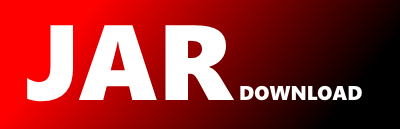
com.stripe.param.PaymentIntentUpdateParams Maven / Gradle / Ivy
// Generated by delombok at Wed Jun 10 17:29:56 PDT 2020
package com.stripe.param;
import com.google.gson.annotations.SerializedName;
import com.stripe.net.ApiRequestParams;
import com.stripe.net.ApiRequestParams.EnumParam;
import com.stripe.param.common.EmptyParam;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class PaymentIntentUpdateParams extends ApiRequestParams {
/**
* Amount intended to be collected by this PaymentIntent. A positive integer representing how much
* to charge in the smallest currency
* unit (e.g., 100 cents to charge $1.00 or 100 to charge ¥100, a zero-decimal currency). The
* minimum amount is $0.50 US or equivalent in
* charge currency. The amount value supports up to eight digits (e.g., a value of 99999999
* for a USD charge of $999,999.99).
*/
@SerializedName("amount")
Long amount;
/**
* The amount of the application fee (if any) for the resulting payment. See the PaymentIntents use case for connected accounts
* for details.
*/
@SerializedName("application_fee_amount")
Object applicationFeeAmount;
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@SerializedName("currency")
Object currency;
/**
* ID of the Customer this PaymentIntent belongs to, if one exists.
*
* Payment methods attached to other Customers cannot be used with this PaymentIntent.
*
*
If present in combination with setup_future_usage,
* this PaymentIntent's payment method will be attached to the Customer after the PaymentIntent
* has been confirmed and any required actions from the user are complete.
*/
@SerializedName("customer")
Object customer;
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
*/
@SerializedName("description")
Object description;
/**
* Specifies which fields in the response should be expanded.
*/
@SerializedName("expand")
List expand;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset by
* posting an empty value to them. All keys can be unset by posting an empty value to {@code
* metadata}.
*/
@SerializedName("metadata")
Object metadata;
/**
* ID of the payment method (a PaymentMethod, Card, or compatible Source
* object) to attach to this PaymentIntent.
*/
@SerializedName("payment_method")
Object paymentMethod;
/**
* Payment-method-specific configuration for this PaymentIntent.
*/
@SerializedName("payment_method_options")
PaymentMethodOptions paymentMethodOptions;
/**
* The list of payment method types (e.g. card) that this PaymentIntent is allowed to use.
*/
@SerializedName("payment_method_types")
List paymentMethodTypes;
/**
* Email address that the receipt for the resulting payment will be sent to.
*/
@SerializedName("receipt_email")
Object receiptEmail;
/**
* If the PaymentIntent has a {@code payment_method} and a {@code customer} or if you're attaching
* a payment method to the PaymentIntent in this request, you can pass {@code
* save_payment_method=true} to save the payment method to the customer immediately.
*
* If the payment method is already saved to a customer, this parameter does nothing. If this
* type of payment method cannot be saved to a customer, the request will error.
*
*
Saving a payment method using this parameter is not recommended because it will
* save the payment method even if it cannot be charged (e.g. the user made a typo). To ensure
* that only payment methods which are likely to be chargeable are saved to a customer, use the
* (setup_future_usage)[#payment_intents/object#payment_intent_object-setup_future_usage]
* property, which saves the payment method after the PaymentIntent has been confirmed and all
* required actions by the customer are complete.
*/
@SerializedName("save_payment_method")
Boolean savePaymentMethod;
/**
* Indicates that you intend to make future payments with this PaymentIntent's payment method.
*
*
Providing this parameter will attach the payment method to
* the PaymentIntent's Customer, if present, after the PaymentIntent is confirmed and any required
* actions from the user are complete. If no Customer was provided, the payment method can still
* be attached to a Customer
* after the transaction completes.
*
*
When processing card payments, Stripe also uses {@code setup_future_usage} to dynamically
* optimize your payment flow and comply with regional legislation and network rules, such as SCA.
*
*
If {@code setup_future_usage} is already set and you are performing a request using a
* publishable key, you may only update the value from {@code on_session} to {@code off_session}.
*/
@SerializedName("setup_future_usage")
EnumParam setupFutureUsage;
/**
* Shipping information for this PaymentIntent.
*/
@SerializedName("shipping")
Object shipping;
/**
* This is a legacy field that will be removed in the future. It is the ID of the Source object to
* attach to this PaymentIntent. Please use the {@code payment_method} field instead, which also
* supports Cards and compatible Source
* objects.
*/
@SerializedName("source")
Object source;
/**
* For non-card charges, you can use this value as the complete description that appears on your
* customers’ statements. Must contain at least one letter, maximum 22 characters.
*/
@SerializedName("statement_descriptor")
Object statementDescriptor;
/**
* Provides information about a card payment that customers see on their statements. Concatenated
* with the prefix (shortened descriptor) or statement descriptor that’s set on the account to
* form the complete statement descriptor. Maximum 22 characters for the concatenated descriptor.
*/
@SerializedName("statement_descriptor_suffix")
Object statementDescriptorSuffix;
/**
* The parameters used to automatically create a Transfer when the payment succeeds. For more
* information, see the PaymentIntents use case for connected accounts.
*/
@SerializedName("transfer_data")
TransferData transferData;
/**
* A string that identifies the resulting payment as part of a group. {@code transfer_group} may
* only be provided if it has not been set. See the PaymentIntents use case for connected accounts
* for details.
*/
@SerializedName("transfer_group")
Object transferGroup;
private PaymentIntentUpdateParams(Long amount, Object applicationFeeAmount, Object currency, Object customer, Object description, List expand, Map extraParams, Object metadata, Object paymentMethod, PaymentMethodOptions paymentMethodOptions, List paymentMethodTypes, Object receiptEmail, Boolean savePaymentMethod, EnumParam setupFutureUsage, Object shipping, Object source, Object statementDescriptor, Object statementDescriptorSuffix, TransferData transferData, Object transferGroup) {
this.amount = amount;
this.applicationFeeAmount = applicationFeeAmount;
this.currency = currency;
this.customer = customer;
this.description = description;
this.expand = expand;
this.extraParams = extraParams;
this.metadata = metadata;
this.paymentMethod = paymentMethod;
this.paymentMethodOptions = paymentMethodOptions;
this.paymentMethodTypes = paymentMethodTypes;
this.receiptEmail = receiptEmail;
this.savePaymentMethod = savePaymentMethod;
this.setupFutureUsage = setupFutureUsage;
this.shipping = shipping;
this.source = source;
this.statementDescriptor = statementDescriptor;
this.statementDescriptorSuffix = statementDescriptorSuffix;
this.transferData = transferData;
this.transferGroup = transferGroup;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Long amount;
private Object applicationFeeAmount;
private Object currency;
private Object customer;
private Object description;
private List expand;
private Map extraParams;
private Object metadata;
private Object paymentMethod;
private PaymentMethodOptions paymentMethodOptions;
private List paymentMethodTypes;
private Object receiptEmail;
private Boolean savePaymentMethod;
private EnumParam setupFutureUsage;
private Object shipping;
private Object source;
private Object statementDescriptor;
private Object statementDescriptorSuffix;
private TransferData transferData;
private Object transferGroup;
/**
* Finalize and obtain parameter instance from this builder.
*/
public PaymentIntentUpdateParams build() {
return new PaymentIntentUpdateParams(this.amount, this.applicationFeeAmount, this.currency, this.customer, this.description, this.expand, this.extraParams, this.metadata, this.paymentMethod, this.paymentMethodOptions, this.paymentMethodTypes, this.receiptEmail, this.savePaymentMethod, this.setupFutureUsage, this.shipping, this.source, this.statementDescriptor, this.statementDescriptorSuffix, this.transferData, this.transferGroup);
}
/**
* Amount intended to be collected by this PaymentIntent. A positive integer representing how
* much to charge in the smallest
* currency unit (e.g., 100 cents to charge $1.00 or 100 to charge ¥100, a zero-decimal
* currency). The minimum amount is $0.50 US or equivalent in
* charge currency. The amount value supports up to eight digits (e.g., a value of 99999999
* for a USD charge of $999,999.99).
*/
public Builder setAmount(Long amount) {
this.amount = amount;
return this;
}
/**
* The amount of the application fee (if any) for the resulting payment. See the PaymentIntents
* use case for connected
* accounts for details.
*/
public Builder setApplicationFeeAmount(Long applicationFeeAmount) {
this.applicationFeeAmount = applicationFeeAmount;
return this;
}
/**
* The amount of the application fee (if any) for the resulting payment. See the PaymentIntents
* use case for connected
* accounts for details.
*/
public Builder setApplicationFeeAmount(EmptyParam applicationFeeAmount) {
this.applicationFeeAmount = applicationFeeAmount;
return this;
}
/**
* Three-letter ISO currency
* code, in lowercase. Must be a supported
* currency.
*/
public Builder setCurrency(String currency) {
this.currency = currency;
return this;
}
/**
* Three-letter ISO currency
* code, in lowercase. Must be a supported
* currency.
*/
public Builder setCurrency(EmptyParam currency) {
this.currency = currency;
return this;
}
/**
* ID of the Customer this PaymentIntent belongs to, if one exists.
*
* Payment methods attached to other Customers cannot be used with this PaymentIntent.
*
*
If present in combination with setup_future_usage,
* this PaymentIntent's payment method will be attached to the Customer after the PaymentIntent
* has been confirmed and any required actions from the user are complete.
*/
public Builder setCustomer(String customer) {
this.customer = customer;
return this;
}
/**
* ID of the Customer this PaymentIntent belongs to, if one exists.
*
*
Payment methods attached to other Customers cannot be used with this PaymentIntent.
*
*
If present in combination with setup_future_usage,
* this PaymentIntent's payment method will be attached to the Customer after the PaymentIntent
* has been confirmed and any required actions from the user are complete.
*/
public Builder setCustomer(EmptyParam customer) {
this.customer = customer;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
*/
public Builder setDescription(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
*/
public Builder setDescription(EmptyParam description) {
this.description = description;
return this;
}
/**
* Add an element to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* PaymentIntentUpdateParams#expand} for the field documentation.
*/
public Builder addExpand(String element) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.add(element);
return this;
}
/**
* Add all elements to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* PaymentIntentUpdateParams#expand} for the field documentation.
*/
public Builder addAllExpand(List elements) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.addAll(elements);
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* PaymentIntentUpdateParams#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link PaymentIntentUpdateParams#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Add a key/value pair to `metadata` map. A map is initialized for the first `put/putAll` call,
* and subsequent calls add additional key/value pairs to the original map. See {@link
* PaymentIntentUpdateParams#metadata} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder putMetadata(String key, String value) {
if (this.metadata == null || this.metadata instanceof EmptyParam) {
this.metadata = new HashMap();
}
((Map) this.metadata).put(key, value);
return this;
}
/**
* Add all map key/value pairs to `metadata` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link PaymentIntentUpdateParams#metadata} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder putAllMetadata(Map map) {
if (this.metadata == null || this.metadata instanceof EmptyParam) {
this.metadata = new HashMap();
}
((Map) this.metadata).putAll(map);
return this;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset
* by posting an empty value to them. All keys can be unset by posting an empty value to {@code
* metadata}.
*/
public Builder setMetadata(EmptyParam metadata) {
this.metadata = metadata;
return this;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset
* by posting an empty value to them. All keys can be unset by posting an empty value to {@code
* metadata}.
*/
public Builder setMetadata(Map metadata) {
this.metadata = metadata;
return this;
}
/**
* ID of the payment method (a PaymentMethod, Card, or compatible Source
* object) to attach to this PaymentIntent.
*/
public Builder setPaymentMethod(String paymentMethod) {
this.paymentMethod = paymentMethod;
return this;
}
/**
* ID of the payment method (a PaymentMethod, Card, or compatible Source
* object) to attach to this PaymentIntent.
*/
public Builder setPaymentMethod(EmptyParam paymentMethod) {
this.paymentMethod = paymentMethod;
return this;
}
/**
* Payment-method-specific configuration for this PaymentIntent.
*/
public Builder setPaymentMethodOptions(PaymentMethodOptions paymentMethodOptions) {
this.paymentMethodOptions = paymentMethodOptions;
return this;
}
/**
* Add an element to `paymentMethodTypes` list. A list is initialized for the first `add/addAll`
* call, and subsequent calls adds additional elements to the original list. See {@link
* PaymentIntentUpdateParams#paymentMethodTypes} for the field documentation.
*/
public Builder addPaymentMethodType(String element) {
if (this.paymentMethodTypes == null) {
this.paymentMethodTypes = new ArrayList<>();
}
this.paymentMethodTypes.add(element);
return this;
}
/**
* Add all elements to `paymentMethodTypes` list. A list is initialized for the first
* `add/addAll` call, and subsequent calls adds additional elements to the original list. See
* {@link PaymentIntentUpdateParams#paymentMethodTypes} for the field documentation.
*/
public Builder addAllPaymentMethodType(List elements) {
if (this.paymentMethodTypes == null) {
this.paymentMethodTypes = new ArrayList<>();
}
this.paymentMethodTypes.addAll(elements);
return this;
}
/**
* Email address that the receipt for the resulting payment will be sent to.
*/
public Builder setReceiptEmail(String receiptEmail) {
this.receiptEmail = receiptEmail;
return this;
}
/**
* Email address that the receipt for the resulting payment will be sent to.
*/
public Builder setReceiptEmail(EmptyParam receiptEmail) {
this.receiptEmail = receiptEmail;
return this;
}
/**
* If the PaymentIntent has a {@code payment_method} and a {@code customer} or if you're
* attaching a payment method to the PaymentIntent in this request, you can pass {@code
* save_payment_method=true} to save the payment method to the customer immediately.
*
* If the payment method is already saved to a customer, this parameter does nothing. If this
* type of payment method cannot be saved to a customer, the request will error.
*
*
Saving a payment method using this parameter is not recommended because it will
* save the payment method even if it cannot be charged (e.g. the user made a typo). To ensure
* that only payment methods which are likely to be chargeable are saved to a customer, use the
* (setup_future_usage)[#payment_intents/object#payment_intent_object-setup_future_usage]
* property, which saves the payment method after the PaymentIntent has been confirmed and all
* required actions by the customer are complete.
*/
public Builder setSavePaymentMethod(Boolean savePaymentMethod) {
this.savePaymentMethod = savePaymentMethod;
return this;
}
/**
* Indicates that you intend to make future payments with this PaymentIntent's payment method.
*
*
Providing this parameter will attach the payment method to
* the PaymentIntent's Customer, if present, after the PaymentIntent is confirmed and any
* required actions from the user are complete. If no Customer was provided, the payment method
* can still be attached to a
* Customer after the transaction completes.
*
*
When processing card payments, Stripe also uses {@code setup_future_usage} to dynamically
* optimize your payment flow and comply with regional legislation and network rules, such as SCA.
*
*
If {@code setup_future_usage} is already set and you are performing a request using a
* publishable key, you may only update the value from {@code on_session} to {@code
* off_session}.
*/
public Builder setSetupFutureUsage(SetupFutureUsage setupFutureUsage) {
this.setupFutureUsage = setupFutureUsage;
return this;
}
/**
* Indicates that you intend to make future payments with this PaymentIntent's payment method.
*
*
Providing this parameter will attach the payment method to
* the PaymentIntent's Customer, if present, after the PaymentIntent is confirmed and any
* required actions from the user are complete. If no Customer was provided, the payment method
* can still be attached to a
* Customer after the transaction completes.
*
*
When processing card payments, Stripe also uses {@code setup_future_usage} to dynamically
* optimize your payment flow and comply with regional legislation and network rules, such as SCA.
*
*
If {@code setup_future_usage} is already set and you are performing a request using a
* publishable key, you may only update the value from {@code on_session} to {@code
* off_session}.
*/
public Builder setSetupFutureUsage(EmptyParam setupFutureUsage) {
this.setupFutureUsage = setupFutureUsage;
return this;
}
/**
* Shipping information for this PaymentIntent.
*/
public Builder setShipping(Shipping shipping) {
this.shipping = shipping;
return this;
}
/**
* Shipping information for this PaymentIntent.
*/
public Builder setShipping(EmptyParam shipping) {
this.shipping = shipping;
return this;
}
/**
* This is a legacy field that will be removed in the future. It is the ID of the Source object
* to attach to this PaymentIntent. Please use the {@code payment_method} field instead, which
* also supports Cards and compatible Source
* objects.
*/
public Builder setSource(String source) {
this.source = source;
return this;
}
/**
* This is a legacy field that will be removed in the future. It is the ID of the Source object
* to attach to this PaymentIntent. Please use the {@code payment_method} field instead, which
* also supports Cards and compatible Source
* objects.
*/
public Builder setSource(EmptyParam source) {
this.source = source;
return this;
}
/**
* For non-card charges, you can use this value as the complete description that appears on your
* customers’ statements. Must contain at least one letter, maximum 22 characters.
*/
public Builder setStatementDescriptor(String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
return this;
}
/**
* For non-card charges, you can use this value as the complete description that appears on your
* customers’ statements. Must contain at least one letter, maximum 22 characters.
*/
public Builder setStatementDescriptor(EmptyParam statementDescriptor) {
this.statementDescriptor = statementDescriptor;
return this;
}
/**
* Provides information about a card payment that customers see on their statements.
* Concatenated with the prefix (shortened descriptor) or statement descriptor that’s set on the
* account to form the complete statement descriptor. Maximum 22 characters for the concatenated
* descriptor.
*/
public Builder setStatementDescriptorSuffix(String statementDescriptorSuffix) {
this.statementDescriptorSuffix = statementDescriptorSuffix;
return this;
}
/**
* Provides information about a card payment that customers see on their statements.
* Concatenated with the prefix (shortened descriptor) or statement descriptor that’s set on the
* account to form the complete statement descriptor. Maximum 22 characters for the concatenated
* descriptor.
*/
public Builder setStatementDescriptorSuffix(EmptyParam statementDescriptorSuffix) {
this.statementDescriptorSuffix = statementDescriptorSuffix;
return this;
}
/**
* The parameters used to automatically create a Transfer when the payment succeeds. For more
* information, see the PaymentIntents use case for connected
* accounts.
*/
public Builder setTransferData(TransferData transferData) {
this.transferData = transferData;
return this;
}
/**
* A string that identifies the resulting payment as part of a group. {@code transfer_group} may
* only be provided if it has not been set. See the PaymentIntents use case for connected
* accounts for details.
*/
public Builder setTransferGroup(String transferGroup) {
this.transferGroup = transferGroup;
return this;
}
/**
* A string that identifies the resulting payment as part of a group. {@code transfer_group} may
* only be provided if it has not been set. See the PaymentIntents use case for connected
* accounts for details.
*/
public Builder setTransferGroup(EmptyParam transferGroup) {
this.transferGroup = transferGroup;
return this;
}
}
public static class PaymentMethodOptions {
/**
* If this is a {@code bancontact} PaymentMethod, this sub-hash contains details about the
* Bancontact payment method options.
*/
@SerializedName("bancontact")
Object bancontact;
/**
* Configuration for any card payments attempted on this PaymentIntent.
*/
@SerializedName("card")
Object card;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
private PaymentMethodOptions(Object bancontact, Object card, Map extraParams) {
this.bancontact = bancontact;
this.card = card;
this.extraParams = extraParams;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Object bancontact;
private Object card;
private Map extraParams;
/**
* Finalize and obtain parameter instance from this builder.
*/
public PaymentMethodOptions build() {
return new PaymentMethodOptions(this.bancontact, this.card, this.extraParams);
}
/**
* If this is a {@code bancontact} PaymentMethod, this sub-hash contains details about the
* Bancontact payment method options.
*/
public Builder setBancontact(Bancontact bancontact) {
this.bancontact = bancontact;
return this;
}
/**
* If this is a {@code bancontact} PaymentMethod, this sub-hash contains details about the
* Bancontact payment method options.
*/
public Builder setBancontact(EmptyParam bancontact) {
this.bancontact = bancontact;
return this;
}
/**
* Configuration for any card payments attempted on this PaymentIntent.
*/
public Builder setCard(Card card) {
this.card = card;
return this;
}
/**
* Configuration for any card payments attempted on this PaymentIntent.
*/
public Builder setCard(EmptyParam card) {
this.card = card;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* PaymentIntentUpdateParams.PaymentMethodOptions#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link PaymentIntentUpdateParams.PaymentMethodOptions#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
}
public static class Bancontact {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Preferred language of the Bancontact authorization page that the customer is redirected to.
*/
@SerializedName("preferred_language")
PreferredLanguage preferredLanguage;
private Bancontact(Map extraParams, PreferredLanguage preferredLanguage) {
this.extraParams = extraParams;
this.preferredLanguage = preferredLanguage;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private PreferredLanguage preferredLanguage;
/**
* Finalize and obtain parameter instance from this builder.
*/
public Bancontact build() {
return new Bancontact(this.extraParams, this.preferredLanguage);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link PaymentIntentUpdateParams.PaymentMethodOptions.Bancontact#extraParams}
* for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link PaymentIntentUpdateParams.PaymentMethodOptions.Bancontact#extraParams}
* for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Preferred language of the Bancontact authorization page that the customer is redirected
* to.
*/
public Builder setPreferredLanguage(PreferredLanguage preferredLanguage) {
this.preferredLanguage = preferredLanguage;
return this;
}
}
public enum PreferredLanguage implements ApiRequestParams.EnumParam {
@SerializedName("de")
DE("de"), @SerializedName("en")
EN("en"), @SerializedName("fr")
FR("fr"), @SerializedName("nl")
NL("nl");
private final String value;
PreferredLanguage(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Preferred language of the Bancontact authorization page that the customer is redirected to.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PreferredLanguage getPreferredLanguage() {
return this.preferredLanguage;
}
}
public static class Card {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Installment configuration for payments attempted on this PaymentIntent (Mexico Only).
*
* For more information, see the installments integration guide.
*/
@SerializedName("installments")
Installments installments;
/**
* When specified, this parameter indicates that a transaction will be marked as MOTO (Mail
* Order Telephone Order) and thus out of scope for SCA. This parameter can only be provided
* during confirmation.
*/
@SerializedName("moto")
Boolean moto;
/**
* Selected network to process this PaymentIntent on. Depends on the available networks of the
* card attached to the PaymentIntent. Can be only set confirm-time.
*/
@SerializedName("network")
Network network;
/**
* We strongly recommend that you rely on our SCA Engine to automatically prompt your
* customers for authentication based on risk level and other requirements.
* However, if you wish to request 3D Secure based on logic from your own fraud engine,
* provide this option. Permitted values include: {@code automatic} or {@code any}. If not
* provided, defaults to {@code automatic}. Read our guide on manually requesting 3D
* Secure for more information on how this configuration interacts with Radar and our SCA
* Engine.
*/
@SerializedName("request_three_d_secure")
RequestThreeDSecure requestThreeDSecure;
private Card(Map extraParams, Installments installments, Boolean moto, Network network, RequestThreeDSecure requestThreeDSecure) {
this.extraParams = extraParams;
this.installments = installments;
this.moto = moto;
this.network = network;
this.requestThreeDSecure = requestThreeDSecure;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private Installments installments;
private Boolean moto;
private Network network;
private RequestThreeDSecure requestThreeDSecure;
/** Finalize and obtain parameter instance from this builder. */
public Card build() {
return new Card(this.extraParams, this.installments, this.moto, this.network, this.requestThreeDSecure);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link PaymentIntentUpdateParams.PaymentMethodOptions.Card#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link PaymentIntentUpdateParams.PaymentMethodOptions.Card#extraParams} for the
* field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Installment configuration for payments attempted on this PaymentIntent (Mexico Only).
*
* For more information, see the installments integration guide.
*/
public Builder setInstallments(Installments installments) {
this.installments = installments;
return this;
}
/**
* When specified, this parameter indicates that a transaction will be marked as MOTO (Mail
* Order Telephone Order) and thus out of scope for SCA. This parameter can only be provided
* during confirmation.
*/
public Builder setMoto(Boolean moto) {
this.moto = moto;
return this;
}
/**
* Selected network to process this PaymentIntent on. Depends on the available networks of
* the card attached to the PaymentIntent. Can be only set confirm-time.
*/
public Builder setNetwork(Network network) {
this.network = network;
return this;
}
/**
* We strongly recommend that you rely on our SCA Engine to automatically prompt your
* customers for authentication based on risk level and other requirements.
* However, if you wish to request 3D Secure based on logic from your own fraud engine,
* provide this option. Permitted values include: {@code automatic} or {@code any}. If not
* provided, defaults to {@code automatic}. Read our guide on manually requesting 3D
* Secure for more information on how this configuration interacts with Radar and our
* SCA Engine.
*/
public Builder setRequestThreeDSecure(RequestThreeDSecure requestThreeDSecure) {
this.requestThreeDSecure = requestThreeDSecure;
return this;
}
}
public static class Installments {
/**
* Setting to true enables installments for this PaymentIntent. This will cause the response
* to contain a list of available installment plans. Setting to false will prevent any
* selected plan from applying to a charge.
*/
@SerializedName("enabled")
Boolean enabled;
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field
* (serialized) name in this param object. Effectively, this map is flattened to its parent
* instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* The selected installment plan to use for this payment attempt. This parameter can only be
* provided during confirmation.
*/
@SerializedName("plan")
Object plan;
private Installments(Boolean enabled, Map extraParams, Object plan) {
this.enabled = enabled;
this.extraParams = extraParams;
this.plan = plan;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Boolean enabled;
private Map extraParams;
private Object plan;
/** Finalize and obtain parameter instance from this builder. */
public Installments build() {
return new Installments(this.enabled, this.extraParams, this.plan);
}
/**
* Setting to true enables installments for this PaymentIntent. This will cause the
* response to contain a list of available installment plans. Setting to false will
* prevent any selected plan from applying to a charge.
*/
public Builder setEnabled(Boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* PaymentIntentUpdateParams.PaymentMethodOptions.Card.Installments#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* PaymentIntentUpdateParams.PaymentMethodOptions.Card.Installments#extraParams} for the
* field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* The selected installment plan to use for this payment attempt. This parameter can only
* be provided during confirmation.
*/
public Builder setPlan(Plan plan) {
this.plan = plan;
return this;
}
/**
* The selected installment plan to use for this payment attempt. This parameter can only
* be provided during confirmation.
*/
public Builder setPlan(EmptyParam plan) {
this.plan = plan;
return this;
}
}
public static class Plan {
/**
* For {@code fixed_count} installment plans, this is the number of installment payments
* your customer will make to their credit card.
*/
@SerializedName("count")
Long count;
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field
* (serialized) name in this param object. Effectively, this map is flattened to its
* parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* For {@code fixed_count} installment plans, this is the interval between installment
* payments your customer will make to their credit card. One of {@code month}.
*/
@SerializedName("interval")
Interval interval;
/** Type of installment plan, one of {@code fixed_count}. */
@SerializedName("type")
Type type;
private Plan(Long count, Map extraParams, Interval interval, Type type) {
this.count = count;
this.extraParams = extraParams;
this.interval = interval;
this.type = type;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Long count;
private Map extraParams;
private Interval interval;
private Type type;
/** Finalize and obtain parameter instance from this builder. */
public Plan build() {
return new Plan(this.count, this.extraParams, this.interval, this.type);
}
/**
* For {@code fixed_count} installment plans, this is the number of installment payments
* your customer will make to their credit card.
*/
public Builder setCount(Long count) {
this.count = count;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the
* original map. See {@link
* PaymentIntentUpdateParams.PaymentMethodOptions.Card.Installments.Plan#extraParams}
* for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the
* original map. See {@link
* PaymentIntentUpdateParams.PaymentMethodOptions.Card.Installments.Plan#extraParams}
* for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* For {@code fixed_count} installment plans, this is the interval between installment
* payments your customer will make to their credit card. One of {@code month}.
*/
public Builder setInterval(Interval interval) {
this.interval = interval;
return this;
}
public Builder setType(Type type) {
this.type = type;
return this;
}
}
public enum Interval implements ApiRequestParams.EnumParam {
@SerializedName("month")
MONTH("month");
private final String value;
Interval(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum Type implements ApiRequestParams.EnumParam {
@SerializedName("fixed_count")
FIXED_COUNT("fixed_count");
private final String value;
Type(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCount() {
return this.count;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Interval getInterval() {
return this.interval;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Type getType() {
return this.type;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getEnabled() {
return this.enabled;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getPlan() {
return this.plan;
}
}
public enum Network implements ApiRequestParams.EnumParam {
@SerializedName("amex")
AMEX("amex"), @SerializedName("cartes_bancaires")
CARTES_BANCAIRES("cartes_bancaires"), @SerializedName("diners")
DINERS("diners"), @SerializedName("discover")
DISCOVER("discover"), @SerializedName("interac")
INTERAC("interac"), @SerializedName("jcb")
JCB("jcb"), @SerializedName("mastercard")
MASTERCARD("mastercard"), @SerializedName("unionpay")
UNIONPAY("unionpay"), @SerializedName("unknown")
UNKNOWN("unknown"), @SerializedName("visa")
VISA("visa");
private final String value;
Network(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum RequestThreeDSecure implements ApiRequestParams.EnumParam {
@SerializedName("any")
ANY("any"), @SerializedName("automatic")
AUTOMATIC("automatic");
private final String value;
RequestThreeDSecure(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Installments getInstallments() {
return this.installments;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getMoto() {
return this.moto;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Network getNetwork() {
return this.network;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public RequestThreeDSecure getRequestThreeDSecure() {
return this.requestThreeDSecure;
}
}
/**
* If this is a {@code bancontact} PaymentMethod, this sub-hash contains details about the
* Bancontact payment method options.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getBancontact() {
return this.bancontact;
}
/**
* Configuration for any card payments attempted on this PaymentIntent.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getCard() {
return this.card;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
}
/**
* Type of installment plan, one of {@code fixed_count}.
*/
public static class Shipping {
/**
* Shipping address.
*/
@SerializedName("address")
Address address;
/**
* The delivery service that shipped a physical product, such as Fedex, UPS, USPS, etc.
*/
@SerializedName("carrier")
Object carrier;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Recipient name.
*/
@SerializedName("name")
Object name;
/**
* Recipient phone (including extension).
*/
@SerializedName("phone")
Object phone;
/**
* The tracking number for a physical product, obtained from the delivery service. If multiple
* tracking numbers were generated for this purchase, please separate them with commas.
*/
@SerializedName("tracking_number")
Object trackingNumber;
private Shipping(Address address, Object carrier, Map extraParams, Object name, Object phone, Object trackingNumber) {
this.address = address;
this.carrier = carrier;
this.extraParams = extraParams;
this.name = name;
this.phone = phone;
this.trackingNumber = trackingNumber;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Address address;
private Object carrier;
private Map extraParams;
private Object name;
private Object phone;
private Object trackingNumber;
/**
* Finalize and obtain parameter instance from this builder.
*/
public Shipping build() {
return new Shipping(this.address, this.carrier, this.extraParams, this.name, this.phone, this.trackingNumber);
}
/**
* Shipping address.
*/
public Builder setAddress(Address address) {
this.address = address;
return this;
}
/**
* The delivery service that shipped a physical product, such as Fedex, UPS, USPS, etc.
*/
public Builder setCarrier(String carrier) {
this.carrier = carrier;
return this;
}
/**
* The delivery service that shipped a physical product, such as Fedex, UPS, USPS, etc.
*/
public Builder setCarrier(EmptyParam carrier) {
this.carrier = carrier;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* PaymentIntentUpdateParams.Shipping#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link PaymentIntentUpdateParams.Shipping#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Recipient name.
*/
public Builder setName(String name) {
this.name = name;
return this;
}
/**
* Recipient name.
*/
public Builder setName(EmptyParam name) {
this.name = name;
return this;
}
/**
* Recipient phone (including extension).
*/
public Builder setPhone(String phone) {
this.phone = phone;
return this;
}
/**
* Recipient phone (including extension).
*/
public Builder setPhone(EmptyParam phone) {
this.phone = phone;
return this;
}
/**
* The tracking number for a physical product, obtained from the delivery service. If multiple
* tracking numbers were generated for this purchase, please separate them with commas.
*/
public Builder setTrackingNumber(String trackingNumber) {
this.trackingNumber = trackingNumber;
return this;
}
/**
* The tracking number for a physical product, obtained from the delivery service. If multiple
* tracking numbers were generated for this purchase, please separate them with commas.
*/
public Builder setTrackingNumber(EmptyParam trackingNumber) {
this.trackingNumber = trackingNumber;
return this;
}
}
public static class Address {
/** City, district, suburb, town, or village. */
@SerializedName("city")
Object city;
/**
* Two-letter country code (ISO
* 3166-1 alpha-2).
*/
@SerializedName("country")
Object country;
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/** Address line 1 (e.g., street, PO Box, or company name). */
@SerializedName("line1")
Object line1;
/** Address line 2 (e.g., apartment, suite, unit, or building). */
@SerializedName("line2")
Object line2;
/** ZIP or postal code. */
@SerializedName("postal_code")
Object postalCode;
/** State, county, province, or region. */
@SerializedName("state")
Object state;
private Address(Object city, Object country, Map extraParams, Object line1, Object line2, Object postalCode, Object state) {
this.city = city;
this.country = country;
this.extraParams = extraParams;
this.line1 = line1;
this.line2 = line2;
this.postalCode = postalCode;
this.state = state;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Object city;
private Object country;
private Map extraParams;
private Object line1;
private Object line2;
private Object postalCode;
private Object state;
/** Finalize and obtain parameter instance from this builder. */
public Address build() {
return new Address(this.city, this.country, this.extraParams, this.line1, this.line2, this.postalCode, this.state);
}
/** City, district, suburb, town, or village. */
public Builder setCity(String city) {
this.city = city;
return this;
}
/** City, district, suburb, town, or village. */
public Builder setCity(EmptyParam city) {
this.city = city;
return this;
}
/**
* Two-letter country code (ISO
* 3166-1 alpha-2).
*/
public Builder setCountry(String country) {
this.country = country;
return this;
}
/**
* Two-letter country code (ISO
* 3166-1 alpha-2).
*/
public Builder setCountry(EmptyParam country) {
this.country = country;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link PaymentIntentUpdateParams.Shipping.Address#extraParams} for the field
* documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link PaymentIntentUpdateParams.Shipping.Address#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/** Address line 1 (e.g., street, PO Box, or company name). */
public Builder setLine1(String line1) {
this.line1 = line1;
return this;
}
/** Address line 1 (e.g., street, PO Box, or company name). */
public Builder setLine1(EmptyParam line1) {
this.line1 = line1;
return this;
}
/** Address line 2 (e.g., apartment, suite, unit, or building). */
public Builder setLine2(String line2) {
this.line2 = line2;
return this;
}
/** Address line 2 (e.g., apartment, suite, unit, or building). */
public Builder setLine2(EmptyParam line2) {
this.line2 = line2;
return this;
}
/** ZIP or postal code. */
public Builder setPostalCode(String postalCode) {
this.postalCode = postalCode;
return this;
}
/** ZIP or postal code. */
public Builder setPostalCode(EmptyParam postalCode) {
this.postalCode = postalCode;
return this;
}
/** State, county, province, or region. */
public Builder setState(String state) {
this.state = state;
return this;
}
public Builder setState(EmptyParam state) {
this.state = state;
return this;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getCity() {
return this.city;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getCountry() {
return this.country;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getLine1() {
return this.line1;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getLine2() {
return this.line2;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getPostalCode() {
return this.postalCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getState() {
return this.state;
}
}
/**
* Shipping address.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Address getAddress() {
return this.address;
}
/**
* The delivery service that shipped a physical product, such as Fedex, UPS, USPS, etc.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getCarrier() {
return this.carrier;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Recipient name.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getName() {
return this.name;
}
/**
* Recipient phone (including extension).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getPhone() {
return this.phone;
}
/**
* The tracking number for a physical product, obtained from the delivery service. If multiple
* tracking numbers were generated for this purchase, please separate them with commas.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getTrackingNumber() {
return this.trackingNumber;
}
}
/**
* State, county, province, or region.
*/
public static class TransferData {
/**
* The amount that will be transferred automatically when a charge succeeds.
*/
@SerializedName("amount")
Long amount;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
private TransferData(Long amount, Map extraParams) {
this.amount = amount;
this.extraParams = extraParams;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Long amount;
private Map extraParams;
/** Finalize and obtain parameter instance from this builder. */
public TransferData build() {
return new TransferData(this.amount, this.extraParams);
}
/** The amount that will be transferred automatically when a charge succeeds. */
public Builder setAmount(Long amount) {
this.amount = amount;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* PaymentIntentUpdateParams.TransferData#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
}
/**
* The amount that will be transferred automatically when a charge succeeds.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link PaymentIntentUpdateParams.TransferData#extraParams} for the field documentation.
*/
public enum SetupFutureUsage implements ApiRequestParams.EnumParam {
@SerializedName("off_session")
OFF_SESSION("off_session"), @SerializedName("on_session")
ON_SESSION("on_session");
private final String value;
SetupFutureUsage(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
/**
* Amount intended to be collected by this PaymentIntent. A positive integer representing how much
* to charge in the smallest currency
* unit (e.g., 100 cents to charge $1.00 or 100 to charge ¥100, a zero-decimal currency). The
* minimum amount is $0.50 US or equivalent in
* charge currency. The amount value supports up to eight digits (e.g., a value of 99999999
* for a USD charge of $999,999.99).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
/**
* The amount of the application fee (if any) for the resulting payment. See the PaymentIntents use case for connected accounts
* for details.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getApplicationFeeAmount() {
return this.applicationFeeAmount;
}
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getCurrency() {
return this.currency;
}
/**
* ID of the Customer this PaymentIntent belongs to, if one exists.
*
* Payment methods attached to other Customers cannot be used with this PaymentIntent.
*
*
If present in combination with setup_future_usage,
* this PaymentIntent's payment method will be attached to the Customer after the PaymentIntent
* has been confirmed and any required actions from the user are complete.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getCustomer() {
return this.customer;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getDescription() {
return this.description;
}
/**
* Specifies which fields in the response should be expanded.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getExpand() {
return this.expand;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format. Individual keys can be unset by
* posting an empty value to them. All keys can be unset by posting an empty value to {@code
* metadata}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getMetadata() {
return this.metadata;
}
/**
* ID of the payment method (a PaymentMethod, Card, or compatible Source
* object) to attach to this PaymentIntent.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getPaymentMethod() {
return this.paymentMethod;
}
/**
* Payment-method-specific configuration for this PaymentIntent.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PaymentMethodOptions getPaymentMethodOptions() {
return this.paymentMethodOptions;
}
/**
* The list of payment method types (e.g. card) that this PaymentIntent is allowed to use.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getPaymentMethodTypes() {
return this.paymentMethodTypes;
}
/**
* Email address that the receipt for the resulting payment will be sent to.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getReceiptEmail() {
return this.receiptEmail;
}
/**
* If the PaymentIntent has a {@code payment_method} and a {@code customer} or if you're attaching
* a payment method to the PaymentIntent in this request, you can pass {@code
* save_payment_method=true} to save the payment method to the customer immediately.
*
* If the payment method is already saved to a customer, this parameter does nothing. If this
* type of payment method cannot be saved to a customer, the request will error.
*
*
Saving a payment method using this parameter is not recommended because it will
* save the payment method even if it cannot be charged (e.g. the user made a typo). To ensure
* that only payment methods which are likely to be chargeable are saved to a customer, use the
* (setup_future_usage)[#payment_intents/object#payment_intent_object-setup_future_usage]
* property, which saves the payment method after the PaymentIntent has been confirmed and all
* required actions by the customer are complete.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getSavePaymentMethod() {
return this.savePaymentMethod;
}
/**
* Indicates that you intend to make future payments with this PaymentIntent's payment method.
*
*
Providing this parameter will attach the payment method to
* the PaymentIntent's Customer, if present, after the PaymentIntent is confirmed and any required
* actions from the user are complete. If no Customer was provided, the payment method can still
* be attached to a Customer
* after the transaction completes.
*
*
When processing card payments, Stripe also uses {@code setup_future_usage} to dynamically
* optimize your payment flow and comply with regional legislation and network rules, such as SCA.
*
*
If {@code setup_future_usage} is already set and you are performing a request using a
* publishable key, you may only update the value from {@code on_session} to {@code off_session}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public EnumParam getSetupFutureUsage() {
return this.setupFutureUsage;
}
/**
* Shipping information for this PaymentIntent.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getShipping() {
return this.shipping;
}
/**
* This is a legacy field that will be removed in the future. It is the ID of the Source object to
* attach to this PaymentIntent. Please use the {@code payment_method} field instead, which also
* supports Cards and compatible Source
* objects.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getSource() {
return this.source;
}
/**
* For non-card charges, you can use this value as the complete description that appears on your
* customers’ statements. Must contain at least one letter, maximum 22 characters.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getStatementDescriptor() {
return this.statementDescriptor;
}
/**
* Provides information about a card payment that customers see on their statements. Concatenated
* with the prefix (shortened descriptor) or statement descriptor that’s set on the account to
* form the complete statement descriptor. Maximum 22 characters for the concatenated descriptor.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getStatementDescriptorSuffix() {
return this.statementDescriptorSuffix;
}
/**
* The parameters used to automatically create a Transfer when the payment succeeds. For more
* information, see the PaymentIntents use case for connected accounts.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TransferData getTransferData() {
return this.transferData;
}
/**
* A string that identifies the resulting payment as part of a group. {@code transfer_group} may
* only be provided if it has not been set. See the PaymentIntents use case for connected accounts
* for details.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getTransferGroup() {
return this.transferGroup;
}
}