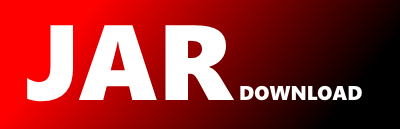
com.stripe.model.Balance Maven / Gradle / Ivy
// Generated by delombok at Wed Aug 05 16:28:33 PDT 2020
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.BalanceRetrieveParams;
import java.util.List;
import java.util.Map;
public class Balance extends ApiResource {
/**
* Funds that are available to be transferred or paid out, whether automatically by Stripe or
* explicitly via the Transfers API or Payouts API. The available balance for each
* currency and payment type can be found in the {@code source_types} property.
*/
@SerializedName("available")
List available;
/**
* Funds held due to negative balances on connected Custom accounts. The connect reserve balance
* for each currency and payment type can be found in the {@code source_types} property.
*/
@SerializedName("connect_reserved")
List connectReserved;
@SerializedName("issuing")
Details issuing;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code balance}.
*/
@SerializedName("object")
String object;
/**
* Funds that are not yet available in the balance, due to the 7-day rolling pay cycle. The
* pending balance for each currency, and for each payment type, can be found in the {@code
* source_types} property.
*/
@SerializedName("pending")
List pending;
/**
* Retrieves the current account balance, based on the authentication that was used to make the
* request. For a sample request, see Accounting
* for negative balances.
*/
public static Balance retrieve() throws StripeException {
return retrieve((Map) null, (RequestOptions) null);
}
/**
* Retrieves the current account balance, based on the authentication that was used to make the
* request. For a sample request, see Accounting
* for negative balances.
*/
public static Balance retrieve(RequestOptions options) throws StripeException {
return retrieve((Map) null, options);
}
/**
* Retrieves the current account balance, based on the authentication that was used to make the
* request. For a sample request, see Accounting
* for negative balances.
*/
public static Balance retrieve(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/balance");
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Balance.class, options);
}
/**
* Retrieves the current account balance, based on the authentication that was used to make the
* request. For a sample request, see Accounting
* for negative balances.
*/
public static Balance retrieve(BalanceRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/balance");
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Balance.class, options);
}
public static class Details extends StripeObject {
/**
* Funds that are available for use.
*/
@SerializedName("available")
List available;
/**
* Funds that are available for use.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getAvailable() {
return this.available;
}
/**
* Funds that are available for use.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAvailable(final List available) {
this.available = available;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Balance.Details)) return false;
final Balance.Details other = (Balance.Details) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$available = this.getAvailable();
final java.lang.Object other$available = other.getAvailable();
if (this$available == null ? other$available != null : !this$available.equals(other$available)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Balance.Details;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $available = this.getAvailable();
result = result * PRIME + ($available == null ? 43 : $available.hashCode());
return result;
}
}
public static class Money extends StripeObject {
/** Balance amount. */
@SerializedName("amount")
Long amount;
/**
* Three-letter ISO currency
* code, in lowercase. Must be a supported
* currency.
*/
@SerializedName("currency")
String currency;
@SerializedName("source_types")
SourceTypes sourceTypes;
public static class SourceTypes extends StripeObject {
/** Amount for bank account. */
@SerializedName("bank_account")
Long bankAccount;
/** Amount for card. */
@SerializedName("card")
Long card;
/** Amount for FPX. */
@SerializedName("fpx")
Long fpx;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getBankAccount() {
return this.bankAccount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCard() {
return this.card;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getFpx() {
return this.fpx;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBankAccount(final Long bankAccount) {
this.bankAccount = bankAccount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCard(final Long card) {
this.card = card;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFpx(final Long fpx) {
this.fpx = fpx;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Balance.Money.SourceTypes)) return false;
final Balance.Money.SourceTypes other = (Balance.Money.SourceTypes) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$bankAccount = this.getBankAccount();
final java.lang.Object other$bankAccount = other.getBankAccount();
if (this$bankAccount == null ? other$bankAccount != null : !this$bankAccount.equals(other$bankAccount)) return false;
final java.lang.Object this$card = this.getCard();
final java.lang.Object other$card = other.getCard();
if (this$card == null ? other$card != null : !this$card.equals(other$card)) return false;
final java.lang.Object this$fpx = this.getFpx();
final java.lang.Object other$fpx = other.getFpx();
if (this$fpx == null ? other$fpx != null : !this$fpx.equals(other$fpx)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Balance.Money.SourceTypes;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $bankAccount = this.getBankAccount();
result = result * PRIME + ($bankAccount == null ? 43 : $bankAccount.hashCode());
final java.lang.Object $card = this.getCard();
result = result * PRIME + ($card == null ? 43 : $card.hashCode());
final java.lang.Object $fpx = this.getFpx();
result = result * PRIME + ($fpx == null ? 43 : $fpx.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SourceTypes getSourceTypes() {
return this.sourceTypes;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmount(final Long amount) {
this.amount = amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSourceTypes(final SourceTypes sourceTypes) {
this.sourceTypes = sourceTypes;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Balance.Money)) return false;
final Balance.Money other = (Balance.Money) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
final java.lang.Object this$sourceTypes = this.getSourceTypes();
final java.lang.Object other$sourceTypes = other.getSourceTypes();
if (this$sourceTypes == null ? other$sourceTypes != null : !this$sourceTypes.equals(other$sourceTypes)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Balance.Money;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
final java.lang.Object $sourceTypes = this.getSourceTypes();
result = result * PRIME + ($sourceTypes == null ? 43 : $sourceTypes.hashCode());
return result;
}
}
/**
* Funds that are available to be transferred or paid out, whether automatically by Stripe or
* explicitly via the Transfers API or Payouts API. The available balance for each
* currency and payment type can be found in the {@code source_types} property.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getAvailable() {
return this.available;
}
/**
* Funds held due to negative balances on connected Custom accounts. The connect reserve balance
* for each currency and payment type can be found in the {@code source_types} property.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getConnectReserved() {
return this.connectReserved;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Details getIssuing() {
return this.issuing;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code balance}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* Funds that are not yet available in the balance, due to the 7-day rolling pay cycle. The
* pending balance for each currency, and for each payment type, can be found in the {@code
* source_types} property.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getPending() {
return this.pending;
}
/**
* Funds that are available to be transferred or paid out, whether automatically by Stripe or
* explicitly via the Transfers API or Payouts API. The available balance for each
* currency and payment type can be found in the {@code source_types} property.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAvailable(final List available) {
this.available = available;
}
/**
* Funds held due to negative balances on connected Custom accounts. The connect reserve balance
* for each currency and payment type can be found in the {@code source_types} property.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setConnectReserved(final List connectReserved) {
this.connectReserved = connectReserved;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIssuing(final Details issuing) {
this.issuing = issuing;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code balance}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* Funds that are not yet available in the balance, due to the 7-day rolling pay cycle. The
* pending balance for each currency, and for each payment type, can be found in the {@code
* source_types} property.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPending(final List pending) {
this.pending = pending;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Balance)) return false;
final Balance other = (Balance) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$available = this.getAvailable();
final java.lang.Object other$available = other.getAvailable();
if (this$available == null ? other$available != null : !this$available.equals(other$available)) return false;
final java.lang.Object this$connectReserved = this.getConnectReserved();
final java.lang.Object other$connectReserved = other.getConnectReserved();
if (this$connectReserved == null ? other$connectReserved != null : !this$connectReserved.equals(other$connectReserved)) return false;
final java.lang.Object this$issuing = this.getIssuing();
final java.lang.Object other$issuing = other.getIssuing();
if (this$issuing == null ? other$issuing != null : !this$issuing.equals(other$issuing)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$pending = this.getPending();
final java.lang.Object other$pending = other.getPending();
if (this$pending == null ? other$pending != null : !this$pending.equals(other$pending)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Balance;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $available = this.getAvailable();
result = result * PRIME + ($available == null ? 43 : $available.hashCode());
final java.lang.Object $connectReserved = this.getConnectReserved();
result = result * PRIME + ($connectReserved == null ? 43 : $connectReserved.hashCode());
final java.lang.Object $issuing = this.getIssuing();
result = result * PRIME + ($issuing == null ? 43 : $issuing.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $pending = this.getPending();
result = result * PRIME + ($pending == null ? 43 : $pending.hashCode());
return result;
}
}