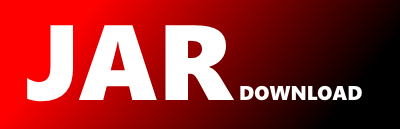
com.stripe.model.Discount Maven / Gradle / Ivy
// Generated by delombok at Fri Nov 19 19:06:04 EST 2021
// File generated from our OpenAPI spec
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
import com.stripe.net.ApiResource;
public class Discount extends StripeObject implements HasId {
/**
* The Checkout session that this coupon is applied to, if it is applied to a particular session
* in payment mode. Will not be present for subscription mode.
*/
@SerializedName("checkout_session")
String checkoutSession;
/**
* A coupon contains information about a percent-off or amount-off discount you might want to
* apply to a customer. Coupons may be applied to invoices or orders. Coupons do not work with
* conventional one-off charges.
*/
@SerializedName("coupon")
Coupon coupon;
/**
* The ID of the customer associated with this discount.
*/
@SerializedName("customer")
ExpandableField customer;
/**
* Always true for a deleted object.
*/
@SerializedName("deleted")
Boolean deleted;
/**
* If the coupon has a duration of {@code repeating}, the date that this discount will end. If the
* coupon has a duration of {@code once} or {@code forever}, this attribute will be null.
*/
@SerializedName("end")
Long end;
/**
* The ID of the discount object. Discounts cannot be fetched by ID. Use {@code
* expand[]=discounts} in API calls to expand discount IDs in an array.
*/
@SerializedName("id")
String id;
/**
* The invoice that the discount's coupon was applied to, if it was applied directly to a
* particular invoice.
*/
@SerializedName("invoice")
String invoice;
/**
* The invoice item {@code id} (or invoice line item {@code id} for invoice line items of
* type='subscription') that the discount's coupon was applied to, if it was applied directly to a
* particular invoice item or invoice line item.
*/
@SerializedName("invoice_item")
String invoiceItem;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code discount}.
*/
@SerializedName("object")
String object;
/**
* The promotion code applied to create this discount.
*/
@SerializedName("promotion_code")
ExpandableField promotionCode;
/**
* Date that the coupon was applied.
*/
@SerializedName("start")
Long start;
/**
* The subscription that this coupon is applied to, if it is applied to a particular subscription.
*/
@SerializedName("subscription")
String subscription;
/**
* Get ID of expandable {@code customer} object.
*/
public String getCustomer() {
return (this.customer != null) ? this.customer.getId() : null;
}
public void setCustomer(String id) {
this.customer = ApiResource.setExpandableFieldId(id, this.customer);
}
/**
* Get expanded {@code customer}.
*/
public Customer getCustomerObject() {
return (this.customer != null) ? this.customer.getExpanded() : null;
}
public void setCustomerObject(Customer expandableObject) {
this.customer = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code promotionCode} object.
*/
public String getPromotionCode() {
return (this.promotionCode != null) ? this.promotionCode.getId() : null;
}
public void setPromotionCode(String id) {
this.promotionCode = ApiResource.setExpandableFieldId(id, this.promotionCode);
}
/**
* Get expanded {@code promotionCode}.
*/
public PromotionCode getPromotionCodeObject() {
return (this.promotionCode != null) ? this.promotionCode.getExpanded() : null;
}
public void setPromotionCodeObject(PromotionCode expandableObject) {
this.promotionCode = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* The Checkout session that this coupon is applied to, if it is applied to a particular session
* in payment mode. Will not be present for subscription mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCheckoutSession() {
return this.checkoutSession;
}
/**
* A coupon contains information about a percent-off or amount-off discount you might want to
* apply to a customer. Coupons may be applied to invoices or orders. Coupons do not work with
* conventional one-off charges.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Coupon getCoupon() {
return this.coupon;
}
/**
* Always true for a deleted object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getDeleted() {
return this.deleted;
}
/**
* If the coupon has a duration of {@code repeating}, the date that this discount will end. If the
* coupon has a duration of {@code once} or {@code forever}, this attribute will be null.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getEnd() {
return this.end;
}
/**
* The invoice that the discount's coupon was applied to, if it was applied directly to a
* particular invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getInvoice() {
return this.invoice;
}
/**
* The invoice item {@code id} (or invoice line item {@code id} for invoice line items of
* type='subscription') that the discount's coupon was applied to, if it was applied directly to a
* particular invoice item or invoice line item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getInvoiceItem() {
return this.invoiceItem;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code discount}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* Date that the coupon was applied.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getStart() {
return this.start;
}
/**
* The subscription that this coupon is applied to, if it is applied to a particular subscription.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSubscription() {
return this.subscription;
}
/**
* The Checkout session that this coupon is applied to, if it is applied to a particular session
* in payment mode. Will not be present for subscription mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCheckoutSession(final String checkoutSession) {
this.checkoutSession = checkoutSession;
}
/**
* A coupon contains information about a percent-off or amount-off discount you might want to
* apply to a customer. Coupons may be applied to invoices or orders. Coupons do not work with
* conventional one-off charges.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCoupon(final Coupon coupon) {
this.coupon = coupon;
}
/**
* Always true for a deleted object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDeleted(final Boolean deleted) {
this.deleted = deleted;
}
/**
* If the coupon has a duration of {@code repeating}, the date that this discount will end. If the
* coupon has a duration of {@code once} or {@code forever}, this attribute will be null.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEnd(final Long end) {
this.end = end;
}
/**
* The ID of the discount object. Discounts cannot be fetched by ID. Use {@code
* expand[]=discounts} in API calls to expand discount IDs in an array.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* The invoice that the discount's coupon was applied to, if it was applied directly to a
* particular invoice.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setInvoice(final String invoice) {
this.invoice = invoice;
}
/**
* The invoice item {@code id} (or invoice line item {@code id} for invoice line items of
* type='subscription') that the discount's coupon was applied to, if it was applied directly to a
* particular invoice item or invoice line item.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setInvoiceItem(final String invoiceItem) {
this.invoiceItem = invoiceItem;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code discount}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* Date that the coupon was applied.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStart(final Long start) {
this.start = start;
}
/**
* The subscription that this coupon is applied to, if it is applied to a particular subscription.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSubscription(final String subscription) {
this.subscription = subscription;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Discount)) return false;
final Discount other = (Discount) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$deleted = this.getDeleted();
final java.lang.Object other$deleted = other.getDeleted();
if (this$deleted == null ? other$deleted != null : !this$deleted.equals(other$deleted)) return false;
final java.lang.Object this$end = this.getEnd();
final java.lang.Object other$end = other.getEnd();
if (this$end == null ? other$end != null : !this$end.equals(other$end)) return false;
final java.lang.Object this$start = this.getStart();
final java.lang.Object other$start = other.getStart();
if (this$start == null ? other$start != null : !this$start.equals(other$start)) return false;
final java.lang.Object this$checkoutSession = this.getCheckoutSession();
final java.lang.Object other$checkoutSession = other.getCheckoutSession();
if (this$checkoutSession == null ? other$checkoutSession != null : !this$checkoutSession.equals(other$checkoutSession)) return false;
final java.lang.Object this$coupon = this.getCoupon();
final java.lang.Object other$coupon = other.getCoupon();
if (this$coupon == null ? other$coupon != null : !this$coupon.equals(other$coupon)) return false;
final java.lang.Object this$customer = this.getCustomer();
final java.lang.Object other$customer = other.getCustomer();
if (this$customer == null ? other$customer != null : !this$customer.equals(other$customer)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$invoice = this.getInvoice();
final java.lang.Object other$invoice = other.getInvoice();
if (this$invoice == null ? other$invoice != null : !this$invoice.equals(other$invoice)) return false;
final java.lang.Object this$invoiceItem = this.getInvoiceItem();
final java.lang.Object other$invoiceItem = other.getInvoiceItem();
if (this$invoiceItem == null ? other$invoiceItem != null : !this$invoiceItem.equals(other$invoiceItem)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$promotionCode = this.getPromotionCode();
final java.lang.Object other$promotionCode = other.getPromotionCode();
if (this$promotionCode == null ? other$promotionCode != null : !this$promotionCode.equals(other$promotionCode)) return false;
final java.lang.Object this$subscription = this.getSubscription();
final java.lang.Object other$subscription = other.getSubscription();
if (this$subscription == null ? other$subscription != null : !this$subscription.equals(other$subscription)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Discount;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $deleted = this.getDeleted();
result = result * PRIME + ($deleted == null ? 43 : $deleted.hashCode());
final java.lang.Object $end = this.getEnd();
result = result * PRIME + ($end == null ? 43 : $end.hashCode());
final java.lang.Object $start = this.getStart();
result = result * PRIME + ($start == null ? 43 : $start.hashCode());
final java.lang.Object $checkoutSession = this.getCheckoutSession();
result = result * PRIME + ($checkoutSession == null ? 43 : $checkoutSession.hashCode());
final java.lang.Object $coupon = this.getCoupon();
result = result * PRIME + ($coupon == null ? 43 : $coupon.hashCode());
final java.lang.Object $customer = this.getCustomer();
result = result * PRIME + ($customer == null ? 43 : $customer.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $invoice = this.getInvoice();
result = result * PRIME + ($invoice == null ? 43 : $invoice.hashCode());
final java.lang.Object $invoiceItem = this.getInvoiceItem();
result = result * PRIME + ($invoiceItem == null ? 43 : $invoiceItem.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $promotionCode = this.getPromotionCode();
result = result * PRIME + ($promotionCode == null ? 43 : $promotionCode.hashCode());
final java.lang.Object $subscription = this.getSubscription();
result = result * PRIME + ($subscription == null ? 43 : $subscription.hashCode());
return result;
}
/**
* The ID of the discount object. Discounts cannot be fetched by ID. Use {@code
* expand[]=discounts} in API calls to expand discount IDs in an array.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
}