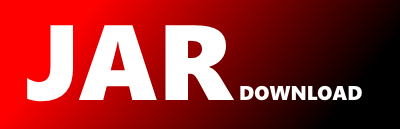
com.stripe.model.billingportal.Session Maven / Gradle / Ivy
// Generated by delombok at Thu Oct 07 18:42:35 EDT 2021
// File generated from our OpenAPI spec
package com.stripe.model.billingportal;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.model.ExpandableField;
import com.stripe.model.HasId;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.billingportal.SessionCreateParams;
import java.util.Map;
public class Session extends ApiResource implements HasId {
/**
* The configuration used by this session, describing the features available.
*/
@SerializedName("configuration")
ExpandableField configuration;
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* The ID of the customer for this session.
*/
@SerializedName("customer")
String customer;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* The IETF language tag of the locale Customer Portal is displayed in. If blank or auto, the
* customer’s {@code preferred_locales} or browser’s locale is used.
*
* One of {@code auto}, {@code bg}, {@code cs}, {@code da}, {@code de}, {@code el}, {@code en},
* {@code en-AU}, {@code en-CA}, {@code en-GB}, {@code en-IE}, {@code en-IN}, {@code en-NZ},
* {@code en-SG}, {@code es}, {@code es-419}, {@code et}, {@code fi}, {@code fil}, {@code fr},
* {@code fr-CA}, {@code hr}, {@code hu}, {@code id}, {@code it}, {@code ja}, {@code ko}, {@code
* lt}, {@code lv}, {@code ms}, {@code mt}, {@code nb}, {@code nl}, {@code pl}, {@code pt}, {@code
* pt-BR}, {@code ro}, {@code ru}, {@code sk}, {@code sl}, {@code sv}, {@code th}, {@code tr},
* {@code vi}, {@code zh}, {@code zh-HK}, or {@code zh-TW}.
*/
@SerializedName("locale")
String locale;
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code billing_portal.session}.
*/
@SerializedName("object")
String object;
/**
* The account for which the session was created on behalf of. When specified, only subscriptions
* and invoices with this {@code on_behalf_of} account appear in the portal. For more information,
* see the docs. Use
* the Accounts
* API to modify the {@code on_behalf_of} account's branding settings, which the portal
* displays.
*/
@SerializedName("on_behalf_of")
String onBehalfOf;
/**
* The URL to redirect customers to when they click on the portal's link to return to your
* website.
*/
@SerializedName("return_url")
String returnUrl;
/**
* The short-lived URL of the session that gives customers access to the customer portal.
*/
@SerializedName("url")
String url;
/**
* Get ID of expandable {@code configuration} object.
*/
public String getConfiguration() {
return (this.configuration != null) ? this.configuration.getId() : null;
}
public void setConfiguration(String id) {
this.configuration = ApiResource.setExpandableFieldId(id, this.configuration);
}
/**
* Get expanded {@code configuration}.
*/
public Configuration getConfigurationObject() {
return (this.configuration != null) ? this.configuration.getExpanded() : null;
}
public void setConfigurationObject(Configuration expandableObject) {
this.configuration = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Creates a session of the customer portal.
*/
public static Session create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Creates a session of the customer portal.
*/
public static Session create(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/billing_portal/sessions");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Session.class, options);
}
/**
* Creates a session of the customer portal.
*/
public static Session create(SessionCreateParams params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Creates a session of the customer portal.
*/
public static Session create(SessionCreateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/billing_portal/sessions");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Session.class, options);
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
/**
* The ID of the customer for this session.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCustomer() {
return this.customer;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* The IETF language tag of the locale Customer Portal is displayed in. If blank or auto, the
* customer’s {@code preferred_locales} or browser’s locale is used.
*
* One of {@code auto}, {@code bg}, {@code cs}, {@code da}, {@code de}, {@code el}, {@code en},
* {@code en-AU}, {@code en-CA}, {@code en-GB}, {@code en-IE}, {@code en-IN}, {@code en-NZ},
* {@code en-SG}, {@code es}, {@code es-419}, {@code et}, {@code fi}, {@code fil}, {@code fr},
* {@code fr-CA}, {@code hr}, {@code hu}, {@code id}, {@code it}, {@code ja}, {@code ko}, {@code
* lt}, {@code lv}, {@code ms}, {@code mt}, {@code nb}, {@code nl}, {@code pl}, {@code pt}, {@code
* pt-BR}, {@code ro}, {@code ru}, {@code sk}, {@code sl}, {@code sv}, {@code th}, {@code tr},
* {@code vi}, {@code zh}, {@code zh-HK}, or {@code zh-TW}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLocale() {
return this.locale;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code billing_portal.session}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* The account for which the session was created on behalf of. When specified, only subscriptions
* and invoices with this {@code on_behalf_of} account appear in the portal. For more information,
* see the docs. Use
* the Accounts
* API to modify the {@code on_behalf_of} account's branding settings, which the portal
* displays.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getOnBehalfOf() {
return this.onBehalfOf;
}
/**
* The URL to redirect customers to when they click on the portal's link to return to your
* website.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReturnUrl() {
return this.returnUrl;
}
/**
* The short-lived URL of the session that gives customers access to the customer portal.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUrl() {
return this.url;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* The ID of the customer for this session.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCustomer(final String customer) {
this.customer = customer;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* The IETF language tag of the locale Customer Portal is displayed in. If blank or auto, the
* customer’s {@code preferred_locales} or browser’s locale is used.
*
*
One of {@code auto}, {@code bg}, {@code cs}, {@code da}, {@code de}, {@code el}, {@code en},
* {@code en-AU}, {@code en-CA}, {@code en-GB}, {@code en-IE}, {@code en-IN}, {@code en-NZ},
* {@code en-SG}, {@code es}, {@code es-419}, {@code et}, {@code fi}, {@code fil}, {@code fr},
* {@code fr-CA}, {@code hr}, {@code hu}, {@code id}, {@code it}, {@code ja}, {@code ko}, {@code
* lt}, {@code lv}, {@code ms}, {@code mt}, {@code nb}, {@code nl}, {@code pl}, {@code pt}, {@code
* pt-BR}, {@code ro}, {@code ru}, {@code sk}, {@code sl}, {@code sv}, {@code th}, {@code tr},
* {@code vi}, {@code zh}, {@code zh-HK}, or {@code zh-TW}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLocale(final String locale) {
this.locale = locale;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code billing_portal.session}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* The account for which the session was created on behalf of. When specified, only subscriptions
* and invoices with this {@code on_behalf_of} account appear in the portal. For more information,
* see the docs. Use
* the Accounts
* API to modify the {@code on_behalf_of} account's branding settings, which the portal
* displays.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setOnBehalfOf(final String onBehalfOf) {
this.onBehalfOf = onBehalfOf;
}
/**
* The URL to redirect customers to when they click on the portal's link to return to your
* website.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReturnUrl(final String returnUrl) {
this.returnUrl = returnUrl;
}
/**
* The short-lived URL of the session that gives customers access to the customer portal.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUrl(final String url) {
this.url = url;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Session)) return false;
final Session other = (Session) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$configuration = this.getConfiguration();
final java.lang.Object other$configuration = other.getConfiguration();
if (this$configuration == null ? other$configuration != null : !this$configuration.equals(other$configuration)) return false;
final java.lang.Object this$customer = this.getCustomer();
final java.lang.Object other$customer = other.getCustomer();
if (this$customer == null ? other$customer != null : !this$customer.equals(other$customer)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$locale = this.getLocale();
final java.lang.Object other$locale = other.getLocale();
if (this$locale == null ? other$locale != null : !this$locale.equals(other$locale)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$onBehalfOf = this.getOnBehalfOf();
final java.lang.Object other$onBehalfOf = other.getOnBehalfOf();
if (this$onBehalfOf == null ? other$onBehalfOf != null : !this$onBehalfOf.equals(other$onBehalfOf)) return false;
final java.lang.Object this$returnUrl = this.getReturnUrl();
final java.lang.Object other$returnUrl = other.getReturnUrl();
if (this$returnUrl == null ? other$returnUrl != null : !this$returnUrl.equals(other$returnUrl)) return false;
final java.lang.Object this$url = this.getUrl();
final java.lang.Object other$url = other.getUrl();
if (this$url == null ? other$url != null : !this$url.equals(other$url)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Session;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $configuration = this.getConfiguration();
result = result * PRIME + ($configuration == null ? 43 : $configuration.hashCode());
final java.lang.Object $customer = this.getCustomer();
result = result * PRIME + ($customer == null ? 43 : $customer.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $locale = this.getLocale();
result = result * PRIME + ($locale == null ? 43 : $locale.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $onBehalfOf = this.getOnBehalfOf();
result = result * PRIME + ($onBehalfOf == null ? 43 : $onBehalfOf.hashCode());
final java.lang.Object $returnUrl = this.getReturnUrl();
result = result * PRIME + ($returnUrl == null ? 43 : $returnUrl.hashCode());
final java.lang.Object $url = this.getUrl();
result = result * PRIME + ($url == null ? 43 : $url.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
}