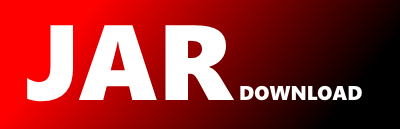
com.stripe.model.checkout.Session Maven / Gradle / Ivy
// Generated by delombok at Thu Mar 12 08:35:04 PDT 2020
package com.stripe.model.checkout;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.model.Customer;
import com.stripe.model.ExpandableField;
import com.stripe.model.HasId;
import com.stripe.model.PaymentIntent;
import com.stripe.model.Plan;
import com.stripe.model.SetupIntent;
import com.stripe.model.Sku;
import com.stripe.model.StripeObject;
import com.stripe.model.Subscription;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.checkout.SessionCreateParams;
import com.stripe.param.checkout.SessionListParams;
import com.stripe.param.checkout.SessionRetrieveParams;
import java.util.List;
import java.util.Map;
public class Session extends ApiResource implements HasId {
/**
* The value ({@code auto} or {@code required}) for whether Checkout collected the customer's
* billing address.
*/
@SerializedName("billing_address_collection")
String billingAddressCollection;
/**
* The URL the customer will be directed to if they decide to cancel payment and return to your
* website.
*/
@SerializedName("cancel_url")
String cancelUrl;
/**
* A unique string to reference the Checkout Session. This can be a customer ID, a cart ID, or
* similar, and can be used to reconcile the session with your internal systems.
*/
@SerializedName("client_reference_id")
String clientReferenceId;
/**
* The ID of the customer for this session. For Checkout Sessions in {@code payment} or {@code
* subscription} mode, Checkout will create a new customer object based on information provided
* during the session unless an existing customer was provided when the session was created.
*/
@SerializedName("customer")
ExpandableField customer;
/**
* If provided, this value will be used when the Customer object is created. If not provided,
* customers will be asked to enter their email address. Use this parameter to prefill customer
* data if you already have an email on file. To access information about the customer once a
* session is complete, use the {@code customer} field.
*/
@SerializedName("customer_email")
String customerEmail;
/**
* The line items, plans, or SKUs purchased by the customer.
*/
@SerializedName("display_items")
List displayItems;
/**
* Unique identifier for the object. Used to pass to {@code redirectToCheckout} in Stripe.js.
*/
@SerializedName("id")
String id;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* The IETF language tag of the locale Checkout is displayed in. If blank or {@code auto}, the
* browser's locale is used.
*
* One of {@code auto}, {@code da}, {@code de}, {@code en}, {@code es}, {@code fi}, {@code fr},
* {@code it}, {@code ja}, {@code ms}, {@code nb}, {@code nl}, {@code pl}, {@code pt}, {@code sv},
* or {@code zh}.
*/
@SerializedName("locale")
String locale;
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* The mode of the Checkout Session, one of {@code payment}, {@code setup}, or {@code
* subscription}.
*/
@SerializedName("mode")
String mode;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code checkout.session}.
*/
@SerializedName("object")
String object;
/**
* The ID of the PaymentIntent for Checkout Sessions in {@code payment} mode.
*/
@SerializedName("payment_intent")
ExpandableField paymentIntent;
/**
* A list of the types of payment methods (e.g. card) this Checkout Session is allowed to accept.
*/
@SerializedName("payment_method_types")
List paymentMethodTypes;
/**
* The ID of the SetupIntent for Checkout Sessions in {@code setup} mode.
*/
@SerializedName("setup_intent")
ExpandableField setupIntent;
/**
* Describes the type of transaction being performed by Checkout in order to customize relevant
* text on the page, such as the submit button. {@code submit_type} can only be specified on
* Checkout Sessions in {@code payment} mode, but not Checkout Sessions in {@code subscription} or
* {@code setup} mode.
*
* One of {@code auto}, {@code book}, {@code donate}, or {@code pay}.
*/
@SerializedName("submit_type")
String submitType;
/**
* The ID of the subscription for Checkout Sessions in {@code subscription} mode.
*/
@SerializedName("subscription")
ExpandableField subscription;
/**
* The URL the customer will be directed to after the payment or subscription creation is
* successful.
*/
@SerializedName("success_url")
String successUrl;
/**
* Get ID of expandable {@code customer} object.
*/
public String getCustomer() {
return (this.customer != null) ? this.customer.getId() : null;
}
public void setCustomer(String id) {
this.customer = ApiResource.setExpandableFieldId(id, this.customer);
}
/**
* Get expanded {@code customer}.
*/
public Customer getCustomerObject() {
return (this.customer != null) ? this.customer.getExpanded() : null;
}
public void setCustomerObject(Customer expandableObject) {
this.customer = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code paymentIntent} object.
*/
public String getPaymentIntent() {
return (this.paymentIntent != null) ? this.paymentIntent.getId() : null;
}
public void setPaymentIntent(String id) {
this.paymentIntent = ApiResource.setExpandableFieldId(id, this.paymentIntent);
}
/**
* Get expanded {@code paymentIntent}.
*/
public PaymentIntent getPaymentIntentObject() {
return (this.paymentIntent != null) ? this.paymentIntent.getExpanded() : null;
}
public void setPaymentIntentObject(PaymentIntent expandableObject) {
this.paymentIntent = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code setupIntent} object.
*/
public String getSetupIntent() {
return (this.setupIntent != null) ? this.setupIntent.getId() : null;
}
public void setSetupIntent(String id) {
this.setupIntent = ApiResource.setExpandableFieldId(id, this.setupIntent);
}
/**
* Get expanded {@code setupIntent}.
*/
public SetupIntent getSetupIntentObject() {
return (this.setupIntent != null) ? this.setupIntent.getExpanded() : null;
}
public void setSetupIntentObject(SetupIntent expandableObject) {
this.setupIntent = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code subscription} object.
*/
public String getSubscription() {
return (this.subscription != null) ? this.subscription.getId() : null;
}
public void setSubscription(String id) {
this.subscription = ApiResource.setExpandableFieldId(id, this.subscription);
}
/**
* Get expanded {@code subscription}.
*/
public Subscription getSubscriptionObject() {
return (this.subscription != null) ? this.subscription.getExpanded() : null;
}
public void setSubscriptionObject(Subscription expandableObject) {
this.subscription = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Returns a list of Checkout Sessions.
*/
public static SessionCollection list(Map params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of Checkout Sessions.
*/
public static SessionCollection list(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/checkout/sessions");
return ApiResource.requestCollection(url, params, SessionCollection.class, options);
}
/**
* Returns a list of Checkout Sessions.
*/
public static SessionCollection list(SessionListParams params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of Checkout Sessions.
*/
public static SessionCollection list(SessionListParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/checkout/sessions");
return ApiResource.requestCollection(url, params, SessionCollection.class, options);
}
/**
* Retrieves a Session object.
*/
public static Session retrieve(String session) throws StripeException {
return retrieve(session, (Map) null, (RequestOptions) null);
}
/**
* Retrieves a Session object.
*/
public static Session retrieve(String session, RequestOptions options) throws StripeException {
return retrieve(session, (Map) null, options);
}
/**
* Retrieves a Session object.
*/
public static Session retrieve(String session, Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/checkout/sessions/%s", ApiResource.urlEncodeId(session)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Session.class, options);
}
/**
* Retrieves a Session object.
*/
public static Session retrieve(String session, SessionRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/checkout/sessions/%s", ApiResource.urlEncodeId(session)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Session.class, options);
}
/**
* Creates a Session object.
*/
public static Session create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Creates a Session object.
*/
public static Session create(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/checkout/sessions");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Session.class, options);
}
/**
* Creates a Session object.
*/
public static Session create(SessionCreateParams params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Creates a Session object.
*/
public static Session create(SessionCreateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/checkout/sessions");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Session.class, options);
}
public static class DisplayItem extends StripeObject {
/** Amount for the display item. */
@SerializedName("amount")
Long amount;
/**
* Three-letter ISO currency
* code, in lowercase. Must be a supported
* currency.
*/
@SerializedName("currency")
String currency;
@SerializedName("custom")
Custom custom;
/**
* Plans define the base price, currency, and billing cycle for subscriptions. For example, you
* might have a <currency>5</currency>/month plan that provides limited access to
* your products, and a <currency>15</currency>/month plan that allows full access.
*
* Related guide: Managing Products and
* Plans.
*/
@SerializedName("plan")
Plan plan;
/** Quantity of the display item being purchased. */
@SerializedName("quantity")
Long quantity;
/**
* Stores representations of stock
* keeping units. SKUs describe specific product variations, taking into account any
* combination of: attributes, currency, and cost. For example, a product may be a T-shirt,
* whereas a specific SKU represents the {@code size: large}, {@code color: red} version of that
* shirt.
*
*
Can also be used to manage inventory.
*
*
Related guide: Tax, Shipping, and Inventory.
*/
@SerializedName("sku")
Sku sku;
/** The type of display item. One of {@code custom}, {@code plan} or {@code sku} */
@SerializedName("type")
String type;
public static class Custom extends StripeObject {
/** The description of the line item. */
@SerializedName("description")
String description;
/** The images of the line item. */
@SerializedName("images")
List images;
/** The name of the line item. */
@SerializedName("name")
String name;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDescription() {
return this.description;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getImages() {
return this.images;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getName() {
return this.name;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDescription(final String description) {
this.description = description;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setImages(final List images) {
this.images = images;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setName(final String name) {
this.name = name;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Session.DisplayItem.Custom)) return false;
final Session.DisplayItem.Custom other = (Session.DisplayItem.Custom) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$images = this.getImages();
final java.lang.Object other$images = other.getImages();
if (this$images == null ? other$images != null : !this$images.equals(other$images)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Session.DisplayItem.Custom;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $images = this.getImages();
result = result * PRIME + ($images == null ? 43 : $images.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Custom getCustom() {
return this.custom;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Plan getPlan() {
return this.plan;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getQuantity() {
return this.quantity;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Sku getSku() {
return this.sku;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmount(final Long amount) {
this.amount = amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCustom(final Custom custom) {
this.custom = custom;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPlan(final Plan plan) {
this.plan = plan;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setQuantity(final Long quantity) {
this.quantity = quantity;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSku(final Sku sku) {
this.sku = sku;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Session.DisplayItem)) return false;
final Session.DisplayItem other = (Session.DisplayItem) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
final java.lang.Object this$custom = this.getCustom();
final java.lang.Object other$custom = other.getCustom();
if (this$custom == null ? other$custom != null : !this$custom.equals(other$custom)) return false;
final java.lang.Object this$plan = this.getPlan();
final java.lang.Object other$plan = other.getPlan();
if (this$plan == null ? other$plan != null : !this$plan.equals(other$plan)) return false;
final java.lang.Object this$quantity = this.getQuantity();
final java.lang.Object other$quantity = other.getQuantity();
if (this$quantity == null ? other$quantity != null : !this$quantity.equals(other$quantity)) return false;
final java.lang.Object this$sku = this.getSku();
final java.lang.Object other$sku = other.getSku();
if (this$sku == null ? other$sku != null : !this$sku.equals(other$sku)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Session.DisplayItem;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
final java.lang.Object $custom = this.getCustom();
result = result * PRIME + ($custom == null ? 43 : $custom.hashCode());
final java.lang.Object $plan = this.getPlan();
result = result * PRIME + ($plan == null ? 43 : $plan.hashCode());
final java.lang.Object $quantity = this.getQuantity();
result = result * PRIME + ($quantity == null ? 43 : $quantity.hashCode());
final java.lang.Object $sku = this.getSku();
result = result * PRIME + ($sku == null ? 43 : $sku.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
return result;
}
}
/**
* The value ({@code auto} or {@code required}) for whether Checkout collected the customer's
* billing address.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBillingAddressCollection() {
return this.billingAddressCollection;
}
/**
* The URL the customer will be directed to if they decide to cancel payment and return to your
* website.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCancelUrl() {
return this.cancelUrl;
}
/**
* A unique string to reference the Checkout Session. This can be a customer ID, a cart ID, or
* similar, and can be used to reconcile the session with your internal systems.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getClientReferenceId() {
return this.clientReferenceId;
}
/**
* If provided, this value will be used when the Customer object is created. If not provided,
* customers will be asked to enter their email address. Use this parameter to prefill customer
* data if you already have an email on file. To access information about the customer once a
* session is complete, use the {@code customer} field.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCustomerEmail() {
return this.customerEmail;
}
/**
* The line items, plans, or SKUs purchased by the customer.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getDisplayItems() {
return this.displayItems;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* The IETF language tag of the locale Checkout is displayed in. If blank or {@code auto}, the
* browser's locale is used.
*
* One of {@code auto}, {@code da}, {@code de}, {@code en}, {@code es}, {@code fi}, {@code fr},
* {@code it}, {@code ja}, {@code ms}, {@code nb}, {@code nl}, {@code pl}, {@code pt}, {@code sv},
* or {@code zh}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLocale() {
return this.locale;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
/**
* The mode of the Checkout Session, one of {@code payment}, {@code setup}, or {@code
* subscription}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getMode() {
return this.mode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code checkout.session}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* A list of the types of payment methods (e.g. card) this Checkout Session is allowed to accept.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getPaymentMethodTypes() {
return this.paymentMethodTypes;
}
/**
* Describes the type of transaction being performed by Checkout in order to customize relevant
* text on the page, such as the submit button. {@code submit_type} can only be specified on
* Checkout Sessions in {@code payment} mode, but not Checkout Sessions in {@code subscription} or
* {@code setup} mode.
*
* One of {@code auto}, {@code book}, {@code donate}, or {@code pay}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSubmitType() {
return this.submitType;
}
/**
* The URL the customer will be directed to after the payment or subscription creation is
* successful.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSuccessUrl() {
return this.successUrl;
}
/**
* The value ({@code auto} or {@code required}) for whether Checkout collected the customer's
* billing address.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBillingAddressCollection(final String billingAddressCollection) {
this.billingAddressCollection = billingAddressCollection;
}
/**
* The URL the customer will be directed to if they decide to cancel payment and return to your
* website.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCancelUrl(final String cancelUrl) {
this.cancelUrl = cancelUrl;
}
/**
* A unique string to reference the Checkout Session. This can be a customer ID, a cart ID, or
* similar, and can be used to reconcile the session with your internal systems.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setClientReferenceId(final String clientReferenceId) {
this.clientReferenceId = clientReferenceId;
}
/**
* If provided, this value will be used when the Customer object is created. If not provided,
* customers will be asked to enter their email address. Use this parameter to prefill customer
* data if you already have an email on file. To access information about the customer once a
* session is complete, use the {@code customer} field.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCustomerEmail(final String customerEmail) {
this.customerEmail = customerEmail;
}
/**
* The line items, plans, or SKUs purchased by the customer.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDisplayItems(final List displayItems) {
this.displayItems = displayItems;
}
/**
* Unique identifier for the object. Used to pass to {@code redirectToCheckout} in Stripe.js.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* The IETF language tag of the locale Checkout is displayed in. If blank or {@code auto}, the
* browser's locale is used.
*
* One of {@code auto}, {@code da}, {@code de}, {@code en}, {@code es}, {@code fi}, {@code fr},
* {@code it}, {@code ja}, {@code ms}, {@code nb}, {@code nl}, {@code pl}, {@code pt}, {@code sv},
* or {@code zh}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLocale(final String locale) {
this.locale = locale;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
/**
* The mode of the Checkout Session, one of {@code payment}, {@code setup}, or {@code
* subscription}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMode(final String mode) {
this.mode = mode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code checkout.session}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* A list of the types of payment methods (e.g. card) this Checkout Session is allowed to accept.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPaymentMethodTypes(final List paymentMethodTypes) {
this.paymentMethodTypes = paymentMethodTypes;
}
/**
* Describes the type of transaction being performed by Checkout in order to customize relevant
* text on the page, such as the submit button. {@code submit_type} can only be specified on
* Checkout Sessions in {@code payment} mode, but not Checkout Sessions in {@code subscription} or
* {@code setup} mode.
*
* One of {@code auto}, {@code book}, {@code donate}, or {@code pay}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSubmitType(final String submitType) {
this.submitType = submitType;
}
/**
* The URL the customer will be directed to after the payment or subscription creation is
* successful.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSuccessUrl(final String successUrl) {
this.successUrl = successUrl;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Session)) return false;
final Session other = (Session) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$billingAddressCollection = this.getBillingAddressCollection();
final java.lang.Object other$billingAddressCollection = other.getBillingAddressCollection();
if (this$billingAddressCollection == null ? other$billingAddressCollection != null : !this$billingAddressCollection.equals(other$billingAddressCollection)) return false;
final java.lang.Object this$cancelUrl = this.getCancelUrl();
final java.lang.Object other$cancelUrl = other.getCancelUrl();
if (this$cancelUrl == null ? other$cancelUrl != null : !this$cancelUrl.equals(other$cancelUrl)) return false;
final java.lang.Object this$clientReferenceId = this.getClientReferenceId();
final java.lang.Object other$clientReferenceId = other.getClientReferenceId();
if (this$clientReferenceId == null ? other$clientReferenceId != null : !this$clientReferenceId.equals(other$clientReferenceId)) return false;
final java.lang.Object this$customer = this.getCustomer();
final java.lang.Object other$customer = other.getCustomer();
if (this$customer == null ? other$customer != null : !this$customer.equals(other$customer)) return false;
final java.lang.Object this$customerEmail = this.getCustomerEmail();
final java.lang.Object other$customerEmail = other.getCustomerEmail();
if (this$customerEmail == null ? other$customerEmail != null : !this$customerEmail.equals(other$customerEmail)) return false;
final java.lang.Object this$displayItems = this.getDisplayItems();
final java.lang.Object other$displayItems = other.getDisplayItems();
if (this$displayItems == null ? other$displayItems != null : !this$displayItems.equals(other$displayItems)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$locale = this.getLocale();
final java.lang.Object other$locale = other.getLocale();
if (this$locale == null ? other$locale != null : !this$locale.equals(other$locale)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$mode = this.getMode();
final java.lang.Object other$mode = other.getMode();
if (this$mode == null ? other$mode != null : !this$mode.equals(other$mode)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$paymentIntent = this.getPaymentIntent();
final java.lang.Object other$paymentIntent = other.getPaymentIntent();
if (this$paymentIntent == null ? other$paymentIntent != null : !this$paymentIntent.equals(other$paymentIntent)) return false;
final java.lang.Object this$paymentMethodTypes = this.getPaymentMethodTypes();
final java.lang.Object other$paymentMethodTypes = other.getPaymentMethodTypes();
if (this$paymentMethodTypes == null ? other$paymentMethodTypes != null : !this$paymentMethodTypes.equals(other$paymentMethodTypes)) return false;
final java.lang.Object this$setupIntent = this.getSetupIntent();
final java.lang.Object other$setupIntent = other.getSetupIntent();
if (this$setupIntent == null ? other$setupIntent != null : !this$setupIntent.equals(other$setupIntent)) return false;
final java.lang.Object this$submitType = this.getSubmitType();
final java.lang.Object other$submitType = other.getSubmitType();
if (this$submitType == null ? other$submitType != null : !this$submitType.equals(other$submitType)) return false;
final java.lang.Object this$subscription = this.getSubscription();
final java.lang.Object other$subscription = other.getSubscription();
if (this$subscription == null ? other$subscription != null : !this$subscription.equals(other$subscription)) return false;
final java.lang.Object this$successUrl = this.getSuccessUrl();
final java.lang.Object other$successUrl = other.getSuccessUrl();
if (this$successUrl == null ? other$successUrl != null : !this$successUrl.equals(other$successUrl)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Session;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $billingAddressCollection = this.getBillingAddressCollection();
result = result * PRIME + ($billingAddressCollection == null ? 43 : $billingAddressCollection.hashCode());
final java.lang.Object $cancelUrl = this.getCancelUrl();
result = result * PRIME + ($cancelUrl == null ? 43 : $cancelUrl.hashCode());
final java.lang.Object $clientReferenceId = this.getClientReferenceId();
result = result * PRIME + ($clientReferenceId == null ? 43 : $clientReferenceId.hashCode());
final java.lang.Object $customer = this.getCustomer();
result = result * PRIME + ($customer == null ? 43 : $customer.hashCode());
final java.lang.Object $customerEmail = this.getCustomerEmail();
result = result * PRIME + ($customerEmail == null ? 43 : $customerEmail.hashCode());
final java.lang.Object $displayItems = this.getDisplayItems();
result = result * PRIME + ($displayItems == null ? 43 : $displayItems.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $locale = this.getLocale();
result = result * PRIME + ($locale == null ? 43 : $locale.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $mode = this.getMode();
result = result * PRIME + ($mode == null ? 43 : $mode.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $paymentIntent = this.getPaymentIntent();
result = result * PRIME + ($paymentIntent == null ? 43 : $paymentIntent.hashCode());
final java.lang.Object $paymentMethodTypes = this.getPaymentMethodTypes();
result = result * PRIME + ($paymentMethodTypes == null ? 43 : $paymentMethodTypes.hashCode());
final java.lang.Object $setupIntent = this.getSetupIntent();
result = result * PRIME + ($setupIntent == null ? 43 : $setupIntent.hashCode());
final java.lang.Object $submitType = this.getSubmitType();
result = result * PRIME + ($submitType == null ? 43 : $submitType.hashCode());
final java.lang.Object $subscription = this.getSubscription();
result = result * PRIME + ($subscription == null ? 43 : $subscription.hashCode());
final java.lang.Object $successUrl = this.getSuccessUrl();
result = result * PRIME + ($successUrl == null ? 43 : $successUrl.hashCode());
return result;
}
/**
* Unique identifier for the object. Used to pass to {@code redirectToCheckout} in Stripe.js.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
}