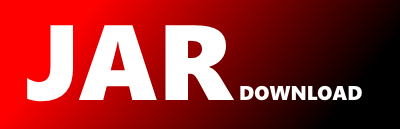
com.stripe.param.CustomerCreateParams Maven / Gradle / Ivy
// Generated by delombok at Wed Jun 10 17:29:56 PDT 2020
package com.stripe.param;
import com.google.gson.annotations.SerializedName;
import com.stripe.net.ApiRequestParams;
import com.stripe.net.ApiRequestParams.EnumParam;
import com.stripe.param.common.EmptyParam;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class CustomerCreateParams extends ApiRequestParams {
/**
* The customer's address.
*/
@SerializedName("address")
Object address;
/**
* An integer amount in %s that represents the customer's current balance, which affect the
* customer's future invoices. A negative amount represents a credit that decreases the amount due
* on an invoice; a positive amount increases the amount due on an invoice.
*/
@SerializedName("balance")
Long balance;
@SerializedName("coupon")
String coupon;
/**
* An arbitrary string that you can attach to a customer object. It is displayed alongside the
* customer in the dashboard.
*/
@SerializedName("description")
String description;
/**
* Customer's email address. It's displayed alongside the customer in your dashboard and can be
* useful for searching and tracking. This may be up to 512 characters.
*/
@SerializedName("email")
String email;
/**
* Specifies which fields in the response should be expanded.
*/
@SerializedName("expand")
List expand;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* The prefix for the customer used to generate unique invoice numbers. Must be 3–12 uppercase
* letters or numbers.
*/
@SerializedName("invoice_prefix")
String invoicePrefix;
/**
* Default invoice settings for this customer.
*/
@SerializedName("invoice_settings")
InvoiceSettings invoiceSettings;
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset by
* posting an empty value to them. All keys can be unset by posting an empty value to {@code
* metadata}.
*/
@SerializedName("metadata")
Object metadata;
/**
* The customer's full name or business name.
*/
@SerializedName("name")
String name;
/**
* The sequence to be used on the customer's next invoice. Defaults to 1.
*/
@SerializedName("next_invoice_sequence")
Long nextInvoiceSequence;
@SerializedName("payment_method")
String paymentMethod;
/**
* The customer's phone number.
*/
@SerializedName("phone")
String phone;
/**
* Customer's preferred languages, ordered by preference.
*/
@SerializedName("preferred_locales")
List preferredLocales;
/**
* The customer's shipping information. Appears on invoices emailed to this customer.
*/
@SerializedName("shipping")
Object shipping;
@SerializedName("source")
String source;
/**
* The customer's tax exemption. One of {@code none}, {@code exempt}, or {@code reverse}.
*/
@SerializedName("tax_exempt")
EnumParam taxExempt;
/**
* The customer's tax IDs.
*/
@SerializedName("tax_id_data")
List taxIdData;
private CustomerCreateParams(Object address, Long balance, String coupon, String description, String email, List expand, Map extraParams, String invoicePrefix, InvoiceSettings invoiceSettings, Object metadata, String name, Long nextInvoiceSequence, String paymentMethod, String phone, List preferredLocales, Object shipping, String source, EnumParam taxExempt, List taxIdData) {
this.address = address;
this.balance = balance;
this.coupon = coupon;
this.description = description;
this.email = email;
this.expand = expand;
this.extraParams = extraParams;
this.invoicePrefix = invoicePrefix;
this.invoiceSettings = invoiceSettings;
this.metadata = metadata;
this.name = name;
this.nextInvoiceSequence = nextInvoiceSequence;
this.paymentMethod = paymentMethod;
this.phone = phone;
this.preferredLocales = preferredLocales;
this.shipping = shipping;
this.source = source;
this.taxExempt = taxExempt;
this.taxIdData = taxIdData;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Object address;
private Long balance;
private String coupon;
private String description;
private String email;
private List expand;
private Map extraParams;
private String invoicePrefix;
private InvoiceSettings invoiceSettings;
private Object metadata;
private String name;
private Long nextInvoiceSequence;
private String paymentMethod;
private String phone;
private List preferredLocales;
private Object shipping;
private String source;
private EnumParam taxExempt;
private List taxIdData;
/**
* Finalize and obtain parameter instance from this builder.
*/
public CustomerCreateParams build() {
return new CustomerCreateParams(this.address, this.balance, this.coupon, this.description, this.email, this.expand, this.extraParams, this.invoicePrefix, this.invoiceSettings, this.metadata, this.name, this.nextInvoiceSequence, this.paymentMethod, this.phone, this.preferredLocales, this.shipping, this.source, this.taxExempt, this.taxIdData);
}
/**
* The customer's address.
*/
public Builder setAddress(Address address) {
this.address = address;
return this;
}
/**
* The customer's address.
*/
public Builder setAddress(EmptyParam address) {
this.address = address;
return this;
}
/**
* An integer amount in %s that represents the customer's current balance, which affect the
* customer's future invoices. A negative amount represents a credit that decreases the amount
* due on an invoice; a positive amount increases the amount due on an invoice.
*/
public Builder setBalance(Long balance) {
this.balance = balance;
return this;
}
public Builder setCoupon(String coupon) {
this.coupon = coupon;
return this;
}
/**
* An arbitrary string that you can attach to a customer object. It is displayed alongside the
* customer in the dashboard.
*/
public Builder setDescription(String description) {
this.description = description;
return this;
}
/**
* Customer's email address. It's displayed alongside the customer in your dashboard and can be
* useful for searching and tracking. This may be up to 512 characters.
*/
public Builder setEmail(String email) {
this.email = email;
return this;
}
/**
* Add an element to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* CustomerCreateParams#expand} for the field documentation.
*/
public Builder addExpand(String element) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.add(element);
return this;
}
/**
* Add all elements to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* CustomerCreateParams#expand} for the field documentation.
*/
public Builder addAllExpand(List elements) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.addAll(elements);
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* CustomerCreateParams#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link CustomerCreateParams#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* The prefix for the customer used to generate unique invoice numbers. Must be 3–12 uppercase
* letters or numbers.
*/
public Builder setInvoicePrefix(String invoicePrefix) {
this.invoicePrefix = invoicePrefix;
return this;
}
/**
* Default invoice settings for this customer.
*/
public Builder setInvoiceSettings(InvoiceSettings invoiceSettings) {
this.invoiceSettings = invoiceSettings;
return this;
}
/**
* Add a key/value pair to `metadata` map. A map is initialized for the first `put/putAll` call,
* and subsequent calls add additional key/value pairs to the original map. See {@link
* CustomerCreateParams#metadata} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder putMetadata(String key, String value) {
if (this.metadata == null || this.metadata instanceof EmptyParam) {
this.metadata = new HashMap();
}
((Map) this.metadata).put(key, value);
return this;
}
/**
* Add all map key/value pairs to `metadata` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link CustomerCreateParams#metadata} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder putAllMetadata(Map map) {
if (this.metadata == null || this.metadata instanceof EmptyParam) {
this.metadata = new HashMap();
}
((Map) this.metadata).putAll(map);
return this;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset
* by posting an empty value to them. All keys can be unset by posting an empty value to {@code
* metadata}.
*/
public Builder setMetadata(EmptyParam metadata) {
this.metadata = metadata;
return this;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset
* by posting an empty value to them. All keys can be unset by posting an empty value to {@code
* metadata}.
*/
public Builder setMetadata(Map metadata) {
this.metadata = metadata;
return this;
}
/**
* The customer's full name or business name.
*/
public Builder setName(String name) {
this.name = name;
return this;
}
/**
* The sequence to be used on the customer's next invoice. Defaults to 1.
*/
public Builder setNextInvoiceSequence(Long nextInvoiceSequence) {
this.nextInvoiceSequence = nextInvoiceSequence;
return this;
}
public Builder setPaymentMethod(String paymentMethod) {
this.paymentMethod = paymentMethod;
return this;
}
/**
* The customer's phone number.
*/
public Builder setPhone(String phone) {
this.phone = phone;
return this;
}
/**
* Add an element to `preferredLocales` list. A list is initialized for the first `add/addAll`
* call, and subsequent calls adds additional elements to the original list. See {@link
* CustomerCreateParams#preferredLocales} for the field documentation.
*/
public Builder addPreferredLocale(String element) {
if (this.preferredLocales == null) {
this.preferredLocales = new ArrayList<>();
}
this.preferredLocales.add(element);
return this;
}
/**
* Add all elements to `preferredLocales` list. A list is initialized for the first `add/addAll`
* call, and subsequent calls adds additional elements to the original list. See {@link
* CustomerCreateParams#preferredLocales} for the field documentation.
*/
public Builder addAllPreferredLocale(List elements) {
if (this.preferredLocales == null) {
this.preferredLocales = new ArrayList<>();
}
this.preferredLocales.addAll(elements);
return this;
}
/**
* The customer's shipping information. Appears on invoices emailed to this customer.
*/
public Builder setShipping(Shipping shipping) {
this.shipping = shipping;
return this;
}
/**
* The customer's shipping information. Appears on invoices emailed to this customer.
*/
public Builder setShipping(EmptyParam shipping) {
this.shipping = shipping;
return this;
}
public Builder setSource(String source) {
this.source = source;
return this;
}
/**
* The customer's tax exemption. One of {@code none}, {@code exempt}, or {@code reverse}.
*/
public Builder setTaxExempt(TaxExempt taxExempt) {
this.taxExempt = taxExempt;
return this;
}
/**
* The customer's tax exemption. One of {@code none}, {@code exempt}, or {@code reverse}.
*/
public Builder setTaxExempt(EmptyParam taxExempt) {
this.taxExempt = taxExempt;
return this;
}
/**
* Add an element to `taxIdData` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* CustomerCreateParams#taxIdData} for the field documentation.
*/
public Builder addTaxIdData(TaxIdData element) {
if (this.taxIdData == null) {
this.taxIdData = new ArrayList<>();
}
this.taxIdData.add(element);
return this;
}
/**
* Add all elements to `taxIdData` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* CustomerCreateParams#taxIdData} for the field documentation.
*/
public Builder addAllTaxIdData(List elements) {
if (this.taxIdData == null) {
this.taxIdData = new ArrayList<>();
}
this.taxIdData.addAll(elements);
return this;
}
}
public static class Address {
/**
* City, district, suburb, town, or village.
*/
@SerializedName("city")
String city;
/**
* Two-letter country code (ISO
* 3166-1 alpha-2).
*/
@SerializedName("country")
String country;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Address line 1 (e.g., street, PO Box, or company name).
*/
@SerializedName("line1")
String line1;
/**
* Address line 2 (e.g., apartment, suite, unit, or building).
*/
@SerializedName("line2")
String line2;
/**
* ZIP or postal code.
*/
@SerializedName("postal_code")
String postalCode;
/**
* State, county, province, or region.
*/
@SerializedName("state")
String state;
private Address(String city, String country, Map extraParams, String line1, String line2, String postalCode, String state) {
this.city = city;
this.country = country;
this.extraParams = extraParams;
this.line1 = line1;
this.line2 = line2;
this.postalCode = postalCode;
this.state = state;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private String city;
private String country;
private Map extraParams;
private String line1;
private String line2;
private String postalCode;
private String state;
/** Finalize and obtain parameter instance from this builder. */
public Address build() {
return new Address(this.city, this.country, this.extraParams, this.line1, this.line2, this.postalCode, this.state);
}
/** City, district, suburb, town, or village. */
public Builder setCity(String city) {
this.city = city;
return this;
}
/**
* Two-letter country code (ISO
* 3166-1 alpha-2).
*/
public Builder setCountry(String country) {
this.country = country;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* CustomerCreateParams.Address#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link CustomerCreateParams.Address#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/** Address line 1 (e.g., street, PO Box, or company name). */
public Builder setLine1(String line1) {
this.line1 = line1;
return this;
}
/** Address line 2 (e.g., apartment, suite, unit, or building). */
public Builder setLine2(String line2) {
this.line2 = line2;
return this;
}
/** ZIP or postal code. */
public Builder setPostalCode(String postalCode) {
this.postalCode = postalCode;
return this;
}
public Builder setState(String state) {
this.state = state;
return this;
}
}
/**
* City, district, suburb, town, or village.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCity() {
return this.city;
}
/**
* Two-letter country code (ISO
* 3166-1 alpha-2).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCountry() {
return this.country;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Address line 1 (e.g., street, PO Box, or company name).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLine1() {
return this.line1;
}
/**
* Address line 2 (e.g., apartment, suite, unit, or building).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLine2() {
return this.line2;
}
/**
* ZIP or postal code.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPostalCode() {
return this.postalCode;
}
/**
* State, county, province, or region.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getState() {
return this.state;
}
}
/**
* State, county, province, or region.
*/
public static class InvoiceSettings {
/**
* Default custom fields to be displayed on invoices for this customer. When updating, pass an
* empty string to remove previously-defined fields.
*/
@SerializedName("custom_fields")
Object customFields;
/**
* ID of a payment method that's attached to the customer, to be used as the customer's default
* payment method for subscriptions and invoices.
*/
@SerializedName("default_payment_method")
String defaultPaymentMethod;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Default footer to be displayed on invoices for this customer.
*/
@SerializedName("footer")
String footer;
private InvoiceSettings(Object customFields, String defaultPaymentMethod, Map extraParams, String footer) {
this.customFields = customFields;
this.defaultPaymentMethod = defaultPaymentMethod;
this.extraParams = extraParams;
this.footer = footer;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Object customFields;
private String defaultPaymentMethod;
private Map extraParams;
private String footer;
/**
* Finalize and obtain parameter instance from this builder.
*/
public InvoiceSettings build() {
return new InvoiceSettings(this.customFields, this.defaultPaymentMethod, this.extraParams, this.footer);
}
/**
* Add an element to `customFields` list. A list is initialized for the first `add/addAll`
* call, and subsequent calls adds additional elements to the original list. See {@link
* CustomerCreateParams.InvoiceSettings#customFields} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addCustomField(CustomField element) {
if (this.customFields == null || this.customFields instanceof EmptyParam) {
this.customFields = new ArrayList();
}
((List) this.customFields).add(element);
return this;
}
/**
* Add all elements to `customFields` list. A list is initialized for the first `add/addAll`
* call, and subsequent calls adds additional elements to the original list. See {@link
* CustomerCreateParams.InvoiceSettings#customFields} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addAllCustomField(List elements) {
if (this.customFields == null || this.customFields instanceof EmptyParam) {
this.customFields = new ArrayList();
}
((List) this.customFields).addAll(elements);
return this;
}
/**
* Default custom fields to be displayed on invoices for this customer. When updating, pass an
* empty string to remove previously-defined fields.
*/
public Builder setCustomFields(EmptyParam customFields) {
this.customFields = customFields;
return this;
}
/**
* Default custom fields to be displayed on invoices for this customer. When updating, pass an
* empty string to remove previously-defined fields.
*/
public Builder setCustomFields(List customFields) {
this.customFields = customFields;
return this;
}
/**
* ID of a payment method that's attached to the customer, to be used as the customer's
* default payment method for subscriptions and invoices.
*/
public Builder setDefaultPaymentMethod(String defaultPaymentMethod) {
this.defaultPaymentMethod = defaultPaymentMethod;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* CustomerCreateParams.InvoiceSettings#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link CustomerCreateParams.InvoiceSettings#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Default footer to be displayed on invoices for this customer.
*/
public Builder setFooter(String footer) {
this.footer = footer;
return this;
}
}
public static class CustomField {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/** The name of the custom field. This may be up to 30 characters. */
@SerializedName("name")
String name;
/** The value of the custom field. This may be up to 30 characters. */
@SerializedName("value")
String value;
private CustomField(Map extraParams, String name, String value) {
this.extraParams = extraParams;
this.name = name;
this.value = value;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private String name;
private String value;
/** Finalize and obtain parameter instance from this builder. */
public CustomField build() {
return new CustomField(this.extraParams, this.name, this.value);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link CustomerCreateParams.InvoiceSettings.CustomField#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link CustomerCreateParams.InvoiceSettings.CustomField#extraParams} for the
* field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/** The name of the custom field. This may be up to 30 characters. */
public Builder setName(String name) {
this.name = name;
return this;
}
public Builder setValue(String value) {
this.value = value;
return this;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getName() {
return this.name;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
/**
* Default custom fields to be displayed on invoices for this customer. When updating, pass an
* empty string to remove previously-defined fields.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getCustomFields() {
return this.customFields;
}
/**
* ID of a payment method that's attached to the customer, to be used as the customer's default
* payment method for subscriptions and invoices.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDefaultPaymentMethod() {
return this.defaultPaymentMethod;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Default footer to be displayed on invoices for this customer.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFooter() {
return this.footer;
}
}
/**
* The value of the custom field. This may be up to 30 characters.
*/
public static class Shipping {
/**
* Customer shipping address.
*/
@SerializedName("address")
Address address;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Customer name.
*/
@SerializedName("name")
String name;
/**
* Customer phone (including extension).
*/
@SerializedName("phone")
String phone;
private Shipping(Address address, Map extraParams, String name, String phone) {
this.address = address;
this.extraParams = extraParams;
this.name = name;
this.phone = phone;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Address address;
private Map extraParams;
private String name;
private String phone;
/**
* Finalize and obtain parameter instance from this builder.
*/
public Shipping build() {
return new Shipping(this.address, this.extraParams, this.name, this.phone);
}
/**
* Customer shipping address.
*/
public Builder setAddress(Address address) {
this.address = address;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* CustomerCreateParams.Shipping#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link CustomerCreateParams.Shipping#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Customer name.
*/
public Builder setName(String name) {
this.name = name;
return this;
}
/**
* Customer phone (including extension).
*/
public Builder setPhone(String phone) {
this.phone = phone;
return this;
}
}
public static class Address {
/** City, district, suburb, town, or village. */
@SerializedName("city")
String city;
/**
* Two-letter country code (ISO
* 3166-1 alpha-2).
*/
@SerializedName("country")
String country;
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/** Address line 1 (e.g., street, PO Box, or company name). */
@SerializedName("line1")
String line1;
/** Address line 2 (e.g., apartment, suite, unit, or building). */
@SerializedName("line2")
String line2;
/** ZIP or postal code. */
@SerializedName("postal_code")
String postalCode;
/** State, county, province, or region. */
@SerializedName("state")
String state;
private Address(String city, String country, Map extraParams, String line1, String line2, String postalCode, String state) {
this.city = city;
this.country = country;
this.extraParams = extraParams;
this.line1 = line1;
this.line2 = line2;
this.postalCode = postalCode;
this.state = state;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private String city;
private String country;
private Map extraParams;
private String line1;
private String line2;
private String postalCode;
private String state;
/** Finalize and obtain parameter instance from this builder. */
public Address build() {
return new Address(this.city, this.country, this.extraParams, this.line1, this.line2, this.postalCode, this.state);
}
/** City, district, suburb, town, or village. */
public Builder setCity(String city) {
this.city = city;
return this;
}
/**
* Two-letter country code (ISO
* 3166-1 alpha-2).
*/
public Builder setCountry(String country) {
this.country = country;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link CustomerCreateParams.Shipping.Address#extraParams} for the field
* documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link CustomerCreateParams.Shipping.Address#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/** Address line 1 (e.g., street, PO Box, or company name). */
public Builder setLine1(String line1) {
this.line1 = line1;
return this;
}
/** Address line 2 (e.g., apartment, suite, unit, or building). */
public Builder setLine2(String line2) {
this.line2 = line2;
return this;
}
/** ZIP or postal code. */
public Builder setPostalCode(String postalCode) {
this.postalCode = postalCode;
return this;
}
public Builder setState(String state) {
this.state = state;
return this;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCity() {
return this.city;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCountry() {
return this.country;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLine1() {
return this.line1;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLine2() {
return this.line2;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPostalCode() {
return this.postalCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getState() {
return this.state;
}
}
/**
* Customer shipping address.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Address getAddress() {
return this.address;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Customer name.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getName() {
return this.name;
}
/**
* Customer phone (including extension).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPhone() {
return this.phone;
}
}
/**
* State, county, province, or region.
*/
public static class TaxIdData {
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Type of the tax ID, one of {@code eu_vat}, {@code br_cnpj}, {@code br_cpf}, {@code nz_gst},
* {@code au_abn}, {@code in_gst}, {@code no_vat}, {@code za_vat}, {@code ch_vat}, {@code
* mx_rfc}, {@code sg_uen}, {@code ru_inn}, {@code ca_bn}, {@code hk_br}, {@code es_cif}, {@code
* tw_vat}, {@code th_vat}, {@code jp_cn}, {@code li_uid}, {@code my_itn}, {@code us_ein},
* {@code kr_brn}, {@code ca_qst}, {@code my_sst}, {@code sg_gst}, {@code ae_trn}, {@code
* cl_tin}, {@code sa_vat}, {@code id_npwp}, or {@code my_frp}.
*/
@SerializedName("type")
Type type;
/**
* Value of the tax ID.
*/
@SerializedName("value")
String value;
private TaxIdData(Map extraParams, Type type, String value) {
this.extraParams = extraParams;
this.type = type;
this.value = value;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private Type type;
private String value;
/**
* Finalize and obtain parameter instance from this builder.
*/
public TaxIdData build() {
return new TaxIdData(this.extraParams, this.type, this.value);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* CustomerCreateParams.TaxIdData#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link CustomerCreateParams.TaxIdData#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Type of the tax ID, one of {@code eu_vat}, {@code br_cnpj}, {@code br_cpf}, {@code nz_gst},
* {@code au_abn}, {@code in_gst}, {@code no_vat}, {@code za_vat}, {@code ch_vat}, {@code
* mx_rfc}, {@code sg_uen}, {@code ru_inn}, {@code ca_bn}, {@code hk_br}, {@code es_cif},
* {@code tw_vat}, {@code th_vat}, {@code jp_cn}, {@code li_uid}, {@code my_itn}, {@code
* us_ein}, {@code kr_brn}, {@code ca_qst}, {@code my_sst}, {@code sg_gst}, {@code ae_trn},
* {@code cl_tin}, {@code sa_vat}, {@code id_npwp}, or {@code my_frp}.
*/
public Builder setType(Type type) {
this.type = type;
return this;
}
/**
* Value of the tax ID.
*/
public Builder setValue(String value) {
this.value = value;
return this;
}
}
public enum Type implements ApiRequestParams.EnumParam {
@SerializedName("ae_trn")
AE_TRN("ae_trn"), @SerializedName("au_abn")
AU_ABN("au_abn"), @SerializedName("br_cnpj")
BR_CNPJ("br_cnpj"), @SerializedName("br_cpf")
BR_CPF("br_cpf"), @SerializedName("ca_bn")
CA_BN("ca_bn"), @SerializedName("ca_qst")
CA_QST("ca_qst"), @SerializedName("ch_vat")
CH_VAT("ch_vat"), @SerializedName("cl_tin")
CL_TIN("cl_tin"), @SerializedName("es_cif")
ES_CIF("es_cif"), @SerializedName("eu_vat")
EU_VAT("eu_vat"), @SerializedName("hk_br")
HK_BR("hk_br"), @SerializedName("id_npwp")
ID_NPWP("id_npwp"), @SerializedName("in_gst")
IN_GST("in_gst"), @SerializedName("jp_cn")
JP_CN("jp_cn"), @SerializedName("kr_brn")
KR_BRN("kr_brn"), @SerializedName("li_uid")
LI_UID("li_uid"), @SerializedName("mx_rfc")
MX_RFC("mx_rfc"), @SerializedName("my_frp")
MY_FRP("my_frp"), @SerializedName("my_itn")
MY_ITN("my_itn"), @SerializedName("my_sst")
MY_SST("my_sst"), @SerializedName("no_vat")
NO_VAT("no_vat"), @SerializedName("nz_gst")
NZ_GST("nz_gst"), @SerializedName("ru_inn")
RU_INN("ru_inn"), @SerializedName("sa_vat")
SA_VAT("sa_vat"), @SerializedName("sg_gst")
SG_GST("sg_gst"), @SerializedName("sg_uen")
SG_UEN("sg_uen"), @SerializedName("th_vat")
TH_VAT("th_vat"), @SerializedName("tw_vat")
TW_VAT("tw_vat"), @SerializedName("us_ein")
US_EIN("us_ein"), @SerializedName("za_vat")
ZA_VAT("za_vat");
private final String value;
Type(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Type of the tax ID, one of {@code eu_vat}, {@code br_cnpj}, {@code br_cpf}, {@code nz_gst},
* {@code au_abn}, {@code in_gst}, {@code no_vat}, {@code za_vat}, {@code ch_vat}, {@code
* mx_rfc}, {@code sg_uen}, {@code ru_inn}, {@code ca_bn}, {@code hk_br}, {@code es_cif}, {@code
* tw_vat}, {@code th_vat}, {@code jp_cn}, {@code li_uid}, {@code my_itn}, {@code us_ein},
* {@code kr_brn}, {@code ca_qst}, {@code my_sst}, {@code sg_gst}, {@code ae_trn}, {@code
* cl_tin}, {@code sa_vat}, {@code id_npwp}, or {@code my_frp}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Type getType() {
return this.type;
}
/**
* Value of the tax ID.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum TaxExempt implements ApiRequestParams.EnumParam {
@SerializedName("exempt")
EXEMPT("exempt"), @SerializedName("none")
NONE("none"), @SerializedName("reverse")
REVERSE("reverse");
private final String value;
TaxExempt(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
/**
* The customer's address.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getAddress() {
return this.address;
}
/**
* An integer amount in %s that represents the customer's current balance, which affect the
* customer's future invoices. A negative amount represents a credit that decreases the amount due
* on an invoice; a positive amount increases the amount due on an invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getBalance() {
return this.balance;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCoupon() {
return this.coupon;
}
/**
* An arbitrary string that you can attach to a customer object. It is displayed alongside the
* customer in the dashboard.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDescription() {
return this.description;
}
/**
* Customer's email address. It's displayed alongside the customer in your dashboard and can be
* useful for searching and tracking. This may be up to 512 characters.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getEmail() {
return this.email;
}
/**
* Specifies which fields in the response should be expanded.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getExpand() {
return this.expand;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* The prefix for the customer used to generate unique invoice numbers. Must be 3–12 uppercase
* letters or numbers.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getInvoicePrefix() {
return this.invoicePrefix;
}
/**
* Default invoice settings for this customer.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public InvoiceSettings getInvoiceSettings() {
return this.invoiceSettings;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset by
* posting an empty value to them. All keys can be unset by posting an empty value to {@code
* metadata}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getMetadata() {
return this.metadata;
}
/**
* The customer's full name or business name.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getName() {
return this.name;
}
/**
* The sequence to be used on the customer's next invoice. Defaults to 1.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getNextInvoiceSequence() {
return this.nextInvoiceSequence;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPaymentMethod() {
return this.paymentMethod;
}
/**
* The customer's phone number.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPhone() {
return this.phone;
}
/**
* Customer's preferred languages, ordered by preference.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getPreferredLocales() {
return this.preferredLocales;
}
/**
* The customer's shipping information. Appears on invoices emailed to this customer.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getShipping() {
return this.shipping;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSource() {
return this.source;
}
/**
* The customer's tax exemption. One of {@code none}, {@code exempt}, or {@code reverse}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public EnumParam getTaxExempt() {
return this.taxExempt;
}
/**
* The customer's tax IDs.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getTaxIdData() {
return this.taxIdData;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy