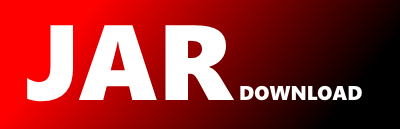
com.stripe.param.SubscriptionUpdateParams Maven / Gradle / Ivy
// Generated by delombok at Thu Oct 07 18:42:34 EDT 2021
// File generated from our OpenAPI spec
package com.stripe.param;
import com.google.gson.annotations.SerializedName;
import com.stripe.net.ApiRequestParams;
import com.stripe.param.common.EmptyParam;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class SubscriptionUpdateParams extends ApiRequestParams {
/**
* A list of prices and quantities that will generate invoice items appended to the first invoice
* for this subscription. You may pass up to 20 items.
*/
@SerializedName("add_invoice_items")
List addInvoiceItems;
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents the
* percentage of the subscription invoice subtotal that will be transferred to the application
* owner's Stripe account. The request must be made by a platform account on a connected account
* in order to set an application fee percentage. For more information, see the application fees
* documentation.
*/
@SerializedName("application_fee_percent")
BigDecimal applicationFeePercent;
/**
* Automatic tax settings for this subscription.
*/
@SerializedName("automatic_tax")
AutomaticTax automaticTax;
/**
* Either {@code now} or {@code unchanged}. Setting the value to {@code now} resets the
* subscription's billing cycle anchor to the current time. For more information, see the billing
* cycle documentation.
*/
@SerializedName("billing_cycle_anchor")
BillingCycleAnchor billingCycleAnchor;
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. Pass an empty string to remove previously-defined thresholds.
*/
@SerializedName("billing_thresholds")
Object billingThresholds;
/**
* A timestamp at which the subscription should cancel. If set to a date before the current period
* ends, this will cause a proration if prorations have been enabled using {@code
* proration_behavior}. If set during a future period, this will always cause a proration for that
* period.
*/
@SerializedName("cancel_at")
Object cancelAt;
/**
* Boolean indicating whether this subscription should cancel at the end of the current period.
*/
@SerializedName("cancel_at_period_end")
Boolean cancelAtPeriodEnd;
/**
* Either {@code charge_automatically}, or {@code send_invoice}. When charging automatically,
* Stripe will attempt to pay this subscription at the end of the cycle using the default source
* attached to the customer. When sending an invoice, Stripe will email your customer an invoice
* with payment instructions. Defaults to {@code charge_automatically}.
*/
@SerializedName("collection_method")
CollectionMethod collectionMethod;
/**
* The ID of the coupon to apply to this subscription. A coupon applied to a subscription will
* only affect invoices created for that particular subscription.
*/
@SerializedName("coupon")
Object coupon;
/**
* Number of days a customer has to pay invoices generated by this subscription. Valid only for
* subscriptions where {@code collection_method} is set to {@code send_invoice}.
*/
@SerializedName("days_until_due")
Long daysUntilDue;
/**
* ID of the default payment method for the subscription. It must belong to the customer
* associated with the subscription. This takes precedence over {@code default_source}. If neither
* are set, invoices will use the customer's invoice_settings.default_payment_method
* or default_source.
*/
@SerializedName("default_payment_method")
Object defaultPaymentMethod;
/**
* ID of the default payment source for the subscription. It must belong to the customer
* associated with the subscription and be in a chargeable state. If {@code
* default_payment_method} is also set, {@code default_payment_method} will take precedence. If
* neither are set, invoices will use the customer's invoice_settings.default_payment_method
* or default_source.
*/
@SerializedName("default_source")
Object defaultSource;
/**
* The tax rates that will apply to any subscription item that does not have {@code tax_rates}
* set. Invoices created will have their {@code default_tax_rates} populated from the
* subscription. Pass an empty string to remove previously-defined tax rates.
*/
@SerializedName("default_tax_rates")
Object defaultTaxRates;
/**
* Specifies which fields in the response should be expanded.
*/
@SerializedName("expand")
List expand;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* A list of up to 20 subscription items, each with an attached price.
*/
@SerializedName("items")
List- items;
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format. Individual keys can be unset by posting an empty value to them. All keys can
* be unset by posting an empty value to {@code metadata}.
*/
@SerializedName("metadata")
Object metadata;
/**
* Indicates if a customer is on or off-session while an invoice payment is attempted.
*/
@SerializedName("off_session")
Boolean offSession;
/**
* If specified, payment collection for this subscription will be paused.
*/
@SerializedName("pause_collection")
Object pauseCollection;
/**
* Use {@code allow_incomplete} to transition the subscription to {@code status=past_due} if a
* payment is required but cannot be paid. This allows you to manage scenarios where additional
* user actions are needed to pay a subscription's invoice. For example, SCA regulation may
* require 3DS authentication to complete payment. See the SCA Migration
* Guide for Billing to learn more. This is the default behavior.
*
*
Use {@code default_incomplete} to transition the subscription to {@code status=past_due}
* when payment is required and await explicit confirmation of the invoice's payment intent. This
* allows simpler management of scenarios where additional user actions are needed to pay a
* subscription’s invoice. Such as failed payments, SCA
* regulation, or collecting a mandate for a bank debit payment method.
*
*
Use {@code pending_if_incomplete} to update the subscription using pending updates. When
* you use {@code pending_if_incomplete} you can only pass the parameters supported
* by pending updates.
*
*
Use {@code error_if_incomplete} if you want Stripe to return an HTTP 402 status code if a
* subscription's invoice cannot be paid. For example, if a payment method requires 3DS
* authentication due to SCA regulation and further user action is needed, this parameter does not
* update the subscription and returns an error instead. This was the default behavior for API
* versions prior to 2019-03-14. See the changelog to learn more.
*/
@SerializedName("payment_behavior")
PaymentBehavior paymentBehavior;
/**
* Payment settings to pass to invoices created by the subscription.
*/
@SerializedName("payment_settings")
PaymentSettings paymentSettings;
/**
* Specifies an interval for how often to bill for any pending invoice items. It is analogous to
* calling Create an invoice for the
* given subscription at the specified interval.
*/
@SerializedName("pending_invoice_item_interval")
Object pendingInvoiceItemInterval;
/**
* The promotion code to apply to this subscription. A promotion code applied to a subscription
* will only affect invoices created for that particular subscription.
*/
@SerializedName("promotion_code")
Object promotionCode;
/**
* Determines how to handle prorations when the
* billing cycle changes (e.g., when switching plans, resetting {@code billing_cycle_anchor=now},
* or starting a trial), or if an item's {@code quantity} changes. Valid values are {@code
* create_prorations}, {@code none}, or {@code always_invoice}.
*
*
Passing {@code create_prorations} will cause proration invoice items to be created when
* applicable. These proration items will only be invoiced immediately under certain
* conditions. In order to always invoice immediately for prorations, pass {@code
* always_invoice}.
*
*
Prorations can be disabled by passing {@code none}.
*/
@SerializedName("proration_behavior")
ProrationBehavior prorationBehavior;
/**
* If set, the proration will be calculated as though the subscription was updated at the given
* time. This can be used to apply exactly the same proration that was previewed with upcoming invoice endpoint. It
* can also be used to implement custom proration logic, such as prorating by day instead of by
* second, by providing the time that you wish to use for proration calculations.
*/
@SerializedName("proration_date")
Long prorationDate;
/**
* If specified, the funds from the subscription's invoices will be transferred to the destination
* and the ID of the resulting transfers will be found on the resulting charges. This will be
* unset if you POST an empty value.
*/
@SerializedName("transfer_data")
Object transferData;
/**
* Unix timestamp representing the end of the trial period the customer will get before being
* charged for the first time. This will always overwrite any trials that might apply via a
* subscribed plan. If set, trial_end will override the default trial period of the plan the
* customer is being subscribed to. The special value {@code now} can be provided to end the
* customer's trial immediately. Can be at most two years from {@code billing_cycle_anchor}.
*/
@SerializedName("trial_end")
Object trialEnd;
/**
* Indicates if a plan's {@code trial_period_days} should be applied to the subscription. Setting
* {@code trial_end} per subscription is preferred, and this defaults to {@code false}. Setting
* this flag to {@code true} together with {@code trial_end} is not allowed.
*/
@SerializedName("trial_from_plan")
Boolean trialFromPlan;
private SubscriptionUpdateParams(List addInvoiceItems, BigDecimal applicationFeePercent, AutomaticTax automaticTax, BillingCycleAnchor billingCycleAnchor, Object billingThresholds, Object cancelAt, Boolean cancelAtPeriodEnd, CollectionMethod collectionMethod, Object coupon, Long daysUntilDue, Object defaultPaymentMethod, Object defaultSource, Object defaultTaxRates, List expand, Map extraParams, List- items, Object metadata, Boolean offSession, Object pauseCollection, PaymentBehavior paymentBehavior, PaymentSettings paymentSettings, Object pendingInvoiceItemInterval, Object promotionCode, ProrationBehavior prorationBehavior, Long prorationDate, Object transferData, Object trialEnd, Boolean trialFromPlan) {
this.addInvoiceItems = addInvoiceItems;
this.applicationFeePercent = applicationFeePercent;
this.automaticTax = automaticTax;
this.billingCycleAnchor = billingCycleAnchor;
this.billingThresholds = billingThresholds;
this.cancelAt = cancelAt;
this.cancelAtPeriodEnd = cancelAtPeriodEnd;
this.collectionMethod = collectionMethod;
this.coupon = coupon;
this.daysUntilDue = daysUntilDue;
this.defaultPaymentMethod = defaultPaymentMethod;
this.defaultSource = defaultSource;
this.defaultTaxRates = defaultTaxRates;
this.expand = expand;
this.extraParams = extraParams;
this.items = items;
this.metadata = metadata;
this.offSession = offSession;
this.pauseCollection = pauseCollection;
this.paymentBehavior = paymentBehavior;
this.paymentSettings = paymentSettings;
this.pendingInvoiceItemInterval = pendingInvoiceItemInterval;
this.promotionCode = promotionCode;
this.prorationBehavior = prorationBehavior;
this.prorationDate = prorationDate;
this.transferData = transferData;
this.trialEnd = trialEnd;
this.trialFromPlan = trialFromPlan;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private List
addInvoiceItems;
private BigDecimal applicationFeePercent;
private AutomaticTax automaticTax;
private BillingCycleAnchor billingCycleAnchor;
private Object billingThresholds;
private Object cancelAt;
private Boolean cancelAtPeriodEnd;
private CollectionMethod collectionMethod;
private Object coupon;
private Long daysUntilDue;
private Object defaultPaymentMethod;
private Object defaultSource;
private Object defaultTaxRates;
private List expand;
private Map extraParams;
private List- items;
private Object metadata;
private Boolean offSession;
private Object pauseCollection;
private PaymentBehavior paymentBehavior;
private PaymentSettings paymentSettings;
private Object pendingInvoiceItemInterval;
private Object promotionCode;
private ProrationBehavior prorationBehavior;
private Long prorationDate;
private Object transferData;
private Object trialEnd;
private Boolean trialFromPlan;
/**
* Finalize and obtain parameter instance from this builder.
*/
public SubscriptionUpdateParams build() {
return new SubscriptionUpdateParams(this.addInvoiceItems, this.applicationFeePercent, this.automaticTax, this.billingCycleAnchor, this.billingThresholds, this.cancelAt, this.cancelAtPeriodEnd, this.collectionMethod, this.coupon, this.daysUntilDue, this.defaultPaymentMethod, this.defaultSource, this.defaultTaxRates, this.expand, this.extraParams, this.items, this.metadata, this.offSession, this.pauseCollection, this.paymentBehavior, this.paymentSettings, this.pendingInvoiceItemInterval, this.promotionCode, this.prorationBehavior, this.prorationDate, this.transferData, this.trialEnd, this.trialFromPlan);
}
/**
* Add an element to `addInvoiceItems` list. A list is initialized for the first `add/addAll`
* call, and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams#addInvoiceItems} for the field documentation.
*/
public Builder addAddInvoiceItem(AddInvoiceItem element) {
if (this.addInvoiceItems == null) {
this.addInvoiceItems = new ArrayList<>();
}
this.addInvoiceItems.add(element);
return this;
}
/**
* Add all elements to `addInvoiceItems` list. A list is initialized for the first `add/addAll`
* call, and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams#addInvoiceItems} for the field documentation.
*/
public Builder addAllAddInvoiceItem(List
elements) {
if (this.addInvoiceItems == null) {
this.addInvoiceItems = new ArrayList<>();
}
this.addInvoiceItems.addAll(elements);
return this;
}
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents
* the percentage of the subscription invoice subtotal that will be transferred to the
* application owner's Stripe account. The request must be made by a platform account on a
* connected account in order to set an application fee percentage. For more information, see
* the application fees documentation.
*/
public Builder setApplicationFeePercent(BigDecimal applicationFeePercent) {
this.applicationFeePercent = applicationFeePercent;
return this;
}
/**
* Automatic tax settings for this subscription.
*/
public Builder setAutomaticTax(AutomaticTax automaticTax) {
this.automaticTax = automaticTax;
return this;
}
/**
* Either {@code now} or {@code unchanged}. Setting the value to {@code now} resets the
* subscription's billing cycle anchor to the current time. For more information, see the
* billing cycle documentation.
*/
public Builder setBillingCycleAnchor(BillingCycleAnchor billingCycleAnchor) {
this.billingCycleAnchor = billingCycleAnchor;
return this;
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. Pass an empty string to remove previously-defined thresholds.
*/
public Builder setBillingThresholds(BillingThresholds billingThresholds) {
this.billingThresholds = billingThresholds;
return this;
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. Pass an empty string to remove previously-defined thresholds.
*/
public Builder setBillingThresholds(EmptyParam billingThresholds) {
this.billingThresholds = billingThresholds;
return this;
}
/**
* A timestamp at which the subscription should cancel. If set to a date before the current
* period ends, this will cause a proration if prorations have been enabled using {@code
* proration_behavior}. If set during a future period, this will always cause a proration for
* that period.
*/
public Builder setCancelAt(Long cancelAt) {
this.cancelAt = cancelAt;
return this;
}
/**
* A timestamp at which the subscription should cancel. If set to a date before the current
* period ends, this will cause a proration if prorations have been enabled using {@code
* proration_behavior}. If set during a future period, this will always cause a proration for
* that period.
*/
public Builder setCancelAt(EmptyParam cancelAt) {
this.cancelAt = cancelAt;
return this;
}
/**
* Boolean indicating whether this subscription should cancel at the end of the current period.
*/
public Builder setCancelAtPeriodEnd(Boolean cancelAtPeriodEnd) {
this.cancelAtPeriodEnd = cancelAtPeriodEnd;
return this;
}
/**
* Either {@code charge_automatically}, or {@code send_invoice}. When charging automatically,
* Stripe will attempt to pay this subscription at the end of the cycle using the default source
* attached to the customer. When sending an invoice, Stripe will email your customer an invoice
* with payment instructions. Defaults to {@code charge_automatically}.
*/
public Builder setCollectionMethod(CollectionMethod collectionMethod) {
this.collectionMethod = collectionMethod;
return this;
}
/**
* The ID of the coupon to apply to this subscription. A coupon applied to a subscription will
* only affect invoices created for that particular subscription.
*/
public Builder setCoupon(String coupon) {
this.coupon = coupon;
return this;
}
/**
* The ID of the coupon to apply to this subscription. A coupon applied to a subscription will
* only affect invoices created for that particular subscription.
*/
public Builder setCoupon(EmptyParam coupon) {
this.coupon = coupon;
return this;
}
/**
* Number of days a customer has to pay invoices generated by this subscription. Valid only for
* subscriptions where {@code collection_method} is set to {@code send_invoice}.
*/
public Builder setDaysUntilDue(Long daysUntilDue) {
this.daysUntilDue = daysUntilDue;
return this;
}
/**
* ID of the default payment method for the subscription. It must belong to the customer
* associated with the subscription. This takes precedence over {@code default_source}. If
* neither are set, invoices will use the customer's invoice_settings.default_payment_method
* or default_source.
*/
public Builder setDefaultPaymentMethod(String defaultPaymentMethod) {
this.defaultPaymentMethod = defaultPaymentMethod;
return this;
}
/**
* ID of the default payment method for the subscription. It must belong to the customer
* associated with the subscription. This takes precedence over {@code default_source}. If
* neither are set, invoices will use the customer's invoice_settings.default_payment_method
* or default_source.
*/
public Builder setDefaultPaymentMethod(EmptyParam defaultPaymentMethod) {
this.defaultPaymentMethod = defaultPaymentMethod;
return this;
}
/**
* ID of the default payment source for the subscription. It must belong to the customer
* associated with the subscription and be in a chargeable state. If {@code
* default_payment_method} is also set, {@code default_payment_method} will take precedence. If
* neither are set, invoices will use the customer's invoice_settings.default_payment_method
* or default_source.
*/
public Builder setDefaultSource(String defaultSource) {
this.defaultSource = defaultSource;
return this;
}
/**
* ID of the default payment source for the subscription. It must belong to the customer
* associated with the subscription and be in a chargeable state. If {@code
* default_payment_method} is also set, {@code default_payment_method} will take precedence. If
* neither are set, invoices will use the customer's invoice_settings.default_payment_method
* or default_source.
*/
public Builder setDefaultSource(EmptyParam defaultSource) {
this.defaultSource = defaultSource;
return this;
}
/**
* Add an element to `defaultTaxRates` list. A list is initialized for the first `add/addAll`
* call, and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams#defaultTaxRates} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addDefaultTaxRate(String element) {
if (this.defaultTaxRates == null || this.defaultTaxRates instanceof EmptyParam) {
this.defaultTaxRates = new ArrayList();
}
((List) this.defaultTaxRates).add(element);
return this;
}
/**
* Add all elements to `defaultTaxRates` list. A list is initialized for the first `add/addAll`
* call, and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams#defaultTaxRates} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addAllDefaultTaxRate(List elements) {
if (this.defaultTaxRates == null || this.defaultTaxRates instanceof EmptyParam) {
this.defaultTaxRates = new ArrayList();
}
((List) this.defaultTaxRates).addAll(elements);
return this;
}
/**
* The tax rates that will apply to any subscription item that does not have {@code tax_rates}
* set. Invoices created will have their {@code default_tax_rates} populated from the
* subscription. Pass an empty string to remove previously-defined tax rates.
*/
public Builder setDefaultTaxRates(EmptyParam defaultTaxRates) {
this.defaultTaxRates = defaultTaxRates;
return this;
}
/**
* The tax rates that will apply to any subscription item that does not have {@code tax_rates}
* set. Invoices created will have their {@code default_tax_rates} populated from the
* subscription. Pass an empty string to remove previously-defined tax rates.
*/
public Builder setDefaultTaxRates(List defaultTaxRates) {
this.defaultTaxRates = defaultTaxRates;
return this;
}
/**
* Add an element to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams#expand} for the field documentation.
*/
public Builder addExpand(String element) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.add(element);
return this;
}
/**
* Add all elements to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams#expand} for the field documentation.
*/
public Builder addAllExpand(List elements) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.addAll(elements);
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionUpdateParams#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionUpdateParams#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Add an element to `items` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams#items} for the field documentation.
*/
public Builder addItem(Item element) {
if (this.items == null) {
this.items = new ArrayList<>();
}
this.items.add(element);
return this;
}
/**
* Add all elements to `items` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams#items} for the field documentation.
*/
public Builder addAllItem(List- elements) {
if (this.items == null) {
this.items = new ArrayList<>();
}
this.items.addAll(elements);
return this;
}
/**
* Add a key/value pair to `metadata` map. A map is initialized for the first `put/putAll` call,
* and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionUpdateParams#metadata} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder putMetadata(String key, String value) {
if (this.metadata == null || this.metadata instanceof EmptyParam) {
this.metadata = new HashMap
();
}
((Map) this.metadata).put(key, value);
return this;
}
/**
* Add all map key/value pairs to `metadata` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionUpdateParams#metadata} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder putAllMetadata(Map map) {
if (this.metadata == null || this.metadata instanceof EmptyParam) {
this.metadata = new HashMap();
}
((Map) this.metadata).putAll(map);
return this;
}
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format. Individual keys can be unset by posting an empty value to them. All keys
* can be unset by posting an empty value to {@code metadata}.
*/
public Builder setMetadata(EmptyParam metadata) {
this.metadata = metadata;
return this;
}
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format. Individual keys can be unset by posting an empty value to them. All keys
* can be unset by posting an empty value to {@code metadata}.
*/
public Builder setMetadata(Map metadata) {
this.metadata = metadata;
return this;
}
/**
* Indicates if a customer is on or off-session while an invoice payment is attempted.
*/
public Builder setOffSession(Boolean offSession) {
this.offSession = offSession;
return this;
}
/**
* If specified, payment collection for this subscription will be paused.
*/
public Builder setPauseCollection(PauseCollection pauseCollection) {
this.pauseCollection = pauseCollection;
return this;
}
/**
* If specified, payment collection for this subscription will be paused.
*/
public Builder setPauseCollection(EmptyParam pauseCollection) {
this.pauseCollection = pauseCollection;
return this;
}
/**
* Use {@code allow_incomplete} to transition the subscription to {@code status=past_due} if a
* payment is required but cannot be paid. This allows you to manage scenarios where additional
* user actions are needed to pay a subscription's invoice. For example, SCA regulation may
* require 3DS authentication to complete payment. See the SCA Migration
* Guide for Billing to learn more. This is the default behavior.
*
* Use {@code default_incomplete} to transition the subscription to {@code status=past_due}
* when payment is required and await explicit confirmation of the invoice's payment intent.
* This allows simpler management of scenarios where additional user actions are needed to pay a
* subscription’s invoice. Such as failed payments, SCA
* regulation, or collecting a mandate for a bank debit payment method.
*
*
Use {@code pending_if_incomplete} to update the subscription using pending updates.
* When you use {@code pending_if_incomplete} you can only pass the parameters supported
* by pending updates.
*
*
Use {@code error_if_incomplete} if you want Stripe to return an HTTP 402 status code if a
* subscription's invoice cannot be paid. For example, if a payment method requires 3DS
* authentication due to SCA regulation and further user action is needed, this parameter does
* not update the subscription and returns an error instead. This was the default behavior for
* API versions prior to 2019-03-14. See the changelog to learn more.
*/
public Builder setPaymentBehavior(PaymentBehavior paymentBehavior) {
this.paymentBehavior = paymentBehavior;
return this;
}
/**
* Payment settings to pass to invoices created by the subscription.
*/
public Builder setPaymentSettings(PaymentSettings paymentSettings) {
this.paymentSettings = paymentSettings;
return this;
}
/**
* Specifies an interval for how often to bill for any pending invoice items. It is analogous to
* calling Create an invoice for the
* given subscription at the specified interval.
*/
public Builder setPendingInvoiceItemInterval(PendingInvoiceItemInterval pendingInvoiceItemInterval) {
this.pendingInvoiceItemInterval = pendingInvoiceItemInterval;
return this;
}
/**
* Specifies an interval for how often to bill for any pending invoice items. It is analogous to
* calling Create an invoice for the
* given subscription at the specified interval.
*/
public Builder setPendingInvoiceItemInterval(EmptyParam pendingInvoiceItemInterval) {
this.pendingInvoiceItemInterval = pendingInvoiceItemInterval;
return this;
}
/**
* The promotion code to apply to this subscription. A promotion code applied to a subscription
* will only affect invoices created for that particular subscription.
*/
public Builder setPromotionCode(String promotionCode) {
this.promotionCode = promotionCode;
return this;
}
/**
* The promotion code to apply to this subscription. A promotion code applied to a subscription
* will only affect invoices created for that particular subscription.
*/
public Builder setPromotionCode(EmptyParam promotionCode) {
this.promotionCode = promotionCode;
return this;
}
/**
* Determines how to handle prorations when the
* billing cycle changes (e.g., when switching plans, resetting {@code
* billing_cycle_anchor=now}, or starting a trial), or if an item's {@code quantity} changes.
* Valid values are {@code create_prorations}, {@code none}, or {@code always_invoice}.
*
*
Passing {@code create_prorations} will cause proration invoice items to be created when
* applicable. These proration items will only be invoiced immediately under certain
* conditions. In order to always invoice immediately for prorations, pass {@code
* always_invoice}.
*
*
Prorations can be disabled by passing {@code none}.
*/
public Builder setProrationBehavior(ProrationBehavior prorationBehavior) {
this.prorationBehavior = prorationBehavior;
return this;
}
/**
* If set, the proration will be calculated as though the subscription was updated at the given
* time. This can be used to apply exactly the same proration that was previewed with upcoming invoice endpoint.
* It can also be used to implement custom proration logic, such as prorating by day instead of
* by second, by providing the time that you wish to use for proration calculations.
*/
public Builder setProrationDate(Long prorationDate) {
this.prorationDate = prorationDate;
return this;
}
/**
* If specified, the funds from the subscription's invoices will be transferred to the
* destination and the ID of the resulting transfers will be found on the resulting charges.
* This will be unset if you POST an empty value.
*/
public Builder setTransferData(TransferData transferData) {
this.transferData = transferData;
return this;
}
/**
* If specified, the funds from the subscription's invoices will be transferred to the
* destination and the ID of the resulting transfers will be found on the resulting charges.
* This will be unset if you POST an empty value.
*/
public Builder setTransferData(EmptyParam transferData) {
this.transferData = transferData;
return this;
}
/**
* Unix timestamp representing the end of the trial period the customer will get before being
* charged for the first time. This will always overwrite any trials that might apply via a
* subscribed plan. If set, trial_end will override the default trial period of the plan the
* customer is being subscribed to. The special value {@code now} can be provided to end the
* customer's trial immediately. Can be at most two years from {@code billing_cycle_anchor}.
*/
public Builder setTrialEnd(TrialEnd trialEnd) {
this.trialEnd = trialEnd;
return this;
}
/**
* Unix timestamp representing the end of the trial period the customer will get before being
* charged for the first time. This will always overwrite any trials that might apply via a
* subscribed plan. If set, trial_end will override the default trial period of the plan the
* customer is being subscribed to. The special value {@code now} can be provided to end the
* customer's trial immediately. Can be at most two years from {@code billing_cycle_anchor}.
*/
public Builder setTrialEnd(Long trialEnd) {
this.trialEnd = trialEnd;
return this;
}
/**
* Indicates if a plan's {@code trial_period_days} should be applied to the subscription.
* Setting {@code trial_end} per subscription is preferred, and this defaults to {@code false}.
* Setting this flag to {@code true} together with {@code trial_end} is not allowed.
*/
public Builder setTrialFromPlan(Boolean trialFromPlan) {
this.trialFromPlan = trialFromPlan;
return this;
}
}
public static class AddInvoiceItem {
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* The ID of the price object.
*/
@SerializedName("price")
Object price;
/**
* Data used to generate a new Price object
* inline.
*/
@SerializedName("price_data")
PriceData priceData;
/**
* Quantity for this item. Defaults to 1.
*/
@SerializedName("quantity")
Long quantity;
/**
* The tax rates which apply to the item. When set, the {@code default_tax_rates} do not apply
* to this item.
*/
@SerializedName("tax_rates")
Object taxRates;
private AddInvoiceItem(Map extraParams, Object price, PriceData priceData, Long quantity, Object taxRates) {
this.extraParams = extraParams;
this.price = price;
this.priceData = priceData;
this.quantity = quantity;
this.taxRates = taxRates;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private Object price;
private PriceData priceData;
private Long quantity;
private Object taxRates;
/**
* Finalize and obtain parameter instance from this builder.
*/
public AddInvoiceItem build() {
return new AddInvoiceItem(this.extraParams, this.price, this.priceData, this.quantity, this.taxRates);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionUpdateParams.AddInvoiceItem#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionUpdateParams.AddInvoiceItem#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* The ID of the price object.
*/
public Builder setPrice(String price) {
this.price = price;
return this;
}
/**
* The ID of the price object.
*/
public Builder setPrice(EmptyParam price) {
this.price = price;
return this;
}
/**
* Data used to generate a new Price object
* inline.
*/
public Builder setPriceData(PriceData priceData) {
this.priceData = priceData;
return this;
}
/**
* Quantity for this item. Defaults to 1.
*/
public Builder setQuantity(Long quantity) {
this.quantity = quantity;
return this;
}
/**
* Add an element to `taxRates` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams.AddInvoiceItem#taxRates} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addTaxRate(String element) {
if (this.taxRates == null || this.taxRates instanceof EmptyParam) {
this.taxRates = new ArrayList();
}
((List) this.taxRates).add(element);
return this;
}
/**
* Add all elements to `taxRates` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams.AddInvoiceItem#taxRates} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addAllTaxRate(List elements) {
if (this.taxRates == null || this.taxRates instanceof EmptyParam) {
this.taxRates = new ArrayList();
}
((List) this.taxRates).addAll(elements);
return this;
}
/**
* The tax rates which apply to the item. When set, the {@code default_tax_rates} do not apply
* to this item.
*/
public Builder setTaxRates(EmptyParam taxRates) {
this.taxRates = taxRates;
return this;
}
/**
* The tax rates which apply to the item. When set, the {@code default_tax_rates} do not apply
* to this item.
*/
public Builder setTaxRates(List taxRates) {
this.taxRates = taxRates;
return this;
}
}
public static class PriceData {
/**
* Three-letter ISO currency
* code, in lowercase. Must be a supported
* currency.
*/
@SerializedName("currency")
Object currency;
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/** The ID of the product that this price will belong to. */
@SerializedName("product")
Object product;
/**
* Specifies whether the price is considered inclusive of taxes or exclusive of taxes. One of
* {@code inclusive}, {@code exclusive}, or {@code unspecified}. Once specified as either
* {@code inclusive} or {@code exclusive}, it cannot be changed.
*/
@SerializedName("tax_behavior")
TaxBehavior taxBehavior;
/** A positive integer in %s (or 0 for a free price) representing how much to charge. */
@SerializedName("unit_amount")
Long unitAmount;
/**
* Same as {@code unit_amount}, but accepts a decimal value in %s with at most 12 decimal
* places. Only one of {@code unit_amount} and {@code unit_amount_decimal} can be set.
*/
@SerializedName("unit_amount_decimal")
Object unitAmountDecimal;
private PriceData(Object currency, Map extraParams, Object product, TaxBehavior taxBehavior, Long unitAmount, Object unitAmountDecimal) {
this.currency = currency;
this.extraParams = extraParams;
this.product = product;
this.taxBehavior = taxBehavior;
this.unitAmount = unitAmount;
this.unitAmountDecimal = unitAmountDecimal;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Object currency;
private Map extraParams;
private Object product;
private TaxBehavior taxBehavior;
private Long unitAmount;
private Object unitAmountDecimal;
/** Finalize and obtain parameter instance from this builder. */
public PriceData build() {
return new PriceData(this.currency, this.extraParams, this.product, this.taxBehavior, this.unitAmount, this.unitAmountDecimal);
}
/**
* Three-letter ISO currency
* code, in lowercase. Must be a supported
* currency.
*/
public Builder setCurrency(String currency) {
this.currency = currency;
return this;
}
/**
* Three-letter ISO currency
* code, in lowercase. Must be a supported
* currency.
*/
public Builder setCurrency(EmptyParam currency) {
this.currency = currency;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SubscriptionUpdateParams.AddInvoiceItem.PriceData#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SubscriptionUpdateParams.AddInvoiceItem.PriceData#extraParams} for the
* field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/** The ID of the product that this price will belong to. */
public Builder setProduct(String product) {
this.product = product;
return this;
}
/** The ID of the product that this price will belong to. */
public Builder setProduct(EmptyParam product) {
this.product = product;
return this;
}
/**
* Specifies whether the price is considered inclusive of taxes or exclusive of taxes. One
* of {@code inclusive}, {@code exclusive}, or {@code unspecified}. Once specified as either
* {@code inclusive} or {@code exclusive}, it cannot be changed.
*/
public Builder setTaxBehavior(TaxBehavior taxBehavior) {
this.taxBehavior = taxBehavior;
return this;
}
/** A positive integer in %s (or 0 for a free price) representing how much to charge. */
public Builder setUnitAmount(Long unitAmount) {
this.unitAmount = unitAmount;
return this;
}
/**
* Same as {@code unit_amount}, but accepts a decimal value in %s with at most 12 decimal
* places. Only one of {@code unit_amount} and {@code unit_amount_decimal} can be set.
*/
public Builder setUnitAmountDecimal(BigDecimal unitAmountDecimal) {
this.unitAmountDecimal = unitAmountDecimal;
return this;
}
public Builder setUnitAmountDecimal(EmptyParam unitAmountDecimal) {
this.unitAmountDecimal = unitAmountDecimal;
return this;
}
}
public enum TaxBehavior implements ApiRequestParams.EnumParam {
@SerializedName("exclusive")
EXCLUSIVE("exclusive"), @SerializedName("inclusive")
INCLUSIVE("inclusive"), @SerializedName("unspecified")
UNSPECIFIED("unspecified");
private final String value;
TaxBehavior(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getCurrency() {
return this.currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getProduct() {
return this.product;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TaxBehavior getTaxBehavior() {
return this.taxBehavior;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getUnitAmount() {
return this.unitAmount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getUnitAmountDecimal() {
return this.unitAmountDecimal;
}
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* The ID of the price object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getPrice() {
return this.price;
}
/**
* Data used to generate a new Price object
* inline.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PriceData getPriceData() {
return this.priceData;
}
/**
* Quantity for this item. Defaults to 1.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getQuantity() {
return this.quantity;
}
/**
* The tax rates which apply to the item. When set, the {@code default_tax_rates} do not apply
* to this item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getTaxRates() {
return this.taxRates;
}
}
/**
* Same as {@code unit_amount}, but accepts a decimal value in %s with at most 12 decimal
* places. Only one of {@code unit_amount} and {@code unit_amount_decimal} can be set.
*/
public static class AutomaticTax {
/**
* Enabled automatic tax calculation which will automatically compute tax rates on all invoices
* generated by the subscription.
*/
@SerializedName("enabled")
Boolean enabled;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
private AutomaticTax(Boolean enabled, Map extraParams) {
this.enabled = enabled;
this.extraParams = extraParams;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Boolean enabled;
private Map extraParams;
/** Finalize and obtain parameter instance from this builder. */
public AutomaticTax build() {
return new AutomaticTax(this.enabled, this.extraParams);
}
/**
* Enabled automatic tax calculation which will automatically compute tax rates on all
* invoices generated by the subscription.
*/
public Builder setEnabled(Boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionUpdateParams.AutomaticTax#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
}
/**
* Enabled automatic tax calculation which will automatically compute tax rates on all invoices
* generated by the subscription.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getEnabled() {
return this.enabled;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionUpdateParams.AutomaticTax#extraParams} for the field documentation.
*/
public static class BillingThresholds {
/**
* Monetary threshold that triggers the subscription to advance to a new billing period.
*/
@SerializedName("amount_gte")
Long amountGte;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Indicates if the {@code billing_cycle_anchor} should be reset when a threshold is reached. If
* true, {@code billing_cycle_anchor} will be updated to the date/time the threshold was last
* reached; otherwise, the value will remain unchanged.
*/
@SerializedName("reset_billing_cycle_anchor")
Boolean resetBillingCycleAnchor;
private BillingThresholds(Long amountGte, Map extraParams, Boolean resetBillingCycleAnchor) {
this.amountGte = amountGte;
this.extraParams = extraParams;
this.resetBillingCycleAnchor = resetBillingCycleAnchor;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Long amountGte;
private Map extraParams;
private Boolean resetBillingCycleAnchor;
/** Finalize and obtain parameter instance from this builder. */
public BillingThresholds build() {
return new BillingThresholds(this.amountGte, this.extraParams, this.resetBillingCycleAnchor);
}
/** Monetary threshold that triggers the subscription to advance to a new billing period. */
public Builder setAmountGte(Long amountGte) {
this.amountGte = amountGte;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionUpdateParams.BillingThresholds#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionUpdateParams.BillingThresholds#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
public Builder setResetBillingCycleAnchor(Boolean resetBillingCycleAnchor) {
this.resetBillingCycleAnchor = resetBillingCycleAnchor;
return this;
}
}
/**
* Monetary threshold that triggers the subscription to advance to a new billing period.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountGte() {
return this.amountGte;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Indicates if the {@code billing_cycle_anchor} should be reset when a threshold is reached. If
* true, {@code billing_cycle_anchor} will be updated to the date/time the threshold was last
* reached; otherwise, the value will remain unchanged.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getResetBillingCycleAnchor() {
return this.resetBillingCycleAnchor;
}
}
/**
* Indicates if the {@code billing_cycle_anchor} should be reset when a threshold is reached.
* If true, {@code billing_cycle_anchor} will be updated to the date/time the threshold was
* last reached; otherwise, the value will remain unchanged.
*/
public static class Item {
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. When updating, pass an empty string to remove previously-defined thresholds.
*/
@SerializedName("billing_thresholds")
Object billingThresholds;
/**
* Delete all usage for a given subscription item. Allowed only when {@code deleted} is set to
* {@code true} and the current plan's {@code usage_type} is {@code metered}.
*/
@SerializedName("clear_usage")
Boolean clearUsage;
/**
* A flag that, if set to {@code true}, will delete the specified item.
*/
@SerializedName("deleted")
Boolean deleted;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Subscription item to update.
*/
@SerializedName("id")
Object id;
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format. Individual keys can be unset by posting an empty value to them. All keys
* can be unset by posting an empty value to {@code metadata}.
*/
@SerializedName("metadata")
Object metadata;
/**
* Plan ID for this item, as a string.
*/
@SerializedName("plan")
Object plan;
/**
* The ID of the price object. When changing a subscription item's price, {@code quantity} is
* set to 1 unless a {@code quantity} parameter is provided.
*/
@SerializedName("price")
Object price;
/**
* Data used to generate a new Price object
* inline.
*/
@SerializedName("price_data")
PriceData priceData;
/**
* Quantity for this item.
*/
@SerializedName("quantity")
Long quantity;
/**
* A list of Tax Rate ids. These Tax Rates
* will override the {@code
* default_tax_rates} on the Subscription. When updating, pass an empty string to remove
* previously-defined tax rates.
*/
@SerializedName("tax_rates")
Object taxRates;
private Item(Object billingThresholds, Boolean clearUsage, Boolean deleted, Map extraParams, Object id, Object metadata, Object plan, Object price, PriceData priceData, Long quantity, Object taxRates) {
this.billingThresholds = billingThresholds;
this.clearUsage = clearUsage;
this.deleted = deleted;
this.extraParams = extraParams;
this.id = id;
this.metadata = metadata;
this.plan = plan;
this.price = price;
this.priceData = priceData;
this.quantity = quantity;
this.taxRates = taxRates;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Object billingThresholds;
private Boolean clearUsage;
private Boolean deleted;
private Map extraParams;
private Object id;
private Object metadata;
private Object plan;
private Object price;
private PriceData priceData;
private Long quantity;
private Object taxRates;
/**
* Finalize and obtain parameter instance from this builder.
*/
public Item build() {
return new Item(this.billingThresholds, this.clearUsage, this.deleted, this.extraParams, this.id, this.metadata, this.plan, this.price, this.priceData, this.quantity, this.taxRates);
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. When updating, pass an empty string to remove previously-defined
* thresholds.
*/
public Builder setBillingThresholds(BillingThresholds billingThresholds) {
this.billingThresholds = billingThresholds;
return this;
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. When updating, pass an empty string to remove previously-defined
* thresholds.
*/
public Builder setBillingThresholds(EmptyParam billingThresholds) {
this.billingThresholds = billingThresholds;
return this;
}
/**
* Delete all usage for a given subscription item. Allowed only when {@code deleted} is set to
* {@code true} and the current plan's {@code usage_type} is {@code metered}.
*/
public Builder setClearUsage(Boolean clearUsage) {
this.clearUsage = clearUsage;
return this;
}
/**
* A flag that, if set to {@code true}, will delete the specified item.
*/
public Builder setDeleted(Boolean deleted) {
this.deleted = deleted;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionUpdateParams.Item#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionUpdateParams.Item#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Subscription item to update.
*/
public Builder setId(String id) {
this.id = id;
return this;
}
/**
* Subscription item to update.
*/
public Builder setId(EmptyParam id) {
this.id = id;
return this;
}
/**
* Add a key/value pair to `metadata` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionUpdateParams.Item#metadata} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder putMetadata(String key, String value) {
if (this.metadata == null || this.metadata instanceof EmptyParam) {
this.metadata = new HashMap();
}
((Map) this.metadata).put(key, value);
return this;
}
/**
* Add all map key/value pairs to `metadata` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionUpdateParams.Item#metadata} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder putAllMetadata(Map map) {
if (this.metadata == null || this.metadata instanceof EmptyParam) {
this.metadata = new HashMap();
}
((Map) this.metadata).putAll(map);
return this;
}
/**
* Set of key-value pairs that you can
* attach to an object. This can be useful for storing additional information about the object
* in a structured format. Individual keys can be unset by posting an empty value to them. All
* keys can be unset by posting an empty value to {@code metadata}.
*/
public Builder setMetadata(EmptyParam metadata) {
this.metadata = metadata;
return this;
}
/**
* Set of key-value pairs that you can
* attach to an object. This can be useful for storing additional information about the object
* in a structured format. Individual keys can be unset by posting an empty value to them. All
* keys can be unset by posting an empty value to {@code metadata}.
*/
public Builder setMetadata(Map metadata) {
this.metadata = metadata;
return this;
}
/**
* Plan ID for this item, as a string.
*/
public Builder setPlan(String plan) {
this.plan = plan;
return this;
}
/**
* Plan ID for this item, as a string.
*/
public Builder setPlan(EmptyParam plan) {
this.plan = plan;
return this;
}
/**
* The ID of the price object. When changing a subscription item's price, {@code quantity} is
* set to 1 unless a {@code quantity} parameter is provided.
*/
public Builder setPrice(String price) {
this.price = price;
return this;
}
/**
* The ID of the price object. When changing a subscription item's price, {@code quantity} is
* set to 1 unless a {@code quantity} parameter is provided.
*/
public Builder setPrice(EmptyParam price) {
this.price = price;
return this;
}
/**
* Data used to generate a new Price object
* inline.
*/
public Builder setPriceData(PriceData priceData) {
this.priceData = priceData;
return this;
}
/**
* Quantity for this item.
*/
public Builder setQuantity(Long quantity) {
this.quantity = quantity;
return this;
}
/**
* Add an element to `taxRates` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams.Item#taxRates} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addTaxRate(String element) {
if (this.taxRates == null || this.taxRates instanceof EmptyParam) {
this.taxRates = new ArrayList();
}
((List) this.taxRates).add(element);
return this;
}
/**
* Add all elements to `taxRates` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionUpdateParams.Item#taxRates} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addAllTaxRate(List elements) {
if (this.taxRates == null || this.taxRates instanceof EmptyParam) {
this.taxRates = new ArrayList();
}
((List) this.taxRates).addAll(elements);
return this;
}
/**
* A list of Tax Rate ids. These Tax Rates
* will override the {@code
* default_tax_rates} on the Subscription. When updating, pass an empty string to remove
* previously-defined tax rates.
*/
public Builder setTaxRates(EmptyParam taxRates) {
this.taxRates = taxRates;
return this;
}
/**
* A list of Tax Rate ids. These Tax Rates
* will override the {@code
* default_tax_rates} on the Subscription. When updating, pass an empty string to remove
* previously-defined tax rates.
*/
public Builder setTaxRates(List taxRates) {
this.taxRates = taxRates;
return this;
}
}
public static class BillingThresholds {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Usage threshold that triggers the subscription to advance to a new billing period.
*/
@SerializedName("usage_gte")
Long usageGte;
private BillingThresholds(Map extraParams, Long usageGte) {
this.extraParams = extraParams;
this.usageGte = usageGte;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private Long usageGte;
/** Finalize and obtain parameter instance from this builder. */
public BillingThresholds build() {
return new BillingThresholds(this.extraParams, this.usageGte);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SubscriptionUpdateParams.Item.BillingThresholds#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SubscriptionUpdateParams.Item.BillingThresholds#extraParams} for the
* field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
public Builder setUsageGte(Long usageGte) {
this.usageGte = usageGte;
return this;
}
}
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Usage threshold that triggers the subscription to advance to a new billing period.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getUsageGte() {
return this.usageGte;
}
}
/**
* Usage threshold that triggers the subscription to advance to a new billing period.
*/
public static class PriceData {
/**
* Three-letter ISO currency
* code, in lowercase. Must be a supported
* currency.
*/
@SerializedName("currency")
Object currency;
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/** The ID of the product that this price will belong to. */
@SerializedName("product")
Object product;
/** The recurring components of a price such as {@code interval} and {@code usage_type}. */
@SerializedName("recurring")
Recurring recurring;
/**
* Specifies whether the price is considered inclusive of taxes or exclusive of taxes. One of
* {@code inclusive}, {@code exclusive}, or {@code unspecified}. Once specified as either
* {@code inclusive} or {@code exclusive}, it cannot be changed.
*/
@SerializedName("tax_behavior")
TaxBehavior taxBehavior;
/** A positive integer in %s (or 0 for a free price) representing how much to charge. */
@SerializedName("unit_amount")
Long unitAmount;
/**
* Same as {@code unit_amount}, but accepts a decimal value in %s with at most 12 decimal
* places. Only one of {@code unit_amount} and {@code unit_amount_decimal} can be set.
*/
@SerializedName("unit_amount_decimal")
Object unitAmountDecimal;
private PriceData(Object currency, Map extraParams, Object product, Recurring recurring, TaxBehavior taxBehavior, Long unitAmount, Object unitAmountDecimal) {
this.currency = currency;
this.extraParams = extraParams;
this.product = product;
this.recurring = recurring;
this.taxBehavior = taxBehavior;
this.unitAmount = unitAmount;
this.unitAmountDecimal = unitAmountDecimal;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Object currency;
private Map extraParams;
private Object product;
private Recurring recurring;
private TaxBehavior taxBehavior;
private Long unitAmount;
private Object unitAmountDecimal;
/** Finalize and obtain parameter instance from this builder. */
public PriceData build() {
return new PriceData(this.currency, this.extraParams, this.product, this.recurring, this.taxBehavior, this.unitAmount, this.unitAmountDecimal);
}
/**
* Three-letter ISO currency
* code, in lowercase. Must be a supported
* currency.
*/
public Builder setCurrency(String currency) {
this.currency = currency;
return this;
}
/**
* Three-letter ISO currency
* code, in lowercase. Must be a supported
* currency.
*/
public Builder setCurrency(EmptyParam currency) {
this.currency = currency;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SubscriptionUpdateParams.Item.PriceData#extraParams} for the field
* documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SubscriptionUpdateParams.Item.PriceData#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/** The ID of the product that this price will belong to. */
public Builder setProduct(String product) {
this.product = product;
return this;
}
/** The ID of the product that this price will belong to. */
public Builder setProduct(EmptyParam product) {
this.product = product;
return this;
}
/** The recurring components of a price such as {@code interval} and {@code usage_type}. */
public Builder setRecurring(Recurring recurring) {
this.recurring = recurring;
return this;
}
/**
* Specifies whether the price is considered inclusive of taxes or exclusive of taxes. One
* of {@code inclusive}, {@code exclusive}, or {@code unspecified}. Once specified as either
* {@code inclusive} or {@code exclusive}, it cannot be changed.
*/
public Builder setTaxBehavior(TaxBehavior taxBehavior) {
this.taxBehavior = taxBehavior;
return this;
}
/** A positive integer in %s (or 0 for a free price) representing how much to charge. */
public Builder setUnitAmount(Long unitAmount) {
this.unitAmount = unitAmount;
return this;
}
/**
* Same as {@code unit_amount}, but accepts a decimal value in %s with at most 12 decimal
* places. Only one of {@code unit_amount} and {@code unit_amount_decimal} can be set.
*/
public Builder setUnitAmountDecimal(BigDecimal unitAmountDecimal) {
this.unitAmountDecimal = unitAmountDecimal;
return this;
}
/**
* Same as {@code unit_amount}, but accepts a decimal value in %s with at most 12 decimal
* places. Only one of {@code unit_amount} and {@code unit_amount_decimal} can be set.
*/
public Builder setUnitAmountDecimal(EmptyParam unitAmountDecimal) {
this.unitAmountDecimal = unitAmountDecimal;
return this;
}
}
public static class Recurring {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field
* (serialized) name in this param object. Effectively, this map is flattened to its parent
* instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Specifies billing frequency. Either {@code day}, {@code week}, {@code month} or {@code
* year}.
*/
@SerializedName("interval")
Interval interval;
/**
* The number of intervals between subscription billings. For example, {@code
* interval=month} and {@code interval_count=3} bills every 3 months. Maximum of one year
* interval allowed (1 year, 12 months, or 52 weeks).
*/
@SerializedName("interval_count")
Long intervalCount;
private Recurring(Map extraParams, Interval interval, Long intervalCount) {
this.extraParams = extraParams;
this.interval = interval;
this.intervalCount = intervalCount;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private Interval interval;
private Long intervalCount;
/** Finalize and obtain parameter instance from this builder. */
public Recurring build() {
return new Recurring(this.extraParams, this.interval, this.intervalCount);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SubscriptionUpdateParams.Item.PriceData.Recurring#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SubscriptionUpdateParams.Item.PriceData.Recurring#extraParams} for the
* field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Specifies billing frequency. Either {@code day}, {@code week}, {@code month} or {@code
* year}.
*/
public Builder setInterval(Interval interval) {
this.interval = interval;
return this;
}
public Builder setIntervalCount(Long intervalCount) {
this.intervalCount = intervalCount;
return this;
}
}
public enum Interval implements ApiRequestParams.EnumParam {
@SerializedName("day")
DAY("day"), @SerializedName("month")
MONTH("month"), @SerializedName("week")
WEEK("week"), @SerializedName("year")
YEAR("year");
private final String value;
Interval(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Interval getInterval() {
return this.interval;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getIntervalCount() {
return this.intervalCount;
}
}
public enum TaxBehavior implements ApiRequestParams.EnumParam {
@SerializedName("exclusive")
EXCLUSIVE("exclusive"), @SerializedName("inclusive")
INCLUSIVE("inclusive"), @SerializedName("unspecified")
UNSPECIFIED("unspecified");
private final String value;
TaxBehavior(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getCurrency() {
return this.currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getProduct() {
return this.product;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Recurring getRecurring() {
return this.recurring;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TaxBehavior getTaxBehavior() {
return this.taxBehavior;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getUnitAmount() {
return this.unitAmount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getUnitAmountDecimal() {
return this.unitAmountDecimal;
}
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. When updating, pass an empty string to remove previously-defined thresholds.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getBillingThresholds() {
return this.billingThresholds;
}
/**
* Delete all usage for a given subscription item. Allowed only when {@code deleted} is set to
* {@code true} and the current plan's {@code usage_type} is {@code metered}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getClearUsage() {
return this.clearUsage;
}
/**
* A flag that, if set to {@code true}, will delete the specified item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getDeleted() {
return this.deleted;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Subscription item to update.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getId() {
return this.id;
}
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format. Individual keys can be unset by posting an empty value to them. All keys
* can be unset by posting an empty value to {@code metadata}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getMetadata() {
return this.metadata;
}
/**
* Plan ID for this item, as a string.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getPlan() {
return this.plan;
}
/**
* The ID of the price object. When changing a subscription item's price, {@code quantity} is
* set to 1 unless a {@code quantity} parameter is provided.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getPrice() {
return this.price;
}
/**
* Data used to generate a new Price object
* inline.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PriceData getPriceData() {
return this.priceData;
}
/**
* Quantity for this item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getQuantity() {
return this.quantity;
}
/**
* A list of Tax Rate ids. These Tax Rates
* will override the {@code
* default_tax_rates} on the Subscription. When updating, pass an empty string to remove
* previously-defined tax rates.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getTaxRates() {
return this.taxRates;
}
}
/**
* The number of intervals between subscription billings. For example, {@code
* interval=month} and {@code interval_count=3} bills every 3 months. Maximum of one year
* interval allowed (1 year, 12 months, or 52 weeks).
*/
public static class PauseCollection {
/**
* The payment collection behavior for this subscription while paused. One of {@code
* keep_as_draft}, {@code mark_uncollectible}, or {@code void}.
*/
@SerializedName("behavior")
Behavior behavior;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* The time after which the subscription will resume collecting payments.
*/
@SerializedName("resumes_at")
Long resumesAt;
private PauseCollection(Behavior behavior, Map extraParams, Long resumesAt) {
this.behavior = behavior;
this.extraParams = extraParams;
this.resumesAt = resumesAt;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Behavior behavior;
private Map extraParams;
private Long resumesAt;
/**
* Finalize and obtain parameter instance from this builder.
*/
public PauseCollection build() {
return new PauseCollection(this.behavior, this.extraParams, this.resumesAt);
}
/**
* The payment collection behavior for this subscription while paused. One of {@code
* keep_as_draft}, {@code mark_uncollectible}, or {@code void}.
*/
public Builder setBehavior(Behavior behavior) {
this.behavior = behavior;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionUpdateParams.PauseCollection#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionUpdateParams.PauseCollection#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* The time after which the subscription will resume collecting payments.
*/
public Builder setResumesAt(Long resumesAt) {
this.resumesAt = resumesAt;
return this;
}
}
public enum Behavior implements ApiRequestParams.EnumParam {
@SerializedName("keep_as_draft")
KEEP_AS_DRAFT("keep_as_draft"), @SerializedName("mark_uncollectible")
MARK_UNCOLLECTIBLE("mark_uncollectible"), @SerializedName("void")
VOID("void");
private final String value;
Behavior(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
/**
* The payment collection behavior for this subscription while paused. One of {@code
* keep_as_draft}, {@code mark_uncollectible}, or {@code void}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Behavior getBehavior() {
return this.behavior;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* The time after which the subscription will resume collecting payments.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getResumesAt() {
return this.resumesAt;
}
}
public static class PaymentSettings {
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Payment-method-specific configuration to provide to invoices created by the subscription.
*/
@SerializedName("payment_method_options")
PaymentMethodOptions paymentMethodOptions;
/**
* The list of payment method types (e.g. card) to provide to the invoice’s PaymentIntent. If
* not set, Stripe attempts to automatically determine the types to use by looking at the
* invoice’s default payment method, the subscription’s default payment method, the customer’s
* default payment method, and your invoice template settings.
*/
@SerializedName("payment_method_types")
Object paymentMethodTypes;
private PaymentSettings(Map extraParams, PaymentMethodOptions paymentMethodOptions, Object paymentMethodTypes) {
this.extraParams = extraParams;
this.paymentMethodOptions = paymentMethodOptions;
this.paymentMethodTypes = paymentMethodTypes;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private PaymentMethodOptions paymentMethodOptions;
private Object paymentMethodTypes;
/**
* Finalize and obtain parameter instance from this builder.
*/
public PaymentSettings build() {
return new PaymentSettings(this.extraParams, this.paymentMethodOptions, this.paymentMethodTypes);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionUpdateParams.PaymentSettings#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionUpdateParams.PaymentSettings#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Payment-method-specific configuration to provide to invoices created by the subscription.
*/
public Builder setPaymentMethodOptions(PaymentMethodOptions paymentMethodOptions) {
this.paymentMethodOptions = paymentMethodOptions;
return this;
}
/**
* Add an element to `paymentMethodTypes` list. A list is initialized for the first
* `add/addAll` call, and subsequent calls adds additional elements to the original list. See
* {@link SubscriptionUpdateParams.PaymentSettings#paymentMethodTypes} for the field
* documentation.
*/
@SuppressWarnings("unchecked")
public Builder addPaymentMethodType(PaymentMethodType element) {
if (this.paymentMethodTypes == null || this.paymentMethodTypes instanceof EmptyParam) {
this.paymentMethodTypes = new ArrayList();
}
((List) this.paymentMethodTypes).add(element);
return this;
}
/**
* Add all elements to `paymentMethodTypes` list. A list is initialized for the first
* `add/addAll` call, and subsequent calls adds additional elements to the original list. See
* {@link SubscriptionUpdateParams.PaymentSettings#paymentMethodTypes} for the field
* documentation.
*/
@SuppressWarnings("unchecked")
public Builder addAllPaymentMethodType(List elements) {
if (this.paymentMethodTypes == null || this.paymentMethodTypes instanceof EmptyParam) {
this.paymentMethodTypes = new ArrayList();
}
((List) this.paymentMethodTypes).addAll(elements);
return this;
}
/**
* The list of payment method types (e.g. card) to provide to the invoice’s PaymentIntent. If
* not set, Stripe attempts to automatically determine the types to use by looking at the
* invoice’s default payment method, the subscription’s default payment method, the customer’s
* default payment method, and your invoice template settings.
*/
public Builder setPaymentMethodTypes(EmptyParam paymentMethodTypes) {
this.paymentMethodTypes = paymentMethodTypes;
return this;
}
/**
* The list of payment method types (e.g. card) to provide to the invoice’s PaymentIntent. If
* not set, Stripe attempts to automatically determine the types to use by looking at the
* invoice’s default payment method, the subscription’s default payment method, the customer’s
* default payment method, and your invoice template settings.
*/
public Builder setPaymentMethodTypes(List paymentMethodTypes) {
this.paymentMethodTypes = paymentMethodTypes;
return this;
}
}
public static class PaymentMethodOptions {
/**
* This sub-hash contains details about the Canadian pre-authorized debit payment method
* options to pass to the invoice’s PaymentIntent.
*/
@SerializedName("acss_debit")
Object acssDebit;
/**
* This sub-hash contains details about the Bancontact payment method options to pass to the
* invoice’s PaymentIntent.
*/
@SerializedName("bancontact")
Object bancontact;
/**
* This sub-hash contains details about the Card payment method options to pass to the
* invoice’s PaymentIntent.
*/
@SerializedName("card")
Object card;
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
private PaymentMethodOptions(Object acssDebit, Object bancontact, Object card, Map extraParams) {
this.acssDebit = acssDebit;
this.bancontact = bancontact;
this.card = card;
this.extraParams = extraParams;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Object acssDebit;
private Object bancontact;
private Object card;
private Map extraParams;
/**
* Finalize and obtain parameter instance from this builder.
*/
public PaymentMethodOptions build() {
return new PaymentMethodOptions(this.acssDebit, this.bancontact, this.card, this.extraParams);
}
/**
* This sub-hash contains details about the Canadian pre-authorized debit payment method
* options to pass to the invoice’s PaymentIntent.
*/
public Builder setAcssDebit(AcssDebit acssDebit) {
this.acssDebit = acssDebit;
return this;
}
/**
* This sub-hash contains details about the Canadian pre-authorized debit payment method
* options to pass to the invoice’s PaymentIntent.
*/
public Builder setAcssDebit(EmptyParam acssDebit) {
this.acssDebit = acssDebit;
return this;
}
/**
* This sub-hash contains details about the Bancontact payment method options to pass to the
* invoice’s PaymentIntent.
*/
public Builder setBancontact(Bancontact bancontact) {
this.bancontact = bancontact;
return this;
}
/**
* This sub-hash contains details about the Bancontact payment method options to pass to the
* invoice’s PaymentIntent.
*/
public Builder setBancontact(EmptyParam bancontact) {
this.bancontact = bancontact;
return this;
}
/**
* This sub-hash contains details about the Card payment method options to pass to the
* invoice’s PaymentIntent.
*/
public Builder setCard(Card card) {
this.card = card;
return this;
}
/**
* This sub-hash contains details about the Card payment method options to pass to the
* invoice’s PaymentIntent.
*/
public Builder setCard(EmptyParam card) {
this.card = card;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* SubscriptionUpdateParams.PaymentSettings.PaymentMethodOptions#extraParams} for the field
* documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* SubscriptionUpdateParams.PaymentSettings.PaymentMethodOptions#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
}
public static class AcssDebit {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field
* (serialized) name in this param object. Effectively, this map is flattened to its parent
* instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Additional fields for Mandate creation.
*/
@SerializedName("mandate_options")
MandateOptions mandateOptions;
/**
* Verification method for the intent.
*/
@SerializedName("verification_method")
VerificationMethod verificationMethod;
private AcssDebit(Map extraParams, MandateOptions mandateOptions, VerificationMethod verificationMethod) {
this.extraParams = extraParams;
this.mandateOptions = mandateOptions;
this.verificationMethod = verificationMethod;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private MandateOptions mandateOptions;
private VerificationMethod verificationMethod;
/**
* Finalize and obtain parameter instance from this builder.
*/
public AcssDebit build() {
return new AcssDebit(this.extraParams, this.mandateOptions, this.verificationMethod);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* SubscriptionUpdateParams.PaymentSettings.PaymentMethodOptions.AcssDebit#extraParams}
* for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* SubscriptionUpdateParams.PaymentSettings.PaymentMethodOptions.AcssDebit#extraParams}
* for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Additional fields for Mandate creation.
*/
public Builder setMandateOptions(MandateOptions mandateOptions) {
this.mandateOptions = mandateOptions;
return this;
}
/**
* Verification method for the intent.
*/
public Builder setVerificationMethod(VerificationMethod verificationMethod) {
this.verificationMethod = verificationMethod;
return this;
}
}
public static class MandateOptions {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field
* (serialized) name in this param object. Effectively, this map is flattened to its
* parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Transaction type of the mandate.
*/
@SerializedName("transaction_type")
TransactionType transactionType;
private MandateOptions(Map extraParams, TransactionType transactionType) {
this.extraParams = extraParams;
this.transactionType = transactionType;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private TransactionType transactionType;
/**
* Finalize and obtain parameter instance from this builder.
*/
public MandateOptions build() {
return new MandateOptions(this.extraParams, this.transactionType);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the
* original map. See {@link
* SubscriptionUpdateParams.PaymentSettings.PaymentMethodOptions.AcssDebit.MandateOptions#extraParams}
* for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the
* original map. See {@link
* SubscriptionUpdateParams.PaymentSettings.PaymentMethodOptions.AcssDebit.MandateOptions#extraParams}
* for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Transaction type of the mandate.
*/
public Builder setTransactionType(TransactionType transactionType) {
this.transactionType = transactionType;
return this;
}
}
public enum TransactionType implements ApiRequestParams.EnumParam {
@SerializedName("business")
BUSINESS("business"), @SerializedName("personal")
PERSONAL("personal");
private final String value;
TransactionType(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field
* (serialized) name in this param object. Effectively, this map is flattened to its
* parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Transaction type of the mandate.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TransactionType getTransactionType() {
return this.transactionType;
}
}
public enum VerificationMethod implements ApiRequestParams.EnumParam {
@SerializedName("automatic")
AUTOMATIC("automatic"), @SerializedName("instant")
INSTANT("instant"), @SerializedName("microdeposits")
MICRODEPOSITS("microdeposits");
private final String value;
VerificationMethod(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field
* (serialized) name in this param object. Effectively, this map is flattened to its parent
* instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Additional fields for Mandate creation.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public MandateOptions getMandateOptions() {
return this.mandateOptions;
}
/**
* Verification method for the intent.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public VerificationMethod getVerificationMethod() {
return this.verificationMethod;
}
}
public static class Bancontact {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field
* (serialized) name in this param object. Effectively, this map is flattened to its parent
* instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Preferred language of the Bancontact authorization page that the customer is redirected
* to.
*/
@SerializedName("preferred_language")
PreferredLanguage preferredLanguage;
private Bancontact(Map extraParams, PreferredLanguage preferredLanguage) {
this.extraParams = extraParams;
this.preferredLanguage = preferredLanguage;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private PreferredLanguage preferredLanguage;
/**
* Finalize and obtain parameter instance from this builder.
*/
public Bancontact build() {
return new Bancontact(this.extraParams, this.preferredLanguage);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* SubscriptionUpdateParams.PaymentSettings.PaymentMethodOptions.Bancontact#extraParams}
* for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* SubscriptionUpdateParams.PaymentSettings.PaymentMethodOptions.Bancontact#extraParams}
* for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Preferred language of the Bancontact authorization page that the customer is redirected
* to.
*/
public Builder setPreferredLanguage(PreferredLanguage preferredLanguage) {
this.preferredLanguage = preferredLanguage;
return this;
}
}
public enum PreferredLanguage implements ApiRequestParams.EnumParam {
@SerializedName("de")
DE("de"), @SerializedName("en")
EN("en"), @SerializedName("fr")
FR("fr"), @SerializedName("nl")
NL("nl");
private final String value;
PreferredLanguage(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field
* (serialized) name in this param object. Effectively, this map is flattened to its parent
* instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Preferred language of the Bancontact authorization page that the customer is redirected
* to.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PreferredLanguage getPreferredLanguage() {
return this.preferredLanguage;
}
}
public static class Card {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field
* (serialized) name in this param object. Effectively, this map is flattened to its parent
* instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* We strongly recommend that you rely on our SCA Engine to automatically prompt your
* customers for authentication based on risk level and other requirements.
* However, if you wish to request 3D Secure based on logic from your own fraud engine,
* provide this option. Read our guide on manually requesting 3D
* Secure for more information on how this configuration interacts with Radar and our
* SCA Engine.
*/
@SerializedName("request_three_d_secure")
RequestThreeDSecure requestThreeDSecure;
private Card(Map extraParams, RequestThreeDSecure requestThreeDSecure) {
this.extraParams = extraParams;
this.requestThreeDSecure = requestThreeDSecure;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private RequestThreeDSecure requestThreeDSecure;
/** Finalize and obtain parameter instance from this builder. */
public Card build() {
return new Card(this.extraParams, this.requestThreeDSecure);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* SubscriptionUpdateParams.PaymentSettings.PaymentMethodOptions.Card#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link
* SubscriptionUpdateParams.PaymentSettings.PaymentMethodOptions.Card#extraParams} for the
* field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
public Builder setRequestThreeDSecure(RequestThreeDSecure requestThreeDSecure) {
this.requestThreeDSecure = requestThreeDSecure;
return this;
}
}
public enum RequestThreeDSecure implements ApiRequestParams.EnumParam {
@SerializedName("any")
ANY("any"), @SerializedName("automatic")
AUTOMATIC("automatic");
private final String value;
RequestThreeDSecure(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public RequestThreeDSecure getRequestThreeDSecure() {
return this.requestThreeDSecure;
}
}
/**
* This sub-hash contains details about the Canadian pre-authorized debit payment method
* options to pass to the invoice’s PaymentIntent.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getAcssDebit() {
return this.acssDebit;
}
/**
* This sub-hash contains details about the Bancontact payment method options to pass to the
* invoice’s PaymentIntent.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getBancontact() {
return this.bancontact;
}
/**
* This sub-hash contains details about the Card payment method options to pass to the
* invoice’s PaymentIntent.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getCard() {
return this.card;
}
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
}
/**
* We strongly recommend that you rely on our SCA Engine to automatically prompt your
* customers for authentication based on risk level and other requirements.
* However, if you wish to request 3D Secure based on logic from your own fraud engine,
* provide this option. Read our guide on manually requesting
* 3D Secure for more information on how this configuration interacts with Radar and
* our SCA Engine.
*/
public enum PaymentMethodType implements ApiRequestParams.EnumParam {
@SerializedName("ach_credit_transfer")
ACH_CREDIT_TRANSFER("ach_credit_transfer"), @SerializedName("ach_debit")
ACH_DEBIT("ach_debit"), @SerializedName("acss_debit")
ACSS_DEBIT("acss_debit"), @SerializedName("au_becs_debit")
AU_BECS_DEBIT("au_becs_debit"), @SerializedName("bacs_debit")
BACS_DEBIT("bacs_debit"), @SerializedName("bancontact")
BANCONTACT("bancontact"), @SerializedName("boleto")
BOLETO("boleto"), @SerializedName("card")
CARD("card"), @SerializedName("fpx")
FPX("fpx"), @SerializedName("giropay")
GIROPAY("giropay"), @SerializedName("ideal")
IDEAL("ideal"), @SerializedName("sepa_credit_transfer")
SEPA_CREDIT_TRANSFER("sepa_credit_transfer"), @SerializedName("sepa_debit")
SEPA_DEBIT("sepa_debit"), @SerializedName("sofort")
SOFORT("sofort"), @SerializedName("wechat_pay")
WECHAT_PAY("wechat_pay");
private final String value;
PaymentMethodType(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Payment-method-specific configuration to provide to invoices created by the subscription.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PaymentMethodOptions getPaymentMethodOptions() {
return this.paymentMethodOptions;
}
/**
* The list of payment method types (e.g. card) to provide to the invoice’s PaymentIntent. If
* not set, Stripe attempts to automatically determine the types to use by looking at the
* invoice’s default payment method, the subscription’s default payment method, the customer’s
* default payment method, and your invoice template settings.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getPaymentMethodTypes() {
return this.paymentMethodTypes;
}
}
public static class PendingInvoiceItemInterval {
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Specifies invoicing frequency. Either {@code day}, {@code week}, {@code month} or {@code
* year}.
*/
@SerializedName("interval")
Interval interval;
/**
* The number of intervals between invoices. For example, {@code interval=month} and {@code
* interval_count=3} bills every 3 months. Maximum of one year interval allowed (1 year, 12
* months, or 52 weeks).
*/
@SerializedName("interval_count")
Long intervalCount;
private PendingInvoiceItemInterval(Map extraParams, Interval interval, Long intervalCount) {
this.extraParams = extraParams;
this.interval = interval;
this.intervalCount = intervalCount;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private Interval interval;
private Long intervalCount;
/**
* Finalize and obtain parameter instance from this builder.
*/
public PendingInvoiceItemInterval build() {
return new PendingInvoiceItemInterval(this.extraParams, this.interval, this.intervalCount);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionUpdateParams.PendingInvoiceItemInterval#extraParams} for the field
* documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionUpdateParams.PendingInvoiceItemInterval#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Specifies invoicing frequency. Either {@code day}, {@code week}, {@code month} or {@code
* year}.
*/
public Builder setInterval(Interval interval) {
this.interval = interval;
return this;
}
/**
* The number of intervals between invoices. For example, {@code interval=month} and {@code
* interval_count=3} bills every 3 months. Maximum of one year interval allowed (1 year, 12
* months, or 52 weeks).
*/
public Builder setIntervalCount(Long intervalCount) {
this.intervalCount = intervalCount;
return this;
}
}
public enum Interval implements ApiRequestParams.EnumParam {
@SerializedName("day")
DAY("day"), @SerializedName("month")
MONTH("month"), @SerializedName("week")
WEEK("week"), @SerializedName("year")
YEAR("year");
private final String value;
Interval(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* Specifies invoicing frequency. Either {@code day}, {@code week}, {@code month} or {@code
* year}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Interval getInterval() {
return this.interval;
}
/**
* The number of intervals between invoices. For example, {@code interval=month} and {@code
* interval_count=3} bills every 3 months. Maximum of one year interval allowed (1 year, 12
* months, or 52 weeks).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getIntervalCount() {
return this.intervalCount;
}
}
public static class TransferData {
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents
* the percentage of the subscription invoice subtotal that will be transferred to the
* destination account. By default, the entire amount is transferred to the destination.
*/
@SerializedName("amount_percent")
BigDecimal amountPercent;
/**
* ID of an existing, connected Stripe account.
*/
@SerializedName("destination")
Object destination;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
private TransferData(BigDecimal amountPercent, Object destination, Map extraParams) {
this.amountPercent = amountPercent;
this.destination = destination;
this.extraParams = extraParams;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private BigDecimal amountPercent;
private Object destination;
private Map extraParams;
/** Finalize and obtain parameter instance from this builder. */
public TransferData build() {
return new TransferData(this.amountPercent, this.destination, this.extraParams);
}
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents
* the percentage of the subscription invoice subtotal that will be transferred to the
* destination account. By default, the entire amount is transferred to the destination.
*/
public Builder setAmountPercent(BigDecimal amountPercent) {
this.amountPercent = amountPercent;
return this;
}
/** ID of an existing, connected Stripe account. */
public Builder setDestination(String destination) {
this.destination = destination;
return this;
}
/** ID of an existing, connected Stripe account. */
public Builder setDestination(EmptyParam destination) {
this.destination = destination;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionUpdateParams.TransferData#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
}
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents
* the percentage of the subscription invoice subtotal that will be transferred to the
* destination account. By default, the entire amount is transferred to the destination.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BigDecimal getAmountPercent() {
return this.amountPercent;
}
/**
* ID of an existing, connected Stripe account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getDestination() {
return this.destination;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionUpdateParams.TransferData#extraParams} for the field documentation.
*/
public enum BillingCycleAnchor implements ApiRequestParams.EnumParam {
@SerializedName("now")
NOW("now"), @SerializedName("unchanged")
UNCHANGED("unchanged");
private final String value;
BillingCycleAnchor(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum CollectionMethod implements ApiRequestParams.EnumParam {
@SerializedName("charge_automatically")
CHARGE_AUTOMATICALLY("charge_automatically"), @SerializedName("send_invoice")
SEND_INVOICE("send_invoice");
private final String value;
CollectionMethod(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum PaymentBehavior implements ApiRequestParams.EnumParam {
@SerializedName("allow_incomplete")
ALLOW_INCOMPLETE("allow_incomplete"), @SerializedName("default_incomplete")
DEFAULT_INCOMPLETE("default_incomplete"), @SerializedName("error_if_incomplete")
ERROR_IF_INCOMPLETE("error_if_incomplete"), @SerializedName("pending_if_incomplete")
PENDING_IF_INCOMPLETE("pending_if_incomplete");
private final String value;
PaymentBehavior(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum ProrationBehavior implements ApiRequestParams.EnumParam {
@SerializedName("always_invoice")
ALWAYS_INVOICE("always_invoice"), @SerializedName("create_prorations")
CREATE_PRORATIONS("create_prorations"), @SerializedName("none")
NONE("none");
private final String value;
ProrationBehavior(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum TrialEnd implements ApiRequestParams.EnumParam {
@SerializedName("now")
NOW("now");
private final String value;
TrialEnd(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
/**
* A list of prices and quantities that will generate invoice items appended to the first invoice
* for this subscription. You may pass up to 20 items.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getAddInvoiceItems() {
return this.addInvoiceItems;
}
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents the
* percentage of the subscription invoice subtotal that will be transferred to the application
* owner's Stripe account. The request must be made by a platform account on a connected account
* in order to set an application fee percentage. For more information, see the application fees
* documentation.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BigDecimal getApplicationFeePercent() {
return this.applicationFeePercent;
}
/**
* Automatic tax settings for this subscription.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public AutomaticTax getAutomaticTax() {
return this.automaticTax;
}
/**
* Either {@code now} or {@code unchanged}. Setting the value to {@code now} resets the
* subscription's billing cycle anchor to the current time. For more information, see the billing
* cycle documentation.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BillingCycleAnchor getBillingCycleAnchor() {
return this.billingCycleAnchor;
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. Pass an empty string to remove previously-defined thresholds.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getBillingThresholds() {
return this.billingThresholds;
}
/**
* A timestamp at which the subscription should cancel. If set to a date before the current period
* ends, this will cause a proration if prorations have been enabled using {@code
* proration_behavior}. If set during a future period, this will always cause a proration for that
* period.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getCancelAt() {
return this.cancelAt;
}
/**
* Boolean indicating whether this subscription should cancel at the end of the current period.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getCancelAtPeriodEnd() {
return this.cancelAtPeriodEnd;
}
/**
* Either {@code charge_automatically}, or {@code send_invoice}. When charging automatically,
* Stripe will attempt to pay this subscription at the end of the cycle using the default source
* attached to the customer. When sending an invoice, Stripe will email your customer an invoice
* with payment instructions. Defaults to {@code charge_automatically}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public CollectionMethod getCollectionMethod() {
return this.collectionMethod;
}
/**
* The ID of the coupon to apply to this subscription. A coupon applied to a subscription will
* only affect invoices created for that particular subscription.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getCoupon() {
return this.coupon;
}
/**
* Number of days a customer has to pay invoices generated by this subscription. Valid only for
* subscriptions where {@code collection_method} is set to {@code send_invoice}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDaysUntilDue() {
return this.daysUntilDue;
}
/**
* ID of the default payment method for the subscription. It must belong to the customer
* associated with the subscription. This takes precedence over {@code default_source}. If neither
* are set, invoices will use the customer's invoice_settings.default_payment_method
* or default_source.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getDefaultPaymentMethod() {
return this.defaultPaymentMethod;
}
/**
* ID of the default payment source for the subscription. It must belong to the customer
* associated with the subscription and be in a chargeable state. If {@code
* default_payment_method} is also set, {@code default_payment_method} will take precedence. If
* neither are set, invoices will use the customer's invoice_settings.default_payment_method
* or default_source.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getDefaultSource() {
return this.defaultSource;
}
/**
* The tax rates that will apply to any subscription item that does not have {@code tax_rates}
* set. Invoices created will have their {@code default_tax_rates} populated from the
* subscription. Pass an empty string to remove previously-defined tax rates.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getDefaultTaxRates() {
return this.defaultTaxRates;
}
/**
* Specifies which fields in the response should be expanded.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getExpand() {
return this.expand;
}
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getExtraParams() {
return this.extraParams;
}
/**
* A list of up to 20 subscription items, each with an attached price.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List- getItems() {
return this.items;
}
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format. Individual keys can be unset by posting an empty value to them. All keys can
* be unset by posting an empty value to {@code metadata}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getMetadata() {
return this.metadata;
}
/**
* Indicates if a customer is on or off-session while an invoice payment is attempted.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getOffSession() {
return this.offSession;
}
/**
* If specified, payment collection for this subscription will be paused.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getPauseCollection() {
return this.pauseCollection;
}
/**
* Use {@code allow_incomplete} to transition the subscription to {@code status=past_due} if a
* payment is required but cannot be paid. This allows you to manage scenarios where additional
* user actions are needed to pay a subscription's invoice. For example, SCA regulation may
* require 3DS authentication to complete payment. See the SCA Migration
* Guide for Billing to learn more. This is the default behavior.
*
*
Use {@code default_incomplete} to transition the subscription to {@code status=past_due}
* when payment is required and await explicit confirmation of the invoice's payment intent. This
* allows simpler management of scenarios where additional user actions are needed to pay a
* subscription’s invoice. Such as failed payments, SCA
* regulation, or collecting a mandate for a bank debit payment method.
*
*
Use {@code pending_if_incomplete} to update the subscription using pending updates. When
* you use {@code pending_if_incomplete} you can only pass the parameters supported
* by pending updates.
*
*
Use {@code error_if_incomplete} if you want Stripe to return an HTTP 402 status code if a
* subscription's invoice cannot be paid. For example, if a payment method requires 3DS
* authentication due to SCA regulation and further user action is needed, this parameter does not
* update the subscription and returns an error instead. This was the default behavior for API
* versions prior to 2019-03-14. See the changelog to learn more.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PaymentBehavior getPaymentBehavior() {
return this.paymentBehavior;
}
/**
* Payment settings to pass to invoices created by the subscription.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PaymentSettings getPaymentSettings() {
return this.paymentSettings;
}
/**
* Specifies an interval for how often to bill for any pending invoice items. It is analogous to
* calling Create an invoice for the
* given subscription at the specified interval.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getPendingInvoiceItemInterval() {
return this.pendingInvoiceItemInterval;
}
/**
* The promotion code to apply to this subscription. A promotion code applied to a subscription
* will only affect invoices created for that particular subscription.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getPromotionCode() {
return this.promotionCode;
}
/**
* Determines how to handle prorations when the
* billing cycle changes (e.g., when switching plans, resetting {@code billing_cycle_anchor=now},
* or starting a trial), or if an item's {@code quantity} changes. Valid values are {@code
* create_prorations}, {@code none}, or {@code always_invoice}.
*
*
Passing {@code create_prorations} will cause proration invoice items to be created when
* applicable. These proration items will only be invoiced immediately under certain
* conditions. In order to always invoice immediately for prorations, pass {@code
* always_invoice}.
*
*
Prorations can be disabled by passing {@code none}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ProrationBehavior getProrationBehavior() {
return this.prorationBehavior;
}
/**
* If set, the proration will be calculated as though the subscription was updated at the given
* time. This can be used to apply exactly the same proration that was previewed with upcoming invoice endpoint. It
* can also be used to implement custom proration logic, such as prorating by day instead of by
* second, by providing the time that you wish to use for proration calculations.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getProrationDate() {
return this.prorationDate;
}
/**
* If specified, the funds from the subscription's invoices will be transferred to the destination
* and the ID of the resulting transfers will be found on the resulting charges. This will be
* unset if you POST an empty value.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getTransferData() {
return this.transferData;
}
/**
* Unix timestamp representing the end of the trial period the customer will get before being
* charged for the first time. This will always overwrite any trials that might apply via a
* subscribed plan. If set, trial_end will override the default trial period of the plan the
* customer is being subscribed to. The special value {@code now} can be provided to end the
* customer's trial immediately. Can be at most two years from {@code billing_cycle_anchor}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Object getTrialEnd() {
return this.trialEnd;
}
/**
* Indicates if a plan's {@code trial_period_days} should be applied to the subscription. Setting
* {@code trial_end} per subscription is preferred, and this defaults to {@code false}. Setting
* this flag to {@code true} together with {@code trial_end} is not allowed.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getTrialFromPlan() {
return this.trialFromPlan;
}
}