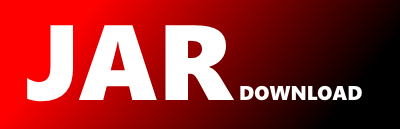
com.stripe.model.radar.EarlyFraudWarning Maven / Gradle / Ivy
// Generated by delombok at Wed Aug 05 16:28:33 PDT 2020
package com.stripe.model.radar;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.model.Charge;
import com.stripe.model.ExpandableField;
import com.stripe.model.HasId;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.radar.EarlyFraudWarningListParams;
import com.stripe.param.radar.EarlyFraudWarningRetrieveParams;
import java.util.Map;
public class EarlyFraudWarning extends ApiResource implements HasId {
/**
* An EFW is actionable if it has not received a dispute and has not been fully refunded. You may
* wish to proactively refund a charge that receives an EFW, in order to avoid receiving a dispute
* later.
*/
@SerializedName("actionable")
Boolean actionable;
/**
* ID of the charge this early fraud warning is for, optionally expanded.
*/
@SerializedName("charge")
ExpandableField charge;
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* The type of fraud labelled by the issuer. One of {@code card_never_received}, {@code
* fraudulent_card_application}, {@code made_with_counterfeit_card}, {@code made_with_lost_card},
* {@code made_with_stolen_card}, {@code misc}, {@code unauthorized_use_of_card}.
*/
@SerializedName("fraud_type")
String fraudType;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code radar.early_fraud_warning}.
*/
@SerializedName("object")
String object;
/**
* Get ID of expandable {@code charge} object.
*/
public String getCharge() {
return (this.charge != null) ? this.charge.getId() : null;
}
public void setCharge(String id) {
this.charge = ApiResource.setExpandableFieldId(id, this.charge);
}
/**
* Get expanded {@code charge}.
*/
public Charge getChargeObject() {
return (this.charge != null) ? this.charge.getExpanded() : null;
}
public void setChargeObject(Charge expandableObject) {
this.charge = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Returns a list of early fraud warnings.
*/
public static EarlyFraudWarningCollection list(Map params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of early fraud warnings.
*/
public static EarlyFraudWarningCollection list(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/radar/early_fraud_warnings");
return ApiResource.requestCollection(url, params, EarlyFraudWarningCollection.class, options);
}
/**
* Returns a list of early fraud warnings.
*/
public static EarlyFraudWarningCollection list(EarlyFraudWarningListParams params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of early fraud warnings.
*/
public static EarlyFraudWarningCollection list(EarlyFraudWarningListParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/radar/early_fraud_warnings");
return ApiResource.requestCollection(url, params, EarlyFraudWarningCollection.class, options);
}
/**
* Retrieves the details of an early fraud warning that has previously been created.
*
* Please refer to the early
* fraud warning object reference for more details.
*/
public static EarlyFraudWarning retrieve(String earlyFraudWarning) throws StripeException {
return retrieve(earlyFraudWarning, (Map) null, (RequestOptions) null);
}
/**
* Retrieves the details of an early fraud warning that has previously been created.
*
* Please refer to the early
* fraud warning object reference for more details.
*/
public static EarlyFraudWarning retrieve(String earlyFraudWarning, RequestOptions options) throws StripeException {
return retrieve(earlyFraudWarning, (Map) null, options);
}
/**
* Retrieves the details of an early fraud warning that has previously been created.
*
* Please refer to the early
* fraud warning object reference for more details.
*/
public static EarlyFraudWarning retrieve(String earlyFraudWarning, Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/radar/early_fraud_warnings/%s", ApiResource.urlEncodeId(earlyFraudWarning)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, EarlyFraudWarning.class, options);
}
/**
* Retrieves the details of an early fraud warning that has previously been created.
*
* Please refer to the early
* fraud warning object reference for more details.
*/
public static EarlyFraudWarning retrieve(String earlyFraudWarning, EarlyFraudWarningRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/radar/early_fraud_warnings/%s", ApiResource.urlEncodeId(earlyFraudWarning)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, EarlyFraudWarning.class, options);
}
/**
* An EFW is actionable if it has not received a dispute and has not been fully refunded. You may
* wish to proactively refund a charge that receives an EFW, in order to avoid receiving a dispute
* later.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getActionable() {
return this.actionable;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
/**
* The type of fraud labelled by the issuer. One of {@code card_never_received}, {@code
* fraudulent_card_application}, {@code made_with_counterfeit_card}, {@code made_with_lost_card},
* {@code made_with_stolen_card}, {@code misc}, {@code unauthorized_use_of_card}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFraudType() {
return this.fraudType;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code radar.early_fraud_warning}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* An EFW is actionable if it has not received a dispute and has not been fully refunded. You may
* wish to proactively refund a charge that receives an EFW, in order to avoid receiving a dispute
* later.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setActionable(final Boolean actionable) {
this.actionable = actionable;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* The type of fraud labelled by the issuer. One of {@code card_never_received}, {@code
* fraudulent_card_application}, {@code made_with_counterfeit_card}, {@code made_with_lost_card},
* {@code made_with_stolen_card}, {@code misc}, {@code unauthorized_use_of_card}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFraudType(final String fraudType) {
this.fraudType = fraudType;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code radar.early_fraud_warning}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof EarlyFraudWarning)) return false;
final EarlyFraudWarning other = (EarlyFraudWarning) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$actionable = this.getActionable();
final java.lang.Object other$actionable = other.getActionable();
if (this$actionable == null ? other$actionable != null : !this$actionable.equals(other$actionable)) return false;
final java.lang.Object this$charge = this.getCharge();
final java.lang.Object other$charge = other.getCharge();
if (this$charge == null ? other$charge != null : !this$charge.equals(other$charge)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$fraudType = this.getFraudType();
final java.lang.Object other$fraudType = other.getFraudType();
if (this$fraudType == null ? other$fraudType != null : !this$fraudType.equals(other$fraudType)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof EarlyFraudWarning;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $actionable = this.getActionable();
result = result * PRIME + ($actionable == null ? 43 : $actionable.hashCode());
final java.lang.Object $charge = this.getCharge();
result = result * PRIME + ($charge == null ? 43 : $charge.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $fraudType = this.getFraudType();
result = result * PRIME + ($fraudType == null ? 43 : $fraudType.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
}