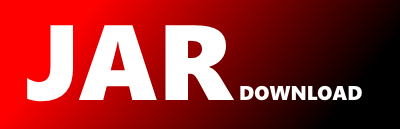
com.stripe.model.issuing.Transaction Maven / Gradle / Ivy
// Generated by delombok at Wed Jun 30 19:13:17 EDT 2021
// File generated from our OpenAPI spec
package com.stripe.model.issuing;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.model.BalanceTransaction;
import com.stripe.model.BalanceTransactionSource;
import com.stripe.model.ExpandableField;
import com.stripe.model.MetadataStore;
import com.stripe.model.StripeObject;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.issuing.TransactionListParams;
import com.stripe.param.issuing.TransactionRetrieveParams;
import com.stripe.param.issuing.TransactionUpdateParams;
import java.math.BigDecimal;
import java.util.List;
import java.util.Map;
public class Transaction extends ApiResource implements MetadataStore, BalanceTransactionSource {
/**
* The transaction amount, which will be reflected in your balance. This amount is in your
* currency and in the smallest currency
* unit.
*/
@SerializedName("amount")
Long amount;
/**
* Detailed breakdown of amount components. These amounts are denominated in {@code currency} and
* in the smallest currency unit.
*/
@SerializedName("amount_details")
AmountDetails amountDetails;
/**
* The {@code Authorization} object that led to this transaction.
*/
@SerializedName("authorization")
ExpandableField authorization;
/**
* ID of the balance transaction
* associated with this transaction.
*/
@SerializedName("balance_transaction")
ExpandableField balanceTransaction;
/**
* The card used to make this transaction.
*/
@SerializedName("card")
ExpandableField card;
/**
* The cardholder to whom this transaction belongs.
*/
@SerializedName("cardholder")
ExpandableField cardholder;
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@SerializedName("currency")
String currency;
/**
* If you've disputed the transaction, the ID of the dispute.
*/
@SerializedName("dispute")
ExpandableField dispute;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* The amount that the merchant will receive, denominated in {@code merchant_currency} and in the
* smallest currency unit. It will
* be different from {@code amount} if the merchant is taking payment in a different currency.
*/
@SerializedName("merchant_amount")
Long merchantAmount;
/**
* The currency with which the merchant is taking payment.
*/
@SerializedName("merchant_currency")
String merchantCurrency;
@SerializedName("merchant_data")
Authorization.MerchantData merchantData;
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code issuing.transaction}.
*/
@SerializedName("object")
String object;
/**
* Additional purchase information that is optionally provided by the merchant.
*/
@SerializedName("purchase_details")
PurchaseDetails purchaseDetails;
/**
* The nature of the transaction.
*
*
One of {@code capture}, or {@code refund}.
*/
@SerializedName("type")
String type;
/**
* Get ID of expandable {@code authorization} object.
*/
public String getAuthorization() {
return (this.authorization != null) ? this.authorization.getId() : null;
}
public void setAuthorization(String id) {
this.authorization = ApiResource.setExpandableFieldId(id, this.authorization);
}
/**
* Get expanded {@code authorization}.
*/
public Authorization getAuthorizationObject() {
return (this.authorization != null) ? this.authorization.getExpanded() : null;
}
public void setAuthorizationObject(Authorization expandableObject) {
this.authorization = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code balanceTransaction} object.
*/
public String getBalanceTransaction() {
return (this.balanceTransaction != null) ? this.balanceTransaction.getId() : null;
}
public void setBalanceTransaction(String id) {
this.balanceTransaction = ApiResource.setExpandableFieldId(id, this.balanceTransaction);
}
/**
* Get expanded {@code balanceTransaction}.
*/
public BalanceTransaction getBalanceTransactionObject() {
return (this.balanceTransaction != null) ? this.balanceTransaction.getExpanded() : null;
}
public void setBalanceTransactionObject(BalanceTransaction expandableObject) {
this.balanceTransaction = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code card} object.
*/
public String getCard() {
return (this.card != null) ? this.card.getId() : null;
}
public void setCard(String id) {
this.card = ApiResource.setExpandableFieldId(id, this.card);
}
/**
* Get expanded {@code card}.
*/
public Card getCardObject() {
return (this.card != null) ? this.card.getExpanded() : null;
}
public void setCardObject(Card expandableObject) {
this.card = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code cardholder} object.
*/
public String getCardholder() {
return (this.cardholder != null) ? this.cardholder.getId() : null;
}
public void setCardholder(String id) {
this.cardholder = ApiResource.setExpandableFieldId(id, this.cardholder);
}
/**
* Get expanded {@code cardholder}.
*/
public Cardholder getCardholderObject() {
return (this.cardholder != null) ? this.cardholder.getExpanded() : null;
}
public void setCardholderObject(Cardholder expandableObject) {
this.cardholder = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code dispute} object.
*/
public String getDispute() {
return (this.dispute != null) ? this.dispute.getId() : null;
}
public void setDispute(String id) {
this.dispute = ApiResource.setExpandableFieldId(id, this.dispute);
}
/**
* Get expanded {@code dispute}.
*/
public Dispute getDisputeObject() {
return (this.dispute != null) ? this.dispute.getExpanded() : null;
}
public void setDisputeObject(Dispute expandableObject) {
this.dispute = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Returns a list of Issuing Transaction
objects. The objects are sorted in
* descending order by creation date, with the most recently created object appearing first.
*/
public static TransactionCollection list(Map params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of Issuing Transaction
objects. The objects are sorted in
* descending order by creation date, with the most recently created object appearing first.
*/
public static TransactionCollection list(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/issuing/transactions");
return ApiResource.requestCollection(url, params, TransactionCollection.class, options);
}
/**
* Returns a list of Issuing Transaction
objects. The objects are sorted in
* descending order by creation date, with the most recently created object appearing first.
*/
public static TransactionCollection list(TransactionListParams params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of Issuing Transaction
objects. The objects are sorted in
* descending order by creation date, with the most recently created object appearing first.
*/
public static TransactionCollection list(TransactionListParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/issuing/transactions");
return ApiResource.requestCollection(url, params, TransactionCollection.class, options);
}
/**
* Retrieves an Issuing Transaction
object.
*/
public static Transaction retrieve(String transaction) throws StripeException {
return retrieve(transaction, (Map) null, (RequestOptions) null);
}
/**
* Retrieves an Issuing Transaction
object.
*/
public static Transaction retrieve(String transaction, RequestOptions options) throws StripeException {
return retrieve(transaction, (Map) null, options);
}
/**
* Retrieves an Issuing Transaction
object.
*/
public static Transaction retrieve(String transaction, Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/issuing/transactions/%s", ApiResource.urlEncodeId(transaction)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Transaction.class, options);
}
/**
* Retrieves an Issuing Transaction
object.
*/
public static Transaction retrieve(String transaction, TransactionRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/issuing/transactions/%s", ApiResource.urlEncodeId(transaction)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Transaction.class, options);
}
/**
* Updates the specified Issuing Transaction
object by setting the values of the
* parameters passed. Any parameters not provided will be left unchanged.
*/
@Override
public Transaction update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates the specified Issuing Transaction
object by setting the values of the
* parameters passed. Any parameters not provided will be left unchanged.
*/
@Override
public Transaction update(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/issuing/transactions/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Transaction.class, options);
}
/**
* Updates the specified Issuing Transaction
object by setting the values of the
* parameters passed. Any parameters not provided will be left unchanged.
*/
public Transaction update(TransactionUpdateParams params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates the specified Issuing Transaction
object by setting the values of the
* parameters passed. Any parameters not provided will be left unchanged.
*/
public Transaction update(TransactionUpdateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/issuing/transactions/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Transaction.class, options);
}
public static class AmountDetails extends StripeObject {
/**
* The fee charged by the ATM for the cash withdrawal.
*/
@SerializedName("atm_fee")
Long atmFee;
/**
* The fee charged by the ATM for the cash withdrawal.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAtmFee() {
return this.atmFee;
}
/**
* The fee charged by the ATM for the cash withdrawal.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAtmFee(final Long atmFee) {
this.atmFee = atmFee;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Transaction.AmountDetails)) return false;
final Transaction.AmountDetails other = (Transaction.AmountDetails) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$atmFee = this.getAtmFee();
final java.lang.Object other$atmFee = other.getAtmFee();
if (this$atmFee == null ? other$atmFee != null : !this$atmFee.equals(other$atmFee)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Transaction.AmountDetails;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $atmFee = this.getAtmFee();
result = result * PRIME + ($atmFee == null ? 43 : $atmFee.hashCode());
return result;
}
}
public static class PurchaseDetails extends StripeObject {
/** Information about the flight that was purchased with this transaction. */
@SerializedName("flight")
Flight flight;
/** Information about fuel that was purchased with this transaction. */
@SerializedName("fuel")
Fuel fuel;
/** Information about lodging that was purchased with this transaction. */
@SerializedName("lodging")
Lodging lodging;
/** The line items in the purchase. */
@SerializedName("receipt")
List receipt;
/** A merchant-specific order number. */
@SerializedName("reference")
String reference;
public static class Flight extends StripeObject {
/** The time that the flight departed. */
@SerializedName("departure_at")
Long departureAt;
/** The name of the passenger. */
@SerializedName("passenger_name")
String passengerName;
/** Whether the ticket is refundable. */
@SerializedName("refundable")
Boolean refundable;
/** The legs of the trip. */
@SerializedName("segments")
List segments;
/** The travel agency that issued the ticket. */
@SerializedName("travel_agency")
String travelAgency;
public static class Segments extends StripeObject {
/** The three-letter IATA airport code of the flight's destination. */
@SerializedName("arrival_airport_code")
String arrivalAirportCode;
/** The airline carrier code. */
@SerializedName("carrier")
String carrier;
/** The three-letter IATA airport code that the flight departed from. */
@SerializedName("departure_airport_code")
String departureAirportCode;
/** The flight number. */
@SerializedName("flight_number")
String flightNumber;
/** The flight's service class. */
@SerializedName("service_class")
String serviceClass;
/** Whether a stopover is allowed on this flight. */
@SerializedName("stopover_allowed")
Boolean stopoverAllowed;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getArrivalAirportCode() {
return this.arrivalAirportCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCarrier() {
return this.carrier;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDepartureAirportCode() {
return this.departureAirportCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFlightNumber() {
return this.flightNumber;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getServiceClass() {
return this.serviceClass;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getStopoverAllowed() {
return this.stopoverAllowed;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setArrivalAirportCode(final String arrivalAirportCode) {
this.arrivalAirportCode = arrivalAirportCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCarrier(final String carrier) {
this.carrier = carrier;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDepartureAirportCode(final String departureAirportCode) {
this.departureAirportCode = departureAirportCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFlightNumber(final String flightNumber) {
this.flightNumber = flightNumber;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setServiceClass(final String serviceClass) {
this.serviceClass = serviceClass;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStopoverAllowed(final Boolean stopoverAllowed) {
this.stopoverAllowed = stopoverAllowed;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Transaction.PurchaseDetails.Flight.Segments)) return false;
final Transaction.PurchaseDetails.Flight.Segments other = (Transaction.PurchaseDetails.Flight.Segments) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$stopoverAllowed = this.getStopoverAllowed();
final java.lang.Object other$stopoverAllowed = other.getStopoverAllowed();
if (this$stopoverAllowed == null ? other$stopoverAllowed != null : !this$stopoverAllowed.equals(other$stopoverAllowed)) return false;
final java.lang.Object this$arrivalAirportCode = this.getArrivalAirportCode();
final java.lang.Object other$arrivalAirportCode = other.getArrivalAirportCode();
if (this$arrivalAirportCode == null ? other$arrivalAirportCode != null : !this$arrivalAirportCode.equals(other$arrivalAirportCode)) return false;
final java.lang.Object this$carrier = this.getCarrier();
final java.lang.Object other$carrier = other.getCarrier();
if (this$carrier == null ? other$carrier != null : !this$carrier.equals(other$carrier)) return false;
final java.lang.Object this$departureAirportCode = this.getDepartureAirportCode();
final java.lang.Object other$departureAirportCode = other.getDepartureAirportCode();
if (this$departureAirportCode == null ? other$departureAirportCode != null : !this$departureAirportCode.equals(other$departureAirportCode)) return false;
final java.lang.Object this$flightNumber = this.getFlightNumber();
final java.lang.Object other$flightNumber = other.getFlightNumber();
if (this$flightNumber == null ? other$flightNumber != null : !this$flightNumber.equals(other$flightNumber)) return false;
final java.lang.Object this$serviceClass = this.getServiceClass();
final java.lang.Object other$serviceClass = other.getServiceClass();
if (this$serviceClass == null ? other$serviceClass != null : !this$serviceClass.equals(other$serviceClass)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Transaction.PurchaseDetails.Flight.Segments;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $stopoverAllowed = this.getStopoverAllowed();
result = result * PRIME + ($stopoverAllowed == null ? 43 : $stopoverAllowed.hashCode());
final java.lang.Object $arrivalAirportCode = this.getArrivalAirportCode();
result = result * PRIME + ($arrivalAirportCode == null ? 43 : $arrivalAirportCode.hashCode());
final java.lang.Object $carrier = this.getCarrier();
result = result * PRIME + ($carrier == null ? 43 : $carrier.hashCode());
final java.lang.Object $departureAirportCode = this.getDepartureAirportCode();
result = result * PRIME + ($departureAirportCode == null ? 43 : $departureAirportCode.hashCode());
final java.lang.Object $flightNumber = this.getFlightNumber();
result = result * PRIME + ($flightNumber == null ? 43 : $flightNumber.hashCode());
final java.lang.Object $serviceClass = this.getServiceClass();
result = result * PRIME + ($serviceClass == null ? 43 : $serviceClass.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDepartureAt() {
return this.departureAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPassengerName() {
return this.passengerName;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getRefundable() {
return this.refundable;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getSegments() {
return this.segments;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTravelAgency() {
return this.travelAgency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDepartureAt(final Long departureAt) {
this.departureAt = departureAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPassengerName(final String passengerName) {
this.passengerName = passengerName;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRefundable(final Boolean refundable) {
this.refundable = refundable;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSegments(final List segments) {
this.segments = segments;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTravelAgency(final String travelAgency) {
this.travelAgency = travelAgency;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Transaction.PurchaseDetails.Flight)) return false;
final Transaction.PurchaseDetails.Flight other = (Transaction.PurchaseDetails.Flight) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$departureAt = this.getDepartureAt();
final java.lang.Object other$departureAt = other.getDepartureAt();
if (this$departureAt == null ? other$departureAt != null : !this$departureAt.equals(other$departureAt)) return false;
final java.lang.Object this$refundable = this.getRefundable();
final java.lang.Object other$refundable = other.getRefundable();
if (this$refundable == null ? other$refundable != null : !this$refundable.equals(other$refundable)) return false;
final java.lang.Object this$passengerName = this.getPassengerName();
final java.lang.Object other$passengerName = other.getPassengerName();
if (this$passengerName == null ? other$passengerName != null : !this$passengerName.equals(other$passengerName)) return false;
final java.lang.Object this$segments = this.getSegments();
final java.lang.Object other$segments = other.getSegments();
if (this$segments == null ? other$segments != null : !this$segments.equals(other$segments)) return false;
final java.lang.Object this$travelAgency = this.getTravelAgency();
final java.lang.Object other$travelAgency = other.getTravelAgency();
if (this$travelAgency == null ? other$travelAgency != null : !this$travelAgency.equals(other$travelAgency)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Transaction.PurchaseDetails.Flight;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $departureAt = this.getDepartureAt();
result = result * PRIME + ($departureAt == null ? 43 : $departureAt.hashCode());
final java.lang.Object $refundable = this.getRefundable();
result = result * PRIME + ($refundable == null ? 43 : $refundable.hashCode());
final java.lang.Object $passengerName = this.getPassengerName();
result = result * PRIME + ($passengerName == null ? 43 : $passengerName.hashCode());
final java.lang.Object $segments = this.getSegments();
result = result * PRIME + ($segments == null ? 43 : $segments.hashCode());
final java.lang.Object $travelAgency = this.getTravelAgency();
result = result * PRIME + ($travelAgency == null ? 43 : $travelAgency.hashCode());
return result;
}
}
public static class Fuel extends StripeObject {
/**
* The type of fuel that was purchased. One of {@code diesel}, {@code unleaded_plus}, {@code
* unleaded_regular}, {@code unleaded_super}, or {@code other}.
*/
@SerializedName("type")
String type;
/** The units for {@code volume_decimal}. One of {@code us_gallon} or {@code liter}. */
@SerializedName("unit")
String unit;
/**
* The cost in cents per each unit of fuel, represented as a decimal string with at most 12
* decimal places.
*/
@SerializedName("unit_cost_decimal")
BigDecimal unitCostDecimal;
/**
* The volume of the fuel that was pumped, represented as a decimal string with at most 12
* decimal places.
*/
@SerializedName("volume_decimal")
BigDecimal volumeDecimal;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUnit() {
return this.unit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BigDecimal getUnitCostDecimal() {
return this.unitCostDecimal;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BigDecimal getVolumeDecimal() {
return this.volumeDecimal;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUnit(final String unit) {
this.unit = unit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUnitCostDecimal(final BigDecimal unitCostDecimal) {
this.unitCostDecimal = unitCostDecimal;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVolumeDecimal(final BigDecimal volumeDecimal) {
this.volumeDecimal = volumeDecimal;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Transaction.PurchaseDetails.Fuel)) return false;
final Transaction.PurchaseDetails.Fuel other = (Transaction.PurchaseDetails.Fuel) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final java.lang.Object this$unit = this.getUnit();
final java.lang.Object other$unit = other.getUnit();
if (this$unit == null ? other$unit != null : !this$unit.equals(other$unit)) return false;
final java.lang.Object this$unitCostDecimal = this.getUnitCostDecimal();
final java.lang.Object other$unitCostDecimal = other.getUnitCostDecimal();
if (this$unitCostDecimal == null ? other$unitCostDecimal != null : !this$unitCostDecimal.equals(other$unitCostDecimal)) return false;
final java.lang.Object this$volumeDecimal = this.getVolumeDecimal();
final java.lang.Object other$volumeDecimal = other.getVolumeDecimal();
if (this$volumeDecimal == null ? other$volumeDecimal != null : !this$volumeDecimal.equals(other$volumeDecimal)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Transaction.PurchaseDetails.Fuel;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final java.lang.Object $unit = this.getUnit();
result = result * PRIME + ($unit == null ? 43 : $unit.hashCode());
final java.lang.Object $unitCostDecimal = this.getUnitCostDecimal();
result = result * PRIME + ($unitCostDecimal == null ? 43 : $unitCostDecimal.hashCode());
final java.lang.Object $volumeDecimal = this.getVolumeDecimal();
result = result * PRIME + ($volumeDecimal == null ? 43 : $volumeDecimal.hashCode());
return result;
}
}
public static class Lodging extends StripeObject {
/** The time of checking into the lodging. */
@SerializedName("check_in_at")
Long checkInAt;
/** The number of nights stayed at the lodging. */
@SerializedName("nights")
Long nights;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCheckInAt() {
return this.checkInAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getNights() {
return this.nights;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCheckInAt(final Long checkInAt) {
this.checkInAt = checkInAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setNights(final Long nights) {
this.nights = nights;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Transaction.PurchaseDetails.Lodging)) return false;
final Transaction.PurchaseDetails.Lodging other = (Transaction.PurchaseDetails.Lodging) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$checkInAt = this.getCheckInAt();
final java.lang.Object other$checkInAt = other.getCheckInAt();
if (this$checkInAt == null ? other$checkInAt != null : !this$checkInAt.equals(other$checkInAt)) return false;
final java.lang.Object this$nights = this.getNights();
final java.lang.Object other$nights = other.getNights();
if (this$nights == null ? other$nights != null : !this$nights.equals(other$nights)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Transaction.PurchaseDetails.Lodging;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $checkInAt = this.getCheckInAt();
result = result * PRIME + ($checkInAt == null ? 43 : $checkInAt.hashCode());
final java.lang.Object $nights = this.getNights();
result = result * PRIME + ($nights == null ? 43 : $nights.hashCode());
return result;
}
}
public static class Receipt extends StripeObject {
/** The description of the item. The maximum length of this field is 26 characters. */
@SerializedName("description")
String description;
/** The quantity of the item. */
@SerializedName("quantity")
BigDecimal quantity;
/** The total for this line item in cents. */
@SerializedName("total")
Long total;
/** The unit cost of the item in cents. */
@SerializedName("unit_cost")
Long unitCost;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDescription() {
return this.description;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BigDecimal getQuantity() {
return this.quantity;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getTotal() {
return this.total;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getUnitCost() {
return this.unitCost;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDescription(final String description) {
this.description = description;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setQuantity(final BigDecimal quantity) {
this.quantity = quantity;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTotal(final Long total) {
this.total = total;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUnitCost(final Long unitCost) {
this.unitCost = unitCost;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Transaction.PurchaseDetails.Receipt)) return false;
final Transaction.PurchaseDetails.Receipt other = (Transaction.PurchaseDetails.Receipt) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$total = this.getTotal();
final java.lang.Object other$total = other.getTotal();
if (this$total == null ? other$total != null : !this$total.equals(other$total)) return false;
final java.lang.Object this$unitCost = this.getUnitCost();
final java.lang.Object other$unitCost = other.getUnitCost();
if (this$unitCost == null ? other$unitCost != null : !this$unitCost.equals(other$unitCost)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$quantity = this.getQuantity();
final java.lang.Object other$quantity = other.getQuantity();
if (this$quantity == null ? other$quantity != null : !this$quantity.equals(other$quantity)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Transaction.PurchaseDetails.Receipt;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $total = this.getTotal();
result = result * PRIME + ($total == null ? 43 : $total.hashCode());
final java.lang.Object $unitCost = this.getUnitCost();
result = result * PRIME + ($unitCost == null ? 43 : $unitCost.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $quantity = this.getQuantity();
result = result * PRIME + ($quantity == null ? 43 : $quantity.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Flight getFlight() {
return this.flight;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Fuel getFuel() {
return this.fuel;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Lodging getLodging() {
return this.lodging;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getReceipt() {
return this.receipt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReference() {
return this.reference;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFlight(final Flight flight) {
this.flight = flight;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFuel(final Fuel fuel) {
this.fuel = fuel;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLodging(final Lodging lodging) {
this.lodging = lodging;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReceipt(final List receipt) {
this.receipt = receipt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReference(final String reference) {
this.reference = reference;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Transaction.PurchaseDetails)) return false;
final Transaction.PurchaseDetails other = (Transaction.PurchaseDetails) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$flight = this.getFlight();
final java.lang.Object other$flight = other.getFlight();
if (this$flight == null ? other$flight != null : !this$flight.equals(other$flight)) return false;
final java.lang.Object this$fuel = this.getFuel();
final java.lang.Object other$fuel = other.getFuel();
if (this$fuel == null ? other$fuel != null : !this$fuel.equals(other$fuel)) return false;
final java.lang.Object this$lodging = this.getLodging();
final java.lang.Object other$lodging = other.getLodging();
if (this$lodging == null ? other$lodging != null : !this$lodging.equals(other$lodging)) return false;
final java.lang.Object this$receipt = this.getReceipt();
final java.lang.Object other$receipt = other.getReceipt();
if (this$receipt == null ? other$receipt != null : !this$receipt.equals(other$receipt)) return false;
final java.lang.Object this$reference = this.getReference();
final java.lang.Object other$reference = other.getReference();
if (this$reference == null ? other$reference != null : !this$reference.equals(other$reference)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Transaction.PurchaseDetails;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $flight = this.getFlight();
result = result * PRIME + ($flight == null ? 43 : $flight.hashCode());
final java.lang.Object $fuel = this.getFuel();
result = result * PRIME + ($fuel == null ? 43 : $fuel.hashCode());
final java.lang.Object $lodging = this.getLodging();
result = result * PRIME + ($lodging == null ? 43 : $lodging.hashCode());
final java.lang.Object $receipt = this.getReceipt();
result = result * PRIME + ($receipt == null ? 43 : $receipt.hashCode());
final java.lang.Object $reference = this.getReference();
result = result * PRIME + ($reference == null ? 43 : $reference.hashCode());
return result;
}
}
/**
* The transaction amount, which will be reflected in your balance. This amount is in your
* currency and in the smallest currency
* unit.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
/**
* Detailed breakdown of amount components. These amounts are denominated in {@code currency} and
* in the smallest currency unit.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public AmountDetails getAmountDetails() {
return this.amountDetails;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* The amount that the merchant will receive, denominated in {@code merchant_currency} and in the
* smallest currency unit. It will
* be different from {@code amount} if the merchant is taking payment in a different currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getMerchantAmount() {
return this.merchantAmount;
}
/**
* The currency with which the merchant is taking payment.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getMerchantCurrency() {
return this.merchantCurrency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Authorization.MerchantData getMerchantData() {
return this.merchantData;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code issuing.transaction}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* Additional purchase information that is optionally provided by the merchant.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PurchaseDetails getPurchaseDetails() {
return this.purchaseDetails;
}
/**
* The nature of the transaction.
*
*
One of {@code capture}, or {@code refund}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
/**
* The transaction amount, which will be reflected in your balance. This amount is in your
* currency and in the smallest currency
* unit.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmount(final Long amount) {
this.amount = amount;
}
/**
* Detailed breakdown of amount components. These amounts are denominated in {@code currency} and
* in the smallest currency unit.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountDetails(final AmountDetails amountDetails) {
this.amountDetails = amountDetails;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* The amount that the merchant will receive, denominated in {@code merchant_currency} and in the
* smallest currency unit. It will
* be different from {@code amount} if the merchant is taking payment in a different currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMerchantAmount(final Long merchantAmount) {
this.merchantAmount = merchantAmount;
}
/**
* The currency with which the merchant is taking payment.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMerchantCurrency(final String merchantCurrency) {
this.merchantCurrency = merchantCurrency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMerchantData(final Authorization.MerchantData merchantData) {
this.merchantData = merchantData;
}
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code issuing.transaction}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* Additional purchase information that is optionally provided by the merchant.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPurchaseDetails(final PurchaseDetails purchaseDetails) {
this.purchaseDetails = purchaseDetails;
}
/**
* The nature of the transaction.
*
*
One of {@code capture}, or {@code refund}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Transaction)) return false;
final Transaction other = (Transaction) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$merchantAmount = this.getMerchantAmount();
final java.lang.Object other$merchantAmount = other.getMerchantAmount();
if (this$merchantAmount == null ? other$merchantAmount != null : !this$merchantAmount.equals(other$merchantAmount)) return false;
final java.lang.Object this$amountDetails = this.getAmountDetails();
final java.lang.Object other$amountDetails = other.getAmountDetails();
if (this$amountDetails == null ? other$amountDetails != null : !this$amountDetails.equals(other$amountDetails)) return false;
final java.lang.Object this$authorization = this.getAuthorization();
final java.lang.Object other$authorization = other.getAuthorization();
if (this$authorization == null ? other$authorization != null : !this$authorization.equals(other$authorization)) return false;
final java.lang.Object this$balanceTransaction = this.getBalanceTransaction();
final java.lang.Object other$balanceTransaction = other.getBalanceTransaction();
if (this$balanceTransaction == null ? other$balanceTransaction != null : !this$balanceTransaction.equals(other$balanceTransaction)) return false;
final java.lang.Object this$card = this.getCard();
final java.lang.Object other$card = other.getCard();
if (this$card == null ? other$card != null : !this$card.equals(other$card)) return false;
final java.lang.Object this$cardholder = this.getCardholder();
final java.lang.Object other$cardholder = other.getCardholder();
if (this$cardholder == null ? other$cardholder != null : !this$cardholder.equals(other$cardholder)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
final java.lang.Object this$dispute = this.getDispute();
final java.lang.Object other$dispute = other.getDispute();
if (this$dispute == null ? other$dispute != null : !this$dispute.equals(other$dispute)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$merchantCurrency = this.getMerchantCurrency();
final java.lang.Object other$merchantCurrency = other.getMerchantCurrency();
if (this$merchantCurrency == null ? other$merchantCurrency != null : !this$merchantCurrency.equals(other$merchantCurrency)) return false;
final java.lang.Object this$merchantData = this.getMerchantData();
final java.lang.Object other$merchantData = other.getMerchantData();
if (this$merchantData == null ? other$merchantData != null : !this$merchantData.equals(other$merchantData)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$purchaseDetails = this.getPurchaseDetails();
final java.lang.Object other$purchaseDetails = other.getPurchaseDetails();
if (this$purchaseDetails == null ? other$purchaseDetails != null : !this$purchaseDetails.equals(other$purchaseDetails)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Transaction;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $merchantAmount = this.getMerchantAmount();
result = result * PRIME + ($merchantAmount == null ? 43 : $merchantAmount.hashCode());
final java.lang.Object $amountDetails = this.getAmountDetails();
result = result * PRIME + ($amountDetails == null ? 43 : $amountDetails.hashCode());
final java.lang.Object $authorization = this.getAuthorization();
result = result * PRIME + ($authorization == null ? 43 : $authorization.hashCode());
final java.lang.Object $balanceTransaction = this.getBalanceTransaction();
result = result * PRIME + ($balanceTransaction == null ? 43 : $balanceTransaction.hashCode());
final java.lang.Object $card = this.getCard();
result = result * PRIME + ($card == null ? 43 : $card.hashCode());
final java.lang.Object $cardholder = this.getCardholder();
result = result * PRIME + ($cardholder == null ? 43 : $cardholder.hashCode());
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
final java.lang.Object $dispute = this.getDispute();
result = result * PRIME + ($dispute == null ? 43 : $dispute.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $merchantCurrency = this.getMerchantCurrency();
result = result * PRIME + ($merchantCurrency == null ? 43 : $merchantCurrency.hashCode());
final java.lang.Object $merchantData = this.getMerchantData();
result = result * PRIME + ($merchantData == null ? 43 : $merchantData.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $purchaseDetails = this.getPurchaseDetails();
result = result * PRIME + ($purchaseDetails == null ? 43 : $purchaseDetails.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
}