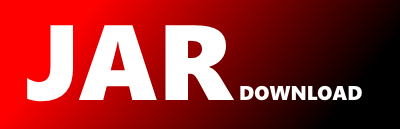
com.stripe.model.TaxId Maven / Gradle / Ivy
// Generated by delombok at Wed Jun 10 17:29:56 PDT 2020
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import java.util.Map;
public class TaxId extends ApiResource implements HasId {
/**
* Two-letter ISO code representing the country of the tax ID.
*/
@SerializedName("country")
String country;
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* ID of the customer.
*/
@SerializedName("customer")
ExpandableField customer;
/**
* Always true for a deleted object.
*/
@SerializedName("deleted")
Boolean deleted;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code tax_id}.
*/
@SerializedName("object")
String object;
/**
* Type of the tax ID, one of {@code ae_trn}, {@code au_abn}, {@code br_cnpj}, {@code br_cpf},
* {@code ca_bn}, {@code ca_qst}, {@code ch_vat}, {@code cl_tin}, {@code es_cif}, {@code eu_vat},
* {@code hk_br}, {@code id_npwp}, {@code in_gst}, {@code jp_cn}, {@code kr_brn}, {@code li_uid},
* {@code mx_rfc}, {@code my_frp}, {@code my_itn}, {@code my_sst}, {@code no_vat}, {@code nz_gst},
* {@code ru_inn}, {@code sa_vat}, {@code sg_gst}, {@code sg_uen}, {@code th_vat}, {@code tw_vat},
* {@code us_ein}, or {@code za_vat}. Note that some legacy tax IDs have type {@code unknown}
*/
@SerializedName("type")
String type;
/**
* Value of the tax ID.
*/
@SerializedName("value")
String value;
@SerializedName("verification")
Verification verification;
/**
* Get ID of expandable {@code customer} object.
*/
public String getCustomer() {
return (this.customer != null) ? this.customer.getId() : null;
}
public void setCustomer(String id) {
this.customer = ApiResource.setExpandableFieldId(id, this.customer);
}
/**
* Get expanded {@code customer}.
*/
public Customer getCustomerObject() {
return (this.customer != null) ? this.customer.getExpanded() : null;
}
public void setCustomerObject(Customer expandableObject) {
this.customer = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Deletes an existing TaxID
object.
*/
public TaxId delete() throws StripeException {
return delete((Map) null, (RequestOptions) null);
}
/**
* Deletes an existing TaxID
object.
*/
public TaxId delete(RequestOptions options) throws StripeException {
return delete((Map) null, options);
}
/**
* Deletes an existing TaxID
object.
*/
public TaxId delete(Map params) throws StripeException {
return delete(params, (RequestOptions) null);
}
/**
* Deletes an existing TaxID
object.
*/
public TaxId delete(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/customers/%s/tax_ids/%s", ApiResource.urlEncodeId(this.getCustomer()), ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.DELETE, url, params, TaxId.class, options);
}
public static class Verification extends StripeObject {
/**
* Verification status, one of {@code pending}, {@code verified}, {@code unverified}, or {@code
* unavailable}.
*/
@SerializedName("status")
String status;
/** Verified address. */
@SerializedName("verified_address")
String verifiedAddress;
/** Verified name. */
@SerializedName("verified_name")
String verifiedName;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getVerifiedAddress() {
return this.verifiedAddress;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getVerifiedName() {
return this.verifiedName;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVerifiedAddress(final String verifiedAddress) {
this.verifiedAddress = verifiedAddress;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVerifiedName(final String verifiedName) {
this.verifiedName = verifiedName;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof TaxId.Verification)) return false;
final TaxId.Verification other = (TaxId.Verification) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$verifiedAddress = this.getVerifiedAddress();
final java.lang.Object other$verifiedAddress = other.getVerifiedAddress();
if (this$verifiedAddress == null ? other$verifiedAddress != null : !this$verifiedAddress.equals(other$verifiedAddress)) return false;
final java.lang.Object this$verifiedName = this.getVerifiedName();
final java.lang.Object other$verifiedName = other.getVerifiedName();
if (this$verifiedName == null ? other$verifiedName != null : !this$verifiedName.equals(other$verifiedName)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof TaxId.Verification;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $verifiedAddress = this.getVerifiedAddress();
result = result * PRIME + ($verifiedAddress == null ? 43 : $verifiedAddress.hashCode());
final java.lang.Object $verifiedName = this.getVerifiedName();
result = result * PRIME + ($verifiedName == null ? 43 : $verifiedName.hashCode());
return result;
}
}
/**
* Two-letter ISO code representing the country of the tax ID.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCountry() {
return this.country;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
/**
* Always true for a deleted object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getDeleted() {
return this.deleted;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code tax_id}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* Type of the tax ID, one of {@code ae_trn}, {@code au_abn}, {@code br_cnpj}, {@code br_cpf},
* {@code ca_bn}, {@code ca_qst}, {@code ch_vat}, {@code cl_tin}, {@code es_cif}, {@code eu_vat},
* {@code hk_br}, {@code id_npwp}, {@code in_gst}, {@code jp_cn}, {@code kr_brn}, {@code li_uid},
* {@code mx_rfc}, {@code my_frp}, {@code my_itn}, {@code my_sst}, {@code no_vat}, {@code nz_gst},
* {@code ru_inn}, {@code sa_vat}, {@code sg_gst}, {@code sg_uen}, {@code th_vat}, {@code tw_vat},
* {@code us_ein}, or {@code za_vat}. Note that some legacy tax IDs have type {@code unknown}
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
/**
* Value of the tax ID.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Verification getVerification() {
return this.verification;
}
/**
* Two-letter ISO code representing the country of the tax ID.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCountry(final String country) {
this.country = country;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* Always true for a deleted object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDeleted(final Boolean deleted) {
this.deleted = deleted;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code tax_id}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* Type of the tax ID, one of {@code ae_trn}, {@code au_abn}, {@code br_cnpj}, {@code br_cpf},
* {@code ca_bn}, {@code ca_qst}, {@code ch_vat}, {@code cl_tin}, {@code es_cif}, {@code eu_vat},
* {@code hk_br}, {@code id_npwp}, {@code in_gst}, {@code jp_cn}, {@code kr_brn}, {@code li_uid},
* {@code mx_rfc}, {@code my_frp}, {@code my_itn}, {@code my_sst}, {@code no_vat}, {@code nz_gst},
* {@code ru_inn}, {@code sa_vat}, {@code sg_gst}, {@code sg_uen}, {@code th_vat}, {@code tw_vat},
* {@code us_ein}, or {@code za_vat}. Note that some legacy tax IDs have type {@code unknown}
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
/**
* Value of the tax ID.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setValue(final String value) {
this.value = value;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVerification(final Verification verification) {
this.verification = verification;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof TaxId)) return false;
final TaxId other = (TaxId) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$country = this.getCountry();
final java.lang.Object other$country = other.getCountry();
if (this$country == null ? other$country != null : !this$country.equals(other$country)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$customer = this.getCustomer();
final java.lang.Object other$customer = other.getCustomer();
if (this$customer == null ? other$customer != null : !this$customer.equals(other$customer)) return false;
final java.lang.Object this$deleted = this.getDeleted();
final java.lang.Object other$deleted = other.getDeleted();
if (this$deleted == null ? other$deleted != null : !this$deleted.equals(other$deleted)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final java.lang.Object this$value = this.getValue();
final java.lang.Object other$value = other.getValue();
if (this$value == null ? other$value != null : !this$value.equals(other$value)) return false;
final java.lang.Object this$verification = this.getVerification();
final java.lang.Object other$verification = other.getVerification();
if (this$verification == null ? other$verification != null : !this$verification.equals(other$verification)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof TaxId;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $country = this.getCountry();
result = result * PRIME + ($country == null ? 43 : $country.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $customer = this.getCustomer();
result = result * PRIME + ($customer == null ? 43 : $customer.hashCode());
final java.lang.Object $deleted = this.getDeleted();
result = result * PRIME + ($deleted == null ? 43 : $deleted.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final java.lang.Object $value = this.getValue();
result = result * PRIME + ($value == null ? 43 : $value.hashCode());
final java.lang.Object $verification = this.getVerification();
result = result * PRIME + ($verification == null ? 43 : $verification.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
}