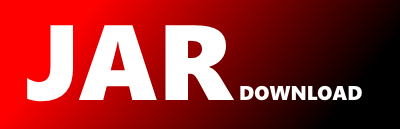
com.stripe.model.SetupAttempt Maven / Gradle / Ivy
// Generated by delombok at Thu Oct 07 18:42:34 EDT 2021
// File generated from our OpenAPI spec
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.SetupAttemptListParams;
import java.util.Map;
public class SetupAttempt extends ApiResource implements HasId {
/**
* The value of application
* on the SetupIntent at the time of this confirmation.
*/
@SerializedName("application")
ExpandableField application;
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* The value of customer
* on the SetupIntent at the time of this confirmation.
*/
@SerializedName("customer")
ExpandableField customer;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code setup_attempt}.
*/
@SerializedName("object")
String object;
/**
* The value of on_behalf_of
* on the SetupIntent at the time of this confirmation.
*/
@SerializedName("on_behalf_of")
ExpandableField onBehalfOf;
/**
* ID of the payment method used with this SetupAttempt.
*/
@SerializedName("payment_method")
ExpandableField paymentMethod;
@SerializedName("payment_method_details")
PaymentMethodDetails paymentMethodDetails;
/**
* The error encountered during this attempt to confirm the SetupIntent, if any.
*/
@SerializedName("setup_error")
StripeError setupError;
/**
* ID of the SetupIntent that this attempt belongs to.
*/
@SerializedName("setup_intent")
ExpandableField setupIntent;
/**
* Status of this SetupAttempt, one of {@code requires_confirmation}, {@code requires_action},
* {@code processing}, {@code succeeded}, {@code failed}, or {@code abandoned}.
*/
@SerializedName("status")
String status;
/**
* The value of usage on
* the SetupIntent at the time of this confirmation, one of {@code off_session} or {@code
* on_session}.
*/
@SerializedName("usage")
String usage;
/**
* Get ID of expandable {@code application} object.
*/
public String getApplication() {
return (this.application != null) ? this.application.getId() : null;
}
public void setApplication(String id) {
this.application = ApiResource.setExpandableFieldId(id, this.application);
}
/**
* Get expanded {@code application}.
*/
public Application getApplicationObject() {
return (this.application != null) ? this.application.getExpanded() : null;
}
public void setApplicationObject(Application expandableObject) {
this.application = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code customer} object.
*/
public String getCustomer() {
return (this.customer != null) ? this.customer.getId() : null;
}
public void setCustomer(String id) {
this.customer = ApiResource.setExpandableFieldId(id, this.customer);
}
/**
* Get expanded {@code customer}.
*/
public Customer getCustomerObject() {
return (this.customer != null) ? this.customer.getExpanded() : null;
}
public void setCustomerObject(Customer expandableObject) {
this.customer = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code onBehalfOf} object.
*/
public String getOnBehalfOf() {
return (this.onBehalfOf != null) ? this.onBehalfOf.getId() : null;
}
public void setOnBehalfOf(String id) {
this.onBehalfOf = ApiResource.setExpandableFieldId(id, this.onBehalfOf);
}
/**
* Get expanded {@code onBehalfOf}.
*/
public Account getOnBehalfOfObject() {
return (this.onBehalfOf != null) ? this.onBehalfOf.getExpanded() : null;
}
public void setOnBehalfOfObject(Account expandableObject) {
this.onBehalfOf = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code paymentMethod} object.
*/
public String getPaymentMethod() {
return (this.paymentMethod != null) ? this.paymentMethod.getId() : null;
}
public void setPaymentMethod(String id) {
this.paymentMethod = ApiResource.setExpandableFieldId(id, this.paymentMethod);
}
/**
* Get expanded {@code paymentMethod}.
*/
public PaymentMethod getPaymentMethodObject() {
return (this.paymentMethod != null) ? this.paymentMethod.getExpanded() : null;
}
public void setPaymentMethodObject(PaymentMethod expandableObject) {
this.paymentMethod = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code setupIntent} object.
*/
public String getSetupIntent() {
return (this.setupIntent != null) ? this.setupIntent.getId() : null;
}
public void setSetupIntent(String id) {
this.setupIntent = ApiResource.setExpandableFieldId(id, this.setupIntent);
}
/**
* Get expanded {@code setupIntent}.
*/
public SetupIntent getSetupIntentObject() {
return (this.setupIntent != null) ? this.setupIntent.getExpanded() : null;
}
public void setSetupIntentObject(SetupIntent expandableObject) {
this.setupIntent = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Returns a list of SetupAttempts associated with a provided SetupIntent.
*/
public static SetupAttemptCollection list(Map params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of SetupAttempts associated with a provided SetupIntent.
*/
public static SetupAttemptCollection list(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/setup_attempts");
return ApiResource.requestCollection(url, params, SetupAttemptCollection.class, options);
}
/**
* Returns a list of SetupAttempts associated with a provided SetupIntent.
*/
public static SetupAttemptCollection list(SetupAttemptListParams params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of SetupAttempts associated with a provided SetupIntent.
*/
public static SetupAttemptCollection list(SetupAttemptListParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/setup_attempts");
return ApiResource.requestCollection(url, params, SetupAttemptCollection.class, options);
}
public static class PaymentMethodDetails extends StripeObject {
@SerializedName("acss_debit")
AcssDebit acssDebit;
@SerializedName("au_becs_debit")
AuBecsDebit auBecsDebit;
@SerializedName("bacs_debit")
BacsDebit bacsDebit;
@SerializedName("bancontact")
Bancontact bancontact;
@SerializedName("card")
Card card;
@SerializedName("card_present")
CardPresent cardPresent;
@SerializedName("ideal")
Ideal ideal;
@SerializedName("sepa_debit")
SepaDebit sepaDebit;
@SerializedName("sofort")
Sofort sofort;
/**
* The type of the payment method used in the SetupIntent (e.g., {@code card}). An additional
* hash is included on {@code payment_method_details} with a name matching this value. It
* contains confirmation-specific information for the payment method.
*/
@SerializedName("type")
String type;
public static class AcssDebit extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SetupAttempt.PaymentMethodDetails.AcssDebit)) return false;
final SetupAttempt.PaymentMethodDetails.AcssDebit other = (SetupAttempt.PaymentMethodDetails.AcssDebit) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SetupAttempt.PaymentMethodDetails.AcssDebit;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class AuBecsDebit extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SetupAttempt.PaymentMethodDetails.AuBecsDebit)) return false;
final SetupAttempt.PaymentMethodDetails.AuBecsDebit other = (SetupAttempt.PaymentMethodDetails.AuBecsDebit) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SetupAttempt.PaymentMethodDetails.AuBecsDebit;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class BacsDebit extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SetupAttempt.PaymentMethodDetails.BacsDebit)) return false;
final SetupAttempt.PaymentMethodDetails.BacsDebit other = (SetupAttempt.PaymentMethodDetails.BacsDebit) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SetupAttempt.PaymentMethodDetails.BacsDebit;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class Bancontact extends StripeObject {
/** Bank code of bank associated with the bank account. */
@SerializedName("bank_code")
String bankCode;
/** Name of the bank associated with the bank account. */
@SerializedName("bank_name")
String bankName;
/** Bank Identifier Code of the bank associated with the bank account. */
@SerializedName("bic")
String bic;
/** The ID of the SEPA Direct Debit PaymentMethod which was generated by this SetupAttempt. */
@SerializedName("generated_sepa_debit")
ExpandableField generatedSepaDebit;
/**
* The mandate for the SEPA Direct Debit PaymentMethod which was generated by this
* SetupAttempt.
*/
@SerializedName("generated_sepa_debit_mandate")
ExpandableField generatedSepaDebitMandate;
/** Last four characters of the IBAN. */
@SerializedName("iban_last4")
String ibanLast4;
/**
* Preferred language of the Bancontact authorization page that the customer is redirected to.
* Can be one of {@code en}, {@code de}, {@code fr}, or {@code nl}
*/
@SerializedName("preferred_language")
String preferredLanguage;
/**
* Owner's verified full name. Values are verified or provided by Bancontact directly (if
* supported) at the time of authorization or settlement. They cannot be set or mutated.
*/
@SerializedName("verified_name")
String verifiedName;
/** Get ID of expandable {@code generatedSepaDebit} object. */
public String getGeneratedSepaDebit() {
return (this.generatedSepaDebit != null) ? this.generatedSepaDebit.getId() : null;
}
public void setGeneratedSepaDebit(String id) {
this.generatedSepaDebit = ApiResource.setExpandableFieldId(id, this.generatedSepaDebit);
}
/** Get expanded {@code generatedSepaDebit}. */
public PaymentMethod getGeneratedSepaDebitObject() {
return (this.generatedSepaDebit != null) ? this.generatedSepaDebit.getExpanded() : null;
}
public void setGeneratedSepaDebitObject(PaymentMethod expandableObject) {
this.generatedSepaDebit = new ExpandableField(expandableObject.getId(), expandableObject);
}
/** Get ID of expandable {@code generatedSepaDebitMandate} object. */
public String getGeneratedSepaDebitMandate() {
return (this.generatedSepaDebitMandate != null) ? this.generatedSepaDebitMandate.getId() : null;
}
public void setGeneratedSepaDebitMandate(String id) {
this.generatedSepaDebitMandate = ApiResource.setExpandableFieldId(id, this.generatedSepaDebitMandate);
}
/** Get expanded {@code generatedSepaDebitMandate}. */
public Mandate getGeneratedSepaDebitMandateObject() {
return (this.generatedSepaDebitMandate != null) ? this.generatedSepaDebitMandate.getExpanded() : null;
}
public void setGeneratedSepaDebitMandateObject(Mandate expandableObject) {
this.generatedSepaDebitMandate = new ExpandableField(expandableObject.getId(), expandableObject);
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBankCode() {
return this.bankCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBankName() {
return this.bankName;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBic() {
return this.bic;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getIbanLast4() {
return this.ibanLast4;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPreferredLanguage() {
return this.preferredLanguage;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getVerifiedName() {
return this.verifiedName;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBankCode(final String bankCode) {
this.bankCode = bankCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBankName(final String bankName) {
this.bankName = bankName;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBic(final String bic) {
this.bic = bic;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIbanLast4(final String ibanLast4) {
this.ibanLast4 = ibanLast4;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPreferredLanguage(final String preferredLanguage) {
this.preferredLanguage = preferredLanguage;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVerifiedName(final String verifiedName) {
this.verifiedName = verifiedName;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SetupAttempt.PaymentMethodDetails.Bancontact)) return false;
final SetupAttempt.PaymentMethodDetails.Bancontact other = (SetupAttempt.PaymentMethodDetails.Bancontact) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$bankCode = this.getBankCode();
final java.lang.Object other$bankCode = other.getBankCode();
if (this$bankCode == null ? other$bankCode != null : !this$bankCode.equals(other$bankCode)) return false;
final java.lang.Object this$bankName = this.getBankName();
final java.lang.Object other$bankName = other.getBankName();
if (this$bankName == null ? other$bankName != null : !this$bankName.equals(other$bankName)) return false;
final java.lang.Object this$bic = this.getBic();
final java.lang.Object other$bic = other.getBic();
if (this$bic == null ? other$bic != null : !this$bic.equals(other$bic)) return false;
final java.lang.Object this$generatedSepaDebit = this.getGeneratedSepaDebit();
final java.lang.Object other$generatedSepaDebit = other.getGeneratedSepaDebit();
if (this$generatedSepaDebit == null ? other$generatedSepaDebit != null : !this$generatedSepaDebit.equals(other$generatedSepaDebit)) return false;
final java.lang.Object this$generatedSepaDebitMandate = this.getGeneratedSepaDebitMandate();
final java.lang.Object other$generatedSepaDebitMandate = other.getGeneratedSepaDebitMandate();
if (this$generatedSepaDebitMandate == null ? other$generatedSepaDebitMandate != null : !this$generatedSepaDebitMandate.equals(other$generatedSepaDebitMandate)) return false;
final java.lang.Object this$ibanLast4 = this.getIbanLast4();
final java.lang.Object other$ibanLast4 = other.getIbanLast4();
if (this$ibanLast4 == null ? other$ibanLast4 != null : !this$ibanLast4.equals(other$ibanLast4)) return false;
final java.lang.Object this$preferredLanguage = this.getPreferredLanguage();
final java.lang.Object other$preferredLanguage = other.getPreferredLanguage();
if (this$preferredLanguage == null ? other$preferredLanguage != null : !this$preferredLanguage.equals(other$preferredLanguage)) return false;
final java.lang.Object this$verifiedName = this.getVerifiedName();
final java.lang.Object other$verifiedName = other.getVerifiedName();
if (this$verifiedName == null ? other$verifiedName != null : !this$verifiedName.equals(other$verifiedName)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SetupAttempt.PaymentMethodDetails.Bancontact;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $bankCode = this.getBankCode();
result = result * PRIME + ($bankCode == null ? 43 : $bankCode.hashCode());
final java.lang.Object $bankName = this.getBankName();
result = result * PRIME + ($bankName == null ? 43 : $bankName.hashCode());
final java.lang.Object $bic = this.getBic();
result = result * PRIME + ($bic == null ? 43 : $bic.hashCode());
final java.lang.Object $generatedSepaDebit = this.getGeneratedSepaDebit();
result = result * PRIME + ($generatedSepaDebit == null ? 43 : $generatedSepaDebit.hashCode());
final java.lang.Object $generatedSepaDebitMandate = this.getGeneratedSepaDebitMandate();
result = result * PRIME + ($generatedSepaDebitMandate == null ? 43 : $generatedSepaDebitMandate.hashCode());
final java.lang.Object $ibanLast4 = this.getIbanLast4();
result = result * PRIME + ($ibanLast4 == null ? 43 : $ibanLast4.hashCode());
final java.lang.Object $preferredLanguage = this.getPreferredLanguage();
result = result * PRIME + ($preferredLanguage == null ? 43 : $preferredLanguage.hashCode());
final java.lang.Object $verifiedName = this.getVerifiedName();
result = result * PRIME + ($verifiedName == null ? 43 : $verifiedName.hashCode());
return result;
}
}
public static class Card extends StripeObject {
/** Populated if this authorization used 3D Secure authentication. */
@SerializedName("three_d_secure")
Charge.PaymentMethodDetails.Card.ThreeDSecure threeDSecure;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Charge.PaymentMethodDetails.Card.ThreeDSecure getThreeDSecure() {
return this.threeDSecure;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setThreeDSecure(final Charge.PaymentMethodDetails.Card.ThreeDSecure threeDSecure) {
this.threeDSecure = threeDSecure;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SetupAttempt.PaymentMethodDetails.Card)) return false;
final SetupAttempt.PaymentMethodDetails.Card other = (SetupAttempt.PaymentMethodDetails.Card) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$threeDSecure = this.getThreeDSecure();
final java.lang.Object other$threeDSecure = other.getThreeDSecure();
if (this$threeDSecure == null ? other$threeDSecure != null : !this$threeDSecure.equals(other$threeDSecure)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SetupAttempt.PaymentMethodDetails.Card;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $threeDSecure = this.getThreeDSecure();
result = result * PRIME + ($threeDSecure == null ? 43 : $threeDSecure.hashCode());
return result;
}
}
public static class CardPresent extends StripeObject {
/** The ID of the Card PaymentMethod which was generated by this SetupAttempt. */
@SerializedName("generated_card")
ExpandableField generatedCard;
/** Get ID of expandable {@code generatedCard} object. */
public String getGeneratedCard() {
return (this.generatedCard != null) ? this.generatedCard.getId() : null;
}
public void setGeneratedCard(String id) {
this.generatedCard = ApiResource.setExpandableFieldId(id, this.generatedCard);
}
/** Get expanded {@code generatedCard}. */
public PaymentMethod getGeneratedCardObject() {
return (this.generatedCard != null) ? this.generatedCard.getExpanded() : null;
}
public void setGeneratedCardObject(PaymentMethod expandableObject) {
this.generatedCard = new ExpandableField(expandableObject.getId(), expandableObject);
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SetupAttempt.PaymentMethodDetails.CardPresent)) return false;
final SetupAttempt.PaymentMethodDetails.CardPresent other = (SetupAttempt.PaymentMethodDetails.CardPresent) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$generatedCard = this.getGeneratedCard();
final java.lang.Object other$generatedCard = other.getGeneratedCard();
if (this$generatedCard == null ? other$generatedCard != null : !this$generatedCard.equals(other$generatedCard)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SetupAttempt.PaymentMethodDetails.CardPresent;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $generatedCard = this.getGeneratedCard();
result = result * PRIME + ($generatedCard == null ? 43 : $generatedCard.hashCode());
return result;
}
}
public static class Ideal extends StripeObject {
/**
* The customer's bank. Can be one of {@code abn_amro}, {@code asn_bank}, {@code bunq}, {@code
* handelsbanken}, {@code ing}, {@code knab}, {@code moneyou}, {@code rabobank}, {@code
* regiobank}, {@code revolut}, {@code sns_bank}, {@code triodos_bank}, or {@code
* van_lanschot}.
*/
@SerializedName("bank")
String bank;
/**
* The Bank Identifier Code of the customer's bank.
*
* One of {@code ABNANL2A}, {@code ASNBNL21}, {@code BUNQNL2A}, {@code FVLBNL22}, {@code
* HANDNL2A}, {@code INGBNL2A}, {@code KNABNL2H}, {@code MOYONL21}, {@code RABONL2U}, {@code
* RBRBNL21}, {@code REVOLT21}, {@code SNSBNL2A}, or {@code TRIONL2U}.
*/
@SerializedName("bic")
String bic;
/** The ID of the SEPA Direct Debit PaymentMethod which was generated by this SetupAttempt. */
@SerializedName("generated_sepa_debit")
ExpandableField generatedSepaDebit;
/**
* The mandate for the SEPA Direct Debit PaymentMethod which was generated by this
* SetupAttempt.
*/
@SerializedName("generated_sepa_debit_mandate")
ExpandableField generatedSepaDebitMandate;
/** Last four characters of the IBAN. */
@SerializedName("iban_last4")
String ibanLast4;
/**
* Owner's verified full name. Values are verified or provided by iDEAL directly (if
* supported) at the time of authorization or settlement. They cannot be set or mutated.
*/
@SerializedName("verified_name")
String verifiedName;
/** Get ID of expandable {@code generatedSepaDebit} object. */
public String getGeneratedSepaDebit() {
return (this.generatedSepaDebit != null) ? this.generatedSepaDebit.getId() : null;
}
public void setGeneratedSepaDebit(String id) {
this.generatedSepaDebit = ApiResource.setExpandableFieldId(id, this.generatedSepaDebit);
}
/** Get expanded {@code generatedSepaDebit}. */
public PaymentMethod getGeneratedSepaDebitObject() {
return (this.generatedSepaDebit != null) ? this.generatedSepaDebit.getExpanded() : null;
}
public void setGeneratedSepaDebitObject(PaymentMethod expandableObject) {
this.generatedSepaDebit = new ExpandableField(expandableObject.getId(), expandableObject);
}
/** Get ID of expandable {@code generatedSepaDebitMandate} object. */
public String getGeneratedSepaDebitMandate() {
return (this.generatedSepaDebitMandate != null) ? this.generatedSepaDebitMandate.getId() : null;
}
public void setGeneratedSepaDebitMandate(String id) {
this.generatedSepaDebitMandate = ApiResource.setExpandableFieldId(id, this.generatedSepaDebitMandate);
}
/** Get expanded {@code generatedSepaDebitMandate}. */
public Mandate getGeneratedSepaDebitMandateObject() {
return (this.generatedSepaDebitMandate != null) ? this.generatedSepaDebitMandate.getExpanded() : null;
}
public void setGeneratedSepaDebitMandateObject(Mandate expandableObject) {
this.generatedSepaDebitMandate = new ExpandableField(expandableObject.getId(), expandableObject);
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBank() {
return this.bank;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBic() {
return this.bic;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getIbanLast4() {
return this.ibanLast4;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getVerifiedName() {
return this.verifiedName;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBank(final String bank) {
this.bank = bank;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBic(final String bic) {
this.bic = bic;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIbanLast4(final String ibanLast4) {
this.ibanLast4 = ibanLast4;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVerifiedName(final String verifiedName) {
this.verifiedName = verifiedName;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SetupAttempt.PaymentMethodDetails.Ideal)) return false;
final SetupAttempt.PaymentMethodDetails.Ideal other = (SetupAttempt.PaymentMethodDetails.Ideal) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$bank = this.getBank();
final java.lang.Object other$bank = other.getBank();
if (this$bank == null ? other$bank != null : !this$bank.equals(other$bank)) return false;
final java.lang.Object this$bic = this.getBic();
final java.lang.Object other$bic = other.getBic();
if (this$bic == null ? other$bic != null : !this$bic.equals(other$bic)) return false;
final java.lang.Object this$generatedSepaDebit = this.getGeneratedSepaDebit();
final java.lang.Object other$generatedSepaDebit = other.getGeneratedSepaDebit();
if (this$generatedSepaDebit == null ? other$generatedSepaDebit != null : !this$generatedSepaDebit.equals(other$generatedSepaDebit)) return false;
final java.lang.Object this$generatedSepaDebitMandate = this.getGeneratedSepaDebitMandate();
final java.lang.Object other$generatedSepaDebitMandate = other.getGeneratedSepaDebitMandate();
if (this$generatedSepaDebitMandate == null ? other$generatedSepaDebitMandate != null : !this$generatedSepaDebitMandate.equals(other$generatedSepaDebitMandate)) return false;
final java.lang.Object this$ibanLast4 = this.getIbanLast4();
final java.lang.Object other$ibanLast4 = other.getIbanLast4();
if (this$ibanLast4 == null ? other$ibanLast4 != null : !this$ibanLast4.equals(other$ibanLast4)) return false;
final java.lang.Object this$verifiedName = this.getVerifiedName();
final java.lang.Object other$verifiedName = other.getVerifiedName();
if (this$verifiedName == null ? other$verifiedName != null : !this$verifiedName.equals(other$verifiedName)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SetupAttempt.PaymentMethodDetails.Ideal;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $bank = this.getBank();
result = result * PRIME + ($bank == null ? 43 : $bank.hashCode());
final java.lang.Object $bic = this.getBic();
result = result * PRIME + ($bic == null ? 43 : $bic.hashCode());
final java.lang.Object $generatedSepaDebit = this.getGeneratedSepaDebit();
result = result * PRIME + ($generatedSepaDebit == null ? 43 : $generatedSepaDebit.hashCode());
final java.lang.Object $generatedSepaDebitMandate = this.getGeneratedSepaDebitMandate();
result = result * PRIME + ($generatedSepaDebitMandate == null ? 43 : $generatedSepaDebitMandate.hashCode());
final java.lang.Object $ibanLast4 = this.getIbanLast4();
result = result * PRIME + ($ibanLast4 == null ? 43 : $ibanLast4.hashCode());
final java.lang.Object $verifiedName = this.getVerifiedName();
result = result * PRIME + ($verifiedName == null ? 43 : $verifiedName.hashCode());
return result;
}
}
public static class SepaDebit extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SetupAttempt.PaymentMethodDetails.SepaDebit)) return false;
final SetupAttempt.PaymentMethodDetails.SepaDebit other = (SetupAttempt.PaymentMethodDetails.SepaDebit) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SetupAttempt.PaymentMethodDetails.SepaDebit;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class Sofort extends StripeObject {
/** Bank code of bank associated with the bank account. */
@SerializedName("bank_code")
String bankCode;
/** Name of the bank associated with the bank account. */
@SerializedName("bank_name")
String bankName;
/** Bank Identifier Code of the bank associated with the bank account. */
@SerializedName("bic")
String bic;
/** The ID of the SEPA Direct Debit PaymentMethod which was generated by this SetupAttempt. */
@SerializedName("generated_sepa_debit")
ExpandableField generatedSepaDebit;
/**
* The mandate for the SEPA Direct Debit PaymentMethod which was generated by this
* SetupAttempt.
*/
@SerializedName("generated_sepa_debit_mandate")
ExpandableField generatedSepaDebitMandate;
/** Last four characters of the IBAN. */
@SerializedName("iban_last4")
String ibanLast4;
/**
* Preferred language of the Sofort authorization page that the customer is redirected to. Can
* be one of {@code en}, {@code de}, {@code fr}, or {@code nl}
*/
@SerializedName("preferred_language")
String preferredLanguage;
/**
* Owner's verified full name. Values are verified or provided by Sofort directly (if
* supported) at the time of authorization or settlement. They cannot be set or mutated.
*/
@SerializedName("verified_name")
String verifiedName;
/** Get ID of expandable {@code generatedSepaDebit} object. */
public String getGeneratedSepaDebit() {
return (this.generatedSepaDebit != null) ? this.generatedSepaDebit.getId() : null;
}
public void setGeneratedSepaDebit(String id) {
this.generatedSepaDebit = ApiResource.setExpandableFieldId(id, this.generatedSepaDebit);
}
/** Get expanded {@code generatedSepaDebit}. */
public PaymentMethod getGeneratedSepaDebitObject() {
return (this.generatedSepaDebit != null) ? this.generatedSepaDebit.getExpanded() : null;
}
public void setGeneratedSepaDebitObject(PaymentMethod expandableObject) {
this.generatedSepaDebit = new ExpandableField(expandableObject.getId(), expandableObject);
}
/** Get ID of expandable {@code generatedSepaDebitMandate} object. */
public String getGeneratedSepaDebitMandate() {
return (this.generatedSepaDebitMandate != null) ? this.generatedSepaDebitMandate.getId() : null;
}
public void setGeneratedSepaDebitMandate(String id) {
this.generatedSepaDebitMandate = ApiResource.setExpandableFieldId(id, this.generatedSepaDebitMandate);
}
/** Get expanded {@code generatedSepaDebitMandate}. */
public Mandate getGeneratedSepaDebitMandateObject() {
return (this.generatedSepaDebitMandate != null) ? this.generatedSepaDebitMandate.getExpanded() : null;
}
public void setGeneratedSepaDebitMandateObject(Mandate expandableObject) {
this.generatedSepaDebitMandate = new ExpandableField(expandableObject.getId(), expandableObject);
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBankCode() {
return this.bankCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBankName() {
return this.bankName;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBic() {
return this.bic;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getIbanLast4() {
return this.ibanLast4;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPreferredLanguage() {
return this.preferredLanguage;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getVerifiedName() {
return this.verifiedName;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBankCode(final String bankCode) {
this.bankCode = bankCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBankName(final String bankName) {
this.bankName = bankName;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBic(final String bic) {
this.bic = bic;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIbanLast4(final String ibanLast4) {
this.ibanLast4 = ibanLast4;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPreferredLanguage(final String preferredLanguage) {
this.preferredLanguage = preferredLanguage;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVerifiedName(final String verifiedName) {
this.verifiedName = verifiedName;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SetupAttempt.PaymentMethodDetails.Sofort)) return false;
final SetupAttempt.PaymentMethodDetails.Sofort other = (SetupAttempt.PaymentMethodDetails.Sofort) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$bankCode = this.getBankCode();
final java.lang.Object other$bankCode = other.getBankCode();
if (this$bankCode == null ? other$bankCode != null : !this$bankCode.equals(other$bankCode)) return false;
final java.lang.Object this$bankName = this.getBankName();
final java.lang.Object other$bankName = other.getBankName();
if (this$bankName == null ? other$bankName != null : !this$bankName.equals(other$bankName)) return false;
final java.lang.Object this$bic = this.getBic();
final java.lang.Object other$bic = other.getBic();
if (this$bic == null ? other$bic != null : !this$bic.equals(other$bic)) return false;
final java.lang.Object this$generatedSepaDebit = this.getGeneratedSepaDebit();
final java.lang.Object other$generatedSepaDebit = other.getGeneratedSepaDebit();
if (this$generatedSepaDebit == null ? other$generatedSepaDebit != null : !this$generatedSepaDebit.equals(other$generatedSepaDebit)) return false;
final java.lang.Object this$generatedSepaDebitMandate = this.getGeneratedSepaDebitMandate();
final java.lang.Object other$generatedSepaDebitMandate = other.getGeneratedSepaDebitMandate();
if (this$generatedSepaDebitMandate == null ? other$generatedSepaDebitMandate != null : !this$generatedSepaDebitMandate.equals(other$generatedSepaDebitMandate)) return false;
final java.lang.Object this$ibanLast4 = this.getIbanLast4();
final java.lang.Object other$ibanLast4 = other.getIbanLast4();
if (this$ibanLast4 == null ? other$ibanLast4 != null : !this$ibanLast4.equals(other$ibanLast4)) return false;
final java.lang.Object this$preferredLanguage = this.getPreferredLanguage();
final java.lang.Object other$preferredLanguage = other.getPreferredLanguage();
if (this$preferredLanguage == null ? other$preferredLanguage != null : !this$preferredLanguage.equals(other$preferredLanguage)) return false;
final java.lang.Object this$verifiedName = this.getVerifiedName();
final java.lang.Object other$verifiedName = other.getVerifiedName();
if (this$verifiedName == null ? other$verifiedName != null : !this$verifiedName.equals(other$verifiedName)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SetupAttempt.PaymentMethodDetails.Sofort;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $bankCode = this.getBankCode();
result = result * PRIME + ($bankCode == null ? 43 : $bankCode.hashCode());
final java.lang.Object $bankName = this.getBankName();
result = result * PRIME + ($bankName == null ? 43 : $bankName.hashCode());
final java.lang.Object $bic = this.getBic();
result = result * PRIME + ($bic == null ? 43 : $bic.hashCode());
final java.lang.Object $generatedSepaDebit = this.getGeneratedSepaDebit();
result = result * PRIME + ($generatedSepaDebit == null ? 43 : $generatedSepaDebit.hashCode());
final java.lang.Object $generatedSepaDebitMandate = this.getGeneratedSepaDebitMandate();
result = result * PRIME + ($generatedSepaDebitMandate == null ? 43 : $generatedSepaDebitMandate.hashCode());
final java.lang.Object $ibanLast4 = this.getIbanLast4();
result = result * PRIME + ($ibanLast4 == null ? 43 : $ibanLast4.hashCode());
final java.lang.Object $preferredLanguage = this.getPreferredLanguage();
result = result * PRIME + ($preferredLanguage == null ? 43 : $preferredLanguage.hashCode());
final java.lang.Object $verifiedName = this.getVerifiedName();
result = result * PRIME + ($verifiedName == null ? 43 : $verifiedName.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public AcssDebit getAcssDebit() {
return this.acssDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public AuBecsDebit getAuBecsDebit() {
return this.auBecsDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BacsDebit getBacsDebit() {
return this.bacsDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Bancontact getBancontact() {
return this.bancontact;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Card getCard() {
return this.card;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public CardPresent getCardPresent() {
return this.cardPresent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Ideal getIdeal() {
return this.ideal;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SepaDebit getSepaDebit() {
return this.sepaDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Sofort getSofort() {
return this.sofort;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAcssDebit(final AcssDebit acssDebit) {
this.acssDebit = acssDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAuBecsDebit(final AuBecsDebit auBecsDebit) {
this.auBecsDebit = auBecsDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBacsDebit(final BacsDebit bacsDebit) {
this.bacsDebit = bacsDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBancontact(final Bancontact bancontact) {
this.bancontact = bancontact;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCard(final Card card) {
this.card = card;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCardPresent(final CardPresent cardPresent) {
this.cardPresent = cardPresent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIdeal(final Ideal ideal) {
this.ideal = ideal;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSepaDebit(final SepaDebit sepaDebit) {
this.sepaDebit = sepaDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSofort(final Sofort sofort) {
this.sofort = sofort;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SetupAttempt.PaymentMethodDetails)) return false;
final SetupAttempt.PaymentMethodDetails other = (SetupAttempt.PaymentMethodDetails) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$acssDebit = this.getAcssDebit();
final java.lang.Object other$acssDebit = other.getAcssDebit();
if (this$acssDebit == null ? other$acssDebit != null : !this$acssDebit.equals(other$acssDebit)) return false;
final java.lang.Object this$auBecsDebit = this.getAuBecsDebit();
final java.lang.Object other$auBecsDebit = other.getAuBecsDebit();
if (this$auBecsDebit == null ? other$auBecsDebit != null : !this$auBecsDebit.equals(other$auBecsDebit)) return false;
final java.lang.Object this$bacsDebit = this.getBacsDebit();
final java.lang.Object other$bacsDebit = other.getBacsDebit();
if (this$bacsDebit == null ? other$bacsDebit != null : !this$bacsDebit.equals(other$bacsDebit)) return false;
final java.lang.Object this$bancontact = this.getBancontact();
final java.lang.Object other$bancontact = other.getBancontact();
if (this$bancontact == null ? other$bancontact != null : !this$bancontact.equals(other$bancontact)) return false;
final java.lang.Object this$card = this.getCard();
final java.lang.Object other$card = other.getCard();
if (this$card == null ? other$card != null : !this$card.equals(other$card)) return false;
final java.lang.Object this$cardPresent = this.getCardPresent();
final java.lang.Object other$cardPresent = other.getCardPresent();
if (this$cardPresent == null ? other$cardPresent != null : !this$cardPresent.equals(other$cardPresent)) return false;
final java.lang.Object this$ideal = this.getIdeal();
final java.lang.Object other$ideal = other.getIdeal();
if (this$ideal == null ? other$ideal != null : !this$ideal.equals(other$ideal)) return false;
final java.lang.Object this$sepaDebit = this.getSepaDebit();
final java.lang.Object other$sepaDebit = other.getSepaDebit();
if (this$sepaDebit == null ? other$sepaDebit != null : !this$sepaDebit.equals(other$sepaDebit)) return false;
final java.lang.Object this$sofort = this.getSofort();
final java.lang.Object other$sofort = other.getSofort();
if (this$sofort == null ? other$sofort != null : !this$sofort.equals(other$sofort)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SetupAttempt.PaymentMethodDetails;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $acssDebit = this.getAcssDebit();
result = result * PRIME + ($acssDebit == null ? 43 : $acssDebit.hashCode());
final java.lang.Object $auBecsDebit = this.getAuBecsDebit();
result = result * PRIME + ($auBecsDebit == null ? 43 : $auBecsDebit.hashCode());
final java.lang.Object $bacsDebit = this.getBacsDebit();
result = result * PRIME + ($bacsDebit == null ? 43 : $bacsDebit.hashCode());
final java.lang.Object $bancontact = this.getBancontact();
result = result * PRIME + ($bancontact == null ? 43 : $bancontact.hashCode());
final java.lang.Object $card = this.getCard();
result = result * PRIME + ($card == null ? 43 : $card.hashCode());
final java.lang.Object $cardPresent = this.getCardPresent();
result = result * PRIME + ($cardPresent == null ? 43 : $cardPresent.hashCode());
final java.lang.Object $ideal = this.getIdeal();
result = result * PRIME + ($ideal == null ? 43 : $ideal.hashCode());
final java.lang.Object $sepaDebit = this.getSepaDebit();
result = result * PRIME + ($sepaDebit == null ? 43 : $sepaDebit.hashCode());
final java.lang.Object $sofort = this.getSofort();
result = result * PRIME + ($sofort == null ? 43 : $sofort.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
return result;
}
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code setup_attempt}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PaymentMethodDetails getPaymentMethodDetails() {
return this.paymentMethodDetails;
}
/**
* The error encountered during this attempt to confirm the SetupIntent, if any.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public StripeError getSetupError() {
return this.setupError;
}
/**
* Status of this SetupAttempt, one of {@code requires_confirmation}, {@code requires_action},
* {@code processing}, {@code succeeded}, {@code failed}, or {@code abandoned}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
/**
* The value of usage on
* the SetupIntent at the time of this confirmation, one of {@code off_session} or {@code
* on_session}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUsage() {
return this.usage;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code setup_attempt}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPaymentMethodDetails(final PaymentMethodDetails paymentMethodDetails) {
this.paymentMethodDetails = paymentMethodDetails;
}
/**
* The error encountered during this attempt to confirm the SetupIntent, if any.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSetupError(final StripeError setupError) {
this.setupError = setupError;
}
/**
* Status of this SetupAttempt, one of {@code requires_confirmation}, {@code requires_action},
* {@code processing}, {@code succeeded}, {@code failed}, or {@code abandoned}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
/**
* The value of usage on
* the SetupIntent at the time of this confirmation, one of {@code off_session} or {@code
* on_session}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsage(final String usage) {
this.usage = usage;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SetupAttempt)) return false;
final SetupAttempt other = (SetupAttempt) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$application = this.getApplication();
final java.lang.Object other$application = other.getApplication();
if (this$application == null ? other$application != null : !this$application.equals(other$application)) return false;
final java.lang.Object this$customer = this.getCustomer();
final java.lang.Object other$customer = other.getCustomer();
if (this$customer == null ? other$customer != null : !this$customer.equals(other$customer)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$onBehalfOf = this.getOnBehalfOf();
final java.lang.Object other$onBehalfOf = other.getOnBehalfOf();
if (this$onBehalfOf == null ? other$onBehalfOf != null : !this$onBehalfOf.equals(other$onBehalfOf)) return false;
final java.lang.Object this$paymentMethod = this.getPaymentMethod();
final java.lang.Object other$paymentMethod = other.getPaymentMethod();
if (this$paymentMethod == null ? other$paymentMethod != null : !this$paymentMethod.equals(other$paymentMethod)) return false;
final java.lang.Object this$paymentMethodDetails = this.getPaymentMethodDetails();
final java.lang.Object other$paymentMethodDetails = other.getPaymentMethodDetails();
if (this$paymentMethodDetails == null ? other$paymentMethodDetails != null : !this$paymentMethodDetails.equals(other$paymentMethodDetails)) return false;
final java.lang.Object this$setupError = this.getSetupError();
final java.lang.Object other$setupError = other.getSetupError();
if (this$setupError == null ? other$setupError != null : !this$setupError.equals(other$setupError)) return false;
final java.lang.Object this$setupIntent = this.getSetupIntent();
final java.lang.Object other$setupIntent = other.getSetupIntent();
if (this$setupIntent == null ? other$setupIntent != null : !this$setupIntent.equals(other$setupIntent)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$usage = this.getUsage();
final java.lang.Object other$usage = other.getUsage();
if (this$usage == null ? other$usage != null : !this$usage.equals(other$usage)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SetupAttempt;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $application = this.getApplication();
result = result * PRIME + ($application == null ? 43 : $application.hashCode());
final java.lang.Object $customer = this.getCustomer();
result = result * PRIME + ($customer == null ? 43 : $customer.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $onBehalfOf = this.getOnBehalfOf();
result = result * PRIME + ($onBehalfOf == null ? 43 : $onBehalfOf.hashCode());
final java.lang.Object $paymentMethod = this.getPaymentMethod();
result = result * PRIME + ($paymentMethod == null ? 43 : $paymentMethod.hashCode());
final java.lang.Object $paymentMethodDetails = this.getPaymentMethodDetails();
result = result * PRIME + ($paymentMethodDetails == null ? 43 : $paymentMethodDetails.hashCode());
final java.lang.Object $setupError = this.getSetupError();
result = result * PRIME + ($setupError == null ? 43 : $setupError.hashCode());
final java.lang.Object $setupIntent = this.getSetupIntent();
result = result * PRIME + ($setupIntent == null ? 43 : $setupIntent.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $usage = this.getUsage();
result = result * PRIME + ($usage == null ? 43 : $usage.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
}