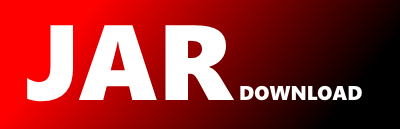
com.stripe.model.identity.VerificationSession Maven / Gradle / Ivy
// Generated by delombok at Thu Nov 04 09:14:18 EDT 2021
// File generated from our OpenAPI spec
package com.stripe.model.identity;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.model.Address;
import com.stripe.model.ExpandableField;
import com.stripe.model.HasId;
import com.stripe.model.MetadataStore;
import com.stripe.model.StripeObject;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.identity.VerificationSessionCancelParams;
import com.stripe.param.identity.VerificationSessionCreateParams;
import com.stripe.param.identity.VerificationSessionListParams;
import com.stripe.param.identity.VerificationSessionRedactParams;
import com.stripe.param.identity.VerificationSessionRetrieveParams;
import com.stripe.param.identity.VerificationSessionUpdateParams;
import java.util.List;
import java.util.Map;
public class VerificationSession extends ApiResource implements HasId, MetadataStore {
/**
* The short-lived client secret used by Stripe.js to show a verification modal inside your app.
* This client secret expires after 24 hours and can only be used once. Don’t store it, log it,
* embed it in a URL, or expose it to anyone other than the user. Make sure that you have TLS
* enabled on any page that includes the client secret. Refer to our docs on passing the client
* secret to the frontend to learn more.
*/
@SerializedName("client_secret")
String clientSecret;
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* If present, this property tells you the last error encountered when processing the
* verification.
*/
@SerializedName("last_error")
LastError lastError;
/**
* ID of the most recent VerificationReport. Learn more about
* accessing detailed verification results.
*/
@SerializedName("last_verification_report")
ExpandableField lastVerificationReport;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code identity.verification_session}.
*/
@SerializedName("object")
String object;
@SerializedName("options")
Options options;
/**
* Redaction status of this VerificationSession. If the VerificationSession is not redacted, this
* field will be null.
*/
@SerializedName("redaction")
Redaction redaction;
/**
* Status of this VerificationSession. Learn more about the lifecycle of
* sessions.
*
*
One of {@code canceled}, {@code processing}, {@code requires_input}, or {@code verified}.
*/
@SerializedName("status")
String status;
/**
* The type of verification
* check to be performed.
*
*
One of {@code document}, or {@code id_number}.
*/
@SerializedName("type")
String type;
/**
* The short-lived URL that you use to redirect a user to Stripe to submit their identity
* information. This URL expires after 48 hours and can only be used once. Don’t store it, log it,
* send it in emails or expose it to anyone other than the user. Refer to our docs on verifying
* identity documents to learn how to redirect users to Stripe.
*/
@SerializedName("url")
String url;
/**
* The user’s verified data.
*/
@SerializedName("verified_outputs")
VerifiedOutputs verifiedOutputs;
/**
* Get ID of expandable {@code lastVerificationReport} object.
*/
public String getLastVerificationReport() {
return (this.lastVerificationReport != null) ? this.lastVerificationReport.getId() : null;
}
public void setLastVerificationReport(String id) {
this.lastVerificationReport = ApiResource.setExpandableFieldId(id, this.lastVerificationReport);
}
/**
* Get expanded {@code lastVerificationReport}.
*/
public VerificationReport getLastVerificationReportObject() {
return (this.lastVerificationReport != null) ? this.lastVerificationReport.getExpanded() : null;
}
public void setLastVerificationReportObject(VerificationReport expandableObject) {
this.lastVerificationReport = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Creates a VerificationSession object.
*
* After the VerificationSession is created, display a verification modal using the session
* client_secret
or send your users to the session’s url
.
*
*
If your API key is in test mode, verification checks won’t actually process, though
* everything else will occur as if in live mode.
*
*
Related guide: Verify
* your users’ identity documents.
*/
public static VerificationSession create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Creates a VerificationSession object.
*
* After the VerificationSession is created, display a verification modal using the session
* client_secret
or send your users to the session’s url
.
*
*
If your API key is in test mode, verification checks won’t actually process, though
* everything else will occur as if in live mode.
*
*
Related guide: Verify
* your users’ identity documents.
*/
public static VerificationSession create(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/identity/verification_sessions");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, VerificationSession.class, options);
}
/**
* Creates a VerificationSession object.
*
* After the VerificationSession is created, display a verification modal using the session
* client_secret
or send your users to the session’s url
.
*
*
If your API key is in test mode, verification checks won’t actually process, though
* everything else will occur as if in live mode.
*
*
Related guide: Verify
* your users’ identity documents.
*/
public static VerificationSession create(VerificationSessionCreateParams params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Creates a VerificationSession object.
*
*
After the VerificationSession is created, display a verification modal using the session
* client_secret
or send your users to the session’s url
.
*
*
If your API key is in test mode, verification checks won’t actually process, though
* everything else will occur as if in live mode.
*
*
Related guide: Verify
* your users’ identity documents.
*/
public static VerificationSession create(VerificationSessionCreateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/identity/verification_sessions");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, VerificationSession.class, options);
}
/**
* Retrieves the details of a VerificationSession that was previously created.
*
*
When the session status is requires_input
, you can use this method to retrieve
* a valid client_secret
or url
to allow re-submission.
*/
public static VerificationSession retrieve(String session) throws StripeException {
return retrieve(session, (Map) null, (RequestOptions) null);
}
/**
* Retrieves the details of a VerificationSession that was previously created.
*
* When the session status is requires_input
, you can use this method to retrieve
* a valid client_secret
or url
to allow re-submission.
*/
public static VerificationSession retrieve(String session, RequestOptions options) throws StripeException {
return retrieve(session, (Map) null, options);
}
/**
* Retrieves the details of a VerificationSession that was previously created.
*
* When the session status is requires_input
, you can use this method to retrieve
* a valid client_secret
or url
to allow re-submission.
*/
public static VerificationSession retrieve(String session, Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/identity/verification_sessions/%s", ApiResource.urlEncodeId(session)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, VerificationSession.class, options);
}
/**
* Retrieves the details of a VerificationSession that was previously created.
*
* When the session status is requires_input
, you can use this method to retrieve
* a valid client_secret
or url
to allow re-submission.
*/
public static VerificationSession retrieve(String session, VerificationSessionRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/identity/verification_sessions/%s", ApiResource.urlEncodeId(session)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, VerificationSession.class, options);
}
/**
* Returns a list of VerificationSessions.
*/
public static VerificationSessionCollection list(Map params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of VerificationSessions.
*/
public static VerificationSessionCollection list(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/identity/verification_sessions");
return ApiResource.requestCollection(url, params, VerificationSessionCollection.class, options);
}
/**
* Returns a list of VerificationSessions.
*/
public static VerificationSessionCollection list(VerificationSessionListParams params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of VerificationSessions.
*/
public static VerificationSessionCollection list(VerificationSessionListParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/identity/verification_sessions");
return ApiResource.requestCollection(url, params, VerificationSessionCollection.class, options);
}
/**
* A VerificationSession object can be canceled when it is in requires_input
status.
*
* Once canceled, future submission attempts are disabled. This cannot be undone. Learn more.
*/
public VerificationSession cancel() throws StripeException {
return cancel((Map) null, (RequestOptions) null);
}
/**
* A VerificationSession object can be canceled when it is in requires_input
status.
*
* Once canceled, future submission attempts are disabled. This cannot be undone. Learn more.
*/
public VerificationSession cancel(RequestOptions options) throws StripeException {
return cancel((Map) null, options);
}
/**
* A VerificationSession object can be canceled when it is in requires_input
status.
*
* Once canceled, future submission attempts are disabled. This cannot be undone. Learn more.
*/
public VerificationSession cancel(Map params) throws StripeException {
return cancel(params, (RequestOptions) null);
}
/**
* A VerificationSession object can be canceled when it is in requires_input
status.
*
* Once canceled, future submission attempts are disabled. This cannot be undone. Learn more.
*/
public VerificationSession cancel(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/identity/verification_sessions/%s/cancel", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, VerificationSession.class, options);
}
/**
* A VerificationSession object can be canceled when it is in requires_input
status.
*
* Once canceled, future submission attempts are disabled. This cannot be undone. Learn more.
*/
public VerificationSession cancel(VerificationSessionCancelParams params) throws StripeException {
return cancel(params, (RequestOptions) null);
}
/**
* A VerificationSession object can be canceled when it is in requires_input
status.
*
*
Once canceled, future submission attempts are disabled. This cannot be undone. Learn more.
*/
public VerificationSession cancel(VerificationSessionCancelParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/identity/verification_sessions/%s/cancel", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, VerificationSession.class, options);
}
/**
* Redact a VerificationSession to remove all collected information from Stripe. This will redact
* the VerificationSession and all objects related to it, including VerificationReports, Events,
* request logs, etc.
*
*
A VerificationSession object can be redacted when it is in requires_input
or
* verified
status.
* Redacting a VerificationSession in requires_action
state will automatically cancel
* it.
*
*
The redaction process may take up to four days. When the redaction process is in progress,
* the VerificationSession’s redaction.status
field will be set to processing
*
; when the process is finished, it will change to redacted
and an
* identity.verification_session.redacted
event will be emitted.
*
*
Redaction is irreversible. Redacted objects are still accessible in the Stripe API, but all
* the fields that contain personal data will be replaced by the string [redacted]
or
* a similar placeholder. The metadata
field will also be erased. Redacted objects
* cannot be updated or used for any purpose.
*
*
Learn more.
*/
public VerificationSession redact() throws StripeException {
return redact((Map) null, (RequestOptions) null);
}
/**
* Redact a VerificationSession to remove all collected information from Stripe. This will redact
* the VerificationSession and all objects related to it, including VerificationReports, Events,
* request logs, etc.
*
* A VerificationSession object can be redacted when it is in requires_input
or
* verified
status.
* Redacting a VerificationSession in requires_action
state will automatically cancel
* it.
*
*
The redaction process may take up to four days. When the redaction process is in progress,
* the VerificationSession’s redaction.status
field will be set to processing
*
; when the process is finished, it will change to redacted
and an
* identity.verification_session.redacted
event will be emitted.
*
*
Redaction is irreversible. Redacted objects are still accessible in the Stripe API, but all
* the fields that contain personal data will be replaced by the string [redacted]
or
* a similar placeholder. The metadata
field will also be erased. Redacted objects
* cannot be updated or used for any purpose.
*
*
Learn more.
*/
public VerificationSession redact(RequestOptions options) throws StripeException {
return redact((Map) null, options);
}
/**
* Redact a VerificationSession to remove all collected information from Stripe. This will redact
* the VerificationSession and all objects related to it, including VerificationReports, Events,
* request logs, etc.
*
* A VerificationSession object can be redacted when it is in requires_input
or
* verified
status.
* Redacting a VerificationSession in requires_action
state will automatically cancel
* it.
*
*
The redaction process may take up to four days. When the redaction process is in progress,
* the VerificationSession’s redaction.status
field will be set to processing
*
; when the process is finished, it will change to redacted
and an
* identity.verification_session.redacted
event will be emitted.
*
*
Redaction is irreversible. Redacted objects are still accessible in the Stripe API, but all
* the fields that contain personal data will be replaced by the string [redacted]
or
* a similar placeholder. The metadata
field will also be erased. Redacted objects
* cannot be updated or used for any purpose.
*
*
Learn more.
*/
public VerificationSession redact(Map params) throws StripeException {
return redact(params, (RequestOptions) null);
}
/**
* Redact a VerificationSession to remove all collected information from Stripe. This will redact
* the VerificationSession and all objects related to it, including VerificationReports, Events,
* request logs, etc.
*
* A VerificationSession object can be redacted when it is in requires_input
or
* verified
status.
* Redacting a VerificationSession in requires_action
state will automatically cancel
* it.
*
*
The redaction process may take up to four days. When the redaction process is in progress,
* the VerificationSession’s redaction.status
field will be set to processing
*
; when the process is finished, it will change to redacted
and an
* identity.verification_session.redacted
event will be emitted.
*
*
Redaction is irreversible. Redacted objects are still accessible in the Stripe API, but all
* the fields that contain personal data will be replaced by the string [redacted]
or
* a similar placeholder. The metadata
field will also be erased. Redacted objects
* cannot be updated or used for any purpose.
*
*
Learn more.
*/
public VerificationSession redact(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/identity/verification_sessions/%s/redact", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, VerificationSession.class, options);
}
/**
* Redact a VerificationSession to remove all collected information from Stripe. This will redact
* the VerificationSession and all objects related to it, including VerificationReports, Events,
* request logs, etc.
*
* A VerificationSession object can be redacted when it is in requires_input
or
* verified
status.
* Redacting a VerificationSession in requires_action
state will automatically cancel
* it.
*
*
The redaction process may take up to four days. When the redaction process is in progress,
* the VerificationSession’s redaction.status
field will be set to processing
*
; when the process is finished, it will change to redacted
and an
* identity.verification_session.redacted
event will be emitted.
*
*
Redaction is irreversible. Redacted objects are still accessible in the Stripe API, but all
* the fields that contain personal data will be replaced by the string [redacted]
or
* a similar placeholder. The metadata
field will also be erased. Redacted objects
* cannot be updated or used for any purpose.
*
*
Learn more.
*/
public VerificationSession redact(VerificationSessionRedactParams params) throws StripeException {
return redact(params, (RequestOptions) null);
}
/**
* Redact a VerificationSession to remove all collected information from Stripe. This will redact
* the VerificationSession and all objects related to it, including VerificationReports, Events,
* request logs, etc.
*
*
A VerificationSession object can be redacted when it is in requires_input
or
* verified
status.
* Redacting a VerificationSession in requires_action
state will automatically cancel
* it.
*
*
The redaction process may take up to four days. When the redaction process is in progress,
* the VerificationSession’s redaction.status
field will be set to processing
*
; when the process is finished, it will change to redacted
and an
* identity.verification_session.redacted
event will be emitted.
*
*
Redaction is irreversible. Redacted objects are still accessible in the Stripe API, but all
* the fields that contain personal data will be replaced by the string [redacted]
or
* a similar placeholder. The metadata
field will also be erased. Redacted objects
* cannot be updated or used for any purpose.
*
*
Learn more.
*/
public VerificationSession redact(VerificationSessionRedactParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/identity/verification_sessions/%s/redact", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, VerificationSession.class, options);
}
/**
* Updates a VerificationSession object.
*
*
When the session status is requires_input
, you can use this method to update
* the verification check and options.
*/
@Override
public VerificationSession update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates a VerificationSession object.
*
* When the session status is requires_input
, you can use this method to update
* the verification check and options.
*/
@Override
public VerificationSession update(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/identity/verification_sessions/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, VerificationSession.class, options);
}
/**
* Updates a VerificationSession object.
*
* When the session status is requires_input
, you can use this method to update
* the verification check and options.
*/
public VerificationSession update(VerificationSessionUpdateParams params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates a VerificationSession object.
*
*
When the session status is requires_input
, you can use this method to update
* the verification check and options.
*/
public VerificationSession update(VerificationSessionUpdateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/identity/verification_sessions/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, VerificationSession.class, options);
}
public static class LastError extends StripeObject {
/**
* A short machine-readable string giving the reason for the verification or user-session
* failure.
*
*
One of {@code abandoned}, {@code consent_declined}, {@code country_not_supported}, {@code
* device_not_supported}, {@code document_expired}, {@code document_type_not_supported}, {@code
* document_unverified_other}, {@code id_number_insufficient_document_data}, {@code
* id_number_mismatch}, {@code id_number_unverified_other}, {@code
* selfie_document_missing_photo}, {@code selfie_face_mismatch}, {@code selfie_manipulated},
* {@code selfie_unverified_other}, or {@code under_supported_age}.
*/
@SerializedName("code")
String code;
/**
* A message that explains the reason for verification or user-session failure.
*/
@SerializedName("reason")
String reason;
/**
* A short machine-readable string giving the reason for the verification or user-session
* failure.
*
*
One of {@code abandoned}, {@code consent_declined}, {@code country_not_supported}, {@code
* device_not_supported}, {@code document_expired}, {@code document_type_not_supported}, {@code
* document_unverified_other}, {@code id_number_insufficient_document_data}, {@code
* id_number_mismatch}, {@code id_number_unverified_other}, {@code
* selfie_document_missing_photo}, {@code selfie_face_mismatch}, {@code selfie_manipulated},
* {@code selfie_unverified_other}, or {@code under_supported_age}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCode() {
return this.code;
}
/**
* A message that explains the reason for verification or user-session failure.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReason() {
return this.reason;
}
/**
* A short machine-readable string giving the reason for the verification or user-session
* failure.
*
*
One of {@code abandoned}, {@code consent_declined}, {@code country_not_supported}, {@code
* device_not_supported}, {@code document_expired}, {@code document_type_not_supported}, {@code
* document_unverified_other}, {@code id_number_insufficient_document_data}, {@code
* id_number_mismatch}, {@code id_number_unverified_other}, {@code
* selfie_document_missing_photo}, {@code selfie_face_mismatch}, {@code selfie_manipulated},
* {@code selfie_unverified_other}, or {@code under_supported_age}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCode(final String code) {
this.code = code;
}
/**
* A message that explains the reason for verification or user-session failure.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReason(final String reason) {
this.reason = reason;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof VerificationSession.LastError)) return false;
final VerificationSession.LastError other = (VerificationSession.LastError) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$code = this.getCode();
final java.lang.Object other$code = other.getCode();
if (this$code == null ? other$code != null : !this$code.equals(other$code)) return false;
final java.lang.Object this$reason = this.getReason();
final java.lang.Object other$reason = other.getReason();
if (this$reason == null ? other$reason != null : !this$reason.equals(other$reason)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof VerificationSession.LastError;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $code = this.getCode();
result = result * PRIME + ($code == null ? 43 : $code.hashCode());
final java.lang.Object $reason = this.getReason();
result = result * PRIME + ($reason == null ? 43 : $reason.hashCode());
return result;
}
}
public static class Options extends StripeObject {
@SerializedName("document")
Document document;
@SerializedName("id_number")
IdNumber idNumber;
public static class Document extends StripeObject {
/**
* Array of strings of allowed identity document types. If the provided identity document
* isn’t one of the allowed types, the verification check will fail with a
* document_type_not_allowed error code.
*/
@SerializedName("allowed_types")
List allowedTypes;
/**
* Collect an ID number and perform an ID number
* check with the document’s extracted name and date of birth.
*/
@SerializedName("require_id_number")
Boolean requireIdNumber;
/**
* Disable image uploads, identity document images have to be captured using the device’s
* camera.
*/
@SerializedName("require_live_capture")
Boolean requireLiveCapture;
/**
* Capture a face image and perform a selfie check
* comparing a photo ID and a picture of your user’s face. Learn more.
*/
@SerializedName("require_matching_selfie")
Boolean requireMatchingSelfie;
/**
* Array of strings of allowed identity document types. If the provided identity document
* isn’t one of the allowed types, the verification check will fail with a
* document_type_not_allowed error code.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getAllowedTypes() {
return this.allowedTypes;
}
/**
* Collect an ID number and perform an ID number
* check with the document’s extracted name and date of birth.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getRequireIdNumber() {
return this.requireIdNumber;
}
/**
* Disable image uploads, identity document images have to be captured using the device’s
* camera.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getRequireLiveCapture() {
return this.requireLiveCapture;
}
/**
* Capture a face image and perform a selfie check
* comparing a photo ID and a picture of your user’s face. Learn more.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getRequireMatchingSelfie() {
return this.requireMatchingSelfie;
}
/**
* Array of strings of allowed identity document types. If the provided identity document
* isn’t one of the allowed types, the verification check will fail with a
* document_type_not_allowed error code.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAllowedTypes(final List allowedTypes) {
this.allowedTypes = allowedTypes;
}
/**
* Collect an ID number and perform an ID number
* check with the document’s extracted name and date of birth.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRequireIdNumber(final Boolean requireIdNumber) {
this.requireIdNumber = requireIdNumber;
}
/**
* Disable image uploads, identity document images have to be captured using the device’s
* camera.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRequireLiveCapture(final Boolean requireLiveCapture) {
this.requireLiveCapture = requireLiveCapture;
}
/**
* Capture a face image and perform a selfie check
* comparing a photo ID and a picture of your user’s face. Learn more.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRequireMatchingSelfie(final Boolean requireMatchingSelfie) {
this.requireMatchingSelfie = requireMatchingSelfie;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof VerificationSession.Options.Document)) return false;
final VerificationSession.Options.Document other = (VerificationSession.Options.Document) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$requireIdNumber = this.getRequireIdNumber();
final java.lang.Object other$requireIdNumber = other.getRequireIdNumber();
if (this$requireIdNumber == null ? other$requireIdNumber != null : !this$requireIdNumber.equals(other$requireIdNumber)) return false;
final java.lang.Object this$requireLiveCapture = this.getRequireLiveCapture();
final java.lang.Object other$requireLiveCapture = other.getRequireLiveCapture();
if (this$requireLiveCapture == null ? other$requireLiveCapture != null : !this$requireLiveCapture.equals(other$requireLiveCapture)) return false;
final java.lang.Object this$requireMatchingSelfie = this.getRequireMatchingSelfie();
final java.lang.Object other$requireMatchingSelfie = other.getRequireMatchingSelfie();
if (this$requireMatchingSelfie == null ? other$requireMatchingSelfie != null : !this$requireMatchingSelfie.equals(other$requireMatchingSelfie)) return false;
final java.lang.Object this$allowedTypes = this.getAllowedTypes();
final java.lang.Object other$allowedTypes = other.getAllowedTypes();
if (this$allowedTypes == null ? other$allowedTypes != null : !this$allowedTypes.equals(other$allowedTypes)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof VerificationSession.Options.Document;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $requireIdNumber = this.getRequireIdNumber();
result = result * PRIME + ($requireIdNumber == null ? 43 : $requireIdNumber.hashCode());
final java.lang.Object $requireLiveCapture = this.getRequireLiveCapture();
result = result * PRIME + ($requireLiveCapture == null ? 43 : $requireLiveCapture.hashCode());
final java.lang.Object $requireMatchingSelfie = this.getRequireMatchingSelfie();
result = result * PRIME + ($requireMatchingSelfie == null ? 43 : $requireMatchingSelfie.hashCode());
final java.lang.Object $allowedTypes = this.getAllowedTypes();
result = result * PRIME + ($allowedTypes == null ? 43 : $allowedTypes.hashCode());
return result;
}
}
public static class IdNumber extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof VerificationSession.Options.IdNumber)) return false;
final VerificationSession.Options.IdNumber other = (VerificationSession.Options.IdNumber) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof VerificationSession.Options.IdNumber;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Document getDocument() {
return this.document;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public IdNumber getIdNumber() {
return this.idNumber;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDocument(final Document document) {
this.document = document;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIdNumber(final IdNumber idNumber) {
this.idNumber = idNumber;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof VerificationSession.Options)) return false;
final VerificationSession.Options other = (VerificationSession.Options) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$document = this.getDocument();
final java.lang.Object other$document = other.getDocument();
if (this$document == null ? other$document != null : !this$document.equals(other$document)) return false;
final java.lang.Object this$idNumber = this.getIdNumber();
final java.lang.Object other$idNumber = other.getIdNumber();
if (this$idNumber == null ? other$idNumber != null : !this$idNumber.equals(other$idNumber)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof VerificationSession.Options;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $document = this.getDocument();
result = result * PRIME + ($document == null ? 43 : $document.hashCode());
final java.lang.Object $idNumber = this.getIdNumber();
result = result * PRIME + ($idNumber == null ? 43 : $idNumber.hashCode());
return result;
}
}
public static class Redaction extends StripeObject {
/**
* Indicates whether this object and its related objects have been redacted or not.
*
* One of {@code processing}, or {@code redacted}.
*/
@SerializedName("status")
String status;
/**
* Indicates whether this object and its related objects have been redacted or not.
*
*
One of {@code processing}, or {@code redacted}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
/**
* Indicates whether this object and its related objects have been redacted or not.
*
*
One of {@code processing}, or {@code redacted}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof VerificationSession.Redaction)) return false;
final VerificationSession.Redaction other = (VerificationSession.Redaction) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof VerificationSession.Redaction;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
return result;
}
}
public static class VerifiedOutputs extends StripeObject {
/** The user's verified address. */
@SerializedName("address")
Address address;
/** The user’s verified date of birth. */
@SerializedName("dob")
DateOfBirth dob;
/** The user's verified first name. */
@SerializedName("first_name")
String firstName;
/** The user's verified id number. */
@SerializedName("id_number")
String idNumber;
/**
* The user's verified id number type.
*
*
One of {@code br_cpf}, {@code sg_nric}, or {@code us_ssn}.
*/
@SerializedName("id_number_type")
String idNumberType;
/** The user's verified last name. */
@SerializedName("last_name")
String lastName;
public static class DateOfBirth extends StripeObject {
/** Numerical day between 1 and 31. */
@SerializedName("day")
Long day;
/** Numerical month between 1 and 12. */
@SerializedName("month")
Long month;
/** The four-digit year. */
@SerializedName("year")
Long year;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDay() {
return this.day;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getMonth() {
return this.month;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getYear() {
return this.year;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDay(final Long day) {
this.day = day;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMonth(final Long month) {
this.month = month;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setYear(final Long year) {
this.year = year;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof VerificationSession.VerifiedOutputs.DateOfBirth)) return false;
final VerificationSession.VerifiedOutputs.DateOfBirth other = (VerificationSession.VerifiedOutputs.DateOfBirth) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$day = this.getDay();
final java.lang.Object other$day = other.getDay();
if (this$day == null ? other$day != null : !this$day.equals(other$day)) return false;
final java.lang.Object this$month = this.getMonth();
final java.lang.Object other$month = other.getMonth();
if (this$month == null ? other$month != null : !this$month.equals(other$month)) return false;
final java.lang.Object this$year = this.getYear();
final java.lang.Object other$year = other.getYear();
if (this$year == null ? other$year != null : !this$year.equals(other$year)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof VerificationSession.VerifiedOutputs.DateOfBirth;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $day = this.getDay();
result = result * PRIME + ($day == null ? 43 : $day.hashCode());
final java.lang.Object $month = this.getMonth();
result = result * PRIME + ($month == null ? 43 : $month.hashCode());
final java.lang.Object $year = this.getYear();
result = result * PRIME + ($year == null ? 43 : $year.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Address getAddress() {
return this.address;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public DateOfBirth getDob() {
return this.dob;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFirstName() {
return this.firstName;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getIdNumber() {
return this.idNumber;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getIdNumberType() {
return this.idNumberType;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLastName() {
return this.lastName;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddress(final Address address) {
this.address = address;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDob(final DateOfBirth dob) {
this.dob = dob;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFirstName(final String firstName) {
this.firstName = firstName;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIdNumber(final String idNumber) {
this.idNumber = idNumber;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIdNumberType(final String idNumberType) {
this.idNumberType = idNumberType;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLastName(final String lastName) {
this.lastName = lastName;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof VerificationSession.VerifiedOutputs)) return false;
final VerificationSession.VerifiedOutputs other = (VerificationSession.VerifiedOutputs) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$address = this.getAddress();
final java.lang.Object other$address = other.getAddress();
if (this$address == null ? other$address != null : !this$address.equals(other$address)) return false;
final java.lang.Object this$dob = this.getDob();
final java.lang.Object other$dob = other.getDob();
if (this$dob == null ? other$dob != null : !this$dob.equals(other$dob)) return false;
final java.lang.Object this$firstName = this.getFirstName();
final java.lang.Object other$firstName = other.getFirstName();
if (this$firstName == null ? other$firstName != null : !this$firstName.equals(other$firstName)) return false;
final java.lang.Object this$idNumber = this.getIdNumber();
final java.lang.Object other$idNumber = other.getIdNumber();
if (this$idNumber == null ? other$idNumber != null : !this$idNumber.equals(other$idNumber)) return false;
final java.lang.Object this$idNumberType = this.getIdNumberType();
final java.lang.Object other$idNumberType = other.getIdNumberType();
if (this$idNumberType == null ? other$idNumberType != null : !this$idNumberType.equals(other$idNumberType)) return false;
final java.lang.Object this$lastName = this.getLastName();
final java.lang.Object other$lastName = other.getLastName();
if (this$lastName == null ? other$lastName != null : !this$lastName.equals(other$lastName)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof VerificationSession.VerifiedOutputs;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $address = this.getAddress();
result = result * PRIME + ($address == null ? 43 : $address.hashCode());
final java.lang.Object $dob = this.getDob();
result = result * PRIME + ($dob == null ? 43 : $dob.hashCode());
final java.lang.Object $firstName = this.getFirstName();
result = result * PRIME + ($firstName == null ? 43 : $firstName.hashCode());
final java.lang.Object $idNumber = this.getIdNumber();
result = result * PRIME + ($idNumber == null ? 43 : $idNumber.hashCode());
final java.lang.Object $idNumberType = this.getIdNumberType();
result = result * PRIME + ($idNumberType == null ? 43 : $idNumberType.hashCode());
final java.lang.Object $lastName = this.getLastName();
result = result * PRIME + ($lastName == null ? 43 : $lastName.hashCode());
return result;
}
}
/**
* The short-lived client secret used by Stripe.js to show a verification modal inside your app.
* This client secret expires after 24 hours and can only be used once. Don’t store it, log it,
* embed it in a URL, or expose it to anyone other than the user. Make sure that you have TLS
* enabled on any page that includes the client secret. Refer to our docs on passing the client
* secret to the frontend to learn more.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getClientSecret() {
return this.clientSecret;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
/**
* If present, this property tells you the last error encountered when processing the
* verification.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public LastError getLastError() {
return this.lastError;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code identity.verification_session}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Options getOptions() {
return this.options;
}
/**
* Redaction status of this VerificationSession. If the VerificationSession is not redacted, this
* field will be null.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Redaction getRedaction() {
return this.redaction;
}
/**
* Status of this VerificationSession. Learn more about the lifecycle of
* sessions.
*
*
One of {@code canceled}, {@code processing}, {@code requires_input}, or {@code verified}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
/**
* The type of verification
* check to be performed.
*
*
One of {@code document}, or {@code id_number}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
/**
* The short-lived URL that you use to redirect a user to Stripe to submit their identity
* information. This URL expires after 48 hours and can only be used once. Don’t store it, log it,
* send it in emails or expose it to anyone other than the user. Refer to our docs on verifying
* identity documents to learn how to redirect users to Stripe.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUrl() {
return this.url;
}
/**
* The user’s verified data.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public VerifiedOutputs getVerifiedOutputs() {
return this.verifiedOutputs;
}
/**
* The short-lived client secret used by Stripe.js to show a verification modal inside your app.
* This client secret expires after 24 hours and can only be used once. Don’t store it, log it,
* embed it in a URL, or expose it to anyone other than the user. Make sure that you have TLS
* enabled on any page that includes the client secret. Refer to our docs on passing the client
* secret to the frontend to learn more.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setClientSecret(final String clientSecret) {
this.clientSecret = clientSecret;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* If present, this property tells you the last error encountered when processing the
* verification.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLastError(final LastError lastError) {
this.lastError = lastError;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code identity.verification_session}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setOptions(final Options options) {
this.options = options;
}
/**
* Redaction status of this VerificationSession. If the VerificationSession is not redacted, this
* field will be null.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRedaction(final Redaction redaction) {
this.redaction = redaction;
}
/**
* Status of this VerificationSession. Learn more about the lifecycle of
* sessions.
*
*
One of {@code canceled}, {@code processing}, {@code requires_input}, or {@code verified}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
/**
* The type of verification
* check to be performed.
*
*
One of {@code document}, or {@code id_number}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
/**
* The short-lived URL that you use to redirect a user to Stripe to submit their identity
* information. This URL expires after 48 hours and can only be used once. Don’t store it, log it,
* send it in emails or expose it to anyone other than the user. Refer to our docs on verifying
* identity documents to learn how to redirect users to Stripe.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUrl(final String url) {
this.url = url;
}
/**
* The user’s verified data.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVerifiedOutputs(final VerifiedOutputs verifiedOutputs) {
this.verifiedOutputs = verifiedOutputs;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof VerificationSession)) return false;
final VerificationSession other = (VerificationSession) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$clientSecret = this.getClientSecret();
final java.lang.Object other$clientSecret = other.getClientSecret();
if (this$clientSecret == null ? other$clientSecret != null : !this$clientSecret.equals(other$clientSecret)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$lastError = this.getLastError();
final java.lang.Object other$lastError = other.getLastError();
if (this$lastError == null ? other$lastError != null : !this$lastError.equals(other$lastError)) return false;
final java.lang.Object this$lastVerificationReport = this.getLastVerificationReport();
final java.lang.Object other$lastVerificationReport = other.getLastVerificationReport();
if (this$lastVerificationReport == null ? other$lastVerificationReport != null : !this$lastVerificationReport.equals(other$lastVerificationReport)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$options = this.getOptions();
final java.lang.Object other$options = other.getOptions();
if (this$options == null ? other$options != null : !this$options.equals(other$options)) return false;
final java.lang.Object this$redaction = this.getRedaction();
final java.lang.Object other$redaction = other.getRedaction();
if (this$redaction == null ? other$redaction != null : !this$redaction.equals(other$redaction)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final java.lang.Object this$url = this.getUrl();
final java.lang.Object other$url = other.getUrl();
if (this$url == null ? other$url != null : !this$url.equals(other$url)) return false;
final java.lang.Object this$verifiedOutputs = this.getVerifiedOutputs();
final java.lang.Object other$verifiedOutputs = other.getVerifiedOutputs();
if (this$verifiedOutputs == null ? other$verifiedOutputs != null : !this$verifiedOutputs.equals(other$verifiedOutputs)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof VerificationSession;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $clientSecret = this.getClientSecret();
result = result * PRIME + ($clientSecret == null ? 43 : $clientSecret.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $lastError = this.getLastError();
result = result * PRIME + ($lastError == null ? 43 : $lastError.hashCode());
final java.lang.Object $lastVerificationReport = this.getLastVerificationReport();
result = result * PRIME + ($lastVerificationReport == null ? 43 : $lastVerificationReport.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $options = this.getOptions();
result = result * PRIME + ($options == null ? 43 : $options.hashCode());
final java.lang.Object $redaction = this.getRedaction();
result = result * PRIME + ($redaction == null ? 43 : $redaction.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final java.lang.Object $url = this.getUrl();
result = result * PRIME + ($url == null ? 43 : $url.hashCode());
final java.lang.Object $verifiedOutputs = this.getVerifiedOutputs();
result = result * PRIME + ($verifiedOutputs == null ? 43 : $verifiedOutputs.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
}