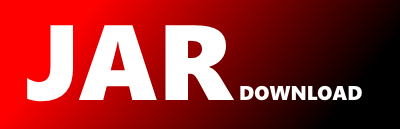
com.stripe.model.billingportal.Configuration Maven / Gradle / Ivy
// Generated by delombok at Wed Jun 30 19:13:17 EDT 2021
// File generated from our OpenAPI spec
package com.stripe.model.billingportal;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.model.HasId;
import com.stripe.model.StripeObject;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.billingportal.ConfigurationCreateParams;
import com.stripe.param.billingportal.ConfigurationListParams;
import com.stripe.param.billingportal.ConfigurationRetrieveParams;
import com.stripe.param.billingportal.ConfigurationUpdateParams;
import java.util.List;
import java.util.Map;
public class Configuration extends ApiResource implements HasId {
/**
* Whether the configuration is active and can be used to create portal sessions.
*/
@SerializedName("active")
Boolean active;
/**
* ID of the Connect Application that created the configuration.
*/
@SerializedName("application")
String application;
@SerializedName("business_profile")
BusinessProfile businessProfile;
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* The default URL to redirect customers to when they click on the portal's link to return to your
* website. This can be overriden
* when creating the session.
*/
@SerializedName("default_return_url")
String defaultReturnUrl;
@SerializedName("features")
Features features;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* Whether the configuration is the default. If {@code true}, this configuration can be managed in
* the Dashboard and portal sessions will use this configuration unless it is overriden when
* creating the session.
*/
@SerializedName("is_default")
Boolean isDefault;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code billing_portal.configuration}.
*/
@SerializedName("object")
String object;
/**
* Time at which the object was last updated. Measured in seconds since the Unix epoch.
*/
@SerializedName("updated")
Long updated;
/**
* Returns a list of configurations that describe the functionality of the customer portal.
*/
public static ConfigurationCollection list(Map params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of configurations that describe the functionality of the customer portal.
*/
public static ConfigurationCollection list(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/billing_portal/configurations");
return ApiResource.requestCollection(url, params, ConfigurationCollection.class, options);
}
/**
* Returns a list of configurations that describe the functionality of the customer portal.
*/
public static ConfigurationCollection list(ConfigurationListParams params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of configurations that describe the functionality of the customer portal.
*/
public static ConfigurationCollection list(ConfigurationListParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/billing_portal/configurations");
return ApiResource.requestCollection(url, params, ConfigurationCollection.class, options);
}
/**
* Creates a configuration that describes the functionality and behavior of a PortalSession.
*/
public static Configuration create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Creates a configuration that describes the functionality and behavior of a PortalSession.
*/
public static Configuration create(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/billing_portal/configurations");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Configuration.class, options);
}
/**
* Creates a configuration that describes the functionality and behavior of a PortalSession.
*/
public static Configuration create(ConfigurationCreateParams params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Creates a configuration that describes the functionality and behavior of a PortalSession.
*/
public static Configuration create(ConfigurationCreateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/billing_portal/configurations");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Configuration.class, options);
}
/**
* Updates a configuration that describes the functionality of the customer portal.
*/
public Configuration update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates a configuration that describes the functionality of the customer portal.
*/
public Configuration update(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/billing_portal/configurations/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Configuration.class, options);
}
/**
* Updates a configuration that describes the functionality of the customer portal.
*/
public Configuration update(ConfigurationUpdateParams params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates a configuration that describes the functionality of the customer portal.
*/
public Configuration update(ConfigurationUpdateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/billing_portal/configurations/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Configuration.class, options);
}
/**
* Retrieves a configuration that describes the functionality of the customer portal.
*/
public static Configuration retrieve(String configuration) throws StripeException {
return retrieve(configuration, (Map) null, (RequestOptions) null);
}
/**
* Retrieves a configuration that describes the functionality of the customer portal.
*/
public static Configuration retrieve(String configuration, RequestOptions options) throws StripeException {
return retrieve(configuration, (Map) null, options);
}
/**
* Retrieves a configuration that describes the functionality of the customer portal.
*/
public static Configuration retrieve(String configuration, Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/billing_portal/configurations/%s", ApiResource.urlEncodeId(configuration)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Configuration.class, options);
}
/**
* Retrieves a configuration that describes the functionality of the customer portal.
*/
public static Configuration retrieve(String configuration, ConfigurationRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/billing_portal/configurations/%s", ApiResource.urlEncodeId(configuration)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Configuration.class, options);
}
public static class BusinessProfile extends StripeObject {
/**
* The messaging shown to customers in the portal.
*/
@SerializedName("headline")
String headline;
/**
* A link to the business’s publicly available privacy policy.
*/
@SerializedName("privacy_policy_url")
String privacyPolicyUrl;
/**
* A link to the business’s publicly available terms of service.
*/
@SerializedName("terms_of_service_url")
String termsOfServiceUrl;
/**
* The messaging shown to customers in the portal.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getHeadline() {
return this.headline;
}
/**
* A link to the business’s publicly available privacy policy.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPrivacyPolicyUrl() {
return this.privacyPolicyUrl;
}
/**
* A link to the business’s publicly available terms of service.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTermsOfServiceUrl() {
return this.termsOfServiceUrl;
}
/**
* The messaging shown to customers in the portal.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setHeadline(final String headline) {
this.headline = headline;
}
/**
* A link to the business’s publicly available privacy policy.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPrivacyPolicyUrl(final String privacyPolicyUrl) {
this.privacyPolicyUrl = privacyPolicyUrl;
}
/**
* A link to the business’s publicly available terms of service.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTermsOfServiceUrl(final String termsOfServiceUrl) {
this.termsOfServiceUrl = termsOfServiceUrl;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Configuration.BusinessProfile)) return false;
final Configuration.BusinessProfile other = (Configuration.BusinessProfile) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$headline = this.getHeadline();
final java.lang.Object other$headline = other.getHeadline();
if (this$headline == null ? other$headline != null : !this$headline.equals(other$headline)) return false;
final java.lang.Object this$privacyPolicyUrl = this.getPrivacyPolicyUrl();
final java.lang.Object other$privacyPolicyUrl = other.getPrivacyPolicyUrl();
if (this$privacyPolicyUrl == null ? other$privacyPolicyUrl != null : !this$privacyPolicyUrl.equals(other$privacyPolicyUrl)) return false;
final java.lang.Object this$termsOfServiceUrl = this.getTermsOfServiceUrl();
final java.lang.Object other$termsOfServiceUrl = other.getTermsOfServiceUrl();
if (this$termsOfServiceUrl == null ? other$termsOfServiceUrl != null : !this$termsOfServiceUrl.equals(other$termsOfServiceUrl)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Configuration.BusinessProfile;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $headline = this.getHeadline();
result = result * PRIME + ($headline == null ? 43 : $headline.hashCode());
final java.lang.Object $privacyPolicyUrl = this.getPrivacyPolicyUrl();
result = result * PRIME + ($privacyPolicyUrl == null ? 43 : $privacyPolicyUrl.hashCode());
final java.lang.Object $termsOfServiceUrl = this.getTermsOfServiceUrl();
result = result * PRIME + ($termsOfServiceUrl == null ? 43 : $termsOfServiceUrl.hashCode());
return result;
}
}
public static class Features extends StripeObject {
@SerializedName("customer_update")
CustomerUpdate customerUpdate;
@SerializedName("invoice_history")
InvoiceHistory invoiceHistory;
@SerializedName("payment_method_update")
PaymentMethodUpdate paymentMethodUpdate;
@SerializedName("subscription_cancel")
SubscriptionCancel subscriptionCancel;
@SerializedName("subscription_pause")
SubscriptionPause subscriptionPause;
@SerializedName("subscription_update")
SubscriptionUpdate subscriptionUpdate;
public static class CustomerUpdate extends StripeObject {
/**
* The types of customer updates that are supported. When empty, customers are not updateable.
*/
@SerializedName("allowed_updates")
List allowedUpdates;
/** Whether the feature is enabled. */
@SerializedName("enabled")
Boolean enabled;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getAllowedUpdates() {
return this.allowedUpdates;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getEnabled() {
return this.enabled;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAllowedUpdates(final List allowedUpdates) {
this.allowedUpdates = allowedUpdates;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEnabled(final Boolean enabled) {
this.enabled = enabled;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Configuration.Features.CustomerUpdate)) return false;
final Configuration.Features.CustomerUpdate other = (Configuration.Features.CustomerUpdate) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$enabled = this.getEnabled();
final java.lang.Object other$enabled = other.getEnabled();
if (this$enabled == null ? other$enabled != null : !this$enabled.equals(other$enabled)) return false;
final java.lang.Object this$allowedUpdates = this.getAllowedUpdates();
final java.lang.Object other$allowedUpdates = other.getAllowedUpdates();
if (this$allowedUpdates == null ? other$allowedUpdates != null : !this$allowedUpdates.equals(other$allowedUpdates)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Configuration.Features.CustomerUpdate;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $enabled = this.getEnabled();
result = result * PRIME + ($enabled == null ? 43 : $enabled.hashCode());
final java.lang.Object $allowedUpdates = this.getAllowedUpdates();
result = result * PRIME + ($allowedUpdates == null ? 43 : $allowedUpdates.hashCode());
return result;
}
}
public static class InvoiceHistory extends StripeObject {
/** Whether the feature is enabled. */
@SerializedName("enabled")
Boolean enabled;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getEnabled() {
return this.enabled;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEnabled(final Boolean enabled) {
this.enabled = enabled;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Configuration.Features.InvoiceHistory)) return false;
final Configuration.Features.InvoiceHistory other = (Configuration.Features.InvoiceHistory) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$enabled = this.getEnabled();
final java.lang.Object other$enabled = other.getEnabled();
if (this$enabled == null ? other$enabled != null : !this$enabled.equals(other$enabled)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Configuration.Features.InvoiceHistory;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $enabled = this.getEnabled();
result = result * PRIME + ($enabled == null ? 43 : $enabled.hashCode());
return result;
}
}
public static class PaymentMethodUpdate extends StripeObject {
/** Whether the feature is enabled. */
@SerializedName("enabled")
Boolean enabled;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getEnabled() {
return this.enabled;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEnabled(final Boolean enabled) {
this.enabled = enabled;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Configuration.Features.PaymentMethodUpdate)) return false;
final Configuration.Features.PaymentMethodUpdate other = (Configuration.Features.PaymentMethodUpdate) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$enabled = this.getEnabled();
final java.lang.Object other$enabled = other.getEnabled();
if (this$enabled == null ? other$enabled != null : !this$enabled.equals(other$enabled)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Configuration.Features.PaymentMethodUpdate;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $enabled = this.getEnabled();
result = result * PRIME + ($enabled == null ? 43 : $enabled.hashCode());
return result;
}
}
public static class SubscriptionCancel extends StripeObject {
/** Whether the feature is enabled. */
@SerializedName("enabled")
Boolean enabled;
/**
* Whether to cancel subscriptions immediately or at the end of the billing period.
*
* One of {@code at_period_end}, or {@code immediately}.
*/
@SerializedName("mode")
String mode;
/**
* Whether to create prorations when canceling subscriptions. Possible values are {@code none}
* and {@code create_prorations}.
*
*
One of {@code always_invoice}, {@code create_prorations}, or {@code none}.
*/
@SerializedName("proration_behavior")
String prorationBehavior;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getEnabled() {
return this.enabled;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getMode() {
return this.mode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getProrationBehavior() {
return this.prorationBehavior;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEnabled(final Boolean enabled) {
this.enabled = enabled;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMode(final String mode) {
this.mode = mode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setProrationBehavior(final String prorationBehavior) {
this.prorationBehavior = prorationBehavior;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Configuration.Features.SubscriptionCancel)) return false;
final Configuration.Features.SubscriptionCancel other = (Configuration.Features.SubscriptionCancel) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$enabled = this.getEnabled();
final java.lang.Object other$enabled = other.getEnabled();
if (this$enabled == null ? other$enabled != null : !this$enabled.equals(other$enabled)) return false;
final java.lang.Object this$mode = this.getMode();
final java.lang.Object other$mode = other.getMode();
if (this$mode == null ? other$mode != null : !this$mode.equals(other$mode)) return false;
final java.lang.Object this$prorationBehavior = this.getProrationBehavior();
final java.lang.Object other$prorationBehavior = other.getProrationBehavior();
if (this$prorationBehavior == null ? other$prorationBehavior != null : !this$prorationBehavior.equals(other$prorationBehavior)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Configuration.Features.SubscriptionCancel;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $enabled = this.getEnabled();
result = result * PRIME + ($enabled == null ? 43 : $enabled.hashCode());
final java.lang.Object $mode = this.getMode();
result = result * PRIME + ($mode == null ? 43 : $mode.hashCode());
final java.lang.Object $prorationBehavior = this.getProrationBehavior();
result = result * PRIME + ($prorationBehavior == null ? 43 : $prorationBehavior.hashCode());
return result;
}
}
public static class SubscriptionPause extends StripeObject {
/** Whether the feature is enabled. */
@SerializedName("enabled")
Boolean enabled;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getEnabled() {
return this.enabled;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEnabled(final Boolean enabled) {
this.enabled = enabled;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Configuration.Features.SubscriptionPause)) return false;
final Configuration.Features.SubscriptionPause other = (Configuration.Features.SubscriptionPause) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$enabled = this.getEnabled();
final java.lang.Object other$enabled = other.getEnabled();
if (this$enabled == null ? other$enabled != null : !this$enabled.equals(other$enabled)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Configuration.Features.SubscriptionPause;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $enabled = this.getEnabled();
result = result * PRIME + ($enabled == null ? 43 : $enabled.hashCode());
return result;
}
}
public static class SubscriptionUpdate extends StripeObject {
/**
* The types of subscription updates that are supported for items listed in the {@code
* products} attribute. When empty, subscriptions are not updateable.
*/
@SerializedName("default_allowed_updates")
List defaultAllowedUpdates;
/** Whether the feature is enabled. */
@SerializedName("enabled")
Boolean enabled;
/** The list of products that support subscription updates. */
@SerializedName("products")
List products;
/**
* Determines how to handle prorations resulting from subscription updates. Valid values are
* {@code none}, {@code create_prorations}, and {@code always_invoice}.
*
* One of {@code always_invoice}, {@code create_prorations}, or {@code none}.
*/
@SerializedName("proration_behavior")
String prorationBehavior;
public static class Product extends StripeObject {
/** The list of price IDs which, when subscribed to, a subscription can be updated. */
@SerializedName("prices")
List prices;
/** The product ID. */
@SerializedName("product")
String product;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getPrices() {
return this.prices;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getProduct() {
return this.product;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPrices(final List prices) {
this.prices = prices;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setProduct(final String product) {
this.product = product;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Configuration.Features.SubscriptionUpdate.Product)) return false;
final Configuration.Features.SubscriptionUpdate.Product other = (Configuration.Features.SubscriptionUpdate.Product) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$prices = this.getPrices();
final java.lang.Object other$prices = other.getPrices();
if (this$prices == null ? other$prices != null : !this$prices.equals(other$prices)) return false;
final java.lang.Object this$product = this.getProduct();
final java.lang.Object other$product = other.getProduct();
if (this$product == null ? other$product != null : !this$product.equals(other$product)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Configuration.Features.SubscriptionUpdate.Product;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $prices = this.getPrices();
result = result * PRIME + ($prices == null ? 43 : $prices.hashCode());
final java.lang.Object $product = this.getProduct();
result = result * PRIME + ($product == null ? 43 : $product.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getDefaultAllowedUpdates() {
return this.defaultAllowedUpdates;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getEnabled() {
return this.enabled;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getProducts() {
return this.products;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getProrationBehavior() {
return this.prorationBehavior;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDefaultAllowedUpdates(final List defaultAllowedUpdates) {
this.defaultAllowedUpdates = defaultAllowedUpdates;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEnabled(final Boolean enabled) {
this.enabled = enabled;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setProducts(final List products) {
this.products = products;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setProrationBehavior(final String prorationBehavior) {
this.prorationBehavior = prorationBehavior;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Configuration.Features.SubscriptionUpdate)) return false;
final Configuration.Features.SubscriptionUpdate other = (Configuration.Features.SubscriptionUpdate) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$enabled = this.getEnabled();
final java.lang.Object other$enabled = other.getEnabled();
if (this$enabled == null ? other$enabled != null : !this$enabled.equals(other$enabled)) return false;
final java.lang.Object this$defaultAllowedUpdates = this.getDefaultAllowedUpdates();
final java.lang.Object other$defaultAllowedUpdates = other.getDefaultAllowedUpdates();
if (this$defaultAllowedUpdates == null ? other$defaultAllowedUpdates != null : !this$defaultAllowedUpdates.equals(other$defaultAllowedUpdates)) return false;
final java.lang.Object this$products = this.getProducts();
final java.lang.Object other$products = other.getProducts();
if (this$products == null ? other$products != null : !this$products.equals(other$products)) return false;
final java.lang.Object this$prorationBehavior = this.getProrationBehavior();
final java.lang.Object other$prorationBehavior = other.getProrationBehavior();
if (this$prorationBehavior == null ? other$prorationBehavior != null : !this$prorationBehavior.equals(other$prorationBehavior)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Configuration.Features.SubscriptionUpdate;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $enabled = this.getEnabled();
result = result * PRIME + ($enabled == null ? 43 : $enabled.hashCode());
final java.lang.Object $defaultAllowedUpdates = this.getDefaultAllowedUpdates();
result = result * PRIME + ($defaultAllowedUpdates == null ? 43 : $defaultAllowedUpdates.hashCode());
final java.lang.Object $products = this.getProducts();
result = result * PRIME + ($products == null ? 43 : $products.hashCode());
final java.lang.Object $prorationBehavior = this.getProrationBehavior();
result = result * PRIME + ($prorationBehavior == null ? 43 : $prorationBehavior.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public CustomerUpdate getCustomerUpdate() {
return this.customerUpdate;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public InvoiceHistory getInvoiceHistory() {
return this.invoiceHistory;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PaymentMethodUpdate getPaymentMethodUpdate() {
return this.paymentMethodUpdate;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SubscriptionCancel getSubscriptionCancel() {
return this.subscriptionCancel;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SubscriptionPause getSubscriptionPause() {
return this.subscriptionPause;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SubscriptionUpdate getSubscriptionUpdate() {
return this.subscriptionUpdate;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCustomerUpdate(final CustomerUpdate customerUpdate) {
this.customerUpdate = customerUpdate;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setInvoiceHistory(final InvoiceHistory invoiceHistory) {
this.invoiceHistory = invoiceHistory;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPaymentMethodUpdate(final PaymentMethodUpdate paymentMethodUpdate) {
this.paymentMethodUpdate = paymentMethodUpdate;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSubscriptionCancel(final SubscriptionCancel subscriptionCancel) {
this.subscriptionCancel = subscriptionCancel;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSubscriptionPause(final SubscriptionPause subscriptionPause) {
this.subscriptionPause = subscriptionPause;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSubscriptionUpdate(final SubscriptionUpdate subscriptionUpdate) {
this.subscriptionUpdate = subscriptionUpdate;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Configuration.Features)) return false;
final Configuration.Features other = (Configuration.Features) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$customerUpdate = this.getCustomerUpdate();
final java.lang.Object other$customerUpdate = other.getCustomerUpdate();
if (this$customerUpdate == null ? other$customerUpdate != null : !this$customerUpdate.equals(other$customerUpdate)) return false;
final java.lang.Object this$invoiceHistory = this.getInvoiceHistory();
final java.lang.Object other$invoiceHistory = other.getInvoiceHistory();
if (this$invoiceHistory == null ? other$invoiceHistory != null : !this$invoiceHistory.equals(other$invoiceHistory)) return false;
final java.lang.Object this$paymentMethodUpdate = this.getPaymentMethodUpdate();
final java.lang.Object other$paymentMethodUpdate = other.getPaymentMethodUpdate();
if (this$paymentMethodUpdate == null ? other$paymentMethodUpdate != null : !this$paymentMethodUpdate.equals(other$paymentMethodUpdate)) return false;
final java.lang.Object this$subscriptionCancel = this.getSubscriptionCancel();
final java.lang.Object other$subscriptionCancel = other.getSubscriptionCancel();
if (this$subscriptionCancel == null ? other$subscriptionCancel != null : !this$subscriptionCancel.equals(other$subscriptionCancel)) return false;
final java.lang.Object this$subscriptionPause = this.getSubscriptionPause();
final java.lang.Object other$subscriptionPause = other.getSubscriptionPause();
if (this$subscriptionPause == null ? other$subscriptionPause != null : !this$subscriptionPause.equals(other$subscriptionPause)) return false;
final java.lang.Object this$subscriptionUpdate = this.getSubscriptionUpdate();
final java.lang.Object other$subscriptionUpdate = other.getSubscriptionUpdate();
if (this$subscriptionUpdate == null ? other$subscriptionUpdate != null : !this$subscriptionUpdate.equals(other$subscriptionUpdate)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Configuration.Features;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $customerUpdate = this.getCustomerUpdate();
result = result * PRIME + ($customerUpdate == null ? 43 : $customerUpdate.hashCode());
final java.lang.Object $invoiceHistory = this.getInvoiceHistory();
result = result * PRIME + ($invoiceHistory == null ? 43 : $invoiceHistory.hashCode());
final java.lang.Object $paymentMethodUpdate = this.getPaymentMethodUpdate();
result = result * PRIME + ($paymentMethodUpdate == null ? 43 : $paymentMethodUpdate.hashCode());
final java.lang.Object $subscriptionCancel = this.getSubscriptionCancel();
result = result * PRIME + ($subscriptionCancel == null ? 43 : $subscriptionCancel.hashCode());
final java.lang.Object $subscriptionPause = this.getSubscriptionPause();
result = result * PRIME + ($subscriptionPause == null ? 43 : $subscriptionPause.hashCode());
final java.lang.Object $subscriptionUpdate = this.getSubscriptionUpdate();
result = result * PRIME + ($subscriptionUpdate == null ? 43 : $subscriptionUpdate.hashCode());
return result;
}
}
/**
* Whether the configuration is active and can be used to create portal sessions.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getActive() {
return this.active;
}
/**
* ID of the Connect Application that created the configuration.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getApplication() {
return this.application;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BusinessProfile getBusinessProfile() {
return this.businessProfile;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
/**
* The default URL to redirect customers to when they click on the portal's link to return to your
* website. This can be overriden
* when creating the session.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDefaultReturnUrl() {
return this.defaultReturnUrl;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Features getFeatures() {
return this.features;
}
/**
* Whether the configuration is the default. If {@code true}, this configuration can be managed in
* the Dashboard and portal sessions will use this configuration unless it is overriden when
* creating the session.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getIsDefault() {
return this.isDefault;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code billing_portal.configuration}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* Time at which the object was last updated. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getUpdated() {
return this.updated;
}
/**
* Whether the configuration is active and can be used to create portal sessions.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setActive(final Boolean active) {
this.active = active;
}
/**
* ID of the Connect Application that created the configuration.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setApplication(final String application) {
this.application = application;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBusinessProfile(final BusinessProfile businessProfile) {
this.businessProfile = businessProfile;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* The default URL to redirect customers to when they click on the portal's link to return to your
* website. This can be overriden
* when creating the session.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDefaultReturnUrl(final String defaultReturnUrl) {
this.defaultReturnUrl = defaultReturnUrl;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFeatures(final Features features) {
this.features = features;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* Whether the configuration is the default. If {@code true}, this configuration can be managed in
* the Dashboard and portal sessions will use this configuration unless it is overriden when
* creating the session.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIsDefault(final Boolean isDefault) {
this.isDefault = isDefault;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code billing_portal.configuration}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* Time at which the object was last updated. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUpdated(final Long updated) {
this.updated = updated;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Configuration)) return false;
final Configuration other = (Configuration) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$active = this.getActive();
final java.lang.Object other$active = other.getActive();
if (this$active == null ? other$active != null : !this$active.equals(other$active)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$isDefault = this.getIsDefault();
final java.lang.Object other$isDefault = other.getIsDefault();
if (this$isDefault == null ? other$isDefault != null : !this$isDefault.equals(other$isDefault)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$updated = this.getUpdated();
final java.lang.Object other$updated = other.getUpdated();
if (this$updated == null ? other$updated != null : !this$updated.equals(other$updated)) return false;
final java.lang.Object this$application = this.getApplication();
final java.lang.Object other$application = other.getApplication();
if (this$application == null ? other$application != null : !this$application.equals(other$application)) return false;
final java.lang.Object this$businessProfile = this.getBusinessProfile();
final java.lang.Object other$businessProfile = other.getBusinessProfile();
if (this$businessProfile == null ? other$businessProfile != null : !this$businessProfile.equals(other$businessProfile)) return false;
final java.lang.Object this$defaultReturnUrl = this.getDefaultReturnUrl();
final java.lang.Object other$defaultReturnUrl = other.getDefaultReturnUrl();
if (this$defaultReturnUrl == null ? other$defaultReturnUrl != null : !this$defaultReturnUrl.equals(other$defaultReturnUrl)) return false;
final java.lang.Object this$features = this.getFeatures();
final java.lang.Object other$features = other.getFeatures();
if (this$features == null ? other$features != null : !this$features.equals(other$features)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Configuration;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $active = this.getActive();
result = result * PRIME + ($active == null ? 43 : $active.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $isDefault = this.getIsDefault();
result = result * PRIME + ($isDefault == null ? 43 : $isDefault.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $updated = this.getUpdated();
result = result * PRIME + ($updated == null ? 43 : $updated.hashCode());
final java.lang.Object $application = this.getApplication();
result = result * PRIME + ($application == null ? 43 : $application.hashCode());
final java.lang.Object $businessProfile = this.getBusinessProfile();
result = result * PRIME + ($businessProfile == null ? 43 : $businessProfile.hashCode());
final java.lang.Object $defaultReturnUrl = this.getDefaultReturnUrl();
result = result * PRIME + ($defaultReturnUrl == null ? 43 : $defaultReturnUrl.hashCode());
final java.lang.Object $features = this.getFeatures();
result = result * PRIME + ($features == null ? 43 : $features.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
}