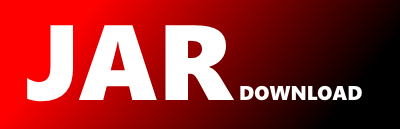
com.stripe.model.Mandate Maven / Gradle / Ivy
// Generated by delombok at Wed Jun 10 17:29:56 PDT 2020
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.MandateRetrieveParams;
import java.util.Map;
public class Mandate extends ApiResource implements HasId {
@SerializedName("customer_acceptance")
CustomerAcceptance customerAcceptance;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
@SerializedName("multi_use")
MultiUse multiUse;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code mandate}.
*/
@SerializedName("object")
String object;
/**
* ID of the payment method associated with this mandate.
*/
@SerializedName("payment_method")
ExpandableField paymentMethod;
@SerializedName("payment_method_details")
PaymentMethodDetails paymentMethodDetails;
@SerializedName("single_use")
SingleUse singleUse;
/**
* The status of the mandate, which indicates whether it can be used to initiate a payment.
*
* One of {@code active}, {@code inactive}, or {@code pending}.
*/
@SerializedName("status")
String status;
/**
* The type of the mandate.
*
*
One of {@code multi_use}, or {@code single_use}.
*/
@SerializedName("type")
String type;
/**
* Get ID of expandable {@code paymentMethod} object.
*/
public String getPaymentMethod() {
return (this.paymentMethod != null) ? this.paymentMethod.getId() : null;
}
public void setPaymentMethod(String id) {
this.paymentMethod = ApiResource.setExpandableFieldId(id, this.paymentMethod);
}
/**
* Get expanded {@code paymentMethod}.
*/
public PaymentMethod getPaymentMethodObject() {
return (this.paymentMethod != null) ? this.paymentMethod.getExpanded() : null;
}
public void setPaymentMethodObject(PaymentMethod expandableObject) {
this.paymentMethod = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Retrieves a Mandate object.
*/
public static Mandate retrieve(String mandate) throws StripeException {
return retrieve(mandate, (Map) null, (RequestOptions) null);
}
/**
* Retrieves a Mandate object.
*/
public static Mandate retrieve(String mandate, RequestOptions options) throws StripeException {
return retrieve(mandate, (Map) null, options);
}
/**
* Retrieves a Mandate object.
*/
public static Mandate retrieve(String mandate, Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/mandates/%s", ApiResource.urlEncodeId(mandate)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Mandate.class, options);
}
/**
* Retrieves a Mandate object.
*/
public static Mandate retrieve(String mandate, MandateRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/mandates/%s", ApiResource.urlEncodeId(mandate)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Mandate.class, options);
}
public static class AuBecsDebit extends StripeObject {
/**
* The URL of the mandate. This URL generally contains sensitive information about the customer
* and should be shared with them exclusively.
*/
@SerializedName("url")
String url;
/**
* The URL of the mandate. This URL generally contains sensitive information about the customer
* and should be shared with them exclusively.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUrl() {
return this.url;
}
/**
* The URL of the mandate. This URL generally contains sensitive information about the customer
* and should be shared with them exclusively.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUrl(final String url) {
this.url = url;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Mandate.AuBecsDebit)) return false;
final Mandate.AuBecsDebit other = (Mandate.AuBecsDebit) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$url = this.getUrl();
final java.lang.Object other$url = other.getUrl();
if (this$url == null ? other$url != null : !this$url.equals(other$url)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Mandate.AuBecsDebit;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $url = this.getUrl();
result = result * PRIME + ($url == null ? 43 : $url.hashCode());
return result;
}
}
public static class BacsDebit extends StripeObject {
/**
* The status of the mandate on the Bacs network. Can be one of {@code pending}, {@code
* revoked}, {@code refused}, or {@code accepted}.
*/
@SerializedName("network_status")
String networkStatus;
/**
* The unique reference identifying the mandate on the Bacs network.
*/
@SerializedName("reference")
String reference;
/**
* The URL that will contain the mandate that the customer has signed.
*/
@SerializedName("url")
String url;
/**
* The status of the mandate on the Bacs network. Can be one of {@code pending}, {@code
* revoked}, {@code refused}, or {@code accepted}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getNetworkStatus() {
return this.networkStatus;
}
/**
* The unique reference identifying the mandate on the Bacs network.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReference() {
return this.reference;
}
/**
* The URL that will contain the mandate that the customer has signed.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUrl() {
return this.url;
}
/**
* The status of the mandate on the Bacs network. Can be one of {@code pending}, {@code
* revoked}, {@code refused}, or {@code accepted}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setNetworkStatus(final String networkStatus) {
this.networkStatus = networkStatus;
}
/**
* The unique reference identifying the mandate on the Bacs network.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReference(final String reference) {
this.reference = reference;
}
/**
* The URL that will contain the mandate that the customer has signed.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUrl(final String url) {
this.url = url;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Mandate.BacsDebit)) return false;
final Mandate.BacsDebit other = (Mandate.BacsDebit) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$networkStatus = this.getNetworkStatus();
final java.lang.Object other$networkStatus = other.getNetworkStatus();
if (this$networkStatus == null ? other$networkStatus != null : !this$networkStatus.equals(other$networkStatus)) return false;
final java.lang.Object this$reference = this.getReference();
final java.lang.Object other$reference = other.getReference();
if (this$reference == null ? other$reference != null : !this$reference.equals(other$reference)) return false;
final java.lang.Object this$url = this.getUrl();
final java.lang.Object other$url = other.getUrl();
if (this$url == null ? other$url != null : !this$url.equals(other$url)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Mandate.BacsDebit;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $networkStatus = this.getNetworkStatus();
result = result * PRIME + ($networkStatus == null ? 43 : $networkStatus.hashCode());
final java.lang.Object $reference = this.getReference();
result = result * PRIME + ($reference == null ? 43 : $reference.hashCode());
final java.lang.Object $url = this.getUrl();
result = result * PRIME + ($url == null ? 43 : $url.hashCode());
return result;
}
}
public static class CustomerAcceptance extends StripeObject {
/**
* The time at which the customer accepted the Mandate.
*/
@SerializedName("accepted_at")
Long acceptedAt;
@SerializedName("offline")
Offline offline;
@SerializedName("online")
Online online;
/**
* The type of customer acceptance information included with the Mandate. One of {@code online}
* or {@code offline}.
*/
@SerializedName("type")
String type;
public static class Offline extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Mandate.CustomerAcceptance.Offline)) return false;
final Mandate.CustomerAcceptance.Offline other = (Mandate.CustomerAcceptance.Offline) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Mandate.CustomerAcceptance.Offline;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class Online extends StripeObject {
/** The IP address from which the Mandate was accepted by the customer. */
@SerializedName("ip_address")
String ipAddress;
@SerializedName("user_agent")
String userAgent;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getIpAddress() {
return this.ipAddress;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUserAgent() {
return this.userAgent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIpAddress(final String ipAddress) {
this.ipAddress = ipAddress;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUserAgent(final String userAgent) {
this.userAgent = userAgent;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Mandate.CustomerAcceptance.Online)) return false;
final Mandate.CustomerAcceptance.Online other = (Mandate.CustomerAcceptance.Online) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$ipAddress = this.getIpAddress();
final java.lang.Object other$ipAddress = other.getIpAddress();
if (this$ipAddress == null ? other$ipAddress != null : !this$ipAddress.equals(other$ipAddress)) return false;
final java.lang.Object this$userAgent = this.getUserAgent();
final java.lang.Object other$userAgent = other.getUserAgent();
if (this$userAgent == null ? other$userAgent != null : !this$userAgent.equals(other$userAgent)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Mandate.CustomerAcceptance.Online;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $ipAddress = this.getIpAddress();
result = result * PRIME + ($ipAddress == null ? 43 : $ipAddress.hashCode());
final java.lang.Object $userAgent = this.getUserAgent();
result = result * PRIME + ($userAgent == null ? 43 : $userAgent.hashCode());
return result;
}
}
/**
* The time at which the customer accepted the Mandate.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAcceptedAt() {
return this.acceptedAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Offline getOffline() {
return this.offline;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Online getOnline() {
return this.online;
}
/**
* The type of customer acceptance information included with the Mandate. One of {@code online}
* or {@code offline}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
/**
* The time at which the customer accepted the Mandate.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAcceptedAt(final Long acceptedAt) {
this.acceptedAt = acceptedAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setOffline(final Offline offline) {
this.offline = offline;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setOnline(final Online online) {
this.online = online;
}
/**
* The type of customer acceptance information included with the Mandate. One of {@code online}
* or {@code offline}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Mandate.CustomerAcceptance)) return false;
final Mandate.CustomerAcceptance other = (Mandate.CustomerAcceptance) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$acceptedAt = this.getAcceptedAt();
final java.lang.Object other$acceptedAt = other.getAcceptedAt();
if (this$acceptedAt == null ? other$acceptedAt != null : !this$acceptedAt.equals(other$acceptedAt)) return false;
final java.lang.Object this$offline = this.getOffline();
final java.lang.Object other$offline = other.getOffline();
if (this$offline == null ? other$offline != null : !this$offline.equals(other$offline)) return false;
final java.lang.Object this$online = this.getOnline();
final java.lang.Object other$online = other.getOnline();
if (this$online == null ? other$online != null : !this$online.equals(other$online)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Mandate.CustomerAcceptance;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $acceptedAt = this.getAcceptedAt();
result = result * PRIME + ($acceptedAt == null ? 43 : $acceptedAt.hashCode());
final java.lang.Object $offline = this.getOffline();
result = result * PRIME + ($offline == null ? 43 : $offline.hashCode());
final java.lang.Object $online = this.getOnline();
result = result * PRIME + ($online == null ? 43 : $online.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
return result;
}
}
/**
* The user agent of the browser from which the Mandate was accepted by the customer.
*/
public static class MultiUse extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Mandate.MultiUse)) return false;
final Mandate.MultiUse other = (Mandate.MultiUse) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Mandate.MultiUse;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class PaymentMethodDetails extends StripeObject {
@SerializedName("au_becs_debit")
AuBecsDebit auBecsDebit;
@SerializedName("bacs_debit")
BacsDebit bacsDebit;
@SerializedName("card")
Card card;
@SerializedName("sepa_debit")
SepaDebit sepaDebit;
/**
* The type of the payment method associated with this mandate. An additional hash is included
* on {@code payment_method_details} with a name matching this value. It contains mandate
* information specific to the payment method.
*/
@SerializedName("type")
String type;
public static class Card extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Mandate.PaymentMethodDetails.Card)) return false;
final Mandate.PaymentMethodDetails.Card other = (Mandate.PaymentMethodDetails.Card) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Mandate.PaymentMethodDetails.Card;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class SepaDebit extends StripeObject {
/** The unique reference of the mandate. */
@SerializedName("reference")
String reference;
@SerializedName("url")
String url;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReference() {
return this.reference;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUrl() {
return this.url;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReference(final String reference) {
this.reference = reference;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUrl(final String url) {
this.url = url;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Mandate.PaymentMethodDetails.SepaDebit)) return false;
final Mandate.PaymentMethodDetails.SepaDebit other = (Mandate.PaymentMethodDetails.SepaDebit) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$reference = this.getReference();
final java.lang.Object other$reference = other.getReference();
if (this$reference == null ? other$reference != null : !this$reference.equals(other$reference)) return false;
final java.lang.Object this$url = this.getUrl();
final java.lang.Object other$url = other.getUrl();
if (this$url == null ? other$url != null : !this$url.equals(other$url)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Mandate.PaymentMethodDetails.SepaDebit;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $reference = this.getReference();
result = result * PRIME + ($reference == null ? 43 : $reference.hashCode());
final java.lang.Object $url = this.getUrl();
result = result * PRIME + ($url == null ? 43 : $url.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public AuBecsDebit getAuBecsDebit() {
return this.auBecsDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BacsDebit getBacsDebit() {
return this.bacsDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Card getCard() {
return this.card;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SepaDebit getSepaDebit() {
return this.sepaDebit;
}
/**
* The type of the payment method associated with this mandate. An additional hash is included
* on {@code payment_method_details} with a name matching this value. It contains mandate
* information specific to the payment method.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAuBecsDebit(final AuBecsDebit auBecsDebit) {
this.auBecsDebit = auBecsDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBacsDebit(final BacsDebit bacsDebit) {
this.bacsDebit = bacsDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCard(final Card card) {
this.card = card;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSepaDebit(final SepaDebit sepaDebit) {
this.sepaDebit = sepaDebit;
}
/**
* The type of the payment method associated with this mandate. An additional hash is included
* on {@code payment_method_details} with a name matching this value. It contains mandate
* information specific to the payment method.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Mandate.PaymentMethodDetails)) return false;
final Mandate.PaymentMethodDetails other = (Mandate.PaymentMethodDetails) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$auBecsDebit = this.getAuBecsDebit();
final java.lang.Object other$auBecsDebit = other.getAuBecsDebit();
if (this$auBecsDebit == null ? other$auBecsDebit != null : !this$auBecsDebit.equals(other$auBecsDebit)) return false;
final java.lang.Object this$bacsDebit = this.getBacsDebit();
final java.lang.Object other$bacsDebit = other.getBacsDebit();
if (this$bacsDebit == null ? other$bacsDebit != null : !this$bacsDebit.equals(other$bacsDebit)) return false;
final java.lang.Object this$card = this.getCard();
final java.lang.Object other$card = other.getCard();
if (this$card == null ? other$card != null : !this$card.equals(other$card)) return false;
final java.lang.Object this$sepaDebit = this.getSepaDebit();
final java.lang.Object other$sepaDebit = other.getSepaDebit();
if (this$sepaDebit == null ? other$sepaDebit != null : !this$sepaDebit.equals(other$sepaDebit)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Mandate.PaymentMethodDetails;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $auBecsDebit = this.getAuBecsDebit();
result = result * PRIME + ($auBecsDebit == null ? 43 : $auBecsDebit.hashCode());
final java.lang.Object $bacsDebit = this.getBacsDebit();
result = result * PRIME + ($bacsDebit == null ? 43 : $bacsDebit.hashCode());
final java.lang.Object $card = this.getCard();
result = result * PRIME + ($card == null ? 43 : $card.hashCode());
final java.lang.Object $sepaDebit = this.getSepaDebit();
result = result * PRIME + ($sepaDebit == null ? 43 : $sepaDebit.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
return result;
}
}
/**
* The URL of the mandate. This URL generally contains sensitive information about the
* customer and should be shared with them exclusively.
*/
public static class SingleUse extends StripeObject {
/** On a single use mandate, the amount of the payment. */
@SerializedName("amount")
Long amount;
/** On a single use mandate, the currency of the payment. */
@SerializedName("currency")
String currency;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmount(final Long amount) {
this.amount = amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Mandate.SingleUse)) return false;
final Mandate.SingleUse other = (Mandate.SingleUse) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Mandate.SingleUse;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public CustomerAcceptance getCustomerAcceptance() {
return this.customerAcceptance;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public MultiUse getMultiUse() {
return this.multiUse;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code mandate}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PaymentMethodDetails getPaymentMethodDetails() {
return this.paymentMethodDetails;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SingleUse getSingleUse() {
return this.singleUse;
}
/**
* The status of the mandate, which indicates whether it can be used to initiate a payment.
*
*
One of {@code active}, {@code inactive}, or {@code pending}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
/**
* The type of the mandate.
*
*
One of {@code multi_use}, or {@code single_use}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCustomerAcceptance(final CustomerAcceptance customerAcceptance) {
this.customerAcceptance = customerAcceptance;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMultiUse(final MultiUse multiUse) {
this.multiUse = multiUse;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code mandate}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPaymentMethodDetails(final PaymentMethodDetails paymentMethodDetails) {
this.paymentMethodDetails = paymentMethodDetails;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSingleUse(final SingleUse singleUse) {
this.singleUse = singleUse;
}
/**
* The status of the mandate, which indicates whether it can be used to initiate a payment.
*
*
One of {@code active}, {@code inactive}, or {@code pending}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
/**
* The type of the mandate.
*
*
One of {@code multi_use}, or {@code single_use}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Mandate)) return false;
final Mandate other = (Mandate) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$customerAcceptance = this.getCustomerAcceptance();
final java.lang.Object other$customerAcceptance = other.getCustomerAcceptance();
if (this$customerAcceptance == null ? other$customerAcceptance != null : !this$customerAcceptance.equals(other$customerAcceptance)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$multiUse = this.getMultiUse();
final java.lang.Object other$multiUse = other.getMultiUse();
if (this$multiUse == null ? other$multiUse != null : !this$multiUse.equals(other$multiUse)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$paymentMethod = this.getPaymentMethod();
final java.lang.Object other$paymentMethod = other.getPaymentMethod();
if (this$paymentMethod == null ? other$paymentMethod != null : !this$paymentMethod.equals(other$paymentMethod)) return false;
final java.lang.Object this$paymentMethodDetails = this.getPaymentMethodDetails();
final java.lang.Object other$paymentMethodDetails = other.getPaymentMethodDetails();
if (this$paymentMethodDetails == null ? other$paymentMethodDetails != null : !this$paymentMethodDetails.equals(other$paymentMethodDetails)) return false;
final java.lang.Object this$singleUse = this.getSingleUse();
final java.lang.Object other$singleUse = other.getSingleUse();
if (this$singleUse == null ? other$singleUse != null : !this$singleUse.equals(other$singleUse)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Mandate;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $customerAcceptance = this.getCustomerAcceptance();
result = result * PRIME + ($customerAcceptance == null ? 43 : $customerAcceptance.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $multiUse = this.getMultiUse();
result = result * PRIME + ($multiUse == null ? 43 : $multiUse.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $paymentMethod = this.getPaymentMethod();
result = result * PRIME + ($paymentMethod == null ? 43 : $paymentMethod.hashCode());
final java.lang.Object $paymentMethodDetails = this.getPaymentMethodDetails();
result = result * PRIME + ($paymentMethodDetails == null ? 43 : $paymentMethodDetails.hashCode());
final java.lang.Object $singleUse = this.getSingleUse();
result = result * PRIME + ($singleUse == null ? 43 : $singleUse.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
}