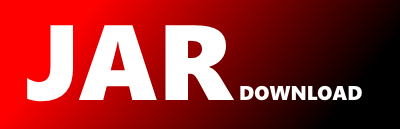
com.stripe.param.PaymentIntentConfirmParams Maven / Gradle / Ivy
// Generated by delombok at Thu Mar 12 08:35:04 PDT 2020
package com.stripe.param;
import com.google.gson.annotations.SerializedName;
import com.stripe.net.ApiRequestParams;
import com.stripe.net.ApiRequestParams.EnumParam;
import com.stripe.param.common.EmptyParam;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class PaymentIntentConfirmParams extends ApiRequestParams {
/**
* Set to {@code true} to fail the payment attempt if the PaymentIntent transitions into {@code
* requires_action}. This parameter is intended for simpler integrations that do not handle
* customer actions, like saving cards without
* authentication.
*/
@SerializedName("error_on_requires_action")
Boolean errorOnRequiresAction;
/**
* Specifies which fields in the response should be expanded.
*/
@SerializedName("expand")
List expand;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* ID of the mandate to be used for this payment.
*/
@SerializedName("mandate")
String mandate;
/**
* This hash contains details about the Mandate to create.
*/
@SerializedName("mandate_data")
Object mandateData;
/**
* Set to {@code true} to indicate that the customer is not in your checkout flow during this
* payment attempt, and therefore is unable to authenticate. This parameter is intended for
* scenarios where you collect card details and charge them later.
*/
@SerializedName("off_session")
Object offSession;
/**
* ID of the payment method (a PaymentMethod, Card, or compatible Source
* object) to attach to this PaymentIntent.
*/
@SerializedName("payment_method")
String paymentMethod;
/**
* Payment-method-specific configuration for this PaymentIntent.
*/
@SerializedName("payment_method_options")
PaymentMethodOptions paymentMethodOptions;
/**
* Email address that the receipt for the resulting payment will be sent to.
*/
@SerializedName("receipt_email")
Object receiptEmail;
/**
* The URL to redirect your customer back to after they authenticate or cancel their payment on
* the payment method's app or site. If you'd prefer to redirect to a mobile application, you can
* alternatively supply an application URI scheme. This parameter is only used for cards and other
* redirect-based payment methods.
*/
@SerializedName("return_url")
String returnUrl;
/**
* If the PaymentIntent has a {@code payment_method} and a {@code customer} or if you're attaching
* a payment method to the PaymentIntent in this request, you can pass {@code
* save_payment_method=true} to save the payment method to the customer. Defaults to {@code
* false}.
*
* If the payment method is already saved to a customer, this does nothing. If this type of
* payment method cannot be saved to a customer, the request will error.
*
*
Note that saving a payment method using this parameter does not guarantee that the
* payment method can be charged. To ensure that only payment methods which can be charged
* are saved to a customer, you can manually save
* the payment method in response to the {@code
* payment_intent.succeeded} webhook.
*/
@SerializedName("save_payment_method")
Boolean savePaymentMethod;
/**
* Indicates that you intend to make future payments with this PaymentIntent's payment method.
*
*
If present, the payment method used with this PaymentIntent can be attached to a Customer, even
* after the transaction completes.
*
*
Use {@code on_session} if you intend to only reuse the payment method when your customer is
* present in your checkout flow. Use {@code off_session} if your customer may or may not be in
* your checkout flow.
*
*
Stripe uses {@code setup_future_usage} to dynamically optimize your payment flow and comply
* with regional legislation and network rules. For example, if your customer is impacted by SCA, using {@code
* off_session} will ensure that they are authenticated while processing this PaymentIntent. You
* will then be able to collect off-session
* payments for this customer.
*
*
If {@code setup_future_usage} is already set and you are performing a request using a
* publishable key, you may only update the value from {@code on_session} to {@code off_session}.
*/
@SerializedName("setup_future_usage")
EnumParam setupFutureUsage;
/**
* Shipping information for this PaymentIntent.
*/
@SerializedName("shipping")
Object shipping;
/**
* This is a legacy field that will be removed in the future. It is the ID of the Source object to
* attach to this PaymentIntent. Please use the {@code payment_method} field instead, which also
* supports Cards and compatible Source
* objects.
*/
@SerializedName("source")
String source;
/**
* Set to {@code true} only when using manual confirmation and the iOS or Android SDKs to handle
* additional authentication steps.
*/
@SerializedName("use_stripe_sdk")
Boolean useStripeSdk;
private PaymentIntentConfirmParams(Boolean errorOnRequiresAction, List expand, Map extraParams, String mandate, Object mandateData, Object offSession, String paymentMethod, PaymentMethodOptions paymentMethodOptions, Object receiptEmail, String returnUrl, Boolean savePaymentMethod, EnumParam setupFutureUsage, Object shipping, String source, Boolean useStripeSdk) {
this.errorOnRequiresAction = errorOnRequiresAction;
this.expand = expand;
this.extraParams = extraParams;
this.mandate = mandate;
this.mandateData = mandateData;
this.offSession = offSession;
this.paymentMethod = paymentMethod;
this.paymentMethodOptions = paymentMethodOptions;
this.receiptEmail = receiptEmail;
this.returnUrl = returnUrl;
this.savePaymentMethod = savePaymentMethod;
this.setupFutureUsage = setupFutureUsage;
this.shipping = shipping;
this.source = source;
this.useStripeSdk = useStripeSdk;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Boolean errorOnRequiresAction;
private List expand;
private Map extraParams;
private String mandate;
private Object mandateData;
private Object offSession;
private String paymentMethod;
private PaymentMethodOptions paymentMethodOptions;
private Object receiptEmail;
private String returnUrl;
private Boolean savePaymentMethod;
private EnumParam setupFutureUsage;
private Object shipping;
private String source;
private Boolean useStripeSdk;
/**
* Finalize and obtain parameter instance from this builder.
*/
public PaymentIntentConfirmParams build() {
return new PaymentIntentConfirmParams(this.errorOnRequiresAction, this.expand, this.extraParams, this.mandate, this.mandateData, this.offSession, this.paymentMethod, this.paymentMethodOptions, this.receiptEmail, this.returnUrl, this.savePaymentMethod, this.setupFutureUsage, this.shipping, this.source, this.useStripeSdk);
}
/**
* Set to {@code true} to fail the payment attempt if the PaymentIntent transitions into {@code
* requires_action}. This parameter is intended for simpler integrations that do not handle
* customer actions, like saving cards without
* authentication.
*/
public Builder setErrorOnRequiresAction(Boolean errorOnRequiresAction) {
this.errorOnRequiresAction = errorOnRequiresAction;
return this;
}
/**
* Add an element to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* PaymentIntentConfirmParams#expand} for the field documentation.
*/
public Builder addExpand(String element) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.add(element);
return this;
}
/**
* Add all elements to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* PaymentIntentConfirmParams#expand} for the field documentation.
*/
public Builder addAllExpand(List elements) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.addAll(elements);
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* PaymentIntentConfirmParams#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link PaymentIntentConfirmParams#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* ID of the mandate to be used for this payment.
*/
public Builder setMandate(String mandate) {
this.mandate = mandate;
return this;
}
/**
* This hash contains details about the Mandate to create.
*/
public Builder setMandateData(MandateData mandateData) {
this.mandateData = mandateData;
return this;
}
/**
* Set to {@code true} to indicate that the customer is not in your checkout flow during this
* payment attempt, and therefore is unable to authenticate. This parameter is intended for
* scenarios where you collect card details and charge them later.
*/
public Builder setOffSession(Boolean offSession) {
this.offSession = offSession;
return this;
}
/**
* Set to {@code true} to indicate that the customer is not in your checkout flow during this
* payment attempt, and therefore is unable to authenticate. This parameter is intended for
* scenarios where you collect card details and charge them later.
*/
public Builder setOffSession(OffSession offSession) {
this.offSession = offSession;
return this;
}
/**
* ID of the payment method (a PaymentMethod, Card, or compatible Source
* object) to attach to this PaymentIntent.
*/
public Builder setPaymentMethod(String paymentMethod) {
this.paymentMethod = paymentMethod;
return this;
}
/**
* Payment-method-specific configuration for this PaymentIntent.
*/
public Builder setPaymentMethodOptions(PaymentMethodOptions paymentMethodOptions) {
this.paymentMethodOptions = paymentMethodOptions;
return this;
}
/**
* Email address that the receipt for the resulting payment will be sent to.
*/
public Builder setReceiptEmail(String receiptEmail) {
this.receiptEmail = receiptEmail;
return this;
}
/**
* Email address that the receipt for the resulting payment will be sent to.
*/
public Builder setReceiptEmail(EmptyParam receiptEmail) {
this.receiptEmail = receiptEmail;
return this;
}
/**
* The URL to redirect your customer back to after they authenticate or cancel their payment on
* the payment method's app or site. If you'd prefer to redirect to a mobile application, you
* can alternatively supply an application URI scheme. This parameter is only used for cards and
* other redirect-based payment methods.
*/
public Builder setReturnUrl(String returnUrl) {
this.returnUrl = returnUrl;
return this;
}
/**
* If the PaymentIntent has a {@code payment_method} and a {@code customer} or if you're
* attaching a payment method to the PaymentIntent in this request, you can pass {@code
* save_payment_method=true} to save the payment method to the customer. Defaults to {@code
* false}.
*
* If the payment method is already saved to a customer, this does nothing. If this type of
* payment method cannot be saved to a customer, the request will error.
*
*
Note that saving a payment method using this parameter does not guarantee that the
* payment method can be charged. To ensure that only payment methods which can be charged
* are saved to a customer, you can manually save
* the payment method in response to the {@code
* payment_intent.succeeded} webhook.
*/
public Builder setSavePaymentMethod(Boolean savePaymentMethod) {
this.savePaymentMethod = savePaymentMethod;
return this;
}
/**
* Indicates that you intend to make future payments with this PaymentIntent's payment method.
*
*
If present, the payment method used with this PaymentIntent can be attached to a Customer, even
* after the transaction completes.
*
*
Use {@code on_session} if you intend to only reuse the payment method when your customer
* is present in your checkout flow. Use {@code off_session} if your customer may or may not be
* in your checkout flow.
*
*
Stripe uses {@code setup_future_usage} to dynamically optimize your payment flow and
* comply with regional legislation and network rules. For example, if your customer is impacted
* by SCA, using {@code
* off_session} will ensure that they are authenticated while processing this PaymentIntent. You
* will then be able to collect off-session
* payments for this customer.
*
*
If {@code setup_future_usage} is already set and you are performing a request using a
* publishable key, you may only update the value from {@code on_session} to {@code
* off_session}.
*/
public Builder setSetupFutureUsage(SetupFutureUsage setupFutureUsage) {
this.setupFutureUsage = setupFutureUsage;
return this;
}
/**
* Indicates that you intend to make future payments with this PaymentIntent's payment method.
*
*
If present, the payment method used with this PaymentIntent can be attached to a Customer, even
* after the transaction completes.
*
*
Use {@code on_session} if you intend to only reuse the payment method when your customer
* is present in your checkout flow. Use {@code off_session} if your customer may or may not be
* in your checkout flow.
*
*
Stripe uses {@code setup_future_usage} to dynamically optimize your payment flow and
* comply with regional legislation and network rules. For example, if your customer is impacted
* by SCA, using {@code
* off_session} will ensure that they are authenticated while processing this PaymentIntent. You
* will then be able to collect off-session
* payments for this customer.
*
*
If {@code setup_future_usage} is already set and you are performing a request using a
* publishable key, you may only update the value from {@code on_session} to {@code
* off_session}.
*/
public Builder setSetupFutureUsage(EmptyParam setupFutureUsage) {
this.setupFutureUsage = setupFutureUsage;
return this;
}
/**
* Shipping information for this PaymentIntent.
*/
public Builder setShipping(Shipping shipping) {
this.shipping = shipping;
return this;
}
/**
* Shipping information for this PaymentIntent.
*/
public Builder setShipping(EmptyParam shipping) {
this.shipping = shipping;
return this;
}
/**
* This is a legacy field that will be removed in the future. It is the ID of the Source object
* to attach to this PaymentIntent. Please use the {@code payment_method} field instead, which
* also supports Cards and compatible Source
* objects.
*/
public Builder setSource(String source) {
this.source = source;
return this;
}
/**
* Set to {@code true} only when using manual confirmation and the iOS or Android SDKs to handle
* additional authentication steps.
*/
public Builder setUseStripeSdk(Boolean useStripeSdk) {
this.useStripeSdk = useStripeSdk;
return this;
}
}
public static class MandateData {
/**
* This hash contains details about the customer acceptance of the Mandate.
*/
@SerializedName("customer_acceptance")
CustomerAcceptance customerAcceptance;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
private MandateData(CustomerAcceptance customerAcceptance, Map extraParams) {
this.customerAcceptance = customerAcceptance;
this.extraParams = extraParams;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private CustomerAcceptance customerAcceptance;
private Map extraParams;
/**
* Finalize and obtain parameter instance from this builder.
*/
public MandateData build() {
return new MandateData(this.customerAcceptance, this.extraParams);
}
/**
* This hash contains details about the customer acceptance of the Mandate.
*/
public Builder setCustomerAcceptance(CustomerAcceptance customerAcceptance) {
this.customerAcceptance = customerAcceptance;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* PaymentIntentConfirmParams.MandateData#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link PaymentIntentConfirmParams.MandateData#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
}
public static class CustomerAcceptance {
/** The time at which the customer accepted the Mandate. */
@SerializedName("accepted_at")
Long acceptedAt;
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* If this is a Mandate accepted offline, this hash contains details about the offline
* acceptance.
*/
@SerializedName("offline")
Offline offline;
/**
* If this is a Mandate accepted online, this hash contains details about the online
* acceptance.
*/
@SerializedName("online")
Online online;
/**
* The type of customer acceptance information included with the Mandate. One of {@code
* online} or {@code offline}.
*/
@SerializedName("type")
Type type;
private CustomerAcceptance(Long acceptedAt, Map extraParams, Offline offline, Online online, Type type) {
this.acceptedAt = acceptedAt;
this.extraParams = extraParams;
this.offline = offline;
this.online = online;
this.type = type;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Long acceptedAt;
private Map extraParams;
private Offline offline;
private Online online;
private Type type;
/** Finalize and obtain parameter instance from this builder. */
public CustomerAcceptance build() {
return new CustomerAcceptance(this.acceptedAt, this.extraParams, this.offline, this.online, this.type);
}
/** The time at which the customer accepted the Mandate. */
public Builder setAcceptedAt(Long acceptedAt) {
this.acceptedAt = acceptedAt;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link PaymentIntentConfirmParams.MandateData.CustomerAcceptance#extraParams}
* for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link PaymentIntentConfirmParams.MandateData.CustomerAcceptance#extraParams}
* for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* If this is a Mandate accepted offline, this hash contains details about the offline
* acceptance.
*/
public Builder setOffline(Offline offline) {
this.offline = offline;
return this;
}
/**
* If this is a Mandate accepted online, this hash contains details about the online
* acceptance.
*/
public Builder setOnline(Online online) {
this.online = online;
return this;
}
/**
* The type of customer acceptance information included with the Mandate. One of {@code
* online} or {@code offline}.
*/
public Builder setType(Type type) {
this.type = type;
return this;
}
}
public static class Offline {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field
* (serialized) name in this param object. Effectively, this map is flattened to its parent
* instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
private Offline(Map extraParams) {
this.extraParams = extraParams;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
/** Finalize and obtain parameter instance from this builder. */
public Offline build() {
return new Offline(this.extraParams);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* PaymentIntentConfirmParams.MandateData.CustomerAcceptance.Offline#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* PaymentIntentConfirmParams.MandateData.CustomerAcceptance.Offline#extraParams} for the
* field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
}
public static class Online {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field
* (serialized) name in this param object. Effectively, this map is flattened to its parent
* instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/** The IP address from which the Mandate was accepted by the customer. */
@SerializedName("ip_address")
String ipAddress;
/** The user agent of the browser from which the Mandate was accepted by the customer. */
@SerializedName("user_agent")
String userAgent;
private Online(Map extraParams, String ipAddress, String userAgent) {
this.extraParams = extraParams;
this.ipAddress = ipAddress;
this.userAgent = userAgent;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private String ipAddress;
private String userAgent;
/** Finalize and obtain parameter instance from this builder. */
public Online build() {
return new Online(this.extraParams, this.ipAddress, this.userAgent);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* PaymentIntentConfirmParams.MandateData.CustomerAcceptance.Online#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* PaymentIntentConfirmParams.MandateData.CustomerAcceptance.Online#extraParams} for the
* field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/** The IP address from which the Mandate was accepted by the customer. */
public Builder setIpAddress(String ipAddress) {
this.ipAddress = ipAddress;
return this;
}
public Builder setUserAgent(String userAgent) {
this.userAgent = userAgent;
return this;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getIpAddress() {
return this.ipAddress;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUserAgent() {
return this.userAgent;
}
}
public enum Type implements ApiRequestParams.EnumParam {
@SerializedName("offline")
OFFLINE("offline"), @SerializedName("online")
ONLINE("online");
private final String value;
Type(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAcceptedAt() {
return this.acceptedAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Offline getOffline() {
return this.offline;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Online getOnline() {
return this.online;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Type getType() {
return this.type;
}
}
/**
* This hash contains details about the customer acceptance of the Mandate.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public CustomerAcceptance getCustomerAcceptance() {
return this.customerAcceptance;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
}
/**
* The user agent of the browser from which the Mandate was accepted by the customer.
*/
public static class PaymentMethodOptions {
/**
* Configuration for any card payments attempted on this PaymentIntent.
*/
@SerializedName("card")
Card card;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
private PaymentMethodOptions(Card card, Map extraParams) {
this.card = card;
this.extraParams = extraParams;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Card card;
private Map extraParams;
/**
* Finalize and obtain parameter instance from this builder.
*/
public PaymentMethodOptions build() {
return new PaymentMethodOptions(this.card, this.extraParams);
}
/**
* Configuration for any card payments attempted on this PaymentIntent.
*/
public Builder setCard(Card card) {
this.card = card;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* PaymentIntentConfirmParams.PaymentMethodOptions#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link PaymentIntentConfirmParams.PaymentMethodOptions#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
}
public static class Card {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Installment configuration for payments attempted on this PaymentIntent (Mexico Only).
*
* For more information, see the installments integration guide.
*/
@SerializedName("installments")
Installments installments;
/**
* When specified, this parameter indicates that a transaction will be marked as MOTO (Mail
* Order Telephone Order) and thus out of scope for SCA. This parameter can only be provided
* during confirmation.
*/
@SerializedName("moto")
Boolean moto;
/**
* We strongly recommend that you rely on our SCA Engine to automatically prompt your
* customers for authentication based on risk level and other requirements.
* However, if you wish to request 3D Secure based on logic from your own fraud engine,
* provide this option. Permitted values include: {@code automatic} or {@code any}. If not
* provided, defaults to {@code automatic}. Read our guide on manually requesting 3D
* Secure for more information on how this configuration interacts with Radar and our SCA
* Engine.
*/
@SerializedName("request_three_d_secure")
RequestThreeDSecure requestThreeDSecure;
private Card(Map extraParams, Installments installments, Boolean moto, RequestThreeDSecure requestThreeDSecure) {
this.extraParams = extraParams;
this.installments = installments;
this.moto = moto;
this.requestThreeDSecure = requestThreeDSecure;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private Installments installments;
private Boolean moto;
private RequestThreeDSecure requestThreeDSecure;
/** Finalize and obtain parameter instance from this builder. */
public Card build() {
return new Card(this.extraParams, this.installments, this.moto, this.requestThreeDSecure);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link PaymentIntentConfirmParams.PaymentMethodOptions.Card#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link PaymentIntentConfirmParams.PaymentMethodOptions.Card#extraParams} for the
* field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Installment configuration for payments attempted on this PaymentIntent (Mexico Only).
*
* For more information, see the installments integration guide.
*/
public Builder setInstallments(Installments installments) {
this.installments = installments;
return this;
}
/**
* When specified, this parameter indicates that a transaction will be marked as MOTO (Mail
* Order Telephone Order) and thus out of scope for SCA. This parameter can only be provided
* during confirmation.
*/
public Builder setMoto(Boolean moto) {
this.moto = moto;
return this;
}
/**
* We strongly recommend that you rely on our SCA Engine to automatically prompt your
* customers for authentication based on risk level and other requirements.
* However, if you wish to request 3D Secure based on logic from your own fraud engine,
* provide this option. Permitted values include: {@code automatic} or {@code any}. If not
* provided, defaults to {@code automatic}. Read our guide on manually requesting 3D
* Secure for more information on how this configuration interacts with Radar and our
* SCA Engine.
*/
public Builder setRequestThreeDSecure(RequestThreeDSecure requestThreeDSecure) {
this.requestThreeDSecure = requestThreeDSecure;
return this;
}
}
public static class Installments {
/**
* Setting to true enables installments for this PaymentIntent. This will cause the response
* to contain a list of available installment plans. Setting to false will prevent any
* selected plan from applying to a charge.
*/
@SerializedName("enabled")
Boolean enabled;
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field
* (serialized) name in this param object. Effectively, this map is flattened to its parent
* instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* The selected installment plan to use for this payment attempt. This parameter can only be
* provided during confirmation.
*/
@SerializedName("plan")
Object plan;
private Installments(Boolean enabled, Map extraParams, Object plan) {
this.enabled = enabled;
this.extraParams = extraParams;
this.plan = plan;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Boolean enabled;
private Map extraParams;
private Object plan;
/** Finalize and obtain parameter instance from this builder. */
public Installments build() {
return new Installments(this.enabled, this.extraParams, this.plan);
}
/**
* Setting to true enables installments for this PaymentIntent. This will cause the
* response to contain a list of available installment plans. Setting to false will
* prevent any selected plan from applying to a charge.
*/
public Builder setEnabled(Boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* PaymentIntentConfirmParams.PaymentMethodOptions.Card.Installments#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* PaymentIntentConfirmParams.PaymentMethodOptions.Card.Installments#extraParams} for the
* field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* The selected installment plan to use for this payment attempt. This parameter can only
* be provided during confirmation.
*/
public Builder setPlan(Plan plan) {
this.plan = plan;
return this;
}
/**
* The selected installment plan to use for this payment attempt. This parameter can only
* be provided during confirmation.
*/
public Builder setPlan(EmptyParam plan) {
this.plan = plan;
return this;
}
}
public static class Plan {
/**
* For {@code fixed_count} installment plans, this is the number of installment payments
* your customer will make to their credit card.
*/
@SerializedName("count")
Long count;
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field
* (serialized) name in this param object. Effectively, this map is flattened to its
* parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* For {@code fixed_count} installment plans, this is the interval between installment
* payments your customer will make to their credit card. One of {@code month}.
*/
@SerializedName("interval")
Interval interval;
/** Type of installment plan, one of {@code fixed_count}. */
@SerializedName("type")
Type type;
private Plan(Long count, Map extraParams, Interval interval, Type type) {
this.count = count;
this.extraParams = extraParams;
this.interval = interval;
this.type = type;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Long count;
private Map extraParams;
private Interval interval;
private Type type;
/** Finalize and obtain parameter instance from this builder. */
public Plan build() {
return new Plan(this.count, this.extraParams, this.interval, this.type);
}
/**
* For {@code fixed_count} installment plans, this is the number of installment payments
* your customer will make to their credit card.
*/
public Builder setCount(Long count) {
this.count = count;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the
* original map. See {@link
* PaymentIntentConfirmParams.PaymentMethodOptions.Card.Installments.Plan#extraParams}
* for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the
* original map. See {@link
* PaymentIntentConfirmParams.PaymentMethodOptions.Card.Installments.Plan#extraParams}
* for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* For {@code fixed_count} installment plans, this is the interval between installment
* payments your customer will make to their credit card. One of {@code month}.
*/
public Builder setInterval(Interval interval) {
this.interval = interval;
return this;
}
public Builder setType(Type type) {
this.type = type;
return this;
}
}
public enum Interval implements ApiRequestParams.EnumParam {
@SerializedName("month")
MONTH("month");
private final String value;
Interval(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum Type implements ApiRequestParams.EnumParam {
@SerializedName("fixed_count")
FIXED_COUNT("fixed_count");
private final String value;
Type(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCount() {
return this.count;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Interval getInterval() {
return this.interval;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Type getType() {
return this.type;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getEnabled() {
return this.enabled;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getPlan() {
return this.plan;
}
}
public enum RequestThreeDSecure implements ApiRequestParams.EnumParam {
@SerializedName("any")
ANY("any"), @SerializedName("automatic")
AUTOMATIC("automatic");
private final String value;
RequestThreeDSecure(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Installments getInstallments() {
return this.installments;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getMoto() {
return this.moto;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public RequestThreeDSecure getRequestThreeDSecure() {
return this.requestThreeDSecure;
}
}
/**
* Configuration for any card payments attempted on this PaymentIntent.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Card getCard() {
return this.card;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
}
/**
* Type of installment plan, one of {@code fixed_count}.
*/
public static class Shipping {
/**
* Shipping address.
*/
@SerializedName("address")
Address address;
/**
* The delivery service that shipped a physical product, such as Fedex, UPS, USPS, etc.
*/
@SerializedName("carrier")
String carrier;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Recipient name.
*/
@SerializedName("name")
String name;
/**
* Recipient phone (including extension).
*/
@SerializedName("phone")
String phone;
/**
* The tracking number for a physical product, obtained from the delivery service. If multiple
* tracking numbers were generated for this purchase, please separate them with commas.
*/
@SerializedName("tracking_number")
String trackingNumber;
private Shipping(Address address, String carrier, Map extraParams, String name, String phone, String trackingNumber) {
this.address = address;
this.carrier = carrier;
this.extraParams = extraParams;
this.name = name;
this.phone = phone;
this.trackingNumber = trackingNumber;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Address address;
private String carrier;
private Map extraParams;
private String name;
private String phone;
private String trackingNumber;
/**
* Finalize and obtain parameter instance from this builder.
*/
public Shipping build() {
return new Shipping(this.address, this.carrier, this.extraParams, this.name, this.phone, this.trackingNumber);
}
/**
* Shipping address.
*/
public Builder setAddress(Address address) {
this.address = address;
return this;
}
/**
* The delivery service that shipped a physical product, such as Fedex, UPS, USPS, etc.
*/
public Builder setCarrier(String carrier) {
this.carrier = carrier;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* PaymentIntentConfirmParams.Shipping#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link PaymentIntentConfirmParams.Shipping#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Recipient name.
*/
public Builder setName(String name) {
this.name = name;
return this;
}
/**
* Recipient phone (including extension).
*/
public Builder setPhone(String phone) {
this.phone = phone;
return this;
}
/**
* The tracking number for a physical product, obtained from the delivery service. If multiple
* tracking numbers were generated for this purchase, please separate them with commas.
*/
public Builder setTrackingNumber(String trackingNumber) {
this.trackingNumber = trackingNumber;
return this;
}
}
public static class Address {
/** City, district, suburb, town, or village. */
@SerializedName("city")
String city;
/**
* Two-letter country code (ISO
* 3166-1 alpha-2).
*/
@SerializedName("country")
String country;
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/** Address line 1 (e.g., street, PO Box, or company name). */
@SerializedName("line1")
String line1;
/** Address line 2 (e.g., apartment, suite, unit, or building). */
@SerializedName("line2")
String line2;
/** ZIP or postal code. */
@SerializedName("postal_code")
String postalCode;
/** State, county, province, or region. */
@SerializedName("state")
String state;
private Address(String city, String country, Map extraParams, String line1, String line2, String postalCode, String state) {
this.city = city;
this.country = country;
this.extraParams = extraParams;
this.line1 = line1;
this.line2 = line2;
this.postalCode = postalCode;
this.state = state;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private String city;
private String country;
private Map extraParams;
private String line1;
private String line2;
private String postalCode;
private String state;
/** Finalize and obtain parameter instance from this builder. */
public Address build() {
return new Address(this.city, this.country, this.extraParams, this.line1, this.line2, this.postalCode, this.state);
}
/** City, district, suburb, town, or village. */
public Builder setCity(String city) {
this.city = city;
return this;
}
/**
* Two-letter country code (ISO
* 3166-1 alpha-2).
*/
public Builder setCountry(String country) {
this.country = country;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link PaymentIntentConfirmParams.Shipping.Address#extraParams} for the field
* documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link PaymentIntentConfirmParams.Shipping.Address#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/** Address line 1 (e.g., street, PO Box, or company name). */
public Builder setLine1(String line1) {
this.line1 = line1;
return this;
}
/** Address line 2 (e.g., apartment, suite, unit, or building). */
public Builder setLine2(String line2) {
this.line2 = line2;
return this;
}
/** ZIP or postal code. */
public Builder setPostalCode(String postalCode) {
this.postalCode = postalCode;
return this;
}
public Builder setState(String state) {
this.state = state;
return this;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCity() {
return this.city;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCountry() {
return this.country;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLine1() {
return this.line1;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLine2() {
return this.line2;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPostalCode() {
return this.postalCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getState() {
return this.state;
}
}
/**
* Shipping address.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Address getAddress() {
return this.address;
}
/**
* The delivery service that shipped a physical product, such as Fedex, UPS, USPS, etc.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCarrier() {
return this.carrier;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Recipient name.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getName() {
return this.name;
}
/**
* Recipient phone (including extension).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPhone() {
return this.phone;
}
/**
* The tracking number for a physical product, obtained from the delivery service. If multiple
* tracking numbers were generated for this purchase, please separate them with commas.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTrackingNumber() {
return this.trackingNumber;
}
}
/**
* State, county, province, or region.
*/
public enum OffSession implements ApiRequestParams.EnumParam {
@SerializedName("one_off")
ONE_OFF("one_off"), @SerializedName("recurring")
RECURRING("recurring");
private final String value;
OffSession(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum SetupFutureUsage implements ApiRequestParams.EnumParam {
@SerializedName("off_session")
OFF_SESSION("off_session"), @SerializedName("on_session")
ON_SESSION("on_session");
private final String value;
SetupFutureUsage(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
/**
* Set to {@code true} to fail the payment attempt if the PaymentIntent transitions into {@code
* requires_action}. This parameter is intended for simpler integrations that do not handle
* customer actions, like saving cards without
* authentication.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getErrorOnRequiresAction() {
return this.errorOnRequiresAction;
}
/**
* Specifies which fields in the response should be expanded.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getExpand() {
return this.expand;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* ID of the mandate to be used for this payment.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getMandate() {
return this.mandate;
}
/**
* This hash contains details about the Mandate to create.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getMandateData() {
return this.mandateData;
}
/**
* Set to {@code true} to indicate that the customer is not in your checkout flow during this
* payment attempt, and therefore is unable to authenticate. This parameter is intended for
* scenarios where you collect card details and charge them later.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getOffSession() {
return this.offSession;
}
/**
* ID of the payment method (a PaymentMethod, Card, or compatible Source
* object) to attach to this PaymentIntent.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPaymentMethod() {
return this.paymentMethod;
}
/**
* Payment-method-specific configuration for this PaymentIntent.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PaymentMethodOptions getPaymentMethodOptions() {
return this.paymentMethodOptions;
}
/**
* Email address that the receipt for the resulting payment will be sent to.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getReceiptEmail() {
return this.receiptEmail;
}
/**
* The URL to redirect your customer back to after they authenticate or cancel their payment on
* the payment method's app or site. If you'd prefer to redirect to a mobile application, you can
* alternatively supply an application URI scheme. This parameter is only used for cards and other
* redirect-based payment methods.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReturnUrl() {
return this.returnUrl;
}
/**
* If the PaymentIntent has a {@code payment_method} and a {@code customer} or if you're attaching
* a payment method to the PaymentIntent in this request, you can pass {@code
* save_payment_method=true} to save the payment method to the customer. Defaults to {@code
* false}.
*
* If the payment method is already saved to a customer, this does nothing. If this type of
* payment method cannot be saved to a customer, the request will error.
*
*
Note that saving a payment method using this parameter does not guarantee that the
* payment method can be charged. To ensure that only payment methods which can be charged
* are saved to a customer, you can manually save
* the payment method in response to the {@code
* payment_intent.succeeded} webhook.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getSavePaymentMethod() {
return this.savePaymentMethod;
}
/**
* Indicates that you intend to make future payments with this PaymentIntent's payment method.
*
*
If present, the payment method used with this PaymentIntent can be attached to a Customer, even
* after the transaction completes.
*
*
Use {@code on_session} if you intend to only reuse the payment method when your customer is
* present in your checkout flow. Use {@code off_session} if your customer may or may not be in
* your checkout flow.
*
*
Stripe uses {@code setup_future_usage} to dynamically optimize your payment flow and comply
* with regional legislation and network rules. For example, if your customer is impacted by SCA, using {@code
* off_session} will ensure that they are authenticated while processing this PaymentIntent. You
* will then be able to collect off-session
* payments for this customer.
*
*
If {@code setup_future_usage} is already set and you are performing a request using a
* publishable key, you may only update the value from {@code on_session} to {@code off_session}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public EnumParam getSetupFutureUsage() {
return this.setupFutureUsage;
}
/**
* Shipping information for this PaymentIntent.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getShipping() {
return this.shipping;
}
/**
* This is a legacy field that will be removed in the future. It is the ID of the Source object to
* attach to this PaymentIntent. Please use the {@code payment_method} field instead, which also
* supports Cards and compatible Source
* objects.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSource() {
return this.source;
}
/**
* Set to {@code true} only when using manual confirmation and the iOS or Android SDKs to handle
* additional authentication steps.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getUseStripeSdk() {
return this.useStripeSdk;
}
}