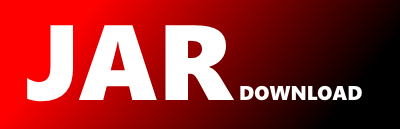
com.stripe.model.CreditNoteLineItem Maven / Gradle / Ivy
// Generated by delombok at Wed Jun 10 17:29:56 PDT 2020
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
import java.math.BigDecimal;
import java.util.List;
public class CreditNoteLineItem extends StripeObject implements HasId {
/**
* The integer amount in %s representing the gross amount being credited for this
* line item, excluding (exclusive) tax and discounts.
*/
@SerializedName("amount")
Long amount;
/**
* Description of the item being credited.
*/
@SerializedName("description")
String description;
/**
* The integer amount in %s representing the discount being credited for this
* line item.
*/
@SerializedName("discount_amount")
Long discountAmount;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* ID of the invoice line item being credited.
*/
@SerializedName("invoice_line_item")
String invoiceLineItem;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code credit_note_line_item}.
*/
@SerializedName("object")
String object;
/**
* The number of units of product being credited.
*/
@SerializedName("quantity")
Long quantity;
/**
* The amount of tax calculated per tax rate for this line item.
*/
@SerializedName("tax_amounts")
List taxAmounts;
/**
* The tax rates which apply to the line item.
*/
@SerializedName("tax_rates")
List taxRates;
/**
* The type of the credit note line item, one of {@code invoice_line_item} or {@code
* custom_line_item}. When the type is {@code invoice_line_item} there is an additional {@code
* invoice_line_item} property on the resource the value of which is the id of the credited line
* item on the invoice.
*/
@SerializedName("type")
String type;
/**
* The cost of each unit of product being credited.
*/
@SerializedName("unit_amount")
Long unitAmount;
/**
* Same as {@code unit_amount}, but contains a decimal value with at most 12 decimal places.
*/
@SerializedName("unit_amount_decimal")
BigDecimal unitAmountDecimal;
/**
* The integer amount in %s representing the gross amount being credited for this
* line item, excluding (exclusive) tax and discounts.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
/**
* Description of the item being credited.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDescription() {
return this.description;
}
/**
* The integer amount in %s representing the discount being credited for this
* line item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDiscountAmount() {
return this.discountAmount;
}
/**
* ID of the invoice line item being credited.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getInvoiceLineItem() {
return this.invoiceLineItem;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code credit_note_line_item}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* The number of units of product being credited.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getQuantity() {
return this.quantity;
}
/**
* The amount of tax calculated per tax rate for this line item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getTaxAmounts() {
return this.taxAmounts;
}
/**
* The tax rates which apply to the line item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getTaxRates() {
return this.taxRates;
}
/**
* The type of the credit note line item, one of {@code invoice_line_item} or {@code
* custom_line_item}. When the type is {@code invoice_line_item} there is an additional {@code
* invoice_line_item} property on the resource the value of which is the id of the credited line
* item on the invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
/**
* The cost of each unit of product being credited.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getUnitAmount() {
return this.unitAmount;
}
/**
* Same as {@code unit_amount}, but contains a decimal value with at most 12 decimal places.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BigDecimal getUnitAmountDecimal() {
return this.unitAmountDecimal;
}
/**
* The integer amount in %s representing the gross amount being credited for this
* line item, excluding (exclusive) tax and discounts.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmount(final Long amount) {
this.amount = amount;
}
/**
* Description of the item being credited.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDescription(final String description) {
this.description = description;
}
/**
* The integer amount in %s representing the discount being credited for this
* line item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDiscountAmount(final Long discountAmount) {
this.discountAmount = discountAmount;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* ID of the invoice line item being credited.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setInvoiceLineItem(final String invoiceLineItem) {
this.invoiceLineItem = invoiceLineItem;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code credit_note_line_item}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* The number of units of product being credited.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setQuantity(final Long quantity) {
this.quantity = quantity;
}
/**
* The amount of tax calculated per tax rate for this line item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxAmounts(final List taxAmounts) {
this.taxAmounts = taxAmounts;
}
/**
* The tax rates which apply to the line item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxRates(final List taxRates) {
this.taxRates = taxRates;
}
/**
* The type of the credit note line item, one of {@code invoice_line_item} or {@code
* custom_line_item}. When the type is {@code invoice_line_item} there is an additional {@code
* invoice_line_item} property on the resource the value of which is the id of the credited line
* item on the invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
/**
* The cost of each unit of product being credited.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUnitAmount(final Long unitAmount) {
this.unitAmount = unitAmount;
}
/**
* Same as {@code unit_amount}, but contains a decimal value with at most 12 decimal places.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUnitAmountDecimal(final BigDecimal unitAmountDecimal) {
this.unitAmountDecimal = unitAmountDecimal;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof CreditNoteLineItem)) return false;
final CreditNoteLineItem other = (CreditNoteLineItem) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$discountAmount = this.getDiscountAmount();
final java.lang.Object other$discountAmount = other.getDiscountAmount();
if (this$discountAmount == null ? other$discountAmount != null : !this$discountAmount.equals(other$discountAmount)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$invoiceLineItem = this.getInvoiceLineItem();
final java.lang.Object other$invoiceLineItem = other.getInvoiceLineItem();
if (this$invoiceLineItem == null ? other$invoiceLineItem != null : !this$invoiceLineItem.equals(other$invoiceLineItem)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$quantity = this.getQuantity();
final java.lang.Object other$quantity = other.getQuantity();
if (this$quantity == null ? other$quantity != null : !this$quantity.equals(other$quantity)) return false;
final java.lang.Object this$taxAmounts = this.getTaxAmounts();
final java.lang.Object other$taxAmounts = other.getTaxAmounts();
if (this$taxAmounts == null ? other$taxAmounts != null : !this$taxAmounts.equals(other$taxAmounts)) return false;
final java.lang.Object this$taxRates = this.getTaxRates();
final java.lang.Object other$taxRates = other.getTaxRates();
if (this$taxRates == null ? other$taxRates != null : !this$taxRates.equals(other$taxRates)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final java.lang.Object this$unitAmount = this.getUnitAmount();
final java.lang.Object other$unitAmount = other.getUnitAmount();
if (this$unitAmount == null ? other$unitAmount != null : !this$unitAmount.equals(other$unitAmount)) return false;
final java.lang.Object this$unitAmountDecimal = this.getUnitAmountDecimal();
final java.lang.Object other$unitAmountDecimal = other.getUnitAmountDecimal();
if (this$unitAmountDecimal == null ? other$unitAmountDecimal != null : !this$unitAmountDecimal.equals(other$unitAmountDecimal)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof CreditNoteLineItem;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $discountAmount = this.getDiscountAmount();
result = result * PRIME + ($discountAmount == null ? 43 : $discountAmount.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $invoiceLineItem = this.getInvoiceLineItem();
result = result * PRIME + ($invoiceLineItem == null ? 43 : $invoiceLineItem.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $quantity = this.getQuantity();
result = result * PRIME + ($quantity == null ? 43 : $quantity.hashCode());
final java.lang.Object $taxAmounts = this.getTaxAmounts();
result = result * PRIME + ($taxAmounts == null ? 43 : $taxAmounts.hashCode());
final java.lang.Object $taxRates = this.getTaxRates();
result = result * PRIME + ($taxRates == null ? 43 : $taxRates.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final java.lang.Object $unitAmount = this.getUnitAmount();
result = result * PRIME + ($unitAmount == null ? 43 : $unitAmount.hashCode());
final java.lang.Object $unitAmountDecimal = this.getUnitAmountDecimal();
result = result * PRIME + ($unitAmountDecimal == null ? 43 : $unitAmountDecimal.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
}