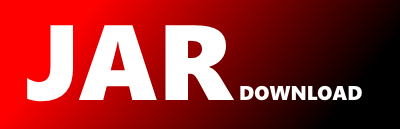
com.stripe.param.SubscriptionListParams Maven / Gradle / Ivy
// Generated by delombok at Wed Aug 05 16:28:32 PDT 2020
package com.stripe.param;
import com.google.gson.annotations.SerializedName;
import com.stripe.net.ApiRequestParams;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class SubscriptionListParams extends ApiRequestParams {
/**
* The collection method of the subscriptions to retrieve. Either {@code charge_automatically} or
* {@code send_invoice}.
*/
@SerializedName("collection_method")
CollectionMethod collectionMethod;
@SerializedName("created")
Object created;
@SerializedName("current_period_end")
Object currentPeriodEnd;
@SerializedName("current_period_start")
Object currentPeriodStart;
/**
* The ID of the customer whose subscriptions will be retrieved.
*/
@SerializedName("customer")
String customer;
/**
* A cursor for use in pagination. {@code ending_before} is an object ID that defines your place
* in the list. For instance, if you make a list request and receive 100 objects, starting with
* {@code obj_bar}, your subsequent call can include {@code ending_before=obj_bar} in order to
* fetch the previous page of the list.
*/
@SerializedName("ending_before")
String endingBefore;
/**
* Specifies which fields in the response should be expanded.
*/
@SerializedName("expand")
List expand;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* A limit on the number of objects to be returned. Limit can range between 1 and 100, and the
* default is 10.
*/
@SerializedName("limit")
Long limit;
/**
* The ID of the plan whose subscriptions will be retrieved.
*/
@SerializedName("plan")
String plan;
/**
* Filter for subscriptions that contain this recurring price ID.
*/
@SerializedName("price")
String price;
/**
* A cursor for use in pagination. {@code starting_after} is an object ID that defines your place
* in the list. For instance, if you make a list request and receive 100 objects, ending with
* {@code obj_foo}, your subsequent call can include {@code starting_after=obj_foo} in order to
* fetch the next page of the list.
*/
@SerializedName("starting_after")
String startingAfter;
/**
* The status of the subscriptions to retrieve. One of: {@code incomplete}, {@code
* incomplete_expired}, {@code trialing}, {@code active}, {@code past_due}, {@code unpaid}, {@code
* canceled}, or {@code all}. Passing in a value of {@code canceled} will return all canceled
* subscriptions, including those belonging to deleted customers. Passing in a value of {@code
* all} will return subscriptions of all statuses.
*/
@SerializedName("status")
Status status;
private SubscriptionListParams(CollectionMethod collectionMethod, Object created, Object currentPeriodEnd, Object currentPeriodStart, String customer, String endingBefore, List expand, Map extraParams, Long limit, String plan, String price, String startingAfter, Status status) {
this.collectionMethod = collectionMethod;
this.created = created;
this.currentPeriodEnd = currentPeriodEnd;
this.currentPeriodStart = currentPeriodStart;
this.customer = customer;
this.endingBefore = endingBefore;
this.expand = expand;
this.extraParams = extraParams;
this.limit = limit;
this.plan = plan;
this.price = price;
this.startingAfter = startingAfter;
this.status = status;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private CollectionMethod collectionMethod;
private Object created;
private Object currentPeriodEnd;
private Object currentPeriodStart;
private String customer;
private String endingBefore;
private List expand;
private Map extraParams;
private Long limit;
private String plan;
private String price;
private String startingAfter;
private Status status;
/**
* Finalize and obtain parameter instance from this builder.
*/
public SubscriptionListParams build() {
return new SubscriptionListParams(this.collectionMethod, this.created, this.currentPeriodEnd, this.currentPeriodStart, this.customer, this.endingBefore, this.expand, this.extraParams, this.limit, this.plan, this.price, this.startingAfter, this.status);
}
/**
* The collection method of the subscriptions to retrieve. Either {@code charge_automatically}
* or {@code send_invoice}.
*/
public Builder setCollectionMethod(CollectionMethod collectionMethod) {
this.collectionMethod = collectionMethod;
return this;
}
public Builder setCreated(Created created) {
this.created = created;
return this;
}
public Builder setCreated(Long created) {
this.created = created;
return this;
}
public Builder setCurrentPeriodEnd(CurrentPeriodEnd currentPeriodEnd) {
this.currentPeriodEnd = currentPeriodEnd;
return this;
}
public Builder setCurrentPeriodEnd(Long currentPeriodEnd) {
this.currentPeriodEnd = currentPeriodEnd;
return this;
}
public Builder setCurrentPeriodStart(CurrentPeriodStart currentPeriodStart) {
this.currentPeriodStart = currentPeriodStart;
return this;
}
public Builder setCurrentPeriodStart(Long currentPeriodStart) {
this.currentPeriodStart = currentPeriodStart;
return this;
}
/**
* The ID of the customer whose subscriptions will be retrieved.
*/
public Builder setCustomer(String customer) {
this.customer = customer;
return this;
}
/**
* A cursor for use in pagination. {@code ending_before} is an object ID that defines your place
* in the list. For instance, if you make a list request and receive 100 objects, starting with
* {@code obj_bar}, your subsequent call can include {@code ending_before=obj_bar} in order to
* fetch the previous page of the list.
*/
public Builder setEndingBefore(String endingBefore) {
this.endingBefore = endingBefore;
return this;
}
/**
* Add an element to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SubscriptionListParams#expand} for the field documentation.
*/
public Builder addExpand(String element) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.add(element);
return this;
}
/**
* Add all elements to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SubscriptionListParams#expand} for the field documentation.
*/
public Builder addAllExpand(List elements) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.addAll(elements);
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionListParams#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionListParams#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* A limit on the number of objects to be returned. Limit can range between 1 and 100, and the
* default is 10.
*/
public Builder setLimit(Long limit) {
this.limit = limit;
return this;
}
/**
* The ID of the plan whose subscriptions will be retrieved.
*/
public Builder setPlan(String plan) {
this.plan = plan;
return this;
}
/**
* Filter for subscriptions that contain this recurring price ID.
*/
public Builder setPrice(String price) {
this.price = price;
return this;
}
/**
* A cursor for use in pagination. {@code starting_after} is an object ID that defines your
* place in the list. For instance, if you make a list request and receive 100 objects, ending
* with {@code obj_foo}, your subsequent call can include {@code starting_after=obj_foo} in
* order to fetch the next page of the list.
*/
public Builder setStartingAfter(String startingAfter) {
this.startingAfter = startingAfter;
return this;
}
/**
* The status of the subscriptions to retrieve. One of: {@code incomplete}, {@code
* incomplete_expired}, {@code trialing}, {@code active}, {@code past_due}, {@code unpaid},
* {@code canceled}, or {@code all}. Passing in a value of {@code canceled} will return all
* canceled subscriptions, including those belonging to deleted customers. Passing in a value of
* {@code all} will return subscriptions of all statuses.
*/
public Builder setStatus(Status status) {
this.status = status;
return this;
}
}
public static class Created {
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Minimum value to filter by (exclusive).
*/
@SerializedName("gt")
Long gt;
/**
* Minimum value to filter by (inclusive).
*/
@SerializedName("gte")
Long gte;
/**
* Maximum value to filter by (exclusive).
*/
@SerializedName("lt")
Long lt;
/**
* Maximum value to filter by (inclusive).
*/
@SerializedName("lte")
Long lte;
private Created(Map extraParams, Long gt, Long gte, Long lt, Long lte) {
this.extraParams = extraParams;
this.gt = gt;
this.gte = gte;
this.lt = lt;
this.lte = lte;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private Long gt;
private Long gte;
private Long lt;
private Long lte;
/** Finalize and obtain parameter instance from this builder. */
public Created build() {
return new Created(this.extraParams, this.gt, this.gte, this.lt, this.lte);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionListParams.Created#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionListParams.Created#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/** Minimum value to filter by (exclusive). */
public Builder setGt(Long gt) {
this.gt = gt;
return this;
}
/** Minimum value to filter by (inclusive). */
public Builder setGte(Long gte) {
this.gte = gte;
return this;
}
/** Maximum value to filter by (exclusive). */
public Builder setLt(Long lt) {
this.lt = lt;
return this;
}
public Builder setLte(Long lte) {
this.lte = lte;
return this;
}
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Minimum value to filter by (exclusive).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getGt() {
return this.gt;
}
/**
* Minimum value to filter by (inclusive).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getGte() {
return this.gte;
}
/**
* Maximum value to filter by (exclusive).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getLt() {
return this.lt;
}
/**
* Maximum value to filter by (inclusive).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getLte() {
return this.lte;
}
}
/**
* Maximum value to filter by (inclusive).
*/
public static class CurrentPeriodEnd {
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Minimum value to filter by (exclusive).
*/
@SerializedName("gt")
Long gt;
/**
* Minimum value to filter by (inclusive).
*/
@SerializedName("gte")
Long gte;
/**
* Maximum value to filter by (exclusive).
*/
@SerializedName("lt")
Long lt;
/**
* Maximum value to filter by (inclusive).
*/
@SerializedName("lte")
Long lte;
private CurrentPeriodEnd(Map extraParams, Long gt, Long gte, Long lt, Long lte) {
this.extraParams = extraParams;
this.gt = gt;
this.gte = gte;
this.lt = lt;
this.lte = lte;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private Long gt;
private Long gte;
private Long lt;
private Long lte;
/** Finalize and obtain parameter instance from this builder. */
public CurrentPeriodEnd build() {
return new CurrentPeriodEnd(this.extraParams, this.gt, this.gte, this.lt, this.lte);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionListParams.CurrentPeriodEnd#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionListParams.CurrentPeriodEnd#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/** Minimum value to filter by (exclusive). */
public Builder setGt(Long gt) {
this.gt = gt;
return this;
}
/** Minimum value to filter by (inclusive). */
public Builder setGte(Long gte) {
this.gte = gte;
return this;
}
/** Maximum value to filter by (exclusive). */
public Builder setLt(Long lt) {
this.lt = lt;
return this;
}
public Builder setLte(Long lte) {
this.lte = lte;
return this;
}
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Minimum value to filter by (exclusive).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getGt() {
return this.gt;
}
/**
* Minimum value to filter by (inclusive).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getGte() {
return this.gte;
}
/**
* Maximum value to filter by (exclusive).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getLt() {
return this.lt;
}
/**
* Maximum value to filter by (inclusive).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getLte() {
return this.lte;
}
}
/**
* Maximum value to filter by (inclusive).
*/
public static class CurrentPeriodStart {
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Minimum value to filter by (exclusive).
*/
@SerializedName("gt")
Long gt;
/**
* Minimum value to filter by (inclusive).
*/
@SerializedName("gte")
Long gte;
/**
* Maximum value to filter by (exclusive).
*/
@SerializedName("lt")
Long lt;
/**
* Maximum value to filter by (inclusive).
*/
@SerializedName("lte")
Long lte;
private CurrentPeriodStart(Map extraParams, Long gt, Long gte, Long lt, Long lte) {
this.extraParams = extraParams;
this.gt = gt;
this.gte = gte;
this.lt = lt;
this.lte = lte;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private Long gt;
private Long gte;
private Long lt;
private Long lte;
/** Finalize and obtain parameter instance from this builder. */
public CurrentPeriodStart build() {
return new CurrentPeriodStart(this.extraParams, this.gt, this.gte, this.lt, this.lte);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionListParams.CurrentPeriodStart#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionListParams.CurrentPeriodStart#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/** Minimum value to filter by (exclusive). */
public Builder setGt(Long gt) {
this.gt = gt;
return this;
}
/** Minimum value to filter by (inclusive). */
public Builder setGte(Long gte) {
this.gte = gte;
return this;
}
/** Maximum value to filter by (exclusive). */
public Builder setLt(Long lt) {
this.lt = lt;
return this;
}
public Builder setLte(Long lte) {
this.lte = lte;
return this;
}
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Minimum value to filter by (exclusive).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getGt() {
return this.gt;
}
/**
* Minimum value to filter by (inclusive).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getGte() {
return this.gte;
}
/**
* Maximum value to filter by (exclusive).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getLt() {
return this.lt;
}
/**
* Maximum value to filter by (inclusive).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getLte() {
return this.lte;
}
}
/**
* Maximum value to filter by (inclusive).
*/
public enum CollectionMethod implements ApiRequestParams.EnumParam {
@SerializedName("charge_automatically")
CHARGE_AUTOMATICALLY("charge_automatically"), @SerializedName("send_invoice")
SEND_INVOICE("send_invoice");
private final String value;
CollectionMethod(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum Status implements ApiRequestParams.EnumParam {
@SerializedName("active")
ACTIVE("active"), @SerializedName("all")
ALL("all"), @SerializedName("canceled")
CANCELED("canceled"), @SerializedName("ended")
ENDED("ended"), @SerializedName("incomplete")
INCOMPLETE("incomplete"), @SerializedName("incomplete_expired")
INCOMPLETE_EXPIRED("incomplete_expired"), @SerializedName("past_due")
PAST_DUE("past_due"), @SerializedName("trialing")
TRIALING("trialing"), @SerializedName("unpaid")
UNPAID("unpaid");
private final String value;
Status(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
/**
* The collection method of the subscriptions to retrieve. Either {@code charge_automatically} or
* {@code send_invoice}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public CollectionMethod getCollectionMethod() {
return this.collectionMethod;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getCreated() {
return this.created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getCurrentPeriodEnd() {
return this.currentPeriodEnd;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getCurrentPeriodStart() {
return this.currentPeriodStart;
}
/**
* The ID of the customer whose subscriptions will be retrieved.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCustomer() {
return this.customer;
}
/**
* A cursor for use in pagination. {@code ending_before} is an object ID that defines your place
* in the list. For instance, if you make a list request and receive 100 objects, starting with
* {@code obj_bar}, your subsequent call can include {@code ending_before=obj_bar} in order to
* fetch the previous page of the list.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getEndingBefore() {
return this.endingBefore;
}
/**
* Specifies which fields in the response should be expanded.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getExpand() {
return this.expand;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* A limit on the number of objects to be returned. Limit can range between 1 and 100, and the
* default is 10.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getLimit() {
return this.limit;
}
/**
* The ID of the plan whose subscriptions will be retrieved.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPlan() {
return this.plan;
}
/**
* Filter for subscriptions that contain this recurring price ID.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPrice() {
return this.price;
}
/**
* A cursor for use in pagination. {@code starting_after} is an object ID that defines your place
* in the list. For instance, if you make a list request and receive 100 objects, ending with
* {@code obj_foo}, your subsequent call can include {@code starting_after=obj_foo} in order to
* fetch the next page of the list.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStartingAfter() {
return this.startingAfter;
}
/**
* The status of the subscriptions to retrieve. One of: {@code incomplete}, {@code
* incomplete_expired}, {@code trialing}, {@code active}, {@code past_due}, {@code unpaid}, {@code
* canceled}, or {@code all}. Passing in a value of {@code canceled} will return all canceled
* subscriptions, including those belonging to deleted customers. Passing in a value of {@code
* all} will return subscriptions of all statuses.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Status getStatus() {
return this.status;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy