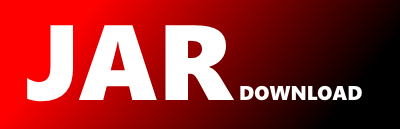
com.stripe.param.SetupIntentConfirmParams Maven / Gradle / Ivy
// Generated by delombok at Wed Jun 10 17:29:55 PDT 2020
package com.stripe.param;
import com.google.gson.annotations.SerializedName;
import com.stripe.net.ApiRequestParams;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class SetupIntentConfirmParams extends ApiRequestParams {
/**
* Specifies which fields in the response should be expanded.
*/
@SerializedName("expand")
List expand;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* This hash contains details about the Mandate to create.
*/
@SerializedName("mandate_data")
Object mandateData;
/**
* ID of the payment method (a PaymentMethod, Card, or saved Source object) to attach to this
* SetupIntent.
*/
@SerializedName("payment_method")
String paymentMethod;
/**
* Payment-method-specific configuration for this SetupIntent.
*/
@SerializedName("payment_method_options")
PaymentMethodOptions paymentMethodOptions;
/**
* The URL to redirect your customer back to after they authenticate on the payment method's app
* or site. If you'd prefer to redirect to a mobile application, you can alternatively supply an
* application URI scheme. This parameter is only used for cards and other redirect-based payment
* methods.
*/
@SerializedName("return_url")
String returnUrl;
private SetupIntentConfirmParams(List expand, Map extraParams, Object mandateData, String paymentMethod, PaymentMethodOptions paymentMethodOptions, String returnUrl) {
this.expand = expand;
this.extraParams = extraParams;
this.mandateData = mandateData;
this.paymentMethod = paymentMethod;
this.paymentMethodOptions = paymentMethodOptions;
this.returnUrl = returnUrl;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private List expand;
private Map extraParams;
private Object mandateData;
private String paymentMethod;
private PaymentMethodOptions paymentMethodOptions;
private String returnUrl;
/**
* Finalize and obtain parameter instance from this builder.
*/
public SetupIntentConfirmParams build() {
return new SetupIntentConfirmParams(this.expand, this.extraParams, this.mandateData, this.paymentMethod, this.paymentMethodOptions, this.returnUrl);
}
/**
* Add an element to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SetupIntentConfirmParams#expand} for the field documentation.
*/
public Builder addExpand(String element) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.add(element);
return this;
}
/**
* Add all elements to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SetupIntentConfirmParams#expand} for the field documentation.
*/
public Builder addAllExpand(List elements) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.addAll(elements);
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SetupIntentConfirmParams#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SetupIntentConfirmParams#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* This hash contains details about the Mandate to create.
*/
public Builder setMandateData(MandateData mandateData) {
this.mandateData = mandateData;
return this;
}
/**
* ID of the payment method (a PaymentMethod, Card, or saved Source object) to attach to this
* SetupIntent.
*/
public Builder setPaymentMethod(String paymentMethod) {
this.paymentMethod = paymentMethod;
return this;
}
/**
* Payment-method-specific configuration for this SetupIntent.
*/
public Builder setPaymentMethodOptions(PaymentMethodOptions paymentMethodOptions) {
this.paymentMethodOptions = paymentMethodOptions;
return this;
}
/**
* The URL to redirect your customer back to after they authenticate on the payment method's app
* or site. If you'd prefer to redirect to a mobile application, you can alternatively supply an
* application URI scheme. This parameter is only used for cards and other redirect-based
* payment methods.
*/
public Builder setReturnUrl(String returnUrl) {
this.returnUrl = returnUrl;
return this;
}
}
public static class MandateData {
/**
* This hash contains details about the customer acceptance of the Mandate.
*/
@SerializedName("customer_acceptance")
CustomerAcceptance customerAcceptance;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
private MandateData(CustomerAcceptance customerAcceptance, Map extraParams) {
this.customerAcceptance = customerAcceptance;
this.extraParams = extraParams;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private CustomerAcceptance customerAcceptance;
private Map extraParams;
/**
* Finalize and obtain parameter instance from this builder.
*/
public MandateData build() {
return new MandateData(this.customerAcceptance, this.extraParams);
}
/**
* This hash contains details about the customer acceptance of the Mandate.
*/
public Builder setCustomerAcceptance(CustomerAcceptance customerAcceptance) {
this.customerAcceptance = customerAcceptance;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SetupIntentConfirmParams.MandateData#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SetupIntentConfirmParams.MandateData#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
}
public static class CustomerAcceptance {
/** The time at which the customer accepted the Mandate. */
@SerializedName("accepted_at")
Long acceptedAt;
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* If this is a Mandate accepted offline, this hash contains details about the offline
* acceptance.
*/
@SerializedName("offline")
Offline offline;
/**
* If this is a Mandate accepted online, this hash contains details about the online
* acceptance.
*/
@SerializedName("online")
Online online;
/**
* The type of customer acceptance information included with the Mandate. One of {@code
* online} or {@code offline}.
*/
@SerializedName("type")
Type type;
private CustomerAcceptance(Long acceptedAt, Map extraParams, Offline offline, Online online, Type type) {
this.acceptedAt = acceptedAt;
this.extraParams = extraParams;
this.offline = offline;
this.online = online;
this.type = type;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Long acceptedAt;
private Map extraParams;
private Offline offline;
private Online online;
private Type type;
/** Finalize and obtain parameter instance from this builder. */
public CustomerAcceptance build() {
return new CustomerAcceptance(this.acceptedAt, this.extraParams, this.offline, this.online, this.type);
}
/** The time at which the customer accepted the Mandate. */
public Builder setAcceptedAt(Long acceptedAt) {
this.acceptedAt = acceptedAt;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SetupIntentConfirmParams.MandateData.CustomerAcceptance#extraParams} for
* the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SetupIntentConfirmParams.MandateData.CustomerAcceptance#extraParams} for
* the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* If this is a Mandate accepted offline, this hash contains details about the offline
* acceptance.
*/
public Builder setOffline(Offline offline) {
this.offline = offline;
return this;
}
/**
* If this is a Mandate accepted online, this hash contains details about the online
* acceptance.
*/
public Builder setOnline(Online online) {
this.online = online;
return this;
}
/**
* The type of customer acceptance information included with the Mandate. One of {@code
* online} or {@code offline}.
*/
public Builder setType(Type type) {
this.type = type;
return this;
}
}
public static class Offline {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field
* (serialized) name in this param object. Effectively, this map is flattened to its parent
* instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
private Offline(Map extraParams) {
this.extraParams = extraParams;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
/** Finalize and obtain parameter instance from this builder. */
public Offline build() {
return new Offline(this.extraParams);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* SetupIntentConfirmParams.MandateData.CustomerAcceptance.Offline#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* SetupIntentConfirmParams.MandateData.CustomerAcceptance.Offline#extraParams} for the
* field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
}
public static class Online {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field
* (serialized) name in this param object. Effectively, this map is flattened to its parent
* instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/** The IP address from which the Mandate was accepted by the customer. */
@SerializedName("ip_address")
String ipAddress;
/** The user agent of the browser from which the Mandate was accepted by the customer. */
@SerializedName("user_agent")
String userAgent;
private Online(Map extraParams, String ipAddress, String userAgent) {
this.extraParams = extraParams;
this.ipAddress = ipAddress;
this.userAgent = userAgent;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private String ipAddress;
private String userAgent;
/** Finalize and obtain parameter instance from this builder. */
public Online build() {
return new Online(this.extraParams, this.ipAddress, this.userAgent);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* SetupIntentConfirmParams.MandateData.CustomerAcceptance.Online#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* SetupIntentConfirmParams.MandateData.CustomerAcceptance.Online#extraParams} for the
* field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/** The IP address from which the Mandate was accepted by the customer. */
public Builder setIpAddress(String ipAddress) {
this.ipAddress = ipAddress;
return this;
}
public Builder setUserAgent(String userAgent) {
this.userAgent = userAgent;
return this;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getIpAddress() {
return this.ipAddress;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUserAgent() {
return this.userAgent;
}
}
public enum Type implements ApiRequestParams.EnumParam {
@SerializedName("offline")
OFFLINE("offline"), @SerializedName("online")
ONLINE("online");
private final String value;
Type(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAcceptedAt() {
return this.acceptedAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Offline getOffline() {
return this.offline;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Online getOnline() {
return this.online;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Type getType() {
return this.type;
}
}
/**
* This hash contains details about the customer acceptance of the Mandate.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public CustomerAcceptance getCustomerAcceptance() {
return this.customerAcceptance;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
}
/**
* The user agent of the browser from which the Mandate was accepted by the customer.
*/
public static class PaymentMethodOptions {
/** Configuration for any card setup attempted on this SetupIntent. */
@SerializedName("card")
Card card;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
private PaymentMethodOptions(Card card, Map extraParams) {
this.card = card;
this.extraParams = extraParams;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Card card;
private Map extraParams;
/** Finalize and obtain parameter instance from this builder. */
public PaymentMethodOptions build() {
return new PaymentMethodOptions(this.card, this.extraParams);
}
/** Configuration for any card setup attempted on this SetupIntent. */
public Builder setCard(Card card) {
this.card = card;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SetupIntentConfirmParams.PaymentMethodOptions#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SetupIntentConfirmParams.PaymentMethodOptions#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
}
public static class Card {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* When specified, this parameter signals that a card has been collected as MOTO (Mail Order
* Telephone Order) and thus out of scope for SCA. This parameter can only be provided during
* confirmation.
*/
@SerializedName("moto")
Boolean moto;
/**
* We strongly recommend that you rely on our SCA Engine to automatically prompt your
* customers for authentication based on risk level and other requirements.
* However, if you wish to request 3D Secure based on logic from your own fraud engine,
* provide this option. Permitted values include: {@code automatic} or {@code any}. If not
* provided, defaults to {@code automatic}. Read our guide on manually requesting 3D
* Secure for more information on how this configuration interacts with Radar and our SCA
* Engine.
*/
@SerializedName("request_three_d_secure")
RequestThreeDSecure requestThreeDSecure;
private Card(Map extraParams, Boolean moto, RequestThreeDSecure requestThreeDSecure) {
this.extraParams = extraParams;
this.moto = moto;
this.requestThreeDSecure = requestThreeDSecure;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private Boolean moto;
private RequestThreeDSecure requestThreeDSecure;
/** Finalize and obtain parameter instance from this builder. */
public Card build() {
return new Card(this.extraParams, this.moto, this.requestThreeDSecure);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SetupIntentConfirmParams.PaymentMethodOptions.Card#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SetupIntentConfirmParams.PaymentMethodOptions.Card#extraParams} for the
* field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* When specified, this parameter signals that a card has been collected as MOTO (Mail Order
* Telephone Order) and thus out of scope for SCA. This parameter can only be provided
* during confirmation.
*/
public Builder setMoto(Boolean moto) {
this.moto = moto;
return this;
}
/**
* We strongly recommend that you rely on our SCA Engine to automatically prompt your
* customers for authentication based on risk level and other requirements.
* However, if you wish to request 3D Secure based on logic from your own fraud engine,
* provide this option. Permitted values include: {@code automatic} or {@code any}. If not
* provided, defaults to {@code automatic}. Read our guide on manually requesting 3D
* Secure for more information on how this configuration interacts with Radar and our
* SCA Engine.
*/
public Builder setRequestThreeDSecure(RequestThreeDSecure requestThreeDSecure) {
this.requestThreeDSecure = requestThreeDSecure;
return this;
}
}
public enum RequestThreeDSecure implements ApiRequestParams.EnumParam {
@SerializedName("any")
ANY("any"), @SerializedName("automatic")
AUTOMATIC("automatic");
private final String value;
RequestThreeDSecure(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getMoto() {
return this.moto;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public RequestThreeDSecure getRequestThreeDSecure() {
return this.requestThreeDSecure;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Card getCard() {
return this.card;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
}
/**
* Specifies which fields in the response should be expanded.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getExpand() {
return this.expand;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* This hash contains details about the Mandate to create.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getMandateData() {
return this.mandateData;
}
/**
* ID of the payment method (a PaymentMethod, Card, or saved Source object) to attach to this
* SetupIntent.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPaymentMethod() {
return this.paymentMethod;
}
/**
* Payment-method-specific configuration for this SetupIntent.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PaymentMethodOptions getPaymentMethodOptions() {
return this.paymentMethodOptions;
}
/**
* The URL to redirect your customer back to after they authenticate on the payment method's app
* or site. If you'd prefer to redirect to a mobile application, you can alternatively supply an
* application URI scheme. This parameter is only used for cards and other redirect-based payment
* methods.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReturnUrl() {
return this.returnUrl;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy