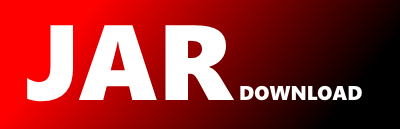
com.stripe.model.Card Maven / Gradle / Ivy
// Generated by delombok at Mon Feb 24 16:13:36 PST 2020
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.InvalidRequestException;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.CardUpdateOnAccountParams;
import com.stripe.param.CardUpdateOnCustomerParams;
import java.util.List;
import java.util.Map;
public class Card extends ApiResource implements MetadataStore, ExternalAccount, PaymentSource {
/**
* The account this card belongs to. This attribute will not be in the card object if the card
* belongs to a customer or recipient instead.
*/
@SerializedName("account")
ExpandableField account;
/**
* City/District/Suburb/Town/Village.
*/
@SerializedName("address_city")
String addressCity;
/**
* Billing address country, if provided when creating card.
*/
@SerializedName("address_country")
String addressCountry;
/**
* Address line 1 (Street address/PO Box/Company name).
*/
@SerializedName("address_line1")
String addressLine1;
/**
* If {@code address_line1} was provided, results of the check: {@code pass}, {@code fail}, {@code
* unavailable}, or {@code unchecked}.
*/
@SerializedName("address_line1_check")
String addressLine1Check;
/**
* Address line 2 (Apartment/Suite/Unit/Building).
*/
@SerializedName("address_line2")
String addressLine2;
/**
* State/County/Province/Region.
*/
@SerializedName("address_state")
String addressState;
/**
* ZIP or postal code.
*/
@SerializedName("address_zip")
String addressZip;
/**
* If {@code address_zip} was provided, results of the check: {@code pass}, {@code fail}, {@code
* unavailable}, or {@code unchecked}.
*/
@SerializedName("address_zip_check")
String addressZipCheck;
/**
* A set of available payout methods for this card. Will be either {@code ["standard"]} or {@code
* ["standard", "instant"]}. Only values from this set should be passed as the {@code method} when
* creating a transfer.
*/
@SerializedName("available_payout_methods")
List availablePayoutMethods;
/**
* Card brand. Can be {@code American Express}, {@code Diners Club}, {@code Discover}, {@code
* JCB}, {@code MasterCard}, {@code UnionPay}, {@code Visa}, or {@code Unknown}.
*/
@SerializedName("brand")
String brand;
/**
* Two-letter ISO code representing the country of the card. You could use this attribute to get a
* sense of the international breakdown of cards you've collected.
*/
@SerializedName("country")
String country;
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@SerializedName("currency")
String currency;
/**
* The customer that this card belongs to. This attribute will not be in the card object if the
* card belongs to an account or recipient instead.
*/
@SerializedName("customer")
ExpandableField customer;
/**
* If a CVC was provided, results of the check: {@code pass}, {@code fail}, {@code unavailable},
* or {@code unchecked}.
*/
@SerializedName("cvc_check")
String cvcCheck;
/**
* Whether this card is the default external account for its currency.
*/
@SerializedName("default_for_currency")
Boolean defaultForCurrency;
/**
* Always true for a deleted object.
*/
@SerializedName("deleted")
Boolean deleted;
/**
* Card description. (Only for internal use only and not typically available in standard API
* requests.)
*/
@SerializedName("description")
String description;
/**
* (For tokenized numbers only.) The last four digits of the device account number.
*/
@SerializedName("dynamic_last4")
String dynamicLast4;
/**
* Two-digit number representing the card's expiration month.
*/
@SerializedName("exp_month")
Long expMonth;
/**
* Four-digit number representing the card's expiration year.
*/
@SerializedName("exp_year")
Long expYear;
/**
* Uniquely identifies this particular card number. You can use this attribute to check whether
* two customers who've signed up with you are using the same card number, for example.
*/
@SerializedName("fingerprint")
String fingerprint;
/**
* Card funding type. Can be {@code credit}, {@code debit}, {@code prepaid}, or {@code unknown}.
*/
@SerializedName("funding")
String funding;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* Issuer identification number of the card. (Only for internal use only and not typically
* available in standard API requests.)
*/
@SerializedName("iin")
String iin;
/**
* Issuer bank name of the card. (Only for internal use only and not typically available in
* standard API requests.)
*/
@SerializedName("issuer")
String issuer;
/**
* The last four digits of the card.
*/
@SerializedName("last4")
String last4;
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* Cardholder name.
*/
@SerializedName("name")
String name;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code card}.
*/
@SerializedName("object")
String object;
/**
* The recipient that this card belongs to. This attribute will not be in the card object if the
* card belongs to a customer or account instead.
*/
@SerializedName("recipient")
ExpandableField recipient;
/**
* If the card number is tokenized, this is the method that was used. Can be {@code
* amex_express_checkout}, {@code android_pay} (includes Google Pay), {@code apple_pay}, {@code
* masterpass}, {@code visa_checkout}, or null.
*/
@SerializedName("tokenization_method")
String tokenizationMethod;
/**
* Get ID of expandable {@code account} object.
*/
public String getAccount() {
return (this.account != null) ? this.account.getId() : null;
}
public void setAccount(String id) {
this.account = ApiResource.setExpandableFieldId(id, this.account);
}
/**
* Get expanded {@code account}.
*/
public Account getAccountObject() {
return (this.account != null) ? this.account.getExpanded() : null;
}
public void setAccountObject(Account expandableObject) {
this.account = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code customer} object.
*/
public String getCustomer() {
return (this.customer != null) ? this.customer.getId() : null;
}
public void setCustomer(String id) {
this.customer = ApiResource.setExpandableFieldId(id, this.customer);
}
/**
* Get expanded {@code customer}.
*/
public Customer getCustomerObject() {
return (this.customer != null) ? this.customer.getExpanded() : null;
}
public void setCustomerObject(Customer expandableObject) {
this.customer = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code recipient} object.
*/
public String getRecipient() {
return (this.recipient != null) ? this.recipient.getId() : null;
}
public void setRecipient(String id) {
this.recipient = ApiResource.setExpandableFieldId(id, this.recipient);
}
/**
* Get expanded {@code recipient}.
*/
public Recipient getRecipientObject() {
return (this.recipient != null) ? this.recipient.getExpanded() : null;
}
public void setRecipientObject(Recipient expandableObject) {
this.recipient = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* If you need to update only some card details, like the billing address or expiration date, you
* can do so without having to re-enter the full card details. Stripe also works directly with
* card networks so that your customers can continue using your
* service without interruption.
*
* Updates a specified card for a given customer.
*/
@Override
public Card update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* If you need to update only some card details, like the billing address or expiration date, you
* can do so without having to re-enter the full card details. Stripe also works directly with
* card networks so that your customers can continue using your
* service without interruption.
*
* Updates a specified card for a given customer.
*/
@Override
public Card update(Map params, RequestOptions options) throws StripeException {
String url;
if (this.getAccount() != null) {
url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/accounts/%s/external_accounts/%s", ApiResource.urlEncodeId(this.getAccount()), ApiResource.urlEncodeId(this.getId())));
} else if (this.getCustomer() != null) {
url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/customers/%s/sources/%s", ApiResource.urlEncodeId(this.getCustomer()), ApiResource.urlEncodeId(this.getId())));
} else {
throw new InvalidRequestException("Unable to construct url because [account, customer] field(s) are all null", null, null, null, 0, null);
}
return request(ApiResource.RequestMethod.POST, url, params, Card.class, options);
}
/**
* If you need to update only some card details, like the billing address or expiration date, you
* can do so without having to re-enter the full card details. Stripe also works directly with
* card networks so that your customers can continue using your
* service without interruption.
*
* Updates a specified card for a given customer.
*/
public Card update(CardUpdateOnAccountParams params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* If you need to update only some card details, like the billing address or expiration date, you
* can do so without having to re-enter the full card details. Stripe also works directly with
* card networks so that your customers can continue using your
* service without interruption.
*
*
Updates a specified card for a given customer.
*/
public Card update(CardUpdateOnAccountParams params, RequestOptions options) throws StripeException {
String url;
if (this.getAccount() != null) {
url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/accounts/%s/external_accounts/%s", ApiResource.urlEncodeId(this.getAccount()), ApiResource.urlEncodeId(this.getId())));
} else {
throw new InvalidRequestException("Unable to construct url because [account] field(s) are all null", null, null, null, 0, null);
}
return request(ApiResource.RequestMethod.POST, url, params, Card.class, options);
}
/**
* If you need to update only some card details, like the billing address or expiration date, you
* can do so without having to re-enter the full card details. Stripe also works directly with
* card networks so that your customers can continue using your
* service without interruption.
*
*
Updates a specified card for a given customer.
*/
public Card update(CardUpdateOnCustomerParams params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* If you need to update only some card details, like the billing address or expiration date, you
* can do so without having to re-enter the full card details. Stripe also works directly with
* card networks so that your customers can continue using your
* service without interruption.
*
*
Updates a specified card for a given customer.
*/
public Card update(CardUpdateOnCustomerParams params, RequestOptions options) throws StripeException {
String url;
if (this.getCustomer() != null) {
url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/customers/%s/sources/%s", ApiResource.urlEncodeId(this.getCustomer()), ApiResource.urlEncodeId(this.getId())));
} else {
throw new InvalidRequestException("Unable to construct url because [customer] field(s) are all null", null, null, null, 0, null);
}
return request(ApiResource.RequestMethod.POST, url, params, Card.class, options);
}
/**
* Delete a specified external account for a given account.
*
*
Delete a specified source for a given customer.
*/
@Override
public Card delete() throws StripeException {
return delete((Map) null, (RequestOptions) null);
}
/**
* Delete a specified external account for a given account.
*
* Delete a specified source for a given customer.
*/
@Override
public Card delete(RequestOptions options) throws StripeException {
return delete((Map) null, options);
}
/**
* Delete a specified external account for a given account.
*
* Delete a specified source for a given customer.
*/
@Override
public Card delete(Map params) throws StripeException {
return delete(params, (RequestOptions) null);
}
/**
* Delete a specified external account for a given account.
*
* Delete a specified source for a given customer.
*/
@Override
public Card delete(Map params, RequestOptions options) throws StripeException {
String url;
if (this.getAccount() != null) {
url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/accounts/%s/external_accounts/%s", ApiResource.urlEncodeId(this.getAccount()), ApiResource.urlEncodeId(this.getId())));
} else if (this.getCustomer() != null) {
url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/customers/%s/sources/%s", ApiResource.urlEncodeId(this.getCustomer()), ApiResource.urlEncodeId(this.getId())));
} else {
throw new InvalidRequestException("Unable to construct url because [account, customer] field(s) are all null", null, null, null, 0, null);
}
return request(ApiResource.RequestMethod.DELETE, url, params, Card.class, options);
}
/**
* City/District/Suburb/Town/Village.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAddressCity() {
return this.addressCity;
}
/**
* Billing address country, if provided when creating card.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAddressCountry() {
return this.addressCountry;
}
/**
* Address line 1 (Street address/PO Box/Company name).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAddressLine1() {
return this.addressLine1;
}
/**
* If {@code address_line1} was provided, results of the check: {@code pass}, {@code fail}, {@code
* unavailable}, or {@code unchecked}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAddressLine1Check() {
return this.addressLine1Check;
}
/**
* Address line 2 (Apartment/Suite/Unit/Building).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAddressLine2() {
return this.addressLine2;
}
/**
* State/County/Province/Region.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAddressState() {
return this.addressState;
}
/**
* ZIP or postal code.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAddressZip() {
return this.addressZip;
}
/**
* If {@code address_zip} was provided, results of the check: {@code pass}, {@code fail}, {@code
* unavailable}, or {@code unchecked}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAddressZipCheck() {
return this.addressZipCheck;
}
/**
* A set of available payout methods for this card. Will be either {@code ["standard"]} or {@code
* ["standard", "instant"]}. Only values from this set should be passed as the {@code method} when
* creating a transfer.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getAvailablePayoutMethods() {
return this.availablePayoutMethods;
}
/**
* Card brand. Can be {@code American Express}, {@code Diners Club}, {@code Discover}, {@code
* JCB}, {@code MasterCard}, {@code UnionPay}, {@code Visa}, or {@code Unknown}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBrand() {
return this.brand;
}
/**
* Two-letter ISO code representing the country of the card. You could use this attribute to get a
* sense of the international breakdown of cards you've collected.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCountry() {
return this.country;
}
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
/**
* If a CVC was provided, results of the check: {@code pass}, {@code fail}, {@code unavailable},
* or {@code unchecked}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCvcCheck() {
return this.cvcCheck;
}
/**
* Whether this card is the default external account for its currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getDefaultForCurrency() {
return this.defaultForCurrency;
}
/**
* Always true for a deleted object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getDeleted() {
return this.deleted;
}
/**
* Card description. (Only for internal use only and not typically available in standard API
* requests.)
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDescription() {
return this.description;
}
/**
* (For tokenized numbers only.) The last four digits of the device account number.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDynamicLast4() {
return this.dynamicLast4;
}
/**
* Two-digit number representing the card's expiration month.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getExpMonth() {
return this.expMonth;
}
/**
* Four-digit number representing the card's expiration year.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getExpYear() {
return this.expYear;
}
/**
* Uniquely identifies this particular card number. You can use this attribute to check whether
* two customers who've signed up with you are using the same card number, for example.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFingerprint() {
return this.fingerprint;
}
/**
* Card funding type. Can be {@code credit}, {@code debit}, {@code prepaid}, or {@code unknown}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFunding() {
return this.funding;
}
/**
* Issuer identification number of the card. (Only for internal use only and not typically
* available in standard API requests.)
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getIin() {
return this.iin;
}
/**
* Issuer bank name of the card. (Only for internal use only and not typically available in
* standard API requests.)
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getIssuer() {
return this.issuer;
}
/**
* The last four digits of the card.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLast4() {
return this.last4;
}
/**
* Cardholder name.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getName() {
return this.name;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code card}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* If the card number is tokenized, this is the method that was used. Can be {@code
* amex_express_checkout}, {@code android_pay} (includes Google Pay), {@code apple_pay}, {@code
* masterpass}, {@code visa_checkout}, or null.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTokenizationMethod() {
return this.tokenizationMethod;
}
/**
* City/District/Suburb/Town/Village.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddressCity(final String addressCity) {
this.addressCity = addressCity;
}
/**
* Billing address country, if provided when creating card.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddressCountry(final String addressCountry) {
this.addressCountry = addressCountry;
}
/**
* Address line 1 (Street address/PO Box/Company name).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddressLine1(final String addressLine1) {
this.addressLine1 = addressLine1;
}
/**
* If {@code address_line1} was provided, results of the check: {@code pass}, {@code fail}, {@code
* unavailable}, or {@code unchecked}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddressLine1Check(final String addressLine1Check) {
this.addressLine1Check = addressLine1Check;
}
/**
* Address line 2 (Apartment/Suite/Unit/Building).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddressLine2(final String addressLine2) {
this.addressLine2 = addressLine2;
}
/**
* State/County/Province/Region.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddressState(final String addressState) {
this.addressState = addressState;
}
/**
* ZIP or postal code.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddressZip(final String addressZip) {
this.addressZip = addressZip;
}
/**
* If {@code address_zip} was provided, results of the check: {@code pass}, {@code fail}, {@code
* unavailable}, or {@code unchecked}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddressZipCheck(final String addressZipCheck) {
this.addressZipCheck = addressZipCheck;
}
/**
* A set of available payout methods for this card. Will be either {@code ["standard"]} or {@code
* ["standard", "instant"]}. Only values from this set should be passed as the {@code method} when
* creating a transfer.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAvailablePayoutMethods(final List availablePayoutMethods) {
this.availablePayoutMethods = availablePayoutMethods;
}
/**
* Card brand. Can be {@code American Express}, {@code Diners Club}, {@code Discover}, {@code
* JCB}, {@code MasterCard}, {@code UnionPay}, {@code Visa}, or {@code Unknown}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBrand(final String brand) {
this.brand = brand;
}
/**
* Two-letter ISO code representing the country of the card. You could use this attribute to get a
* sense of the international breakdown of cards you've collected.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCountry(final String country) {
this.country = country;
}
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
/**
* If a CVC was provided, results of the check: {@code pass}, {@code fail}, {@code unavailable},
* or {@code unchecked}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCvcCheck(final String cvcCheck) {
this.cvcCheck = cvcCheck;
}
/**
* Whether this card is the default external account for its currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDefaultForCurrency(final Boolean defaultForCurrency) {
this.defaultForCurrency = defaultForCurrency;
}
/**
* Always true for a deleted object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDeleted(final Boolean deleted) {
this.deleted = deleted;
}
/**
* Card description. (Only for internal use only and not typically available in standard API
* requests.)
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDescription(final String description) {
this.description = description;
}
/**
* (For tokenized numbers only.) The last four digits of the device account number.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDynamicLast4(final String dynamicLast4) {
this.dynamicLast4 = dynamicLast4;
}
/**
* Two-digit number representing the card's expiration month.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setExpMonth(final Long expMonth) {
this.expMonth = expMonth;
}
/**
* Four-digit number representing the card's expiration year.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setExpYear(final Long expYear) {
this.expYear = expYear;
}
/**
* Uniquely identifies this particular card number. You can use this attribute to check whether
* two customers who've signed up with you are using the same card number, for example.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFingerprint(final String fingerprint) {
this.fingerprint = fingerprint;
}
/**
* Card funding type. Can be {@code credit}, {@code debit}, {@code prepaid}, or {@code unknown}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFunding(final String funding) {
this.funding = funding;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* Issuer identification number of the card. (Only for internal use only and not typically
* available in standard API requests.)
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIin(final String iin) {
this.iin = iin;
}
/**
* Issuer bank name of the card. (Only for internal use only and not typically available in
* standard API requests.)
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIssuer(final String issuer) {
this.issuer = issuer;
}
/**
* The last four digits of the card.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLast4(final String last4) {
this.last4 = last4;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
/**
* Cardholder name.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setName(final String name) {
this.name = name;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code card}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* If the card number is tokenized, this is the method that was used. Can be {@code
* amex_express_checkout}, {@code android_pay} (includes Google Pay), {@code apple_pay}, {@code
* masterpass}, {@code visa_checkout}, or null.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTokenizationMethod(final String tokenizationMethod) {
this.tokenizationMethod = tokenizationMethod;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Card)) return false;
final Card other = (Card) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$account = this.getAccount();
final java.lang.Object other$account = other.getAccount();
if (this$account == null ? other$account != null : !this$account.equals(other$account)) return false;
final java.lang.Object this$addressCity = this.getAddressCity();
final java.lang.Object other$addressCity = other.getAddressCity();
if (this$addressCity == null ? other$addressCity != null : !this$addressCity.equals(other$addressCity)) return false;
final java.lang.Object this$addressCountry = this.getAddressCountry();
final java.lang.Object other$addressCountry = other.getAddressCountry();
if (this$addressCountry == null ? other$addressCountry != null : !this$addressCountry.equals(other$addressCountry)) return false;
final java.lang.Object this$addressLine1 = this.getAddressLine1();
final java.lang.Object other$addressLine1 = other.getAddressLine1();
if (this$addressLine1 == null ? other$addressLine1 != null : !this$addressLine1.equals(other$addressLine1)) return false;
final java.lang.Object this$addressLine1Check = this.getAddressLine1Check();
final java.lang.Object other$addressLine1Check = other.getAddressLine1Check();
if (this$addressLine1Check == null ? other$addressLine1Check != null : !this$addressLine1Check.equals(other$addressLine1Check)) return false;
final java.lang.Object this$addressLine2 = this.getAddressLine2();
final java.lang.Object other$addressLine2 = other.getAddressLine2();
if (this$addressLine2 == null ? other$addressLine2 != null : !this$addressLine2.equals(other$addressLine2)) return false;
final java.lang.Object this$addressState = this.getAddressState();
final java.lang.Object other$addressState = other.getAddressState();
if (this$addressState == null ? other$addressState != null : !this$addressState.equals(other$addressState)) return false;
final java.lang.Object this$addressZip = this.getAddressZip();
final java.lang.Object other$addressZip = other.getAddressZip();
if (this$addressZip == null ? other$addressZip != null : !this$addressZip.equals(other$addressZip)) return false;
final java.lang.Object this$addressZipCheck = this.getAddressZipCheck();
final java.lang.Object other$addressZipCheck = other.getAddressZipCheck();
if (this$addressZipCheck == null ? other$addressZipCheck != null : !this$addressZipCheck.equals(other$addressZipCheck)) return false;
final java.lang.Object this$availablePayoutMethods = this.getAvailablePayoutMethods();
final java.lang.Object other$availablePayoutMethods = other.getAvailablePayoutMethods();
if (this$availablePayoutMethods == null ? other$availablePayoutMethods != null : !this$availablePayoutMethods.equals(other$availablePayoutMethods)) return false;
final java.lang.Object this$brand = this.getBrand();
final java.lang.Object other$brand = other.getBrand();
if (this$brand == null ? other$brand != null : !this$brand.equals(other$brand)) return false;
final java.lang.Object this$country = this.getCountry();
final java.lang.Object other$country = other.getCountry();
if (this$country == null ? other$country != null : !this$country.equals(other$country)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
final java.lang.Object this$customer = this.getCustomer();
final java.lang.Object other$customer = other.getCustomer();
if (this$customer == null ? other$customer != null : !this$customer.equals(other$customer)) return false;
final java.lang.Object this$cvcCheck = this.getCvcCheck();
final java.lang.Object other$cvcCheck = other.getCvcCheck();
if (this$cvcCheck == null ? other$cvcCheck != null : !this$cvcCheck.equals(other$cvcCheck)) return false;
final java.lang.Object this$defaultForCurrency = this.getDefaultForCurrency();
final java.lang.Object other$defaultForCurrency = other.getDefaultForCurrency();
if (this$defaultForCurrency == null ? other$defaultForCurrency != null : !this$defaultForCurrency.equals(other$defaultForCurrency)) return false;
final java.lang.Object this$deleted = this.getDeleted();
final java.lang.Object other$deleted = other.getDeleted();
if (this$deleted == null ? other$deleted != null : !this$deleted.equals(other$deleted)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$dynamicLast4 = this.getDynamicLast4();
final java.lang.Object other$dynamicLast4 = other.getDynamicLast4();
if (this$dynamicLast4 == null ? other$dynamicLast4 != null : !this$dynamicLast4.equals(other$dynamicLast4)) return false;
final java.lang.Object this$expMonth = this.getExpMonth();
final java.lang.Object other$expMonth = other.getExpMonth();
if (this$expMonth == null ? other$expMonth != null : !this$expMonth.equals(other$expMonth)) return false;
final java.lang.Object this$expYear = this.getExpYear();
final java.lang.Object other$expYear = other.getExpYear();
if (this$expYear == null ? other$expYear != null : !this$expYear.equals(other$expYear)) return false;
final java.lang.Object this$fingerprint = this.getFingerprint();
final java.lang.Object other$fingerprint = other.getFingerprint();
if (this$fingerprint == null ? other$fingerprint != null : !this$fingerprint.equals(other$fingerprint)) return false;
final java.lang.Object this$funding = this.getFunding();
final java.lang.Object other$funding = other.getFunding();
if (this$funding == null ? other$funding != null : !this$funding.equals(other$funding)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$iin = this.getIin();
final java.lang.Object other$iin = other.getIin();
if (this$iin == null ? other$iin != null : !this$iin.equals(other$iin)) return false;
final java.lang.Object this$issuer = this.getIssuer();
final java.lang.Object other$issuer = other.getIssuer();
if (this$issuer == null ? other$issuer != null : !this$issuer.equals(other$issuer)) return false;
final java.lang.Object this$last4 = this.getLast4();
final java.lang.Object other$last4 = other.getLast4();
if (this$last4 == null ? other$last4 != null : !this$last4.equals(other$last4)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$recipient = this.getRecipient();
final java.lang.Object other$recipient = other.getRecipient();
if (this$recipient == null ? other$recipient != null : !this$recipient.equals(other$recipient)) return false;
final java.lang.Object this$tokenizationMethod = this.getTokenizationMethod();
final java.lang.Object other$tokenizationMethod = other.getTokenizationMethod();
if (this$tokenizationMethod == null ? other$tokenizationMethod != null : !this$tokenizationMethod.equals(other$tokenizationMethod)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Card;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $account = this.getAccount();
result = result * PRIME + ($account == null ? 43 : $account.hashCode());
final java.lang.Object $addressCity = this.getAddressCity();
result = result * PRIME + ($addressCity == null ? 43 : $addressCity.hashCode());
final java.lang.Object $addressCountry = this.getAddressCountry();
result = result * PRIME + ($addressCountry == null ? 43 : $addressCountry.hashCode());
final java.lang.Object $addressLine1 = this.getAddressLine1();
result = result * PRIME + ($addressLine1 == null ? 43 : $addressLine1.hashCode());
final java.lang.Object $addressLine1Check = this.getAddressLine1Check();
result = result * PRIME + ($addressLine1Check == null ? 43 : $addressLine1Check.hashCode());
final java.lang.Object $addressLine2 = this.getAddressLine2();
result = result * PRIME + ($addressLine2 == null ? 43 : $addressLine2.hashCode());
final java.lang.Object $addressState = this.getAddressState();
result = result * PRIME + ($addressState == null ? 43 : $addressState.hashCode());
final java.lang.Object $addressZip = this.getAddressZip();
result = result * PRIME + ($addressZip == null ? 43 : $addressZip.hashCode());
final java.lang.Object $addressZipCheck = this.getAddressZipCheck();
result = result * PRIME + ($addressZipCheck == null ? 43 : $addressZipCheck.hashCode());
final java.lang.Object $availablePayoutMethods = this.getAvailablePayoutMethods();
result = result * PRIME + ($availablePayoutMethods == null ? 43 : $availablePayoutMethods.hashCode());
final java.lang.Object $brand = this.getBrand();
result = result * PRIME + ($brand == null ? 43 : $brand.hashCode());
final java.lang.Object $country = this.getCountry();
result = result * PRIME + ($country == null ? 43 : $country.hashCode());
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
final java.lang.Object $customer = this.getCustomer();
result = result * PRIME + ($customer == null ? 43 : $customer.hashCode());
final java.lang.Object $cvcCheck = this.getCvcCheck();
result = result * PRIME + ($cvcCheck == null ? 43 : $cvcCheck.hashCode());
final java.lang.Object $defaultForCurrency = this.getDefaultForCurrency();
result = result * PRIME + ($defaultForCurrency == null ? 43 : $defaultForCurrency.hashCode());
final java.lang.Object $deleted = this.getDeleted();
result = result * PRIME + ($deleted == null ? 43 : $deleted.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $dynamicLast4 = this.getDynamicLast4();
result = result * PRIME + ($dynamicLast4 == null ? 43 : $dynamicLast4.hashCode());
final java.lang.Object $expMonth = this.getExpMonth();
result = result * PRIME + ($expMonth == null ? 43 : $expMonth.hashCode());
final java.lang.Object $expYear = this.getExpYear();
result = result * PRIME + ($expYear == null ? 43 : $expYear.hashCode());
final java.lang.Object $fingerprint = this.getFingerprint();
result = result * PRIME + ($fingerprint == null ? 43 : $fingerprint.hashCode());
final java.lang.Object $funding = this.getFunding();
result = result * PRIME + ($funding == null ? 43 : $funding.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $iin = this.getIin();
result = result * PRIME + ($iin == null ? 43 : $iin.hashCode());
final java.lang.Object $issuer = this.getIssuer();
result = result * PRIME + ($issuer == null ? 43 : $issuer.hashCode());
final java.lang.Object $last4 = this.getLast4();
result = result * PRIME + ($last4 == null ? 43 : $last4.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $recipient = this.getRecipient();
result = result * PRIME + ($recipient == null ? 43 : $recipient.hashCode());
final java.lang.Object $tokenizationMethod = this.getTokenizationMethod();
result = result * PRIME + ($tokenizationMethod == null ? 43 : $tokenizationMethod.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
}