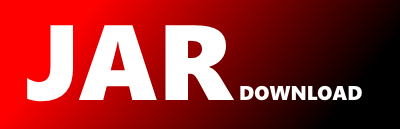
com.stripe.param.InvoiceItemCreateParams Maven / Gradle / Ivy
// Generated by delombok at Mon May 18 17:31:35 PDT 2020
package com.stripe.param;
import com.google.gson.annotations.SerializedName;
import com.stripe.net.ApiRequestParams;
import com.stripe.param.common.EmptyParam;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class InvoiceItemCreateParams extends ApiRequestParams {
/**
* The integer amount in %s of the charge to be applied to the upcoming invoice.
* Passing in a negative {@code amount} will reduce the {@code amount_due} on the invoice.
*/
@SerializedName("amount")
Long amount;
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@SerializedName("currency")
String currency;
/**
* The ID of the customer who will be billed when this invoice item is billed.
*/
@SerializedName("customer")
String customer;
/**
* An arbitrary string which you can attach to the invoice item. The description is displayed in
* the invoice for easy tracking.
*/
@SerializedName("description")
String description;
/**
* Controls whether discounts apply to this invoice item. Defaults to false for prorations or
* negative invoice items, and true for all other invoice items.
*/
@SerializedName("discountable")
Boolean discountable;
/**
* Specifies which fields in the response should be expanded.
*/
@SerializedName("expand")
List expand;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* The ID of an existing invoice to add this invoice item to. When left blank, the invoice item
* will be added to the next upcoming scheduled invoice. This is useful when adding invoice items
* in response to an invoice.created webhook. You can only add invoice items to draft invoices.
*/
@SerializedName("invoice")
String invoice;
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset by
* posting an empty value to them. All keys can be unset by posting an empty value to {@code
* metadata}.
*/
@SerializedName("metadata")
Object metadata;
/**
* The period associated with this invoice item.
*/
@SerializedName("period")
Period period;
/**
* The ID of the price object.
*/
@SerializedName("price")
String price;
/**
* Data used to generate a new price object inline.
*/
@SerializedName("price_data")
PriceData priceData;
/**
* Non-negative integer. The quantity of units for the invoice item.
*/
@SerializedName("quantity")
Long quantity;
/**
* The ID of a subscription to add this invoice item to. When left blank, the invoice item will be
* be added to the next upcoming scheduled invoice. When set, scheduled invoices for subscriptions
* other than the specified subscription will ignore the invoice item. Use this when you want to
* express that an invoice item has been accrued within the context of a particular subscription.
*/
@SerializedName("subscription")
String subscription;
/**
* The tax rates which apply to the invoice item. When set, the {@code default_tax_rates} on the
* invoice do not apply to this invoice item.
*/
@SerializedName("tax_rates")
List taxRates;
/**
* The integer unit amount in %s of the charge to be applied to the upcoming
* invoice. This {@code unit_amount} will be multiplied by the quantity to get the full amount.
* Passing in a negative {@code unit_amount} will reduce the {@code amount_due} on the invoice.
*/
@SerializedName("unit_amount")
Long unitAmount;
/**
* Same as {@code unit_amount}, but accepts a decimal value with at most 12 decimal places. Only
* one of {@code unit_amount} and {@code unit_amount_decimal} can be set.
*/
@SerializedName("unit_amount_decimal")
BigDecimal unitAmountDecimal;
private InvoiceItemCreateParams(Long amount, String currency, String customer, String description, Boolean discountable, List expand, Map extraParams, String invoice, Object metadata, Period period, String price, PriceData priceData, Long quantity, String subscription, List taxRates, Long unitAmount, BigDecimal unitAmountDecimal) {
this.amount = amount;
this.currency = currency;
this.customer = customer;
this.description = description;
this.discountable = discountable;
this.expand = expand;
this.extraParams = extraParams;
this.invoice = invoice;
this.metadata = metadata;
this.period = period;
this.price = price;
this.priceData = priceData;
this.quantity = quantity;
this.subscription = subscription;
this.taxRates = taxRates;
this.unitAmount = unitAmount;
this.unitAmountDecimal = unitAmountDecimal;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Long amount;
private String currency;
private String customer;
private String description;
private Boolean discountable;
private List expand;
private Map extraParams;
private String invoice;
private Object metadata;
private Period period;
private String price;
private PriceData priceData;
private Long quantity;
private String subscription;
private List taxRates;
private Long unitAmount;
private BigDecimal unitAmountDecimal;
/**
* Finalize and obtain parameter instance from this builder.
*/
public InvoiceItemCreateParams build() {
return new InvoiceItemCreateParams(this.amount, this.currency, this.customer, this.description, this.discountable, this.expand, this.extraParams, this.invoice, this.metadata, this.period, this.price, this.priceData, this.quantity, this.subscription, this.taxRates, this.unitAmount, this.unitAmountDecimal);
}
/**
* The integer amount in %s of the charge to be applied to the upcoming
* invoice. Passing in a negative {@code amount} will reduce the {@code amount_due} on the
* invoice.
*/
public Builder setAmount(Long amount) {
this.amount = amount;
return this;
}
/**
* Three-letter ISO currency
* code, in lowercase. Must be a supported
* currency.
*/
public Builder setCurrency(String currency) {
this.currency = currency;
return this;
}
/**
* The ID of the customer who will be billed when this invoice item is billed.
*/
public Builder setCustomer(String customer) {
this.customer = customer;
return this;
}
/**
* An arbitrary string which you can attach to the invoice item. The description is displayed in
* the invoice for easy tracking.
*/
public Builder setDescription(String description) {
this.description = description;
return this;
}
/**
* Controls whether discounts apply to this invoice item. Defaults to false for prorations or
* negative invoice items, and true for all other invoice items.
*/
public Builder setDiscountable(Boolean discountable) {
this.discountable = discountable;
return this;
}
/**
* Add an element to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* InvoiceItemCreateParams#expand} for the field documentation.
*/
public Builder addExpand(String element) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.add(element);
return this;
}
/**
* Add all elements to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* InvoiceItemCreateParams#expand} for the field documentation.
*/
public Builder addAllExpand(List elements) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.addAll(elements);
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* InvoiceItemCreateParams#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link InvoiceItemCreateParams#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* The ID of an existing invoice to add this invoice item to. When left blank, the invoice item
* will be added to the next upcoming scheduled invoice. This is useful when adding invoice
* items in response to an invoice.created webhook. You can only add invoice items to draft
* invoices.
*/
public Builder setInvoice(String invoice) {
this.invoice = invoice;
return this;
}
/**
* Add a key/value pair to `metadata` map. A map is initialized for the first `put/putAll` call,
* and subsequent calls add additional key/value pairs to the original map. See {@link
* InvoiceItemCreateParams#metadata} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder putMetadata(String key, String value) {
if (this.metadata == null || this.metadata instanceof EmptyParam) {
this.metadata = new HashMap();
}
((Map) this.metadata).put(key, value);
return this;
}
/**
* Add all map key/value pairs to `metadata` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link InvoiceItemCreateParams#metadata} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder putAllMetadata(Map map) {
if (this.metadata == null || this.metadata instanceof EmptyParam) {
this.metadata = new HashMap();
}
((Map) this.metadata).putAll(map);
return this;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset
* by posting an empty value to them. All keys can be unset by posting an empty value to {@code
* metadata}.
*/
public Builder setMetadata(EmptyParam metadata) {
this.metadata = metadata;
return this;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset
* by posting an empty value to them. All keys can be unset by posting an empty value to {@code
* metadata}.
*/
public Builder setMetadata(Map metadata) {
this.metadata = metadata;
return this;
}
/**
* The period associated with this invoice item.
*/
public Builder setPeriod(Period period) {
this.period = period;
return this;
}
/**
* The ID of the price object.
*/
public Builder setPrice(String price) {
this.price = price;
return this;
}
/**
* Data used to generate a new price object inline.
*/
public Builder setPriceData(PriceData priceData) {
this.priceData = priceData;
return this;
}
/**
* Non-negative integer. The quantity of units for the invoice item.
*/
public Builder setQuantity(Long quantity) {
this.quantity = quantity;
return this;
}
/**
* The ID of a subscription to add this invoice item to. When left blank, the invoice item will
* be be added to the next upcoming scheduled invoice. When set, scheduled invoices for
* subscriptions other than the specified subscription will ignore the invoice item. Use this
* when you want to express that an invoice item has been accrued within the context of a
* particular subscription.
*/
public Builder setSubscription(String subscription) {
this.subscription = subscription;
return this;
}
/**
* Add an element to `taxRates` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* InvoiceItemCreateParams#taxRates} for the field documentation.
*/
public Builder addTaxRate(String element) {
if (this.taxRates == null) {
this.taxRates = new ArrayList<>();
}
this.taxRates.add(element);
return this;
}
/**
* Add all elements to `taxRates` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* InvoiceItemCreateParams#taxRates} for the field documentation.
*/
public Builder addAllTaxRate(List elements) {
if (this.taxRates == null) {
this.taxRates = new ArrayList<>();
}
this.taxRates.addAll(elements);
return this;
}
/**
* The integer unit amount in %s of the charge to be applied to the upcoming
* invoice. This {@code unit_amount} will be multiplied by the quantity to get the full amount.
* Passing in a negative {@code unit_amount} will reduce the {@code amount_due} on the invoice.
*/
public Builder setUnitAmount(Long unitAmount) {
this.unitAmount = unitAmount;
return this;
}
/**
* Same as {@code unit_amount}, but accepts a decimal value with at most 12 decimal places. Only
* one of {@code unit_amount} and {@code unit_amount_decimal} can be set.
*/
public Builder setUnitAmountDecimal(BigDecimal unitAmountDecimal) {
this.unitAmountDecimal = unitAmountDecimal;
return this;
}
}
public static class Period {
/**
* The end of the period, which must be greater than or equal to the start.
*/
@SerializedName("end")
Long end;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* The start of the period.
*/
@SerializedName("start")
Long start;
private Period(Long end, Map extraParams, Long start) {
this.end = end;
this.extraParams = extraParams;
this.start = start;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Long end;
private Map extraParams;
private Long start;
/** Finalize and obtain parameter instance from this builder. */
public Period build() {
return new Period(this.end, this.extraParams, this.start);
}
/** The end of the period, which must be greater than or equal to the start. */
public Builder setEnd(Long end) {
this.end = end;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* InvoiceItemCreateParams.Period#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link InvoiceItemCreateParams.Period#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
public Builder setStart(Long start) {
this.start = start;
return this;
}
}
/**
* The end of the period, which must be greater than or equal to the start.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getEnd() {
return this.end;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* The start of the period.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getStart() {
return this.start;
}
}
/**
* The start of the period.
*/
public static class PriceData {
/**
* Three-letter ISO currency
* code, in lowercase. Must be a supported
* currency.
*/
@SerializedName("currency")
String currency;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/** The ID of the product that this price will belong to. */
@SerializedName("product")
String product;
/** A positive integer in %s (or 0 for a free price) representing how much to charge. */
@SerializedName("unit_amount")
Long unitAmount;
/**
* Same as {@code unit_amount}, but accepts a decimal value with at most 12 decimal places. Only
* one of {@code unit_amount} and {@code unit_amount_decimal} can be set.
*/
@SerializedName("unit_amount_decimal")
BigDecimal unitAmountDecimal;
private PriceData(String currency, Map extraParams, String product, Long unitAmount, BigDecimal unitAmountDecimal) {
this.currency = currency;
this.extraParams = extraParams;
this.product = product;
this.unitAmount = unitAmount;
this.unitAmountDecimal = unitAmountDecimal;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private String currency;
private Map extraParams;
private String product;
private Long unitAmount;
private BigDecimal unitAmountDecimal;
/** Finalize and obtain parameter instance from this builder. */
public PriceData build() {
return new PriceData(this.currency, this.extraParams, this.product, this.unitAmount, this.unitAmountDecimal);
}
/**
* Three-letter ISO currency
* code, in lowercase. Must be a supported
* currency.
*/
public Builder setCurrency(String currency) {
this.currency = currency;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* InvoiceItemCreateParams.PriceData#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link InvoiceItemCreateParams.PriceData#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/** The ID of the product that this price will belong to. */
public Builder setProduct(String product) {
this.product = product;
return this;
}
/** A positive integer in %s (or 0 for a free price) representing how much to charge. */
public Builder setUnitAmount(Long unitAmount) {
this.unitAmount = unitAmount;
return this;
}
/**
* Same as {@code unit_amount}, but accepts a decimal value with at most 12 decimal places.
* Only one of {@code unit_amount} and {@code unit_amount_decimal} can be set.
*/
public Builder setUnitAmountDecimal(BigDecimal unitAmountDecimal) {
this.unitAmountDecimal = unitAmountDecimal;
return this;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getProduct() {
return this.product;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getUnitAmount() {
return this.unitAmount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BigDecimal getUnitAmountDecimal() {
return this.unitAmountDecimal;
}
}
/**
* The integer amount in %s of the charge to be applied to the upcoming invoice.
* Passing in a negative {@code amount} will reduce the {@code amount_due} on the invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
/**
* The ID of the customer who will be billed when this invoice item is billed.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCustomer() {
return this.customer;
}
/**
* An arbitrary string which you can attach to the invoice item. The description is displayed in
* the invoice for easy tracking.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDescription() {
return this.description;
}
/**
* Controls whether discounts apply to this invoice item. Defaults to false for prorations or
* negative invoice items, and true for all other invoice items.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getDiscountable() {
return this.discountable;
}
/**
* Specifies which fields in the response should be expanded.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getExpand() {
return this.expand;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* The ID of an existing invoice to add this invoice item to. When left blank, the invoice item
* will be added to the next upcoming scheduled invoice. This is useful when adding invoice items
* in response to an invoice.created webhook. You can only add invoice items to draft invoices.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getInvoice() {
return this.invoice;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset by
* posting an empty value to them. All keys can be unset by posting an empty value to {@code
* metadata}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getMetadata() {
return this.metadata;
}
/**
* The period associated with this invoice item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Period getPeriod() {
return this.period;
}
/**
* The ID of the price object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPrice() {
return this.price;
}
/**
* Data used to generate a new price object inline.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PriceData getPriceData() {
return this.priceData;
}
/**
* Non-negative integer. The quantity of units for the invoice item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getQuantity() {
return this.quantity;
}
/**
* The ID of a subscription to add this invoice item to. When left blank, the invoice item will be
* be added to the next upcoming scheduled invoice. When set, scheduled invoices for subscriptions
* other than the specified subscription will ignore the invoice item. Use this when you want to
* express that an invoice item has been accrued within the context of a particular subscription.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSubscription() {
return this.subscription;
}
/**
* The tax rates which apply to the invoice item. When set, the {@code default_tax_rates} on the
* invoice do not apply to this invoice item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getTaxRates() {
return this.taxRates;
}
/**
* The integer unit amount in %s of the charge to be applied to the upcoming
* invoice. This {@code unit_amount} will be multiplied by the quantity to get the full amount.
* Passing in a negative {@code unit_amount} will reduce the {@code amount_due} on the invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getUnitAmount() {
return this.unitAmount;
}
/**
* Same as {@code unit_amount}, but accepts a decimal value with at most 12 decimal places. Only
* one of {@code unit_amount} and {@code unit_amount_decimal} can be set.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BigDecimal getUnitAmountDecimal() {
return this.unitAmountDecimal;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy