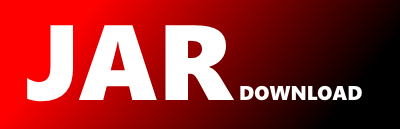
com.stripe.model.reporting.ReportRun Maven / Gradle / Ivy
// Generated by delombok at Thu Oct 07 18:42:34 EDT 2021
// File generated from our OpenAPI spec
package com.stripe.model.reporting;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.model.File;
import com.stripe.model.HasId;
import com.stripe.model.StripeObject;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.reporting.ReportRunCreateParams;
import com.stripe.param.reporting.ReportRunListParams;
import com.stripe.param.reporting.ReportRunRetrieveParams;
import java.util.List;
import java.util.Map;
public class ReportRun extends ApiResource implements HasId {
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* If something should go wrong during the run, a message about the failure (populated when {@code
* status=failed}).
*/
@SerializedName("error")
String error;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* {@code true} if the report is run on live mode data and {@code false} if it is run on test mode
* data.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code reporting.report_run}.
*/
@SerializedName("object")
String object;
@SerializedName("parameters")
Parameters parameters;
/**
* The ID of the report type to run,
* such as {@code "balance.summary.1"}.
*/
@SerializedName("report_type")
String reportType;
/**
* The file object representing the result of the report run (populated when {@code
* status=succeeded}).
*/
@SerializedName("result")
File result;
/**
* Status of this report run. This will be {@code pending} when the run is initially created. When
* the run finishes, this will be set to {@code succeeded} and the {@code result} field will be
* populated. Rarely, we may encounter an error, at which point this will be set to {@code failed}
* and the {@code error} field will be populated.
*/
@SerializedName("status")
String status;
/**
* Timestamp at which this run successfully finished (populated when {@code status=succeeded}).
* Measured in seconds since the Unix epoch.
*/
@SerializedName("succeeded_at")
Long succeededAt;
/**
* Retrieves the details of an existing Report Run.
*/
public static ReportRun retrieve(String reportRun) throws StripeException {
return retrieve(reportRun, (Map) null, (RequestOptions) null);
}
/**
* Retrieves the details of an existing Report Run.
*/
public static ReportRun retrieve(String reportRun, RequestOptions options) throws StripeException {
return retrieve(reportRun, (Map) null, options);
}
/**
* Retrieves the details of an existing Report Run.
*/
public static ReportRun retrieve(String reportRun, Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/reporting/report_runs/%s", ApiResource.urlEncodeId(reportRun)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, ReportRun.class, options);
}
/**
* Retrieves the details of an existing Report Run.
*/
public static ReportRun retrieve(String reportRun, ReportRunRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/reporting/report_runs/%s", ApiResource.urlEncodeId(reportRun)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, ReportRun.class, options);
}
/**
* Creates a new object and begin running the report. (Certain report types require a live-mode API key.)
*/
public static ReportRun create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Creates a new object and begin running the report. (Certain report types require a live-mode API key.)
*/
public static ReportRun create(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/reporting/report_runs");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, ReportRun.class, options);
}
/**
* Creates a new object and begin running the report. (Certain report types require a live-mode API key.)
*/
public static ReportRun create(ReportRunCreateParams params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Creates a new object and begin running the report. (Certain report types require a live-mode API key.)
*/
public static ReportRun create(ReportRunCreateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/reporting/report_runs");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, ReportRun.class, options);
}
/**
* Returns a list of Report Runs, with the most recent appearing first.
*/
public static ReportRunCollection list(Map params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of Report Runs, with the most recent appearing first.
*/
public static ReportRunCollection list(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/reporting/report_runs");
return ApiResource.requestCollection(url, params, ReportRunCollection.class, options);
}
/**
* Returns a list of Report Runs, with the most recent appearing first.
*/
public static ReportRunCollection list(ReportRunListParams params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of Report Runs, with the most recent appearing first.
*/
public static ReportRunCollection list(ReportRunListParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/reporting/report_runs");
return ApiResource.requestCollection(url, params, ReportRunCollection.class, options);
}
public static class Parameters extends StripeObject {
/** The set of output columns requested for inclusion in the report run. */
@SerializedName("columns")
List columns;
/** Connected account ID by which to filter the report run. */
@SerializedName("connected_account")
String connectedAccount;
/** Currency of objects to be included in the report run. */
@SerializedName("currency")
String currency;
/** Ending timestamp of data to be included in the report run (exclusive). */
@SerializedName("interval_end")
Long intervalEnd;
/** Starting timestamp of data to be included in the report run. */
@SerializedName("interval_start")
Long intervalStart;
/** Payout ID by which to filter the report run. */
@SerializedName("payout")
String payout;
/** Category of balance transactions to be included in the report run. */
@SerializedName("reporting_category")
String reportingCategory;
/**
* Defaults to {@code Etc/UTC}. The output timezone for all timestamps in the report. A list of
* possible time zone values is maintained at the IANA
* Time Zone Database. Has no effect on {@code interval_start} or {@code interval_end}.
*/
@SerializedName("timezone")
String timezone;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getColumns() {
return this.columns;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getConnectedAccount() {
return this.connectedAccount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getIntervalEnd() {
return this.intervalEnd;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getIntervalStart() {
return this.intervalStart;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPayout() {
return this.payout;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReportingCategory() {
return this.reportingCategory;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTimezone() {
return this.timezone;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setColumns(final List columns) {
this.columns = columns;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setConnectedAccount(final String connectedAccount) {
this.connectedAccount = connectedAccount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIntervalEnd(final Long intervalEnd) {
this.intervalEnd = intervalEnd;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIntervalStart(final Long intervalStart) {
this.intervalStart = intervalStart;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPayout(final String payout) {
this.payout = payout;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReportingCategory(final String reportingCategory) {
this.reportingCategory = reportingCategory;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTimezone(final String timezone) {
this.timezone = timezone;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof ReportRun.Parameters)) return false;
final ReportRun.Parameters other = (ReportRun.Parameters) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$intervalEnd = this.getIntervalEnd();
final java.lang.Object other$intervalEnd = other.getIntervalEnd();
if (this$intervalEnd == null ? other$intervalEnd != null : !this$intervalEnd.equals(other$intervalEnd)) return false;
final java.lang.Object this$intervalStart = this.getIntervalStart();
final java.lang.Object other$intervalStart = other.getIntervalStart();
if (this$intervalStart == null ? other$intervalStart != null : !this$intervalStart.equals(other$intervalStart)) return false;
final java.lang.Object this$columns = this.getColumns();
final java.lang.Object other$columns = other.getColumns();
if (this$columns == null ? other$columns != null : !this$columns.equals(other$columns)) return false;
final java.lang.Object this$connectedAccount = this.getConnectedAccount();
final java.lang.Object other$connectedAccount = other.getConnectedAccount();
if (this$connectedAccount == null ? other$connectedAccount != null : !this$connectedAccount.equals(other$connectedAccount)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
final java.lang.Object this$payout = this.getPayout();
final java.lang.Object other$payout = other.getPayout();
if (this$payout == null ? other$payout != null : !this$payout.equals(other$payout)) return false;
final java.lang.Object this$reportingCategory = this.getReportingCategory();
final java.lang.Object other$reportingCategory = other.getReportingCategory();
if (this$reportingCategory == null ? other$reportingCategory != null : !this$reportingCategory.equals(other$reportingCategory)) return false;
final java.lang.Object this$timezone = this.getTimezone();
final java.lang.Object other$timezone = other.getTimezone();
if (this$timezone == null ? other$timezone != null : !this$timezone.equals(other$timezone)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof ReportRun.Parameters;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $intervalEnd = this.getIntervalEnd();
result = result * PRIME + ($intervalEnd == null ? 43 : $intervalEnd.hashCode());
final java.lang.Object $intervalStart = this.getIntervalStart();
result = result * PRIME + ($intervalStart == null ? 43 : $intervalStart.hashCode());
final java.lang.Object $columns = this.getColumns();
result = result * PRIME + ($columns == null ? 43 : $columns.hashCode());
final java.lang.Object $connectedAccount = this.getConnectedAccount();
result = result * PRIME + ($connectedAccount == null ? 43 : $connectedAccount.hashCode());
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
final java.lang.Object $payout = this.getPayout();
result = result * PRIME + ($payout == null ? 43 : $payout.hashCode());
final java.lang.Object $reportingCategory = this.getReportingCategory();
result = result * PRIME + ($reportingCategory == null ? 43 : $reportingCategory.hashCode());
final java.lang.Object $timezone = this.getTimezone();
result = result * PRIME + ($timezone == null ? 43 : $timezone.hashCode());
return result;
}
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
/**
* If something should go wrong during the run, a message about the failure (populated when {@code
* status=failed}).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getError() {
return this.error;
}
/**
* {@code true} if the report is run on live mode data and {@code false} if it is run on test mode
* data.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code reporting.report_run}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Parameters getParameters() {
return this.parameters;
}
/**
* The ID of the report type to run,
* such as {@code "balance.summary.1"}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReportType() {
return this.reportType;
}
/**
* The file object representing the result of the report run (populated when {@code
* status=succeeded}).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public File getResult() {
return this.result;
}
/**
* Status of this report run. This will be {@code pending} when the run is initially created. When
* the run finishes, this will be set to {@code succeeded} and the {@code result} field will be
* populated. Rarely, we may encounter an error, at which point this will be set to {@code failed}
* and the {@code error} field will be populated.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
/**
* Timestamp at which this run successfully finished (populated when {@code status=succeeded}).
* Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getSucceededAt() {
return this.succeededAt;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* If something should go wrong during the run, a message about the failure (populated when {@code
* status=failed}).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setError(final String error) {
this.error = error;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* {@code true} if the report is run on live mode data and {@code false} if it is run on test mode
* data.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code reporting.report_run}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setParameters(final Parameters parameters) {
this.parameters = parameters;
}
/**
* The ID of the report type to run,
* such as {@code "balance.summary.1"}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReportType(final String reportType) {
this.reportType = reportType;
}
/**
* The file object representing the result of the report run (populated when {@code
* status=succeeded}).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setResult(final File result) {
this.result = result;
}
/**
* Status of this report run. This will be {@code pending} when the run is initially created. When
* the run finishes, this will be set to {@code succeeded} and the {@code result} field will be
* populated. Rarely, we may encounter an error, at which point this will be set to {@code failed}
* and the {@code error} field will be populated.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
/**
* Timestamp at which this run successfully finished (populated when {@code status=succeeded}).
* Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSucceededAt(final Long succeededAt) {
this.succeededAt = succeededAt;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof ReportRun)) return false;
final ReportRun other = (ReportRun) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$succeededAt = this.getSucceededAt();
final java.lang.Object other$succeededAt = other.getSucceededAt();
if (this$succeededAt == null ? other$succeededAt != null : !this$succeededAt.equals(other$succeededAt)) return false;
final java.lang.Object this$error = this.getError();
final java.lang.Object other$error = other.getError();
if (this$error == null ? other$error != null : !this$error.equals(other$error)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$parameters = this.getParameters();
final java.lang.Object other$parameters = other.getParameters();
if (this$parameters == null ? other$parameters != null : !this$parameters.equals(other$parameters)) return false;
final java.lang.Object this$reportType = this.getReportType();
final java.lang.Object other$reportType = other.getReportType();
if (this$reportType == null ? other$reportType != null : !this$reportType.equals(other$reportType)) return false;
final java.lang.Object this$result = this.getResult();
final java.lang.Object other$result = other.getResult();
if (this$result == null ? other$result != null : !this$result.equals(other$result)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof ReportRun;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $succeededAt = this.getSucceededAt();
result = result * PRIME + ($succeededAt == null ? 43 : $succeededAt.hashCode());
final java.lang.Object $error = this.getError();
result = result * PRIME + ($error == null ? 43 : $error.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $parameters = this.getParameters();
result = result * PRIME + ($parameters == null ? 43 : $parameters.hashCode());
final java.lang.Object $reportType = this.getReportType();
result = result * PRIME + ($reportType == null ? 43 : $reportType.hashCode());
final java.lang.Object $result = this.getResult();
result = result * PRIME + ($result == null ? 43 : $result.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
}