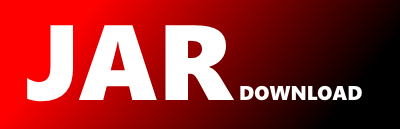
com.stripe.model.ThreeDSecure Maven / Gradle / Ivy
// Generated by delombok at Mon Feb 24 16:13:36 PST 2020
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.ThreeDSecureCreateParams;
import com.stripe.param.ThreeDSecureRetrieveParams;
import java.util.Map;
public class ThreeDSecure extends ApiResource implements HasId {
/**
* Amount of the charge that you will create when authentication completes.
*/
@SerializedName("amount")
Long amount;
/**
* True if the cardholder went through the authentication flow and their bank indicated that
* authentication succeeded.
*/
@SerializedName("authenticated")
Boolean authenticated;
/**
* You can store multiple cards on a customer in order to charge the customer later. You can also
* store multiple debit cards on a recipient in order to transfer to those cards later.
*
* Related guide: Card Payments with
* Sources.
*/
@SerializedName("card")
Card card;
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@SerializedName("currency")
String currency;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code three_d_secure}.
*/
@SerializedName("object")
String object;
/**
* If present, this is the URL that you should send the cardholder to for authentication. If you
* are going to use Stripe.js to display the authentication page in an iframe, you should use the
* value "_callback".
*/
@SerializedName("redirect_url")
String redirectUrl;
/**
* Possible values are {@code redirect_pending}, {@code succeeded}, or {@code failed}. When the
* cardholder can be authenticated, the object starts with status {@code redirect_pending}. When
* liability will be shifted to the cardholder's bank (either because the cardholder was
* successfully authenticated, or because the bank has not implemented 3D Secure, the object wlil
* be in status {@code succeeded}. {@code failed} indicates that authentication was attempted
* unsuccessfully.
*/
@SerializedName("status")
String status;
/**
* Retrieves a 3D Secure object.
*/
public static ThreeDSecure retrieve(String threeDSecure) throws StripeException {
return retrieve(threeDSecure, (Map) null, (RequestOptions) null);
}
/**
* Retrieves a 3D Secure object.
*/
public static ThreeDSecure retrieve(String threeDSecure, RequestOptions options) throws StripeException {
return retrieve(threeDSecure, (Map) null, options);
}
/**
* Retrieves a 3D Secure object.
*/
public static ThreeDSecure retrieve(String threeDSecure, Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/3d_secure/%s", ApiResource.urlEncodeId(threeDSecure)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, ThreeDSecure.class, options);
}
/**
* Retrieves a 3D Secure object.
*/
public static ThreeDSecure retrieve(String threeDSecure, ThreeDSecureRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/3d_secure/%s", ApiResource.urlEncodeId(threeDSecure)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, ThreeDSecure.class, options);
}
/**
* Initiate 3D Secure authentication.
*/
public static ThreeDSecure create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Initiate 3D Secure authentication.
*/
public static ThreeDSecure create(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/3d_secure");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, ThreeDSecure.class, options);
}
/**
* Initiate 3D Secure authentication.
*/
public static ThreeDSecure create(ThreeDSecureCreateParams params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Initiate 3D Secure authentication.
*/
public static ThreeDSecure create(ThreeDSecureCreateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/3d_secure");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, ThreeDSecure.class, options);
}
/**
* Amount of the charge that you will create when authentication completes.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
/**
* True if the cardholder went through the authentication flow and their bank indicated that
* authentication succeeded.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getAuthenticated() {
return this.authenticated;
}
/**
* You can store multiple cards on a customer in order to charge the customer later. You can also
* store multiple debit cards on a recipient in order to transfer to those cards later.
*
* Related guide: Card Payments with
* Sources.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Card getCard() {
return this.card;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code three_d_secure}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* If present, this is the URL that you should send the cardholder to for authentication. If you
* are going to use Stripe.js to display the authentication page in an iframe, you should use the
* value "_callback".
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getRedirectUrl() {
return this.redirectUrl;
}
/**
* Possible values are {@code redirect_pending}, {@code succeeded}, or {@code failed}. When the
* cardholder can be authenticated, the object starts with status {@code redirect_pending}. When
* liability will be shifted to the cardholder's bank (either because the cardholder was
* successfully authenticated, or because the bank has not implemented 3D Secure, the object wlil
* be in status {@code succeeded}. {@code failed} indicates that authentication was attempted
* unsuccessfully.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
/**
* Amount of the charge that you will create when authentication completes.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmount(final Long amount) {
this.amount = amount;
}
/**
* True if the cardholder went through the authentication flow and their bank indicated that
* authentication succeeded.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAuthenticated(final Boolean authenticated) {
this.authenticated = authenticated;
}
/**
* You can store multiple cards on a customer in order to charge the customer later. You can also
* store multiple debit cards on a recipient in order to transfer to those cards later.
*
*
Related guide: Card Payments with
* Sources.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCard(final Card card) {
this.card = card;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code three_d_secure}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* If present, this is the URL that you should send the cardholder to for authentication. If you
* are going to use Stripe.js to display the authentication page in an iframe, you should use the
* value "_callback".
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRedirectUrl(final String redirectUrl) {
this.redirectUrl = redirectUrl;
}
/**
* Possible values are {@code redirect_pending}, {@code succeeded}, or {@code failed}. When the
* cardholder can be authenticated, the object starts with status {@code redirect_pending}. When
* liability will be shifted to the cardholder's bank (either because the cardholder was
* successfully authenticated, or because the bank has not implemented 3D Secure, the object wlil
* be in status {@code succeeded}. {@code failed} indicates that authentication was attempted
* unsuccessfully.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof ThreeDSecure)) return false;
final ThreeDSecure other = (ThreeDSecure) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$authenticated = this.getAuthenticated();
final java.lang.Object other$authenticated = other.getAuthenticated();
if (this$authenticated == null ? other$authenticated != null : !this$authenticated.equals(other$authenticated)) return false;
final java.lang.Object this$card = this.getCard();
final java.lang.Object other$card = other.getCard();
if (this$card == null ? other$card != null : !this$card.equals(other$card)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$redirectUrl = this.getRedirectUrl();
final java.lang.Object other$redirectUrl = other.getRedirectUrl();
if (this$redirectUrl == null ? other$redirectUrl != null : !this$redirectUrl.equals(other$redirectUrl)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof ThreeDSecure;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $authenticated = this.getAuthenticated();
result = result * PRIME + ($authenticated == null ? 43 : $authenticated.hashCode());
final java.lang.Object $card = this.getCard();
result = result * PRIME + ($card == null ? 43 : $card.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $redirectUrl = this.getRedirectUrl();
result = result * PRIME + ($redirectUrl == null ? 43 : $redirectUrl.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
}