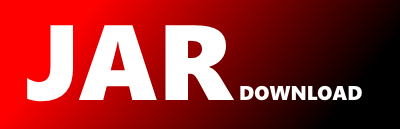
com.stripe.exception.StripeException Maven / Gradle / Ivy
// Generated by delombok at Fri Nov 19 19:06:04 EST 2021
package com.stripe.exception;
import com.stripe.model.StripeError;
public abstract class StripeException extends Exception {
private static final long serialVersionUID = 2L;
// transient so the exception can be serialized, as StripeObject does not
// implement Serializable
/**
* The error resource returned by Stripe's API that caused the exception.
*/
transient StripeError stripeError;
private String code;
private String requestId;
private Integer statusCode;
protected StripeException(String message, String requestId, String code, Integer statusCode) {
this(message, requestId, code, statusCode, null);
}
/**
* Constructs a new Stripe exception with the specified details.
*/
protected StripeException(String message, String requestId, String code, Integer statusCode, Throwable e) {
super(message, e);
this.code = code;
this.requestId = requestId;
this.statusCode = statusCode;
}
/**
* Returns a description of the exception, including the HTTP status code and request ID (if
* applicable).
*
* @return a string representation of the exception.
*/
@Override
public String getMessage() {
String additionalInfo = "";
if (code != null) {
additionalInfo += "; code: " + code;
}
if (requestId != null) {
additionalInfo += "; request-id: " + requestId;
}
return super.getMessage() + additionalInfo;
}
/**
* Returns a description of the user facing exception
*
* @return a string representation of the user facing exception.
*/
public String getUserMessage() {
return super.getMessage();
}
/**
* The error resource returned by Stripe's API that caused the exception.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public StripeError getStripeError() {
return this.stripeError;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCode() {
return this.code;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getRequestId() {
return this.requestId;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Integer getStatusCode() {
return this.statusCode;
}
/**
* The error resource returned by Stripe's API that caused the exception.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStripeError(final StripeError stripeError) {
this.stripeError = stripeError;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy