData(EmptyParam transferData) {
this.transferData = transferData;
return this;
}
/**
* Unix timestamp representing the end of the trial period the customer will get before being
* charged for the first time. This will always overwrite any trials that might apply via a
* subscribed plan. If set, trial_end will override the default trial period of the plan the
* customer is being subscribed to. The special value `now` can be provided to end the
* customer's trial immediately. Can be at most two years from `billing_cycle_anchor`.
*/
public Builder setTrialEnd(TrialEnd trialEnd) {
this.trialEnd = trialEnd;
return this;
}
/**
* Unix timestamp representing the end of the trial period the customer will get before being
* charged for the first time. This will always overwrite any trials that might apply via a
* subscribed plan. If set, trial_end will override the default trial period of the plan the
* customer is being subscribed to. The special value `now` can be provided to end the
* customer's trial immediately. Can be at most two years from `billing_cycle_anchor`.
*/
public Builder setTrialEnd(Long trialEnd) {
this.trialEnd = trialEnd;
return this;
}
/**
* Indicates if a plan's `trial_period_days` should be applied to the subscription. Setting
* `trial_end` per subscription is preferred, and this defaults to `false`. Setting this flag to
* `true` together with `trial_end` is not allowed.
*/
public Builder setTrialFromPlan(Boolean trialFromPlan) {
this.trialFromPlan = trialFromPlan;
return this;
}
}
public static class BillingThresholds {
/**
* Monetary threshold that triggers the subscription to advance to a new billing period.
*/
@SerializedName("amount_gte")
Long amountGte;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Indicates if the `billing_cycle_anchor` should be reset when a threshold is reached. If true,
* `billing_cycle_anchor` will be updated to the date/time the threshold was last reached;
* otherwise, the value will remain unchanged.
*/
@SerializedName("reset_billing_cycle_anchor")
Boolean resetBillingCycleAnchor;
private BillingThresholds(Long amountGte, Map extraParams, Boolean resetBillingCycleAnchor) {
this.amountGte = amountGte;
this.extraParams = extraParams;
this.resetBillingCycleAnchor = resetBillingCycleAnchor;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Long amountGte;
private Map extraParams;
private Boolean resetBillingCycleAnchor;
/** Finalize and obtain parameter instance from this builder. */
public BillingThresholds build() {
return new BillingThresholds(this.amountGte, this.extraParams, this.resetBillingCycleAnchor);
}
/** Monetary threshold that triggers the subscription to advance to a new billing period. */
public Builder setAmountGte(Long amountGte) {
this.amountGte = amountGte;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionUpdateParams.BillingThresholds#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionUpdateParams.BillingThresholds#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
public Builder setResetBillingCycleAnchor(Boolean resetBillingCycleAnchor) {
this.resetBillingCycleAnchor = resetBillingCycleAnchor;
return this;
}
}
/**
* Monetary threshold that triggers the subscription to advance to a new billing period.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountGte() {
return this.amountGte;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Indicates if the `billing_cycle_anchor` should be reset when a threshold is reached. If true,
* `billing_cycle_anchor` will be updated to the date/time the threshold was last reached;
* otherwise, the value will remain unchanged.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getResetBillingCycleAnchor() {
return this.resetBillingCycleAnchor;
}
}
/**
* Indicates if the `billing_cycle_anchor` should be reset when a threshold is reached. If
* true, `billing_cycle_anchor` will be updated to the date/time the threshold was last
* reached; otherwise, the value will remain unchanged.
*/
public static class Item {
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. When updating, pass an empty string to remove previously-defined thresholds.
*/
@SerializedName("billing_thresholds")
Object billingThresholds;
/**
* Delete all usage for a given subscription item. Allowed only when `deleted` is set to `true`
* and the current plan's `usage_type` is `metered`.
*/
@SerializedName("clear_usage")
Boolean clearUsage;
/**
* A flag that, if set to `true`, will delete the specified item.
*/
@SerializedName("deleted")
Boolean deleted;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Subscription item to update.
*/
@SerializedName("id")
Object id;
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset
* by posting an empty value to them. All keys can be unset by posting an empty value to
* `metadata`.
*/
@SerializedName("metadata")
Map metadata;
/**
* Plan ID for this item, as a string.
*/
@SerializedName("plan")
Object plan;
/**
* Quantity for this item.
*/
@SerializedName("quantity")
Long quantity;
/**
* A list of [Tax Rate](https://stripe.com/docs/api/tax_rates) ids. These Tax Rates will
* override the
* [`default_tax_rates`](https://stripe.com/docs/api/subscriptions/create#create_subscription-default_tax_rates)
* on the Subscription. When updating, pass an empty string to remove previously-defined tax
* rates.
*/
@SerializedName("tax_rates")
Object taxRates;
private Item(Object billingThresholds, Boolean clearUsage, Boolean deleted, Map extraParams, Object id, Map metadata, Object plan, Long quantity, Object taxRates) {
this.billingThresholds = billingThresholds;
this.clearUsage = clearUsage;
this.deleted = deleted;
this.extraParams = extraParams;
this.id = id;
this.metadata = metadata;
this.plan = plan;
this.quantity = quantity;
this.taxRates = taxRates;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Object billingThresholds;
private Boolean clearUsage;
private Boolean deleted;
private Map extraParams;
private Object id;
private Map metadata;
private Object plan;
private Long quantity;
private Object taxRates;
/**
* Finalize and obtain parameter instance from this builder.
*/
public Item build() {
return new Item(this.billingThresholds, this.clearUsage, this.deleted, this.extraParams, this.id, this.metadata, this.plan, this.quantity, this.taxRates);
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. When updating, pass an empty string to remove previously-defined
* thresholds.
*/
public Builder setBillingThresholds(BillingThresholds billingThresholds) {
this.billingThresholds = billingThresholds;
return this;
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. When updating, pass an empty string to remove previously-defined
* thresholds.
*/
public Builder setBillingThresholds(EmptyParam billingThresholds) {
this.billingThresholds = billingThresholds;
return this;
}
/**
* Delete all usage for a given subscription item. Allowed only when `deleted` is set to
* `true` and the current plan's `usage_type` is `metered`.
*/
public Builder setClearUsage(Boolean clearUsage) {
this.clearUsage = clearUsage;
return this;
}
/**
* A flag that, if set to `true`, will delete the specified item.
*/
public Builder setDeleted(Boolean deleted) {
this.deleted = deleted;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionUpdateParams.Item#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionUpdateParams.Item#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Subscription item to update.
*/
public Builder setId(String id) {
this.id = id;
return this;
}
/**
* Subscription item to update.
*/
public Builder setId(EmptyParam id) {
this.id = id;
return this;
}
/**
* Add a key/value pair to `metadata` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionUpdateParams.Item#metadata} for the field documentation.
*/
public Builder putMetadata(String key, String value) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `metadata` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionUpdateParams.Item#metadata} for the field documentation.
*/
public Builder putAllMetadata(Map map) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.putAll(map);
return this;
}
/**
* Plan ID for this item, as a string.
*/
public Builder setPlan(String plan) {
this.plan = plan;
return this;
}
/**
* Plan ID for this item, as a string.
*/
public Builder setPlan(EmptyParam plan) {
this.plan = plan;
return this;
}
/**
* Quantity for this item.
*/
public Builder setQuantity(Long quantity) {
this.quantity = quantity;
return this;
}
/**
* Add an element to `taxRates` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams.Item#taxRates} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addTaxRate(String element) {
if (this.taxRates == null || this.taxRates instanceof EmptyParam) {
this.taxRates = new ArrayList();
}
((List) this.taxRates).add(element);
return this;
}
/**
* Add all elements to `taxRates` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams.Item#taxRates} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addAllTaxRate(List elements) {
if (this.taxRates == null || this.taxRates instanceof EmptyParam) {
this.taxRates = new ArrayList();
}
((List) this.taxRates).addAll(elements);
return this;
}
/**
* A list of [Tax Rate](https://stripe.com/docs/api/tax_rates) ids. These Tax Rates will
* override the
* [`default_tax_rates`](https://stripe.com/docs/api/subscriptions/create#create_subscription-default_tax_rates)
* on the Subscription. When updating, pass an empty string to remove previously-defined tax
* rates.
*/
public Builder setTaxRates(EmptyParam taxRates) {
this.taxRates = taxRates;
return this;
}
/**
* A list of [Tax Rate](https://stripe.com/docs/api/tax_rates) ids. These Tax Rates will
* override the
* [`default_tax_rates`](https://stripe.com/docs/api/subscriptions/create#create_subscription-default_tax_rates)
* on the Subscription. When updating, pass an empty string to remove previously-defined tax
* rates.
*/
public Builder setTaxRates(List taxRates) {
this.taxRates = taxRates;
return this;
}
}
public static class BillingThresholds {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/** Usage threshold that triggers the subscription to advance to a new billing period. */
@SerializedName("usage_gte")
Long usageGte;
private BillingThresholds(Map extraParams, Long usageGte) {
this.extraParams = extraParams;
this.usageGte = usageGte;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private Long usageGte;
/** Finalize and obtain parameter instance from this builder. */
public BillingThresholds build() {
return new BillingThresholds(this.extraParams, this.usageGte);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SubscriptionUpdateParams.Item.BillingThresholds#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SubscriptionUpdateParams.Item.BillingThresholds#extraParams} for the
* field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
public Builder setUsageGte(Long usageGte) {
this.usageGte = usageGte;
return this;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getUsageGte() {
return this.usageGte;
}
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. When updating, pass an empty string to remove previously-defined thresholds.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getBillingThresholds() {
return this.billingThresholds;
}
/**
* Delete all usage for a given subscription item. Allowed only when `deleted` is set to `true`
* and the current plan's `usage_type` is `metered`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getClearUsage() {
return this.clearUsage;
}
/**
* A flag that, if set to `true`, will delete the specified item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getDeleted() {
return this.deleted;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Subscription item to update.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getId() {
return this.id;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset
* by posting an empty value to them. All keys can be unset by posting an empty value to
* `metadata`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
/**
* Plan ID for this item, as a string.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getPlan() {
return this.plan;
}
/**
* Quantity for this item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getQuantity() {
return this.quantity;
}
/**
* A list of [Tax Rate](https://stripe.com/docs/api/tax_rates) ids. These Tax Rates will
* override the
* [`default_tax_rates`](https://stripe.com/docs/api/subscriptions/create#create_subscription-default_tax_rates)
* on the Subscription. When updating, pass an empty string to remove previously-defined tax
* rates.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getTaxRates() {
return this.taxRates;
}
}
/**
* Usage threshold that triggers the subscription to advance to a new billing period.
*/
public static class PendingInvoiceItemInterval {
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Specifies invoicing frequency. Either `day`, `week`, `month` or `year`.
*/
@SerializedName("interval")
Interval interval;
/**
* The number of intervals between invoices. For example, `interval=month` and
* `interval_count=3` bills every 3 months. Maximum of one year interval allowed (1 year, 12
* months, or 52 weeks).
*/
@SerializedName("interval_count")
Long intervalCount;
private PendingInvoiceItemInterval(Map extraParams, Interval interval, Long intervalCount) {
this.extraParams = extraParams;
this.interval = interval;
this.intervalCount = intervalCount;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private Interval interval;
private Long intervalCount;
/**
* Finalize and obtain parameter instance from this builder.
*/
public PendingInvoiceItemInterval build() {
return new PendingInvoiceItemInterval(this.extraParams, this.interval, this.intervalCount);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionUpdateParams.PendingInvoiceItemInterval#extraParams} for the field
* documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionUpdateParams.PendingInvoiceItemInterval#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Specifies invoicing frequency. Either `day`, `week`, `month` or `year`.
*/
public Builder setInterval(Interval interval) {
this.interval = interval;
return this;
}
/**
* The number of intervals between invoices. For example, `interval=month` and
* `interval_count=3` bills every 3 months. Maximum of one year interval allowed (1 year, 12
* months, or 52 weeks).
*/
public Builder setIntervalCount(Long intervalCount) {
this.intervalCount = intervalCount;
return this;
}
}
public enum Interval implements ApiRequestParams.EnumParam {
@SerializedName("day")
DAY("day"), @SerializedName("month")
MONTH("month"), @SerializedName("week")
WEEK("week"), @SerializedName("year")
YEAR("year");
private final String value;
Interval(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Specifies invoicing frequency. Either `day`, `week`, `month` or `year`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Interval getInterval() {
return this.interval;
}
/**
* The number of intervals between invoices. For example, `interval=month` and
* `interval_count=3` bills every 3 months. Maximum of one year interval allowed (1 year, 12
* months, or 52 weeks).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getIntervalCount() {
return this.intervalCount;
}
}
public static class TransferData {
/**
* ID of an existing, connected Stripe account.
*/
@SerializedName("destination")
Object destination;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
private TransferData(Object destination, Map extraParams) {
this.destination = destination;
this.extraParams = extraParams;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Object destination;
private Map extraParams;
/** Finalize and obtain parameter instance from this builder. */
public TransferData build() {
return new TransferData(this.destination, this.extraParams);
}
/** ID of an existing, connected Stripe account. */
public Builder setDestination(String destination) {
this.destination = destination;
return this;
}
/** ID of an existing, connected Stripe account. */
public Builder setDestination(EmptyParam destination) {
this.destination = destination;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionUpdateParams.TransferData#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
}
/**
* ID of an existing, connected Stripe account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getDestination() {
return this.destination;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionUpdateParams.TransferData#extraParams} for the field documentation.
*/
public enum BillingCycleAnchor implements ApiRequestParams.EnumParam {
@SerializedName("now")
NOW("now"), @SerializedName("unchanged")
UNCHANGED("unchanged");
private final String value;
BillingCycleAnchor(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum CollectionMethod implements ApiRequestParams.EnumParam {
@SerializedName("charge_automatically")
CHARGE_AUTOMATICALLY("charge_automatically"), @SerializedName("send_invoice")
SEND_INVOICE("send_invoice");
private final String value;
CollectionMethod(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum PaymentBehavior implements ApiRequestParams.EnumParam {
@SerializedName("allow_incomplete")
ALLOW_INCOMPLETE("allow_incomplete"), @SerializedName("error_if_incomplete")
ERROR_IF_INCOMPLETE("error_if_incomplete"), @SerializedName("pending_if_incomplete")
PENDING_IF_INCOMPLETE("pending_if_incomplete");
private final String value;
PaymentBehavior(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum ProrationBehavior implements ApiRequestParams.EnumParam {
@SerializedName("always_invoice")
ALWAYS_INVOICE("always_invoice"), @SerializedName("create_prorations")
CREATE_PRORATIONS("create_prorations"), @SerializedName("none")
NONE("none");
private final String value;
ProrationBehavior(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum TrialEnd implements ApiRequestParams.EnumParam {
@SerializedName("now")
NOW("now");
private final String value;
TrialEnd(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents the
* percentage of the subscription invoice subtotal that will be transferred to the application
* owner's Stripe account. The request must be made by a platform account on a connected account
* in order to set an application fee percentage. For more information, see the application fees
* [documentation](https://stripe.com/docs/connect/subscriptions#collecting-fees-on-subscriptions).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BigDecimal getApplicationFeePercent() {
return this.applicationFeePercent;
}
/**
* Either `now` or `unchanged`. Setting the value to `now` resets the subscription's billing cycle
* anchor to the current time. For more information, see the billing cycle
* [documentation](https://stripe.com/docs/billing/subscriptions/billing-cycle).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BillingCycleAnchor getBillingCycleAnchor() {
return this.billingCycleAnchor;
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. Pass an empty string to remove previously-defined thresholds.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getBillingThresholds() {
return this.billingThresholds;
}
/**
* A timestamp at which the subscription should cancel. If set to a date before the current period
* ends this will cause a proration if `prorate=true`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getCancelAt() {
return this.cancelAt;
}
/**
* Boolean indicating whether this subscription should cancel at the end of the current period.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getCancelAtPeriodEnd() {
return this.cancelAtPeriodEnd;
}
/**
* Either `charge_automatically`, or `send_invoice`. When charging automatically, Stripe will
* attempt to pay this subscription at the end of the cycle using the default source attached to
* the customer. When sending an invoice, Stripe will email your customer an invoice with payment
* instructions. Defaults to `charge_automatically`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public CollectionMethod getCollectionMethod() {
return this.collectionMethod;
}
/**
* The code of the coupon to apply to this subscription. A coupon applied to a subscription will
* only affect invoices created for that particular subscription.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getCoupon() {
return this.coupon;
}
/**
* Number of days a customer has to pay invoices generated by this subscription. Valid only for
* subscriptions where `collection_method` is set to `send_invoice`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDaysUntilDue() {
return this.daysUntilDue;
}
/**
* ID of the default payment method for the subscription. It must belong to the customer
* associated with the subscription. If not set, invoices will use the default payment method in
* the customer's invoice settings.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getDefaultPaymentMethod() {
return this.defaultPaymentMethod;
}
/**
* ID of the default payment source for the subscription. It must belong to the customer
* associated with the subscription and be in a chargeable state. If not set, defaults to the
* customer's default source.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getDefaultSource() {
return this.defaultSource;
}
/**
* The tax rates that will apply to any subscription item that does not have `tax_rates` set.
* Invoices created will have their `default_tax_rates` populated from the subscription. Pass an
* empty string to remove previously-defined tax rates.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getDefaultTaxRates() {
return this.defaultTaxRates;
}
/**
* Specifies which fields in the response should be expanded.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getExpand() {
return this.expand;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* List of subscription items, each with an attached plan.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List- getItems() {
return this.items;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset by
* posting an empty value to them. All keys can be unset by posting an empty value to `metadata`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map
getMetadata() {
return this.metadata;
}
/**
* Indicates if a customer is on or off-session while an invoice payment is attempted.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getOffSession() {
return this.offSession;
}
/**
* Use `allow_incomplete` to create subscriptions with `status=incomplete` if the first invoice
* cannot be paid. Creating subscriptions with this status allows you to manage scenarios where
* additional user actions are needed to pay a subscription's invoice. For example, SCA regulation
* may require 3DS authentication to complete payment. See the [SCA Migration
* Guide](https://stripe.com/docs/billing/migration/strong-customer-authentication) for Billing to
* learn more. This is the default behavior.
*
* Use `error_if_incomplete` if you want Stripe to return an HTTP 402 status code if a
* subscription's first invoice cannot be paid. For example, if a payment method requires 3DS
* authentication due to SCA regulation and further user action is needed, this parameter does not
* create a subscription and returns an error instead. This was the default behavior for API
* versions prior to 2019-03-14. See the [changelog](https://stripe.com/docs/upgrades#2019-03-14)
* to learn more.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PaymentBehavior getPaymentBehavior() {
return this.paymentBehavior;
}
/**
* Specifies an interval for how often to bill for any pending invoice items. It is analogous to
* calling [Create an invoice](https://stripe.com/docs/api#create_invoice) for the given
* subscription at the specified interval.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getPendingInvoiceItemInterval() {
return this.pendingInvoiceItemInterval;
}
/**
* Boolean (defaults to `true`) telling us whether to [credit for unused
* time](https://stripe.com/docs/subscriptions/billing-cycle#prorations) when the billing cycle
* changes (e.g. when switching plans, resetting `billing_cycle_anchor=now`, or starting a trial),
* or if an item's `quantity` changes. If `false`, the anchor period will be free (similar to a
* trial) and no proration adjustments will be created.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getProrate() {
return this.prorate;
}
/**
* Determines how to handle
* [prorations](https://stripe.com/docs/subscriptions/billing-cycle#prorations) when the billing
* cycle changes (e.g., when switching plans, resetting `billing_cycle_anchor=now`, or starting a
* trial), or if an item's `quantity` changes. The value defaults to `create_prorations`,
* indicating that proration invoice items should be created. Prorations can be disabled by
* setting the value to `none`. Passing `always_invoice` will cause an invoice to immediately be
* created for any prorations.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ProrationBehavior getProrationBehavior() {
return this.prorationBehavior;
}
/**
* If set, the proration will be calculated as though the subscription was updated at the given
* time. This can be used to apply exactly the same proration that was previewed with [upcoming
* invoice](#retrieve_customer_invoice) endpoint. It can also be used to implement custom
* proration logic, such as prorating by day instead of by second, by providing the time that you
* wish to use for proration calculations.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getProrationDate() {
return this.prorationDate;
}
/**
* A non-negative decimal (with at most four decimal places) between 0 and 100. This represents
* the percentage of the subscription invoice subtotal that will be calculated and added as tax to
* the final amount in each billing period. For example, a plan which charges $10/month with a
* `tax_percent` of `20.0` will charge $12 per invoice. To unset a previously-set value, pass an
* empty string. This field has been deprecated and will be removed in a future API version, for
* further information view the [migration docs](https://stripe.com/docs/billing/migration/taxes)
* for `tax_rates`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getTaxPercent() {
return this.taxPercent;
}
/**
* If specified, the funds from the subscription's invoices will be transferred to the destination
* and the ID of the resulting transfers will be found on the resulting charges. This will be
* unset if you POST an empty value.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getTransferData() {
return this.transferData;
}
/**
* Unix timestamp representing the end of the trial period the customer will get before being
* charged for the first time. This will always overwrite any trials that might apply via a
* subscribed plan. If set, trial_end will override the default trial period of the plan the
* customer is being subscribed to. The special value `now` can be provided to end the customer's
* trial immediately. Can be at most two years from `billing_cycle_anchor`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getTrialEnd() {
return this.trialEnd;
}
/**
* Indicates if a plan's `trial_period_days` should be applied to the subscription. Setting
* `trial_end` per subscription is preferred, and this defaults to `false`. Setting this flag to
* `true` together with `trial_end` is not allowed.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getTrialFromPlan() {
return this.trialFromPlan;
}
}