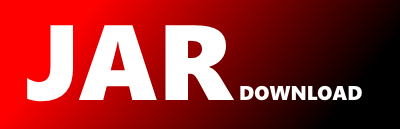
com.stripe.model.StripeError Maven / Gradle / Ivy
// Generated by delombok at Thu Oct 07 18:42:34 EDT 2021
// File generated from our OpenAPI spec
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
public class StripeError extends StripeObject {
/**
* For card errors, the ID of the failed charge.
*/
@SerializedName("charge")
String charge;
/**
* For some errors that could be handled programmatically, a short string indicating the error code reported.
*/
@SerializedName("code")
String code;
/**
* For card errors resulting from a card issuer decline, a short string indicating the card issuer's reason for the
* decline if they provide one.
*/
@SerializedName("decline_code")
String declineCode;
/**
* A URL to more information about the error
* code reported.
*/
@SerializedName("doc_url")
String docUrl;
/**
* A human-readable message providing more details about the error. For card errors, these
* messages can be shown to your users.
*/
@SerializedName("message")
String message;
/**
* If the error is parameter-specific, the parameter related to the error. For example, you can
* use this to display a message near the correct form field.
*/
@SerializedName("param")
String param;
/**
* A PaymentIntent guides you through the process of collecting a payment from your customer. We
* recommend that you create exactly one PaymentIntent for each order or customer session in your
* system. You can reference the PaymentIntent later to see the history of payment attempts for a
* particular session.
*
* A PaymentIntent transitions through multiple statuses
* throughout its lifetime as it interfaces with Stripe.js to perform authentication flows and
* ultimately creates at most one successful charge.
*
*
Related guide: Payment Intents
* API.
*/
@SerializedName("payment_intent")
PaymentIntent paymentIntent;
/**
* PaymentMethod objects represent your customer's payment instruments. They can be used with PaymentIntents to collect payments
* or saved to Customer objects to store instrument details for future payments.
*
*
Related guides: Payment
* Methods and More Payment
* Scenarios.
*/
@SerializedName("payment_method")
PaymentMethod paymentMethod;
/**
* If the error is specific to the type of payment method, the payment method type that had a
* problem. This field is only populated for invoice-related errors.
*/
@SerializedName("payment_method_type")
String paymentMethodType;
/**
* A SetupIntent guides you through the process of setting up and saving a customer's payment
* credentials for future payments. For example, you could use a SetupIntent to set up and save
* your customer's card without immediately collecting a payment. Later, you can use PaymentIntents to drive the payment
* flow.
*
*
Create a SetupIntent as soon as you're ready to collect your customer's payment credentials.
* Do not maintain long-lived, unconfirmed SetupIntents as they may no longer be valid. The
* SetupIntent then transitions through multiple statuses as it guides you
* through the setup process.
*
*
Successful SetupIntents result in payment credentials that are optimized for future
* payments. For example, cardholders in certain regions may need to
* be run through Strong Customer
* Authentication at the time of payment method collection in order to streamline later off-session payments. If the
* SetupIntent is used with a Customer, upon success, it
* will automatically attach the resulting payment method to that Customer. We recommend using
* SetupIntents or setup_future_usage
* on PaymentIntents to save payment methods in order to prevent saving invalid or unoptimized
* payment methods.
*
*
By using SetupIntents, you ensure that your customers experience the minimum set of required
* friction, even as regulations change over time.
*
*
Related guide: Setup Intents
* API.
*/
@SerializedName("setup_intent")
SetupIntent setupIntent;
@SerializedName("source")
PaymentSource source;
/**
* The type of error returned. One of {@code api_error}, {@code card_error}, {@code
* idempotency_error}, or {@code invalid_request_error}
*/
@SerializedName("type")
String type;
/**
* For card errors, the ID of the failed charge.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCharge() {
return this.charge;
}
/**
* For some errors that could be handled programmatically, a short string indicating the error code reported.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCode() {
return this.code;
}
/**
* For card errors resulting from a card issuer decline, a short string indicating the card issuer's reason for the
* decline if they provide one.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDeclineCode() {
return this.declineCode;
}
/**
* A URL to more information about the error
* code reported.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDocUrl() {
return this.docUrl;
}
/**
* A human-readable message providing more details about the error. For card errors, these
* messages can be shown to your users.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getMessage() {
return this.message;
}
/**
* If the error is parameter-specific, the parameter related to the error. For example, you can
* use this to display a message near the correct form field.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getParam() {
return this.param;
}
/**
* A PaymentIntent guides you through the process of collecting a payment from your customer. We
* recommend that you create exactly one PaymentIntent for each order or customer session in your
* system. You can reference the PaymentIntent later to see the history of payment attempts for a
* particular session.
*
*
A PaymentIntent transitions through multiple statuses
* throughout its lifetime as it interfaces with Stripe.js to perform authentication flows and
* ultimately creates at most one successful charge.
*
*
Related guide: Payment Intents
* API.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PaymentIntent getPaymentIntent() {
return this.paymentIntent;
}
/**
* PaymentMethod objects represent your customer's payment instruments. They can be used with PaymentIntents to collect payments
* or saved to Customer objects to store instrument details for future payments.
*
*
Related guides: Payment
* Methods and More Payment
* Scenarios.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PaymentMethod getPaymentMethod() {
return this.paymentMethod;
}
/**
* If the error is specific to the type of payment method, the payment method type that had a
* problem. This field is only populated for invoice-related errors.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPaymentMethodType() {
return this.paymentMethodType;
}
/**
* A SetupIntent guides you through the process of setting up and saving a customer's payment
* credentials for future payments. For example, you could use a SetupIntent to set up and save
* your customer's card without immediately collecting a payment. Later, you can use PaymentIntents to drive the payment
* flow.
*
*
Create a SetupIntent as soon as you're ready to collect your customer's payment credentials.
* Do not maintain long-lived, unconfirmed SetupIntents as they may no longer be valid. The
* SetupIntent then transitions through multiple statuses as it guides you
* through the setup process.
*
*
Successful SetupIntents result in payment credentials that are optimized for future
* payments. For example, cardholders in certain regions may need to
* be run through Strong Customer
* Authentication at the time of payment method collection in order to streamline later off-session payments. If the
* SetupIntent is used with a Customer, upon success, it
* will automatically attach the resulting payment method to that Customer. We recommend using
* SetupIntents or setup_future_usage
* on PaymentIntents to save payment methods in order to prevent saving invalid or unoptimized
* payment methods.
*
*
By using SetupIntents, you ensure that your customers experience the minimum set of required
* friction, even as regulations change over time.
*
*
Related guide: Setup Intents
* API.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SetupIntent getSetupIntent() {
return this.setupIntent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PaymentSource getSource() {
return this.source;
}
/**
* The type of error returned. One of {@code api_error}, {@code card_error}, {@code
* idempotency_error}, or {@code invalid_request_error}
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
/**
* For card errors, the ID of the failed charge.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCharge(final String charge) {
this.charge = charge;
}
/**
* For some errors that could be handled programmatically, a short string indicating the error code reported.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCode(final String code) {
this.code = code;
}
/**
* For card errors resulting from a card issuer decline, a short string indicating the card issuer's reason for the
* decline if they provide one.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDeclineCode(final String declineCode) {
this.declineCode = declineCode;
}
/**
* A URL to more information about the error
* code reported.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDocUrl(final String docUrl) {
this.docUrl = docUrl;
}
/**
* A human-readable message providing more details about the error. For card errors, these
* messages can be shown to your users.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMessage(final String message) {
this.message = message;
}
/**
* If the error is parameter-specific, the parameter related to the error. For example, you can
* use this to display a message near the correct form field.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setParam(final String param) {
this.param = param;
}
/**
* A PaymentIntent guides you through the process of collecting a payment from your customer. We
* recommend that you create exactly one PaymentIntent for each order or customer session in your
* system. You can reference the PaymentIntent later to see the history of payment attempts for a
* particular session.
*
*
A PaymentIntent transitions through multiple statuses
* throughout its lifetime as it interfaces with Stripe.js to perform authentication flows and
* ultimately creates at most one successful charge.
*
*
Related guide: Payment Intents
* API.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPaymentIntent(final PaymentIntent paymentIntent) {
this.paymentIntent = paymentIntent;
}
/**
* PaymentMethod objects represent your customer's payment instruments. They can be used with PaymentIntents to collect payments
* or saved to Customer objects to store instrument details for future payments.
*
*
Related guides: Payment
* Methods and More Payment
* Scenarios.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPaymentMethod(final PaymentMethod paymentMethod) {
this.paymentMethod = paymentMethod;
}
/**
* If the error is specific to the type of payment method, the payment method type that had a
* problem. This field is only populated for invoice-related errors.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPaymentMethodType(final String paymentMethodType) {
this.paymentMethodType = paymentMethodType;
}
/**
* A SetupIntent guides you through the process of setting up and saving a customer's payment
* credentials for future payments. For example, you could use a SetupIntent to set up and save
* your customer's card without immediately collecting a payment. Later, you can use PaymentIntents to drive the payment
* flow.
*
*
Create a SetupIntent as soon as you're ready to collect your customer's payment credentials.
* Do not maintain long-lived, unconfirmed SetupIntents as they may no longer be valid. The
* SetupIntent then transitions through multiple statuses as it guides you
* through the setup process.
*
*
Successful SetupIntents result in payment credentials that are optimized for future
* payments. For example, cardholders in certain regions may need to
* be run through Strong Customer
* Authentication at the time of payment method collection in order to streamline later off-session payments. If the
* SetupIntent is used with a Customer, upon success, it
* will automatically attach the resulting payment method to that Customer. We recommend using
* SetupIntents or setup_future_usage
* on PaymentIntents to save payment methods in order to prevent saving invalid or unoptimized
* payment methods.
*
*
By using SetupIntents, you ensure that your customers experience the minimum set of required
* friction, even as regulations change over time.
*
*
Related guide: Setup Intents
* API.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSetupIntent(final SetupIntent setupIntent) {
this.setupIntent = setupIntent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSource(final PaymentSource source) {
this.source = source;
}
/**
* The type of error returned. One of {@code api_error}, {@code card_error}, {@code
* idempotency_error}, or {@code invalid_request_error}
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof StripeError)) return false;
final StripeError other = (StripeError) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$charge = this.getCharge();
final java.lang.Object other$charge = other.getCharge();
if (this$charge == null ? other$charge != null : !this$charge.equals(other$charge)) return false;
final java.lang.Object this$code = this.getCode();
final java.lang.Object other$code = other.getCode();
if (this$code == null ? other$code != null : !this$code.equals(other$code)) return false;
final java.lang.Object this$declineCode = this.getDeclineCode();
final java.lang.Object other$declineCode = other.getDeclineCode();
if (this$declineCode == null ? other$declineCode != null : !this$declineCode.equals(other$declineCode)) return false;
final java.lang.Object this$docUrl = this.getDocUrl();
final java.lang.Object other$docUrl = other.getDocUrl();
if (this$docUrl == null ? other$docUrl != null : !this$docUrl.equals(other$docUrl)) return false;
final java.lang.Object this$message = this.getMessage();
final java.lang.Object other$message = other.getMessage();
if (this$message == null ? other$message != null : !this$message.equals(other$message)) return false;
final java.lang.Object this$param = this.getParam();
final java.lang.Object other$param = other.getParam();
if (this$param == null ? other$param != null : !this$param.equals(other$param)) return false;
final java.lang.Object this$paymentIntent = this.getPaymentIntent();
final java.lang.Object other$paymentIntent = other.getPaymentIntent();
if (this$paymentIntent == null ? other$paymentIntent != null : !this$paymentIntent.equals(other$paymentIntent)) return false;
final java.lang.Object this$paymentMethod = this.getPaymentMethod();
final java.lang.Object other$paymentMethod = other.getPaymentMethod();
if (this$paymentMethod == null ? other$paymentMethod != null : !this$paymentMethod.equals(other$paymentMethod)) return false;
final java.lang.Object this$paymentMethodType = this.getPaymentMethodType();
final java.lang.Object other$paymentMethodType = other.getPaymentMethodType();
if (this$paymentMethodType == null ? other$paymentMethodType != null : !this$paymentMethodType.equals(other$paymentMethodType)) return false;
final java.lang.Object this$setupIntent = this.getSetupIntent();
final java.lang.Object other$setupIntent = other.getSetupIntent();
if (this$setupIntent == null ? other$setupIntent != null : !this$setupIntent.equals(other$setupIntent)) return false;
final java.lang.Object this$source = this.getSource();
final java.lang.Object other$source = other.getSource();
if (this$source == null ? other$source != null : !this$source.equals(other$source)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof StripeError;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $charge = this.getCharge();
result = result * PRIME + ($charge == null ? 43 : $charge.hashCode());
final java.lang.Object $code = this.getCode();
result = result * PRIME + ($code == null ? 43 : $code.hashCode());
final java.lang.Object $declineCode = this.getDeclineCode();
result = result * PRIME + ($declineCode == null ? 43 : $declineCode.hashCode());
final java.lang.Object $docUrl = this.getDocUrl();
result = result * PRIME + ($docUrl == null ? 43 : $docUrl.hashCode());
final java.lang.Object $message = this.getMessage();
result = result * PRIME + ($message == null ? 43 : $message.hashCode());
final java.lang.Object $param = this.getParam();
result = result * PRIME + ($param == null ? 43 : $param.hashCode());
final java.lang.Object $paymentIntent = this.getPaymentIntent();
result = result * PRIME + ($paymentIntent == null ? 43 : $paymentIntent.hashCode());
final java.lang.Object $paymentMethod = this.getPaymentMethod();
result = result * PRIME + ($paymentMethod == null ? 43 : $paymentMethod.hashCode());
final java.lang.Object $paymentMethodType = this.getPaymentMethodType();
result = result * PRIME + ($paymentMethodType == null ? 43 : $paymentMethodType.hashCode());
final java.lang.Object $setupIntent = this.getSetupIntent();
result = result * PRIME + ($setupIntent == null ? 43 : $setupIntent.hashCode());
final java.lang.Object $source = this.getSource();
result = result * PRIME + ($source == null ? 43 : $source.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
return result;
}
}