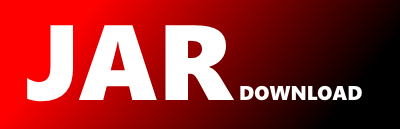
com.stripe.param.SubscriptionCreateParams Maven / Gradle / Ivy
// Generated by delombok at Mon Feb 24 16:13:36 PST 2020
package com.stripe.param;
import com.google.gson.annotations.SerializedName;
import com.stripe.net.ApiRequestParams;
import com.stripe.param.common.EmptyParam;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class SubscriptionCreateParams extends ApiRequestParams {
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents the
* percentage of the subscription invoice subtotal that will be transferred to the application
* owner's Stripe account. The request must be made by a platform account on a connected account
* in order to set an application fee percentage. For more information, see the application fees
* documentation.
*/
@SerializedName("application_fee_percent")
BigDecimal applicationFeePercent;
/**
* For new subscriptions, a past timestamp to backdate the subscription's start date to. If set,
* the first invoice will contain a proration for the timespan between the start date and the
* current time. Can be combined with trials and the billing cycle anchor.
*/
@SerializedName("backdate_start_date")
Long backdateStartDate;
/**
* A future timestamp to anchor the subscription's billing cycle. This is used to
* determine the date of the first full invoice, and, for plans with {@code month} or {@code year}
* intervals, the day of the month for subsequent invoices.
*/
@SerializedName("billing_cycle_anchor")
Long billingCycleAnchor;
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. Pass an empty string to remove previously-defined thresholds.
*/
@SerializedName("billing_thresholds")
Object billingThresholds;
/**
* A timestamp at which the subscription should cancel. If set to a date before the current period
* ends, this will cause a proration if prorations have been enabled using {@code
* proration_behavior}. If set during a future period, this will always cause a proration for that
* period.
*/
@SerializedName("cancel_at")
Long cancelAt;
/**
* Boolean indicating whether this subscription should cancel at the end of the current period.
*/
@SerializedName("cancel_at_period_end")
Boolean cancelAtPeriodEnd;
/**
* Either {@code charge_automatically}, or {@code send_invoice}. When charging automatically,
* Stripe will attempt to pay this subscription at the end of the cycle using the default source
* attached to the customer. When sending an invoice, Stripe will email your customer an invoice
* with payment instructions. Defaults to {@code charge_automatically}.
*/
@SerializedName("collection_method")
CollectionMethod collectionMethod;
/**
* The code of the coupon to apply to this subscription. A coupon applied to a subscription will
* only affect invoices created for that particular subscription.
*/
@SerializedName("coupon")
String coupon;
/**
* The identifier of the customer to subscribe.
*/
@SerializedName("customer")
String customer;
/**
* Number of days a customer has to pay invoices generated by this subscription. Valid only for
* subscriptions where {@code collection_method} is set to {@code send_invoice}.
*/
@SerializedName("days_until_due")
Long daysUntilDue;
/**
* ID of the default payment method for the subscription. It must belong to the customer
* associated with the subscription. If not set, invoices will use the default payment method in
* the customer's invoice settings.
*/
@SerializedName("default_payment_method")
String defaultPaymentMethod;
/**
* ID of the default payment source for the subscription. It must belong to the customer
* associated with the subscription and be in a chargeable state. If not set, defaults to the
* customer's default source.
*/
@SerializedName("default_source")
String defaultSource;
/**
* The tax rates that will apply to any subscription item that does not have {@code tax_rates}
* set. Invoices created will have their {@code default_tax_rates} populated from the
* subscription.
*/
@SerializedName("default_tax_rates")
Object defaultTaxRates;
/**
* Specifies which fields in the response should be expanded.
*/
@SerializedName("expand")
List expand;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* A list of up to 20 subscription items, each with an attached plan.
*/
@SerializedName("items")
List- items;
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset by
* posting an empty value to them. All keys can be unset by posting an empty value to {@code
* metadata}.
*/
@SerializedName("metadata")
Map
metadata;
/**
* Indicates if a customer is on or off-session while an invoice payment is attempted.
*/
@SerializedName("off_session")
Boolean offSession;
/**
* Use {@code allow_incomplete} to create subscriptions with {@code status=incomplete} if the
* first invoice cannot be paid. Creating subscriptions with this status allows you to manage
* scenarios where additional user actions are needed to pay a subscription's invoice. For
* example, SCA regulation may require 3DS authentication to complete payment. See the SCA Migration
* Guide for Billing to learn more. This is the default behavior.
*
* Use {@code error_if_incomplete} if you want Stripe to return an HTTP 402 status code if a
* subscription's first invoice cannot be paid. For example, if a payment method requires 3DS
* authentication due to SCA regulation and further user action is needed, this parameter does not
* create a subscription and returns an error instead. This was the default behavior for API
* versions prior to 2019-03-14. See the changelog to learn more.
*/
@SerializedName("payment_behavior")
PaymentBehavior paymentBehavior;
/**
* Specifies an interval for how often to bill for any pending invoice items. It is analogous to
* calling Create an invoice for the
* given subscription at the specified interval.
*/
@SerializedName("pending_invoice_item_interval")
Object pendingInvoiceItemInterval;
/**
* This field has been renamed to {@code proration_behavior}. {@code prorate=true} can be replaced
* with {@code proration_behavior=create_prorations} and {@code prorate=false} can be replaced
* with {@code proration_behavior=none}.
*/
@SerializedName("prorate")
Boolean prorate;
/**
* Determines how to handle prorations resulting
* from the {@code billing_cycle_anchor}. Valid values are {@code create_prorations} or {@code
* none}.
*
*
Passing {@code create_prorations} will cause proration invoice items to be created when
* applicable. Prorations can be disabled by passing {@code none}. If no value is passed, the
* default is {@code create_prorations}.
*/
@SerializedName("proration_behavior")
ProrationBehavior prorationBehavior;
/**
* A non-negative decimal (with at most four decimal places) between 0 and 100. This represents
* the percentage of the subscription invoice subtotal that will be calculated and added as tax to
* the final amount in each billing period. For example, a plan which charges $10/month with a
* {@code tax_percent} of {@code 20.0} will charge $12 per invoice. To unset a previously-set
* value, pass an empty string. This field has been deprecated and will be removed in a future API
* version, for further information view the migration docs for {@code
* tax_rates}.
*/
@SerializedName("tax_percent")
Object taxPercent;
/**
* If specified, the funds from the subscription's invoices will be transferred to the destination
* and the ID of the resulting transfers will be found on the resulting charges. This will be
* unset if you POST an empty value.
*/
@SerializedName("transfer_data")
TransferData transferData;
/**
* Unix timestamp representing the end of the trial period the customer will get before being
* charged for the first time. This will always overwrite any trials that might apply via a
* subscribed plan. If set, trial_end will override the default trial period of the plan the
* customer is being subscribed to. The special value {@code now} can be provided to end the
* customer's trial immediately. Can be at most two years from {@code billing_cycle_anchor}.
*/
@SerializedName("trial_end")
Object trialEnd;
/**
* Indicates if a plan's {@code trial_period_days} should be applied to the subscription. Setting
* {@code trial_end} per subscription is preferred, and this defaults to {@code false}. Setting
* this flag to {@code true} together with {@code trial_end} is not allowed.
*/
@SerializedName("trial_from_plan")
Boolean trialFromPlan;
/**
* Integer representing the number of trial period days before the customer is charged for the
* first time. This will always overwrite any trials that might apply via a subscribed plan.
*/
@SerializedName("trial_period_days")
Long trialPeriodDays;
private SubscriptionCreateParams(BigDecimal applicationFeePercent, Long backdateStartDate, Long billingCycleAnchor, Object billingThresholds, Long cancelAt, Boolean cancelAtPeriodEnd, CollectionMethod collectionMethod, String coupon, String customer, Long daysUntilDue, String defaultPaymentMethod, String defaultSource, Object defaultTaxRates, List expand, Map extraParams, List- items, Map
metadata, Boolean offSession, PaymentBehavior paymentBehavior, Object pendingInvoiceItemInterval, Boolean prorate, ProrationBehavior prorationBehavior, Object taxPercent, TransferData transferData, Object trialEnd, Boolean trialFromPlan, Long trialPeriodDays) {
this.applicationFeePercent = applicationFeePercent;
this.backdateStartDate = backdateStartDate;
this.billingCycleAnchor = billingCycleAnchor;
this.billingThresholds = billingThresholds;
this.cancelAt = cancelAt;
this.cancelAtPeriodEnd = cancelAtPeriodEnd;
this.collectionMethod = collectionMethod;
this.coupon = coupon;
this.customer = customer;
this.daysUntilDue = daysUntilDue;
this.defaultPaymentMethod = defaultPaymentMethod;
this.defaultSource = defaultSource;
this.defaultTaxRates = defaultTaxRates;
this.expand = expand;
this.extraParams = extraParams;
this.items = items;
this.metadata = metadata;
this.offSession = offSession;
this.paymentBehavior = paymentBehavior;
this.pendingInvoiceItemInterval = pendingInvoiceItemInterval;
this.prorate = prorate;
this.prorationBehavior = prorationBehavior;
this.taxPercent = taxPercent;
this.transferData = transferData;
this.trialEnd = trialEnd;
this.trialFromPlan = trialFromPlan;
this.trialPeriodDays = trialPeriodDays;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private BigDecimal applicationFeePercent;
private Long backdateStartDate;
private Long billingCycleAnchor;
private Object billingThresholds;
private Long cancelAt;
private Boolean cancelAtPeriodEnd;
private CollectionMethod collectionMethod;
private String coupon;
private String customer;
private Long daysUntilDue;
private String defaultPaymentMethod;
private String defaultSource;
private Object defaultTaxRates;
private List expand;
private Map extraParams;
private List- items;
private Map
metadata;
private Boolean offSession;
private PaymentBehavior paymentBehavior;
private Object pendingInvoiceItemInterval;
private Boolean prorate;
private ProrationBehavior prorationBehavior;
private Object taxPercent;
private TransferData transferData;
private Object trialEnd;
private Boolean trialFromPlan;
private Long trialPeriodDays;
/**
* Finalize and obtain parameter instance from this builder.
*/
public SubscriptionCreateParams build() {
return new SubscriptionCreateParams(this.applicationFeePercent, this.backdateStartDate, this.billingCycleAnchor, this.billingThresholds, this.cancelAt, this.cancelAtPeriodEnd, this.collectionMethod, this.coupon, this.customer, this.daysUntilDue, this.defaultPaymentMethod, this.defaultSource, this.defaultTaxRates, this.expand, this.extraParams, this.items, this.metadata, this.offSession, this.paymentBehavior, this.pendingInvoiceItemInterval, this.prorate, this.prorationBehavior, this.taxPercent, this.transferData, this.trialEnd, this.trialFromPlan, this.trialPeriodDays);
}
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents
* the percentage of the subscription invoice subtotal that will be transferred to the
* application owner's Stripe account. The request must be made by a platform account on a
* connected account in order to set an application fee percentage. For more information, see
* the application fees documentation.
*/
public Builder setApplicationFeePercent(BigDecimal applicationFeePercent) {
this.applicationFeePercent = applicationFeePercent;
return this;
}
/**
* For new subscriptions, a past timestamp to backdate the subscription's start date to. If set,
* the first invoice will contain a proration for the timespan between the start date and the
* current time. Can be combined with trials and the billing cycle anchor.
*/
public Builder setBackdateStartDate(Long backdateStartDate) {
this.backdateStartDate = backdateStartDate;
return this;
}
/**
* A future timestamp to anchor the subscription's billing cycle. This is used to
* determine the date of the first full invoice, and, for plans with {@code month} or {@code
* year} intervals, the day of the month for subsequent invoices.
*/
public Builder setBillingCycleAnchor(Long billingCycleAnchor) {
this.billingCycleAnchor = billingCycleAnchor;
return this;
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. Pass an empty string to remove previously-defined thresholds.
*/
public Builder setBillingThresholds(BillingThresholds billingThresholds) {
this.billingThresholds = billingThresholds;
return this;
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. Pass an empty string to remove previously-defined thresholds.
*/
public Builder setBillingThresholds(EmptyParam billingThresholds) {
this.billingThresholds = billingThresholds;
return this;
}
/**
* A timestamp at which the subscription should cancel. If set to a date before the current
* period ends, this will cause a proration if prorations have been enabled using {@code
* proration_behavior}. If set during a future period, this will always cause a proration for
* that period.
*/
public Builder setCancelAt(Long cancelAt) {
this.cancelAt = cancelAt;
return this;
}
/**
* Boolean indicating whether this subscription should cancel at the end of the current period.
*/
public Builder setCancelAtPeriodEnd(Boolean cancelAtPeriodEnd) {
this.cancelAtPeriodEnd = cancelAtPeriodEnd;
return this;
}
/**
* Either {@code charge_automatically}, or {@code send_invoice}. When charging automatically,
* Stripe will attempt to pay this subscription at the end of the cycle using the default source
* attached to the customer. When sending an invoice, Stripe will email your customer an invoice
* with payment instructions. Defaults to {@code charge_automatically}.
*/
public Builder setCollectionMethod(CollectionMethod collectionMethod) {
this.collectionMethod = collectionMethod;
return this;
}
/**
* The code of the coupon to apply to this subscription. A coupon applied to a subscription will
* only affect invoices created for that particular subscription.
*/
public Builder setCoupon(String coupon) {
this.coupon = coupon;
return this;
}
/**
* The identifier of the customer to subscribe.
*/
public Builder setCustomer(String customer) {
this.customer = customer;
return this;
}
/**
* Number of days a customer has to pay invoices generated by this subscription. Valid only for
* subscriptions where {@code collection_method} is set to {@code send_invoice}.
*/
public Builder setDaysUntilDue(Long daysUntilDue) {
this.daysUntilDue = daysUntilDue;
return this;
}
/**
* ID of the default payment method for the subscription. It must belong to the customer
* associated with the subscription. If not set, invoices will use the default payment method in
* the customer's invoice settings.
*/
public Builder setDefaultPaymentMethod(String defaultPaymentMethod) {
this.defaultPaymentMethod = defaultPaymentMethod;
return this;
}
/**
* ID of the default payment source for the subscription. It must belong to the customer
* associated with the subscription and be in a chargeable state. If not set, defaults to the
* customer's default source.
*/
public Builder setDefaultSource(String defaultSource) {
this.defaultSource = defaultSource;
return this;
}
/**
* Add an element to `defaultTaxRates` list. A list is initialized for the first `add/addAll`
* call, and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionCreateParams#defaultTaxRates} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addDefaultTaxRate(String element) {
if (this.defaultTaxRates == null || this.defaultTaxRates instanceof EmptyParam) {
this.defaultTaxRates = new ArrayList();
}
((List) this.defaultTaxRates).add(element);
return this;
}
/**
* Add all elements to `defaultTaxRates` list. A list is initialized for the first `add/addAll`
* call, and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionCreateParams#defaultTaxRates} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addAllDefaultTaxRate(List elements) {
if (this.defaultTaxRates == null || this.defaultTaxRates instanceof EmptyParam) {
this.defaultTaxRates = new ArrayList();
}
((List) this.defaultTaxRates).addAll(elements);
return this;
}
/**
* The tax rates that will apply to any subscription item that does not have {@code tax_rates}
* set. Invoices created will have their {@code default_tax_rates} populated from the
* subscription.
*/
public Builder setDefaultTaxRates(EmptyParam defaultTaxRates) {
this.defaultTaxRates = defaultTaxRates;
return this;
}
/**
* The tax rates that will apply to any subscription item that does not have {@code tax_rates}
* set. Invoices created will have their {@code default_tax_rates} populated from the
* subscription.
*/
public Builder setDefaultTaxRates(List defaultTaxRates) {
this.defaultTaxRates = defaultTaxRates;
return this;
}
/**
* Add an element to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SubscriptionCreateParams#expand} for the field documentation.
*/
public Builder addExpand(String element) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.add(element);
return this;
}
/**
* Add all elements to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SubscriptionCreateParams#expand} for the field documentation.
*/
public Builder addAllExpand(List elements) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.addAll(elements);
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionCreateParams#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionCreateParams#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Add an element to `items` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SubscriptionCreateParams#items} for the field documentation.
*/
public Builder addItem(Item element) {
if (this.items == null) {
this.items = new ArrayList<>();
}
this.items.add(element);
return this;
}
/**
* Add all elements to `items` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SubscriptionCreateParams#items} for the field documentation.
*/
public Builder addAllItem(List- elements) {
if (this.items == null) {
this.items = new ArrayList<>();
}
this.items.addAll(elements);
return this;
}
/**
* Add a key/value pair to `metadata` map. A map is initialized for the first `put/putAll` call,
* and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionCreateParams#metadata} for the field documentation.
*/
public Builder putMetadata(String key, String value) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `metadata` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionCreateParams#metadata} for the field documentation.
*/
public Builder putAllMetadata(Map
map) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.putAll(map);
return this;
}
/**
* Indicates if a customer is on or off-session while an invoice payment is attempted.
*/
public Builder setOffSession(Boolean offSession) {
this.offSession = offSession;
return this;
}
/**
* Use {@code allow_incomplete} to create subscriptions with {@code status=incomplete} if the
* first invoice cannot be paid. Creating subscriptions with this status allows you to manage
* scenarios where additional user actions are needed to pay a subscription's invoice. For
* example, SCA regulation may require 3DS authentication to complete payment. See the SCA Migration
* Guide for Billing to learn more. This is the default behavior.
*
* Use {@code error_if_incomplete} if you want Stripe to return an HTTP 402 status code if a
* subscription's first invoice cannot be paid. For example, if a payment method requires 3DS
* authentication due to SCA regulation and further user action is needed, this parameter does
* not create a subscription and returns an error instead. This was the default behavior for API
* versions prior to 2019-03-14. See the changelog to learn more.
*/
public Builder setPaymentBehavior(PaymentBehavior paymentBehavior) {
this.paymentBehavior = paymentBehavior;
return this;
}
/**
* Specifies an interval for how often to bill for any pending invoice items. It is analogous to
* calling Create an invoice for the
* given subscription at the specified interval.
*/
public Builder setPendingInvoiceItemInterval(PendingInvoiceItemInterval pendingInvoiceItemInterval) {
this.pendingInvoiceItemInterval = pendingInvoiceItemInterval;
return this;
}
/**
* Specifies an interval for how often to bill for any pending invoice items. It is analogous to
* calling Create an invoice for the
* given subscription at the specified interval.
*/
public Builder setPendingInvoiceItemInterval(EmptyParam pendingInvoiceItemInterval) {
this.pendingInvoiceItemInterval = pendingInvoiceItemInterval;
return this;
}
/**
* This field has been renamed to {@code proration_behavior}. {@code prorate=true} can be
* replaced with {@code proration_behavior=create_prorations} and {@code prorate=false} can be
* replaced with {@code proration_behavior=none}.
*/
public Builder setProrate(Boolean prorate) {
this.prorate = prorate;
return this;
}
/**
* Determines how to handle prorations
* resulting from the {@code billing_cycle_anchor}. Valid values are {@code create_prorations}
* or {@code none}.
*
*
Passing {@code create_prorations} will cause proration invoice items to be created when
* applicable. Prorations can be disabled by passing {@code none}. If no value is passed, the
* default is {@code create_prorations}.
*/
public Builder setProrationBehavior(ProrationBehavior prorationBehavior) {
this.prorationBehavior = prorationBehavior;
return this;
}
/**
* A non-negative decimal (with at most four decimal places) between 0 and 100. This represents
* the percentage of the subscription invoice subtotal that will be calculated and added as tax
* to the final amount in each billing period. For example, a plan which charges $10/month with
* a {@code tax_percent} of {@code 20.0} will charge $12 per invoice. To unset a previously-set
* value, pass an empty string. This field has been deprecated and will be removed in a future
* API version, for further information view the migration docs for {@code
* tax_rates}.
*/
public Builder setTaxPercent(BigDecimal taxPercent) {
this.taxPercent = taxPercent;
return this;
}
/**
* A non-negative decimal (with at most four decimal places) between 0 and 100. This represents
* the percentage of the subscription invoice subtotal that will be calculated and added as tax
* to the final amount in each billing period. For example, a plan which charges $10/month with
* a {@code tax_percent} of {@code 20.0} will charge $12 per invoice. To unset a previously-set
* value, pass an empty string. This field has been deprecated and will be removed in a future
* API version, for further information view the migration docs for {@code
* tax_rates}.
*/
public Builder setTaxPercent(EmptyParam taxPercent) {
this.taxPercent = taxPercent;
return this;
}
/**
* If specified, the funds from the subscription's invoices will be transferred to the
* destination and the ID of the resulting transfers will be found on the resulting charges.
* This will be unset if you POST an empty value.
*/
public Builder setTransferData(TransferData transferData) {
this.transferData = transferData;
return this;
}
/**
* Unix timestamp representing the end of the trial period the customer will get before being
* charged for the first time. This will always overwrite any trials that might apply via a
* subscribed plan. If set, trial_end will override the default trial period of the plan the
* customer is being subscribed to. The special value {@code now} can be provided to end the
* customer's trial immediately. Can be at most two years from {@code billing_cycle_anchor}.
*/
public Builder setTrialEnd(TrialEnd trialEnd) {
this.trialEnd = trialEnd;
return this;
}
/**
* Unix timestamp representing the end of the trial period the customer will get before being
* charged for the first time. This will always overwrite any trials that might apply via a
* subscribed plan. If set, trial_end will override the default trial period of the plan the
* customer is being subscribed to. The special value {@code now} can be provided to end the
* customer's trial immediately. Can be at most two years from {@code billing_cycle_anchor}.
*/
public Builder setTrialEnd(Long trialEnd) {
this.trialEnd = trialEnd;
return this;
}
/**
* Indicates if a plan's {@code trial_period_days} should be applied to the subscription.
* Setting {@code trial_end} per subscription is preferred, and this defaults to {@code false}.
* Setting this flag to {@code true} together with {@code trial_end} is not allowed.
*/
public Builder setTrialFromPlan(Boolean trialFromPlan) {
this.trialFromPlan = trialFromPlan;
return this;
}
/**
* Integer representing the number of trial period days before the customer is charged for the
* first time. This will always overwrite any trials that might apply via a subscribed plan.
*/
public Builder setTrialPeriodDays(Long trialPeriodDays) {
this.trialPeriodDays = trialPeriodDays;
return this;
}
}
public static class BillingThresholds {
/**
* Monetary threshold that triggers the subscription to advance to a new billing period.
*/
@SerializedName("amount_gte")
Long amountGte;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Indicates if the {@code billing_cycle_anchor} should be reset when a threshold is reached. If
* true, {@code billing_cycle_anchor} will be updated to the date/time the threshold was last
* reached; otherwise, the value will remain unchanged.
*/
@SerializedName("reset_billing_cycle_anchor")
Boolean resetBillingCycleAnchor;
private BillingThresholds(Long amountGte, Map extraParams, Boolean resetBillingCycleAnchor) {
this.amountGte = amountGte;
this.extraParams = extraParams;
this.resetBillingCycleAnchor = resetBillingCycleAnchor;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Long amountGte;
private Map extraParams;
private Boolean resetBillingCycleAnchor;
/** Finalize and obtain parameter instance from this builder. */
public BillingThresholds build() {
return new BillingThresholds(this.amountGte, this.extraParams, this.resetBillingCycleAnchor);
}
/** Monetary threshold that triggers the subscription to advance to a new billing period. */
public Builder setAmountGte(Long amountGte) {
this.amountGte = amountGte;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionCreateParams.BillingThresholds#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionCreateParams.BillingThresholds#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
public Builder setResetBillingCycleAnchor(Boolean resetBillingCycleAnchor) {
this.resetBillingCycleAnchor = resetBillingCycleAnchor;
return this;
}
}
/**
* Monetary threshold that triggers the subscription to advance to a new billing period.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountGte() {
return this.amountGte;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Indicates if the {@code billing_cycle_anchor} should be reset when a threshold is reached. If
* true, {@code billing_cycle_anchor} will be updated to the date/time the threshold was last
* reached; otherwise, the value will remain unchanged.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getResetBillingCycleAnchor() {
return this.resetBillingCycleAnchor;
}
}
/**
* Indicates if the {@code billing_cycle_anchor} should be reset when a threshold is reached.
* If true, {@code billing_cycle_anchor} will be updated to the date/time the threshold was
* last reached; otherwise, the value will remain unchanged.
*/
public static class Item {
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. When updating, pass an empty string to remove previously-defined thresholds.
*/
@SerializedName("billing_thresholds")
Object billingThresholds;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset
* by posting an empty value to them. All keys can be unset by posting an empty value to {@code
* metadata}.
*/
@SerializedName("metadata")
Map metadata;
/**
* Plan ID for this item, as a string.
*/
@SerializedName("plan")
String plan;
/**
* Quantity for this item.
*/
@SerializedName("quantity")
Long quantity;
/**
* A list of Tax Rate ids. These Tax Rates
* will override the {@code
* default_tax_rates} on the Subscription. When updating, pass an empty string to remove
* previously-defined tax rates.
*/
@SerializedName("tax_rates")
Object taxRates;
private Item(Object billingThresholds, Map extraParams, Map metadata, String plan, Long quantity, Object taxRates) {
this.billingThresholds = billingThresholds;
this.extraParams = extraParams;
this.metadata = metadata;
this.plan = plan;
this.quantity = quantity;
this.taxRates = taxRates;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Object billingThresholds;
private Map extraParams;
private Map metadata;
private String plan;
private Long quantity;
private Object taxRates;
/**
* Finalize and obtain parameter instance from this builder.
*/
public Item build() {
return new Item(this.billingThresholds, this.extraParams, this.metadata, this.plan, this.quantity, this.taxRates);
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. When updating, pass an empty string to remove previously-defined
* thresholds.
*/
public Builder setBillingThresholds(BillingThresholds billingThresholds) {
this.billingThresholds = billingThresholds;
return this;
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. When updating, pass an empty string to remove previously-defined
* thresholds.
*/
public Builder setBillingThresholds(EmptyParam billingThresholds) {
this.billingThresholds = billingThresholds;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionCreateParams.Item#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionCreateParams.Item#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Add a key/value pair to `metadata` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionCreateParams.Item#metadata} for the field documentation.
*/
public Builder putMetadata(String key, String value) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `metadata` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionCreateParams.Item#metadata} for the field documentation.
*/
public Builder putAllMetadata(Map map) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.putAll(map);
return this;
}
/**
* Plan ID for this item, as a string.
*/
public Builder setPlan(String plan) {
this.plan = plan;
return this;
}
/**
* Quantity for this item.
*/
public Builder setQuantity(Long quantity) {
this.quantity = quantity;
return this;
}
/**
* Add an element to `taxRates` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionCreateParams.Item#taxRates} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addTaxRate(String element) {
if (this.taxRates == null || this.taxRates instanceof EmptyParam) {
this.taxRates = new ArrayList();
}
((List) this.taxRates).add(element);
return this;
}
/**
* Add all elements to `taxRates` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionCreateParams.Item#taxRates} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addAllTaxRate(List elements) {
if (this.taxRates == null || this.taxRates instanceof EmptyParam) {
this.taxRates = new ArrayList();
}
((List) this.taxRates).addAll(elements);
return this;
}
/**
* A list of Tax Rate ids. These Tax Rates
* will override the {@code
* default_tax_rates} on the Subscription. When updating, pass an empty string to remove
* previously-defined tax rates.
*/
public Builder setTaxRates(EmptyParam taxRates) {
this.taxRates = taxRates;
return this;
}
/**
* A list of Tax Rate ids. These Tax Rates
* will override the {@code
* default_tax_rates} on the Subscription. When updating, pass an empty string to remove
* previously-defined tax rates.
*/
public Builder setTaxRates(List taxRates) {
this.taxRates = taxRates;
return this;
}
}
public static class BillingThresholds {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/** Usage threshold that triggers the subscription to advance to a new billing period. */
@SerializedName("usage_gte")
Long usageGte;
private BillingThresholds(Map extraParams, Long usageGte) {
this.extraParams = extraParams;
this.usageGte = usageGte;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private Long usageGte;
/** Finalize and obtain parameter instance from this builder. */
public BillingThresholds build() {
return new BillingThresholds(this.extraParams, this.usageGte);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SubscriptionCreateParams.Item.BillingThresholds#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SubscriptionCreateParams.Item.BillingThresholds#extraParams} for the
* field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
public Builder setUsageGte(Long usageGte) {
this.usageGte = usageGte;
return this;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getUsageGte() {
return this.usageGte;
}
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. When updating, pass an empty string to remove previously-defined thresholds.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getBillingThresholds() {
return this.billingThresholds;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset
* by posting an empty value to them. All keys can be unset by posting an empty value to {@code
* metadata}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
/**
* Plan ID for this item, as a string.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPlan() {
return this.plan;
}
/**
* Quantity for this item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getQuantity() {
return this.quantity;
}
/**
* A list of Tax Rate ids. These Tax Rates
* will override the {@code
* default_tax_rates} on the Subscription. When updating, pass an empty string to remove
* previously-defined tax rates.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getTaxRates() {
return this.taxRates;
}
}
/**
* Usage threshold that triggers the subscription to advance to a new billing period.
*/
public static class PendingInvoiceItemInterval {
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Specifies invoicing frequency. Either {@code day}, {@code week}, {@code month} or {@code
* year}.
*/
@SerializedName("interval")
Interval interval;
/**
* The number of intervals between invoices. For example, {@code interval=month} and {@code
* interval_count=3} bills every 3 months. Maximum of one year interval allowed (1 year, 12
* months, or 52 weeks).
*/
@SerializedName("interval_count")
Long intervalCount;
private PendingInvoiceItemInterval(Map extraParams, Interval interval, Long intervalCount) {
this.extraParams = extraParams;
this.interval = interval;
this.intervalCount = intervalCount;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private Interval interval;
private Long intervalCount;
/**
* Finalize and obtain parameter instance from this builder.
*/
public PendingInvoiceItemInterval build() {
return new PendingInvoiceItemInterval(this.extraParams, this.interval, this.intervalCount);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionCreateParams.PendingInvoiceItemInterval#extraParams} for the field
* documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionCreateParams.PendingInvoiceItemInterval#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Specifies invoicing frequency. Either {@code day}, {@code week}, {@code month} or {@code
* year}.
*/
public Builder setInterval(Interval interval) {
this.interval = interval;
return this;
}
/**
* The number of intervals between invoices. For example, {@code interval=month} and {@code
* interval_count=3} bills every 3 months. Maximum of one year interval allowed (1 year, 12
* months, or 52 weeks).
*/
public Builder setIntervalCount(Long intervalCount) {
this.intervalCount = intervalCount;
return this;
}
}
public enum Interval implements ApiRequestParams.EnumParam {
@SerializedName("day")
DAY("day"), @SerializedName("month")
MONTH("month"), @SerializedName("week")
WEEK("week"), @SerializedName("year")
YEAR("year");
private final String value;
Interval(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Specifies invoicing frequency. Either {@code day}, {@code week}, {@code month} or {@code
* year}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Interval getInterval() {
return this.interval;
}
/**
* The number of intervals between invoices. For example, {@code interval=month} and {@code
* interval_count=3} bills every 3 months. Maximum of one year interval allowed (1 year, 12
* months, or 52 weeks).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getIntervalCount() {
return this.intervalCount;
}
}
public static class TransferData {
/**
* ID of an existing, connected Stripe account.
*/
@SerializedName("destination")
String destination;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
private TransferData(String destination, Map extraParams) {
this.destination = destination;
this.extraParams = extraParams;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private String destination;
private Map extraParams;
/** Finalize and obtain parameter instance from this builder. */
public TransferData build() {
return new TransferData(this.destination, this.extraParams);
}
/** ID of an existing, connected Stripe account. */
public Builder setDestination(String destination) {
this.destination = destination;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionCreateParams.TransferData#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
}
/**
* ID of an existing, connected Stripe account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDestination() {
return this.destination;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionCreateParams.TransferData#extraParams} for the field documentation.
*/
public enum CollectionMethod implements ApiRequestParams.EnumParam {
@SerializedName("charge_automatically")
CHARGE_AUTOMATICALLY("charge_automatically"), @SerializedName("send_invoice")
SEND_INVOICE("send_invoice");
private final String value;
CollectionMethod(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum PaymentBehavior implements ApiRequestParams.EnumParam {
@SerializedName("allow_incomplete")
ALLOW_INCOMPLETE("allow_incomplete"), @SerializedName("error_if_incomplete")
ERROR_IF_INCOMPLETE("error_if_incomplete"), @SerializedName("pending_if_incomplete")
PENDING_IF_INCOMPLETE("pending_if_incomplete");
private final String value;
PaymentBehavior(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum ProrationBehavior implements ApiRequestParams.EnumParam {
@SerializedName("always_invoice")
ALWAYS_INVOICE("always_invoice"), @SerializedName("create_prorations")
CREATE_PRORATIONS("create_prorations"), @SerializedName("none")
NONE("none");
private final String value;
ProrationBehavior(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum TrialEnd implements ApiRequestParams.EnumParam {
@SerializedName("now")
NOW("now");
private final String value;
TrialEnd(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents the
* percentage of the subscription invoice subtotal that will be transferred to the application
* owner's Stripe account. The request must be made by a platform account on a connected account
* in order to set an application fee percentage. For more information, see the application fees
* documentation.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BigDecimal getApplicationFeePercent() {
return this.applicationFeePercent;
}
/**
* For new subscriptions, a past timestamp to backdate the subscription's start date to. If set,
* the first invoice will contain a proration for the timespan between the start date and the
* current time. Can be combined with trials and the billing cycle anchor.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getBackdateStartDate() {
return this.backdateStartDate;
}
/**
* A future timestamp to anchor the subscription's billing cycle. This is used to
* determine the date of the first full invoice, and, for plans with {@code month} or {@code year}
* intervals, the day of the month for subsequent invoices.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getBillingCycleAnchor() {
return this.billingCycleAnchor;
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. Pass an empty string to remove previously-defined thresholds.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getBillingThresholds() {
return this.billingThresholds;
}
/**
* A timestamp at which the subscription should cancel. If set to a date before the current period
* ends, this will cause a proration if prorations have been enabled using {@code
* proration_behavior}. If set during a future period, this will always cause a proration for that
* period.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCancelAt() {
return this.cancelAt;
}
/**
* Boolean indicating whether this subscription should cancel at the end of the current period.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getCancelAtPeriodEnd() {
return this.cancelAtPeriodEnd;
}
/**
* Either {@code charge_automatically}, or {@code send_invoice}. When charging automatically,
* Stripe will attempt to pay this subscription at the end of the cycle using the default source
* attached to the customer. When sending an invoice, Stripe will email your customer an invoice
* with payment instructions. Defaults to {@code charge_automatically}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public CollectionMethod getCollectionMethod() {
return this.collectionMethod;
}
/**
* The code of the coupon to apply to this subscription. A coupon applied to a subscription will
* only affect invoices created for that particular subscription.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCoupon() {
return this.coupon;
}
/**
* The identifier of the customer to subscribe.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCustomer() {
return this.customer;
}
/**
* Number of days a customer has to pay invoices generated by this subscription. Valid only for
* subscriptions where {@code collection_method} is set to {@code send_invoice}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDaysUntilDue() {
return this.daysUntilDue;
}
/**
* ID of the default payment method for the subscription. It must belong to the customer
* associated with the subscription. If not set, invoices will use the default payment method in
* the customer's invoice settings.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDefaultPaymentMethod() {
return this.defaultPaymentMethod;
}
/**
* ID of the default payment source for the subscription. It must belong to the customer
* associated with the subscription and be in a chargeable state. If not set, defaults to the
* customer's default source.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDefaultSource() {
return this.defaultSource;
}
/**
* The tax rates that will apply to any subscription item that does not have {@code tax_rates}
* set. Invoices created will have their {@code default_tax_rates} populated from the
* subscription.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getDefaultTaxRates() {
return this.defaultTaxRates;
}
/**
* Specifies which fields in the response should be expanded.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getExpand() {
return this.expand;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* A list of up to 20 subscription items, each with an attached plan.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List- getItems() {
return this.items;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset by
* posting an empty value to them. All keys can be unset by posting an empty value to {@code
* metadata}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map
getMetadata() {
return this.metadata;
}
/**
* Indicates if a customer is on or off-session while an invoice payment is attempted.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getOffSession() {
return this.offSession;
}
/**
* Use {@code allow_incomplete} to create subscriptions with {@code status=incomplete} if the
* first invoice cannot be paid. Creating subscriptions with this status allows you to manage
* scenarios where additional user actions are needed to pay a subscription's invoice. For
* example, SCA regulation may require 3DS authentication to complete payment. See the SCA Migration
* Guide for Billing to learn more. This is the default behavior.
*
* Use {@code error_if_incomplete} if you want Stripe to return an HTTP 402 status code if a
* subscription's first invoice cannot be paid. For example, if a payment method requires 3DS
* authentication due to SCA regulation and further user action is needed, this parameter does not
* create a subscription and returns an error instead. This was the default behavior for API
* versions prior to 2019-03-14. See the changelog to learn more.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PaymentBehavior getPaymentBehavior() {
return this.paymentBehavior;
}
/**
* Specifies an interval for how often to bill for any pending invoice items. It is analogous to
* calling Create an invoice for the
* given subscription at the specified interval.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getPendingInvoiceItemInterval() {
return this.pendingInvoiceItemInterval;
}
/**
* This field has been renamed to {@code proration_behavior}. {@code prorate=true} can be replaced
* with {@code proration_behavior=create_prorations} and {@code prorate=false} can be replaced
* with {@code proration_behavior=none}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getProrate() {
return this.prorate;
}
/**
* Determines how to handle prorations resulting
* from the {@code billing_cycle_anchor}. Valid values are {@code create_prorations} or {@code
* none}.
*
*
Passing {@code create_prorations} will cause proration invoice items to be created when
* applicable. Prorations can be disabled by passing {@code none}. If no value is passed, the
* default is {@code create_prorations}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ProrationBehavior getProrationBehavior() {
return this.prorationBehavior;
}
/**
* A non-negative decimal (with at most four decimal places) between 0 and 100. This represents
* the percentage of the subscription invoice subtotal that will be calculated and added as tax to
* the final amount in each billing period. For example, a plan which charges $10/month with a
* {@code tax_percent} of {@code 20.0} will charge $12 per invoice. To unset a previously-set
* value, pass an empty string. This field has been deprecated and will be removed in a future API
* version, for further information view the migration docs for {@code
* tax_rates}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getTaxPercent() {
return this.taxPercent;
}
/**
* If specified, the funds from the subscription's invoices will be transferred to the destination
* and the ID of the resulting transfers will be found on the resulting charges. This will be
* unset if you POST an empty value.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TransferData getTransferData() {
return this.transferData;
}
/**
* Unix timestamp representing the end of the trial period the customer will get before being
* charged for the first time. This will always overwrite any trials that might apply via a
* subscribed plan. If set, trial_end will override the default trial period of the plan the
* customer is being subscribed to. The special value {@code now} can be provided to end the
* customer's trial immediately. Can be at most two years from {@code billing_cycle_anchor}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getTrialEnd() {
return this.trialEnd;
}
/**
* Indicates if a plan's {@code trial_period_days} should be applied to the subscription. Setting
* {@code trial_end} per subscription is preferred, and this defaults to {@code false}. Setting
* this flag to {@code true} together with {@code trial_end} is not allowed.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getTrialFromPlan() {
return this.trialFromPlan;
}
/**
* Integer representing the number of trial period days before the customer is charged for the
* first time. This will always overwrite any trials that might apply via a subscribed plan.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getTrialPeriodDays() {
return this.trialPeriodDays;
}
}