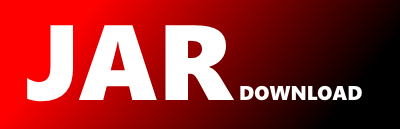
com.stripe.net.StripeRequest Maven / Gradle / Ivy
// Generated by delombok at Wed Aug 05 16:28:32 PDT 2020
package com.stripe.net;
import com.stripe.Stripe;
import com.stripe.exception.ApiConnectionException;
import com.stripe.exception.AuthenticationException;
import com.stripe.exception.StripeException;
import com.stripe.util.StringUtils;
import java.io.IOException;
import java.net.URL;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.UUID;
/**
* A request to Stripe's API.
*/
public final class StripeRequest {
/**
* The HTTP method for the request (GET, POST or DELETE).
*/
private final ApiResource.RequestMethod method;
/**
* The URL for the request. If this is a GET or DELETE request, the URL also includes the request
* parameters in its query string.
*/
private final URL url;
/**
* The body of the request. For POST requests, this will be either a {@code
* application/x-www-form-urlencoded} or a {@code multipart/form-data} payload. For non-POST
* requests, this will be {@code null}.
*/
private final HttpContent content;
/**
* The HTTP headers of the request ({@code Authorization}, {@code Stripe-Version}, {@code
* Stripe-Account}, {@code Idempotency-Key}...).
*/
private final HttpHeaders headers;
/**
* The parameters of the request (as an unmodifiable map).
*/
private final Map params;
/**
* The special modifiers of the request.
*/
private final RequestOptions options;
/**
* Initializes a new instance of the {@link StripeRequest} class.
*
* @param method the HTTP method
* @param url the URL of the request
* @param params the parameters of the request
* @param options the special modifiers of the request
* @throws StripeException if the request cannot be initialized for any reason
*/
public StripeRequest(ApiResource.RequestMethod method, String url, Map params, RequestOptions options) throws StripeException {
try {
this.params = (params != null) ? Collections.unmodifiableMap(params) : null;
this.options = (options != null) ? options : RequestOptions.getDefault();
this.method = method;
this.url = buildURL(method, url, params);
this.content = buildContent(method, params);
this.headers = buildHeaders(method, this.options);
} catch (IOException e) {
throw new ApiConnectionException(String.format("IOException during API request to Stripe (%s): %s Please check your internet connection and try again. If this problem persists,you should check Stripe\'s service status at https://twitter.com/stripestatus, or let us know at [email protected].", Stripe.getApiBase(), e.getMessage()), e);
}
}
/**
* Returns a new {@link StripeRequest} instance with an additional header.
*
* @param name the additional header's name
* @param value the additional header's value
* @return the new {@link StripeRequest} instance
*/
public StripeRequest withAdditionalHeader(String name, String value) {
return new StripeRequest(this.method, this.url, this.content, this.headers.withAdditionalHeader(name, value), this.params, this.options);
}
private static URL buildURL(ApiResource.RequestMethod method, String spec, Map params) throws IOException {
StringBuilder sb = new StringBuilder();
sb.append(spec);
if ((method != ApiResource.RequestMethod.POST) && (params != null)) {
String queryString = FormEncoder.createQueryString(params);
if (queryString != null && !queryString.isEmpty()) {
sb.append("?");
sb.append(queryString);
}
}
return new URL(sb.toString());
}
private static HttpContent buildContent(ApiResource.RequestMethod method, Map params) throws IOException {
if (method != ApiResource.RequestMethod.POST) {
return null;
}
return FormEncoder.createHttpContent(params);
}
private static HttpHeaders buildHeaders(ApiResource.RequestMethod method, RequestOptions options) throws AuthenticationException {
Map> headerMap = new HashMap>();
// Accept
headerMap.put("Accept", Arrays.asList("application/json"));
// Accept-Charset
headerMap.put("Accept-Charset", Arrays.asList(ApiResource.CHARSET.name()));
// Authorization
String apiKey = options.getApiKey();
if (apiKey == null) {
throw new AuthenticationException("No API key provided. Set your API key using `Stripe.apiKey = \"\"`. You can generate API keys from the Stripe Dashboard. See https://stripe.com/docs/api/authentication for details or contact support at https://support.stripe.com/email if you have any questions.", null, null, 0);
} else if (apiKey.isEmpty()) {
throw new AuthenticationException("Your API key is invalid, as it is an empty string. You can double-check your API key from the Stripe Dashboard. See https://stripe.com/docs/api/authentication for details or contact support at https://support.stripe.com/email if you have any questions.", null, null, 0);
} else if (StringUtils.containsWhitespace(apiKey)) {
throw new AuthenticationException("Your API key is invalid, as it contains whitespace. You can double-check your API key from the Stripe Dashboard. See https://stripe.com/docs/api/authentication for details or contact support at https://support.stripe.com/email if you have any questions.", null, null, 0);
}
headerMap.put("Authorization", Arrays.asList(String.format("Bearer %s", apiKey)));
// Stripe-Version
if (options.getStripeVersionOverride() != null) {
headerMap.put("Stripe-Version", Arrays.asList(options.getStripeVersionOverride()));
} else if (options.getStripeVersion() != null) {
headerMap.put("Stripe-Version", Arrays.asList(options.getStripeVersion()));
} else {
throw new IllegalStateException("Either `stripeVersion` or `stripeVersionOverride` value must be set.");
}
// Stripe-Account
if (options.getStripeAccount() != null) {
headerMap.put("Stripe-Account", Arrays.asList(options.getStripeAccount()));
}
// Idempotency-Key
if (options.getIdempotencyKey() != null) {
headerMap.put("Idempotency-Key", Arrays.asList(options.getIdempotencyKey()));
} else if (method == ApiResource.RequestMethod.POST) {
headerMap.put("Idempotency-Key", Arrays.asList(UUID.randomUUID().toString()));
}
return HttpHeaders.of(headerMap);
}
/**
* The HTTP method for the request (GET, POST or DELETE).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ApiResource.RequestMethod method() {
return this.method;
}
/**
* The URL for the request. If this is a GET or DELETE request, the URL also includes the request
* parameters in its query string.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public URL url() {
return this.url;
}
/**
* The body of the request. For POST requests, this will be either a {@code
* application/x-www-form-urlencoded} or a {@code multipart/form-data} payload. For non-POST
* requests, this will be {@code null}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public HttpContent content() {
return this.content;
}
/**
* The HTTP headers of the request ({@code Authorization}, {@code Stripe-Version}, {@code
* Stripe-Account}, {@code Idempotency-Key}...).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public HttpHeaders headers() {
return this.headers;
}
/**
* The parameters of the request (as an unmodifiable map).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map params() {
return this.params;
}
/**
* The special modifiers of the request.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public RequestOptions options() {
return this.options;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof StripeRequest)) return false;
final StripeRequest other = (StripeRequest) o;
final java.lang.Object this$method = this.method();
final java.lang.Object other$method = other.method();
if (this$method == null ? other$method != null : !this$method.equals(other$method)) return false;
final java.lang.Object this$url = this.url();
final java.lang.Object other$url = other.url();
if (this$url == null ? other$url != null : !this$url.equals(other$url)) return false;
final java.lang.Object this$content = this.content();
final java.lang.Object other$content = other.content();
if (this$content == null ? other$content != null : !this$content.equals(other$content)) return false;
final java.lang.Object this$headers = this.headers();
final java.lang.Object other$headers = other.headers();
if (this$headers == null ? other$headers != null : !this$headers.equals(other$headers)) return false;
final java.lang.Object this$params = this.params();
final java.lang.Object other$params = other.params();
if (this$params == null ? other$params != null : !this$params.equals(other$params)) return false;
final java.lang.Object this$options = this.options();
final java.lang.Object other$options = other.options();
if (this$options == null ? other$options != null : !this$options.equals(other$options)) return false;
return true;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $method = this.method();
result = result * PRIME + ($method == null ? 43 : $method.hashCode());
final java.lang.Object $url = this.url();
result = result * PRIME + ($url == null ? 43 : $url.hashCode());
final java.lang.Object $content = this.content();
result = result * PRIME + ($content == null ? 43 : $content.hashCode());
final java.lang.Object $headers = this.headers();
result = result * PRIME + ($headers == null ? 43 : $headers.hashCode());
final java.lang.Object $params = this.params();
result = result * PRIME + ($params == null ? 43 : $params.hashCode());
final java.lang.Object $options = this.options();
result = result * PRIME + ($options == null ? 43 : $options.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "StripeRequest(method=" + this.method() + ", url=" + this.url() + ", content=" + this.content() + ", headers=" + this.headers() + ", params=" + this.params() + ", options=" + this.options() + ")";
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected StripeRequest(final ApiResource.RequestMethod method, final URL url, final HttpContent content, final HttpHeaders headers, final Map params, final RequestOptions options) {
this.method = method;
this.url = url;
this.content = content;
this.headers = headers;
this.params = params;
this.options = options;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy