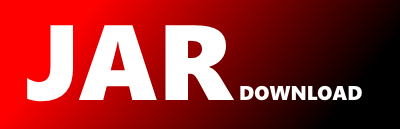
com.stripe.model.LineItem Maven / Gradle / Ivy
// Generated by delombok at Mon May 18 17:31:35 PDT 2020
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
import java.util.List;
public class LineItem extends StripeObject implements HasId {
/**
* Total before any discounts or taxes is applied.
*/
@SerializedName("amount_subtotal")
Long amountSubtotal;
/**
* Total after discounts and taxes.
*/
@SerializedName("amount_total")
Long amountTotal;
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@SerializedName("currency")
String currency;
/**
* An arbitrary string attached to the object. Often useful for displaying to users. Defaults to
* product name.
*/
@SerializedName("description")
String description;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code item}.
*/
@SerializedName("object")
String object;
/**
* Prices define the unit cost, currency, and (optional) billing cycle for both recurring and
* one-time purchases of products. Products
* help you track inventory or provisioning, and prices help you track payment terms. Different
* physical goods or levels of service should be represented by products, and pricing options
* should be represented by prices. This approach lets you change prices without having to change
* your provisioning scheme.
*
*
For example, you might have a single "gold" product that has prices for $10/month,
* $100/year, and €9 once.
*
*
Related guides: Set up a
* subscription, create an
* invoice, and more about products and
* prices.
*/
@SerializedName("price")
Price price;
/**
* The quantity of products being purchased.
*/
@SerializedName("quantity")
Long quantity;
/**
* The taxes applied to the line item.
*/
@SerializedName("taxes")
List taxes;
public static class Tax extends StripeObject {
/** Amount of tax for this line item. */
@SerializedName("amount")
Long amount;
/**
* Tax rates can be applied to invoices and subscriptions to collect tax.
*
* Related guide: Tax Rates.
*/
@SerializedName("rate")
TaxRate rate;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TaxRate getRate() {
return this.rate;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmount(final Long amount) {
this.amount = amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRate(final TaxRate rate) {
this.rate = rate;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof LineItem.Tax)) return false;
final LineItem.Tax other = (LineItem.Tax) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$rate = this.getRate();
final java.lang.Object other$rate = other.getRate();
if (this$rate == null ? other$rate != null : !this$rate.equals(other$rate)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof LineItem.Tax;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $rate = this.getRate();
result = result * PRIME + ($rate == null ? 43 : $rate.hashCode());
return result;
}
}
/**
* Total before any discounts or taxes is applied.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountSubtotal() {
return this.amountSubtotal;
}
/**
* Total after discounts and taxes.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountTotal() {
return this.amountTotal;
}
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users. Defaults to
* product name.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDescription() {
return this.description;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code item}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* Prices define the unit cost, currency, and (optional) billing cycle for both recurring and
* one-time purchases of products. Products
* help you track inventory or provisioning, and prices help you track payment terms. Different
* physical goods or levels of service should be represented by products, and pricing options
* should be represented by prices. This approach lets you change prices without having to change
* your provisioning scheme.
*
*
For example, you might have a single "gold" product that has prices for $10/month,
* $100/year, and €9 once.
*
*
Related guides: Set up a
* subscription, create an
* invoice, and more about products and
* prices.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Price getPrice() {
return this.price;
}
/**
* The quantity of products being purchased.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getQuantity() {
return this.quantity;
}
/**
* The taxes applied to the line item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getTaxes() {
return this.taxes;
}
/**
* Total before any discounts or taxes is applied.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountSubtotal(final Long amountSubtotal) {
this.amountSubtotal = amountSubtotal;
}
/**
* Total after discounts and taxes.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountTotal(final Long amountTotal) {
this.amountTotal = amountTotal;
}
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users. Defaults to
* product name.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDescription(final String description) {
this.description = description;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code item}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* Prices define the unit cost, currency, and (optional) billing cycle for both recurring and
* one-time purchases of products. Products
* help you track inventory or provisioning, and prices help you track payment terms. Different
* physical goods or levels of service should be represented by products, and pricing options
* should be represented by prices. This approach lets you change prices without having to change
* your provisioning scheme.
*
*
For example, you might have a single "gold" product that has prices for $10/month,
* $100/year, and €9 once.
*
*
Related guides: Set up a
* subscription, create an
* invoice, and more about products and
* prices.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPrice(final Price price) {
this.price = price;
}
/**
* The quantity of products being purchased.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setQuantity(final Long quantity) {
this.quantity = quantity;
}
/**
* The taxes applied to the line item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxes(final List taxes) {
this.taxes = taxes;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof LineItem)) return false;
final LineItem other = (LineItem) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amountSubtotal = this.getAmountSubtotal();
final java.lang.Object other$amountSubtotal = other.getAmountSubtotal();
if (this$amountSubtotal == null ? other$amountSubtotal != null : !this$amountSubtotal.equals(other$amountSubtotal)) return false;
final java.lang.Object this$amountTotal = this.getAmountTotal();
final java.lang.Object other$amountTotal = other.getAmountTotal();
if (this$amountTotal == null ? other$amountTotal != null : !this$amountTotal.equals(other$amountTotal)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$price = this.getPrice();
final java.lang.Object other$price = other.getPrice();
if (this$price == null ? other$price != null : !this$price.equals(other$price)) return false;
final java.lang.Object this$quantity = this.getQuantity();
final java.lang.Object other$quantity = other.getQuantity();
if (this$quantity == null ? other$quantity != null : !this$quantity.equals(other$quantity)) return false;
final java.lang.Object this$taxes = this.getTaxes();
final java.lang.Object other$taxes = other.getTaxes();
if (this$taxes == null ? other$taxes != null : !this$taxes.equals(other$taxes)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof LineItem;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amountSubtotal = this.getAmountSubtotal();
result = result * PRIME + ($amountSubtotal == null ? 43 : $amountSubtotal.hashCode());
final java.lang.Object $amountTotal = this.getAmountTotal();
result = result * PRIME + ($amountTotal == null ? 43 : $amountTotal.hashCode());
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $price = this.getPrice();
result = result * PRIME + ($price == null ? 43 : $price.hashCode());
final java.lang.Object $quantity = this.getQuantity();
result = result * PRIME + ($quantity == null ? 43 : $quantity.hashCode());
final java.lang.Object $taxes = this.getTaxes();
result = result * PRIME + ($taxes == null ? 43 : $taxes.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
}