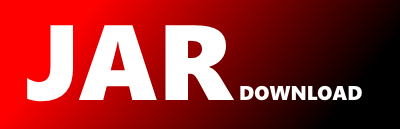
com.stripe.net.StripeResponse Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 24 09:51:01 PST 2020
package com.stripe.net;
import static java.util.Objects.requireNonNull;
import java.time.Instant;
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
import java.util.Optional;
/**
* A response from Stripe's API.
*/
public final class StripeResponse {
/**
* The HTTP status code of the response.
*/
private final int code;
/**
* The HTTP headers of the response.
*/
private final HttpHeaders headers;
/**
* The body of the response.
*/
private final String body;
/**
* Number of times the request was retried. Used for internal tests only.
*/
private int numRetries;
/**
* Initializes a new instance of the {@link StripeResponse} class.
*
* @param code the HTTP status code of the response
* @param headers the HTTP headers of the response
* @param body the body of the response
* @throws NullPointerException if {@code headers} or {@code body} is {@code null}
*/
public StripeResponse(int code, HttpHeaders headers, String body) {
requireNonNull(headers);
requireNonNull(body);
this.code = code;
this.headers = headers;
this.body = body;
}
/**
* Gets the date of the request, as returned by Stripe.
*
* @return the date of the request, as returned by Stripe
*/
public Instant date() {
Optional dateStr = this.headers.firstValue("Date");
if (!dateStr.isPresent()) {
return null;
}
return ZonedDateTime.parse(dateStr.get(), DateTimeFormatter.RFC_1123_DATE_TIME).toInstant();
}
/**
* Gets the idempotency key of the request, as returned by Stripe.
*
* @return the idempotency key of the request, as returned by Stripe
*/
public String idempotencyKey() {
return this.headers.firstValue("Idempotency-Key").orElse(null);
}
/**
* Gets the ID of the request, as returned by Stripe.
*
* @return the ID of the request, as returned by Stripe
*/
public String requestId() {
return this.headers.firstValue("Request-Id").orElse(null);
}
/**
* The HTTP status code of the response.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int code() {
return this.code;
}
/**
* The HTTP headers of the response.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public HttpHeaders headers() {
return this.headers;
}
/**
* The body of the response.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String body() {
return this.body;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof StripeResponse)) return false;
final StripeResponse other = (StripeResponse) o;
if (this.code() != other.code()) return false;
final java.lang.Object this$headers = this.headers();
final java.lang.Object other$headers = other.headers();
if (this$headers == null ? other$headers != null : !this$headers.equals(other$headers)) return false;
final java.lang.Object this$body = this.body();
final java.lang.Object other$body = other.body();
if (this$body == null ? other$body != null : !this$body.equals(other$body)) return false;
if (this.numRetries() != other.numRetries()) return false;
return true;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + this.code();
final java.lang.Object $headers = this.headers();
result = result * PRIME + ($headers == null ? 43 : $headers.hashCode());
final java.lang.Object $body = this.body();
result = result * PRIME + ($body == null ? 43 : $body.hashCode());
result = result * PRIME + this.numRetries();
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "StripeResponse(code=" + this.code() + ", headers=" + this.headers() + ", body=" + this.body() + ", numRetries=" + this.numRetries() + ")";
}
/**
* Number of times the request was retried. Used for internal tests only.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
int numRetries() {
return this.numRetries;
}
/**
* Number of times the request was retried. Used for internal tests only.
* @return this
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
StripeResponse numRetries(final int numRetries) {
this.numRetries = numRetries;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy