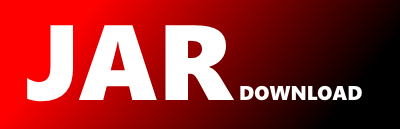
com.stripe.model.checkout.Session Maven / Gradle / Ivy
// Generated by delombok at Wed Jun 30 19:13:17 EDT 2021
// File generated from our OpenAPI spec
package com.stripe.model.checkout;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.model.Customer;
import com.stripe.model.ExpandableField;
import com.stripe.model.HasId;
import com.stripe.model.LineItem;
import com.stripe.model.LineItemCollection;
import com.stripe.model.PaymentIntent;
import com.stripe.model.SetupIntent;
import com.stripe.model.ShippingDetails;
import com.stripe.model.StripeObject;
import com.stripe.model.Subscription;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.checkout.SessionCreateParams;
import com.stripe.param.checkout.SessionListLineItemsParams;
import com.stripe.param.checkout.SessionListParams;
import com.stripe.param.checkout.SessionRetrieveParams;
import java.util.List;
import java.util.Map;
public class Session extends ApiResource implements HasId {
/**
* Enables user redeemable promotion codes.
*/
@SerializedName("allow_promotion_codes")
Boolean allowPromotionCodes;
/**
* Total of all items before discounts or taxes are applied.
*/
@SerializedName("amount_subtotal")
Long amountSubtotal;
/**
* Total of all items after discounts and taxes are applied.
*/
@SerializedName("amount_total")
Long amountTotal;
@SerializedName("automatic_tax")
AutomaticTax automaticTax;
/**
* Describes whether Checkout should collect the customer's billing address.
*
* One of {@code auto}, or {@code required}.
*/
@SerializedName("billing_address_collection")
String billingAddressCollection;
/**
* The URL the customer will be directed to if they decide to cancel payment and return to your
* website.
*/
@SerializedName("cancel_url")
String cancelUrl;
/**
* A unique string to reference the Checkout Session. This can be a customer ID, a cart ID, or
* similar, and can be used to reconcile the Session with your internal systems.
*/
@SerializedName("client_reference_id")
String clientReferenceId;
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@SerializedName("currency")
String currency;
/**
* The ID of the customer for this Session. For Checkout Sessions in {@code payment} or {@code
* subscription} mode, Checkout will create a new customer object based on information provided
* during the payment flow unless an existing customer was provided when the Session was created.
*/
@SerializedName("customer")
ExpandableField customer;
/**
* The customer details including the customer's tax exempt status and the customer's tax IDs.
* Only present on Sessions in {@code payment} or {@code subscription} mode.
*/
@SerializedName("customer_details")
CustomerDetails customerDetails;
/**
* If provided, this value will be used when the Customer object is created. If not provided,
* customers will be asked to enter their email address. Use this parameter to prefill customer
* data if you already have an email on file. To access information about the customer once the
* payment flow is complete, use the {@code customer} attribute.
*/
@SerializedName("customer_email")
String customerEmail;
/**
* Unique identifier for the object. Used to pass to {@code redirectToCheckout} in Stripe.js.
*/
@SerializedName("id")
String id;
/**
* The line items purchased by the customer.
*/
@SerializedName("line_items")
LineItemCollection lineItems;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* The IETF language tag of the locale Checkout is displayed in. If blank or {@code auto}, the
* browser's locale is used.
*
* One of {@code auto}, {@code bg}, {@code cs}, {@code da}, {@code de}, {@code el}, {@code en},
* {@code en-GB}, {@code es}, {@code es-419}, {@code et}, {@code fi}, {@code fr}, {@code fr-CA},
* {@code hu}, {@code id}, {@code it}, {@code ja}, {@code lt}, {@code lv}, {@code ms}, {@code mt},
* {@code nb}, {@code nl}, {@code pl}, {@code pt}, {@code pt-BR}, {@code ro}, {@code ru}, {@code
* sk}, {@code sl}, {@code sv}, {@code th}, {@code tr}, {@code zh}, {@code zh-HK}, or {@code
* zh-TW}.
*/
@SerializedName("locale")
String locale;
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* The mode of the Checkout Session.
*
* One of {@code payment}, {@code setup}, or {@code subscription}.
*/
@SerializedName("mode")
String mode;
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code checkout.session}.
*/
@SerializedName("object")
String object;
/**
* The ID of the PaymentIntent for Checkout Sessions in {@code payment} mode.
*/
@SerializedName("payment_intent")
ExpandableField paymentIntent;
/**
* Payment-method-specific configuration for the PaymentIntent or SetupIntent of this
* CheckoutSession.
*/
@SerializedName("payment_method_options")
PaymentMethodOptions paymentMethodOptions;
/**
* A list of the types of payment methods (e.g. card) this Checkout Session is allowed to accept.
*/
@SerializedName("payment_method_types")
List paymentMethodTypes;
/**
* The payment status of the Checkout Session, one of {@code paid}, {@code unpaid}, or {@code
* no_payment_required}. You can use this value to decide when to fulfill your customer's order.
*/
@SerializedName("payment_status")
String paymentStatus;
/**
* The ID of the SetupIntent for Checkout Sessions in {@code setup} mode.
*/
@SerializedName("setup_intent")
ExpandableField setupIntent;
/**
* Shipping information for this Checkout Session.
*/
@SerializedName("shipping")
ShippingDetails shipping;
/**
* When set, provides configuration for Checkout to collect a shipping address from a customer.
*/
@SerializedName("shipping_address_collection")
ShippingAddressCollection shippingAddressCollection;
/**
* Describes the type of transaction being performed by Checkout in order to customize relevant
* text on the page, such as the submit button. {@code submit_type} can only be specified on
* Checkout Sessions in {@code payment} mode, but not Checkout Sessions in {@code subscription} or
* {@code setup} mode.
*
* One of {@code auto}, {@code book}, {@code donate}, or {@code pay}.
*/
@SerializedName("submit_type")
String submitType;
/**
* The ID of the subscription for Checkout Sessions in {@code subscription} mode.
*/
@SerializedName("subscription")
ExpandableField subscription;
/**
* The URL the customer will be directed to after the payment or subscription creation is
* successful.
*/
@SerializedName("success_url")
String successUrl;
@SerializedName("tax_id_collection")
TaxIDCollection taxIdCollection;
/**
* Tax and discount details for the computed total amount.
*/
@SerializedName("total_details")
TotalDetails totalDetails;
/**
* The URL to the Checkout Session.
*/
@SerializedName("url")
String url;
/**
* Get ID of expandable {@code customer} object.
*/
public String getCustomer() {
return (this.customer != null) ? this.customer.getId() : null;
}
public void setCustomer(String id) {
this.customer = ApiResource.setExpandableFieldId(id, this.customer);
}
/**
* Get expanded {@code customer}.
*/
public Customer getCustomerObject() {
return (this.customer != null) ? this.customer.getExpanded() : null;
}
public void setCustomerObject(Customer expandableObject) {
this.customer = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code paymentIntent} object.
*/
public String getPaymentIntent() {
return (this.paymentIntent != null) ? this.paymentIntent.getId() : null;
}
public void setPaymentIntent(String id) {
this.paymentIntent = ApiResource.setExpandableFieldId(id, this.paymentIntent);
}
/**
* Get expanded {@code paymentIntent}.
*/
public PaymentIntent getPaymentIntentObject() {
return (this.paymentIntent != null) ? this.paymentIntent.getExpanded() : null;
}
public void setPaymentIntentObject(PaymentIntent expandableObject) {
this.paymentIntent = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code setupIntent} object.
*/
public String getSetupIntent() {
return (this.setupIntent != null) ? this.setupIntent.getId() : null;
}
public void setSetupIntent(String id) {
this.setupIntent = ApiResource.setExpandableFieldId(id, this.setupIntent);
}
/**
* Get expanded {@code setupIntent}.
*/
public SetupIntent getSetupIntentObject() {
return (this.setupIntent != null) ? this.setupIntent.getExpanded() : null;
}
public void setSetupIntentObject(SetupIntent expandableObject) {
this.setupIntent = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code subscription} object.
*/
public String getSubscription() {
return (this.subscription != null) ? this.subscription.getId() : null;
}
public void setSubscription(String id) {
this.subscription = ApiResource.setExpandableFieldId(id, this.subscription);
}
/**
* Get expanded {@code subscription}.
*/
public Subscription getSubscriptionObject() {
return (this.subscription != null) ? this.subscription.getExpanded() : null;
}
public void setSubscriptionObject(Subscription expandableObject) {
this.subscription = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Returns a list of Checkout Sessions.
*/
public static SessionCollection list(Map params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of Checkout Sessions.
*/
public static SessionCollection list(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/checkout/sessions");
return ApiResource.requestCollection(url, params, SessionCollection.class, options);
}
/**
* Returns a list of Checkout Sessions.
*/
public static SessionCollection list(SessionListParams params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of Checkout Sessions.
*/
public static SessionCollection list(SessionListParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/checkout/sessions");
return ApiResource.requestCollection(url, params, SessionCollection.class, options);
}
/**
* Retrieves a Session object.
*/
public static Session retrieve(String session) throws StripeException {
return retrieve(session, (Map) null, (RequestOptions) null);
}
/**
* Retrieves a Session object.
*/
public static Session retrieve(String session, RequestOptions options) throws StripeException {
return retrieve(session, (Map) null, options);
}
/**
* Retrieves a Session object.
*/
public static Session retrieve(String session, Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/checkout/sessions/%s", ApiResource.urlEncodeId(session)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Session.class, options);
}
/**
* Retrieves a Session object.
*/
public static Session retrieve(String session, SessionRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/checkout/sessions/%s", ApiResource.urlEncodeId(session)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Session.class, options);
}
/**
* Creates a Session object.
*/
public static Session create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Creates a Session object.
*/
public static Session create(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/checkout/sessions");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Session.class, options);
}
/**
* Creates a Session object.
*/
public static Session create(SessionCreateParams params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Creates a Session object.
*/
public static Session create(SessionCreateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/checkout/sessions");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Session.class, options);
}
/**
* Returns a list of Line Items
*/
public LineItemCollection listLineItems(Map params) throws StripeException {
return listLineItems(params, (RequestOptions) null);
}
/**
* Returns a list of Line Items
*/
public LineItemCollection listLineItems(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/checkout/sessions/%s/line_items", ApiResource.urlEncodeId(this.getId())));
return ApiResource.requestCollection(url, params, LineItemCollection.class, options);
}
/**
* Returns a list of Line Items
*/
public LineItemCollection listLineItems(SessionListLineItemsParams params) throws StripeException {
return listLineItems(params, (RequestOptions) null);
}
/**
* Returns a list of Line Items
*/
public LineItemCollection listLineItems(SessionListLineItemsParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/checkout/sessions/%s/line_items", ApiResource.urlEncodeId(this.getId())));
return ApiResource.requestCollection(url, params, LineItemCollection.class, options);
}
public static class AutomaticTax extends StripeObject {
/**
* Indicates whether automatic tax is enabled for the session.
*/
@SerializedName("enabled")
Boolean enabled;
/**
* The status of the most recent automated tax calculation for this session.
*
* One of {@code complete}, {@code failed}, or {@code requires_location_inputs}.
*/
@SerializedName("status")
String status;
/**
* Indicates whether automatic tax is enabled for the session.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getEnabled() {
return this.enabled;
}
/**
* The status of the most recent automated tax calculation for this session.
*
*
One of {@code complete}, {@code failed}, or {@code requires_location_inputs}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
/**
* Indicates whether automatic tax is enabled for the session.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEnabled(final Boolean enabled) {
this.enabled = enabled;
}
/**
* The status of the most recent automated tax calculation for this session.
*
*
One of {@code complete}, {@code failed}, or {@code requires_location_inputs}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Session.AutomaticTax)) return false;
final Session.AutomaticTax other = (Session.AutomaticTax) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$enabled = this.getEnabled();
final java.lang.Object other$enabled = other.getEnabled();
if (this$enabled == null ? other$enabled != null : !this$enabled.equals(other$enabled)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Session.AutomaticTax;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $enabled = this.getEnabled();
result = result * PRIME + ($enabled == null ? 43 : $enabled.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
return result;
}
}
public static class CustomerDetails extends StripeObject {
/**
* The customer’s email at time of checkout.
*/
@SerializedName("email")
String email;
/**
* The customer’s tax exempt status at time of checkout.
*
*
One of {@code exempt}, {@code none}, or {@code reverse}.
*/
@SerializedName("tax_exempt")
String taxExempt;
/**
* The customer’s tax IDs at time of checkout.
*/
@SerializedName("tax_ids")
List taxIds;
public static class TaxID extends StripeObject {
/**
* The type of the tax ID, one of {@code eu_vat}, {@code br_cnpj}, {@code br_cpf}, {@code
* gb_vat}, {@code nz_gst}, {@code au_abn}, {@code in_gst}, {@code no_vat}, {@code za_vat},
* {@code ch_vat}, {@code mx_rfc}, {@code sg_uen}, {@code ru_inn}, {@code ru_kpp}, {@code
* ca_bn}, {@code hk_br}, {@code es_cif}, {@code tw_vat}, {@code th_vat}, {@code jp_cn},
* {@code jp_rn}, {@code li_uid}, {@code my_itn}, {@code us_ein}, {@code kr_brn}, {@code
* ca_qst}, {@code ca_gst_hst}, {@code ca_pst_bc}, {@code ca_pst_mb}, {@code ca_pst_sk},
* {@code my_sst}, {@code sg_gst}, {@code ae_trn}, {@code cl_tin}, {@code sa_vat}, {@code
* id_npwp}, {@code my_frp}, {@code il_vat}, or {@code unknown}.
*/
@SerializedName("type")
String type;
@SerializedName("value")
String value;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setValue(final String value) {
this.value = value;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Session.CustomerDetails.TaxID)) return false;
final Session.CustomerDetails.TaxID other = (Session.CustomerDetails.TaxID) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final java.lang.Object this$value = this.getValue();
final java.lang.Object other$value = other.getValue();
if (this$value == null ? other$value != null : !this$value.equals(other$value)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Session.CustomerDetails.TaxID;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final java.lang.Object $value = this.getValue();
result = result * PRIME + ($value == null ? 43 : $value.hashCode());
return result;
}
}
/**
* The customer’s email at time of checkout.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getEmail() {
return this.email;
}
/**
* The customer’s tax exempt status at time of checkout.
*
* One of {@code exempt}, {@code none}, or {@code reverse}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTaxExempt() {
return this.taxExempt;
}
/**
* The customer’s tax IDs at time of checkout.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getTaxIds() {
return this.taxIds;
}
/**
* The customer’s email at time of checkout.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEmail(final String email) {
this.email = email;
}
/**
* The customer’s tax exempt status at time of checkout.
*
* One of {@code exempt}, {@code none}, or {@code reverse}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxExempt(final String taxExempt) {
this.taxExempt = taxExempt;
}
/**
* The customer’s tax IDs at time of checkout.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxIds(final List taxIds) {
this.taxIds = taxIds;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Session.CustomerDetails)) return false;
final Session.CustomerDetails other = (Session.CustomerDetails) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$email = this.getEmail();
final java.lang.Object other$email = other.getEmail();
if (this$email == null ? other$email != null : !this$email.equals(other$email)) return false;
final java.lang.Object this$taxExempt = this.getTaxExempt();
final java.lang.Object other$taxExempt = other.getTaxExempt();
if (this$taxExempt == null ? other$taxExempt != null : !this$taxExempt.equals(other$taxExempt)) return false;
final java.lang.Object this$taxIds = this.getTaxIds();
final java.lang.Object other$taxIds = other.getTaxIds();
if (this$taxIds == null ? other$taxIds != null : !this$taxIds.equals(other$taxIds)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Session.CustomerDetails;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $email = this.getEmail();
result = result * PRIME + ($email == null ? 43 : $email.hashCode());
final java.lang.Object $taxExempt = this.getTaxExempt();
result = result * PRIME + ($taxExempt == null ? 43 : $taxExempt.hashCode());
final java.lang.Object $taxIds = this.getTaxIds();
result = result * PRIME + ($taxIds == null ? 43 : $taxIds.hashCode());
return result;
}
}
/**
* The value of the tax ID.
*/
public static class PaymentMethodOptions extends StripeObject {
@SerializedName("acss_debit")
AcssDebit acssDebit;
@SerializedName("boleto")
Boleto boleto;
@SerializedName("oxxo")
Oxxo oxxo;
public static class AcssDebit extends StripeObject {
/**
* Currency supported by the bank account. Returned when the Session is in {@code setup} mode.
*
* One of {@code cad}, or {@code usd}.
*/
@SerializedName("currency")
String currency;
@SerializedName("mandate_options")
MandateOptions mandateOptions;
/**
* Bank account verification method.
*
*
One of {@code automatic}, {@code instant}, or {@code microdeposits}.
*/
@SerializedName("verification_method")
String verificationMethod;
public static class MandateOptions extends StripeObject {
/** A URL for custom mandate text. */
@SerializedName("custom_mandate_url")
String customMandateUrl;
/**
* Description of the interval. Only required if 'payment_schedule' parmeter is 'interval'
* or 'combined'.
*/
@SerializedName("interval_description")
String intervalDescription;
/**
* Payment schedule for the mandate.
*
*
One of {@code combined}, {@code interval}, or {@code sporadic}.
*/
@SerializedName("payment_schedule")
String paymentSchedule;
@SerializedName("transaction_type")
String transactionType;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCustomMandateUrl() {
return this.customMandateUrl;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getIntervalDescription() {
return this.intervalDescription;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPaymentSchedule() {
return this.paymentSchedule;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTransactionType() {
return this.transactionType;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCustomMandateUrl(final String customMandateUrl) {
this.customMandateUrl = customMandateUrl;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIntervalDescription(final String intervalDescription) {
this.intervalDescription = intervalDescription;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPaymentSchedule(final String paymentSchedule) {
this.paymentSchedule = paymentSchedule;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTransactionType(final String transactionType) {
this.transactionType = transactionType;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Session.PaymentMethodOptions.AcssDebit.MandateOptions)) return false;
final Session.PaymentMethodOptions.AcssDebit.MandateOptions other = (Session.PaymentMethodOptions.AcssDebit.MandateOptions) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$customMandateUrl = this.getCustomMandateUrl();
final java.lang.Object other$customMandateUrl = other.getCustomMandateUrl();
if (this$customMandateUrl == null ? other$customMandateUrl != null : !this$customMandateUrl.equals(other$customMandateUrl)) return false;
final java.lang.Object this$intervalDescription = this.getIntervalDescription();
final java.lang.Object other$intervalDescription = other.getIntervalDescription();
if (this$intervalDescription == null ? other$intervalDescription != null : !this$intervalDescription.equals(other$intervalDescription)) return false;
final java.lang.Object this$paymentSchedule = this.getPaymentSchedule();
final java.lang.Object other$paymentSchedule = other.getPaymentSchedule();
if (this$paymentSchedule == null ? other$paymentSchedule != null : !this$paymentSchedule.equals(other$paymentSchedule)) return false;
final java.lang.Object this$transactionType = this.getTransactionType();
final java.lang.Object other$transactionType = other.getTransactionType();
if (this$transactionType == null ? other$transactionType != null : !this$transactionType.equals(other$transactionType)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Session.PaymentMethodOptions.AcssDebit.MandateOptions;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $customMandateUrl = this.getCustomMandateUrl();
result = result * PRIME + ($customMandateUrl == null ? 43 : $customMandateUrl.hashCode());
final java.lang.Object $intervalDescription = this.getIntervalDescription();
result = result * PRIME + ($intervalDescription == null ? 43 : $intervalDescription.hashCode());
final java.lang.Object $paymentSchedule = this.getPaymentSchedule();
result = result * PRIME + ($paymentSchedule == null ? 43 : $paymentSchedule.hashCode());
final java.lang.Object $transactionType = this.getTransactionType();
result = result * PRIME + ($transactionType == null ? 43 : $transactionType.hashCode());
return result;
}
}
/**
* Currency supported by the bank account. Returned when the Session is in {@code setup} mode.
*
*
One of {@code cad}, or {@code usd}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public MandateOptions getMandateOptions() {
return this.mandateOptions;
}
/**
* Bank account verification method.
*
*
One of {@code automatic}, {@code instant}, or {@code microdeposits}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getVerificationMethod() {
return this.verificationMethod;
}
/**
* Currency supported by the bank account. Returned when the Session is in {@code setup} mode.
*
*
One of {@code cad}, or {@code usd}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMandateOptions(final MandateOptions mandateOptions) {
this.mandateOptions = mandateOptions;
}
/**
* Bank account verification method.
*
*
One of {@code automatic}, {@code instant}, or {@code microdeposits}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVerificationMethod(final String verificationMethod) {
this.verificationMethod = verificationMethod;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Session.PaymentMethodOptions.AcssDebit)) return false;
final Session.PaymentMethodOptions.AcssDebit other = (Session.PaymentMethodOptions.AcssDebit) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
final java.lang.Object this$mandateOptions = this.getMandateOptions();
final java.lang.Object other$mandateOptions = other.getMandateOptions();
if (this$mandateOptions == null ? other$mandateOptions != null : !this$mandateOptions.equals(other$mandateOptions)) return false;
final java.lang.Object this$verificationMethod = this.getVerificationMethod();
final java.lang.Object other$verificationMethod = other.getVerificationMethod();
if (this$verificationMethod == null ? other$verificationMethod != null : !this$verificationMethod.equals(other$verificationMethod)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Session.PaymentMethodOptions.AcssDebit;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
final java.lang.Object $mandateOptions = this.getMandateOptions();
result = result * PRIME + ($mandateOptions == null ? 43 : $mandateOptions.hashCode());
final java.lang.Object $verificationMethod = this.getVerificationMethod();
result = result * PRIME + ($verificationMethod == null ? 43 : $verificationMethod.hashCode());
return result;
}
}
/**
* Transaction type of the mandate.
*
*
One of {@code business}, or {@code personal}.
*/
public static class Boleto extends StripeObject {
/**
* The number of calendar days before a Boleto voucher expires. For example, if you create a
* Boleto voucher on Monday and you set expires_after_days to 2, the Boleto voucher will
* expire on Wednesday at 23:59 America/Sao_Paulo time.
*/
@SerializedName("expires_after_days")
Long expiresAfterDays;
/**
* The number of calendar days before a Boleto voucher expires. For example, if you create a
* Boleto voucher on Monday and you set expires_after_days to 2, the Boleto voucher will
* expire on Wednesday at 23:59 America/Sao_Paulo time.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getExpiresAfterDays() {
return this.expiresAfterDays;
}
/**
* The number of calendar days before a Boleto voucher expires. For example, if you create a
* Boleto voucher on Monday and you set expires_after_days to 2, the Boleto voucher will
* expire on Wednesday at 23:59 America/Sao_Paulo time.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setExpiresAfterDays(final Long expiresAfterDays) {
this.expiresAfterDays = expiresAfterDays;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Session.PaymentMethodOptions.Boleto)) return false;
final Session.PaymentMethodOptions.Boleto other = (Session.PaymentMethodOptions.Boleto) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$expiresAfterDays = this.getExpiresAfterDays();
final java.lang.Object other$expiresAfterDays = other.getExpiresAfterDays();
if (this$expiresAfterDays == null ? other$expiresAfterDays != null : !this$expiresAfterDays.equals(other$expiresAfterDays)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Session.PaymentMethodOptions.Boleto;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $expiresAfterDays = this.getExpiresAfterDays();
result = result * PRIME + ($expiresAfterDays == null ? 43 : $expiresAfterDays.hashCode());
return result;
}
}
public static class Oxxo extends StripeObject {
@SerializedName("expires_after_days")
Long expiresAfterDays;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getExpiresAfterDays() {
return this.expiresAfterDays;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setExpiresAfterDays(final Long expiresAfterDays) {
this.expiresAfterDays = expiresAfterDays;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Session.PaymentMethodOptions.Oxxo)) return false;
final Session.PaymentMethodOptions.Oxxo other = (Session.PaymentMethodOptions.Oxxo) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$expiresAfterDays = this.getExpiresAfterDays();
final java.lang.Object other$expiresAfterDays = other.getExpiresAfterDays();
if (this$expiresAfterDays == null ? other$expiresAfterDays != null : !this$expiresAfterDays.equals(other$expiresAfterDays)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Session.PaymentMethodOptions.Oxxo;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $expiresAfterDays = this.getExpiresAfterDays();
result = result * PRIME + ($expiresAfterDays == null ? 43 : $expiresAfterDays.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public AcssDebit getAcssDebit() {
return this.acssDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boleto getBoleto() {
return this.boleto;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Oxxo getOxxo() {
return this.oxxo;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAcssDebit(final AcssDebit acssDebit) {
this.acssDebit = acssDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBoleto(final Boleto boleto) {
this.boleto = boleto;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setOxxo(final Oxxo oxxo) {
this.oxxo = oxxo;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Session.PaymentMethodOptions)) return false;
final Session.PaymentMethodOptions other = (Session.PaymentMethodOptions) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$acssDebit = this.getAcssDebit();
final java.lang.Object other$acssDebit = other.getAcssDebit();
if (this$acssDebit == null ? other$acssDebit != null : !this$acssDebit.equals(other$acssDebit)) return false;
final java.lang.Object this$boleto = this.getBoleto();
final java.lang.Object other$boleto = other.getBoleto();
if (this$boleto == null ? other$boleto != null : !this$boleto.equals(other$boleto)) return false;
final java.lang.Object this$oxxo = this.getOxxo();
final java.lang.Object other$oxxo = other.getOxxo();
if (this$oxxo == null ? other$oxxo != null : !this$oxxo.equals(other$oxxo)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Session.PaymentMethodOptions;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $acssDebit = this.getAcssDebit();
result = result * PRIME + ($acssDebit == null ? 43 : $acssDebit.hashCode());
final java.lang.Object $boleto = this.getBoleto();
result = result * PRIME + ($boleto == null ? 43 : $boleto.hashCode());
final java.lang.Object $oxxo = this.getOxxo();
result = result * PRIME + ($oxxo == null ? 43 : $oxxo.hashCode());
return result;
}
}
/**
* The number of calendar days before an OXXO invoice expires. For example, if you create an
* OXXO invoice on Monday and you set expires_after_days to 2, the OXXO invoice will expire on
* Wednesday at 23:59 America/Mexico_City time.
*/
public static class ShippingAddressCollection extends StripeObject {
/**
* An array of two-letter ISO country codes representing which countries Checkout should provide
* as options for shipping locations. Unsupported country codes: {@code AS, CX, CC, CU, HM, IR,
* KP, MH, FM, NF, MP, PW, SD, SY, UM, VI}.
*/
@SerializedName("allowed_countries")
List allowedCountries;
/**
* An array of two-letter ISO country codes representing which countries Checkout should provide
* as options for shipping locations. Unsupported country codes: {@code AS, CX, CC, CU, HM, IR,
* KP, MH, FM, NF, MP, PW, SD, SY, UM, VI}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getAllowedCountries() {
return this.allowedCountries;
}
/**
* An array of two-letter ISO country codes representing which countries Checkout should provide
* as options for shipping locations. Unsupported country codes: {@code AS, CX, CC, CU, HM, IR,
* KP, MH, FM, NF, MP, PW, SD, SY, UM, VI}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAllowedCountries(final List allowedCountries) {
this.allowedCountries = allowedCountries;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Session.ShippingAddressCollection)) return false;
final Session.ShippingAddressCollection other = (Session.ShippingAddressCollection) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$allowedCountries = this.getAllowedCountries();
final java.lang.Object other$allowedCountries = other.getAllowedCountries();
if (this$allowedCountries == null ? other$allowedCountries != null : !this$allowedCountries.equals(other$allowedCountries)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Session.ShippingAddressCollection;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $allowedCountries = this.getAllowedCountries();
result = result * PRIME + ($allowedCountries == null ? 43 : $allowedCountries.hashCode());
return result;
}
}
public static class TaxIDCollection extends StripeObject {
/**
* Indicates whether tax ID collection is enabled for the session.
*/
@SerializedName("enabled")
Boolean enabled;
/**
* Indicates whether tax ID collection is enabled for the session.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getEnabled() {
return this.enabled;
}
/**
* Indicates whether tax ID collection is enabled for the session.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEnabled(final Boolean enabled) {
this.enabled = enabled;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Session.TaxIDCollection)) return false;
final Session.TaxIDCollection other = (Session.TaxIDCollection) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$enabled = this.getEnabled();
final java.lang.Object other$enabled = other.getEnabled();
if (this$enabled == null ? other$enabled != null : !this$enabled.equals(other$enabled)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Session.TaxIDCollection;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $enabled = this.getEnabled();
result = result * PRIME + ($enabled == null ? 43 : $enabled.hashCode());
return result;
}
}
public static class TotalDetails extends StripeObject {
/** This is the sum of all the line item discounts. */
@SerializedName("amount_discount")
Long amountDiscount;
/** This is the sum of all the line item shipping amounts. */
@SerializedName("amount_shipping")
Long amountShipping;
/** This is the sum of all the line item tax amounts. */
@SerializedName("amount_tax")
Long amountTax;
@SerializedName("breakdown")
Breakdown breakdown;
public static class Breakdown extends StripeObject {
/** The aggregated line item discounts. */
@SerializedName("discounts")
List discounts;
/** The aggregated line item tax amounts by rate. */
@SerializedName("taxes")
List taxes;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getDiscounts() {
return this.discounts;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getTaxes() {
return this.taxes;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDiscounts(final List discounts) {
this.discounts = discounts;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxes(final List taxes) {
this.taxes = taxes;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Session.TotalDetails.Breakdown)) return false;
final Session.TotalDetails.Breakdown other = (Session.TotalDetails.Breakdown) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$discounts = this.getDiscounts();
final java.lang.Object other$discounts = other.getDiscounts();
if (this$discounts == null ? other$discounts != null : !this$discounts.equals(other$discounts)) return false;
final java.lang.Object this$taxes = this.getTaxes();
final java.lang.Object other$taxes = other.getTaxes();
if (this$taxes == null ? other$taxes != null : !this$taxes.equals(other$taxes)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Session.TotalDetails.Breakdown;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $discounts = this.getDiscounts();
result = result * PRIME + ($discounts == null ? 43 : $discounts.hashCode());
final java.lang.Object $taxes = this.getTaxes();
result = result * PRIME + ($taxes == null ? 43 : $taxes.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountDiscount() {
return this.amountDiscount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountShipping() {
return this.amountShipping;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountTax() {
return this.amountTax;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Breakdown getBreakdown() {
return this.breakdown;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountDiscount(final Long amountDiscount) {
this.amountDiscount = amountDiscount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountShipping(final Long amountShipping) {
this.amountShipping = amountShipping;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountTax(final Long amountTax) {
this.amountTax = amountTax;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBreakdown(final Breakdown breakdown) {
this.breakdown = breakdown;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Session.TotalDetails)) return false;
final Session.TotalDetails other = (Session.TotalDetails) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amountDiscount = this.getAmountDiscount();
final java.lang.Object other$amountDiscount = other.getAmountDiscount();
if (this$amountDiscount == null ? other$amountDiscount != null : !this$amountDiscount.equals(other$amountDiscount)) return false;
final java.lang.Object this$amountShipping = this.getAmountShipping();
final java.lang.Object other$amountShipping = other.getAmountShipping();
if (this$amountShipping == null ? other$amountShipping != null : !this$amountShipping.equals(other$amountShipping)) return false;
final java.lang.Object this$amountTax = this.getAmountTax();
final java.lang.Object other$amountTax = other.getAmountTax();
if (this$amountTax == null ? other$amountTax != null : !this$amountTax.equals(other$amountTax)) return false;
final java.lang.Object this$breakdown = this.getBreakdown();
final java.lang.Object other$breakdown = other.getBreakdown();
if (this$breakdown == null ? other$breakdown != null : !this$breakdown.equals(other$breakdown)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Session.TotalDetails;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amountDiscount = this.getAmountDiscount();
result = result * PRIME + ($amountDiscount == null ? 43 : $amountDiscount.hashCode());
final java.lang.Object $amountShipping = this.getAmountShipping();
result = result * PRIME + ($amountShipping == null ? 43 : $amountShipping.hashCode());
final java.lang.Object $amountTax = this.getAmountTax();
result = result * PRIME + ($amountTax == null ? 43 : $amountTax.hashCode());
final java.lang.Object $breakdown = this.getBreakdown();
result = result * PRIME + ($breakdown == null ? 43 : $breakdown.hashCode());
return result;
}
}
/**
* Enables user redeemable promotion codes.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getAllowPromotionCodes() {
return this.allowPromotionCodes;
}
/**
* Total of all items before discounts or taxes are applied.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountSubtotal() {
return this.amountSubtotal;
}
/**
* Total of all items after discounts and taxes are applied.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountTotal() {
return this.amountTotal;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public AutomaticTax getAutomaticTax() {
return this.automaticTax;
}
/**
* Describes whether Checkout should collect the customer's billing address.
*
* One of {@code auto}, or {@code required}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBillingAddressCollection() {
return this.billingAddressCollection;
}
/**
* The URL the customer will be directed to if they decide to cancel payment and return to your
* website.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCancelUrl() {
return this.cancelUrl;
}
/**
* A unique string to reference the Checkout Session. This can be a customer ID, a cart ID, or
* similar, and can be used to reconcile the Session with your internal systems.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getClientReferenceId() {
return this.clientReferenceId;
}
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
/**
* The customer details including the customer's tax exempt status and the customer's tax IDs.
* Only present on Sessions in {@code payment} or {@code subscription} mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public CustomerDetails getCustomerDetails() {
return this.customerDetails;
}
/**
* If provided, this value will be used when the Customer object is created. If not provided,
* customers will be asked to enter their email address. Use this parameter to prefill customer
* data if you already have an email on file. To access information about the customer once the
* payment flow is complete, use the {@code customer} attribute.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCustomerEmail() {
return this.customerEmail;
}
/**
* The line items purchased by the customer.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public LineItemCollection getLineItems() {
return this.lineItems;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* The IETF language tag of the locale Checkout is displayed in. If blank or {@code auto}, the
* browser's locale is used.
*
*
One of {@code auto}, {@code bg}, {@code cs}, {@code da}, {@code de}, {@code el}, {@code en},
* {@code en-GB}, {@code es}, {@code es-419}, {@code et}, {@code fi}, {@code fr}, {@code fr-CA},
* {@code hu}, {@code id}, {@code it}, {@code ja}, {@code lt}, {@code lv}, {@code ms}, {@code mt},
* {@code nb}, {@code nl}, {@code pl}, {@code pt}, {@code pt-BR}, {@code ro}, {@code ru}, {@code
* sk}, {@code sl}, {@code sv}, {@code th}, {@code tr}, {@code zh}, {@code zh-HK}, or {@code
* zh-TW}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLocale() {
return this.locale;
}
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
/**
* The mode of the Checkout Session.
*
* One of {@code payment}, {@code setup}, or {@code subscription}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getMode() {
return this.mode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code checkout.session}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* Payment-method-specific configuration for the PaymentIntent or SetupIntent of this
* CheckoutSession.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PaymentMethodOptions getPaymentMethodOptions() {
return this.paymentMethodOptions;
}
/**
* A list of the types of payment methods (e.g. card) this Checkout Session is allowed to accept.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getPaymentMethodTypes() {
return this.paymentMethodTypes;
}
/**
* The payment status of the Checkout Session, one of {@code paid}, {@code unpaid}, or {@code
* no_payment_required}. You can use this value to decide when to fulfill your customer's order.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPaymentStatus() {
return this.paymentStatus;
}
/**
* Shipping information for this Checkout Session.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ShippingDetails getShipping() {
return this.shipping;
}
/**
* When set, provides configuration for Checkout to collect a shipping address from a customer.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ShippingAddressCollection getShippingAddressCollection() {
return this.shippingAddressCollection;
}
/**
* Describes the type of transaction being performed by Checkout in order to customize relevant
* text on the page, such as the submit button. {@code submit_type} can only be specified on
* Checkout Sessions in {@code payment} mode, but not Checkout Sessions in {@code subscription} or
* {@code setup} mode.
*
* One of {@code auto}, {@code book}, {@code donate}, or {@code pay}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSubmitType() {
return this.submitType;
}
/**
* The URL the customer will be directed to after the payment or subscription creation is
* successful.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSuccessUrl() {
return this.successUrl;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TaxIDCollection getTaxIdCollection() {
return this.taxIdCollection;
}
/**
* Tax and discount details for the computed total amount.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TotalDetails getTotalDetails() {
return this.totalDetails;
}
/**
* The URL to the Checkout Session.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUrl() {
return this.url;
}
/**
* Enables user redeemable promotion codes.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAllowPromotionCodes(final Boolean allowPromotionCodes) {
this.allowPromotionCodes = allowPromotionCodes;
}
/**
* Total of all items before discounts or taxes are applied.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountSubtotal(final Long amountSubtotal) {
this.amountSubtotal = amountSubtotal;
}
/**
* Total of all items after discounts and taxes are applied.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountTotal(final Long amountTotal) {
this.amountTotal = amountTotal;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAutomaticTax(final AutomaticTax automaticTax) {
this.automaticTax = automaticTax;
}
/**
* Describes whether Checkout should collect the customer's billing address.
*
*
One of {@code auto}, or {@code required}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBillingAddressCollection(final String billingAddressCollection) {
this.billingAddressCollection = billingAddressCollection;
}
/**
* The URL the customer will be directed to if they decide to cancel payment and return to your
* website.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCancelUrl(final String cancelUrl) {
this.cancelUrl = cancelUrl;
}
/**
* A unique string to reference the Checkout Session. This can be a customer ID, a cart ID, or
* similar, and can be used to reconcile the Session with your internal systems.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setClientReferenceId(final String clientReferenceId) {
this.clientReferenceId = clientReferenceId;
}
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
/**
* The customer details including the customer's tax exempt status and the customer's tax IDs.
* Only present on Sessions in {@code payment} or {@code subscription} mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCustomerDetails(final CustomerDetails customerDetails) {
this.customerDetails = customerDetails;
}
/**
* If provided, this value will be used when the Customer object is created. If not provided,
* customers will be asked to enter their email address. Use this parameter to prefill customer
* data if you already have an email on file. To access information about the customer once the
* payment flow is complete, use the {@code customer} attribute.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCustomerEmail(final String customerEmail) {
this.customerEmail = customerEmail;
}
/**
* Unique identifier for the object. Used to pass to {@code redirectToCheckout} in Stripe.js.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* The line items purchased by the customer.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLineItems(final LineItemCollection lineItems) {
this.lineItems = lineItems;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* The IETF language tag of the locale Checkout is displayed in. If blank or {@code auto}, the
* browser's locale is used.
*
*
One of {@code auto}, {@code bg}, {@code cs}, {@code da}, {@code de}, {@code el}, {@code en},
* {@code en-GB}, {@code es}, {@code es-419}, {@code et}, {@code fi}, {@code fr}, {@code fr-CA},
* {@code hu}, {@code id}, {@code it}, {@code ja}, {@code lt}, {@code lv}, {@code ms}, {@code mt},
* {@code nb}, {@code nl}, {@code pl}, {@code pt}, {@code pt-BR}, {@code ro}, {@code ru}, {@code
* sk}, {@code sl}, {@code sv}, {@code th}, {@code tr}, {@code zh}, {@code zh-HK}, or {@code
* zh-TW}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLocale(final String locale) {
this.locale = locale;
}
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
/**
* The mode of the Checkout Session.
*
* One of {@code payment}, {@code setup}, or {@code subscription}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMode(final String mode) {
this.mode = mode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code checkout.session}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* Payment-method-specific configuration for the PaymentIntent or SetupIntent of this
* CheckoutSession.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPaymentMethodOptions(final PaymentMethodOptions paymentMethodOptions) {
this.paymentMethodOptions = paymentMethodOptions;
}
/**
* A list of the types of payment methods (e.g. card) this Checkout Session is allowed to accept.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPaymentMethodTypes(final List paymentMethodTypes) {
this.paymentMethodTypes = paymentMethodTypes;
}
/**
* The payment status of the Checkout Session, one of {@code paid}, {@code unpaid}, or {@code
* no_payment_required}. You can use this value to decide when to fulfill your customer's order.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPaymentStatus(final String paymentStatus) {
this.paymentStatus = paymentStatus;
}
/**
* Shipping information for this Checkout Session.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setShipping(final ShippingDetails shipping) {
this.shipping = shipping;
}
/**
* When set, provides configuration for Checkout to collect a shipping address from a customer.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setShippingAddressCollection(final ShippingAddressCollection shippingAddressCollection) {
this.shippingAddressCollection = shippingAddressCollection;
}
/**
* Describes the type of transaction being performed by Checkout in order to customize relevant
* text on the page, such as the submit button. {@code submit_type} can only be specified on
* Checkout Sessions in {@code payment} mode, but not Checkout Sessions in {@code subscription} or
* {@code setup} mode.
*
* One of {@code auto}, {@code book}, {@code donate}, or {@code pay}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSubmitType(final String submitType) {
this.submitType = submitType;
}
/**
* The URL the customer will be directed to after the payment or subscription creation is
* successful.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSuccessUrl(final String successUrl) {
this.successUrl = successUrl;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxIdCollection(final TaxIDCollection taxIdCollection) {
this.taxIdCollection = taxIdCollection;
}
/**
* Tax and discount details for the computed total amount.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTotalDetails(final TotalDetails totalDetails) {
this.totalDetails = totalDetails;
}
/**
* The URL to the Checkout Session.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUrl(final String url) {
this.url = url;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Session)) return false;
final Session other = (Session) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$allowPromotionCodes = this.getAllowPromotionCodes();
final java.lang.Object other$allowPromotionCodes = other.getAllowPromotionCodes();
if (this$allowPromotionCodes == null ? other$allowPromotionCodes != null : !this$allowPromotionCodes.equals(other$allowPromotionCodes)) return false;
final java.lang.Object this$amountSubtotal = this.getAmountSubtotal();
final java.lang.Object other$amountSubtotal = other.getAmountSubtotal();
if (this$amountSubtotal == null ? other$amountSubtotal != null : !this$amountSubtotal.equals(other$amountSubtotal)) return false;
final java.lang.Object this$amountTotal = this.getAmountTotal();
final java.lang.Object other$amountTotal = other.getAmountTotal();
if (this$amountTotal == null ? other$amountTotal != null : !this$amountTotal.equals(other$amountTotal)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$automaticTax = this.getAutomaticTax();
final java.lang.Object other$automaticTax = other.getAutomaticTax();
if (this$automaticTax == null ? other$automaticTax != null : !this$automaticTax.equals(other$automaticTax)) return false;
final java.lang.Object this$billingAddressCollection = this.getBillingAddressCollection();
final java.lang.Object other$billingAddressCollection = other.getBillingAddressCollection();
if (this$billingAddressCollection == null ? other$billingAddressCollection != null : !this$billingAddressCollection.equals(other$billingAddressCollection)) return false;
final java.lang.Object this$cancelUrl = this.getCancelUrl();
final java.lang.Object other$cancelUrl = other.getCancelUrl();
if (this$cancelUrl == null ? other$cancelUrl != null : !this$cancelUrl.equals(other$cancelUrl)) return false;
final java.lang.Object this$clientReferenceId = this.getClientReferenceId();
final java.lang.Object other$clientReferenceId = other.getClientReferenceId();
if (this$clientReferenceId == null ? other$clientReferenceId != null : !this$clientReferenceId.equals(other$clientReferenceId)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
final java.lang.Object this$customer = this.getCustomer();
final java.lang.Object other$customer = other.getCustomer();
if (this$customer == null ? other$customer != null : !this$customer.equals(other$customer)) return false;
final java.lang.Object this$customerDetails = this.getCustomerDetails();
final java.lang.Object other$customerDetails = other.getCustomerDetails();
if (this$customerDetails == null ? other$customerDetails != null : !this$customerDetails.equals(other$customerDetails)) return false;
final java.lang.Object this$customerEmail = this.getCustomerEmail();
final java.lang.Object other$customerEmail = other.getCustomerEmail();
if (this$customerEmail == null ? other$customerEmail != null : !this$customerEmail.equals(other$customerEmail)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$lineItems = this.getLineItems();
final java.lang.Object other$lineItems = other.getLineItems();
if (this$lineItems == null ? other$lineItems != null : !this$lineItems.equals(other$lineItems)) return false;
final java.lang.Object this$locale = this.getLocale();
final java.lang.Object other$locale = other.getLocale();
if (this$locale == null ? other$locale != null : !this$locale.equals(other$locale)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$mode = this.getMode();
final java.lang.Object other$mode = other.getMode();
if (this$mode == null ? other$mode != null : !this$mode.equals(other$mode)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$paymentIntent = this.getPaymentIntent();
final java.lang.Object other$paymentIntent = other.getPaymentIntent();
if (this$paymentIntent == null ? other$paymentIntent != null : !this$paymentIntent.equals(other$paymentIntent)) return false;
final java.lang.Object this$paymentMethodOptions = this.getPaymentMethodOptions();
final java.lang.Object other$paymentMethodOptions = other.getPaymentMethodOptions();
if (this$paymentMethodOptions == null ? other$paymentMethodOptions != null : !this$paymentMethodOptions.equals(other$paymentMethodOptions)) return false;
final java.lang.Object this$paymentMethodTypes = this.getPaymentMethodTypes();
final java.lang.Object other$paymentMethodTypes = other.getPaymentMethodTypes();
if (this$paymentMethodTypes == null ? other$paymentMethodTypes != null : !this$paymentMethodTypes.equals(other$paymentMethodTypes)) return false;
final java.lang.Object this$paymentStatus = this.getPaymentStatus();
final java.lang.Object other$paymentStatus = other.getPaymentStatus();
if (this$paymentStatus == null ? other$paymentStatus != null : !this$paymentStatus.equals(other$paymentStatus)) return false;
final java.lang.Object this$setupIntent = this.getSetupIntent();
final java.lang.Object other$setupIntent = other.getSetupIntent();
if (this$setupIntent == null ? other$setupIntent != null : !this$setupIntent.equals(other$setupIntent)) return false;
final java.lang.Object this$shipping = this.getShipping();
final java.lang.Object other$shipping = other.getShipping();
if (this$shipping == null ? other$shipping != null : !this$shipping.equals(other$shipping)) return false;
final java.lang.Object this$shippingAddressCollection = this.getShippingAddressCollection();
final java.lang.Object other$shippingAddressCollection = other.getShippingAddressCollection();
if (this$shippingAddressCollection == null ? other$shippingAddressCollection != null : !this$shippingAddressCollection.equals(other$shippingAddressCollection)) return false;
final java.lang.Object this$submitType = this.getSubmitType();
final java.lang.Object other$submitType = other.getSubmitType();
if (this$submitType == null ? other$submitType != null : !this$submitType.equals(other$submitType)) return false;
final java.lang.Object this$subscription = this.getSubscription();
final java.lang.Object other$subscription = other.getSubscription();
if (this$subscription == null ? other$subscription != null : !this$subscription.equals(other$subscription)) return false;
final java.lang.Object this$successUrl = this.getSuccessUrl();
final java.lang.Object other$successUrl = other.getSuccessUrl();
if (this$successUrl == null ? other$successUrl != null : !this$successUrl.equals(other$successUrl)) return false;
final java.lang.Object this$taxIdCollection = this.getTaxIdCollection();
final java.lang.Object other$taxIdCollection = other.getTaxIdCollection();
if (this$taxIdCollection == null ? other$taxIdCollection != null : !this$taxIdCollection.equals(other$taxIdCollection)) return false;
final java.lang.Object this$totalDetails = this.getTotalDetails();
final java.lang.Object other$totalDetails = other.getTotalDetails();
if (this$totalDetails == null ? other$totalDetails != null : !this$totalDetails.equals(other$totalDetails)) return false;
final java.lang.Object this$url = this.getUrl();
final java.lang.Object other$url = other.getUrl();
if (this$url == null ? other$url != null : !this$url.equals(other$url)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Session;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $allowPromotionCodes = this.getAllowPromotionCodes();
result = result * PRIME + ($allowPromotionCodes == null ? 43 : $allowPromotionCodes.hashCode());
final java.lang.Object $amountSubtotal = this.getAmountSubtotal();
result = result * PRIME + ($amountSubtotal == null ? 43 : $amountSubtotal.hashCode());
final java.lang.Object $amountTotal = this.getAmountTotal();
result = result * PRIME + ($amountTotal == null ? 43 : $amountTotal.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $automaticTax = this.getAutomaticTax();
result = result * PRIME + ($automaticTax == null ? 43 : $automaticTax.hashCode());
final java.lang.Object $billingAddressCollection = this.getBillingAddressCollection();
result = result * PRIME + ($billingAddressCollection == null ? 43 : $billingAddressCollection.hashCode());
final java.lang.Object $cancelUrl = this.getCancelUrl();
result = result * PRIME + ($cancelUrl == null ? 43 : $cancelUrl.hashCode());
final java.lang.Object $clientReferenceId = this.getClientReferenceId();
result = result * PRIME + ($clientReferenceId == null ? 43 : $clientReferenceId.hashCode());
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
final java.lang.Object $customer = this.getCustomer();
result = result * PRIME + ($customer == null ? 43 : $customer.hashCode());
final java.lang.Object $customerDetails = this.getCustomerDetails();
result = result * PRIME + ($customerDetails == null ? 43 : $customerDetails.hashCode());
final java.lang.Object $customerEmail = this.getCustomerEmail();
result = result * PRIME + ($customerEmail == null ? 43 : $customerEmail.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $lineItems = this.getLineItems();
result = result * PRIME + ($lineItems == null ? 43 : $lineItems.hashCode());
final java.lang.Object $locale = this.getLocale();
result = result * PRIME + ($locale == null ? 43 : $locale.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $mode = this.getMode();
result = result * PRIME + ($mode == null ? 43 : $mode.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $paymentIntent = this.getPaymentIntent();
result = result * PRIME + ($paymentIntent == null ? 43 : $paymentIntent.hashCode());
final java.lang.Object $paymentMethodOptions = this.getPaymentMethodOptions();
result = result * PRIME + ($paymentMethodOptions == null ? 43 : $paymentMethodOptions.hashCode());
final java.lang.Object $paymentMethodTypes = this.getPaymentMethodTypes();
result = result * PRIME + ($paymentMethodTypes == null ? 43 : $paymentMethodTypes.hashCode());
final java.lang.Object $paymentStatus = this.getPaymentStatus();
result = result * PRIME + ($paymentStatus == null ? 43 : $paymentStatus.hashCode());
final java.lang.Object $setupIntent = this.getSetupIntent();
result = result * PRIME + ($setupIntent == null ? 43 : $setupIntent.hashCode());
final java.lang.Object $shipping = this.getShipping();
result = result * PRIME + ($shipping == null ? 43 : $shipping.hashCode());
final java.lang.Object $shippingAddressCollection = this.getShippingAddressCollection();
result = result * PRIME + ($shippingAddressCollection == null ? 43 : $shippingAddressCollection.hashCode());
final java.lang.Object $submitType = this.getSubmitType();
result = result * PRIME + ($submitType == null ? 43 : $submitType.hashCode());
final java.lang.Object $subscription = this.getSubscription();
result = result * PRIME + ($subscription == null ? 43 : $subscription.hashCode());
final java.lang.Object $successUrl = this.getSuccessUrl();
result = result * PRIME + ($successUrl == null ? 43 : $successUrl.hashCode());
final java.lang.Object $taxIdCollection = this.getTaxIdCollection();
result = result * PRIME + ($taxIdCollection == null ? 43 : $taxIdCollection.hashCode());
final java.lang.Object $totalDetails = this.getTotalDetails();
result = result * PRIME + ($totalDetails == null ? 43 : $totalDetails.hashCode());
final java.lang.Object $url = this.getUrl();
result = result * PRIME + ($url == null ? 43 : $url.hashCode());
return result;
}
/**
* Unique identifier for the object. Used to pass to {@code redirectToCheckout} in Stripe.js.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
}