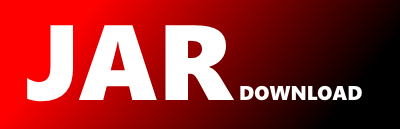
com.stripe.model.StripeSearchResult Maven / Gradle / Ivy
// Generated by delombok at Thu Oct 07 18:42:35 EDT 2021
package com.stripe.model;
import com.stripe.net.RequestOptions;
import java.util.List;
import java.util.Map;
/**
* Provides a representation of a single page worth of data from a Stripe API search method. Please
* note, StripeSearchResult is beta functionality and is subject to change or removal at any time.
*/
public abstract class StripeSearchResult extends StripeObject implements StripeSearchResultInterface {
String object;
List data;
Boolean hasMore;
String url;
String nextPage;
private transient RequestOptions requestOptions;
private Map requestParams;
public Iterable autoPagingIterable() {
return new SearchPagingIterable<>(this);
}
public Iterable autoPagingIterable(Map params) {
this.setRequestParams(params);
return new SearchPagingIterable<>(this);
}
/**
* Constructs an iterable that can be used to iterate across all objects across all pages. As page
* boundaries are encountered, the next page will be fetched automatically for continued
* iteration.
*
* @param params request parameters (will override the parameters from the initial list request)
* @param options request options (will override the options from the initial list request)
*/
public Iterable autoPagingIterable(Map params, RequestOptions options) {
this.setRequestOptions(options);
this.setRequestParams(params);
return new SearchPagingIterable<>(this);
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setData(final List data) {
this.data = data;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setHasMore(final Boolean hasMore) {
this.hasMore = hasMore;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUrl(final String url) {
this.url = url;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setNextPage(final String nextPage) {
this.nextPage = nextPage;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof StripeSearchResult)) return false;
final StripeSearchResult> other = (StripeSearchResult>) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$hasMore = this.getHasMore();
final java.lang.Object other$hasMore = other.getHasMore();
if (this$hasMore == null ? other$hasMore != null : !this$hasMore.equals(other$hasMore)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$data = this.getData();
final java.lang.Object other$data = other.getData();
if (this$data == null ? other$data != null : !this$data.equals(other$data)) return false;
final java.lang.Object this$url = this.getUrl();
final java.lang.Object other$url = other.getUrl();
if (this$url == null ? other$url != null : !this$url.equals(other$url)) return false;
final java.lang.Object this$nextPage = this.getNextPage();
final java.lang.Object other$nextPage = other.getNextPage();
if (this$nextPage == null ? other$nextPage != null : !this$nextPage.equals(other$nextPage)) return false;
final java.lang.Object this$requestParams = this.getRequestParams();
final java.lang.Object other$requestParams = other.getRequestParams();
if (this$requestParams == null ? other$requestParams != null : !this$requestParams.equals(other$requestParams)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof StripeSearchResult;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $hasMore = this.getHasMore();
result = result * PRIME + ($hasMore == null ? 43 : $hasMore.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $data = this.getData();
result = result * PRIME + ($data == null ? 43 : $data.hashCode());
final java.lang.Object $url = this.getUrl();
result = result * PRIME + ($url == null ? 43 : $url.hashCode());
final java.lang.Object $nextPage = this.getNextPage();
result = result * PRIME + ($nextPage == null ? 43 : $nextPage.hashCode());
final java.lang.Object $requestParams = this.getRequestParams();
result = result * PRIME + ($requestParams == null ? 43 : $requestParams.hashCode());
return result;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getData() {
return this.data;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getHasMore() {
return this.hasMore;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUrl() {
return this.url;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getNextPage() {
return this.nextPage;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public RequestOptions getRequestOptions() {
return this.requestOptions;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRequestOptions(final RequestOptions requestOptions) {
this.requestOptions = requestOptions;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getRequestParams() {
return this.requestParams;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRequestParams(final Map requestParams) {
this.requestParams = requestParams;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy