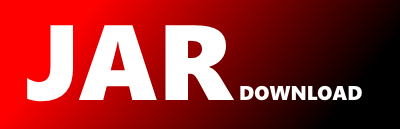
com.stripe.model.SubscriptionSchedule Maven / Gradle / Ivy
// Generated by delombok at Wed Jun 10 17:29:56 PDT 2020
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.SubscriptionScheduleCancelParams;
import com.stripe.param.SubscriptionScheduleCreateParams;
import com.stripe.param.SubscriptionScheduleListParams;
import com.stripe.param.SubscriptionScheduleReleaseParams;
import com.stripe.param.SubscriptionScheduleRetrieveParams;
import com.stripe.param.SubscriptionScheduleUpdateParams;
import java.math.BigDecimal;
import java.util.List;
import java.util.Map;
public class SubscriptionSchedule extends ApiResource implements HasId, MetadataStore {
/**
* Time at which the subscription schedule was canceled. Measured in seconds since the Unix epoch.
*/
@SerializedName("canceled_at")
Long canceledAt;
/**
* Time at which the subscription schedule was completed. Measured in seconds since the Unix
* epoch.
*/
@SerializedName("completed_at")
Long completedAt;
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* Object representing the start and end dates for the current phase of the subscription schedule,
* if it is {@code active}.
*/
@SerializedName("current_phase")
CurrentPhase currentPhase;
/**
* ID of the customer who owns the subscription schedule.
*/
@SerializedName("customer")
ExpandableField customer;
@SerializedName("default_settings")
DefaultSettings defaultSettings;
/**
* Behavior of the subscription schedule and underlying subscription when it ends.
*
* One of {@code cancel}, {@code none}, {@code release}, or {@code renew}.
*/
@SerializedName("end_behavior")
String endBehavior;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code subscription_schedule}.
*/
@SerializedName("object")
String object;
/**
* Configuration for the subscription schedule's phases.
*/
@SerializedName("phases")
List phases;
/**
* Time at which the subscription schedule was released. Measured in seconds since the Unix epoch.
*/
@SerializedName("released_at")
Long releasedAt;
/**
* ID of the subscription once managed by the subscription schedule (if it is released).
*/
@SerializedName("released_subscription")
String releasedSubscription;
/**
* The present status of the subscription schedule. Possible values are {@code not_started},
* {@code active}, {@code completed}, {@code released}, and {@code canceled}. You can read more
* about the different states in our behavior guide.
*
* One of {@code active}, {@code canceled}, {@code completed}, {@code not_started}, or {@code
* released}.
*/
@SerializedName("status")
String status;
/**
* ID of the subscription managed by the subscription schedule.
*/
@SerializedName("subscription")
ExpandableField subscription;
/**
* Get ID of expandable {@code customer} object.
*/
public String getCustomer() {
return (this.customer != null) ? this.customer.getId() : null;
}
public void setCustomer(String id) {
this.customer = ApiResource.setExpandableFieldId(id, this.customer);
}
/**
* Get expanded {@code customer}.
*/
public Customer getCustomerObject() {
return (this.customer != null) ? this.customer.getExpanded() : null;
}
public void setCustomerObject(Customer expandableObject) {
this.customer = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code subscription} object.
*/
public String getSubscription() {
return (this.subscription != null) ? this.subscription.getId() : null;
}
public void setSubscription(String id) {
this.subscription = ApiResource.setExpandableFieldId(id, this.subscription);
}
/**
* Get expanded {@code subscription}.
*/
public Subscription getSubscriptionObject() {
return (this.subscription != null) ? this.subscription.getExpanded() : null;
}
public void setSubscriptionObject(Subscription expandableObject) {
this.subscription = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Retrieves the list of your subscription schedules.
*/
public static SubscriptionScheduleCollection list(Map params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Retrieves the list of your subscription schedules.
*/
public static SubscriptionScheduleCollection list(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/subscription_schedules");
return ApiResource.requestCollection(url, params, SubscriptionScheduleCollection.class, options);
}
/**
* Retrieves the list of your subscription schedules.
*/
public static SubscriptionScheduleCollection list(SubscriptionScheduleListParams params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Retrieves the list of your subscription schedules.
*/
public static SubscriptionScheduleCollection list(SubscriptionScheduleListParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/subscription_schedules");
return ApiResource.requestCollection(url, params, SubscriptionScheduleCollection.class, options);
}
/**
* Creates a new subscription schedule object. Each customer can have up to 25 active or scheduled
* subscriptions.
*/
public static SubscriptionSchedule create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Creates a new subscription schedule object. Each customer can have up to 25 active or scheduled
* subscriptions.
*/
public static SubscriptionSchedule create(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/subscription_schedules");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, SubscriptionSchedule.class, options);
}
/**
* Creates a new subscription schedule object. Each customer can have up to 25 active or scheduled
* subscriptions.
*/
public static SubscriptionSchedule create(SubscriptionScheduleCreateParams params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Creates a new subscription schedule object. Each customer can have up to 25 active or scheduled
* subscriptions.
*/
public static SubscriptionSchedule create(SubscriptionScheduleCreateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/subscription_schedules");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, SubscriptionSchedule.class, options);
}
/**
* Retrieves the details of an existing subscription schedule. You only need to supply the unique
* subscription schedule identifier that was returned upon subscription schedule creation.
*/
public static SubscriptionSchedule retrieve(String schedule) throws StripeException {
return retrieve(schedule, (Map) null, (RequestOptions) null);
}
/**
* Retrieves the details of an existing subscription schedule. You only need to supply the unique
* subscription schedule identifier that was returned upon subscription schedule creation.
*/
public static SubscriptionSchedule retrieve(String schedule, RequestOptions options) throws StripeException {
return retrieve(schedule, (Map) null, options);
}
/**
* Retrieves the details of an existing subscription schedule. You only need to supply the unique
* subscription schedule identifier that was returned upon subscription schedule creation.
*/
public static SubscriptionSchedule retrieve(String schedule, Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/subscription_schedules/%s", ApiResource.urlEncodeId(schedule)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, SubscriptionSchedule.class, options);
}
/**
* Retrieves the details of an existing subscription schedule. You only need to supply the unique
* subscription schedule identifier that was returned upon subscription schedule creation.
*/
public static SubscriptionSchedule retrieve(String schedule, SubscriptionScheduleRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/subscription_schedules/%s", ApiResource.urlEncodeId(schedule)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, SubscriptionSchedule.class, options);
}
/**
* Updates an existing subscription schedule.
*/
@Override
public SubscriptionSchedule update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates an existing subscription schedule.
*/
@Override
public SubscriptionSchedule update(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/subscription_schedules/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, SubscriptionSchedule.class, options);
}
/**
* Updates an existing subscription schedule.
*/
public SubscriptionSchedule update(SubscriptionScheduleUpdateParams params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates an existing subscription schedule.
*/
public SubscriptionSchedule update(SubscriptionScheduleUpdateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/subscription_schedules/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, SubscriptionSchedule.class, options);
}
/**
* Cancels a subscription schedule and its associated subscription immediately (if the
* subscription schedule has an active subscription). A subscription schedule can only be canceled
* if its status is not_started
or active
.
*/
public SubscriptionSchedule cancel() throws StripeException {
return cancel((Map) null, (RequestOptions) null);
}
/**
* Cancels a subscription schedule and its associated subscription immediately (if the
* subscription schedule has an active subscription). A subscription schedule can only be canceled
* if its status is not_started
or active
.
*/
public SubscriptionSchedule cancel(RequestOptions options) throws StripeException {
return cancel((Map) null, options);
}
/**
* Cancels a subscription schedule and its associated subscription immediately (if the
* subscription schedule has an active subscription). A subscription schedule can only be canceled
* if its status is not_started
or active
.
*/
public SubscriptionSchedule cancel(Map params) throws StripeException {
return cancel(params, (RequestOptions) null);
}
/**
* Cancels a subscription schedule and its associated subscription immediately (if the
* subscription schedule has an active subscription). A subscription schedule can only be canceled
* if its status is not_started
or active
.
*/
public SubscriptionSchedule cancel(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/subscription_schedules/%s/cancel", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, SubscriptionSchedule.class, options);
}
/**
* Cancels a subscription schedule and its associated subscription immediately (if the
* subscription schedule has an active subscription). A subscription schedule can only be canceled
* if its status is not_started
or active
.
*/
public SubscriptionSchedule cancel(SubscriptionScheduleCancelParams params) throws StripeException {
return cancel(params, (RequestOptions) null);
}
/**
* Cancels a subscription schedule and its associated subscription immediately (if the
* subscription schedule has an active subscription). A subscription schedule can only be canceled
* if its status is not_started
or active
.
*/
public SubscriptionSchedule cancel(SubscriptionScheduleCancelParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/subscription_schedules/%s/cancel", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, SubscriptionSchedule.class, options);
}
/**
* Releases the subscription schedule immediately, which will stop scheduling of its phases, but
* leave any existing subscription in place. A schedule can only be released if its status is
* not_started
or active
. If the subscription schedule is currently
* associated with a subscription, releasing it will remove its subscription
property
* and set the subscription’s ID to the released_subscription
property.
*/
public SubscriptionSchedule release() throws StripeException {
return release((Map) null, (RequestOptions) null);
}
/**
* Releases the subscription schedule immediately, which will stop scheduling of its phases, but
* leave any existing subscription in place. A schedule can only be released if its status is
* not_started
or active
. If the subscription schedule is currently
* associated with a subscription, releasing it will remove its subscription
property
* and set the subscription’s ID to the released_subscription
property.
*/
public SubscriptionSchedule release(RequestOptions options) throws StripeException {
return release((Map) null, options);
}
/**
* Releases the subscription schedule immediately, which will stop scheduling of its phases, but
* leave any existing subscription in place. A schedule can only be released if its status is
* not_started
or active
. If the subscription schedule is currently
* associated with a subscription, releasing it will remove its subscription
property
* and set the subscription’s ID to the released_subscription
property.
*/
public SubscriptionSchedule release(Map params) throws StripeException {
return release(params, (RequestOptions) null);
}
/**
* Releases the subscription schedule immediately, which will stop scheduling of its phases, but
* leave any existing subscription in place. A schedule can only be released if its status is
* not_started
or active
. If the subscription schedule is currently
* associated with a subscription, releasing it will remove its subscription
property
* and set the subscription’s ID to the released_subscription
property.
*/
public SubscriptionSchedule release(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/subscription_schedules/%s/release", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, SubscriptionSchedule.class, options);
}
/**
* Releases the subscription schedule immediately, which will stop scheduling of its phases, but
* leave any existing subscription in place. A schedule can only be released if its status is
* not_started
or active
. If the subscription schedule is currently
* associated with a subscription, releasing it will remove its subscription
property
* and set the subscription’s ID to the released_subscription
property.
*/
public SubscriptionSchedule release(SubscriptionScheduleReleaseParams params) throws StripeException {
return release(params, (RequestOptions) null);
}
/**
* Releases the subscription schedule immediately, which will stop scheduling of its phases, but
* leave any existing subscription in place. A schedule can only be released if its status is
* not_started
or active
. If the subscription schedule is currently
* associated with a subscription, releasing it will remove its subscription
property
* and set the subscription’s ID to the released_subscription
property.
*/
public SubscriptionSchedule release(SubscriptionScheduleReleaseParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/subscription_schedules/%s/release", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, SubscriptionSchedule.class, options);
}
public static class AddInvoiceItem extends StripeObject {
/**
* ID of the price used to generate the invoice item.
*/
@SerializedName("price")
ExpandableField price;
/**
* The quantity of the invoice item.
*/
@SerializedName("quantity")
Long quantity;
/**
* Get ID of expandable {@code price} object.
*/
public String getPrice() {
return (this.price != null) ? this.price.getId() : null;
}
public void setPrice(String id) {
this.price = ApiResource.setExpandableFieldId(id, this.price);
}
/**
* Get expanded {@code price}.
*/
public Price getPriceObject() {
return (this.price != null) ? this.price.getExpanded() : null;
}
public void setPriceObject(Price expandableObject) {
this.price = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* The quantity of the invoice item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getQuantity() {
return this.quantity;
}
/**
* The quantity of the invoice item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setQuantity(final Long quantity) {
this.quantity = quantity;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SubscriptionSchedule.AddInvoiceItem)) return false;
final SubscriptionSchedule.AddInvoiceItem other = (SubscriptionSchedule.AddInvoiceItem) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$price = this.getPrice();
final java.lang.Object other$price = other.getPrice();
if (this$price == null ? other$price != null : !this$price.equals(other$price)) return false;
final java.lang.Object this$quantity = this.getQuantity();
final java.lang.Object other$quantity = other.getQuantity();
if (this$quantity == null ? other$quantity != null : !this$quantity.equals(other$quantity)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SubscriptionSchedule.AddInvoiceItem;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $price = this.getPrice();
result = result * PRIME + ($price == null ? 43 : $price.hashCode());
final java.lang.Object $quantity = this.getQuantity();
result = result * PRIME + ($quantity == null ? 43 : $quantity.hashCode());
return result;
}
}
public static class CurrentPhase extends StripeObject {
/**
* The end of this phase of the subscription schedule.
*/
@SerializedName("end_date")
Long endDate;
/**
* The start of this phase of the subscription schedule.
*/
@SerializedName("start_date")
Long startDate;
/**
* The end of this phase of the subscription schedule.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getEndDate() {
return this.endDate;
}
/**
* The start of this phase of the subscription schedule.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getStartDate() {
return this.startDate;
}
/**
* The end of this phase of the subscription schedule.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEndDate(final Long endDate) {
this.endDate = endDate;
}
/**
* The start of this phase of the subscription schedule.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStartDate(final Long startDate) {
this.startDate = startDate;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SubscriptionSchedule.CurrentPhase)) return false;
final SubscriptionSchedule.CurrentPhase other = (SubscriptionSchedule.CurrentPhase) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$endDate = this.getEndDate();
final java.lang.Object other$endDate = other.getEndDate();
if (this$endDate == null ? other$endDate != null : !this$endDate.equals(other$endDate)) return false;
final java.lang.Object this$startDate = this.getStartDate();
final java.lang.Object other$startDate = other.getStartDate();
if (this$startDate == null ? other$startDate != null : !this$startDate.equals(other$startDate)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SubscriptionSchedule.CurrentPhase;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $endDate = this.getEndDate();
result = result * PRIME + ($endDate == null ? 43 : $endDate.hashCode());
final java.lang.Object $startDate = this.getStartDate();
result = result * PRIME + ($startDate == null ? 43 : $startDate.hashCode());
return result;
}
}
public static class DefaultSettings extends StripeObject {
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period.
*/
@SerializedName("billing_thresholds")
Subscription.BillingThresholds billingThresholds;
/**
* Either {@code charge_automatically}, or {@code send_invoice}. When charging automatically,
* Stripe will attempt to pay the underlying subscription at the end of each billing cycle using
* the default source attached to the customer. When sending an invoice, Stripe will email your
* customer an invoice with payment instructions.
*
* One of {@code charge_automatically}, or {@code send_invoice}.
*/
@SerializedName("collection_method")
String collectionMethod;
/**
* ID of the default payment method for the subscription schedule. If not set, invoices will use
* the default payment method in the customer's invoice settings.
*/
@SerializedName("default_payment_method")
ExpandableField defaultPaymentMethod;
/**
* The subscription schedule's default invoice settings.
*/
@SerializedName("invoice_settings")
InvoiceSettings invoiceSettings;
/**
* The account (if any) the subscription's payments will be attributed to for tax reporting, and
* where funds from each payment will be transferred to for each of the subscription's invoices.
*/
@SerializedName("transfer_data")
Subscription.TransferData transferData;
/**
* Get ID of expandable {@code defaultPaymentMethod} object.
*/
public String getDefaultPaymentMethod() {
return (this.defaultPaymentMethod != null) ? this.defaultPaymentMethod.getId() : null;
}
public void setDefaultPaymentMethod(String id) {
this.defaultPaymentMethod = ApiResource.setExpandableFieldId(id, this.defaultPaymentMethod);
}
/**
* Get expanded {@code defaultPaymentMethod}.
*/
public PaymentMethod getDefaultPaymentMethodObject() {
return (this.defaultPaymentMethod != null) ? this.defaultPaymentMethod.getExpanded() : null;
}
public void setDefaultPaymentMethodObject(PaymentMethod expandableObject) {
this.defaultPaymentMethod = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Subscription.BillingThresholds getBillingThresholds() {
return this.billingThresholds;
}
/**
* Either {@code charge_automatically}, or {@code send_invoice}. When charging automatically,
* Stripe will attempt to pay the underlying subscription at the end of each billing cycle using
* the default source attached to the customer. When sending an invoice, Stripe will email your
* customer an invoice with payment instructions.
*
* One of {@code charge_automatically}, or {@code send_invoice}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCollectionMethod() {
return this.collectionMethod;
}
/**
* The subscription schedule's default invoice settings.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public InvoiceSettings getInvoiceSettings() {
return this.invoiceSettings;
}
/**
* The account (if any) the subscription's payments will be attributed to for tax reporting, and
* where funds from each payment will be transferred to for each of the subscription's invoices.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Subscription.TransferData getTransferData() {
return this.transferData;
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBillingThresholds(final Subscription.BillingThresholds billingThresholds) {
this.billingThresholds = billingThresholds;
}
/**
* Either {@code charge_automatically}, or {@code send_invoice}. When charging automatically,
* Stripe will attempt to pay the underlying subscription at the end of each billing cycle using
* the default source attached to the customer. When sending an invoice, Stripe will email your
* customer an invoice with payment instructions.
*
*
One of {@code charge_automatically}, or {@code send_invoice}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCollectionMethod(final String collectionMethod) {
this.collectionMethod = collectionMethod;
}
/**
* The subscription schedule's default invoice settings.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setInvoiceSettings(final InvoiceSettings invoiceSettings) {
this.invoiceSettings = invoiceSettings;
}
/**
* The account (if any) the subscription's payments will be attributed to for tax reporting, and
* where funds from each payment will be transferred to for each of the subscription's invoices.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTransferData(final Subscription.TransferData transferData) {
this.transferData = transferData;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SubscriptionSchedule.DefaultSettings)) return false;
final SubscriptionSchedule.DefaultSettings other = (SubscriptionSchedule.DefaultSettings) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$billingThresholds = this.getBillingThresholds();
final java.lang.Object other$billingThresholds = other.getBillingThresholds();
if (this$billingThresholds == null ? other$billingThresholds != null : !this$billingThresholds.equals(other$billingThresholds)) return false;
final java.lang.Object this$collectionMethod = this.getCollectionMethod();
final java.lang.Object other$collectionMethod = other.getCollectionMethod();
if (this$collectionMethod == null ? other$collectionMethod != null : !this$collectionMethod.equals(other$collectionMethod)) return false;
final java.lang.Object this$defaultPaymentMethod = this.getDefaultPaymentMethod();
final java.lang.Object other$defaultPaymentMethod = other.getDefaultPaymentMethod();
if (this$defaultPaymentMethod == null ? other$defaultPaymentMethod != null : !this$defaultPaymentMethod.equals(other$defaultPaymentMethod)) return false;
final java.lang.Object this$invoiceSettings = this.getInvoiceSettings();
final java.lang.Object other$invoiceSettings = other.getInvoiceSettings();
if (this$invoiceSettings == null ? other$invoiceSettings != null : !this$invoiceSettings.equals(other$invoiceSettings)) return false;
final java.lang.Object this$transferData = this.getTransferData();
final java.lang.Object other$transferData = other.getTransferData();
if (this$transferData == null ? other$transferData != null : !this$transferData.equals(other$transferData)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SubscriptionSchedule.DefaultSettings;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $billingThresholds = this.getBillingThresholds();
result = result * PRIME + ($billingThresholds == null ? 43 : $billingThresholds.hashCode());
final java.lang.Object $collectionMethod = this.getCollectionMethod();
result = result * PRIME + ($collectionMethod == null ? 43 : $collectionMethod.hashCode());
final java.lang.Object $defaultPaymentMethod = this.getDefaultPaymentMethod();
result = result * PRIME + ($defaultPaymentMethod == null ? 43 : $defaultPaymentMethod.hashCode());
final java.lang.Object $invoiceSettings = this.getInvoiceSettings();
result = result * PRIME + ($invoiceSettings == null ? 43 : $invoiceSettings.hashCode());
final java.lang.Object $transferData = this.getTransferData();
result = result * PRIME + ($transferData == null ? 43 : $transferData.hashCode());
return result;
}
}
public static class InvoiceSettings extends StripeObject {
/**
* Number of days within which a customer must pay invoices generated by this subscription
* schedule. This value will be {@code null} for subscription schedules where {@code
* billing=charge_automatically}.
*/
@SerializedName("days_until_due")
Long daysUntilDue;
/**
* Number of days within which a customer must pay invoices generated by this subscription
* schedule. This value will be {@code null} for subscription schedules where {@code
* billing=charge_automatically}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDaysUntilDue() {
return this.daysUntilDue;
}
/**
* Number of days within which a customer must pay invoices generated by this subscription
* schedule. This value will be {@code null} for subscription schedules where {@code
* billing=charge_automatically}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDaysUntilDue(final Long daysUntilDue) {
this.daysUntilDue = daysUntilDue;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SubscriptionSchedule.InvoiceSettings)) return false;
final SubscriptionSchedule.InvoiceSettings other = (SubscriptionSchedule.InvoiceSettings) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$daysUntilDue = this.getDaysUntilDue();
final java.lang.Object other$daysUntilDue = other.getDaysUntilDue();
if (this$daysUntilDue == null ? other$daysUntilDue != null : !this$daysUntilDue.equals(other$daysUntilDue)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SubscriptionSchedule.InvoiceSettings;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $daysUntilDue = this.getDaysUntilDue();
result = result * PRIME + ($daysUntilDue == null ? 43 : $daysUntilDue.hashCode());
return result;
}
}
public static class Phase extends StripeObject {
/**
* A list of prices and quantities that will generate invoice items appended to the first
* invoice for this phase.
*/
@SerializedName("add_invoice_items")
List addInvoiceItems;
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents
* the percentage of the subscription invoice subtotal that will be transferred to the
* application owner's Stripe account during this phase of the schedule.
*/
@SerializedName("application_fee_percent")
BigDecimal applicationFeePercent;
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period.
*/
@SerializedName("billing_thresholds")
Subscription.BillingThresholds billingThresholds;
/**
* Either {@code charge_automatically}, or {@code send_invoice}. When charging automatically,
* Stripe will attempt to pay the underlying subscription at the end of each billing cycle using
* the default source attached to the customer. When sending an invoice, Stripe will email your
* customer an invoice with payment instructions.
*
* One of {@code charge_automatically}, or {@code send_invoice}.
*/
@SerializedName("collection_method")
String collectionMethod;
/**
* ID of the coupon to use during this phase of the subscription schedule.
*/
@SerializedName("coupon")
ExpandableField coupon;
/**
* ID of the default payment method for the subscription schedule. It must belong to the
* customer associated with the subscription schedule. If not set, invoices will use the default
* payment method in the customer's invoice settings.
*/
@SerializedName("default_payment_method")
ExpandableField defaultPaymentMethod;
/**
* The default tax rates to apply to the subscription during this phase of the subscription
* schedule.
*/
@SerializedName("default_tax_rates")
List defaultTaxRates;
/**
* The end of this phase of the subscription schedule.
*/
@SerializedName("end_date")
Long endDate;
/**
* The subscription schedule's default invoice settings.
*/
@SerializedName("invoice_settings")
InvoiceSettings invoiceSettings;
/**
* Plans to subscribe during this phase of the subscription schedule.
*/
@SerializedName("plans")
List plans;
/**
* Controls whether or not the subscription schedule will prorate when transitioning to this
* phase. Values are {@code create_prorations} and {@code none}.
*
* One of {@code always_invoice}, {@code create_prorations}, or {@code none}.
*/
@SerializedName("proration_behavior")
String prorationBehavior;
/**
* The start of this phase of the subscription schedule.
*/
@SerializedName("start_date")
Long startDate;
/**
* If provided, each invoice created during this phase of the subscription schedule will apply
* the tax rate, increasing the amount billed to the customer.
*/
@SerializedName("tax_percent")
BigDecimal taxPercent;
/**
* The account (if any) the subscription's payments will be attributed to for tax reporting, and
* where funds from each payment will be transferred to for each of the subscription's invoices.
*/
@SerializedName("transfer_data")
Subscription.TransferData transferData;
/**
* When the trial ends within the phase.
*/
@SerializedName("trial_end")
Long trialEnd;
/**
* Get ID of expandable {@code coupon} object.
*/
public String getCoupon() {
return (this.coupon != null) ? this.coupon.getId() : null;
}
public void setCoupon(String id) {
this.coupon = ApiResource.setExpandableFieldId(id, this.coupon);
}
/**
* Get expanded {@code coupon}.
*/
public Coupon getCouponObject() {
return (this.coupon != null) ? this.coupon.getExpanded() : null;
}
public void setCouponObject(Coupon expandableObject) {
this.coupon = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code defaultPaymentMethod} object.
*/
public String getDefaultPaymentMethod() {
return (this.defaultPaymentMethod != null) ? this.defaultPaymentMethod.getId() : null;
}
public void setDefaultPaymentMethod(String id) {
this.defaultPaymentMethod = ApiResource.setExpandableFieldId(id, this.defaultPaymentMethod);
}
/**
* Get expanded {@code defaultPaymentMethod}.
*/
public PaymentMethod getDefaultPaymentMethodObject() {
return (this.defaultPaymentMethod != null) ? this.defaultPaymentMethod.getExpanded() : null;
}
public void setDefaultPaymentMethodObject(PaymentMethod expandableObject) {
this.defaultPaymentMethod = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* A list of prices and quantities that will generate invoice items appended to the first
* invoice for this phase.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getAddInvoiceItems() {
return this.addInvoiceItems;
}
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents
* the percentage of the subscription invoice subtotal that will be transferred to the
* application owner's Stripe account during this phase of the schedule.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BigDecimal getApplicationFeePercent() {
return this.applicationFeePercent;
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Subscription.BillingThresholds getBillingThresholds() {
return this.billingThresholds;
}
/**
* Either {@code charge_automatically}, or {@code send_invoice}. When charging automatically,
* Stripe will attempt to pay the underlying subscription at the end of each billing cycle using
* the default source attached to the customer. When sending an invoice, Stripe will email your
* customer an invoice with payment instructions.
*
* One of {@code charge_automatically}, or {@code send_invoice}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCollectionMethod() {
return this.collectionMethod;
}
/**
* The default tax rates to apply to the subscription during this phase of the subscription
* schedule.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getDefaultTaxRates() {
return this.defaultTaxRates;
}
/**
* The end of this phase of the subscription schedule.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getEndDate() {
return this.endDate;
}
/**
* The subscription schedule's default invoice settings.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public InvoiceSettings getInvoiceSettings() {
return this.invoiceSettings;
}
/**
* Plans to subscribe during this phase of the subscription schedule.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getPlans() {
return this.plans;
}
/**
* Controls whether or not the subscription schedule will prorate when transitioning to this
* phase. Values are {@code create_prorations} and {@code none}.
*
* One of {@code always_invoice}, {@code create_prorations}, or {@code none}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getProrationBehavior() {
return this.prorationBehavior;
}
/**
* The start of this phase of the subscription schedule.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getStartDate() {
return this.startDate;
}
/**
* If provided, each invoice created during this phase of the subscription schedule will apply
* the tax rate, increasing the amount billed to the customer.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BigDecimal getTaxPercent() {
return this.taxPercent;
}
/**
* The account (if any) the subscription's payments will be attributed to for tax reporting, and
* where funds from each payment will be transferred to for each of the subscription's invoices.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Subscription.TransferData getTransferData() {
return this.transferData;
}
/**
* When the trial ends within the phase.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getTrialEnd() {
return this.trialEnd;
}
/**
* A list of prices and quantities that will generate invoice items appended to the first
* invoice for this phase.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddInvoiceItems(final List addInvoiceItems) {
this.addInvoiceItems = addInvoiceItems;
}
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents
* the percentage of the subscription invoice subtotal that will be transferred to the
* application owner's Stripe account during this phase of the schedule.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setApplicationFeePercent(final BigDecimal applicationFeePercent) {
this.applicationFeePercent = applicationFeePercent;
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBillingThresholds(final Subscription.BillingThresholds billingThresholds) {
this.billingThresholds = billingThresholds;
}
/**
* Either {@code charge_automatically}, or {@code send_invoice}. When charging automatically,
* Stripe will attempt to pay the underlying subscription at the end of each billing cycle using
* the default source attached to the customer. When sending an invoice, Stripe will email your
* customer an invoice with payment instructions.
*
* One of {@code charge_automatically}, or {@code send_invoice}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCollectionMethod(final String collectionMethod) {
this.collectionMethod = collectionMethod;
}
/**
* The default tax rates to apply to the subscription during this phase of the subscription
* schedule.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDefaultTaxRates(final List defaultTaxRates) {
this.defaultTaxRates = defaultTaxRates;
}
/**
* The end of this phase of the subscription schedule.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEndDate(final Long endDate) {
this.endDate = endDate;
}
/**
* The subscription schedule's default invoice settings.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setInvoiceSettings(final InvoiceSettings invoiceSettings) {
this.invoiceSettings = invoiceSettings;
}
/**
* Plans to subscribe during this phase of the subscription schedule.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPlans(final List plans) {
this.plans = plans;
}
/**
* Controls whether or not the subscription schedule will prorate when transitioning to this
* phase. Values are {@code create_prorations} and {@code none}.
*
* One of {@code always_invoice}, {@code create_prorations}, or {@code none}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setProrationBehavior(final String prorationBehavior) {
this.prorationBehavior = prorationBehavior;
}
/**
* The start of this phase of the subscription schedule.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStartDate(final Long startDate) {
this.startDate = startDate;
}
/**
* If provided, each invoice created during this phase of the subscription schedule will apply
* the tax rate, increasing the amount billed to the customer.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxPercent(final BigDecimal taxPercent) {
this.taxPercent = taxPercent;
}
/**
* The account (if any) the subscription's payments will be attributed to for tax reporting, and
* where funds from each payment will be transferred to for each of the subscription's invoices.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTransferData(final Subscription.TransferData transferData) {
this.transferData = transferData;
}
/**
* When the trial ends within the phase.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTrialEnd(final Long trialEnd) {
this.trialEnd = trialEnd;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SubscriptionSchedule.Phase)) return false;
final SubscriptionSchedule.Phase other = (SubscriptionSchedule.Phase) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$addInvoiceItems = this.getAddInvoiceItems();
final java.lang.Object other$addInvoiceItems = other.getAddInvoiceItems();
if (this$addInvoiceItems == null ? other$addInvoiceItems != null : !this$addInvoiceItems.equals(other$addInvoiceItems)) return false;
final java.lang.Object this$applicationFeePercent = this.getApplicationFeePercent();
final java.lang.Object other$applicationFeePercent = other.getApplicationFeePercent();
if (this$applicationFeePercent == null ? other$applicationFeePercent != null : !this$applicationFeePercent.equals(other$applicationFeePercent)) return false;
final java.lang.Object this$billingThresholds = this.getBillingThresholds();
final java.lang.Object other$billingThresholds = other.getBillingThresholds();
if (this$billingThresholds == null ? other$billingThresholds != null : !this$billingThresholds.equals(other$billingThresholds)) return false;
final java.lang.Object this$collectionMethod = this.getCollectionMethod();
final java.lang.Object other$collectionMethod = other.getCollectionMethod();
if (this$collectionMethod == null ? other$collectionMethod != null : !this$collectionMethod.equals(other$collectionMethod)) return false;
final java.lang.Object this$coupon = this.getCoupon();
final java.lang.Object other$coupon = other.getCoupon();
if (this$coupon == null ? other$coupon != null : !this$coupon.equals(other$coupon)) return false;
final java.lang.Object this$defaultPaymentMethod = this.getDefaultPaymentMethod();
final java.lang.Object other$defaultPaymentMethod = other.getDefaultPaymentMethod();
if (this$defaultPaymentMethod == null ? other$defaultPaymentMethod != null : !this$defaultPaymentMethod.equals(other$defaultPaymentMethod)) return false;
final java.lang.Object this$defaultTaxRates = this.getDefaultTaxRates();
final java.lang.Object other$defaultTaxRates = other.getDefaultTaxRates();
if (this$defaultTaxRates == null ? other$defaultTaxRates != null : !this$defaultTaxRates.equals(other$defaultTaxRates)) return false;
final java.lang.Object this$endDate = this.getEndDate();
final java.lang.Object other$endDate = other.getEndDate();
if (this$endDate == null ? other$endDate != null : !this$endDate.equals(other$endDate)) return false;
final java.lang.Object this$invoiceSettings = this.getInvoiceSettings();
final java.lang.Object other$invoiceSettings = other.getInvoiceSettings();
if (this$invoiceSettings == null ? other$invoiceSettings != null : !this$invoiceSettings.equals(other$invoiceSettings)) return false;
final java.lang.Object this$plans = this.getPlans();
final java.lang.Object other$plans = other.getPlans();
if (this$plans == null ? other$plans != null : !this$plans.equals(other$plans)) return false;
final java.lang.Object this$prorationBehavior = this.getProrationBehavior();
final java.lang.Object other$prorationBehavior = other.getProrationBehavior();
if (this$prorationBehavior == null ? other$prorationBehavior != null : !this$prorationBehavior.equals(other$prorationBehavior)) return false;
final java.lang.Object this$startDate = this.getStartDate();
final java.lang.Object other$startDate = other.getStartDate();
if (this$startDate == null ? other$startDate != null : !this$startDate.equals(other$startDate)) return false;
final java.lang.Object this$taxPercent = this.getTaxPercent();
final java.lang.Object other$taxPercent = other.getTaxPercent();
if (this$taxPercent == null ? other$taxPercent != null : !this$taxPercent.equals(other$taxPercent)) return false;
final java.lang.Object this$transferData = this.getTransferData();
final java.lang.Object other$transferData = other.getTransferData();
if (this$transferData == null ? other$transferData != null : !this$transferData.equals(other$transferData)) return false;
final java.lang.Object this$trialEnd = this.getTrialEnd();
final java.lang.Object other$trialEnd = other.getTrialEnd();
if (this$trialEnd == null ? other$trialEnd != null : !this$trialEnd.equals(other$trialEnd)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SubscriptionSchedule.Phase;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $addInvoiceItems = this.getAddInvoiceItems();
result = result * PRIME + ($addInvoiceItems == null ? 43 : $addInvoiceItems.hashCode());
final java.lang.Object $applicationFeePercent = this.getApplicationFeePercent();
result = result * PRIME + ($applicationFeePercent == null ? 43 : $applicationFeePercent.hashCode());
final java.lang.Object $billingThresholds = this.getBillingThresholds();
result = result * PRIME + ($billingThresholds == null ? 43 : $billingThresholds.hashCode());
final java.lang.Object $collectionMethod = this.getCollectionMethod();
result = result * PRIME + ($collectionMethod == null ? 43 : $collectionMethod.hashCode());
final java.lang.Object $coupon = this.getCoupon();
result = result * PRIME + ($coupon == null ? 43 : $coupon.hashCode());
final java.lang.Object $defaultPaymentMethod = this.getDefaultPaymentMethod();
result = result * PRIME + ($defaultPaymentMethod == null ? 43 : $defaultPaymentMethod.hashCode());
final java.lang.Object $defaultTaxRates = this.getDefaultTaxRates();
result = result * PRIME + ($defaultTaxRates == null ? 43 : $defaultTaxRates.hashCode());
final java.lang.Object $endDate = this.getEndDate();
result = result * PRIME + ($endDate == null ? 43 : $endDate.hashCode());
final java.lang.Object $invoiceSettings = this.getInvoiceSettings();
result = result * PRIME + ($invoiceSettings == null ? 43 : $invoiceSettings.hashCode());
final java.lang.Object $plans = this.getPlans();
result = result * PRIME + ($plans == null ? 43 : $plans.hashCode());
final java.lang.Object $prorationBehavior = this.getProrationBehavior();
result = result * PRIME + ($prorationBehavior == null ? 43 : $prorationBehavior.hashCode());
final java.lang.Object $startDate = this.getStartDate();
result = result * PRIME + ($startDate == null ? 43 : $startDate.hashCode());
final java.lang.Object $taxPercent = this.getTaxPercent();
result = result * PRIME + ($taxPercent == null ? 43 : $taxPercent.hashCode());
final java.lang.Object $transferData = this.getTransferData();
result = result * PRIME + ($transferData == null ? 43 : $transferData.hashCode());
final java.lang.Object $trialEnd = this.getTrialEnd();
result = result * PRIME + ($trialEnd == null ? 43 : $trialEnd.hashCode());
return result;
}
}
public static class PhaseItem extends StripeObject {
/**
* Define thresholds at which an invoice will be sent, and the related subscription advanced to
* a new billing period.
*/
@SerializedName("billing_thresholds")
SubscriptionItem.BillingThresholds billingThresholds;
/** ID of the plan to which the customer should be subscribed. */
@SerializedName("plan")
ExpandableField plan;
/** ID of the price to which the customer should be subscribed. */
@SerializedName("price")
ExpandableField price;
/** Quantity of the plan to which the customer should be subscribed. */
@SerializedName("quantity")
Long quantity;
/**
* The tax rates which apply to this {@code phase_item}. When set, the {@code default_tax_rates}
* on the phase do not apply to this {@code phase_item}.
*/
@SerializedName("tax_rates")
List taxRates;
/** Get ID of expandable {@code plan} object. */
public String getPlan() {
return (this.plan != null) ? this.plan.getId() : null;
}
public void setPlan(String id) {
this.plan = ApiResource.setExpandableFieldId(id, this.plan);
}
/** Get expanded {@code plan}. */
public Plan getPlanObject() {
return (this.plan != null) ? this.plan.getExpanded() : null;
}
public void setPlanObject(Plan expandableObject) {
this.plan = new ExpandableField(expandableObject.getId(), expandableObject);
}
/** Get ID of expandable {@code price} object. */
public String getPrice() {
return (this.price != null) ? this.price.getId() : null;
}
public void setPrice(String id) {
this.price = ApiResource.setExpandableFieldId(id, this.price);
}
/** Get expanded {@code price}. */
public Price getPriceObject() {
return (this.price != null) ? this.price.getExpanded() : null;
}
public void setPriceObject(Price expandableObject) {
this.price = new ExpandableField(expandableObject.getId(), expandableObject);
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SubscriptionItem.BillingThresholds getBillingThresholds() {
return this.billingThresholds;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getQuantity() {
return this.quantity;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getTaxRates() {
return this.taxRates;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBillingThresholds(final SubscriptionItem.BillingThresholds billingThresholds) {
this.billingThresholds = billingThresholds;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setQuantity(final Long quantity) {
this.quantity = quantity;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxRates(final List taxRates) {
this.taxRates = taxRates;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SubscriptionSchedule.PhaseItem)) return false;
final SubscriptionSchedule.PhaseItem other = (SubscriptionSchedule.PhaseItem) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$billingThresholds = this.getBillingThresholds();
final java.lang.Object other$billingThresholds = other.getBillingThresholds();
if (this$billingThresholds == null ? other$billingThresholds != null : !this$billingThresholds.equals(other$billingThresholds)) return false;
final java.lang.Object this$plan = this.getPlan();
final java.lang.Object other$plan = other.getPlan();
if (this$plan == null ? other$plan != null : !this$plan.equals(other$plan)) return false;
final java.lang.Object this$price = this.getPrice();
final java.lang.Object other$price = other.getPrice();
if (this$price == null ? other$price != null : !this$price.equals(other$price)) return false;
final java.lang.Object this$quantity = this.getQuantity();
final java.lang.Object other$quantity = other.getQuantity();
if (this$quantity == null ? other$quantity != null : !this$quantity.equals(other$quantity)) return false;
final java.lang.Object this$taxRates = this.getTaxRates();
final java.lang.Object other$taxRates = other.getTaxRates();
if (this$taxRates == null ? other$taxRates != null : !this$taxRates.equals(other$taxRates)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SubscriptionSchedule.PhaseItem;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $billingThresholds = this.getBillingThresholds();
result = result * PRIME + ($billingThresholds == null ? 43 : $billingThresholds.hashCode());
final java.lang.Object $plan = this.getPlan();
result = result * PRIME + ($plan == null ? 43 : $plan.hashCode());
final java.lang.Object $price = this.getPrice();
result = result * PRIME + ($price == null ? 43 : $price.hashCode());
final java.lang.Object $quantity = this.getQuantity();
result = result * PRIME + ($quantity == null ? 43 : $quantity.hashCode());
final java.lang.Object $taxRates = this.getTaxRates();
result = result * PRIME + ($taxRates == null ? 43 : $taxRates.hashCode());
return result;
}
}
/**
* Time at which the subscription schedule was canceled. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCanceledAt() {
return this.canceledAt;
}
/**
* Time at which the subscription schedule was completed. Measured in seconds since the Unix
* epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCompletedAt() {
return this.completedAt;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
/**
* Object representing the start and end dates for the current phase of the subscription schedule,
* if it is {@code active}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public CurrentPhase getCurrentPhase() {
return this.currentPhase;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public DefaultSettings getDefaultSettings() {
return this.defaultSettings;
}
/**
* Behavior of the subscription schedule and underlying subscription when it ends.
*
* One of {@code cancel}, {@code none}, {@code release}, or {@code renew}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getEndBehavior() {
return this.endBehavior;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code subscription_schedule}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* Configuration for the subscription schedule's phases.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getPhases() {
return this.phases;
}
/**
* Time at which the subscription schedule was released. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getReleasedAt() {
return this.releasedAt;
}
/**
* ID of the subscription once managed by the subscription schedule (if it is released).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReleasedSubscription() {
return this.releasedSubscription;
}
/**
* The present status of the subscription schedule. Possible values are {@code not_started},
* {@code active}, {@code completed}, {@code released}, and {@code canceled}. You can read more
* about the different states in our behavior guide.
*
* One of {@code active}, {@code canceled}, {@code completed}, {@code not_started}, or {@code
* released}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
/**
* Time at which the subscription schedule was canceled. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCanceledAt(final Long canceledAt) {
this.canceledAt = canceledAt;
}
/**
* Time at which the subscription schedule was completed. Measured in seconds since the Unix
* epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCompletedAt(final Long completedAt) {
this.completedAt = completedAt;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* Object representing the start and end dates for the current phase of the subscription schedule,
* if it is {@code active}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrentPhase(final CurrentPhase currentPhase) {
this.currentPhase = currentPhase;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDefaultSettings(final DefaultSettings defaultSettings) {
this.defaultSettings = defaultSettings;
}
/**
* Behavior of the subscription schedule and underlying subscription when it ends.
*
*
One of {@code cancel}, {@code none}, {@code release}, or {@code renew}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEndBehavior(final String endBehavior) {
this.endBehavior = endBehavior;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code subscription_schedule}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* Configuration for the subscription schedule's phases.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPhases(final List phases) {
this.phases = phases;
}
/**
* Time at which the subscription schedule was released. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReleasedAt(final Long releasedAt) {
this.releasedAt = releasedAt;
}
/**
* ID of the subscription once managed by the subscription schedule (if it is released).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReleasedSubscription(final String releasedSubscription) {
this.releasedSubscription = releasedSubscription;
}
/**
* The present status of the subscription schedule. Possible values are {@code not_started},
* {@code active}, {@code completed}, {@code released}, and {@code canceled}. You can read more
* about the different states in our behavior guide.
*
* One of {@code active}, {@code canceled}, {@code completed}, {@code not_started}, or {@code
* released}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SubscriptionSchedule)) return false;
final SubscriptionSchedule other = (SubscriptionSchedule) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$canceledAt = this.getCanceledAt();
final java.lang.Object other$canceledAt = other.getCanceledAt();
if (this$canceledAt == null ? other$canceledAt != null : !this$canceledAt.equals(other$canceledAt)) return false;
final java.lang.Object this$completedAt = this.getCompletedAt();
final java.lang.Object other$completedAt = other.getCompletedAt();
if (this$completedAt == null ? other$completedAt != null : !this$completedAt.equals(other$completedAt)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$currentPhase = this.getCurrentPhase();
final java.lang.Object other$currentPhase = other.getCurrentPhase();
if (this$currentPhase == null ? other$currentPhase != null : !this$currentPhase.equals(other$currentPhase)) return false;
final java.lang.Object this$customer = this.getCustomer();
final java.lang.Object other$customer = other.getCustomer();
if (this$customer == null ? other$customer != null : !this$customer.equals(other$customer)) return false;
final java.lang.Object this$defaultSettings = this.getDefaultSettings();
final java.lang.Object other$defaultSettings = other.getDefaultSettings();
if (this$defaultSettings == null ? other$defaultSettings != null : !this$defaultSettings.equals(other$defaultSettings)) return false;
final java.lang.Object this$endBehavior = this.getEndBehavior();
final java.lang.Object other$endBehavior = other.getEndBehavior();
if (this$endBehavior == null ? other$endBehavior != null : !this$endBehavior.equals(other$endBehavior)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$phases = this.getPhases();
final java.lang.Object other$phases = other.getPhases();
if (this$phases == null ? other$phases != null : !this$phases.equals(other$phases)) return false;
final java.lang.Object this$releasedAt = this.getReleasedAt();
final java.lang.Object other$releasedAt = other.getReleasedAt();
if (this$releasedAt == null ? other$releasedAt != null : !this$releasedAt.equals(other$releasedAt)) return false;
final java.lang.Object this$releasedSubscription = this.getReleasedSubscription();
final java.lang.Object other$releasedSubscription = other.getReleasedSubscription();
if (this$releasedSubscription == null ? other$releasedSubscription != null : !this$releasedSubscription.equals(other$releasedSubscription)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$subscription = this.getSubscription();
final java.lang.Object other$subscription = other.getSubscription();
if (this$subscription == null ? other$subscription != null : !this$subscription.equals(other$subscription)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SubscriptionSchedule;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $canceledAt = this.getCanceledAt();
result = result * PRIME + ($canceledAt == null ? 43 : $canceledAt.hashCode());
final java.lang.Object $completedAt = this.getCompletedAt();
result = result * PRIME + ($completedAt == null ? 43 : $completedAt.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $currentPhase = this.getCurrentPhase();
result = result * PRIME + ($currentPhase == null ? 43 : $currentPhase.hashCode());
final java.lang.Object $customer = this.getCustomer();
result = result * PRIME + ($customer == null ? 43 : $customer.hashCode());
final java.lang.Object $defaultSettings = this.getDefaultSettings();
result = result * PRIME + ($defaultSettings == null ? 43 : $defaultSettings.hashCode());
final java.lang.Object $endBehavior = this.getEndBehavior();
result = result * PRIME + ($endBehavior == null ? 43 : $endBehavior.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $phases = this.getPhases();
result = result * PRIME + ($phases == null ? 43 : $phases.hashCode());
final java.lang.Object $releasedAt = this.getReleasedAt();
result = result * PRIME + ($releasedAt == null ? 43 : $releasedAt.hashCode());
final java.lang.Object $releasedSubscription = this.getReleasedSubscription();
result = result * PRIME + ($releasedSubscription == null ? 43 : $releasedSubscription.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $subscription = this.getSubscription();
result = result * PRIME + ($subscription == null ? 43 : $subscription.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
}