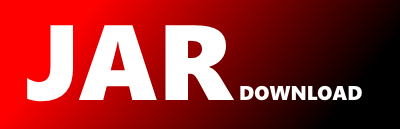
com.stripe.model.PromotionCode Maven / Gradle / Ivy
// Generated by delombok at Wed Aug 05 16:28:33 PDT 2020
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.PromotionCodeCreateParams;
import com.stripe.param.PromotionCodeListParams;
import com.stripe.param.PromotionCodeRetrieveParams;
import com.stripe.param.PromotionCodeUpdateParams;
import java.util.Map;
public class PromotionCode extends ApiResource implements HasId, MetadataStore {
/**
* Whether the promotion code is currently active. A promotion code is only active if the coupon
* is also valid.
*/
@SerializedName("active")
Boolean active;
/**
* The customer-facing code. Regardless of case, this code must be unique across all active
* promotion codes for each customer.
*/
@SerializedName("code")
String code;
/**
* A coupon contains information about a percent-off or amount-off discount you might want to
* apply to a customer. Coupons may be applied to invoices or orders. Coupons do not work with
* conventional one-off charges.
*/
@SerializedName("coupon")
Coupon coupon;
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* The customer that this promotion code can be used by.
*/
@SerializedName("customer")
ExpandableField customer;
/**
* Date at which the promotion code can no longer be redeemed.
*/
@SerializedName("expires_at")
Long expiresAt;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* Maximum number of times this promotion code can be redeemed.
*/
@SerializedName("max_redemptions")
Long maxRedemptions;
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code promotion_code}.
*/
@SerializedName("object")
String object;
@SerializedName("restrictions")
Restrictions restrictions;
/**
* Number of times this promotion code has been used.
*/
@SerializedName("times_redeemed")
Long timesRedeemed;
/**
* Get ID of expandable {@code customer} object.
*/
public String getCustomer() {
return (this.customer != null) ? this.customer.getId() : null;
}
public void setCustomer(String id) {
this.customer = ApiResource.setExpandableFieldId(id, this.customer);
}
/**
* Get expanded {@code customer}.
*/
public Customer getCustomerObject() {
return (this.customer != null) ? this.customer.getExpanded() : null;
}
public void setCustomerObject(Customer expandableObject) {
this.customer = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Retrieves the promotion code with the given ID.
*/
public static PromotionCode retrieve(String promotionCode) throws StripeException {
return retrieve(promotionCode, (Map) null, (RequestOptions) null);
}
/**
* Retrieves the promotion code with the given ID.
*/
public static PromotionCode retrieve(String promotionCode, RequestOptions options) throws StripeException {
return retrieve(promotionCode, (Map) null, options);
}
/**
* Retrieves the promotion code with the given ID.
*/
public static PromotionCode retrieve(String promotionCode, Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/promotion_codes/%s", ApiResource.urlEncodeId(promotionCode)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, PromotionCode.class, options);
}
/**
* Retrieves the promotion code with the given ID.
*/
public static PromotionCode retrieve(String promotionCode, PromotionCodeRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/promotion_codes/%s", ApiResource.urlEncodeId(promotionCode)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, PromotionCode.class, options);
}
/**
* A promotion code points to a coupon. You can optionally restrict the code to a specific
* customer, redemption limit, and expiration date.
*/
public static PromotionCode create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* A promotion code points to a coupon. You can optionally restrict the code to a specific
* customer, redemption limit, and expiration date.
*/
public static PromotionCode create(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/promotion_codes");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, PromotionCode.class, options);
}
/**
* A promotion code points to a coupon. You can optionally restrict the code to a specific
* customer, redemption limit, and expiration date.
*/
public static PromotionCode create(PromotionCodeCreateParams params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* A promotion code points to a coupon. You can optionally restrict the code to a specific
* customer, redemption limit, and expiration date.
*/
public static PromotionCode create(PromotionCodeCreateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/promotion_codes");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, PromotionCode.class, options);
}
/**
* Updates the specified promotion code by setting the values of the parameters passed. Most
* fields are, by design, not editable.
*/
@Override
public PromotionCode update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates the specified promotion code by setting the values of the parameters passed. Most
* fields are, by design, not editable.
*/
@Override
public PromotionCode update(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/promotion_codes/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, PromotionCode.class, options);
}
/**
* Updates the specified promotion code by setting the values of the parameters passed. Most
* fields are, by design, not editable.
*/
public PromotionCode update(PromotionCodeUpdateParams params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates the specified promotion code by setting the values of the parameters passed. Most
* fields are, by design, not editable.
*/
public PromotionCode update(PromotionCodeUpdateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/promotion_codes/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, PromotionCode.class, options);
}
/**
* Returns a list of your promotion codes.
*/
public static PromotionCodeCollection list(Map params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of your promotion codes.
*/
public static PromotionCodeCollection list(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/promotion_codes");
return ApiResource.requestCollection(url, params, PromotionCodeCollection.class, options);
}
/**
* Returns a list of your promotion codes.
*/
public static PromotionCodeCollection list(PromotionCodeListParams params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of your promotion codes.
*/
public static PromotionCodeCollection list(PromotionCodeListParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/promotion_codes");
return ApiResource.requestCollection(url, params, PromotionCodeCollection.class, options);
}
public static class Restrictions extends StripeObject {
/**
* A Boolean indicating if the Promotion Code should only be redeemed for Customers without any
* successful payments or invoices.
*/
@SerializedName("first_time_transaction")
Boolean firstTimeTransaction;
/**
* Minimum amount required to redeem this Promotion Code into a Coupon (e.g., a purchase must be
* $100 or more to work).
*/
@SerializedName("minimum_amount")
Long minimumAmount;
/** Three-letter ISO code for minimum_amount */
@SerializedName("minimum_amount_currency")
String minimumAmountCurrency;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getFirstTimeTransaction() {
return this.firstTimeTransaction;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getMinimumAmount() {
return this.minimumAmount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getMinimumAmountCurrency() {
return this.minimumAmountCurrency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFirstTimeTransaction(final Boolean firstTimeTransaction) {
this.firstTimeTransaction = firstTimeTransaction;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMinimumAmount(final Long minimumAmount) {
this.minimumAmount = minimumAmount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMinimumAmountCurrency(final String minimumAmountCurrency) {
this.minimumAmountCurrency = minimumAmountCurrency;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PromotionCode.Restrictions)) return false;
final PromotionCode.Restrictions other = (PromotionCode.Restrictions) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$firstTimeTransaction = this.getFirstTimeTransaction();
final java.lang.Object other$firstTimeTransaction = other.getFirstTimeTransaction();
if (this$firstTimeTransaction == null ? other$firstTimeTransaction != null : !this$firstTimeTransaction.equals(other$firstTimeTransaction)) return false;
final java.lang.Object this$minimumAmount = this.getMinimumAmount();
final java.lang.Object other$minimumAmount = other.getMinimumAmount();
if (this$minimumAmount == null ? other$minimumAmount != null : !this$minimumAmount.equals(other$minimumAmount)) return false;
final java.lang.Object this$minimumAmountCurrency = this.getMinimumAmountCurrency();
final java.lang.Object other$minimumAmountCurrency = other.getMinimumAmountCurrency();
if (this$minimumAmountCurrency == null ? other$minimumAmountCurrency != null : !this$minimumAmountCurrency.equals(other$minimumAmountCurrency)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PromotionCode.Restrictions;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $firstTimeTransaction = this.getFirstTimeTransaction();
result = result * PRIME + ($firstTimeTransaction == null ? 43 : $firstTimeTransaction.hashCode());
final java.lang.Object $minimumAmount = this.getMinimumAmount();
result = result * PRIME + ($minimumAmount == null ? 43 : $minimumAmount.hashCode());
final java.lang.Object $minimumAmountCurrency = this.getMinimumAmountCurrency();
result = result * PRIME + ($minimumAmountCurrency == null ? 43 : $minimumAmountCurrency.hashCode());
return result;
}
}
/**
* Whether the promotion code is currently active. A promotion code is only active if the coupon
* is also valid.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getActive() {
return this.active;
}
/**
* The customer-facing code. Regardless of case, this code must be unique across all active
* promotion codes for each customer.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCode() {
return this.code;
}
/**
* A coupon contains information about a percent-off or amount-off discount you might want to
* apply to a customer. Coupons may be applied to invoices or orders. Coupons do not work with
* conventional one-off charges.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Coupon getCoupon() {
return this.coupon;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
/**
* Date at which the promotion code can no longer be redeemed.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getExpiresAt() {
return this.expiresAt;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* Maximum number of times this promotion code can be redeemed.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getMaxRedemptions() {
return this.maxRedemptions;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code promotion_code}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Restrictions getRestrictions() {
return this.restrictions;
}
/**
* Number of times this promotion code has been used.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getTimesRedeemed() {
return this.timesRedeemed;
}
/**
* Whether the promotion code is currently active. A promotion code is only active if the coupon
* is also valid.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setActive(final Boolean active) {
this.active = active;
}
/**
* The customer-facing code. Regardless of case, this code must be unique across all active
* promotion codes for each customer.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCode(final String code) {
this.code = code;
}
/**
* A coupon contains information about a percent-off or amount-off discount you might want to
* apply to a customer. Coupons may be applied to invoices or orders. Coupons do not work with
* conventional one-off charges.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCoupon(final Coupon coupon) {
this.coupon = coupon;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* Date at which the promotion code can no longer be redeemed.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setExpiresAt(final Long expiresAt) {
this.expiresAt = expiresAt;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* Maximum number of times this promotion code can be redeemed.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMaxRedemptions(final Long maxRedemptions) {
this.maxRedemptions = maxRedemptions;
}
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code promotion_code}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRestrictions(final Restrictions restrictions) {
this.restrictions = restrictions;
}
/**
* Number of times this promotion code has been used.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTimesRedeemed(final Long timesRedeemed) {
this.timesRedeemed = timesRedeemed;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PromotionCode)) return false;
final PromotionCode other = (PromotionCode) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$active = this.getActive();
final java.lang.Object other$active = other.getActive();
if (this$active == null ? other$active != null : !this$active.equals(other$active)) return false;
final java.lang.Object this$code = this.getCode();
final java.lang.Object other$code = other.getCode();
if (this$code == null ? other$code != null : !this$code.equals(other$code)) return false;
final java.lang.Object this$coupon = this.getCoupon();
final java.lang.Object other$coupon = other.getCoupon();
if (this$coupon == null ? other$coupon != null : !this$coupon.equals(other$coupon)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$customer = this.getCustomer();
final java.lang.Object other$customer = other.getCustomer();
if (this$customer == null ? other$customer != null : !this$customer.equals(other$customer)) return false;
final java.lang.Object this$expiresAt = this.getExpiresAt();
final java.lang.Object other$expiresAt = other.getExpiresAt();
if (this$expiresAt == null ? other$expiresAt != null : !this$expiresAt.equals(other$expiresAt)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$maxRedemptions = this.getMaxRedemptions();
final java.lang.Object other$maxRedemptions = other.getMaxRedemptions();
if (this$maxRedemptions == null ? other$maxRedemptions != null : !this$maxRedemptions.equals(other$maxRedemptions)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$restrictions = this.getRestrictions();
final java.lang.Object other$restrictions = other.getRestrictions();
if (this$restrictions == null ? other$restrictions != null : !this$restrictions.equals(other$restrictions)) return false;
final java.lang.Object this$timesRedeemed = this.getTimesRedeemed();
final java.lang.Object other$timesRedeemed = other.getTimesRedeemed();
if (this$timesRedeemed == null ? other$timesRedeemed != null : !this$timesRedeemed.equals(other$timesRedeemed)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PromotionCode;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $active = this.getActive();
result = result * PRIME + ($active == null ? 43 : $active.hashCode());
final java.lang.Object $code = this.getCode();
result = result * PRIME + ($code == null ? 43 : $code.hashCode());
final java.lang.Object $coupon = this.getCoupon();
result = result * PRIME + ($coupon == null ? 43 : $coupon.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $customer = this.getCustomer();
result = result * PRIME + ($customer == null ? 43 : $customer.hashCode());
final java.lang.Object $expiresAt = this.getExpiresAt();
result = result * PRIME + ($expiresAt == null ? 43 : $expiresAt.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $maxRedemptions = this.getMaxRedemptions();
result = result * PRIME + ($maxRedemptions == null ? 43 : $maxRedemptions.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $restrictions = this.getRestrictions();
result = result * PRIME + ($restrictions == null ? 43 : $restrictions.hashCode());
final java.lang.Object $timesRedeemed = this.getTimesRedeemed();
result = result * PRIME + ($timesRedeemed == null ? 43 : $timesRedeemed.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
}